The `round` function in MATLAB rounds the elements of an array to the nearest integers, with halfway cases rounded away from zero.
Here's a code snippet demonstrating its usage:
% Example of rounding numbers in MATLAB
numbers = [1.5, 2.3, -3.7, 4.5];
roundedNumbers = round(numbers);
disp(roundedNumbers);
Understanding Rounding in MATLAB
What is Rounding?
Rounding is a fundamental concept in numerical analysis, where a number is approximated to a nearby value that has a shorter or simpler representation. In programming and data science, rounding is crucial as it affects data accuracy and representation. The impact of improper rounding can lead to significant discrepancies in computations, particularly in fields such as finance, engineering, or data analytics.
Types of Rounding Methods
MATLAB primarily utilizes different rounding techniques that adhere to specific principles. The most common methods include:
- Round half up: The default rounding method, which rounds to the nearest neighbor, and rounds up if the number is equidistant from both neighbors.
- Round half down: A less common method where numbers that land exactly halfway are rounded down.
- Stochastic rounding: While not directly implemented in MATLAB, this method randomizes rounding to reduce bias in statistical computations.
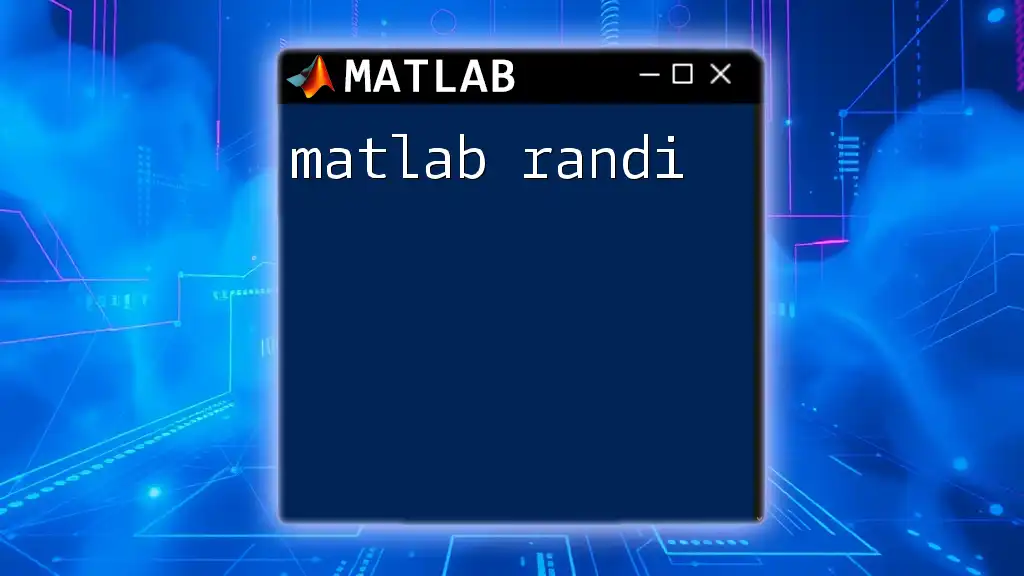
The `round` Function in MATLAB
Syntax of the `round` Function
The basic syntax of the `round` function in MATLAB is straightforward:
B = round(A)
Here, `A` represents the input array, number, or matrix that you wish to round, while `B` is the resulting output after applying rounding.
How to Use the `round` Function
Rounding in Different Dimensions
MATLAB's `round` function is versatile and can handle both scalars and arrays effectively.
Rounding Scalars:
For scalar inputs, the function works seamlessly. Consider the following example:
x = 3.6;
roundedValue = round(x); % returns 4
Here, `3.6` rounds to `4`, demonstrating how MATLAB handles a single floating-point number.
Rounding Arrays:
When dealing with arrays, `round` efficiently processes the entire matrix. For instance:
A = [1.5, 2.3, 3.7; -1.4, -2.8, -2.5];
roundedArray = round(A); % returns [2, 2, 4; -1, -3, -3]
This code illustrates how each element in `A` is rounded to the nearest integer.
Rounding to Specific Decimal Places
A significant feature of the `round` function is its ability to round to a predetermined number of decimal places using an optional second argument:
B = round(A, N)
Here, `N` specifies the number of decimal places desired. For example, rounding to two decimal places is achieved as follows:
A = [1.2565, 2.346, 3.789];
roundedTwoDecimals = round(A, 2); % returns [1.26, 2.35, 3.79]
This flexibility allows for more precise control over numerical data in applications requiring specific formats.
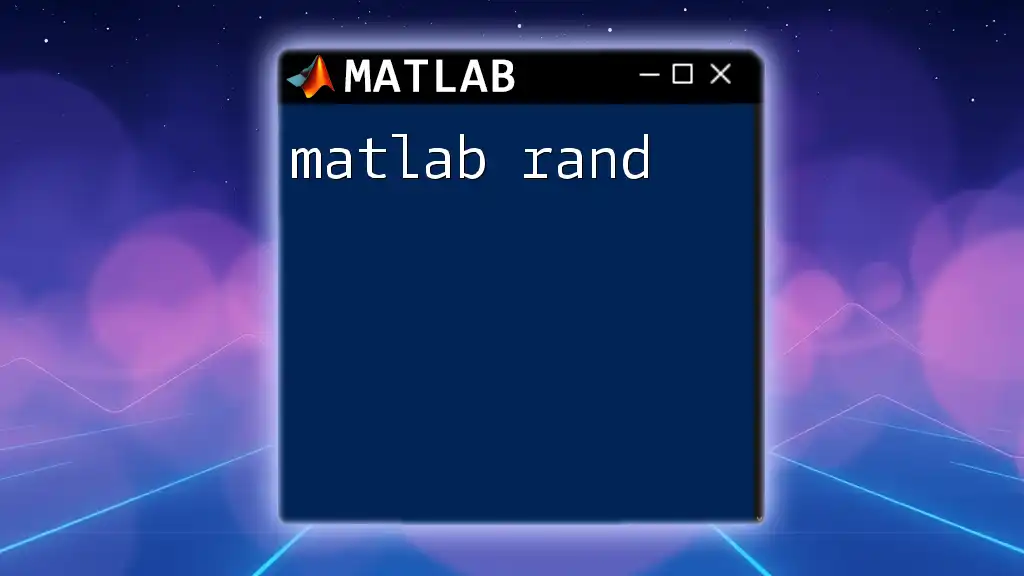
Detailed Example Use Cases
Use Case 1: Financial Calculations
In financial applications, precision is paramount. Rounding plays a pivotal role in determining the accuracy of monetary values. Consider the following code that rounds prices to two decimal places:
prices = [19.99, 2.49, 15.00];
roundedPrices = round(prices, 2); % returns [19.99, 2.49, 15.00]
This ensures that all prices are consistently represented, which is vital for correct financial reporting.
Use Case 2: Statistical Analysis
Rounding is equally essential in statistical analysis, where mean values or other computed statistics must be represented accurately. Here’s an example:
data = [5.1, 4.9, 5.5, 5.0];
meanValue = mean(data);
roundedMean = round(meanValue, 1); % returns 5.0
In this case, the mean is calculated and then rounded to one decimal place, ensuring clarity in reporting statistical results.
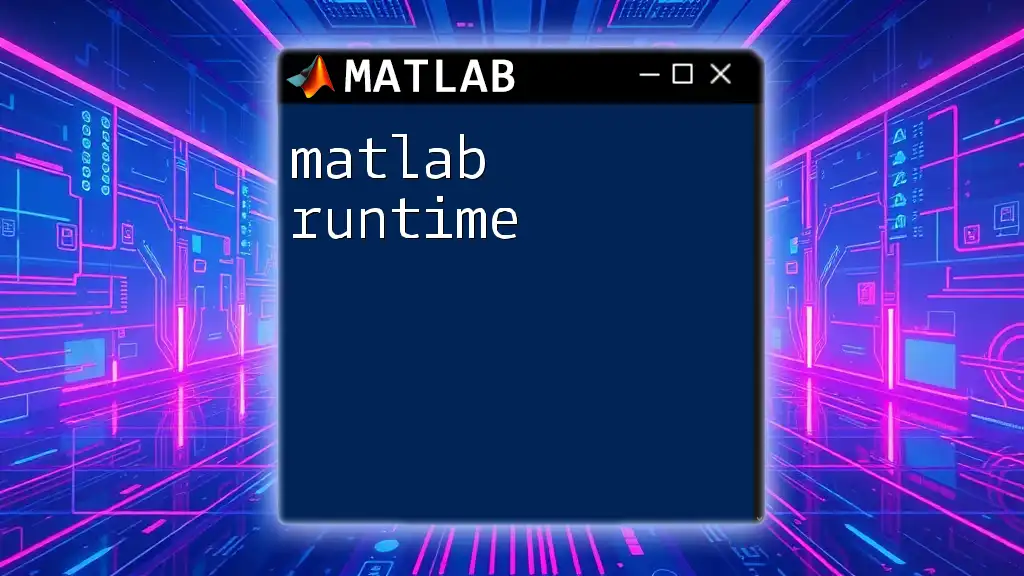
Additional Considerations
Comparing Different Rounding Methods
It's crucial to understand how the `round` function differs from other MATLAB rounding functions like `floor`, `ceil`, and `fix`. Each has its specific behavior, particularly with negative numbers, as illustrated below:
x = 5.7;
floorValue = floor(x); % returns 5
ceilValue = ceil(x); % returns 6
fixValue = fix(x); % returns 5
- `floor` returns the largest integer less than or equal to `x`.
- `ceil` returns the smallest integer greater than or equal to `x`.
- `fix` rounds towards zero, effectively truncating the fractional part.
These distinctions are crucial in applications that require specific rounding behavior.
Performance Tips
When working with large datasets in MATLAB, it’s essential to consider memory efficiency and performance when using the `round` function. To optimize performance:
- Use vectorized operations to process arrays rather than loops.
- Avoid rounding repeatedly; perform rounding only once when necessary.
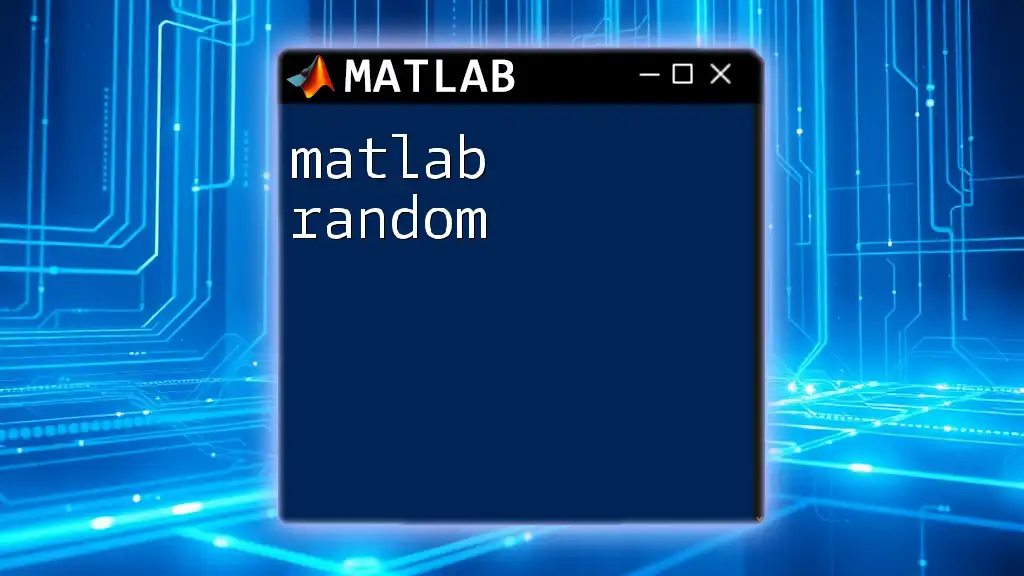
Common Errors and Troubleshooting
Common Issues with `round`
Users often misunderstand how `round` behaves with negative numbers. For instance, rounding `-2.5` results in `-2`, which might seem counterintuitive. Additionally, handling special values like `NaN` requires attention:
A = [NaN, 1.5, -1.5];
roundedValues = round(A); % returns [NaN, 2, -2]
As demonstrated, `NaN` remains unchanged during the rounding process, which is vital in mathematical computations to avoid erroneous results.
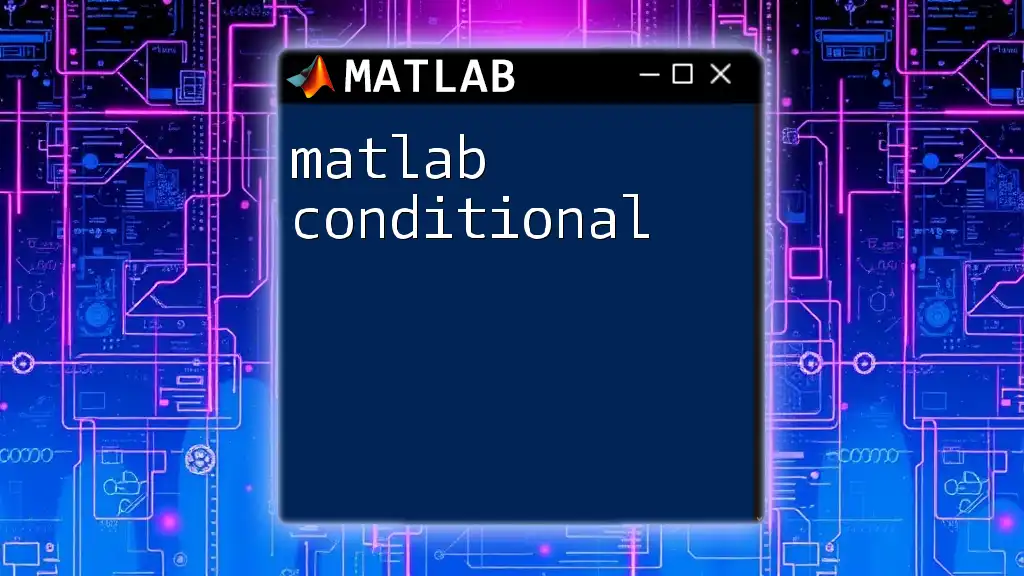
Conclusion
In summary, the `round` function in MATLAB is a critical tool for ensuring numerical precision and clarity across various applications. Understanding its functionalities, including rounding scalars, arrays, and to specific decimal places, plays an essential role in programming and data analysis. By mastering the `round` function, users can significantly improve their data handling and analysis capabilities.
Feel free to explore the `round` function and practice with the diverse examples provided to enhance your MATLAB skills further!