In MATLAB, you can save values from a for loop into an array by indexing the array within the loop, as shown in the following example:
% Initialize an empty array to store results
results = zeros(1, 5);
% Loop to calculate and store values
for i = 1:5
results(i) = i^2; % Save the square of i in the results array
end
% Display the results
disp(results);
Understanding For Loops in MATLAB
What is a For Loop?
A for loop in MATLAB is a powerful control structure that allows you to repeat a sequence of statements a specific number of times. You can think of it as a way to automate repetitive tasks, making your code more efficient and concise.
Structure of a For Loop
A typical for loop in MATLAB consists of:
- Initialization: This is where you define the variable that will manage the loop, often referred to as the loop index.
- Condition: The loop continues to run as long as this condition is satisfied; typically, this is defined by the range of the loop index.
- Increment: After each iteration, the loop index is updated (incremented or decremented) until the condition is no longer valid.
Here’s a simple example of a for loop:
for i = 1:5
disp(i)
end
In this example, MATLAB will display the numbers 1 through 5.
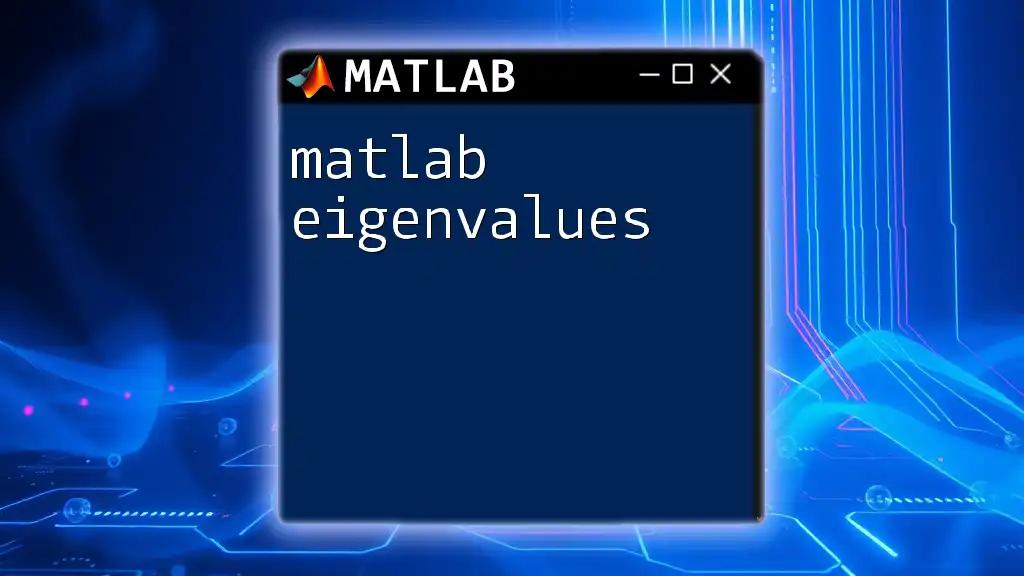
Saving Values from a For Loop
Importance of Saving Values
Saving values generated inside a for loop is crucial for post-loop analysis. Whether you're processing data, simulating experiments, or collecting outputs for further calculations, knowing how to matlab save values from for loop can significantly impact your workflow and the clarity of your code.
How To Save Values
Using Arrays
One of the most common ways to store values computed in a loop is by using arrays. Arrays allow for fast storage and retrieval of numerical data.
Example: Saving loop results in an array:
results = zeros(1, 10); % Preallocate array
for i = 1:10
results(i) = i^2; % Save square of i
end
disp(results); % Display saved values
In this example, we first preallocate the array `results` to improve performance. By using `zeros`, we allocate memory for 10 elements, which speeds up the execution time compared to dynamically resizing the array during each loop iteration.
Using Cell Arrays
For situations where you need to store mixed data types or strings alongside numbers, cell arrays are the way to go. They can handle varying data sizes and types seamlessly.
Example: Saving mixed data types:
results = cell(1, 10); % Preallocate cell array
for i = 1:10
results{i} = sprintf('Number: %d, Square: %d', i, i^2);
end
disp(results);
In this example, each cell in the `results` array can store a formatted string, allowing for greater flexibility in data representation.
Using Structures
When dealing with related data points, structures provide a highly organized way to keep track of multiple variables.
Example: Saving related data in a structure:
results(1:10) = struct('Number', {}, 'Square', {});
for i = 1:10
results(i).Number = i;
results(i).Square = i^2;
end
disp(results);
In this case, each element of the `results` array is a structure containing fields. This allows for a logical relation between the original number and its square, making the data easier to manage and analyze.
Advanced Techniques to Save Loop Values
Dynamic Data Storage
In certain scenarios, you may not know the size of your resulting dataset beforehand. In such cases, you might opt for dynamic concatenation. However, it's important to remember that this technique can lead to decreased performance due to memory allocation overhead.
Example: Storing results dynamically:
results = []; % Start with an empty array
for i = 1:10
results = [results i^2]; % Concatenate square of i
end
disp(results);
While this method works, it's usually more efficient to preallocate your array when possible.
Using Tables
Tables are a newer data type introduced in MATLAB that provide a robust way of organizing data with related variables. They are particularly useful when you want to store labeled data.
Example: Saving data in a table:
results = table(); % Initialize table
for i = 1:10
results(i, :) = table(i, i^2, 'VariableNames', {'Number', 'Square'});
end
disp(results);
Tables allow you to easily manipulate and analyze data while providing clear variable names for better readability.
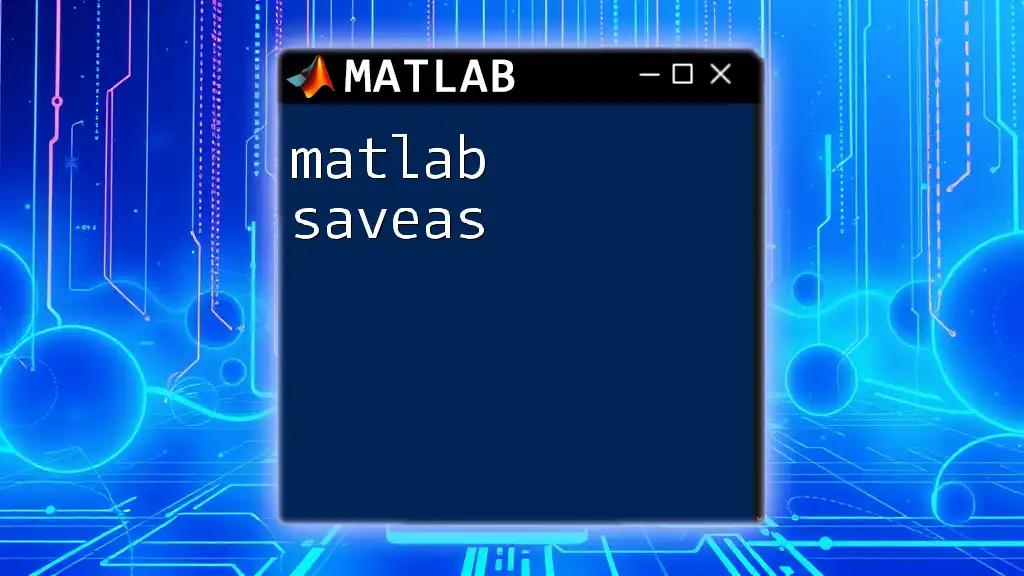
Best Practices for Saving Loop Values
Efficient Memory Management
Always preallocate your arrays whenever possible. Preallocation helps avoid the costly operation of resizing the array during each iteration of the loop, leading to faster execution. MATLAB performs significantly better with preallocated arrays, especially in larger loops.
Code Readability
Keep your code simple and easy to understand. By maintaining clear variable names and providing adequate comments, you not only help yourself in the future but also assist others who may read your code. Well-documented code is much more maintainable.
Error Handling
Be aware of common errors that could occur when saving values in for loops, such as indexing errors. Always ensure your indexes are within valid ranges to avoid runtime errors.

Conclusion
Mastering the technique of how to matlab save values from for loop is essential for efficient programming in MATLAB. Whether using arrays, cell arrays, structures, or tables, each method offers unique benefits that can be applied to various scenarios.
Experiment with different techniques to see which one fits your project best, and take advantage of MATLAB’s extensive documentation for deeper insights into each topic.

Additional Resources
To further your knowledge in MATLAB, explore the official MATLAB documentation and consider enrolling in MATLAB courses, available both online and through reputable institutions. Following the company blog for more tips and tricks can also keep your skills sharp and enhance your learning journey.