The `save` command in MATLAB allows users to save all variables from the workspace to a specified file, preserving their values for future use.
save('myWorkspace.mat')
Understanding MATLAB Workspaces
What is a Workspace?
A workspace in MATLAB is a repository where all the variables and data that you define during a session are stored. Think of it as a virtual table where you can keep all your noted ingredients (variables) while cooking (coding). Everything you declare and calculate exists in this space until you decide to clear it or save it for later use.
Why Save Your Workspace?
When working on a complex project, it’s essential to retain the values and configurations of your variables. Saving the workspace ensures that you don’t lose your progress when you close the MATLAB session. Common scenarios where saving becomes critical include:
- Unexpected Crashes: Computer or software crashes can happen without warnings, and unsaved work can be lost.
- Long-Running Calculations: If you’ve spent a considerable amount of time calculating or processing data, it’s important to preserve that work.
- Research and Documenting: Saving your workspace can help in maintaining continuity in long-term research projects or documentation.
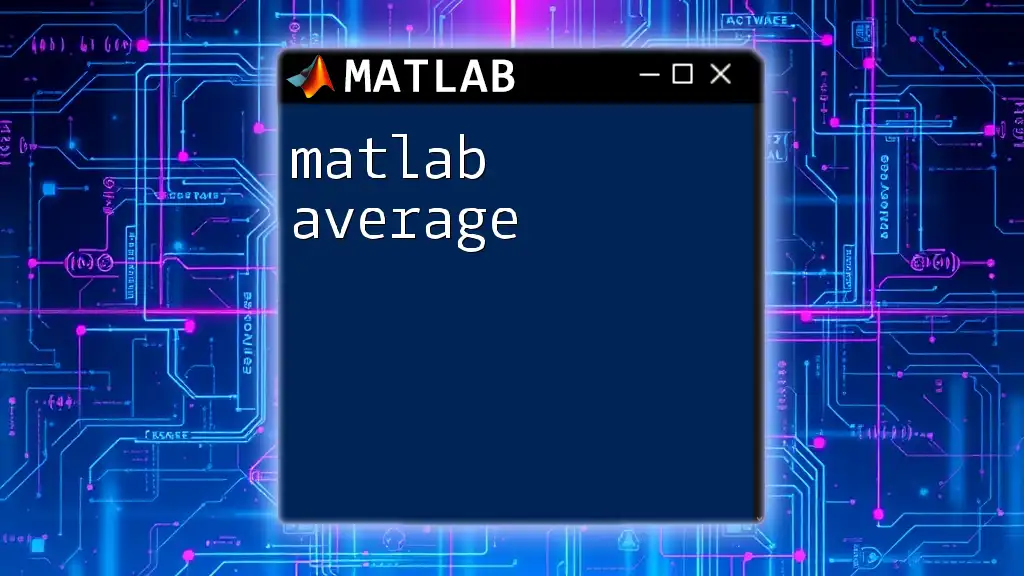
Saving the Workspace in MATLAB
Methods to Save Your Workspace
Using the save Command
The simplest method to save your workspace is by using the `save` command. The basic syntax is as follows:
save('filename.mat')
This command saves all the variables in the current workspace to a specified file (in this case, `filename.mat`). By using the `.mat` extension, you ensure that the file is saved in MATLAB’s standard format, which allows for easy retrieval of data later.
Example
Consider the following scenario where you declare some variables and save them:
x = 5;
y = rand(10);
save('myWorkspace.mat') % Saves x and y to myWorkspace.mat
In this example, the variables `x` and `y` are saved together in `myWorkspace.mat`.
Saving with a Specific Filename
Should you wish to specify a custom filename for better organization, you can easily do so, following the same syntax:
save('customFileName.mat')
This command will result in your variables being saved under the specified name.
Saving Specific Variables
Sometimes, you may want to save only certain variables instead of the entire workspace. This can be accomplished via the following syntax:
save('specificVars.mat', 'var1', 'var2')
This command saves only the specified variables `var1` and `var2` to the `specificVars.mat` file.
Example
For instance, if you wanted to preserve just two variables from a larger set, you could execute:
a = 10;
b = 20;
c = 30;
save('selectedVars.mat', 'a', 'b') % Only saves a and b
Saving to Different Formats
While .mat files are the default and most common format for saving workspaces, you may encounter situations where you'd prefer saving in other formats like CSV or text files. Each format has its specific use cases.
MAT-files vs. Other Formats
MAT-files are binary files that allow you to save workspace variables along with their metadata. However, if you want to export data for use in other applications, converting to formats like CSV can be advantageous.
Code Example
Here’s how you can save a table as a CSV file:
T = table(categorical({'M'; 'F'}), [25; 30], 'VariableNames', {'Gender', 'Age'});
writetable(T, 'data.csv'); % Saves table 'T' as a CSV file
This command converts the table `T` into a CSV file named `data.csv`, which can be opened in software like Excel.
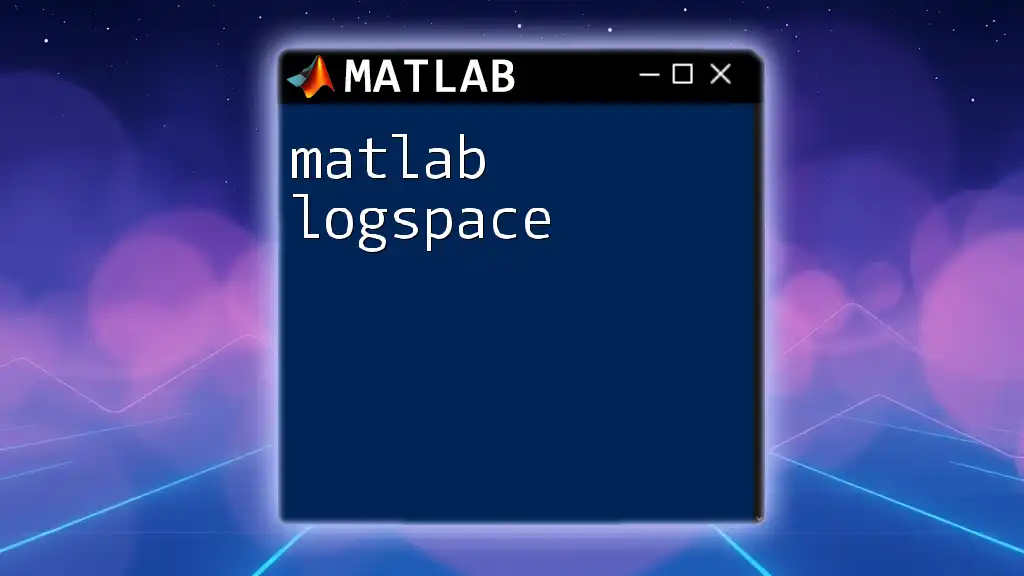
Loading a Saved Workspace
Using the Load Command
To retrieve your previously saved workspace, the `load` command is utilized. The basic syntax for this command is as follows:
load('filename.mat')
This command will restore all variables stored in the specified MAT-file back into your current workspace.
Example
If you saved your workspace as `myWorkspace.mat`, you can load it again with:
load('myWorkspace.mat')
Upon execution, all previously saved variables will be available for use in your current session.
Accessing Loaded Variables
After loading the workspace, accessing the variables is simple: they become available just like any other variable you’ve defined in your current session. You can manipulate or visualize them as needed without having to redefine them.
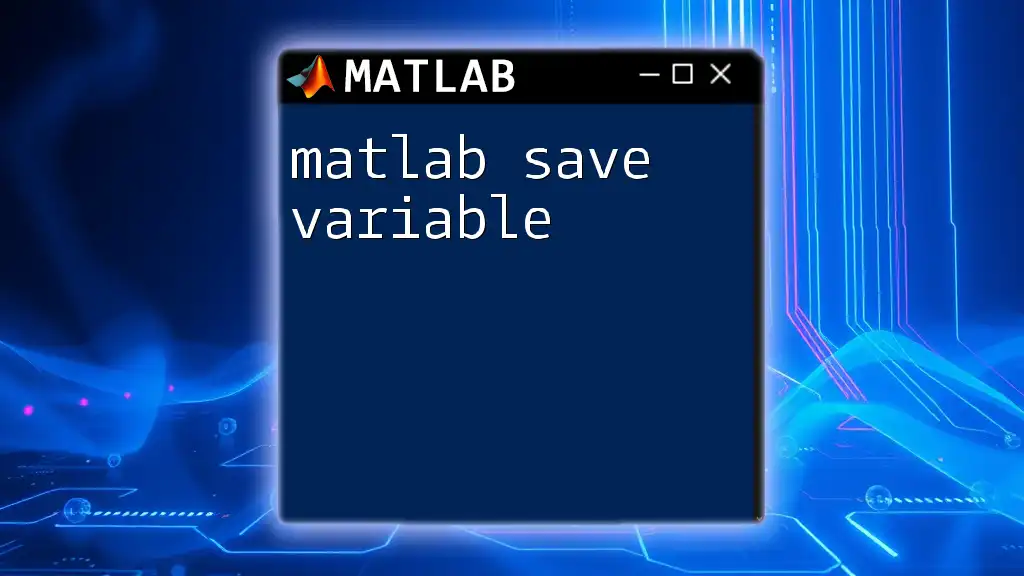
Managing Workspaces in MATLAB
Clearing the Workspace
At some point, you may want to clear your workspace to free up memory or start fresh. You can do this using the `clear` command:
clear
Executing this command will remove all existing variables from your workspace, which is useful for cleanup purposes. However, it’s crucial to remember that this command does not affect saved workspaces; you will lose unwritten changes.
Best Practices for Workspace Management
To maintain a healthy coding environment:
- Regular Saving: Make it a habit to save your workspace regularly, especially after significant changes or calculations.
- Organizing Data: Use meaningful file names to help you quickly identify saved workspaces in the future. This organization is particularly vital when managing multiple projects or research activities.
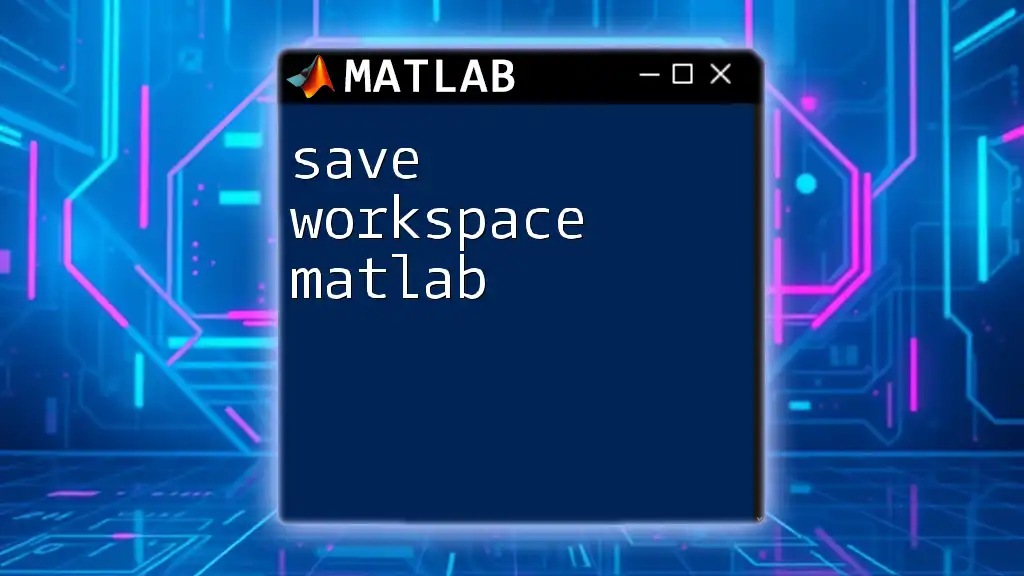
Conclusion
Understanding and utilizing the `matlab save workspace` command effectively allows you to work more efficiently and safeguard your data against unintentional loss. By saving regularly and organizing your workspaces, you can enhance your productivity and ensure smooth progress in your MATLAB projects.
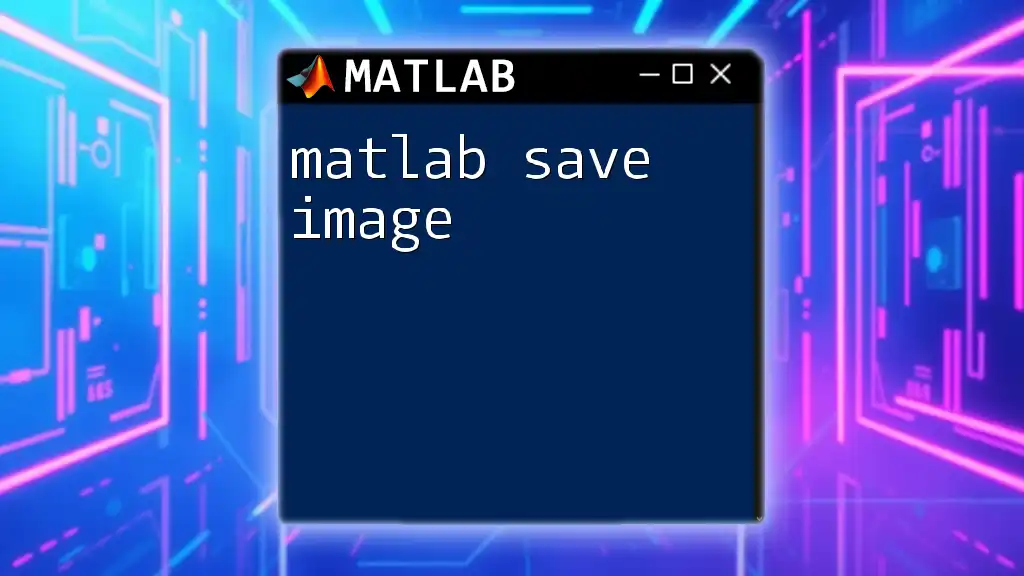
Call to Action
Feel free to share your experiences with saving workspaces in MATLAB, or ask any questions you may have about this essential feature. Engaging and learning from fellow MATLAB users can help you further improve your skills!