In MATLAB, "matlab or" refers to the logical OR operator used to combine two conditions, returning true if at least one of the conditions is true.
result = (a > 5) || (b < 10);
Understanding Logical Operations in MATLAB
What Are Logical Operations?
Logical operations are a fundamental concept in programming that allows the execution of code based on true or false conditions. In MATLAB, logical operations enable users to create conditional statements, filter data, and make decisions based on specific criteria.
The Role of the `or` Operator
The `or` operator in MATLAB, represented as `||` for short-circuit evaluation or `|` for element-wise evaluation, is used to combine multiple conditions. Using the `or` operator allows for greater flexibility when deciding whether certain conditions are met. It evaluates to true if at least one of the operands is true.
This operator is crucial for implementing decision-making processes in code; it provides an efficient way to check multiple conditions without needing nested if-statements, simplifying code readability and reduce complexity.
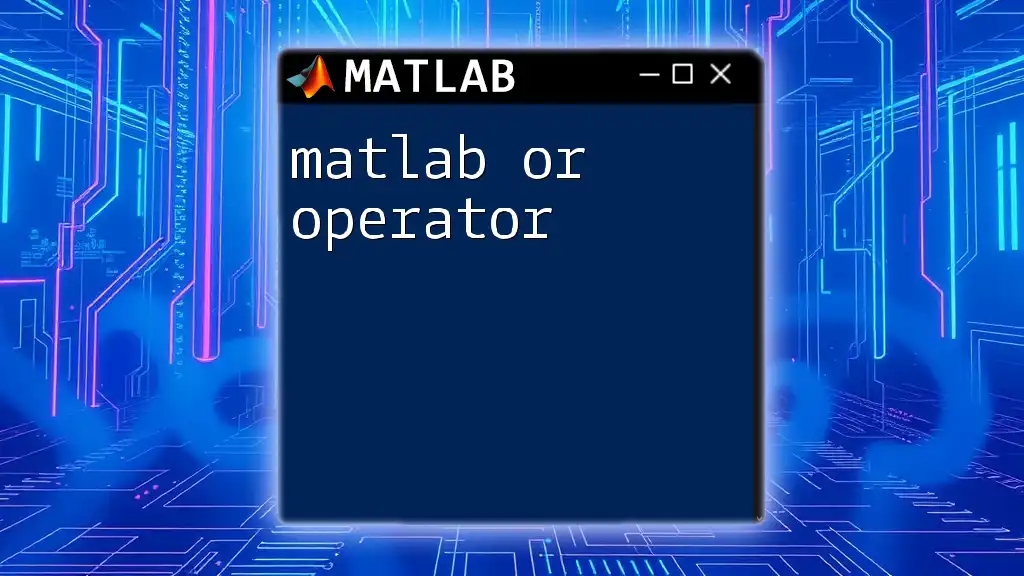
How to Use the `or` Operator
Basic Syntax
To utilize the `or` operator in MATLAB, the general syntax is:
result = A | B;
Here, `A` and `B` can be logical expressions or arrays of logical values. The result will be true wherever at least one of the corresponding elements is true.
Examples of `or` in Action
Simple Boolean Examples
A straightforward way to illustrate the function of the `or` operator is through logical vectors. Consider the following example:
A = [true, false, true];
B = [false, false, true];
C = A | B; % C becomes [true, false, true]
In this example, the resulting vector `C` contains `true` at indices where either `A` or `B` is `true`. This allows for quick logical assessments across multiple conditions.
Conditional Statements
The `or` operator becomes particularly useful in conditional statements, where decisions must be made based on variable assessments. Here's a sample code snippet:
x = 5;
if x < 0 || x > 10
disp('x is out of range.');
else
disp('x is within range.');
end
In this code, the `if` condition checks if `x` is either less than zero or greater than ten. The message displayed will depend on whether at least one of these conditions is true, showcasing the effectiveness of the `or` operator in controlling the flow of logic.
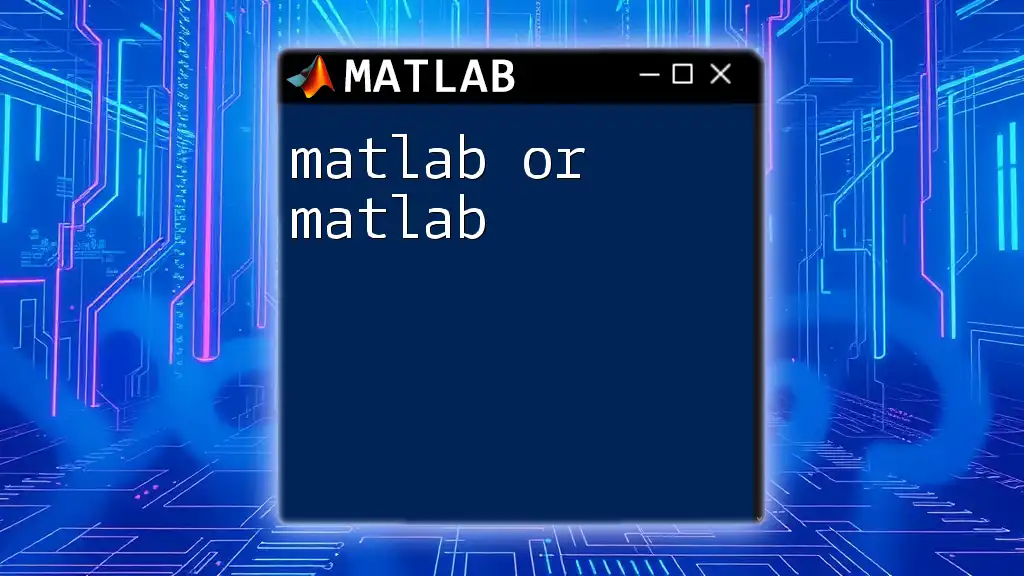
Advanced Uses of the `or` Operator
Combining Conditions
The `or` operator can also be used to combine multiple conditions in a single statement. This is particularly beneficial when you need to check several criteria simultaneously:
a = 10;
b = 20;
c = 30;
if a < 5 || b > 15 || c == 30
disp('At least one condition is true.');
end
In this example, the code checks if any of the conditions involving variables `a`, `b`, or `c` are true. The simplicity of this approach helps maintain clean and efficient code.
Vectorized Operations
Applying `or` Over Arrays
MATLAB's powerful array handling capabilities allow you to apply the `or` operator to entire arrays. Here’s an example:
A = [1, 2, 0, -1];
B = [0, 2, 3, 4];
C = A > 0 | B > 0;
In this case, `C` will evaluate to `[true, true, false, true]` because the `or` operator quickly processes multiple elements in arrays. This vectorization enhances performance by minimizing the need for loops.
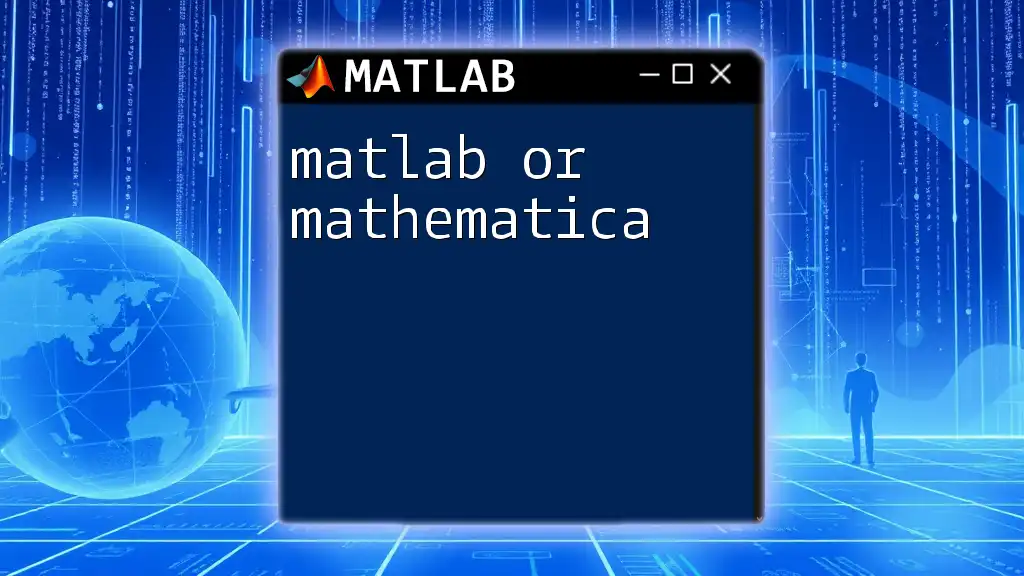
Performance Considerations
Matrix vs. Element-wise Operations
When using logical operators like `or`, it is essential to understand the difference between matrix and element-wise operations. The former is used for complete matrices, while the latter is for corresponding elements within arrays. Misusing these can lead to unexpected results. When using `or` in matrix operations, ensure that the dimensionality of the matrices aligns accordingly to avoid dimension mismatch errors.
Efficiency Tips
To write efficient MATLAB programs that leverage the `or` operator:
- Avoid unnecessary recalculations by storing repetitive expressions in variables.
- Use element-wise logical operations judiciously to enhance performance when processing large datasets.
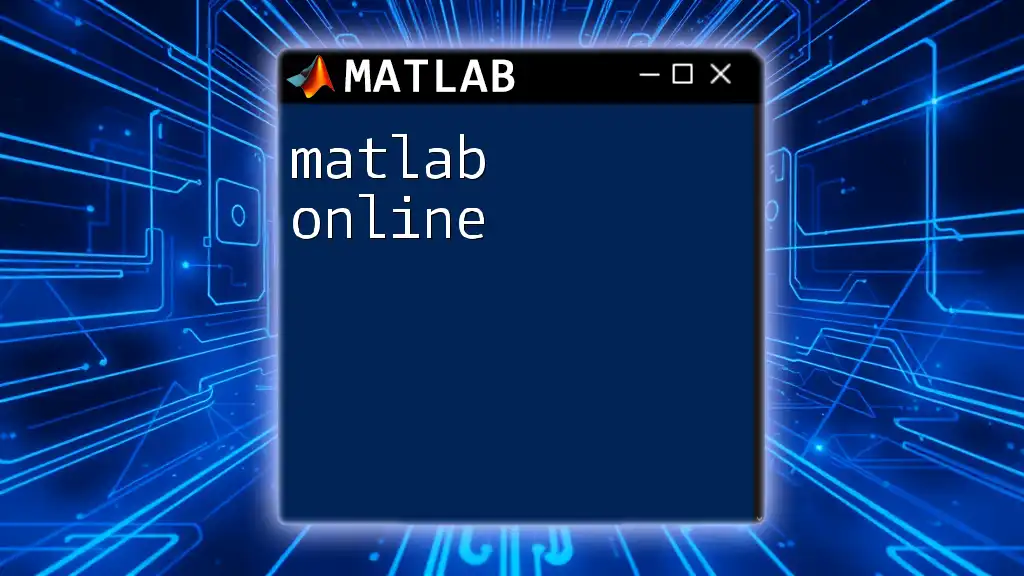
Common Mistakes and Troubleshooting
Common Pitfalls
One common mistake when using the `or` operator arises from misunderstanding operator precedence. For example, if you have mixed logical operations, parentheses may be required to ensure the correct evaluation order. Consider this incorrect approach:
if x < 5 || y > 10 && z == 1
Without parentheses, the `&&` operator takes precedence over `||`. To correct this:
if x < 5 || (y > 10 && z == 1)
Debugging Logical Expressions
When troubleshooting logical expressions that utilize the `or` operator, use MATLAB's debugging features such as breakpoints to inspect variable states and confirm the expected logical values. Understanding what each condition returns is critical in verifying your logical flow.
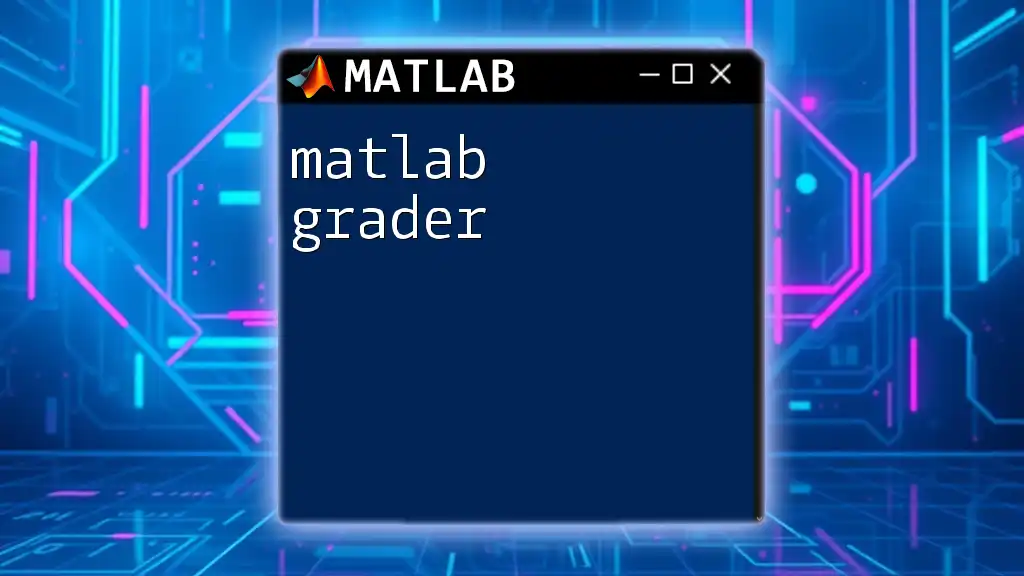
Conclusion
In this guide, we explored the `or` operator in MATLAB, emphasizing its importance in logical operations and conditional statements. Remember that the `or` operator not only simplifies coding but also enhances decision-making efficiency in your MATLAB programs. Whether you are working with simple conditions or complex nested logic, mastering the use of `or` will significantly improve your programming skills.
As you become more familiar with this operator, practice will enhance your confidence in applying it effectively in various scenarios. Be sure to explore additional resources and documentation to deepen your understanding of MATLAB's capabilities.
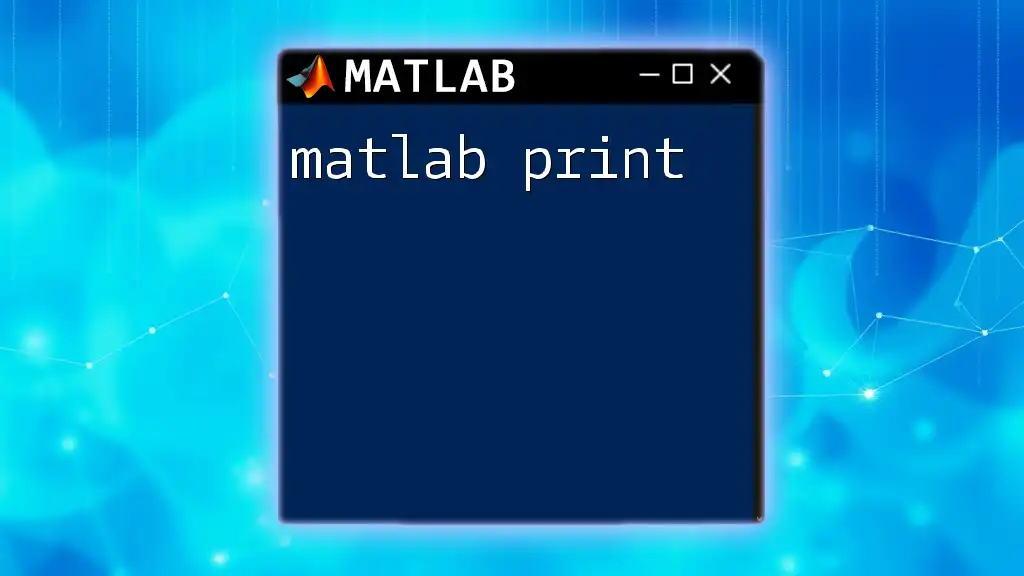
Additional Resources
For further reading, consider visiting the [MATLAB documentation](https://www.mathworks.com/help/matlab/ref/logical.html) for a comprehensive overview of logical operations and practical examples tailored to fit various programming contexts.
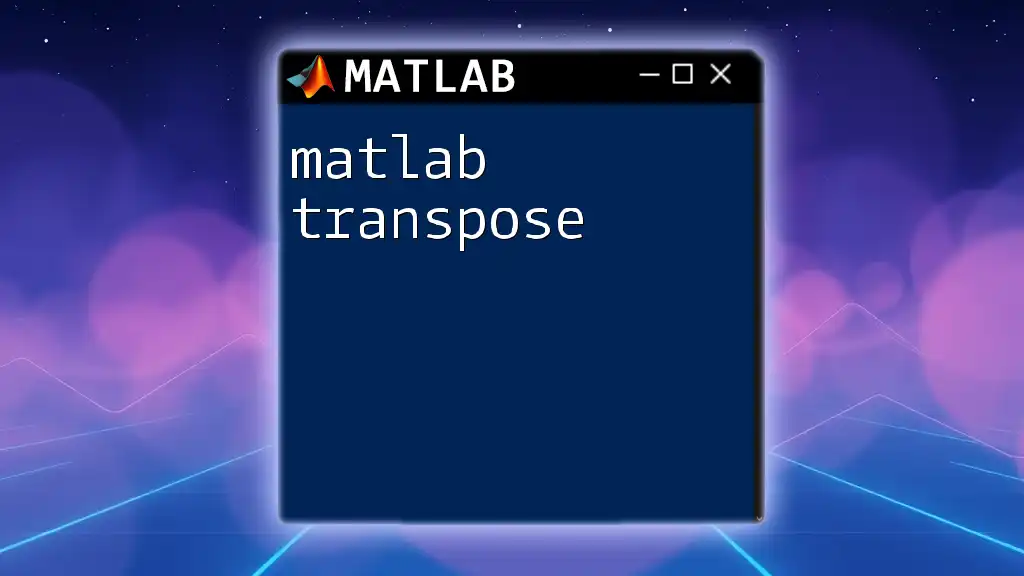
About Us
At [Your Company Name], we are dedicated to helping individuals master MATLAB commands efficiently. Our mission is to provide concise, practical training so you can confidently navigate this powerful tool for your engineering and scientific needs.