In MATLAB, you can split a string by a specific character using the `split` function, which takes the string and the delimiter as inputs. Here's an example:
str = 'Hello,world,how,are,you';
splitStr = split(str, ',');
Understanding Strings in MATLAB
What is a String?
In programming, a string is a sequence of characters used to represent text. In MATLAB, strings can be represented as character arrays or string arrays, allowing for flexible manipulation of textual data. Understanding how to work with strings is crucial for effective data processing in various applications.
Common Use Cases for String Splitting
Splitting strings is an essential operation in many scenarios. Here are some common use cases where splitting strings is beneficial:
- Data cleaning and preparation: When dealing with raw data, strings often need to be parsed to make them useful for analysis.
- Text analysis: Analyzing large bodies of text often requires breaking down sentences into words or phrases to quantify text features.
- Tokenization in Natural Language Processing (NLP): Tokenization involves splitting text into meaningful units (tokens), which is foundational in processing natural language for various machine learning models.
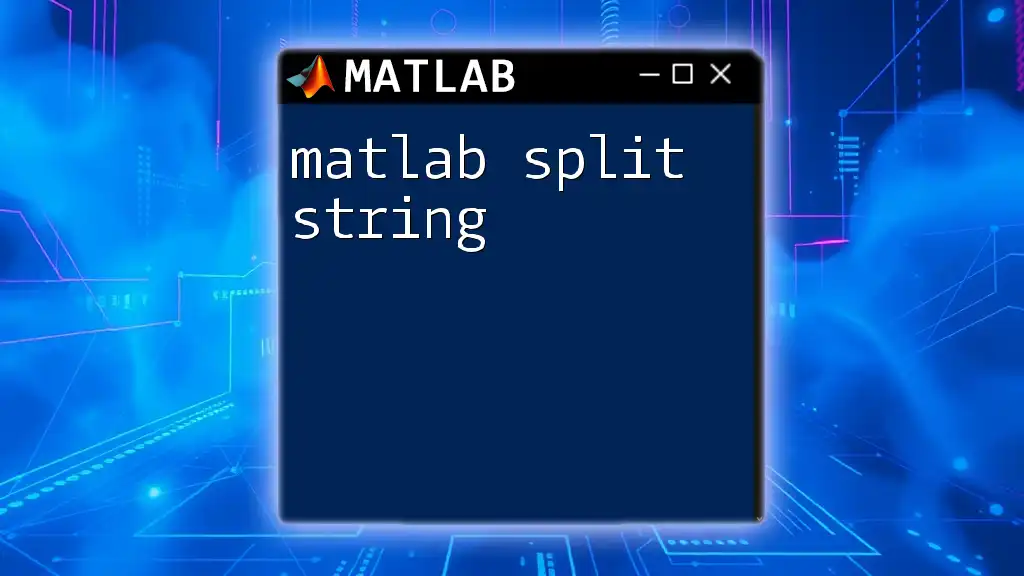
Methods to Split Strings in MATLAB
Using the `strsplit` Function
One of the simplest ways to split strings in MATLAB is by using the `strsplit` function. This function is straightforward and efficient for most cases.
Syntax:
C = strsplit(str, delim)
Example with code snippet
For instance, if you want to split a sentence into individual words, you can use:
sentence = 'MATLAB is great for string Manipulation';
words = strsplit(sentence, ' ');
% Result: {'MATLAB', 'is', 'great', 'for', 'string', 'Manipulation'}
In this example, the string is split at each space, resulting in a cell array containing each word.
Custom Delimiters
MATLAB allows you to use multiple characters as delimiters, enhancing versatility in string manipulation.
Example using commas and spaces
Suppose you have a CSV string from fruit data that you want to split:
csvData = 'apple,banana,cherry, durian, pear';
fruits = strsplit(csvData, {',', ' '});
% Result: {'apple', 'banana', 'cherry', 'durian', 'pear'}
In this case, the string is effectively split using both commas and spaces as delimiters.
Using Regular Expressions with `regexp`
For more complex string splitting tasks, the `regexp` function provides robust capabilities, utilizing regular expressions to find patterns in strings.
Syntax:
C = regexp(str, expr, 'split')
Example with code snippet
Suppose you want to split a string containing sentences separated by punctuation:
text = 'Hello! Are you ready? Yes; I am.';
sentences = regexp(text, '[.!?;]', 'split');
% Result: {'Hello', ' Are you ready', ' Yes', ' I am', ''}
Here, the string is split at each instance of a period, exclamation mark, question mark, or semicolon.
Using the `split` Function (R2016b and later)
In later versions of MATLAB (R2016b onward), you can use the `split` function, which is even simpler for basic splits.
Syntax:
C = split(str, delim)
Example with code snippet
For instance, if you want to split a sentence based on whitespace:
sentence = 'Learn MATLAB easily and effectively';
words = split(sentence);
% Result: "Learn" "MATLAB" "easily" "and" "effectively"
Comparing Methods: When to Use Which
Performance Considerations
When choosing between methods, consider performance. The `strsplit` function is fast for simple splits, while `regexp` can handle more complex patterns but may be slower, especially with large datasets.
Ease of Use
For beginners, `strsplit` and `split` offer straightforward syntax, while `regexp` may have a steeper learning curve due to its reliance on regular expressions.
Flexibility and Customization
Choose `strsplit` for straightforward delimiters and `regexp` for sophisticated pattern matching, depending on your requirements.
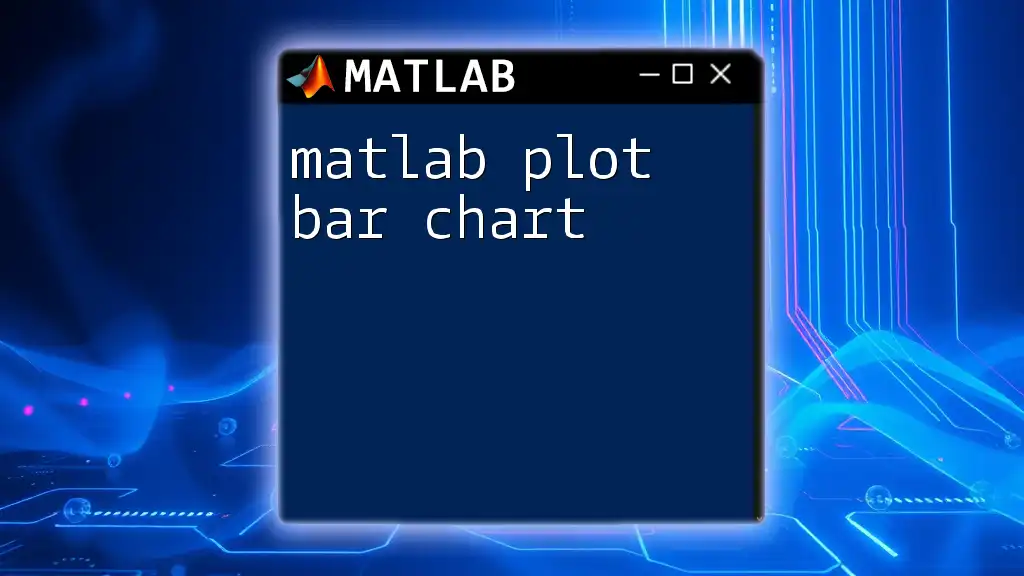
Practical Applications
Data Preparation for Machine Learning
Splitting strings is crucial in preparing textual data for machine learning models. For example, if you have a dataset containing product descriptions, you may need to tokenize these strings into individual words for feature extraction.
Text Analysis in Linguistics
Text analysis often revolves around evaluating sentence structures and frequencies of words. By splitting strings, linguists can better understand language patterns. For example, after splitting a text into words, you can count occurrences to analyze word frequency:
text = 'Data analysis is essential in machine learning. Data helps inform decisions.';
words = strsplit(text);
wordCounts = countcats(categorical(words));
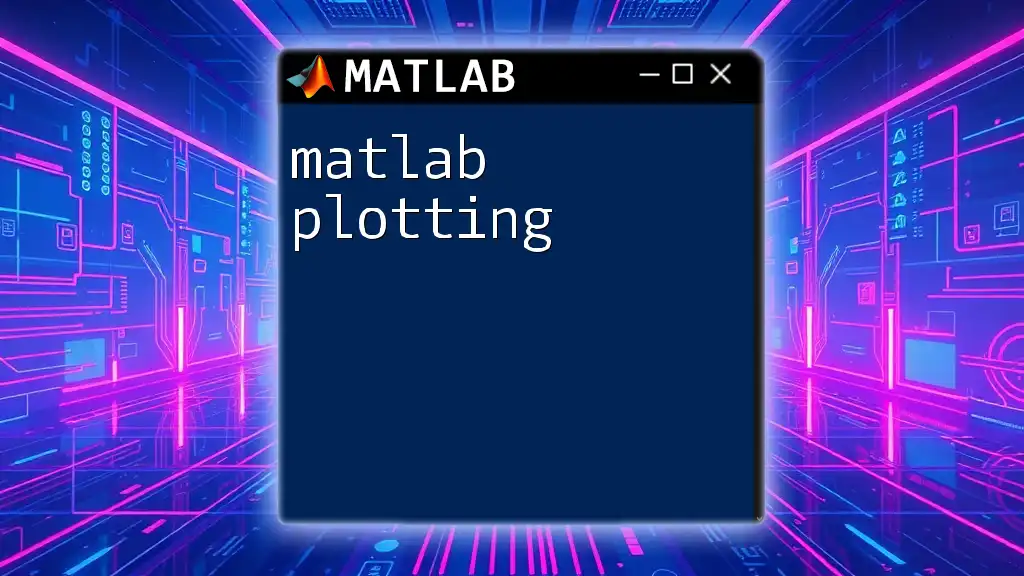
Common Issues and Troubleshooting
Handling Empty Strings
During string splitting, you may encounter empty strings. It's essential to handle these appropriately. You can trim whitespace and remove any empty cells:
trimmedWords = strtrim(words);
Special Characters and Accents
When dealing with strings that contain special characters or accents, you might face issues where the delimiters do not behave as expected. To avoid complications, consider normalizing your strings by removing or replacing special characters before performing splits.
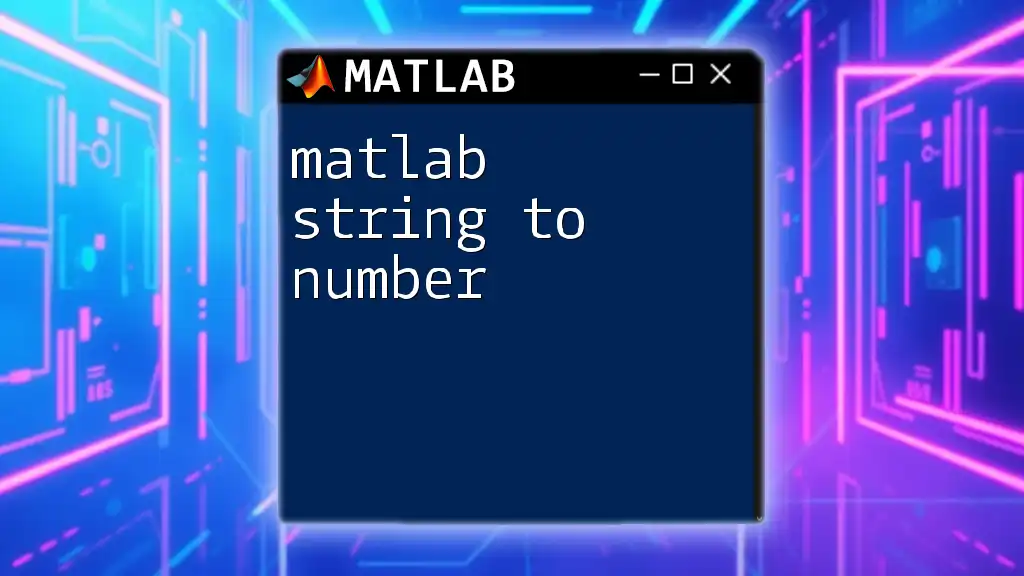
Conclusion
In conclusion, mastering how to matlab split string by character opens the door for effective text manipulation in various tasks. Each method discussed offers unique advantages suited to different needs, from basic splits to complex pattern matching. Experimenting with these string functions will enhance your data processing and analysis skills significantly. Take the next step and dive deeper into MATLAB commands to unlock even more powerful capabilities in your programming journey!
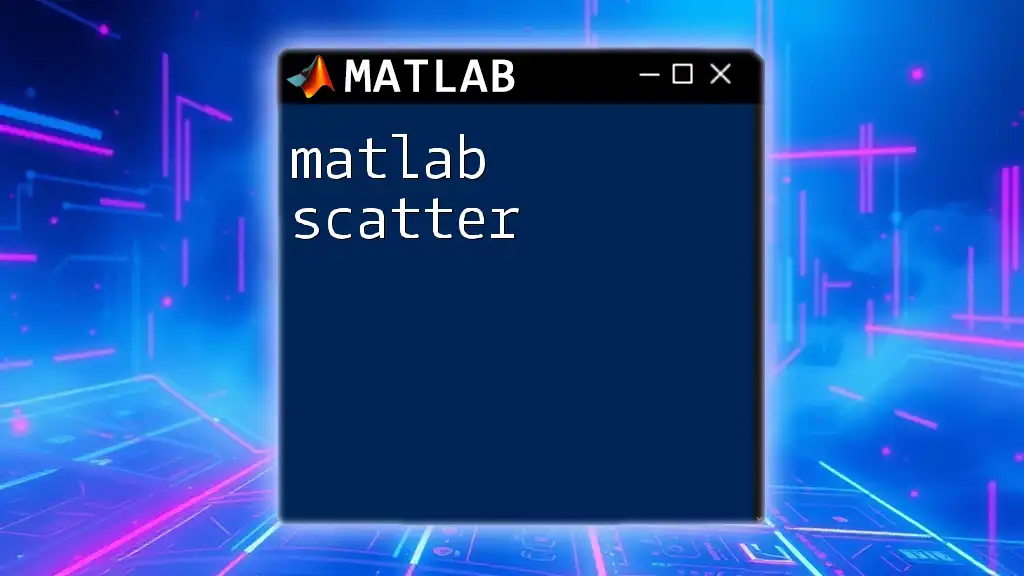
Additional Resources
For further reading, refer to MATLAB's official documentation, online tutorials, and consider interactive courses to enhance your skills in string manipulation and beyond.