The MATLAB `slice` function is used to visualize 3D volumetric data by displaying specific planes through the data cube based on given coordinate values.
% Example of using the slice function in MATLAB
[X,Y,Z] = meshgrid(-3:0.5:3, -3:0.5:3, -3:0.5:3);
V = X.^2 + Y.^2 + Z.^2; % Sample volumetric data
slice(X, Y, Z, V, [0], [0], [0]); % Slicing through the origin
colorbar; % Display colorbar for reference
Understanding the Concept of Slicing in Matlab
What is Slicing?
Slicing in Matlab refers to the technique of retrieving specific portions of arrays or matrices using a defined range or specific criteria. This is particularly beneficial when working with large datasets, where you might only need a subset of the data for analysis.
The importance of slicing cannot be overstated. It allows for efficient data manipulation, crucial for tasks ranging from simple data retrieval to complex data analysis. In a world driven by data, mastering the slicing command can enhance productivity and streamline processes.
The Benefits of Using Slicing
Using slicing provides several advantages:
- Enhanced Data Analysis Efficiency: You can quickly access and operate on subsets of your data, making analysis faster and more focused.
- Simplified Data Retrieval: Accessing specific rows, columns, or elements becomes straightforward, reducing the chances of errors in manual data handling.
- Improved Code Readability and Maintainability: Slicing condenses code, enhancing clarity and making it easier for others (or yourself in the future) to understand what the code is doing.
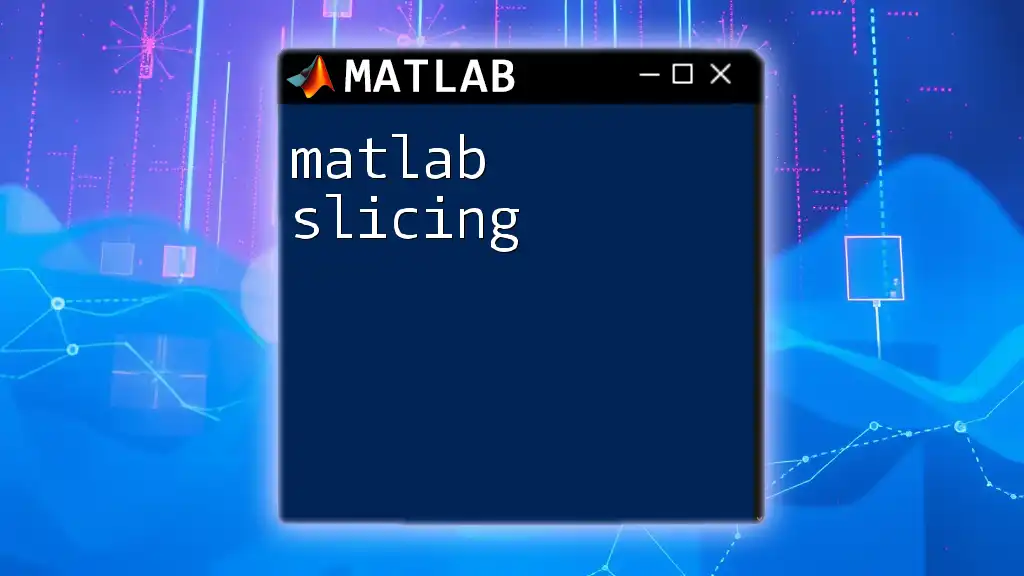
Basic Syntax of Slicing in Matlab
The Slice Operator
The colon operator (`:`) is the core of slicing in Matlab. It allows you to define a range of values to include in your sliced data. The basic syntax is as follows:
A(start:end)
This command retrieves elements from index `start` to index `end`, inclusive.
Index-based Slicing
To slice arrays using specific indices, simply specify the range. For example, consider the code snippet below:
A = [1, 2, 3, 4, 5];
B = A(2:4); % Results in B = [2, 3, 4]
Here, we have extracted the elements from indices 2 through 4.
Multidimensional Array Slicing
Slicing extends to multidimensional arrays, making it possible to extract specific submatrices. For instance:
A = [1 2 3; 4 5 6; 7 8 9];
B = A(1:2, 2:3); % Results in B = [2 3; 5 6]
In this scenario, we sliced two rows and two columns from the matrix `A`. This versatility is crucial when dealing with datasets that contain multiple dimensions.
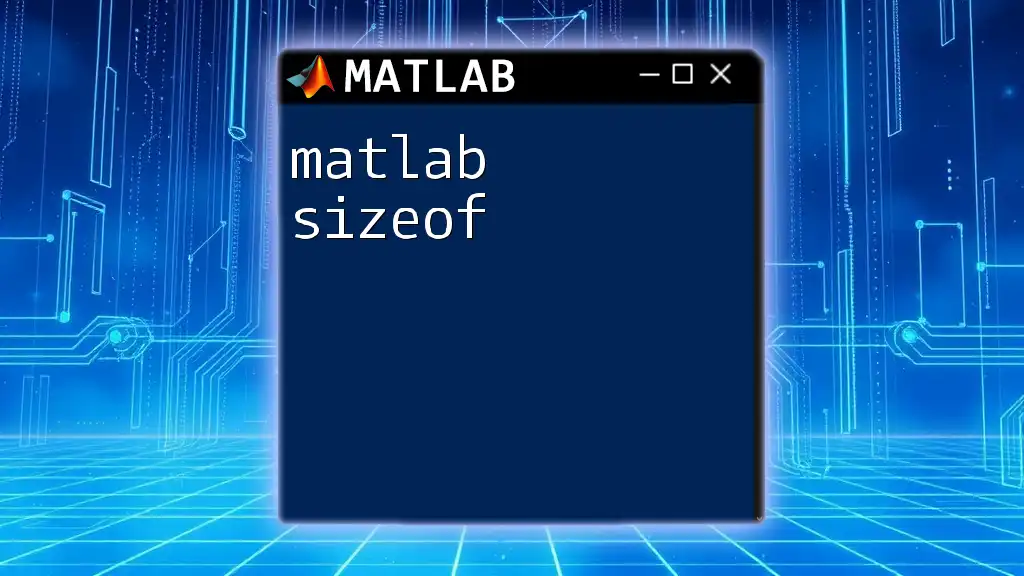
Advanced Slicing Techniques
Logical Indexing
Logical indexing allows you to slice data based on specific conditions. This is particularly powerful for filtering data. For instance:
A = [1, 7, 3, 4, 5];
B = A(A > 4); % Results in B = [7, 5]
In this example, only elements greater than 4 are included in `B`, showcasing how logical conditions can refine your data quickly.
Slicing with Functions
You can enhance slicing capabilities even further by using functions like `find` and `max`. Here's an example:
A = [1, 7, 3, 4, 5];
index = find(A > 4);
B = A(index); % Results in B = [7, 5]
By utilizing the `find` function, you retrieve indices that meet the condition, enabling dynamic and adaptable slicing.
Slicing for Data Visualization
Slicing isn’t limited to data manipulation; it can also be applied for effective data visualization purposes. For example:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
hold on;
% Highlight a specific slice of the data
plot(x(1:50), y(1:50), 'r', 'LineWidth', 2);
hold off;
Here, we plot the sine function and highlight a specific slice of the data, enhancing clarity in visual presentations.
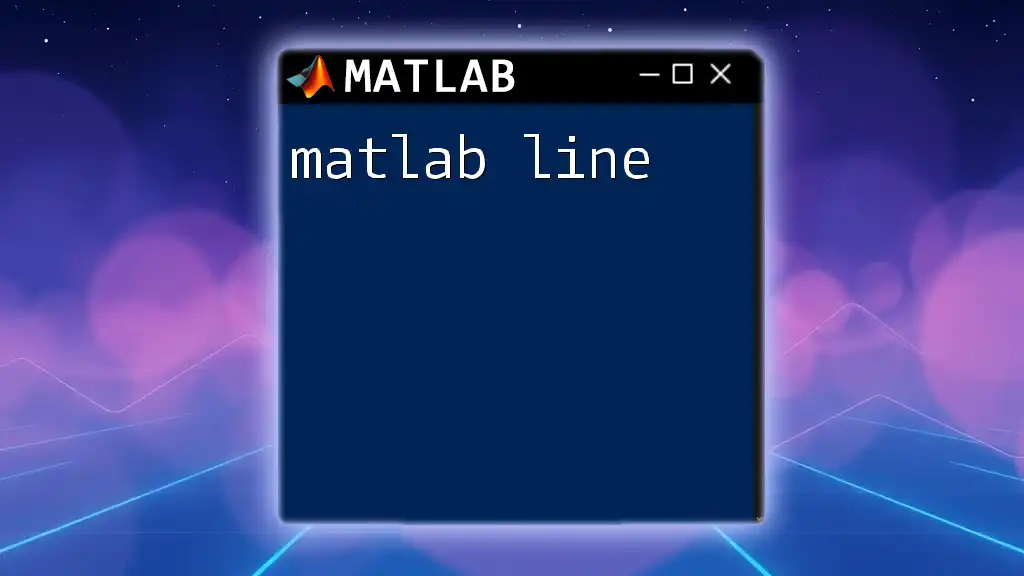
Common Mistakes and How to Avoid Them
Out of Bounds Errors
One of the most common mistakes when slicing is going out of bounds, which occurs when you try to access elements outside the defined limits of the array. Always ensure your start and end indices are within the array’s dimensions.
Misunderstanding Array Dimensions
When working with multidimensional arrays, it’s easy to misunderstand dimensions, leading to incorrect results. Utilize the `size` function to check the dimensions of your data before slicing. This practice reduces the likelihood of errors and improves your code's reliability.
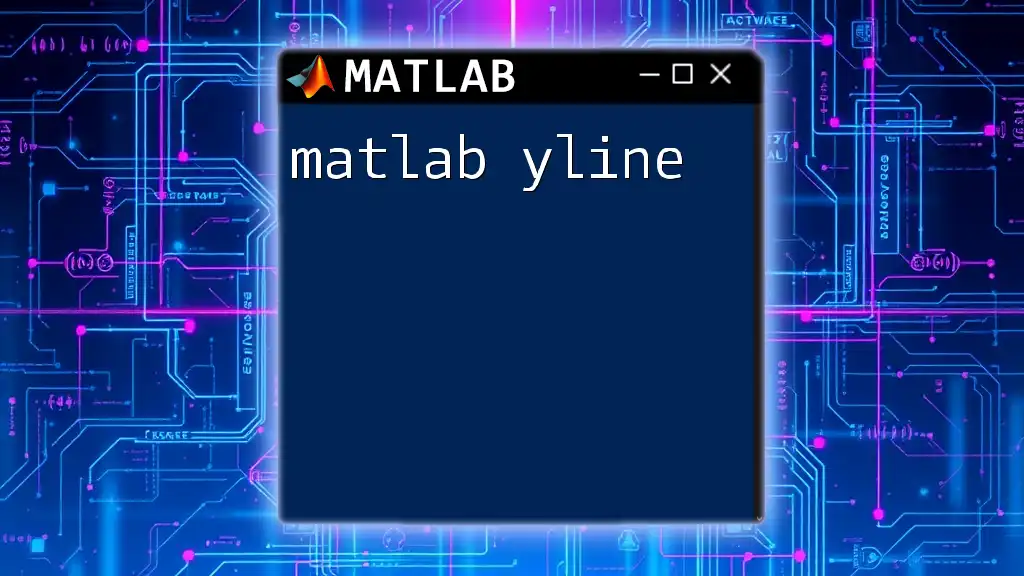
Real-World Applications of Slicing in Matlab
Data Analysis
Slicing is vital in data analysis, particularly in fields such as finance, engineering, and research. For example, to extract certain ranges of entries from a financial dataset for analysis, slicing can streamline the workflow significantly. Consider an example of extracting a specific period from daily stock prices:
dates = datetime(2020, 1, 1):datetime(2020, 12, 31);
prices = rand(1, length(dates)) * 100; % Simulating stock prices
monthly_prices = prices(1:30); % Slicing first 30 days of data
This concise extraction allows for focused analysis on the early month of trading.
Image Processing
In image processing, slicing plays a critical role as well. Consider you want to extract a region of interest from an image:
img = imread('image.jpg');
img_slice = img(100:200, 150:250, :); % Extracting a region of interest
imshow(img_slice);
Here, `img_slice` now holds a specific section of the original image, allowing for localized edits or analysis.
Machine Learning
In machine learning, preparing datasets often involves slicing to separate training and test data. For example, when working with a dataset to feed into a model:
data = rand(100, 5); % Generating a random dataset with 100 samples and 5 features
train_data = data(1:70, :); % 70% for training
test_data = data(71:end, :); % 30% for testing
This efficient slicing ensures that your models are trained and tested appropriately.
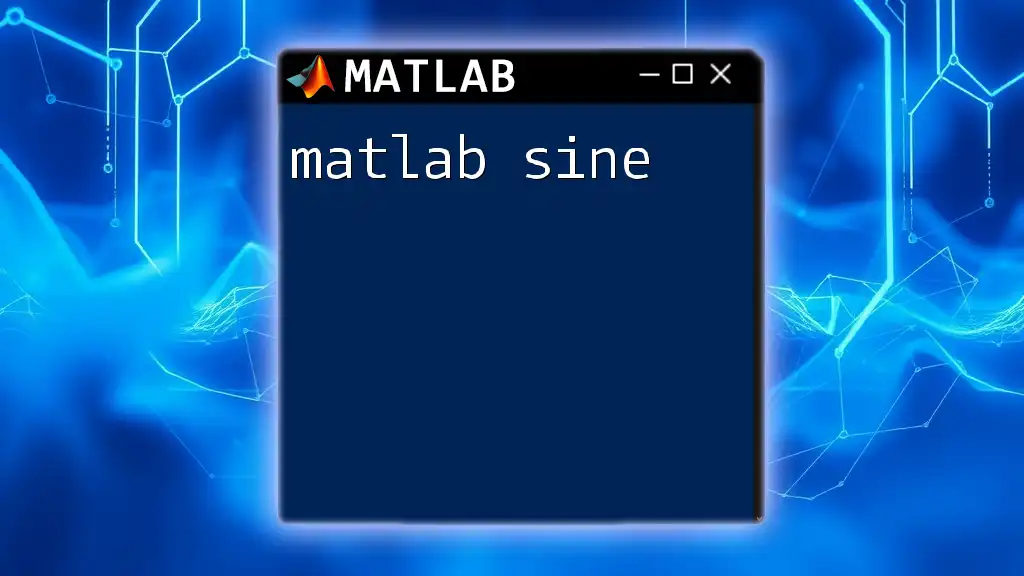
Conclusion
Recap of Key Points
In this comprehensive guide, we explored the concept of `matlab slice`, including its syntax and advanced techniques. The importance of slicing spans efficient data handling across numerous applications, illustrating the versatility and power of this command.
Call to Action
Now that you understand the essential aspects of slicing in Matlab, we encourage you to practice with your datasets. Don’t hesitate to experiment with the slicing techniques discussed here to gain a deeper understanding of data manipulation in your projects.
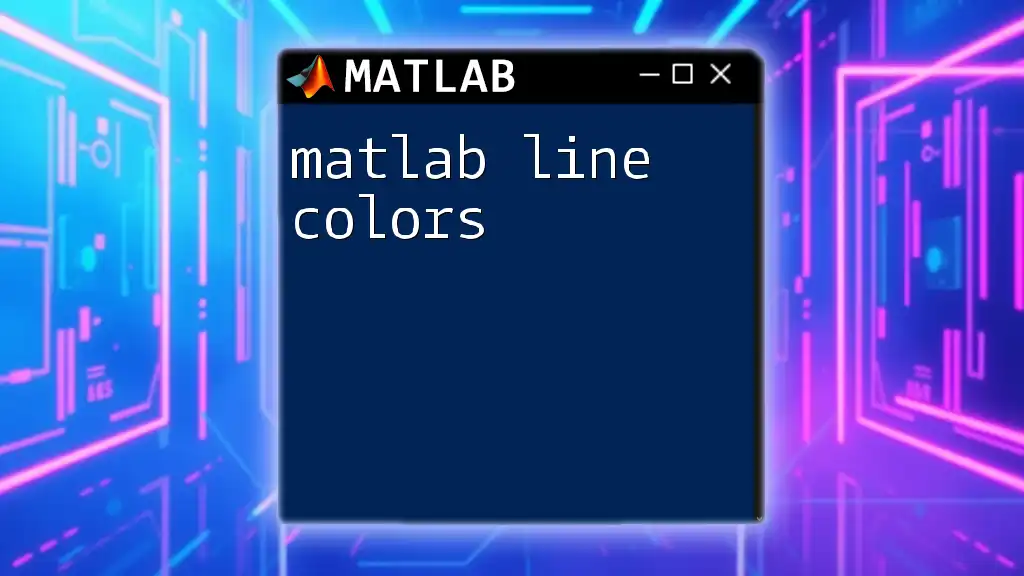
Additional Resources
Suggested Reading
For those wishing to learn more, consider exploring books or online courses focused on Matlab commands and data manipulation techniques.
Community Engagement
Join community forums, such as Matlab Central or Stack Overflow, where you can share your experiences, ask questions, and deepen your knowledge of Matlab slicing and command application.