A MATLAB timer is an object that allows you to execute a specified callback function at regular intervals or after a defined delay.
Here's a simple code snippet to create a timer that displays a message every 2 seconds:
t = timer('ExecutionMode', 'fixedRate', 'Period', 2, 'TimerFcn', @(~,~) disp('Hello, World!'));
start(t);
Remember to stop and delete the timer when you're done to free up system resources:
stop(t);
delete(t);
Understanding the Basics of MATLAB Timer
What is a Timer?
A MATLAB timer is a powerful tool that allows you to execute a specific function at designated intervals or after designated delays. It serves various purposes, such as automating repetitive tasks, performing timed operations in user interfaces, or managing data acquisition in real-time applications. Understanding how a timer works in MATLAB is critical for efficient programming and can significantly enhance your computational processes.
How Timers Work in MATLAB
In MATLAB, a timer is represented as an object, which is created and configured to fulfill your specific requirements. It uses a few fundamental terms, essential for working with timers:
- Timer Object: Represents the timer in MATLAB.
- Callback: The function that executes when the timer fires.
- Execution Modes: Determines how the timer operates (e.g., whether it runs at fixed intervals or after fixed delays).
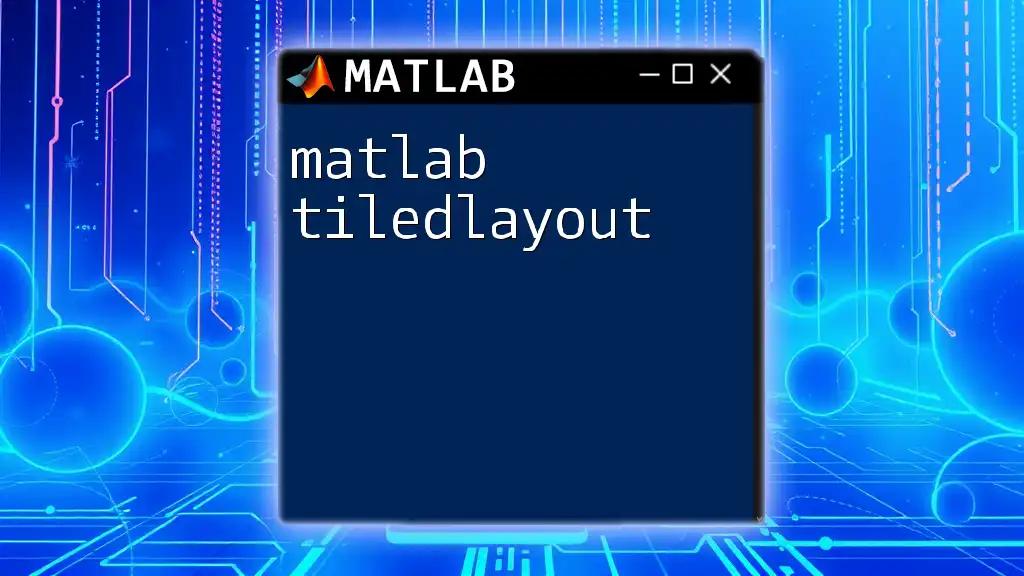
Creating a Timer in MATLAB
Basic Syntax of Timer Creation
Creating a timer in MATLAB is straightforward using the constructor:
t = timer;
This code initializes a timer object, but to make it functional, you'll need to configure its properties.
Setting Timer Properties
To effectively utilize a MATLAB timer, you must set various properties. The crucial properties include:
-
ExecutionMode: Determines how the timer behaves. Options include:
- `'fixedRate'`: Executes the function at a constant rate, regardless of how long the function takes to run.
- `'fixedDelay'`: Executes the function after a given delay, regardless of how long the function runs.
-
StartDelay: This property specifies the duration to wait before starting the timer.
-
Period: The time interval between executions.
-
TimerFcn: This property specifies the function that gets executed when the timer fires.
Here's an example of creating a timer with these fundamental settings:
t = timer('ExecutionMode', 'fixedRate', ...
'Period', 1, ...
'TimerFcn', @(~,~) disp('Timer executed'));
In this example, the timer will execute once every second, displaying the message "Timer executed".

Managing Timers
Starting and Stopping a Timer
Once a MATLAB timer is created and configured, you can manage it using the following methods:
- Start the Timer: Start the timer to initiate its execution.
start(t); % Starts the timer
- Stop the Timer: When you wish to stop the timer's execution, simply call:
stop(t); % Stops the timer
These straightforward commands make it easy to control the timer's lifecycle in your program.
Deleting a Timer
Once you're finished with a timer, or if you need to clean up your workspace, it's essential to delete the timer object to free up resources. Deleting a timer can be performed using:
delete(t); % Deletes the timer object
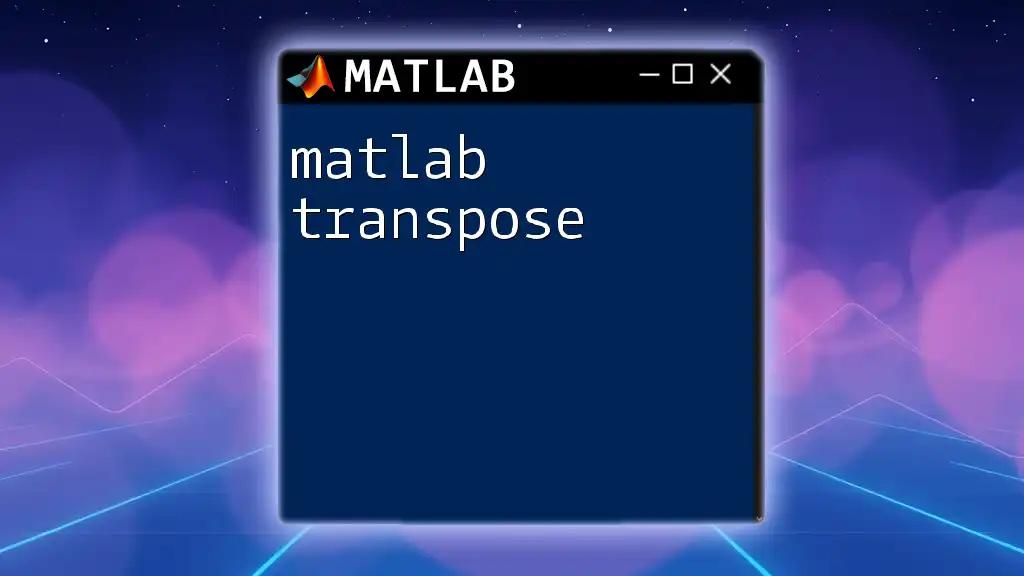
Practical Examples of Using Timers
Example 1: Simple Countdown Timer
Imagine you want to create a simple countdown timer that counts down from 10 to 0. This can be accomplished using the following code:
t = timer('ExecutionMode', 'fixedRate', ...
'Period', 1, ...
'TimerFcn', @(~, event) disp(['Time left: ', num2str(10 - event.CurrentIteration])));
start(t);
In this example, the timer executes every second and decrements a countdown, displaying the remaining time in the console.
Example 2: Periodic Data Logging
A more advanced use case is to log data at regular intervals. Here’s how you can set up a timer for periodic data logging that records random data every five seconds:
dataLog = [];
t = timer('ExecutionMode', 'fixedRate', ...
'Period', 5, ...
'TimerFcn', @(~,~) logData());
start(t);
function logData()
dataLog = [dataLog; rand(1)]; % Simulating data acquisition
disp(['Data logged: ', num2str(dataLog(end))]);
end
This example logs a new random number into the `dataLog` matrix every five seconds, demonstrating how MATLAB timers can facilitate automation in data collection.

Advanced Timer Techniques
Handling Callbacks
Callbacks are integral to the operation of timers. A callback function is executed each time the timer fires. By defining callback functions, you can execute complex logic based on the timer's state or environment.
Multi-timer Setup
Managing multiple timers is possible by creating several timer objects and controlling them independently or in coordination. Each timer can have distinct execution modes, periods, and callback functions.
Timer Error Handling
An essential aspect of using timers is handling potential runtime errors effectively. Using a `try-catch` block within your callback functions allows you to gracefully manage exceptions and avoid crashing your program.

Best Practices for Using Timers
Avoiding Common Pitfalls
When using MATLAB timers, be cautious of the following pitfalls:
- Overlapping Executions: Ensure your callback functions execute quickly enough to prevent overlapping occurrences, which can lead to performance issues.
- Resource Management: Always remember to delete timers when they're no longer needed to free up system resources.
Debugging Timer Issues
Debugging timer-related problems can be tricky, but the following strategies can help identify and resolve issues:
- Use `disp` statements or log messages in your callback functions to trace execution.
- Monitor the state of your timer using properties like `Running` and `TasksExecuted`.
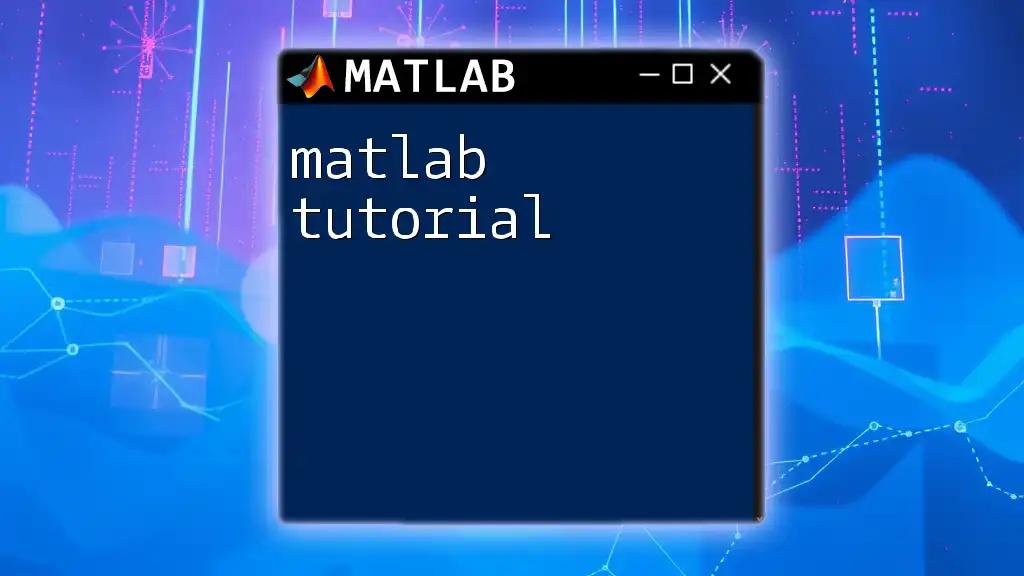
Conclusion
In this article, we explored the functionality and utility of the MATLAB timer. We discussed how to create and configure timers, manage their lifecycle, implement practical examples, and delve into advanced techniques. Timers offer a versatile solution for automating tasks and enhancing the performance of your MATLAB scripts. By experimenting with MATLAB timers, you can unlock new possibilities in your programming endeavors and develop innovative solutions that respond to time-based requirements.
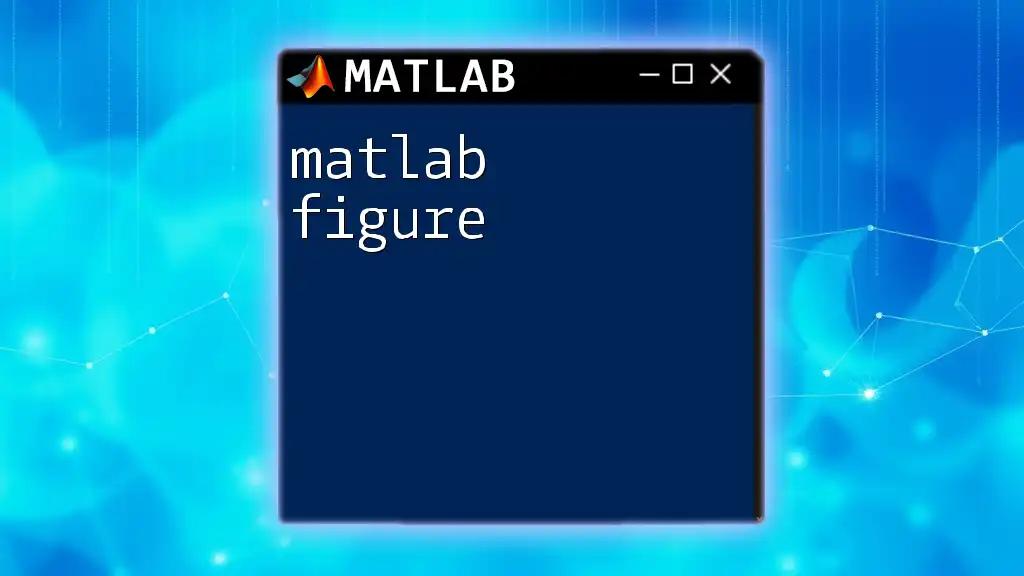
Additional Resources
For further mastering the MATLAB timer, you may explore the official MATLAB documentation, which offers comprehensive insights into the subject. Additionally, consider checking out tutorials and videos that elaborate on using timers to solidify your understanding and application of this powerful tool.