In MATLAB, tables are a data type used to store dataset variables as columns, enabling easy access and manipulation of data within a structured format.
Here's a code snippet to create a simple table:
% Creating a sample table in MATLAB
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 22];
Heights = [65; 70; 68];
T = table(Names, Ages, Heights)
Understanding Tables in MATLAB
What is a Table?
A table in MATLAB is a data type that allows you to store data with mixed types in a column-oriented way. Unlike conventional arrays that can only hold one data type, tables can contain different types across the columns (e.g., numbers, strings, and categorical variables). This structure makes tables especially useful for data analysis and reporting, as they can easily handle real-world datasets that often contain various types of observations.
Table Properties
Tables in MATLAB come with several important properties:
- Data Types Supported: Tables can contain numeric, categorical, and text data. This allows for greater flexibility when dealing with complex datasets.
- Row Names and Variable Names: Each table consists of rows and columns, where each column is called a variable. You can assign names to both rows and columns for easier reference.
- Data Organization: Tables automatically manage the relationship between different data points through variable names, enhancing the readability and organization of your data.
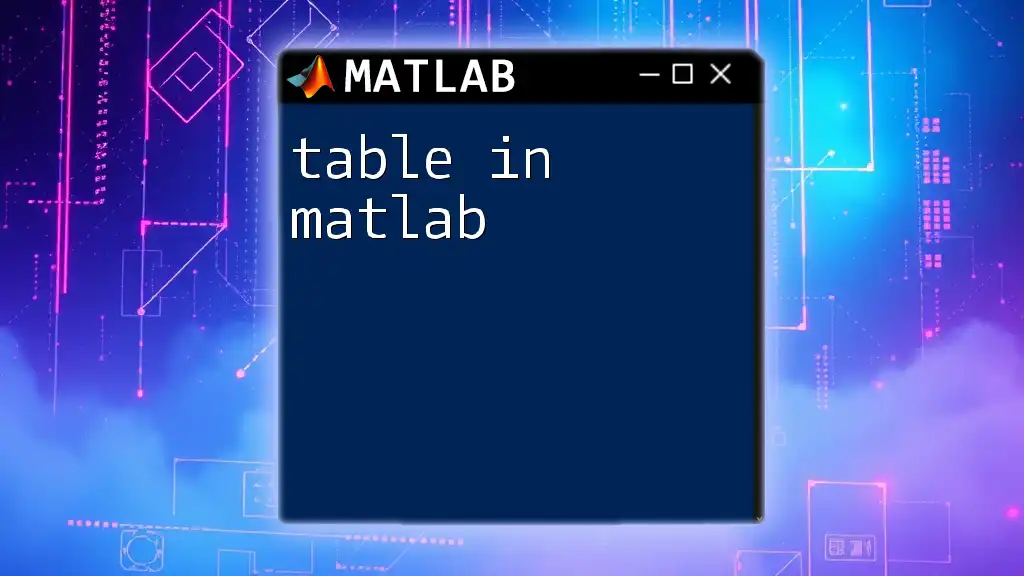
Creating Tables
Creating Tables from Variables
You can create a table using the `table` function directly by passing in the variable data. For example, if you have some data on individuals' ages and heights, you can create a simple table as follows:
age = [24; 30; 22];
height = [180; 175; 172];
names = {'Alice'; 'Bob'; 'Charlie'};
T = table(names, age, height);
In this example, the table `T` comprises three columns: `names`, `age`, and `height`. Each row corresponds to a different individual, making the data easy to read and manipulate.
Importing Data into Tables
You can also import external data into tables. MATLAB provides convenient functions like `readtable` that simplify this process. For instance, if you have a CSV file containing data:
T = readtable('data.csv');
This command will read the data in `data.csv` and convert it into a MATLAB table. This is especially useful when handling large datasets stored in various formats.
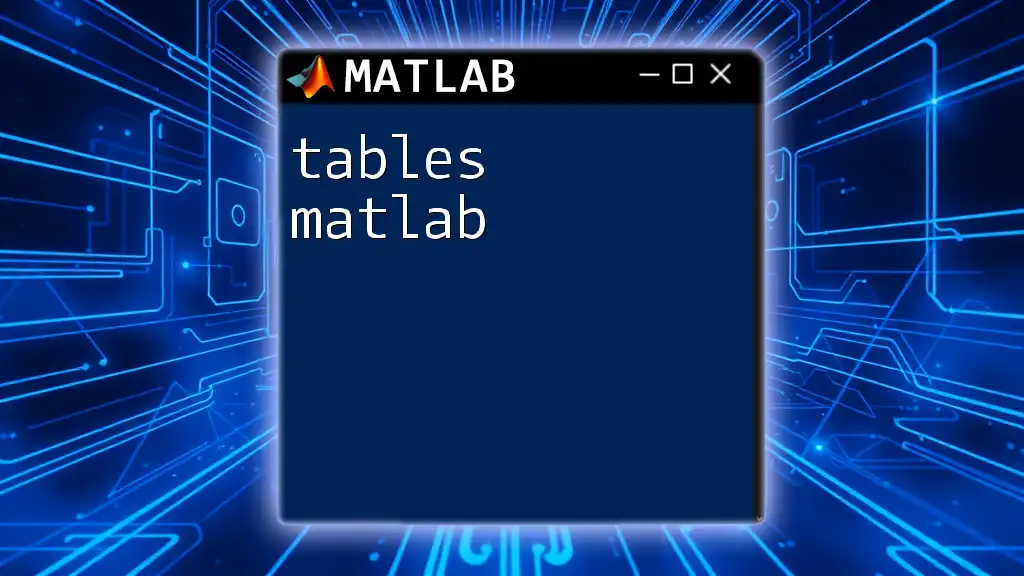
Inspecting Tables
Viewing Table Data
To display the contents of your table, you can use functions like `disp` alongside the variable names. This shows the table's entire content, but when you want to view just a portion of it, the `head` and `tail` functions can be invaluable.
For example:
head(T);
tail(T);
These commands will display the first and last few rows of the table, respectively.
Summary and Statistics
The `summary` function provides a quick statistical overview of your table. It offers insights into each variable's data type, the number of entries, and summary statistics.
summary(T);
Using this command can help you quickly understand the structure and contents of your dataset, identifying potential anomalies or areas requiring further investigation.
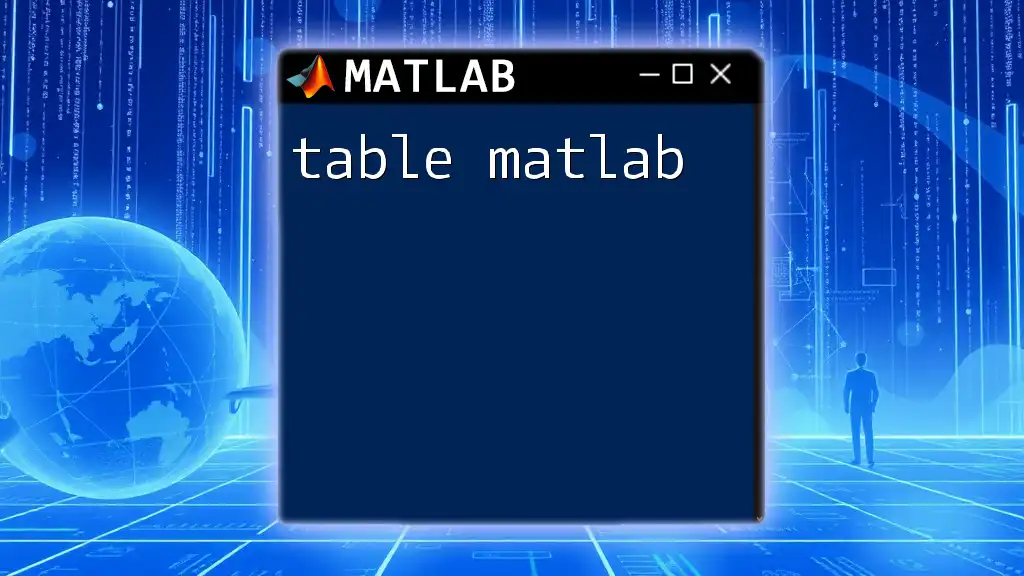
Manipulating Tables
Accessing Table Data
To manipulate a table effectively, you need to access its data efficiently.
Column Access
You can access specific columns by their variable names. For example, to obtain the ages of individuals stored in the previous table:
ages = T.age;
Row Access
Accessing rows can be accomplished using numeric indices or logical indexing. For instance, if you want to access all rows where `age` is greater than 25:
olderThan25 = T(T.age > 25, :);
This command will create a new table, `olderThan25`, containing only those entries that meet the specified condition.
Modifying Tables
Adding and Removing Rows
It is straightforward to append or delete rows in a table. To add a new row:
newRow = table({'Diana'}, 29, 165, 'VariableNames', {'Names', 'Age', 'Height'});
T = [T; newRow];
In this example, we create a new table for `Diana` and concatenate it with the existing table`T`.
Adding and Removing Columns
You can easily add a new variable (column) to your table. For example, to add a column for weights:
weight = [68; 75; 70; 65];
T.Weight = weight;
This modification enriches our dataset by including additional information.
Sorting and Filtering Tables
Tables can be sorted using the `sortrows` function. To sort `T` by the `age` variable:
T = sortrows(T, 'Age');
This rearranges the rows of `T` in ascending order based on the `Age` column.
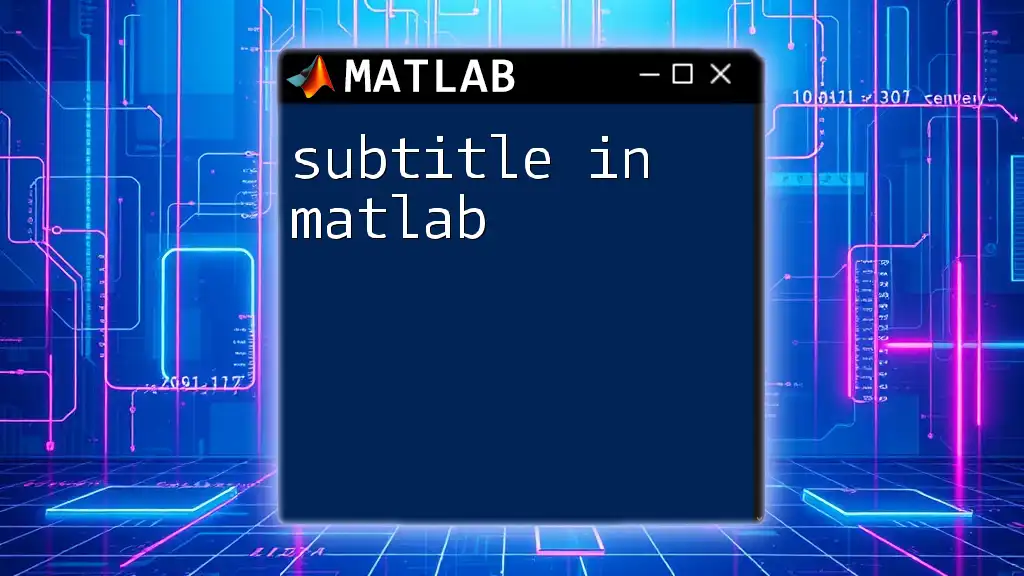
Advanced Table Operations
Joining Tables
Sometimes, you may need to combine multiple tables. MATLAB offers functions such as `innerjoin` and `outerjoin` for this purpose. For instance, to join two tables by a common key:
T1 = table([1; 2; 3], {'A'; 'B'; 'C'}, 'VariableNames', {'ID', 'Value'});
T2 = table([2; 3; 4], {'X'; 'Y'; 'Z'}, 'VariableNames', {'ID', 'Status'});
joinedTable = innerjoin(T1, T2);
This command creates a table that includes only the rows with matching `ID` values from both `T1` and `T2`.
Grouping Data
When you want to analyze subsets of data, grouping is essential. The `groupsummary` function allows you to generate summary statistics based on groups within your data. For example, to compute the average age by group:
T.Group = categorical({'Group1'; 'Group2'; 'Group1'});
summary = groupsummary(T, 'Group', 'mean', 'Age');
This command generates a summary table displaying average ages categorized by groups.
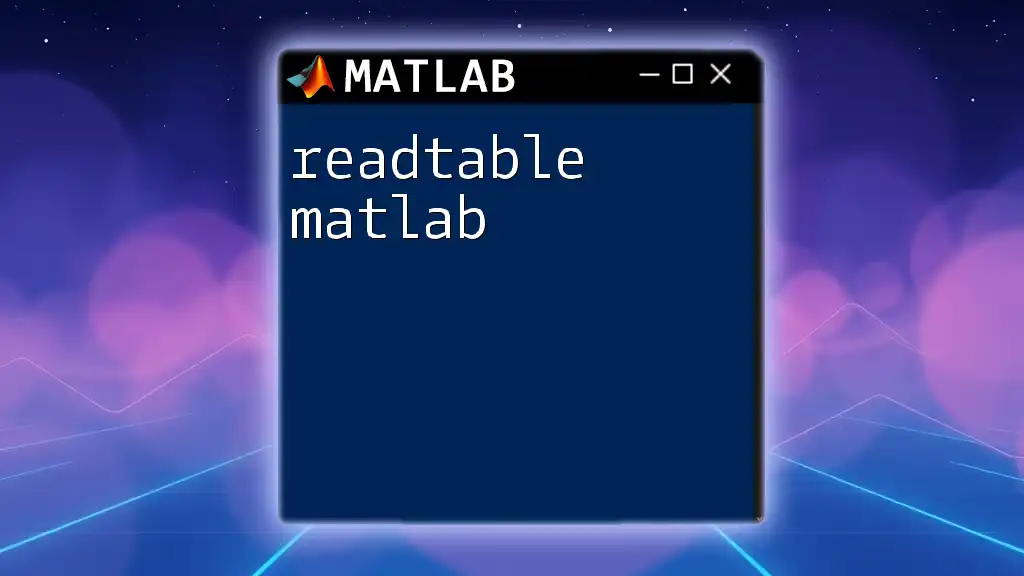
Best Practices for Using Tables
When utilizing tables in MATLAB, several best practices will enhance your experience and ensure data integrity:
- Consistent Naming Conventions: Ensure that you use a uniform naming structure for your variable names. This practice fosters easier referencing and reduces the chances of errors while accessing data.
- Keeping Data Types Uniform: Strive to maintain uniform data types within each column. This consistency can significantly improve performance, especially when performing computations or analyses on the data.
- Descriptive Variable Names: Use clear and descriptive variable names for better readability. This practice will make your data self-explanatory and facilitate collaboration with others.
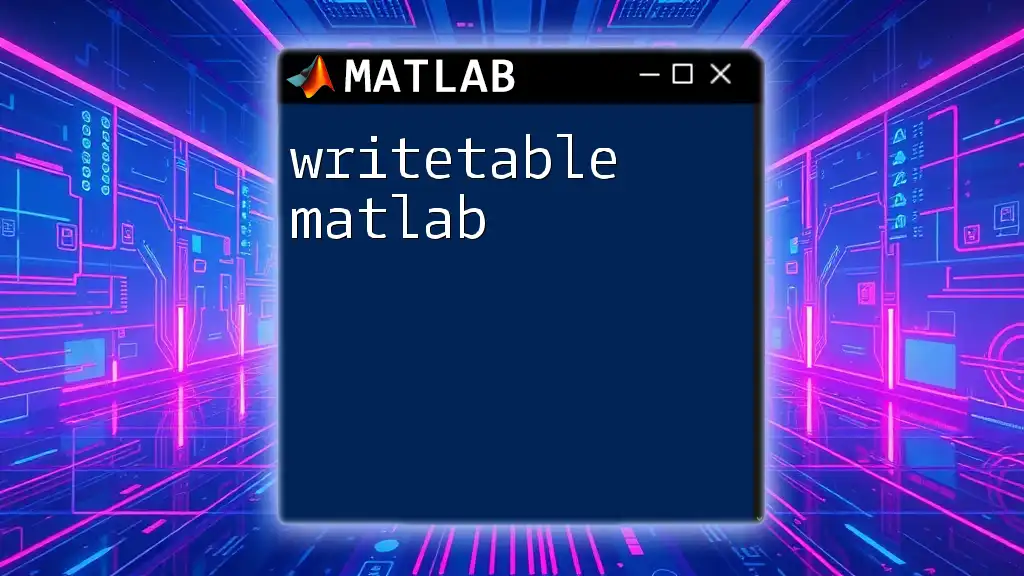
Conclusion
Tables in MATLAB serve as a powerful tool for organizing and analyzing data with mixed types. With their ease of use and versatility, they enable effective data manipulation, exploration, and summary. Embracing tables in your MATLAB workflow can drastically enhance your data analysis capabilities, making complex datasets significantly more manageable. Experimenting with tables will deepen your understanding and allow you to leverage their full potential in MATLAB.
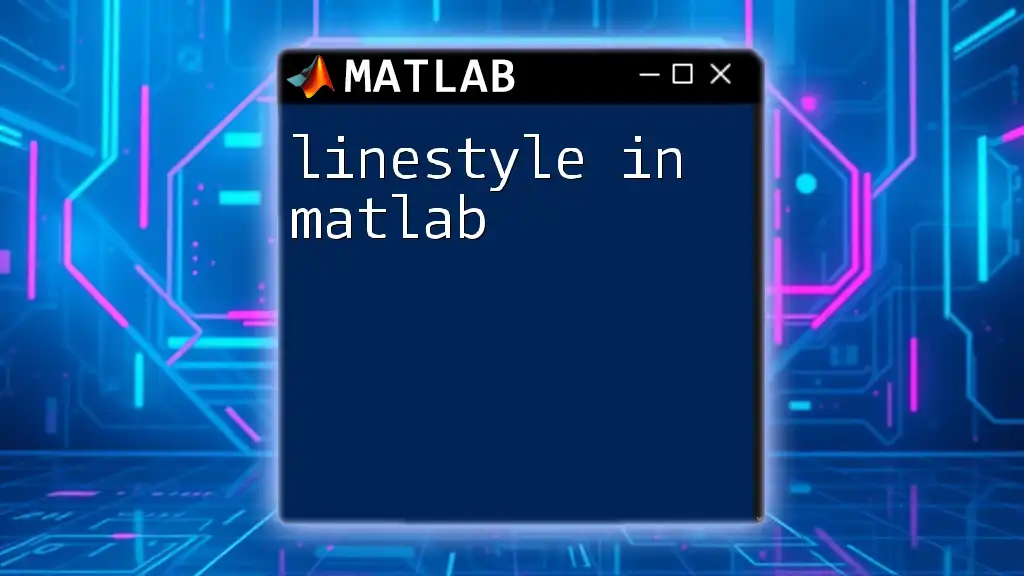
Additional Resources
For further exploration, accessing the MATLAB documentation on tables can provide you with additional insights and usage examples. Additionally, consider seeking out tutorials and seminars focused on tables in MATLAB to expand your knowledge even further.