MathWorks MATLAB is a high-level programming language and interactive environment designed for numerical computation, visualization, and programming, enabling users to analyze data and develop algorithms efficiently.
Here's a simple example demonstrating how to create a plot in MATLAB:
x = 0:0.1:10; % Create an array of values from 0 to 10 with an increment of 0.1
y = sin(x); % Calculate the sine of each value in x
plot(x, y); % Plot the sine wave
title('Sine Wave'); % Add a title to the plot
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
Getting Started with MATLAB
Installation and Setup
System Requirements
Before you embark on your MATLAB journey, ensure that your system meets the requirements. MATLAB typically needs a minimum of 4 GB of RAM (8 GB or more is recommended), reasonable disk space (around 20 GB), and compatibility with major operating systems such as Windows, macOS, and Linux.
Downloading MATLAB
To begin, visit the official [MathWorks website](https://www.mathworks.com/). Create an account, choose the appropriate license type, and initiate the download process. Educational users may benefit from discount options, while students can access MATLAB at a lower price through their institutions.
Installation Process
Once you have the installer, running it is straightforward. Follow the on-screen instructions, and be sure to select components essential to your work. Choose whether to install the MATLAB toolboxes that you may need later on.
First Steps in MATLAB
MATLAB Interface Overview
Upon launching MATLAB, you’ll encounter several key elements. The Command Window is where you type your commands and see the results. The Workspace shows the variables you create during your session, while the Editor is used for writing scripts and functions.
Creating Your First Script
Once you’re familiar with the interface, it’s time to create your first MATLAB script. A simple command to display a greeting can be done as follows:
disp('Hello, World!');
This code snippet will output "Hello, World!" in the Command Window. Understanding how to execute your script and view results is fundamental in your MATLAB journey.
Basic Commands and Functions
Familiarize yourself with essential commands:
- `clc`: Clears the Command Window.
- `clear`: Removes all variables from the workspace.
- `close all`: Closes all figure windows.
These commands are useful for ensuring a clean slate for your coding endeavors.
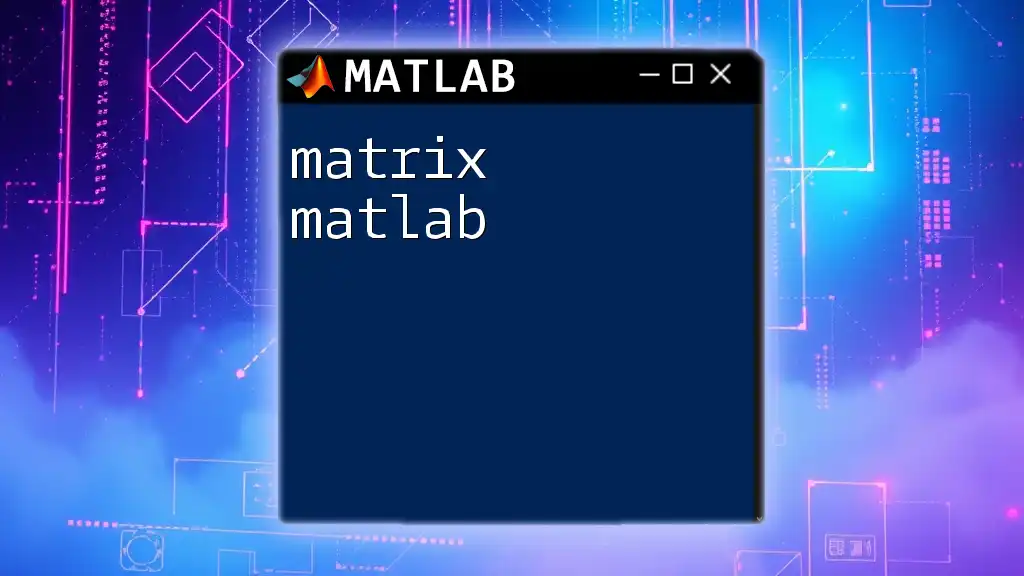
Core MATLAB Concepts
Variables and Data Types
Understanding Variables
Variables store data for processing in MATLAB. To create a variable, simply assign a value using the equal sign `=`. For example:
x = 10;
This line creates a variable named `x` with a value of 10.
Common Data Types
MATLAB primarily operates with:
- Scalars: Single values like `5` or `3.14`.
- Arrays: 1D and 2D matrices, e.g., `A = [1, 2, 3; 4, 5, 6]`.
- Strings: Textual data, expressed as `str = 'Hello, MATLAB!'`.
- Structures: Allows you to group different types of data together.
Each data type has its own set of operations and usage scenarios.
Operators and Expressions
Mathematical Operators
MATLAB supports standard arithmetic operations. For instance:
- Addition: `+`
- Subtraction: `-`
- Multiplication: `*`
- Division: `/`
For example, to add two numbers:
result = 5 + 3; % result will be 8
Relational and Logical Operators
Relational operators help compare values. For instance:
- Equal to: `==`
- Greater than: `>`
- Less than: `<`
An example usage:
a = 10;
b = 5;
result = a > b; % result will be true (1) since 10 is greater than 5
Logical operators include `&&` (and), `||` (or), and `~` (not) for creating complex logical conditions.
Control Structures
Conditional Statements
The use of conditional statements is pivotal in programming. Example:
if x > 0
disp('x is positive');
elseif x < 0
disp('x is negative');
else
disp('x is zero');
end
This code checks the value of `x` and displays a corresponding message depending on its value.
Loops and Iteration
Loops are essential for repeating tasks. A `for` loop example is:
for i = 1:5
disp(i);
end
This will print numbers from 1 to 5 in the Command Window.
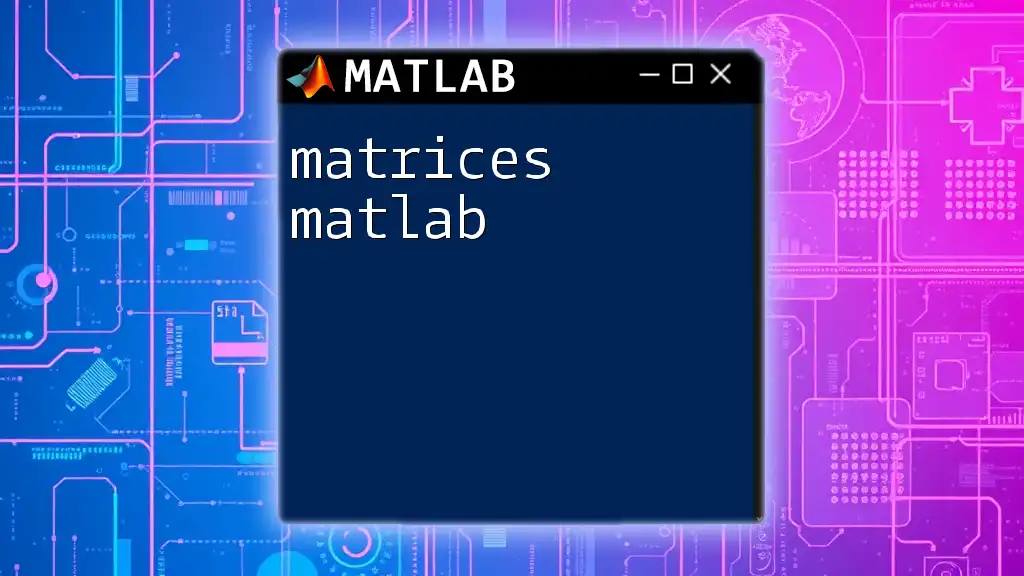
Functions and Scripts
Creating Functions
Defining Functions
Functions encapsulate code for reuse and organization. The syntax starts with the `function` keyword. Below is an example that squares an input value:
function output = myFunction(input)
output = input^2;
end
You can invoke this function in your script by calling `result = myFunction(4);`, which will return `16`.
Scripting vs. Function Files
Scripts are a linear sequence of MATLAB commands, while function files can accept inputs and return outputs. Use scripts for simple tasks, but functions are better for repetitive tasks or complex calculations.
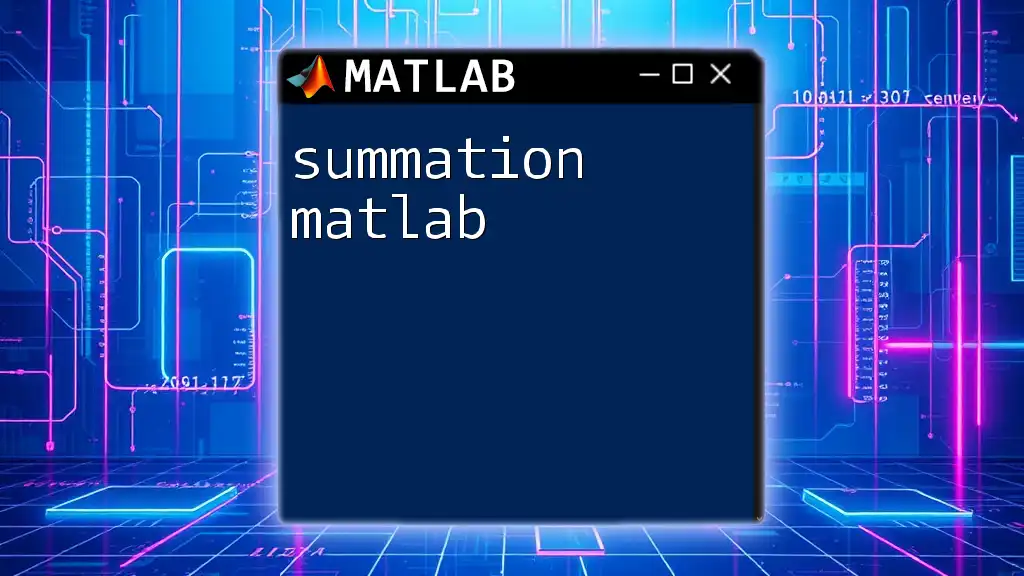
Advanced MATLAB Features
Plotting and Visualization
Basic Plotting Techniques
MATLAB excels in plotting capabilities. A simple sine wave plot can be created with:
x = 0:0.01:2*pi;
y = sin(x);
plot(x, y);
title('Sine Wave');
xlabel('x');
ylabel('sin(x)');
This snippet visualizes the sine function, adding a title and labels for clarity.
Data Import and Export
Importing Data
MATLAB makes it easy to work with external data files. To read data from a `.csv` file, you can use:
data = readtable('yourfile.csv');
This function imports your data into a table format, making it easy to manipulate.
Exporting Data
Once you have processed your data, you may wish to save it. For instance, exporting a matrix to a CSV file:
csvwrite('output.csv', outputMatrix);
This command saves `outputMatrix` to a file called `output.csv`.
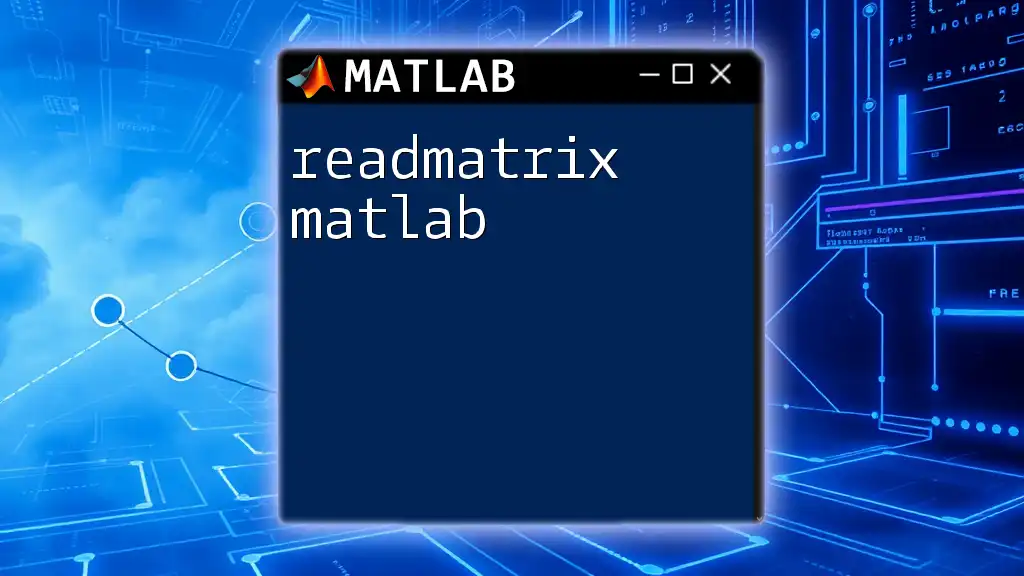
Troubleshooting and Debugging
Common Errors and How to Fix Them
Syntax Errors
Syntax errors often arise from typos or incorrect command usage. MATLAB typically provides error messages that guide you to the line of code containing the issue.
Logic Errors
These arise when the code runs without crashing but produces incorrect results. Debugging tools, such as breakpoints, can be critical in identifying where logic deviates from expectations.
Debugging Tools
Using Breakpoints
Setting breakpoints in the editor allows you to pause execution and analyze code at specific points. It can be done by clicking on the left margin next to the line number in your script.
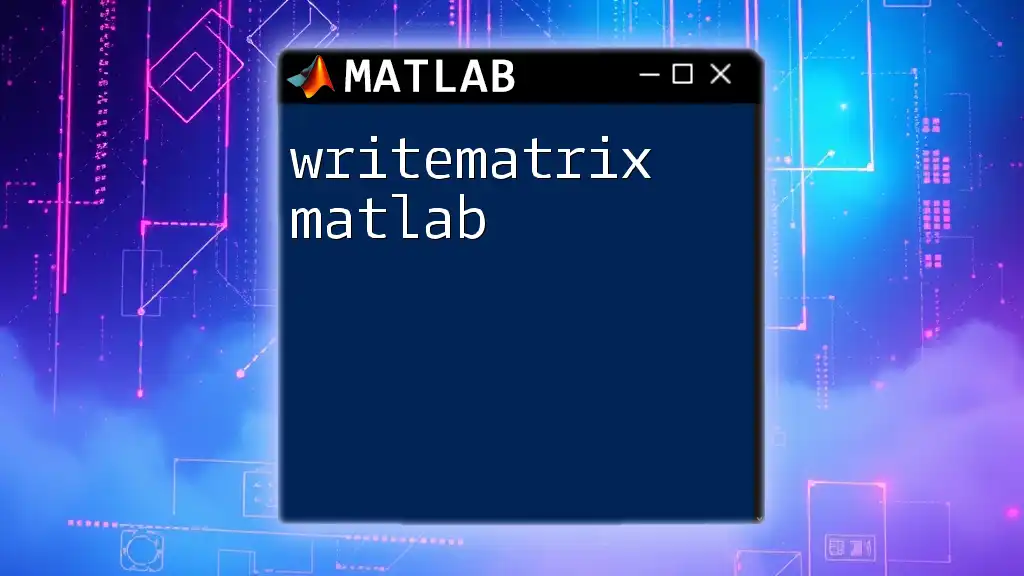
Conclusion
Recap of Key MATLAB Features
This guide covered essential aspects of MathWorks MATLAB, from installation to coding fundamentals, graphics, and data handling. The environment is powerful for anyone looking to perform numerical computations, data analysis, and visualization.
Further Learning Resources
For more in-depth learning, the official [MATLAB Documentation](https://www.mathworks.com/help/matlab/) is immensely valuable. Seek out online platforms offering targeted courses to enhance your skills and apply MATLAB's features in practical situations.
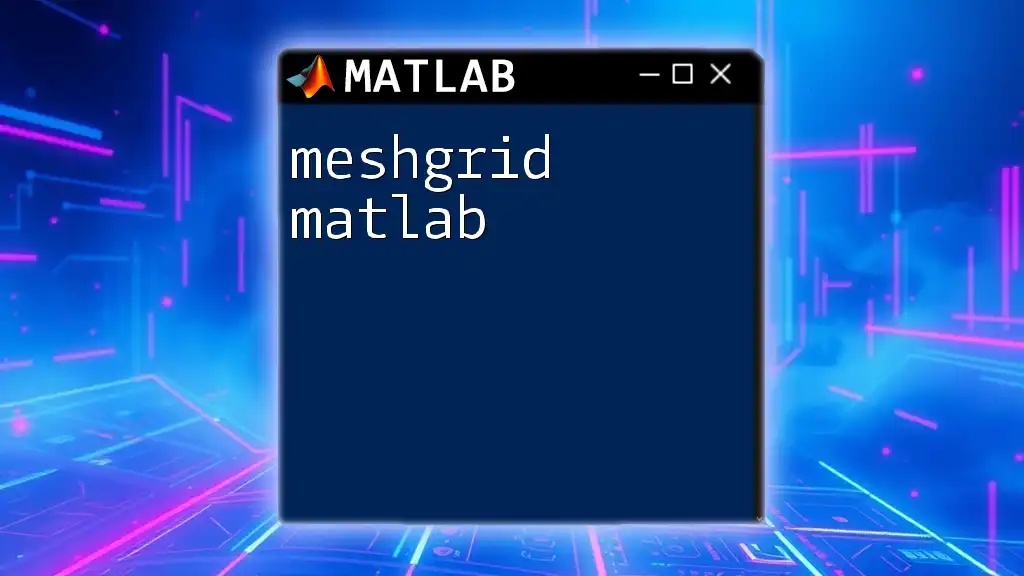
Call to Action
Dive deeper into MATLAB applications, experiment with different functionalities, and consider enrolling in our courses to harness the true potential of MathWorks MATLAB!