A nested for loop in MATLAB allows you to create loops within loops, enabling the iteration over multi-dimensional data structures.
for i = 1:3
for j = 1:2
fprintf('i = %d, j = %d\n', i, j);
end
end
Understanding the Basics of For Loops
What is a For Loop?
A for loop is a fundamental control flow statement in MATLAB that allows you to execute a block of code a specific number of times. It iterates over a sequence of values, which can be particularly useful when you want to automate repetitive tasks or perform operations on arrays and matrices.
The basic syntax of a for loop in MATLAB is:
for index = start:increment:end
% loop body
end
In this structure:
- index is the loop variable that changes with each iteration.
- start is the initial value.
- increment specifies the step size, which is optional and defaults to 1.
- end is the final value that the loop can reach.
Simple For Loop Example
Here is a simple example of a for loop that prints numbers from 1 to 5:
for i = 1:5
disp(i);
end
This loop starts with `i = 1` and continues incrementing `i` by 1 until it reaches 5, displaying each number in the console.
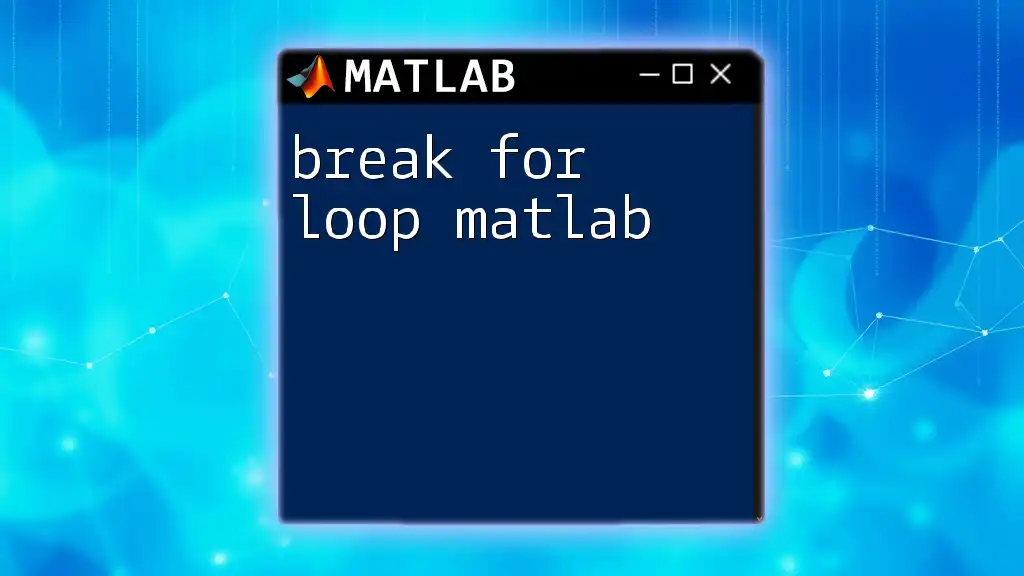
Introduction to Nested For Loops
Definition of Nested For Loops
A nested for loop is a loop inside another loop. This structure allows you to perform complex iterations, such as iterating over a 2D array or generating combinations of items.
Using nested loops can help you efficiently traverse multidimensional data structures. The outer loop runs once for each value of its index, and for each iteration of the outer loop, the inner loop runs completely.
Syntax of Nested For Loops
The basic syntax for a nested for loop is as follows:
for i = 1:n
for j = 1:m
% loop body
end
end
In this case, for every iteration of the outer loop (controlled by `i`), the inner loop (controlled by `j`) iterates through its entire range.
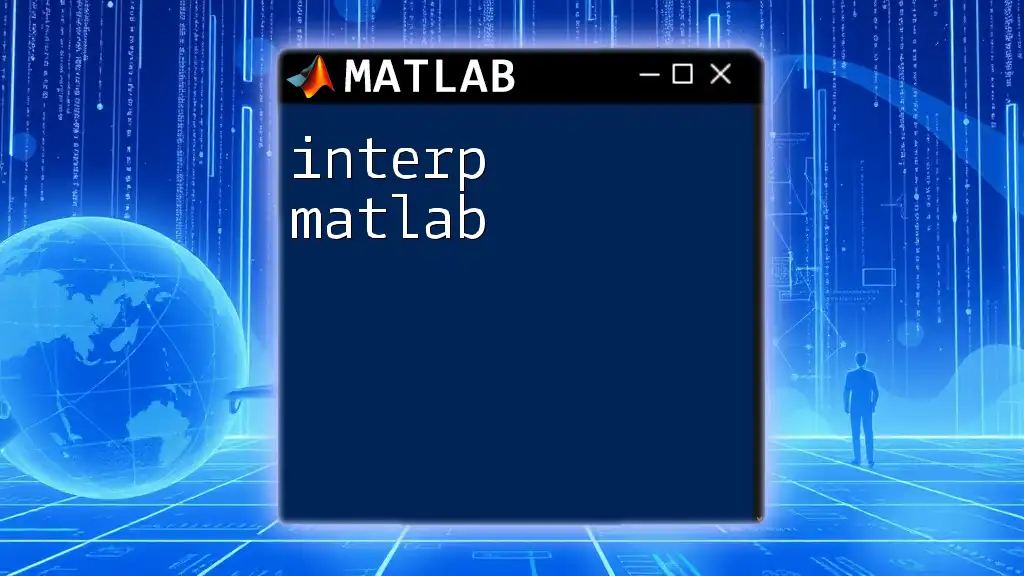
Practical Applications of Nested For Loops
Iterating through Multidimensional Arrays
One of the most common applications of nested for loops is iterating through multidimensional arrays. This capability is invaluable when working with matrices or higher-dimensional datasets.
For example, consider a 3x3 matrix:
A = [1 2 3; 4 5 6; 7 8 9];
for i = 1:size(A, 1)
for j = 1:size(A, 2)
fprintf('Element at (%d, %d): %d\n', i, j, A(i, j));
end
end
In this snippet:
- The outer loop iterates over the rows, and the inner loop iterates over the columns.
- This setup allows you to print every element in the matrix along with its indices.
Generating Combinations
Nested for loops are also useful for generating combinations of items. For instance, if you want to pair colors with sizes, you can use nested loops like this:
colors = {'Red', 'Green', 'Blue'};
sizes = {'S', 'M', 'L'};
for i = 1:length(colors)
for j = 1:length(sizes)
fprintf('Combination: %s %s\n', colors{i}, sizes{j});
end
end
In this example:
- The outer loop iterates through the color array, while the inner loop cycles through the size array, allowing you to print each combination.
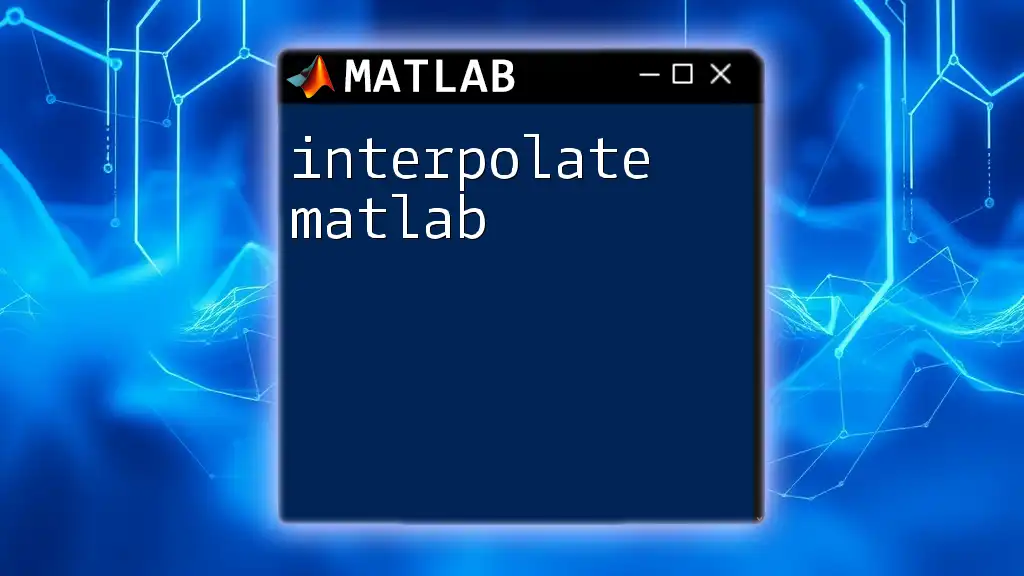
Performance Considerations
Time Complexity of Nested For Loops
When considering nested for loops, it’s essential to understand how they can impact performance. The time complexity grows significantly with the number of nested loops. The general complexity can be represented as O(n * m), where `n` and `m` are the size of the outer and inner loops, respectively. This means that if you double either loop's size, your loop's runtime potentially quadruples.
Tips for Optimizing Nested Loops
Here are some strategies to enhance the performance of your nested loops:
- Minimize Iterations: If possible, reduce the range of your loops or pre-calculate values to avoid repeated calculations.
- Utilize MATLAB Vectorization: MATLAB is optimized for matrix operations. Whenever possible, avoid loops by using array operations or built-in functions which can perform the same tasks more efficiently.
- Cache Results: If you are calculating values that do not change within the loop, caching those results can save time.
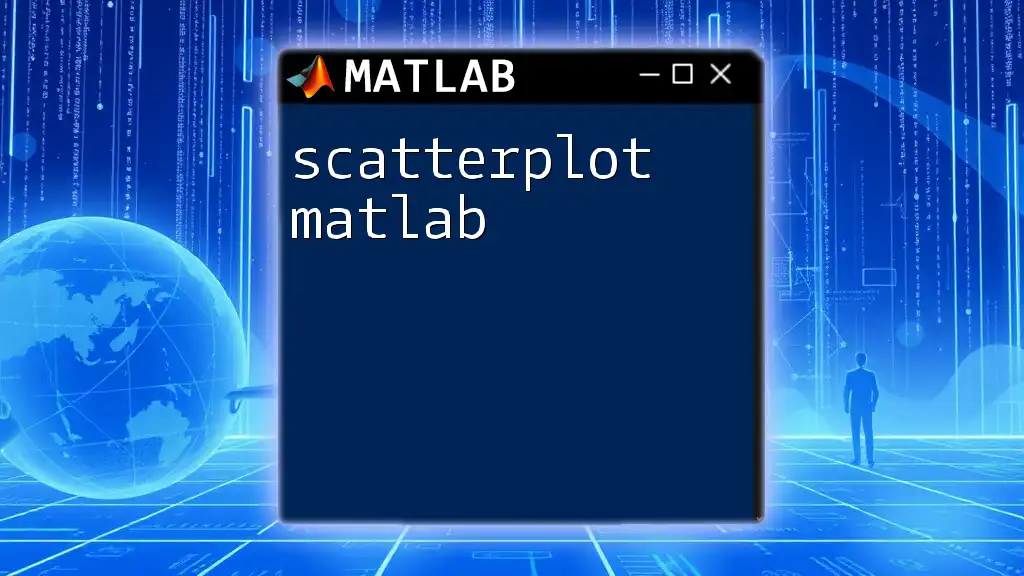
Debugging Nested For Loops
Common Issues and Errors
When working with nested for loops, common pitfalls include:
- Infinite Loops: Forgetting to update the loop variable in the inner loop can lead to infinite execution.
- Index Out of Bounds: Trying to access an element outside the limits of an array can throw an error.
Debugging Techniques
If you encounter issues with your nested loops, consider these debugging techniques:
- Using the MATLAB Debugger: You can set breakpoints in your code to stop execution and inspect variables.
- Verbose Output: Adding `disp()` or `fprintf()` statements can help track variable values at different points in the execution. This can help identify where things might be going wrong.
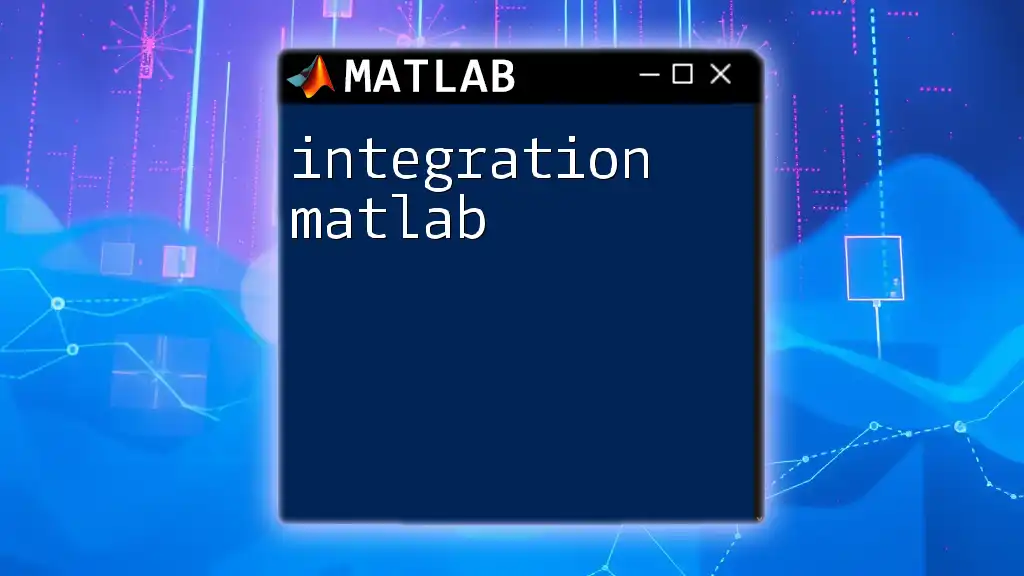
Conclusion
Nested for loops are indispensable tools when dealing with complex data structures in MATLAB. By understanding their syntax, applications, performance considerations, and debugging techniques, you can harness their power effectively. Practicing with nested loops will enhance your MATLAB skillset, making you proficient in data manipulation and iterative processing.
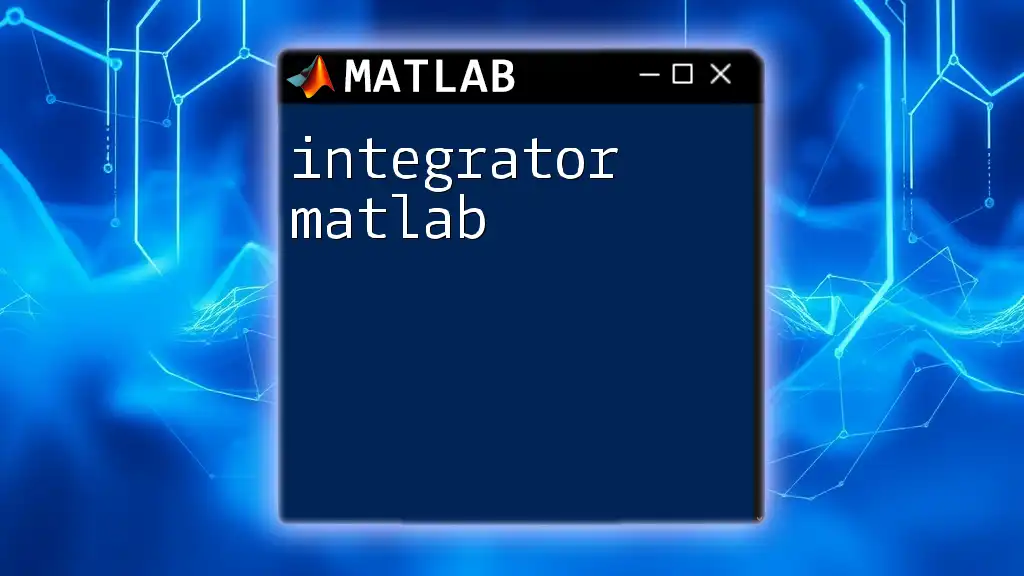
Additional Resources
For those looking to deepen their understanding, exploring MATLAB's official documentation on loops can provide extensive insights and more advanced topics. Additionally, consider blogs and online courses that offer step-by-step tutorials for further practice.
By continuing to engage with MATLAB commands, you will quickly gain confidence in using nested for loops, among other vital concepts.