The `regexprep` function in MATLAB is used to replace occurrences of a specified pattern in strings with a new substring, allowing for powerful text manipulation through regular expressions.
Here’s a simple example of using `regexprep` to replace all occurrences of "cat" with "dog" in a string:
originalString = 'The cat sat on the mat.';
newString = regexprep(originalString, 'cat', 'dog');
disp(newString); % Outputs: The dog sat on the mat.
Understanding Regular Expressions
Regular expressions (regex) are powerful sequences of characters used to match patterns in strings. They provide a flexible and efficient means to search, edit, and manipulate textual data. In MATLAB, regex is implemented through various functions, with `regexprep` being one of the most noteworthy for its ability to perform replacements based on regex matching.
Basics of Regular Expressions
Before diving into `regexprep`, it’s essential to grasp the fundamentals of regular expressions. Here are some common regex tokens:
- `.` - Matches any single character.
- `\d` - Matches any digit; equivalent to `[0-9]`.
- `\w` - Matches any word character; equivalent to `[a-zA-Z0-9_]`.
- `^` - Asserts the start of a line.
- `$` - Asserts the end of a line.
For instance, the regex `\w+` matches one or more consecutive word characters, allowing for convenient word detection.
How Regex Works in MATLAB
MATLAB's regex functionality is robust yet slightly different from implementations in other languages like Python or JavaScript. It is critical to familiarize yourself with these nuances to avoid common pitfalls. For example, MATLAB requires double escaping in certain scenarios, such as `\\d` instead of `\d`.
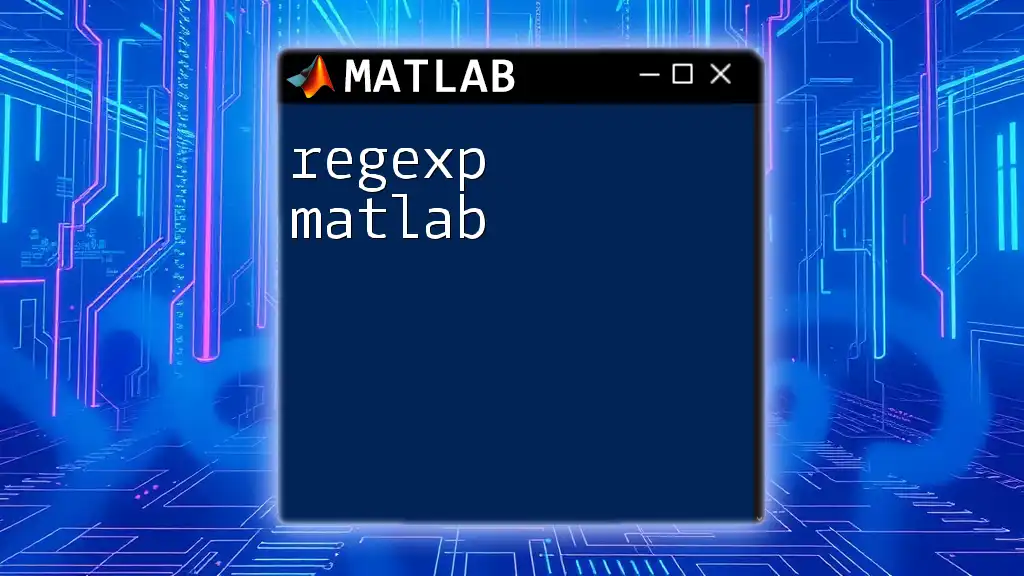
Function Syntax and Structure
To effectively use the `regexprep` function, familiarity with its syntax is crucial. The basic structure is as follows:
newStr = regexprep(str, expression, replace);
This command performs a search-and-replace operation on the string `str`, wherein `expression` defines the regex pattern to be matched, and `replace` indicates the replacement string.
Parameters of `regexprep`
-
`str`: This is the input string that you want to perform replacements on. It could be a character array or a cell array of strings.
-
`expression`: This parameter defines the regex pattern you want to search for within `str`. It can incorporate various regex tokens and flags to optimize your search.
-
`replace`: This specifies what you want to substitute for any matches found. It can include matched groups using `$n` (where `n` refers to the group number).
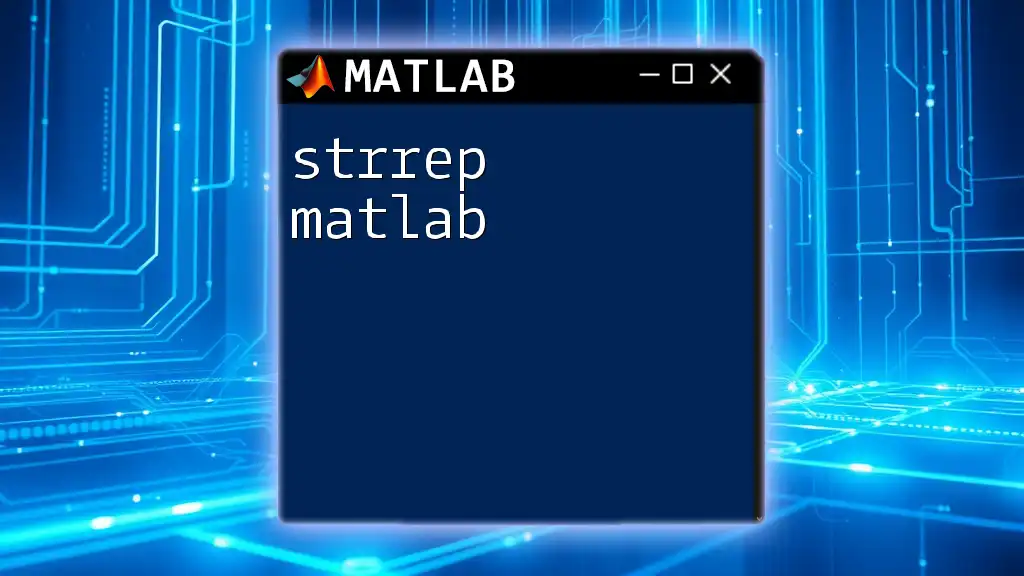
Examples of Using `regexprep`
Basic Example: Replacing Simple Text
To start, consider a straightforward replacement scenario where we replace the word "World" with "MATLAB":
originalStr = 'Hello World';
newStr = regexprep(originalStr, 'World', 'MATLAB');
disp(newStr); % Output: Hello MATLAB
In this example, `regexprep` identifies the word "World" in the string and replaces it with "MATLAB".
Using Regex Patterns for Substitution
Let’s explore a regex pattern that targets digits specifically. The following code replaces each digit in the string "User123" with an asterisk:
originalStr = 'User123';
newStr = regexprep(originalStr, '\d', '*');
disp(newStr); % Output: User***
Here, `\d` is the regex pattern that finds digits, and each occurrence is replaced by `*`, showing how `regexprep` can effectively mask sensitive information.
Advanced Example: Case Insensitive Replacement
For cases requiring case insensitivity, you can utilize the `ignorecase` option. The next snippet demonstrates this by replacing both occurrences of "matlab" regardless of capitalization:
originalStr = 'I love MATLAB and matlab';
newStr = regexprep(originalStr, 'matlab', 'Python', 'ignorecase');
disp(newStr); % Output: I love Python and Python
The output confirms that both variations of "MATLAB" were altered to "Python".
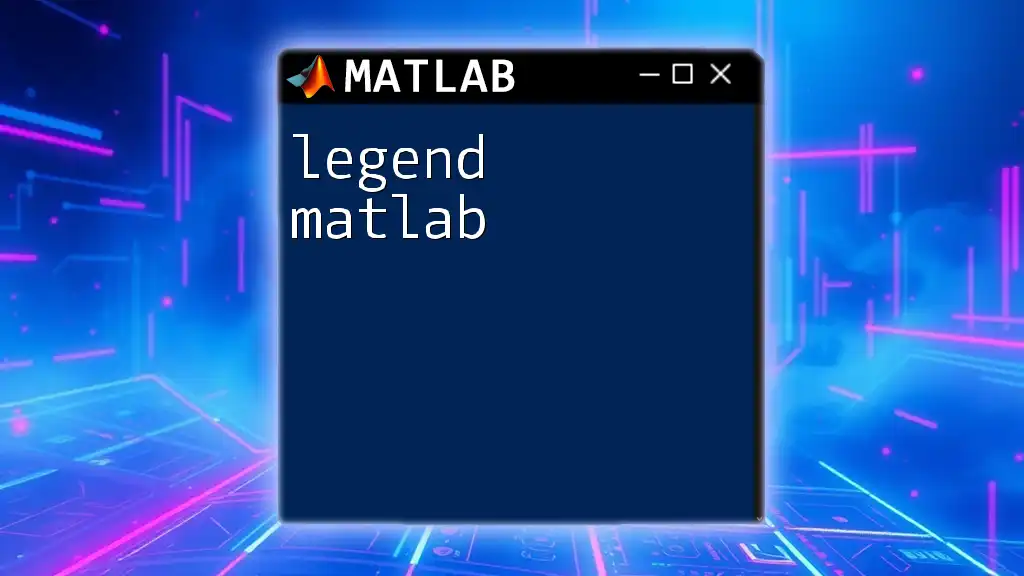
Common Use Cases of `regexprep`
Cleaning Data Strings
Data cleaning is a typical application of `regexprep`. For instance, if you want to remove unwanted punctuation from a string like "Hello!!! MATLAB??", the following command can be employed:
originalStr = 'Hello!!! MATLAB??';
newStr = regexprep(originalStr, '[!?]', '');
disp(newStr); % Output: Hello MATLAB
In this case, the regex pattern `[!?]` matches any exclamation or question mark to facilitate the cleanup of the string.
Formatting Text
Another application involves formatting text. For example, transforming a date string from "2023-10-01" into "01/10/2023" can be achieved with this code:
dateStr = '2023-10-01';
newDateStr = regexprep(dateStr, '(\d{4})-(\d{2})-(\d{2})', '$3/$2/$1');
disp(newDateStr); % Output: 01/10/2023
The capture groups `(\d{4})`, `(\d{2})`, and `(\d{2})` allow us to rearrange the date components effectively.
Validating Strings
Validation is another powerful use of `regexprep`. Suppose you want to check if an email is correctly formatted. Here’s how you can use regex to validate:
emailStr = 'example@example.com';
isValid = ~isempty(regexprep(emailStr, '^[\w.+-]+@[\w-]+\.[a-z]{2,}$', ''));
disp(isValid); % Output: true/false
Here, if `emailStr` matches the regex pattern designated for valid emails, `isValid` will return true, signifying that the email format is correct.
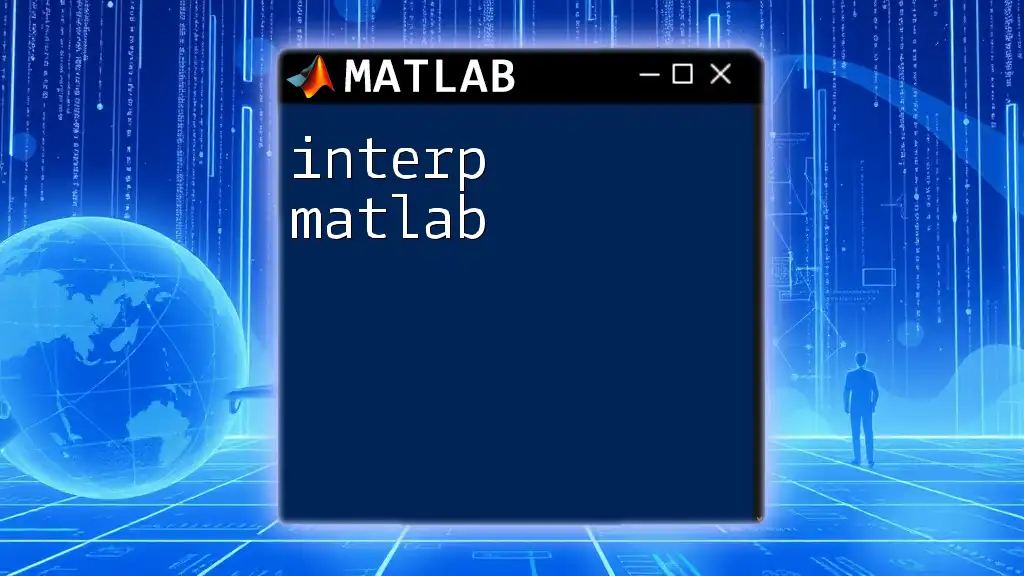
Advanced Features
Using Capture Groups
Capture groups enhance the functionality of regex replacements by allowing dynamic references. For instance, consider this example, which duplicates matched pairs:
originalStr = 'xyxyxy';
newStr = regexprep(originalStr, '(xy)', '$1$1');
disp(newStr); % Output: xyxyxyxyxy
The regex `(xy)` captures instances of "xy" and replaces them with "xyxy", effectively doubling them.
Options and Flags
There are additional options available with `regexprep`, including `lineanchors` that assert the match only at the beginning or end of lines, and `ignorecase`, which allows matches to be case insensitive—both of which enhance your control over the pattern matching.
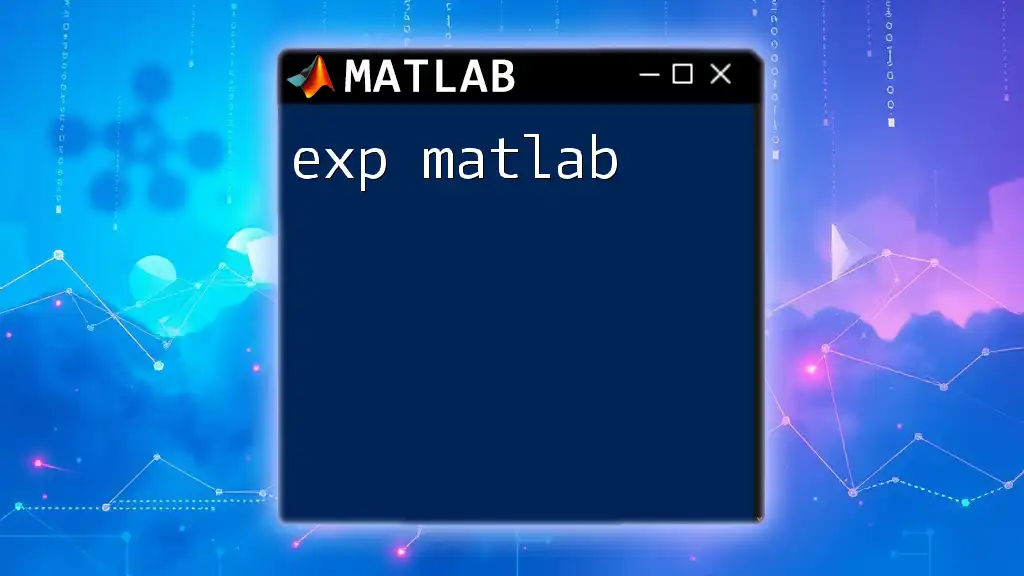
Performance Considerations
While `regexprep` is powerful, it's essential to be mindful of performance, especially when dealing with extensive datasets. Complex regex patterns can lead to slower execution times, so employing simpler patterns where possible can be beneficial. Additionally, pre-compiling frequently used patterns with the `regexp` function can improve performance.
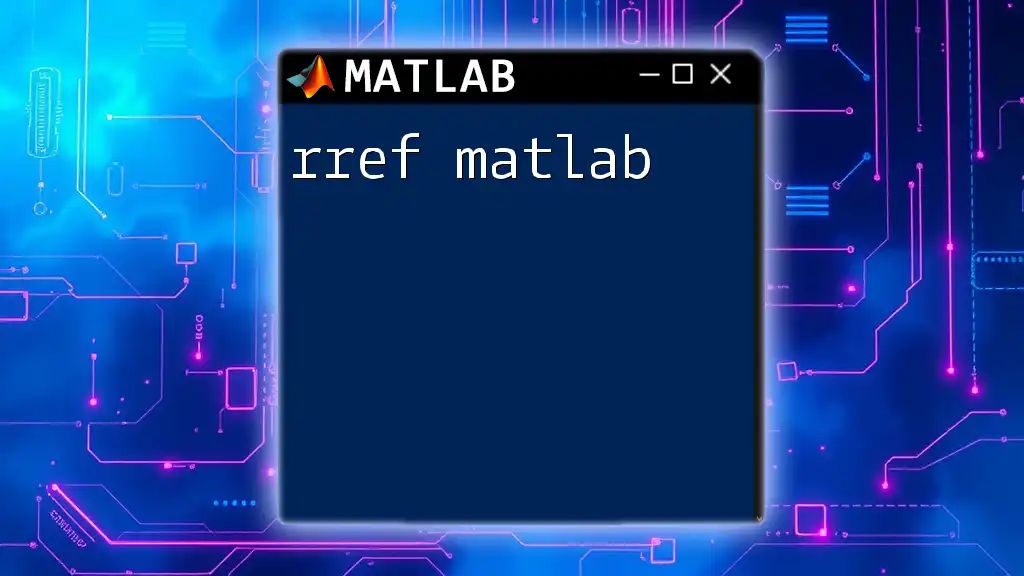
Troubleshooting Common Issues
Common Errors in Regular Expressions
Regular expressions in MATLAB can result in errors if not crafted correctly. For instance, forgetting to escape special characters leads to syntax errors. If you encounter issues, double-check your escape sequences.
Debugging Regex Patterns
A helpful strategy for debugging regex patterns in MATLAB involves using the `regexpi` or `regexp` functions for pattern inspection before applying `regexprep`. This way, you can identify whether your patterns are matching as expected.
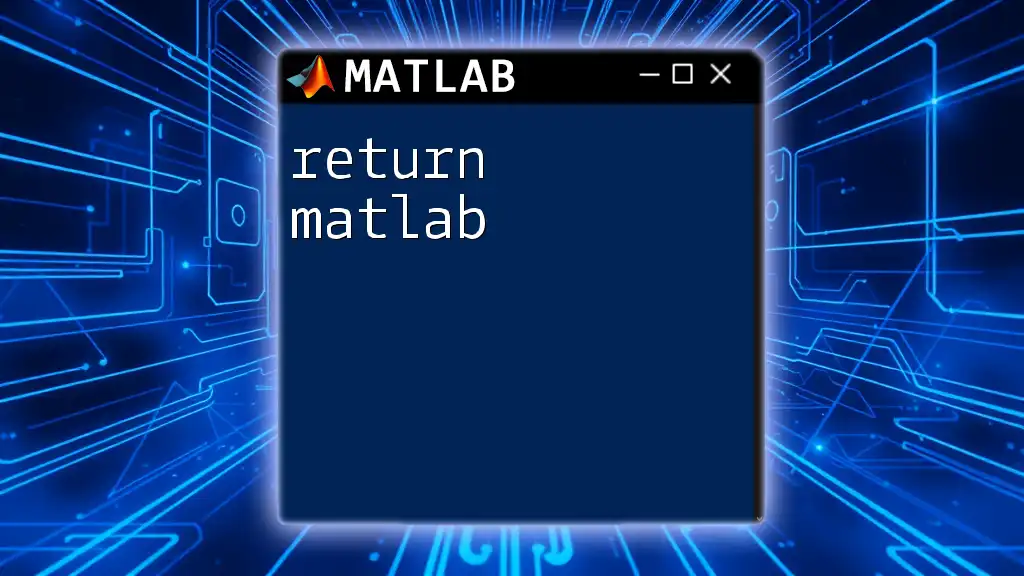
Conclusion
In summary, mastering `regexprep` in MATLAB is an invaluable skill for anyone interested in efficient string manipulation and data processing. From basic replacements to complex regex utilization, the function shines in its versatility and power. Regular practice with various examples will bolster your understanding and mastery of regex within MATLAB. By spending time experimenting with this tool, you'll quickly recognize its potential in streamlining your coding tasks and optimizing data workflows.
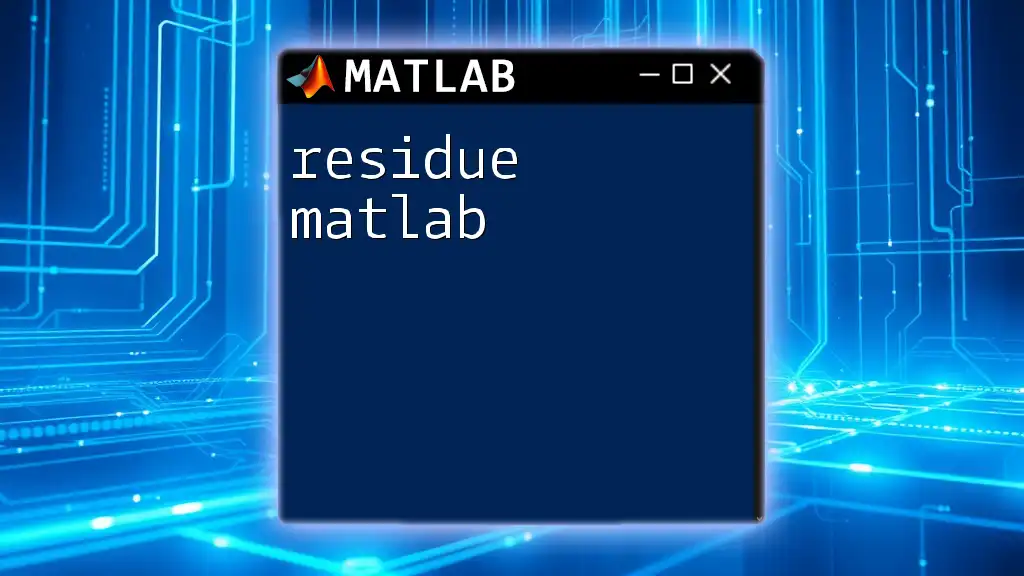
Additional Resources
For those seeking to broaden their knowledge of regular expressions and MATLAB's string manipulation capabilities, consider diving into the official MATLAB documentation. Online resources, forums, and community discussions can also provide valuable insights and support as you hone your skills with `regexprep` and regex concepts in general.