The `exp` function in MATLAB computes the exponential of each element in an array, effectively calculating \( e^{x} \) for every \( x \), which is particularly useful in science and engineering applications. Here's a code snippet demonstrating its use:
x = [0, 1, 2, 3]; % Define an array
y = exp(x); % Calculate the exponential of each element
disp(y); % Display the result
Understanding the Exponential Function
The exponential function is a fundamental mathematical concept, often denoted as \( e^x \), where \( e \) is Euler's number (approximately 2.71828). The exponential function has significant importance in various fields, including mathematics, physics, and engineering due to its unique properties, such as continuous growth and its relationship with logarithmic functions.
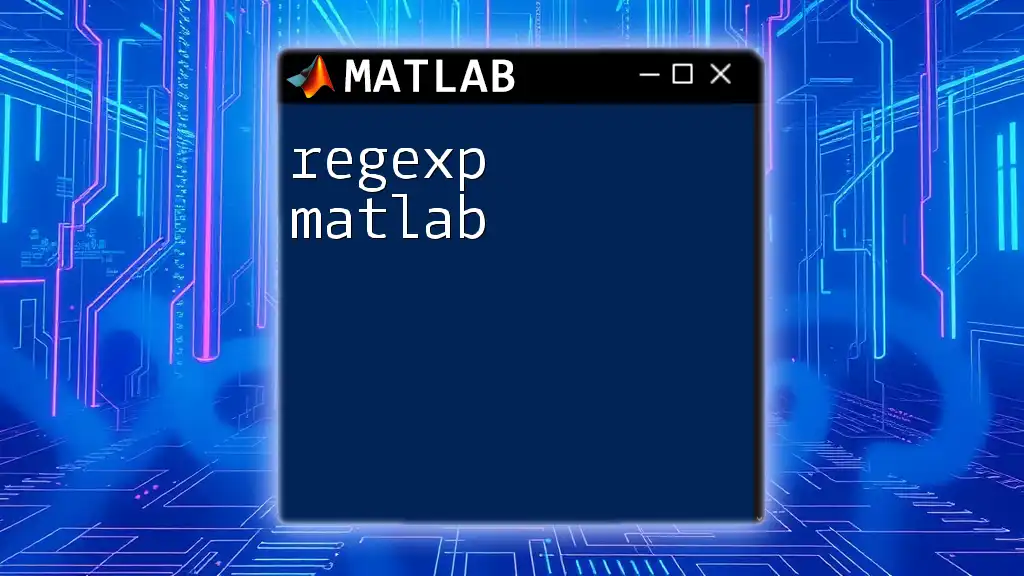
How to Use the `exp` Command in MATLAB
The `exp` function in MATLAB is defined by the following syntax:
Y = exp(X)
In this context, `X` can be any scalar, vector, or matrix, and `Y` will correspondingly be the output.
Examples of Basic Usage
Example 1: Using `exp` with a scalar value
To calculate the exponential of a single number, you can use the following command:
result = exp(1);
disp(result);
This command will return approximately 2.7183, which is \( e^1 \).
Example 2: Using `exp` with a vector
You can also compute the exponential for multiple values at once. Here’s how:
vector_result = exp([1, 2, 3]);
disp(vector_result);
The result will be an array of exponentials corresponding to each entry: [2.7183, 7.3891, 20.0855], which represent \( e^1 \), \( e^2 \), and \( e^3 \) respectively.
Example 3: Using `exp` with a matrix
The `exp` function can be applied to matrices, which is useful when working with multiple data points. Consider the code below:
matrix_result = exp([1 2; 3 4]);
disp(matrix_result);
This will output a 2x2 matrix where each element is computed as the exponential value of its corresponding value in the input matrix.
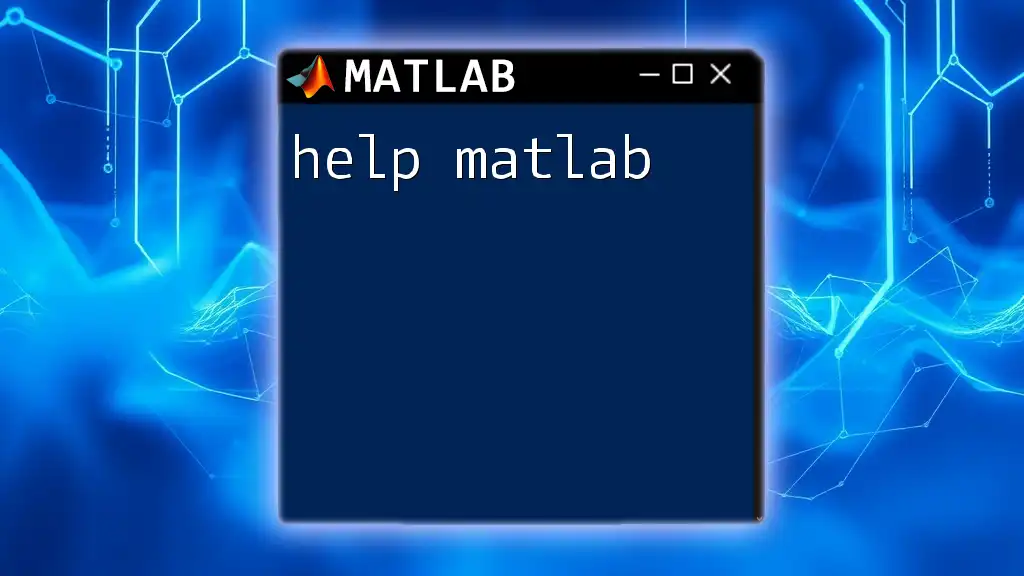
Handling Complex Inputs with `exp`
One of the notable features of the `exp` function in MATLAB is its ability to handle complex numbers. The relationship defined by Euler's formula states that:
\[ e^{i\theta} = \cos(\theta) + i\sin(\theta) \]
Examples with Complex Numbers
Example 4: Using `exp` with a complex number
To illustrate this concept, consider the following code:
complex_result = exp(1i * pi);
disp(complex_result);
The output will be -1, demonstrating that \( e^{i\pi} + 1 = 0 \), a cornerstone of complex analysis known as Euler’s identity.
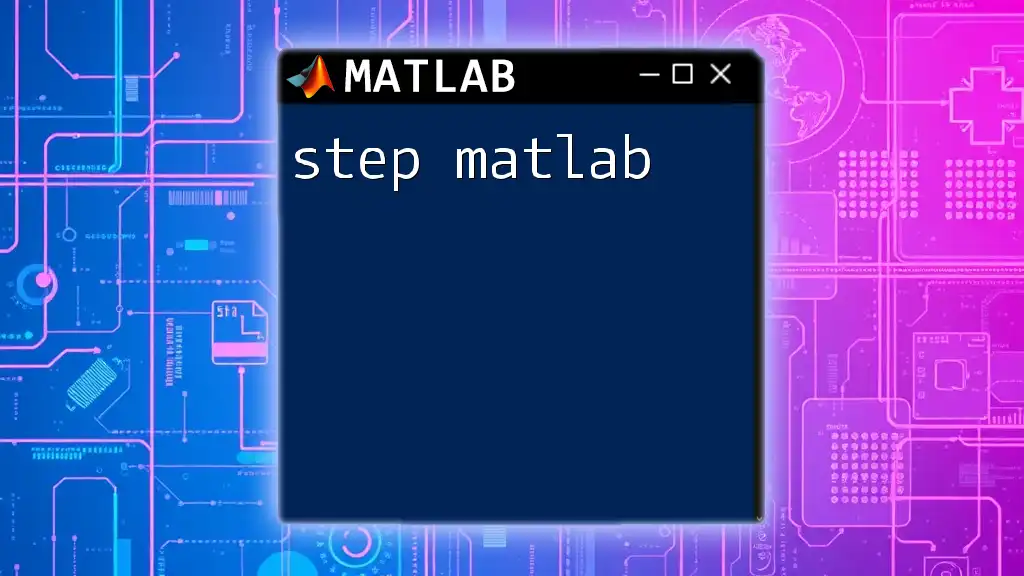
Applications of `exp` in Various Fields
In Mathematics
In mathematics, the `exp` function is crucial for solving various types of differential equations, including both ordinary and partial equations. The solutions often involve exponentials, indicating how quickly a quantity grows or decays over time.
In Engineering
Engineers frequently use the `exp` function in signal processing, particularly in analyzing systems described by exponential decay or growth. For example, in control systems, the response of a circuit can often be modeled using exponentials.
In Finance
In finance, the concept of continuous compounding can be calculated using the `exp` function. For instance, the future value \( FV \) of an investment compounded continuously is given by:
\[ FV = P e^{rt} \]
Here, \( P \) is the principal amount, \( r \) is the interest rate, and \( t \) is the time in years.
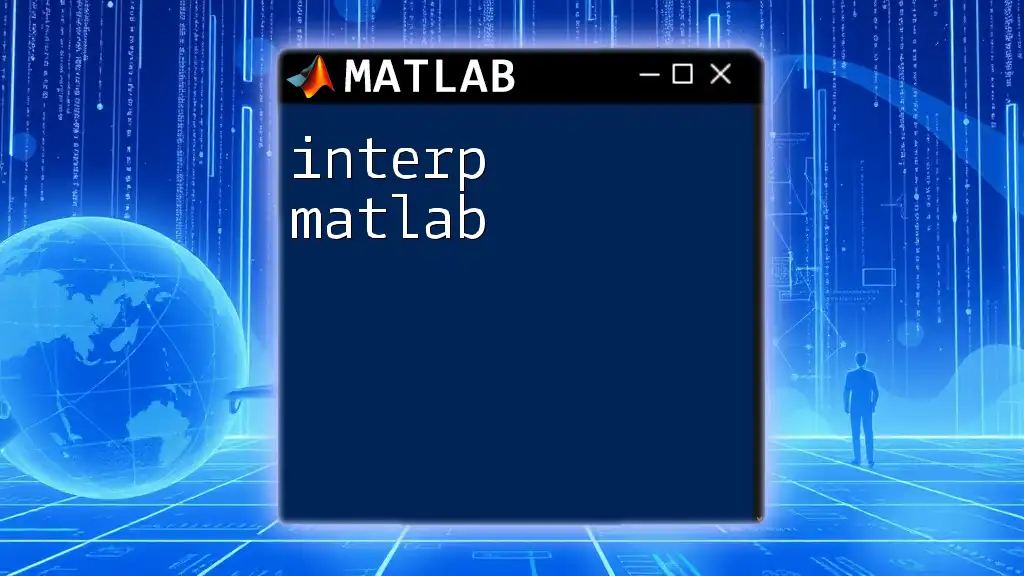
Using `exp` in Vectorized Operations
Vectorization in MATLAB enables efficient computations, particularly when working with large datasets. The capability to apply functions like `exp` across arrays without explicit loops boosts performance significantly.
Example of Vectorized Computations
Suppose you wish to visualize the exponential function over a range of values. You can utilize the following code:
x = 0:0.1:10;
y = exp(x);
plot(x, y);
title('Exponential Function e^x');
xlabel('x');
ylabel('e^x');
This code creates a plot of \( e^x \), illustrating how the function grows rapidly as \( x \) increases.
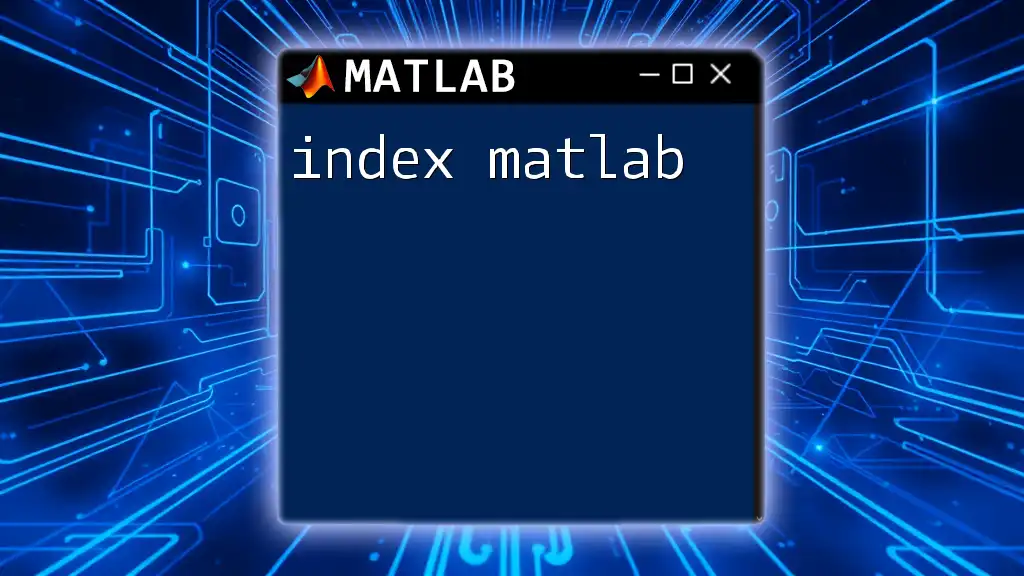
Common Mistakes and Troubleshooting
When using the `exp` function, beginners might encounter common errors related to matrix dimensions. Since MATLAB is dimension-sensitive, it's essential to ensure that the matrices align correctly during operations.
Tips for Debugging
Utilizing commands such as `size` and `length` can be incredibly helpful for identifying dimension-related issues. For example:
size(x)
length(y)
These commands will give insights into the structure of the data being worked with.
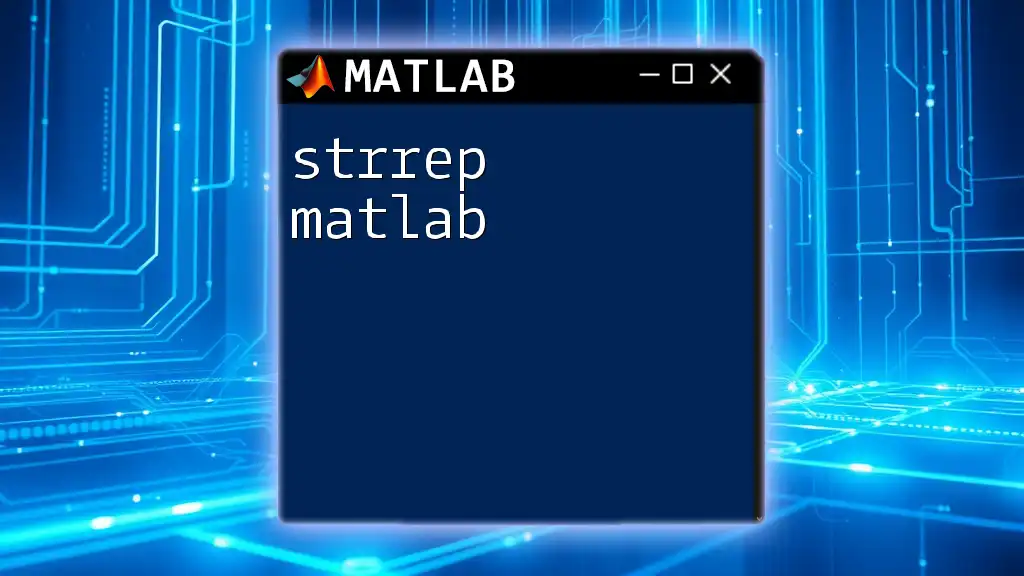
Conclusion
In summary, the `exp` function in MATLAB is not only versatile but also vital across various domains, enhancing the ability to handle both real and complex numerical computations. From mathematical problems to engineering applications and financial modeling, understanding the `exp` function opens up numerous possibilities in computational analysis.
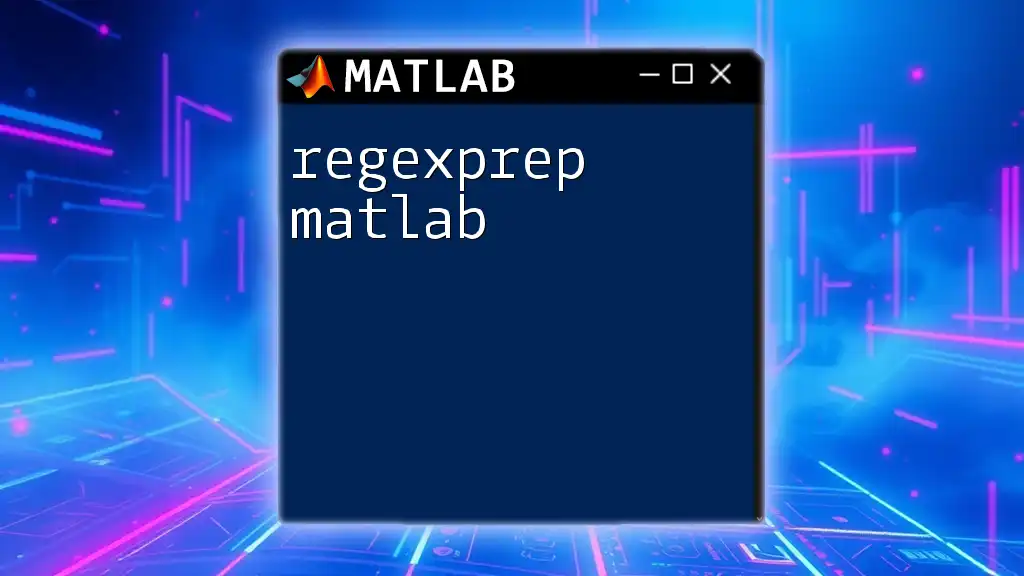
Additional Resources
For further learning about the `exp` function and other MATLAB commands, consider exploring relevant books, online courses, and the MATLAB official documentation. These resources will provide deeper insights and broaden your understanding of MATLAB programming.
Call to Action
Try implementing the examples provided in your MATLAB environment today! Feel free to subscribe for more concise MATLAB tips and command tutorials to enhance your coding skills.