`ode45` in MATLAB is a versatile function used to numerically solve ordinary differential equations (ODEs) using the adaptive Runge-Kutta method.
Here's a simple code snippet demonstrating how to use `ode45` to solve the ODE dy/dt = -2y:
% Define the ODE as a function
odeFunction = @(t, y) -2*y;
% Set the time span and initial condition
tspan = [0 5]; % Time from 0 to 5
y0 = 1; % Initial condition y(0) = 1
% Solve the ODE using ode45
[t, y] = ode45(odeFunction, tspan, y0);
% Plot the results
plot(t, y);
xlabel('Time');
ylabel('y');
title('Solution of dy/dt = -2y using ode45');
Understanding ODEs
What is an Ordinary Differential Equation?
An Ordinary Differential Equation (ODE) is a mathematical equation that relates a function with its derivatives. These equations play a crucial role in various fields such as physics, engineering, biology, and finance, often describing dynamic systems that change over time. ODEs can be classified into different types based on their order:
- First Order: Involves only the first derivative of the function.
- Second Order and Higher: Involves second derivatives or higher.
For example, a first-order ODE might look like this: \[ \frac{dy}{dt} = -k y \] where \( k \) is a constant.
Why Solve ODEs?
Solving ODEs is vital because they model real-world phenomena. For instance, they can describe the motion of physical objects, the dynamics of populations, or the flow of electricity in circuits. In many scenarios, the exact solutions cannot be obtained using traditional analytical methods, making numerical solutions essential.
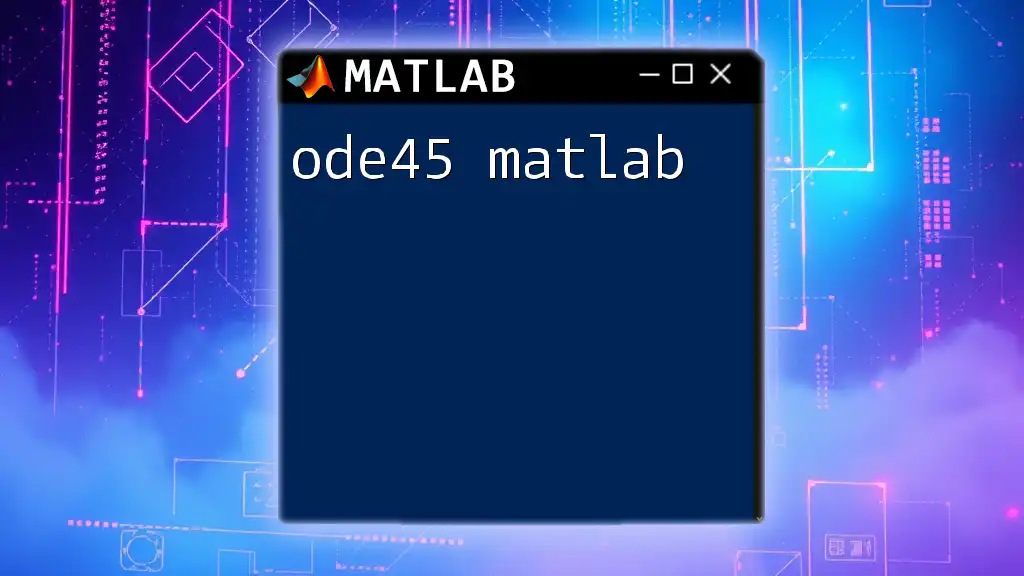
Getting Started with ode45 in MATLAB
What is ode45?
In MATLAB, `ode45` is one of the most widely used functions for solving ODEs. It is based on the Dormand-Prince method, which is an adaptive step size method. This means that `ode45` adjusts the step size during the computation to maintain accuracy while minimizing computation time.
Advantages of using `ode45`:
- Efficiency: Suitable for a wide range of problems.
- Automatic Step Size: Helps achieve better accuracy without manual adjustment.
Basic Syntax of ode45
The general form of calling `ode45` in MATLAB is as follows:
[t, y] = ode45(odefun, tspan, y0)
- odefun: A function that defines the ODE.
- tspan: A vector specifying the time interval for the solution.
- y0: The initial conditions of the ODE.
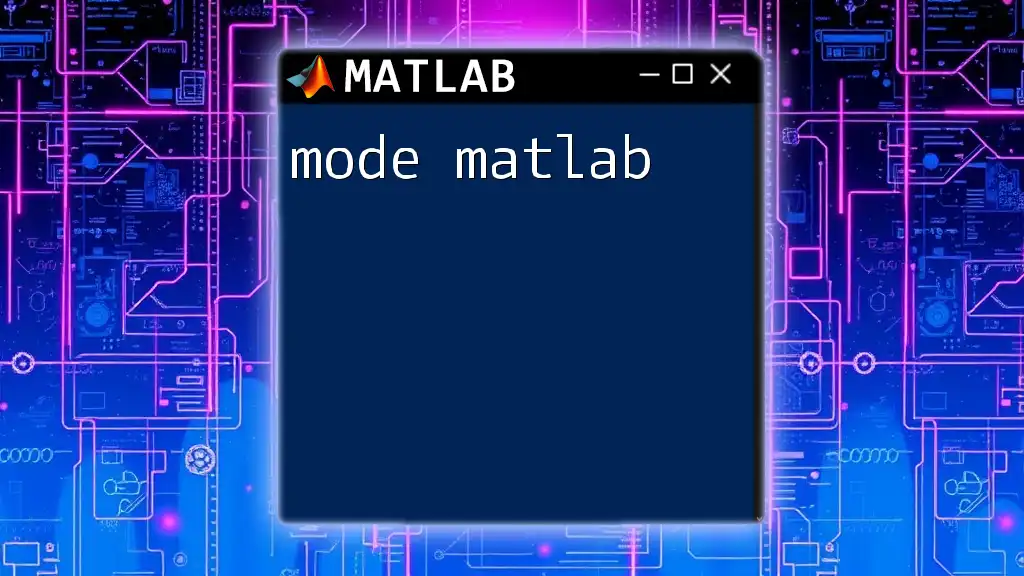
Creating Your First ODE with ode45
Example: Solving a Simple First-Order ODE
Let’s solve the first-order ODE given by: \[ \frac{dy}{dt} = -2y \] with the initial condition \( y(0) = 1 \).
Step-by-Step Code Explanation
First, you need to define the function that describes the ODE:
function dydt = odefun(t, y)
dydt = -2 * y;
end
Next, set the initial conditions and the time span:
% Initial conditions
y0 = 1; % Initial value of y
tspan = [0 5]; % Time interval
Now, use `ode45` to numerically solve the ODE:
% Using ode45 to solve the ODE
[t, y] = ode45(@odefun, tspan, y0);
Finally, plot the results to visualize the solution:
% Plotting the result
plot(t, y)
xlabel('Time t')
ylabel('Solution y')
title('Solution of the ODE dy/dt = -2y')
With this code, you will see an exponential decay of the function \( y \), demonstrating the solution of the ODE visually.
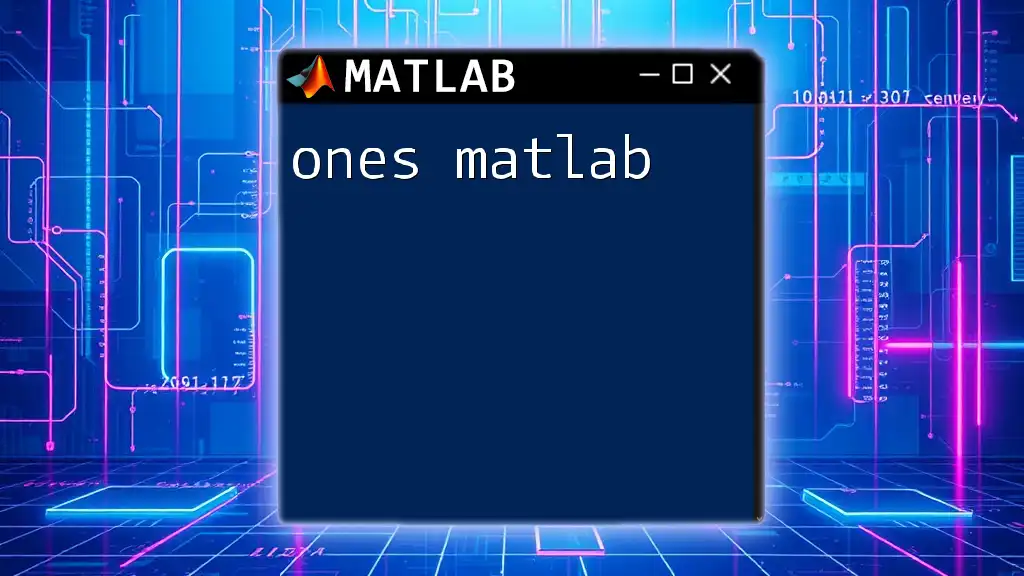
Advanced Concepts in ode45
Handling Systems of ODEs
Sometimes, you need to solve systems of ODEs. For example, consider the system:
\[ \frac{dx}{dt} = x - y \] \[ \frac{dy}{dt} = x + y \]
Code Explanation
Define a function that represents the system:
function dydt = mySystem(t, y)
dydt = [y(1) - y(2); y(1) + y(2)];
end
Set your initial conditions and time span:
% Initial conditions and time span
y0 = [1; 0]; % Initial values of x and y
tspan = [0 10]; % Time interval
Now, call `ode45` to solve the system:
% Solving the system of ODEs
[t, y] = ode45(@mySystem, tspan, y0);
Plot the results for clarity:
% Plotting the result
plot(t, y(1,:), t, y(2,:))
xlabel('Time t')
ylabel('Solutions x and y')
legend('x(t)', 'y(t)')
title('Solution of the system of ODEs')
Using Options with ode45
`ode45` also allows you to customize the solver's behavior using options set via the `odeset` function. You can specify options like relative tolerance and absolute tolerance.
For example, to set custom tolerances, you can do the following:
options = odeset('RelTol',1e-6, 'AbsTol',1e-9);
[t, y] = ode45(@odefun, tspan, y0, options);
This can help achieve higher accuracy for sensitive problems.
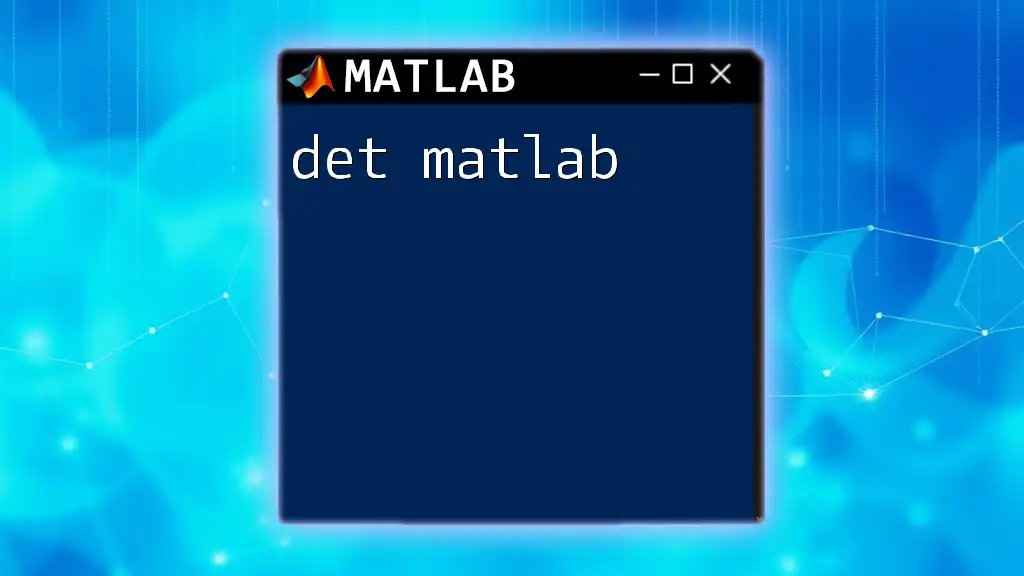
Common Errors and Troubleshooting
When using `ode45`, you might encounter common errors, such as:
- Function Definition Errors: Ensure that your function is defined correctly and returns the appropriate dimensions.
- Mismatched Dimensions: The number of initial conditions must match the number of equations in the system.
Tips for Debugging:
- Always check that your function inputs and outputs are correctly defined.
- Use MATLAB's built-in debugging tools to step through your function and understand where issues may arise.
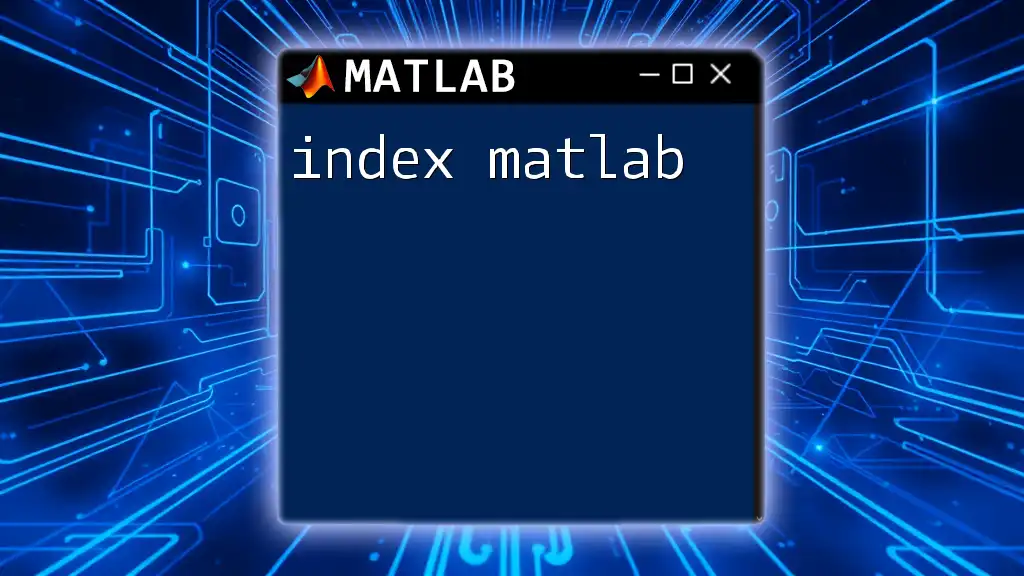
Best Practices for Using ode45
To make the most of `ode45`, consider the following best practices:
Tips for Efficient Computation
- Choose Appropriate Time Intervals: Select time spans that focus on the periods of interest for your solution.
- Understand When to Use `ode45`: Reserve `ode45` for problems that are not stiff. For stiff problems, consider other solvers like `ode15s`.
Plotting Techniques for Better Visualization
To enhance the clarity of your plots:
- Use `subplot` to display multiple plots for different variables.
- Customize labels and titles to make the plots self-explanatory.
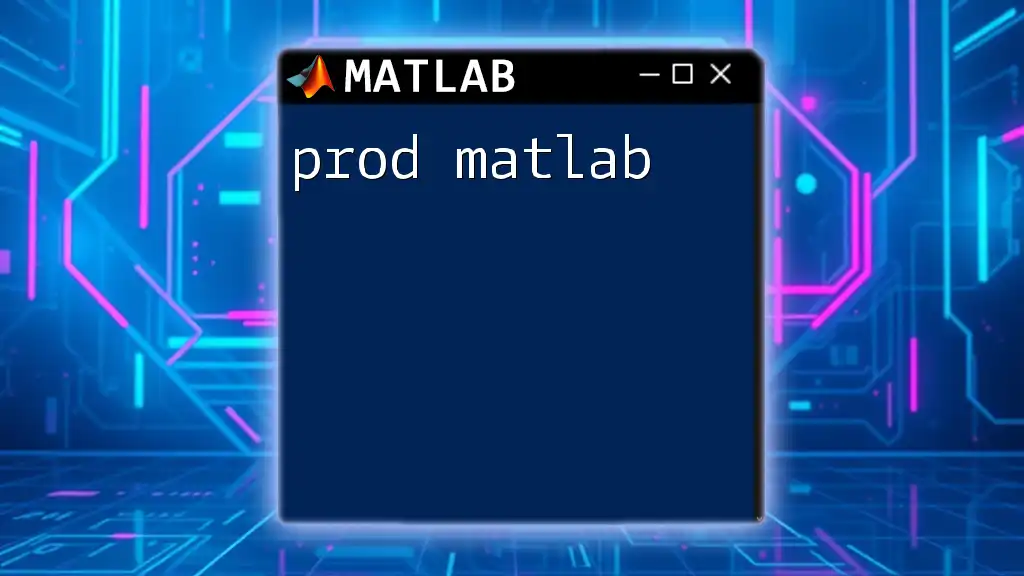
Real-World Applications of ode45
Case Study: Modeling Population Dynamics
A common application of ODEs is in modeling population dynamics, such as the logistic growth model. For the differential equation:
\[ \frac{dP}{dt} = rP(1 - \frac{P}{K}) \]
where \( r \) is the growth rate and \( K \) is the carrying capacity, we can solve it with `ode45`. This will give insights into how populations change over time based on growth rates and resource constraints.
Case Study: Electrical Circuits
Another fascinating application is in electrical circuits. For instance, the dynamics of an RLC circuit can be described by second-order ODEs. Implementing this in MATLAB using `ode45` allows engineers to simulate circuit behavior over time, making it easier to analyze the performance of electronic devices.
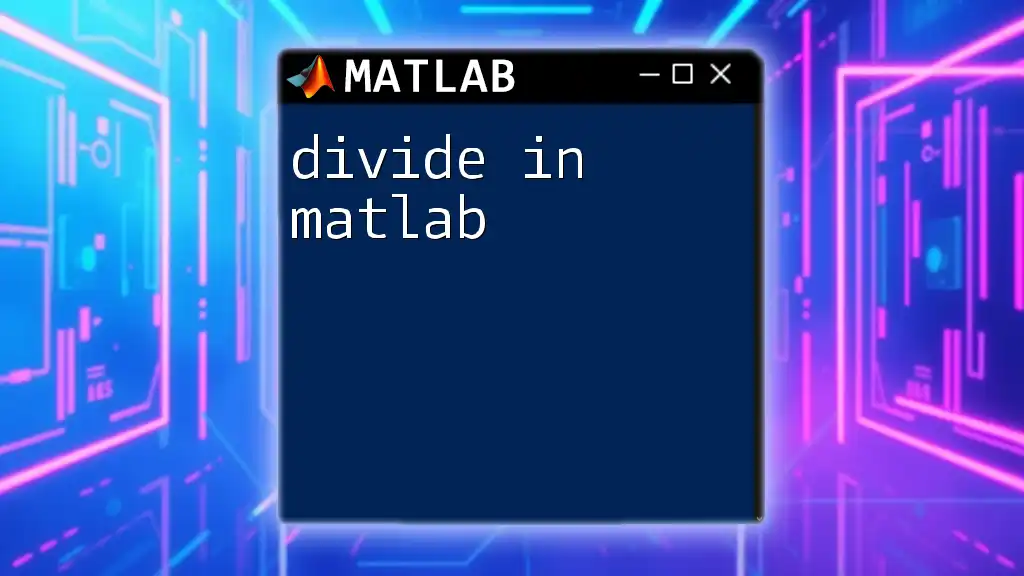
Conclusion
In summary, `ode45` in MATLAB is a powerful tool for solving ordinary differential equations, offering a suitable method for both simple and complex problems. Whether you're working on population models or analyzing electrical circuits, mastering `ode45` will significantly enhance your modeling capabilities. We encourage you to explore its features further and experiment with various types of ODEs to deepen your understanding. Embrace the world of numerical solutions, and let MATLAB be your guide in solving intricate dynamics.