`quadprog` is a MATLAB function used for solving quadratic programming problems, where the objective is to minimize a quadratic function subject to linear constraints.
Here's a code snippet demonstrating its use:
H = [2 -1; -1 2]; % Quadratic coefficients
f = [-2; -5]; % Linear coefficients
A = [1, 2]; % Linear inequality coefficients
b = [2]; % Linear inequality bounds
Aeq = []; % Linear equality coefficients
beq = []; % Linear equality bounds
lb = []; % Lower bounds
ub = []; % Upper bounds
x = quadprog(H, f, A, b, Aeq, beq, lb, ub); % Solve the quadratic programming problem
Understanding the Basics of Quadratic Programming
What is Quadratic Programming?
Quadratic programming is a specialized area of mathematical optimization that deals with problems where the objective function is quadratic and the constraints are linear. It builds upon the principles of linear programming but introduces a quadratic term in the objective function, which can represent various real-world problems more accurately.
Mathematical Formulation of Quadratic Programming
The standard form of a quadratic programming problem can be expressed as:
\[ \text{Minimize } \frac{1}{2} x^T H x + f^T x \] subject to: \[ Ax \leq b \\ A_{eq}x = b_{eq} \\ LB \leq x \leq UB \]
In this formulation:
- \(x\) is the vector of variables to be optimized.
- \(H\) is the Hessian matrix, which is symmetric and defines the curvature of the quadratic term.
- \(f\) is the linear coefficient vector.
- Constraints can include inequality constraints (\(Ax \leq b\)), equality constraints (\(A_{eq}x = b_{eq}\)), as well as lower and upper bounds (\(LB\) and \(UB\)) on the variables.
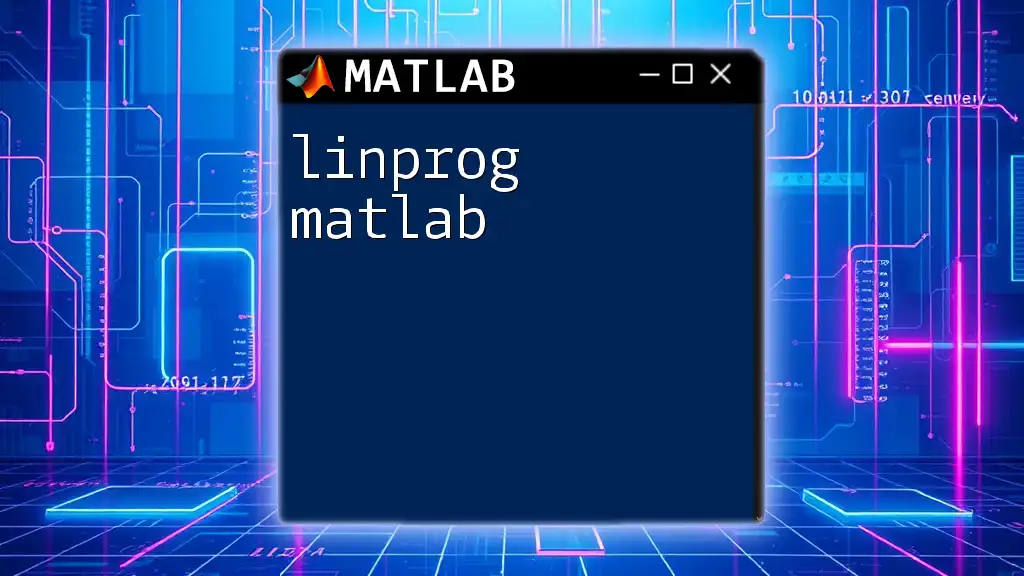
Introducing the `quadprog` Function in MATLAB
What is `quadprog`?
In MATLAB, the `quadprog` function is specifically designed for solving quadratic programming problems efficiently. This function uses advanced optimization techniques that can accommodate large datasets and complex constraints, making it a powerful tool for engineers, data scientists, and analysts.
Syntax and Parameters of `quadprog`
The basic syntax for calling the `quadprog` function in MATLAB is:
x = quadprog(H, f, A, b, Aeq, beq, LB, UB, x0, options)
Each of these parameters plays a crucial role in defining the optimization problem:
- `H`: The Hessian matrix that reflects the curvature of the quadratic objective function.
- `f`: The linear term in the objective function.
- `A`: The matrix representing the coefficients for the inequality constraints.
- `b`: The limit values for the inequality constraints.
- `Aeq`: The matrix for equality constraints.
- `beq`: The limit values for the equality constraints.
- `LB` and `UB`: Vectors specifying lower and upper bounds for the variables.
- `x0`: The initial guess for the variables.
- `options`: A structure specifying the optimization options (e.g., display setting or solver options).
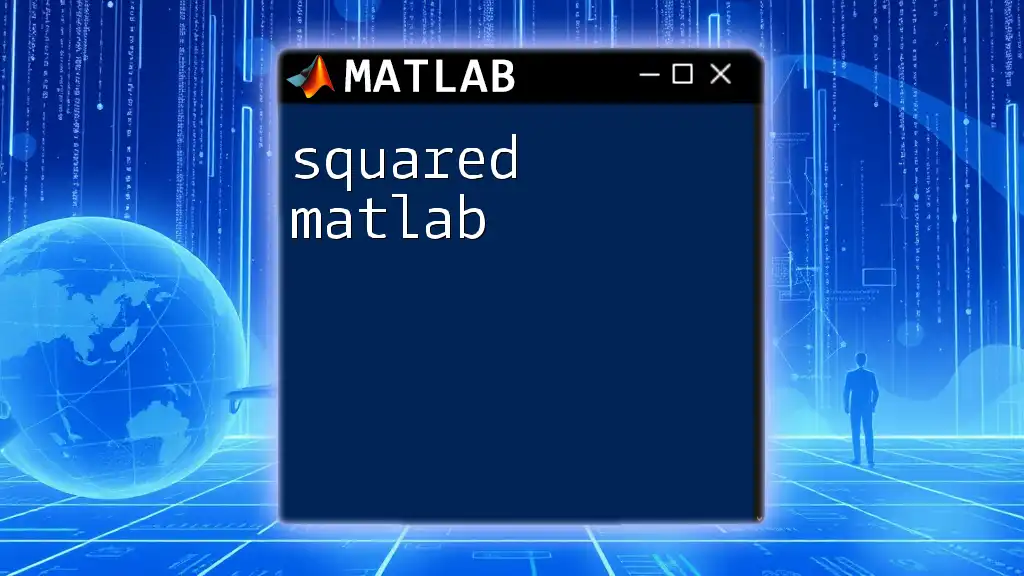
Setting Up a Quadratic Programming Problem
Formulating a Problem Example
To illustrate the use of the `quadprog` function, let’s consider a simple optimization problem. Suppose you are managing a portfolio of investments and want to minimize the risk measured by variance while achieving a target return.
- Define your objective function as minimizing risk while defining constraints to satisfy target returns.
- Based on historical data, you can form the quadratic and linear terms.
Defining Parameters for the `quadprog` Function
Let’s assume a hypothetical example where:
- The Hessian matrix \(H\) represents the covariance of different assets.
- The linear term \(f\) represents expected returns.
A sample set of parameters could look like this:
% Example data setup
H = [2 0; 0 2]; % Hessian matrix (covariance)
f = [-2; -5]; % Linear term (returns)
A = [1, 1; -1, -2; -2, -1]; % Inequality constraints matrix
b = [2; -2; -2]; % Inequality limits (e.g., max risk levels)
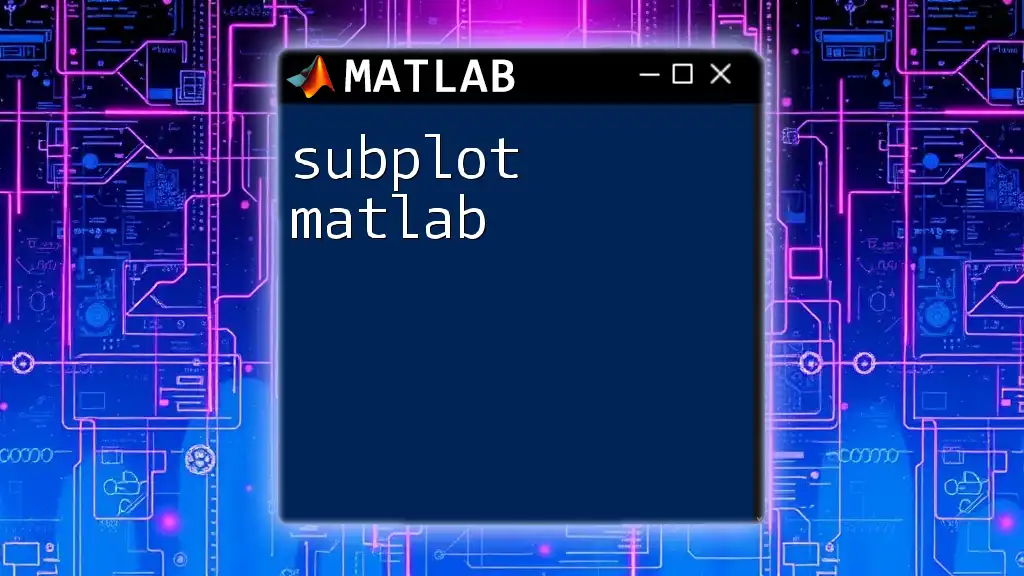
Solving the Quadratic Programming Problem
Running the `quadprog` Function
With the problem formulated and parameters defined, it’s time to implement `quadprog`. To solve the given quadratic programming problem, execute the following command.
% Solve the quadratic programming problem
[x, fval, exitflag, output] = quadprog(H, f, A, b);
disp('Optimal solution:');
disp(x);
Interpreting the Output
When running the `quadprog` function, you receive several output variables:
- `x`: The optimal solution vector that represents the values for your decision variables (e.g., asset weights).
- `fval`: The value of the objective function at the optimal point.
- `exitflag`: Indicates the result of the optimization process, such as successful convergence or failure.
- `output`: A structure containing details about the optimization process (e.g., number of iterations).
The exit flag is particularly important as it informs you whether the optimization was successful or if it encountered issues.
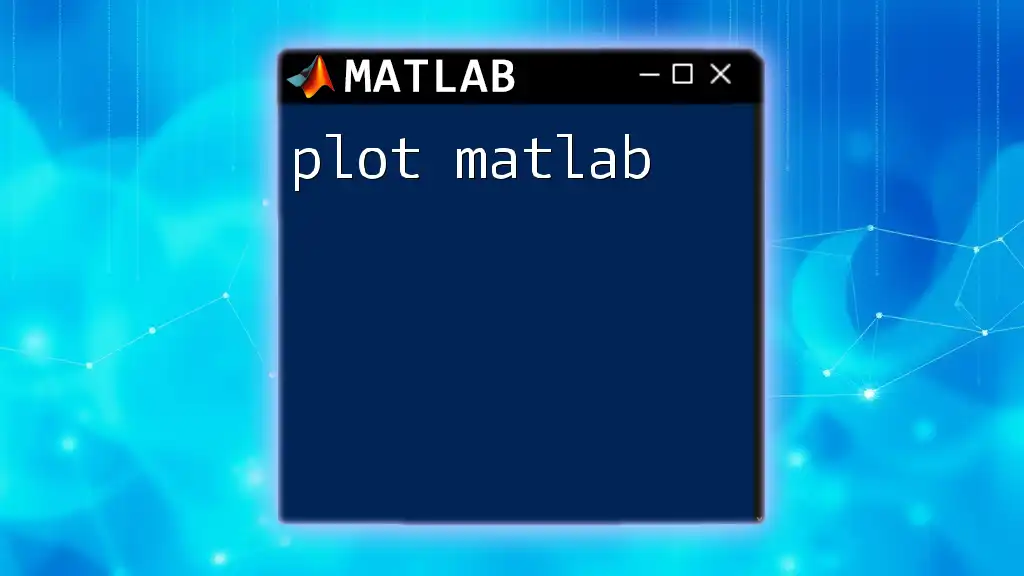
Advanced Topics in Quadratic Programming
Adding Constraints and Bounds
In many practical applications, you may need to include bounds on your variables to represent real-world limits (e.g., maximum investment in an asset). This can be done by defining vectors `LB` and `UB`.
Options Parameter in `quadprog`
The `options` parameter allows you to customize the behavior of the optimization routine to suit your needs better. For example, you might want to increase the iteration limit or change the display settings to monitor progress closely. Here is how you would set options:
options = optimoptions('quadprog', 'Display', 'iter');
Handling Non-Convex Problems
While `quadprog` is powerful, it has limitations—it performs well for convex problems where the Hessian matrix \(H\) is positive definite. In cases of non-convex problems, alternative methods or tools may need to be employed.
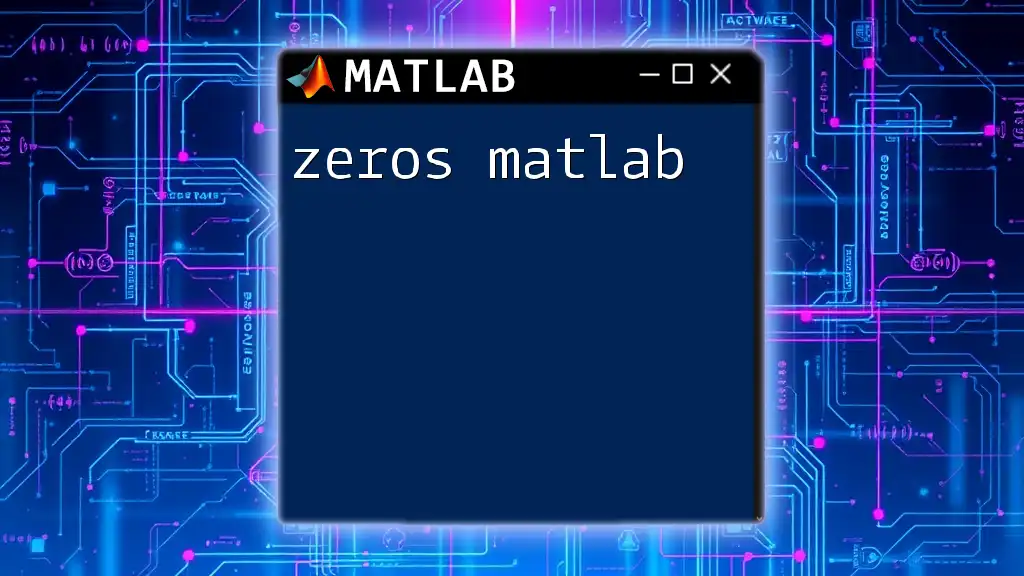
Error Handling and Troubleshooting
Common Errors and Solutions
Users may encounter various errors while using `quadprog`. Some common issues include:
- Misaligned matrix dimensions: Ensure that the dimensions of matrices \(A\), \(b\), and \(H\) match appropriately for the optimization problem.
- Non-positive definite Hessians: Check the entries of the Hessian matrix to ensure it is positive definite.
Best Practices for Successful Optimization
To enhance the likelihood of successful convergence, consider these best practices:
- Scaling your problem: Ensure that the input data is appropriately scaled.
- Providing a sensible initial guess: Use a reasonable starting point to expedite convergence.
- Gradually increasing complexity: Start with simplified versions of your problem to verify formulation and gradually add constraints.
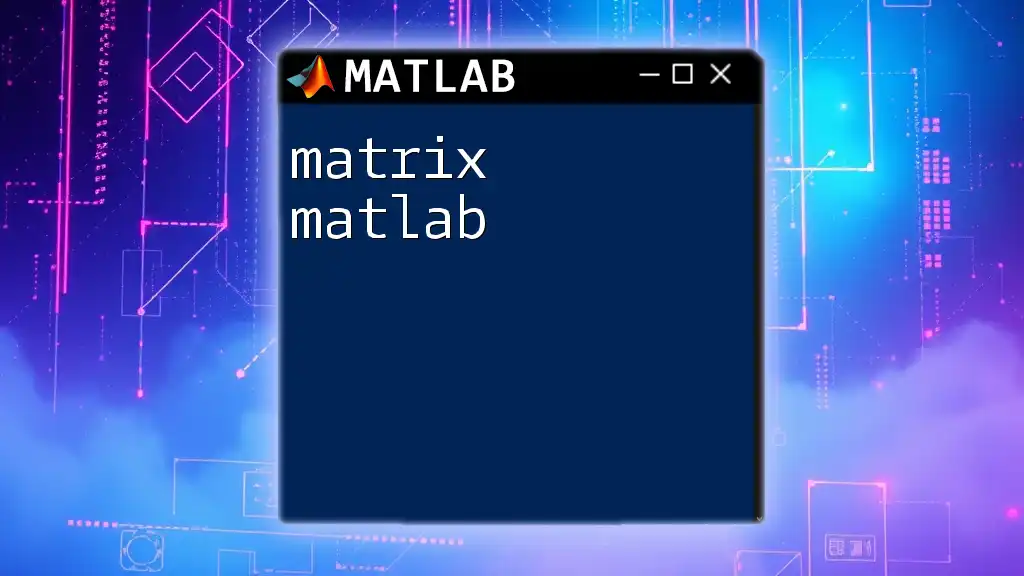
Conclusion
In conclusion, `quadprog` in MATLAB serves as an essential tool for tackling quadratic programming challenges across diverse fields, from finance to engineering and beyond. By understanding its syntax, capabilities, and how to structure optimization problems, users can leverage this function to achieve efficient and effective solutions to complex problems. I encourage you to explore the depths of quadratic programming and apply what you have learned to your own projects!
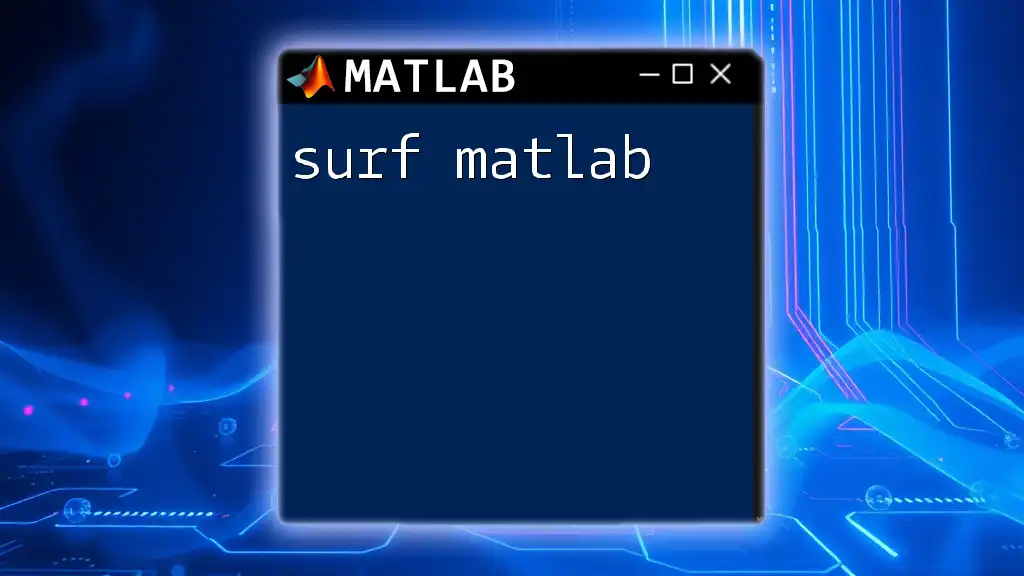
Additional Resources
To further your understanding and practical skills, consult the official MATLAB documentation for `quadprog` and consider exploring recommended books or online courses on mathematical optimization and problem-solving techniques.