In this article, we will explore how to convert Python syntax and functions into their MATLAB equivalents to facilitate a smoother transition for users familiar with Python.
% Python: result = [x**2 for x in range(10)]
result = (0:9).^2;
Understanding the Basics of Both Languages
Python
Python is a high-level programming language renowned for its readability and simplicity. It has gained immense popularity due to its versatility, making it a favorite for tasks ranging from web development to data analysis and artificial intelligence. Its robust ecosystem of libraries, such as NumPy for numerical computations and Pandas for data manipulation, allows for efficient coding practices.
MATLAB
MATLAB (short for MATrix LABoratory) is a specialized programming environment primarily used for mathematical computations and algorithm development. Favored in the academic and engineering fields, MATLAB excels in matrix operations, simulation, and data visualization, making it an invaluable tool for research and development.
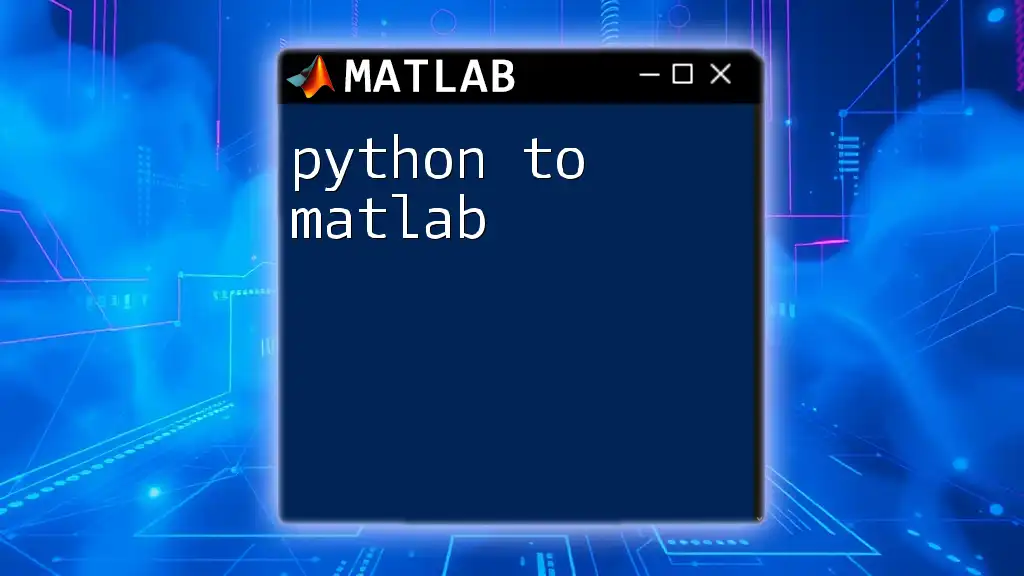
Key Differences Between Python and MATLAB
Syntax Differences
Understanding syntax differences is crucial when tackling python to matlab conversion.
- Variable Assignment: Python utilizes the equals sign for assignment, whereas MATLAB requires a semicolon to prevent output display.
- Python:
x = 10
- MATLAB:
x = 10;
- Python:
Data Types and Structures
Python and MATLAB differ significantly in their data structures:
- Lists and Arrays: Python's lists are flexible and can store mixed data types, while MATLAB uses homogeneous arrays.
- Dictionaries and Structs: Python dictionaries hold key-value pairs, while MATLAB utilizes structures for similar functionality.
An example comparison might look like this:
- Python code using lists and dictionaries:
my_list = [1, 2, 3] my_dict = {'key': 'value'}
- MATLAB code with arrays and structs:
my_array = [1, 2, 3]; my_struct.key = 'value';
Libraries and Functions
Python's extensive libraries enable different operations compared to MATLAB's built-in functions:
- For instance, Python's NumPy offers advanced numerical operations that can be replicated using MATLAB's array functionality. Understanding these equivalent functions can ease the python to matlab conversion process.
Example of Basic Array Operations:
- In Python, you would write:
import numpy as np a = np.array([1, 2, 3]) b = a * 2
- In MATLAB, the equivalent code is:
a = [1, 2, 3]; b = a * 2;
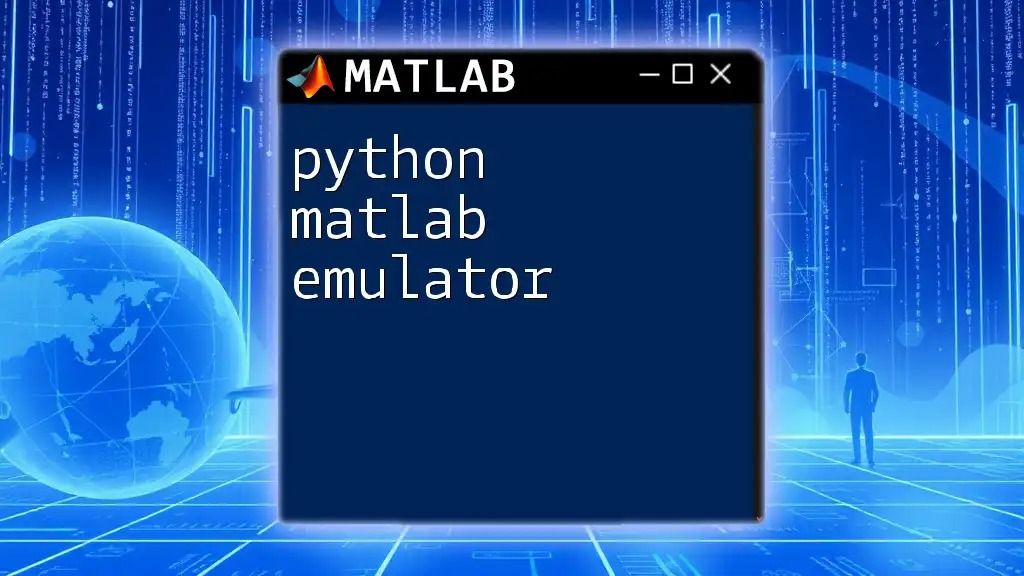
Step-by-Step Conversion Guide
Setting Up the Environment
Before diving into conversion, it’s pivotal to set up your coding environments for both Python and MATLAB. Common options for Python include Anaconda or Jupyter Notebooks, while MATLAB has its built-in IDE that provides extensive tools for code development. Having both established will provide a smoother transition.
Step-by-Step Process
Identifying the Code Structure
Understanding the structure of Python code is essential. When analyzing a Python script, look for functions, loops, and variable usage. This will help streamline the conversion process.
Translating Python Commands to MATLAB
Converting commands involves understanding the syntactical and functional similarities between the languages.
Control Structures: One key area is control structures like if statements, loops, and functions.
-
For example, a Python if statement would look like this:
if x > 10: print("x is greater than 10")
-
The equivalent code in MATLAB:
if x > 10 disp('x is greater than 10') end
Debugging Converted Code
After conversion, debugging is crucial. MATLAB's built-in debugging tools allow you to step through your code and identify any logical errors that arose during the conversion. Errors might include mismatched data types or incorrect function calls, especially if a library function was made in Python.
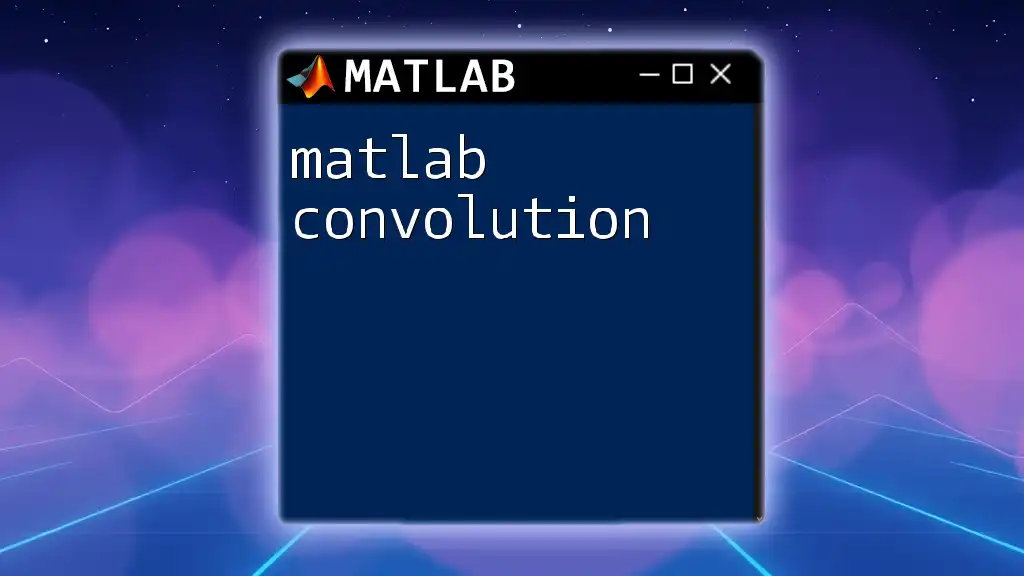
Practical Examples of Python to MATLAB Conversions
Example 1: Data Analysis with Pandas to MATLAB Tables
When converting data analysis routines, one common task is reading and manipulating datasets.
- In Python using Pandas:
import pandas as pd df = pd.read_csv('data.csv')
- The MATLAB equivalent reads a CSV file into a table like so:
df = readtable('data.csv');
These transformations highlight the shift from Python’s object-oriented approach to MATLAB's focus on matrices and tables.
Example 2: Machine Learning Models
As machine learning has grown in popularity, converting ML scripts from Python to MATLAB has become increasingly relevant.
Consider the basic structure of a machine learning model. A sample Python Scikit-Learn model might resemble:
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
In MATLAB, a comparable model fitting would use:
model = fitlm(X_train, y_train);
Understanding these translations can significantly ease data analysis and solution development across platforms.
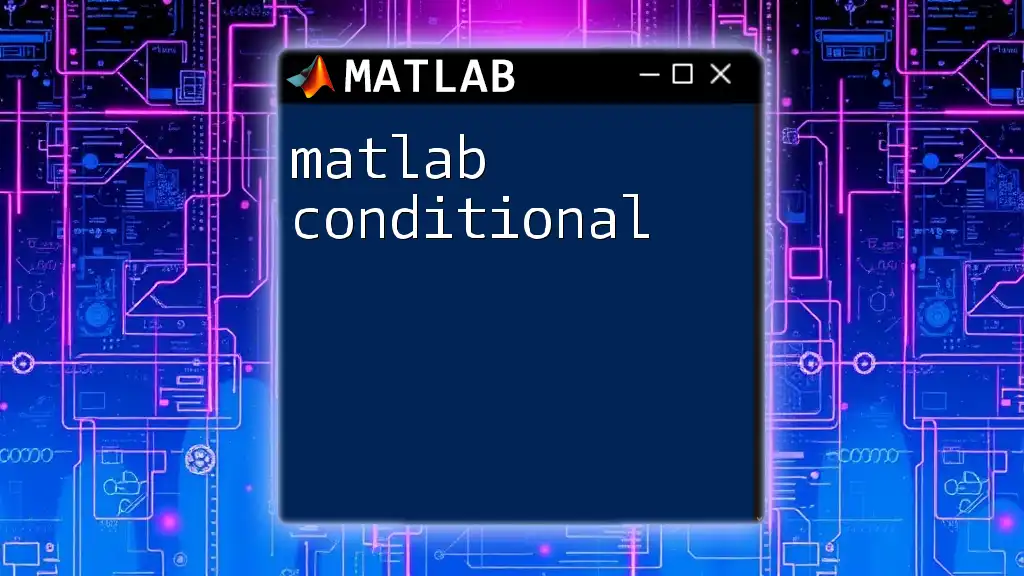
Best Practices for Conversion
To ensure a successful python to matlab conversion, there are several best practices to follow:
-
Maintain Readability: After conversion, focus on clear, readable code. Commenting is essential to help delineate the purpose of various sections and mirror established Python comments and documentation.
-
Optimization: Always consider profiling your MATLAB code for performance. MATLAB has built-in functions to analyze execution time and memory usage, helping you optimize as needed.
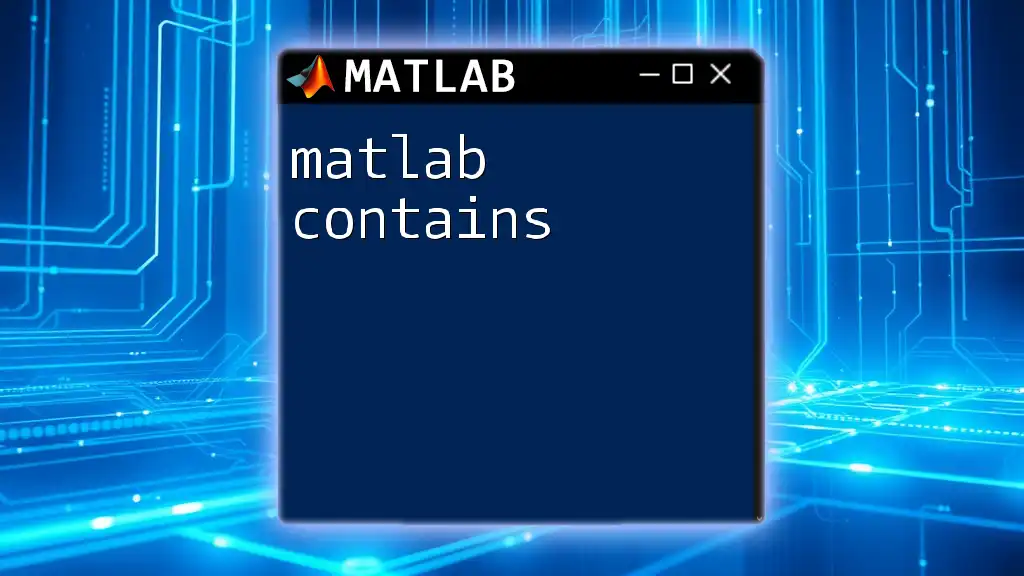
Conclusion
This comprehensive guide has outlined the essential components of python to matlab conversion. Understanding the fundamental differences, alongside practical examples and best practices, will enable you to streamline your coding efforts across both platforms. The key lies in recognizing the core functionality and translating syntax accurately, which will enhance your productivity and expand your programming skill set.
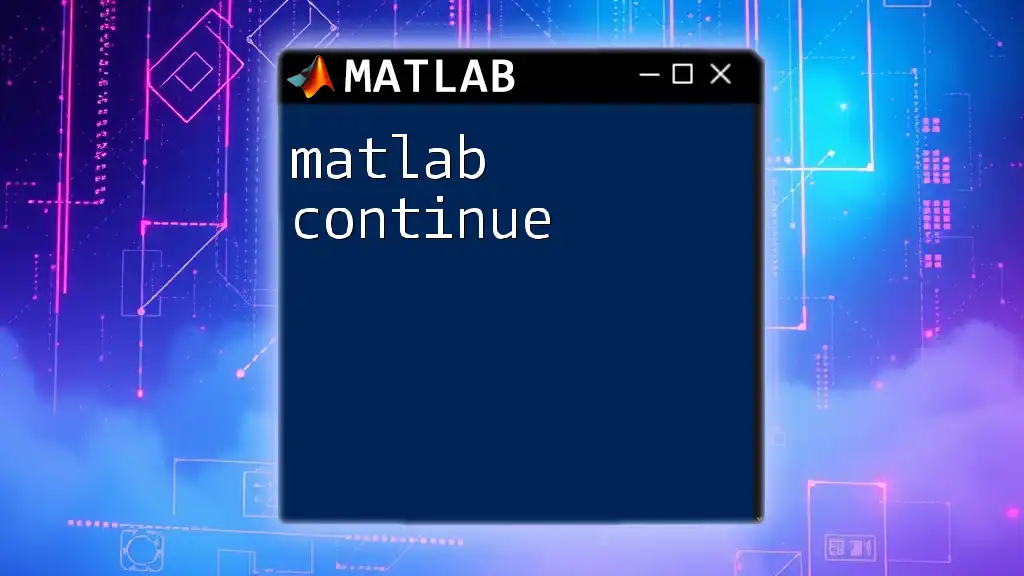
Additional Resources
For more information, consider exploring Python and MATLAB documentation. Engage with online communities through forums and websites dedicated to programming support. These additional resources can provide insights into more complex conversions and allow for ongoing learning in both languages. If you have any questions or need assistance, feel free to reach out to our team for expert guidance.