"Transitioning from Python to MATLAB can enhance your computational capabilities by leveraging MATLAB's specialized functions, such as converting a simple list to a matrix using the following command:"
A = [1, 2, 3; 4, 5, 6]; % Create a 2x3 matrix
Understanding the Basics
What is Python?
Python is a versatile, high-level programming language known for its simplicity and readability. It is widely used in various applications, including web development, data analysis, artificial intelligence, and scientific computing. Python's rich ecosystem of libraries, such as NumPy, Pandas, and Matplotlib, empowers developers to perform complex data manipulations and analyses effortlessly.
What is MATLAB?
MATLAB (Matrix Laboratory) is a proprietary programming language and environment designed primarily for numerical computing and data visualization. Engineers and scientists extensively use MATLAB for its robust mathematical functions, making it ideal for simulations, algorithm development, and data analysis. With built-in functionalities for matrix operations and advanced plot customization, it efficiently handles various mathematical computations.

Key Differences Between Python and MATLAB
Syntax and Structure
Variable Assignment
In Python, variable assignment is straightforward:
x = 10
In contrast, MATLAB requires a semicolon to end the statement:
x = 10;
Data Types
While Python uses lists to create ordered collections of items, MATLAB relies on arrays. For example, a simple list of integers in Python:
my_list = [1, 2, 3, 4]
would correspond to a MATLAB array:
my_array = [1, 2, 3, 4];
Understanding these fundamental data types is crucial to transitioning effectively from Python to MATLAB.
Function Definition
Functions serve as the cornerstone for both Python and MATLAB coding. In Python, a simple function definition looks like this:
def add(a, b):
return a + b
In MATLAB, however, the syntax requires a `function` keyword, with the function name defined at the beginning:
function result = add(a, b)
result = a + b;
end
Control Structures
Control structures like conditionals and loops differ somewhat in structure between the two languages. An example of a conditional statement in Python is as follows:
if x > 0:
print("Positive")
Whereas in MATLAB, it appears as:
if x > 0
disp('Positive');
end
Being familiar with these differences helps in adapting logic from Python to MATLAB.
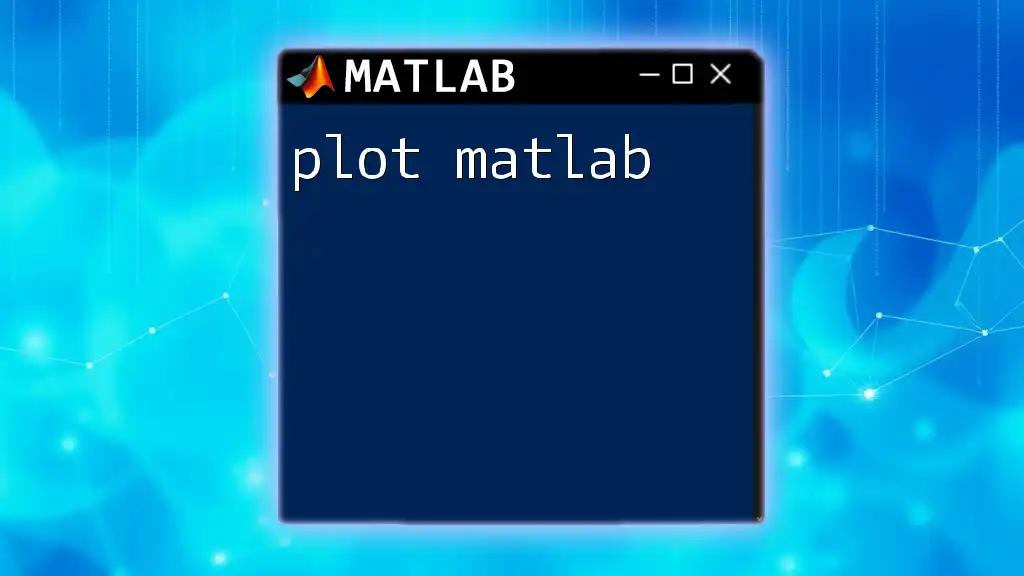
Transitioning from Python to MATLAB
Adapting to MATLAB's Environment
When transitioning from Python to MATLAB, understanding the MATLAB Integrated Development Environment (IDE) is critical. MATLAB’s IDE integrates a workspace for variable management, command window for script execution, and editor for creating functions and scripts. Familiarizing oneself with these features allows for effective coding and debugging.
Basic MATLAB Commands for Python Users
Input/Output Operations
Reading from and writing to files is a common requirement in both languages:
-
Python uses the `open()` function to interact with files:
with open('data.txt', 'r') as file: data = file.read()
-
MATLAB, on the other hand, provides the `load` function to import data:
data = load('data.txt');
Plotting Functions
Graphical representations of data are vital in both languages. The following illustrates how easy it is to create basic plots:
In Python, using Matplotlib:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 1])
plt.show()
In MATLAB, the process is similarly straightforward:
plot([1, 2, 3], [4, 5, 1]);
Both languages provide extensive plotting capabilities, but MATLAB offers built-in functions optimized for engineering applications.
Libraries and Toolboxes
The transition also involves understanding the equivalent libraries or toolboxes. Python developers often turn to:
- NumPy for numerical operations
- SciPy for scientific computing
- Matplotlib for visualization
On the other hand, MATLAB comes equipped with powerful toolboxes like the Statistics and Machine Learning Toolbox, Signal Processing Toolbox, and many others, providing specialized functions suited for various tasks.
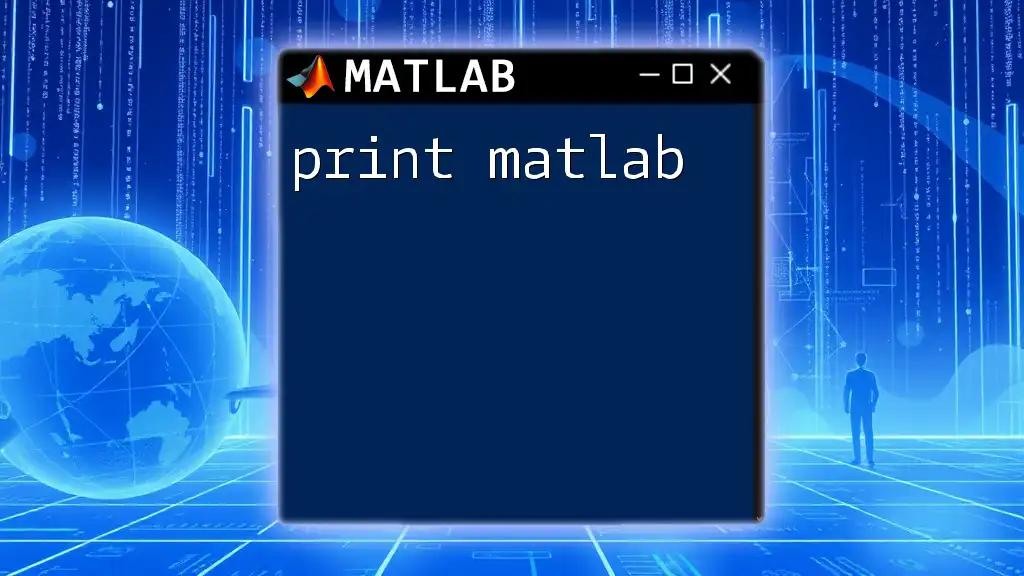
Common MATLAB Functions for Python Users
Array and Matrix Operations
Understanding how to manipulate arrays is crucial. In Python, you would create an array with NumPy:
import numpy as np
arr = np.array([1, 2, 3])
In MATLAB:
arr = [1, 2, 3];
Matrices are handled natively in MATLAB, providing a seamless experience for mathematical operations.
Statistical Functions
For data analysis, transitioning from Python’s pandas library to MATLAB's built-in statistical functions is essential. In Python, calculating the mean of a column can be done as follows:
import pandas as pd
df = pd.DataFrame(data)
mean_value = df['col_1'].mean()
Conversely, in MATLAB:
mean_value = mean(data.col_1);
This clarity in syntax helps maintain efficiency in data manipulation across both platforms.
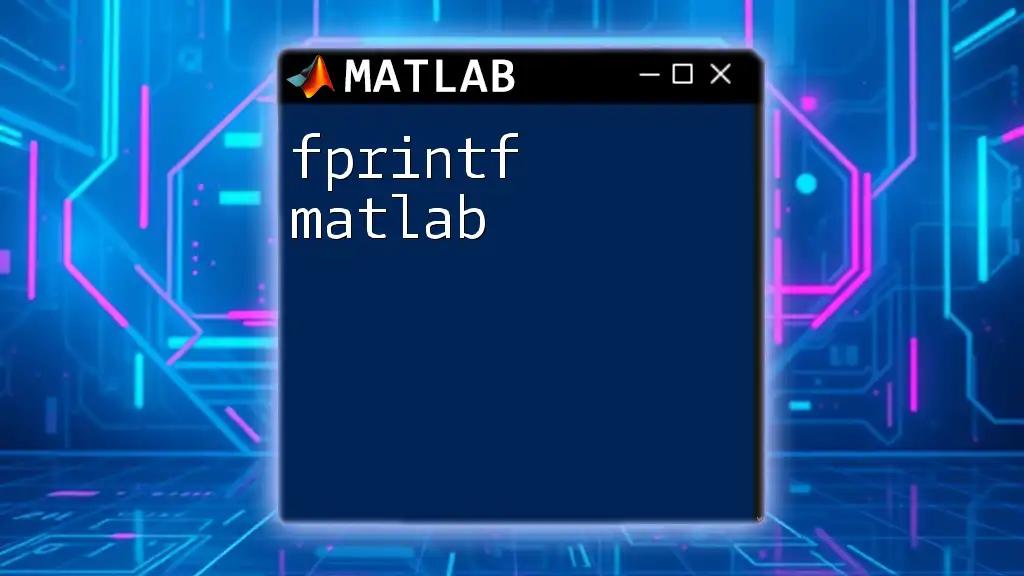
Best Practices for Learning MATLAB
Tips for Python Developers
For Python developers transitioning to MATLAB, it’s vital to embrace MATLAB’s strengths, such as vectorization and native handling of matrices. Getting accustomed to MATLAB’s indexes starting at 1 (instead of 0 in Python) will also improve your coding accuracy. To facilitate learning, invest time in MATLAB’s extensive documentation and leverage the community’s wealth of knowledge.
Practice Examples
Applying learned concepts in practical scenarios enhances retention. Consider engaging in mini-projects or coding challenges, such as recreating familiar algorithms or data visualizations in MATLAB. This proactive approach fosters a deeper understanding of MATLAB's unique features and conventions.
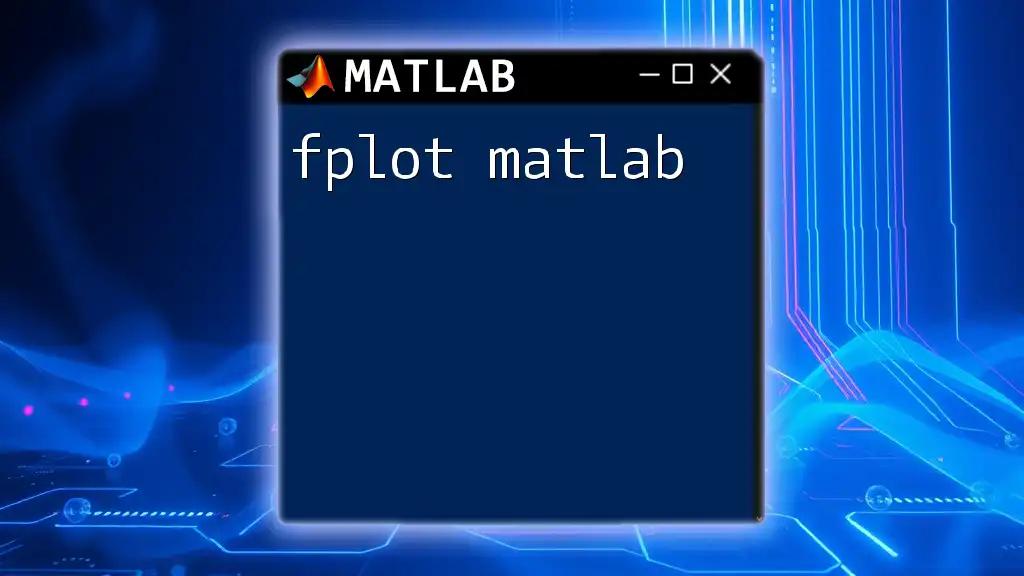
Conclusion
Transitioning from Python to MATLAB may seem daunting at first, but understanding the core differences and similarities between the two languages can significantly ease the process. Armed with this knowledge, you will be better prepared to explore the vast capabilities of MATLAB.
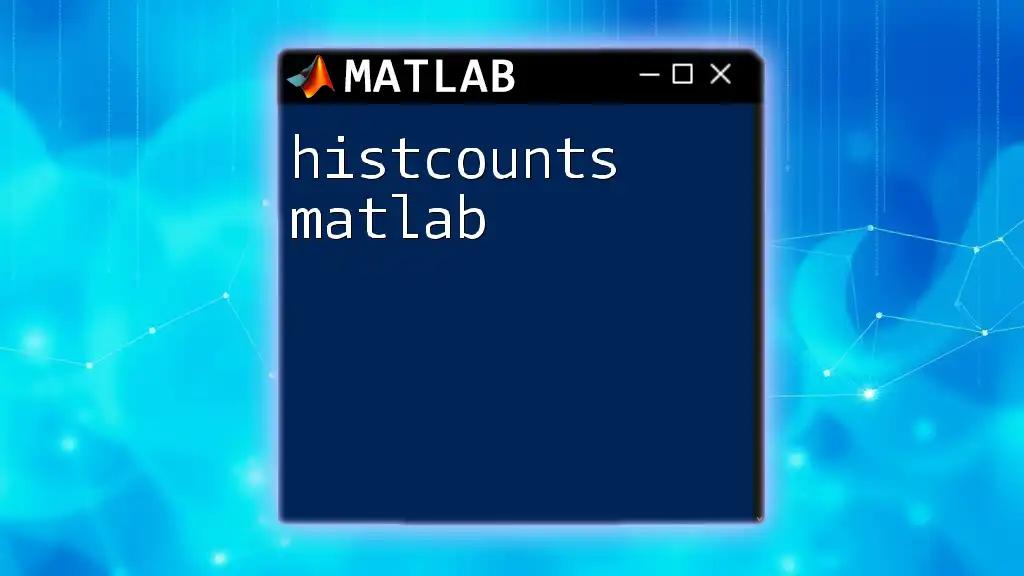
Additional Resources
For those looking to further enhance their skills, access online documentation for both languages. Consider books and video tutorials designed specifically for transitioning developers. Engaging with communities dedicated to MATLAB and Python can also provide valuable insights and support as you explore this new programming landscape.