To read binary data in MATLAB, you can use the `fread` function, which allows you to specify the file identifier, data type, and the number of elements to read.
Here's a code snippet demonstrating this:
% Open a binary file for reading
fileID = fopen('data.bin', 'r');
% Read 10 double-precision floating-point numbers from the file
data = fread(fileID, 10, 'double');
% Close the file
fclose(fileID);
Understanding Binary Format
What is Binary File Format?
Binary files are composed of data in a format that is not human-readable. These files store information in binary digits (0s and 1s), which can represent various types of data such as numbers, images, and audio. Unlike text files, which store data as plain text (ASCII characters), binary files can hold a broader range of information in a more compact format.
For example, a simple image file saved in binary might contain pixel values coded directly as bytes, while a text file would encode these pixels in characters, making it significantly larger.
Common Types of Binary Files
Common binary file types include:
- .bin: A generic binary file format that can be used for various data types.
- .dat: Often used for data storage, varying widely in structure.
- .img: Frequently used for image data, such as raw image files from cameras.
These formats are utilized across various fields, such as medical imaging, digital media, and scientific research, where efficiency in data storage and processing is crucial.
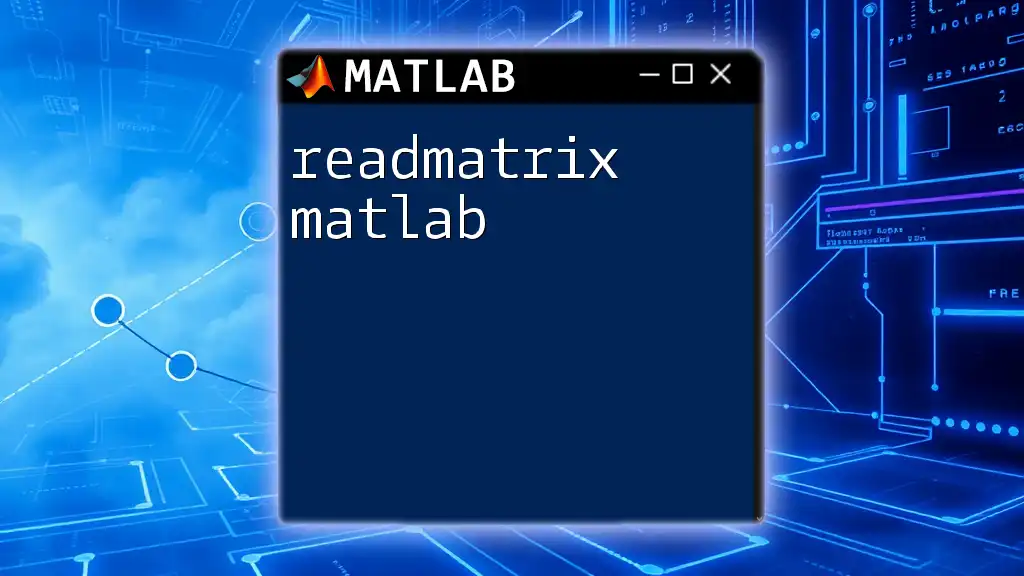
Reading Binary Files in MATLAB
Using MATLAB’s Built-In Functions
MATLAB provides powerful functions to read binary files, mainly through `fopen`, `fread`, and `fclose`. Understanding these functions allows you to effectively read in binary format in MATLAB.
Opening a Binary File
To read a binary file, you first need to open it using `fopen()`. This function returns a file identifier (file ID), which is used for further operations.
fileID = fopen('data.bin', 'rb'); % Open in binary read mode
The `'rb'` argument specifies that the file is opened for reading in binary mode.
Reading Data from Binary Files
The primary function for reading data from binary files is `fread()`. This function allows you to specify the format of the data you're reading as well as the number of elements.
data = fread(fileID, 'double'); % Read data as double precision floats
In this example, `fread()` attempts to read all available data in the file and interprets it as double-precision floating-point numbers.
Closing the File
Once you’ve completed reading from the file, it’s important to close it using `fclose()`. This step ensures that all resources are freed and any potential data loss is avoided.
fclose(fileID); % Closes the file after reading
Example: Reading Different Data Types
MATLAB can handle various data types when reading binary files. Here’s a snippet to demonstrate how to read mixed data types:
fileID = fopen('mixeddata.bin', 'rb');
intData = fread(fileID, 5, 'int32'); % Read 5 integers
doubleData = fread(fileID, 10, 'double'); % Read 10 doubles
charData = fread(fileID, 10, 'char'); % Read 10 characters
fclose(fileID);
In this example, different types of data are read into separate variables specifying the data type in `fread()`.
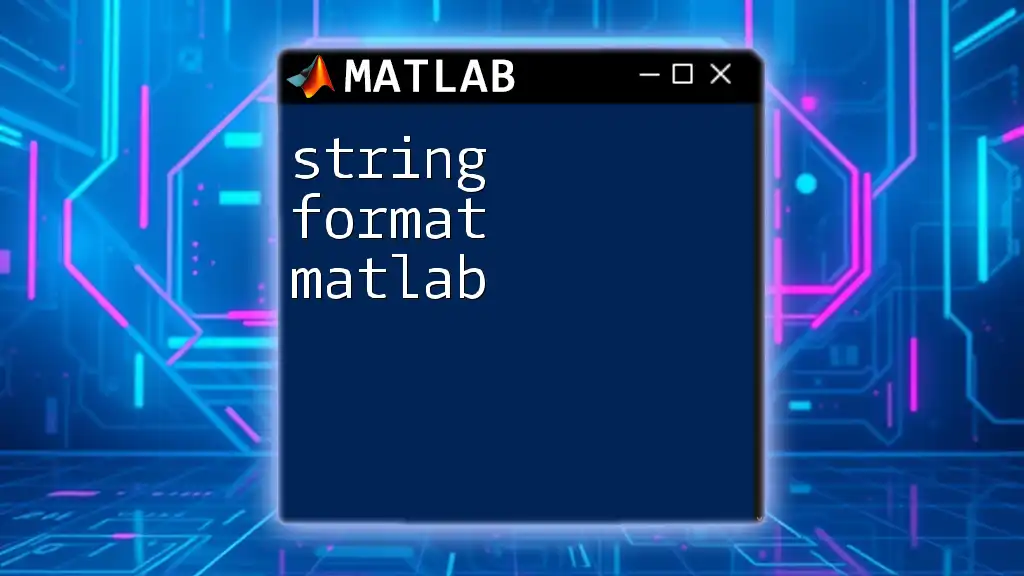
Working with Structure in Binary Files
How to Read Struct Data
Structures allow you to store complex data types in MATLAB. Reading structured data from binary files is straightforward when you understand the format of your binary data.
Defining a Structure
Before reading, you should define the structure format in which your data is stored. A typical structure might look as follows:
student.name = 'John';
student.age = 21;
student.grade = 'A';
This structure includes a name, age, and grade, illustrating how related data can be formatted together.
Reading Structures from Binary
To read a structured binary file, you can utilize `fread()` similarly, but ensure the structure is correctly defined to match the order of the binary data.
fileID = fopen('students.dat', 'rb');
students = fread(fileID, 5, 'struct'); % Read 5 records into a structure
fclose(fileID);
Ensure your binary data is formatted as expected for structures; otherwise, data misalignment can occur.
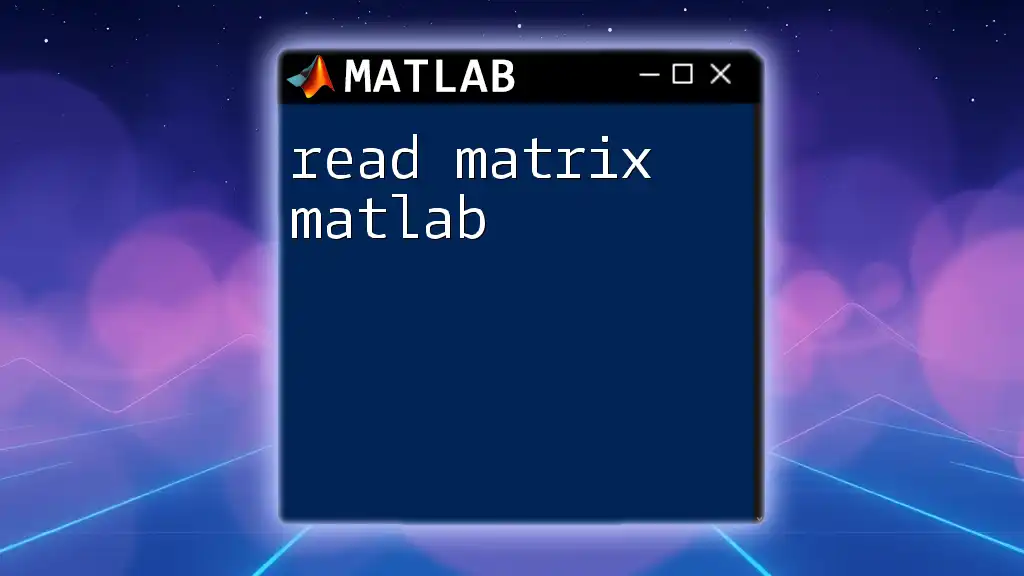
Error Handling in File Operations
Common Errors and Solutions
When reading binary files, common errors include:
- Permission Denied: Ensure your MATLAB session has the necessary permissions to read the file.
- File Not Found: Double-check the file path and name.
Best Practices for File I/O in MATLAB
Using `try-catch` blocks is a best practice when handling file operations to manage potential errors gracefully.
try
fileID = fopen('data.bin', 'rb');
if fileID == -1
error('File could not be opened.');
end
data = fread(fileID, 'double');
catch ME
fprintf('Error reading file: %s\n', ME.message);
end
fclose(fileID);
This example attempts to open the file and read it, providing error feedback if something goes wrong.
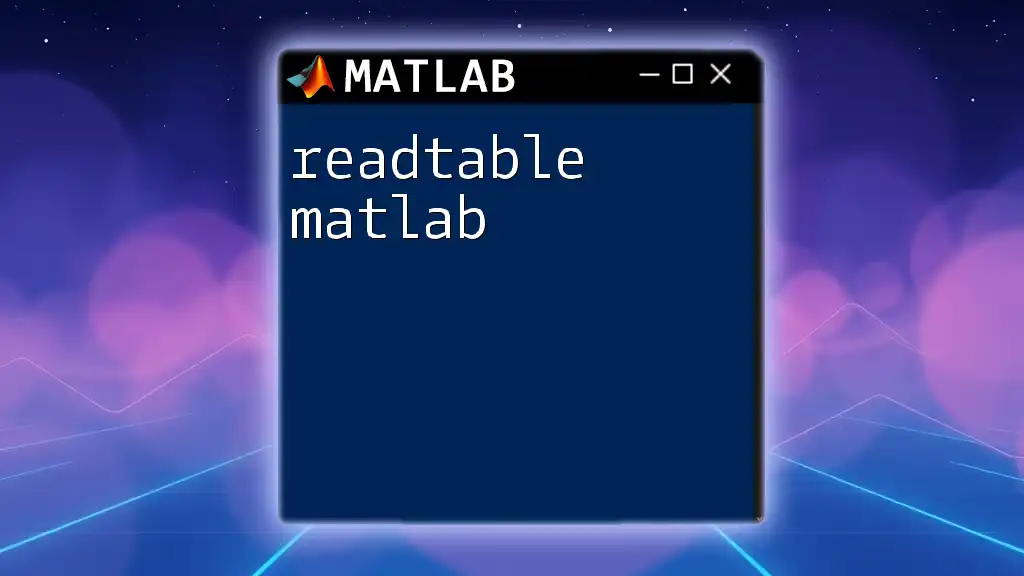
Troubleshooting Tips
Debugging Binary Reads
If you encounter issues during reading, consider checking:
- File Format: Verify that the binary file is structured as expected.
- Data Types: Ensure you are using the correct data type specifiers in `fread()`.
Ensuring Data Integrity
To verify that the data was read correctly, check against known values or check if the length matches expected input.
if ~isequal(originalData, readData)
error('Data integrity compromised');
end
This snippet illustrates how you can compare the original dataset with the read dataset to ensure that no corruption has occurred during the process.
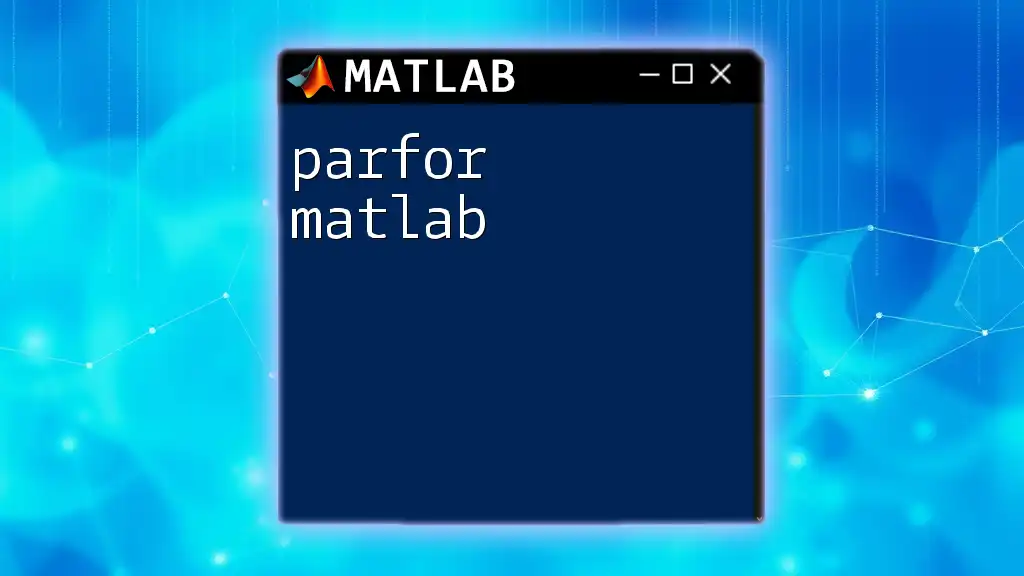
Conclusion
Reading binary files is a powerful feature in MATLAB that opens doors to handling complex data with efficiency and speed. By mastering the built-in functions and structures, as well as understanding error handling and data integrity verification, you can effectively read in binary format in MATLAB and handle various data types with ease. With practice and experimentation, you’ll be well-equipped to incorporate binary data processing into your projects.
Additional Resources
Refer to the MATLAB documentation for more detailed explanations of the functions discussed. Video tutorials and online courses can also provide deeper insights and practical examples for you to explore further. Engaging with the MATLAB community can enhance your learning experience as you share and resolve challenges together.