To read a CSV file into MATLAB, you can use the `readtable` function which imports the data as a table, allowing for easy manipulation and analysis.
data = readtable('filename.csv');
Understanding CSV Files
What is a CSV File?
A CSV file (Comma-Separated Values) is a simple text format for storing tabular data. Each line of the file corresponds to a row in the table, and each value in the line is separated by a comma. The CSV format is widely used due to its simplicity and compatibility with various applications, making it a popular choice for data exchange.
Structure of a CSV File
A typical CSV structure consists of rows and columns, often with the first row acting as a header that provides labels for the columns. For example:
Name, Age, Occupation
John, 28, Engineer
Jane, 32, Doctor
In this structure, we have three columns: Name, Age, and Occupation, with two data entries for individuals.
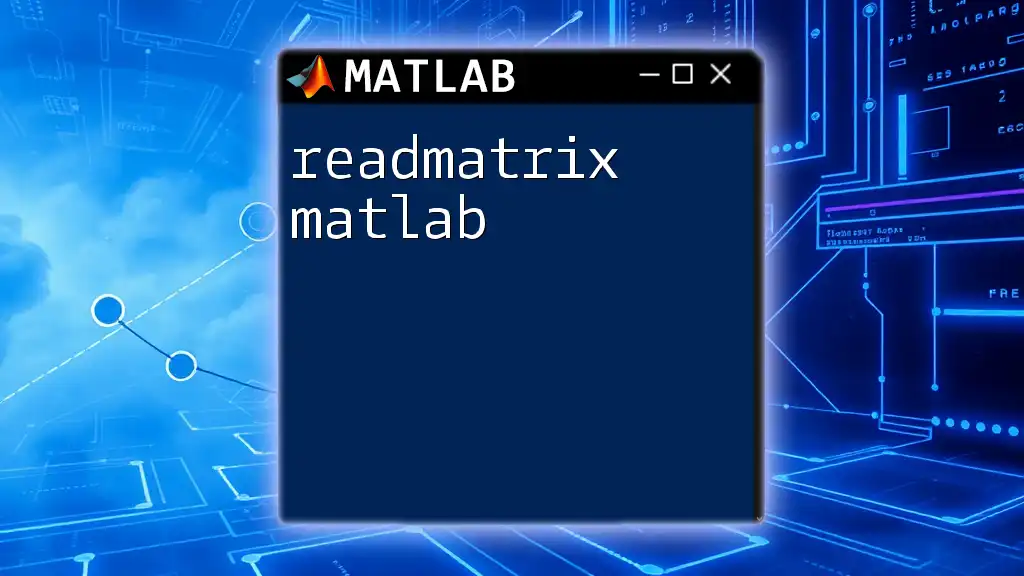
Getting Started with MATLAB
Why Use MATLAB for Data Analysis?
MATLAB is an exceptional environment for mathematical computing, offering powerful functions and tools for data manipulation, analysis, and visualization. With its built-in functions tailored for handling data sets, MATLAB provides an efficient platform for data analysis, particularly when dealing with CSV files.
Installing MATLAB
To begin using MATLAB, download and install it from the official MathWorks website. Ensure that your system meets the requirements outlined during installation. Once installed, launch MATLAB to access its features for data manipulation.
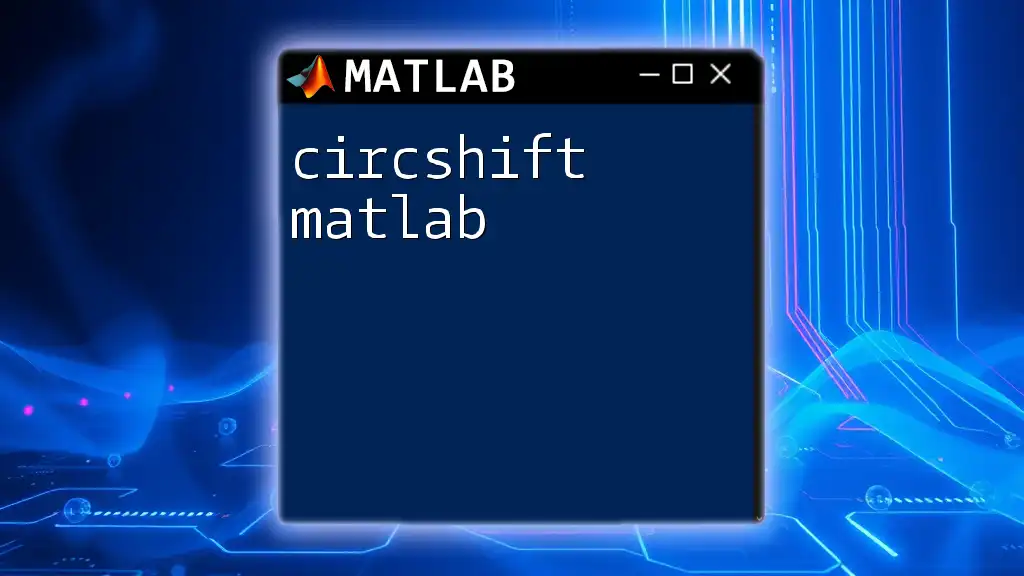
Importing CSV Files into MATLAB
Using `readtable()`
One of the most versatile functions for reading CSV files in MATLAB is `readtable()`. This function imports data from a CSV file into a table, which allows for easy data manipulation and analysis.
-
Syntax and Parameters: You can read a CSV file with the following command:
T = readtable('filename.csv');
-
Example Code Snippet: To read a CSV file named `data.csv`, you would use:
T = readtable('data.csv'); disp(T);
-
Explanation: The output `T` will be a table format that is intuitive for data operations, making it easy to filter, sort, and manipulate data without complex indexing.
Using `csvread()`
Another option for reading CSV data is the `csvread()` function. This function is ideal for numeric matrices but has some limitations when dealing with mixed data types.
-
Syntax and Parameters: The syntax for reading a CSV file using `csvread()` is:
M = csvread('filename.csv', row, col);
-
Example Code Snippet: If you need to read a CSV file and skip the header (first row), you might use:
M = csvread('data.csv', 1, 0); % Skip the header disp(M);
-
Explanation: The output `M` will represent the data as a numeric matrix. This function is most effective when all the data in the CSV file consists solely of numbers.
Using `readmatrix()`
For newer MATLAB versions, `readmatrix()` is a flexible alternative that supports various data types in a CSV file.
-
Syntax and Parameters: To read a CSV file, the syntax is simple:
M = readmatrix('filename.csv');
-
Example Code Snippet: Here's how to read a CSV file:
M = readmatrix('data.csv'); disp(M);
-
Explanation: This function automatically detects the format of data, making it easier to work with files containing various mixed data types.
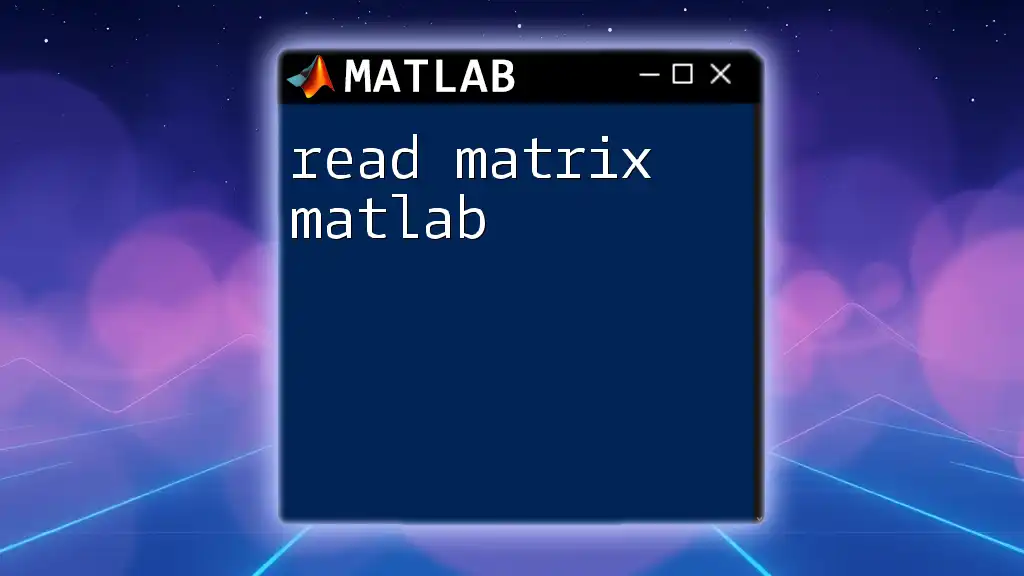
Handling Different CSV Formats
Specifying Delimiters
In some instances, your CSV file might use delimiters other than commas (e.g., semicolons). MATLAB allows you to specify these delimiters.
-
Code Example: If your CSV uses semicolons, you can read it like this:
T = readtable('data.csv', 'Delimiter', ';');
-
Explanation: Specifying a delimiter is crucial for correctly interpreting your data. It ensures that MATLAB distinguishes between different values properly, thus avoiding data misinterpretation.
Reading Specific Rows or Columns
If you want to focus on a specific part of your data, you can select certain rows or columns while reading.
-
Example Code Snippet: To read a specific range from your CSV file, you might use:
T = readtable('data.csv', 'Range', 'A2:C5');
-
Explanation: This can enhance performance by loading only the data you need, reducing memory usage and improving overall script efficiency.
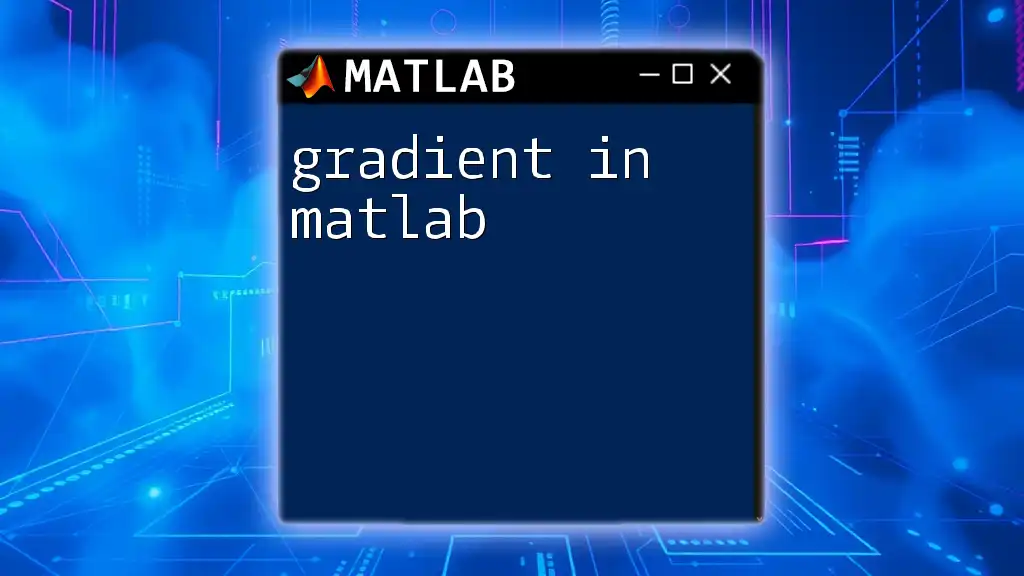
Post-Processing CSV Data in MATLAB
Basic Data Manipulation
After importing your data, basic manipulation can be performed swiftly. For instance, sorting or filtering rows based on certain conditions is incredibly straightforward.
-
Sorting Example: To sort the table by the 'Age' column, you’d use:
sortedData = sortrows(T, 'Age');
Visualizing Data
MATLAB also excels at data visualization. After importing your CSV data, you can create various plots to gain insights.
-
Plotting Example: Here's how you could visualize the Age distribution from the data:
bar(T.Age); xlabel('Individuals'); ylabel('Age'); title('Age Distribution');
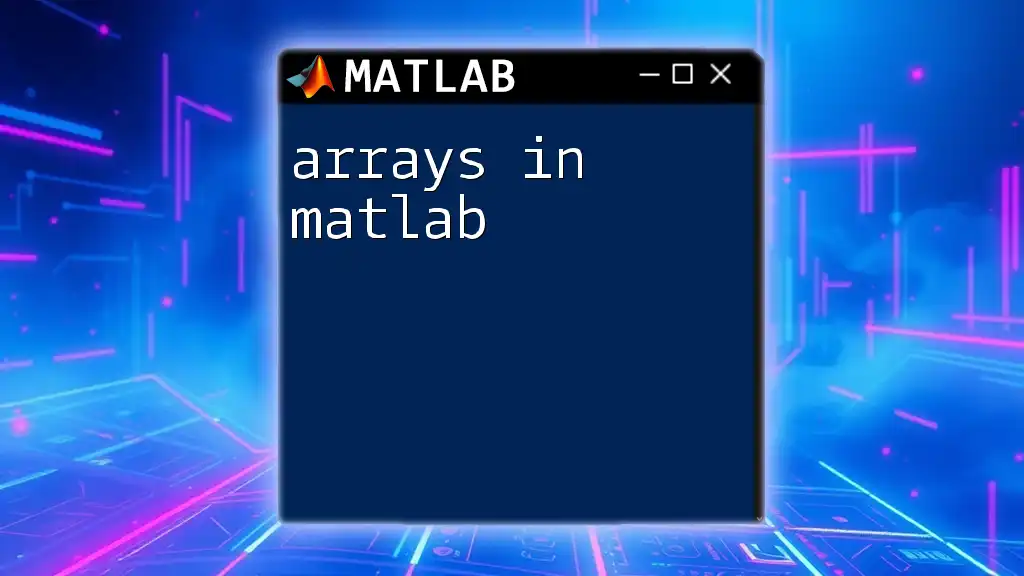
Common Issues and Troubleshooting
Common Errors When Reading CSV files
When working with CSV files, you might encounter errors, such as "file not found" or issues with reading the wrong data types. To handle these, consider the following:
-
File Not Found: Ensure that the file path is correct. It’s often beneficial to specify the full path to avoid confusion.
-
Data Type Confusion: If your CSV data contains mixed types (e.g., strings and numbers), use `readtable()` or `readmatrix()` to seamlessly handle these variations.
-
Debugging: You can implement error handling using try-catch statements:
try T = readtable('data.csv'); catch ME disp('Error reading file:'); disp(ME.message); end
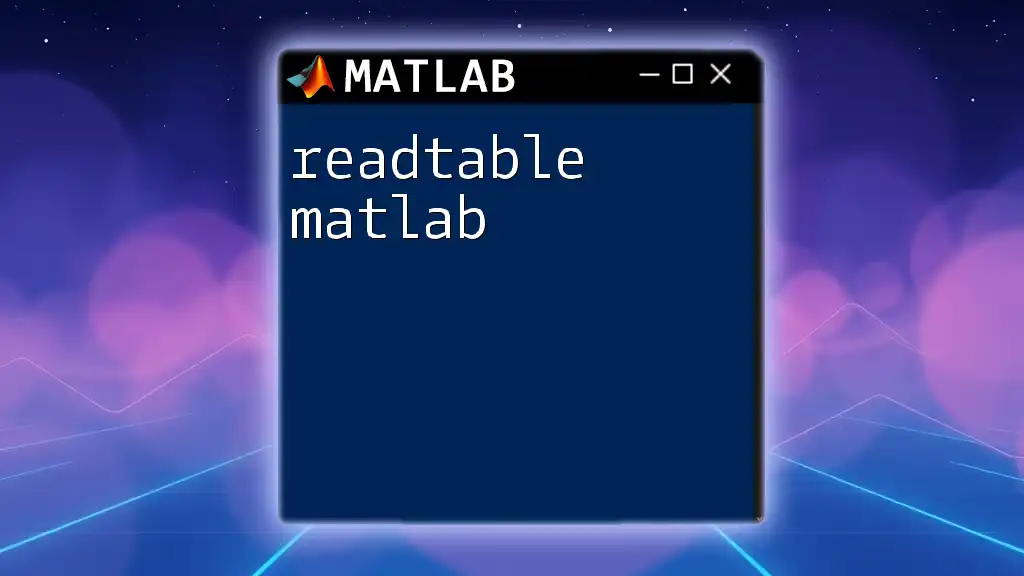
Conclusion
Reading CSV files into MATLAB is a vital skill that can significantly streamline your data analysis process. By utilizing functions like `readtable()`, `csvread()`, and `readmatrix()`, you gain the flexibility to handle data efficiently, allowing for enhanced data manipulation and visualization capabilities. Whether you are a seasoned programmer or a complete novice, understanding how to read CSV into MATLAB will be essential for your data-driven projects. Embrace the power of MATLAB and experiment with the provided code snippets to enhance your analytical skills today!
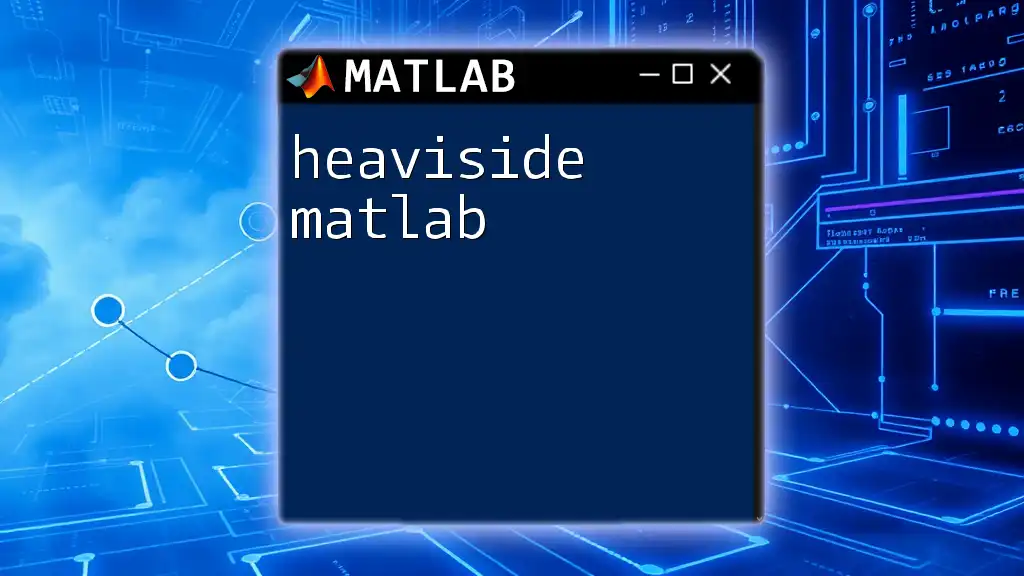
Additional Resources
For further reading and resources, check the official MATLAB documentation for detailed insights on `readtable`, `csvread`, and `readmatrix`. You can also explore additional tutorials for advanced data manipulation in MATLAB.