To separate a string from a number in MATLAB, you can use regular expressions to identify and extract the components from a mixed input string. Here's a code snippet demonstrating this:
inputStr = 'Data123'; % Example input
result = regexp(inputStr, '(\D+)(\d+)', 'tokens'); % Separate into non-digit and digit parts
stringPart = result{1}{1}; % Extract string part
numberPart = str2double(result{1}{2}); % Convert number part to double
Understanding Strings and Numbers in MATLAB
What are Strings in MATLAB?
In MATLAB, strings are sequences of characters that are used to represent text data. Strings can be either character arrays or string arrays. Character arrays are essentially a sequence of characters packed together, whereas string arrays are designed to work more efficiently with sets of strings.
Key characteristics of strings in MATLAB include:
- Strings can contain letters, numbers, symbols, and punctuation marks.
- Character arrays are created using single quotes, while string arrays use double quotes.
For example:
charArray = 'Hello, World!'; % Character Array
strArray = "Hello, World!"; % String Array
What are Numbers in MATLAB?
Numbers in MATLAB represent quantitative data and can be classified into various numeric data types, such as integers, floating-point numbers, and complex numbers. MATLAB handles arithmetic and mathematical computations using these numeric types.
Numeric types in MATLAB can be:
- Scaled (integers) or floating-point (decimals).
- Created directly by assignment or through functions like `zeros`, `ones`, and `rand`.
For instance:
intValue = 42; % Integer
floatValue = 3.14; % Floating-point number
complexValue = 1 + 2i; % Complex number
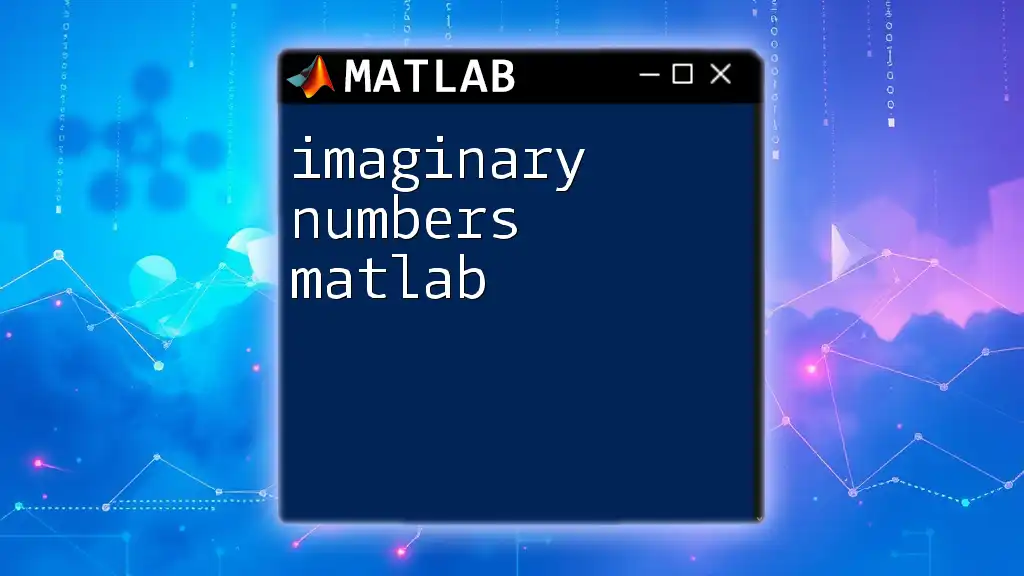
The Need for Separation
Why Separate Strings and Numbers?
Separating strings and numbers is crucial for data preprocessing, especially in analysis and machine learning applications. When dealing with mixed datasets (such as CSV files), having a clear distinction between numeric and text data helps enhance the efficiency of data manipulation, leading to more accurate analysis and predictions.
For example, consider a dataset containing customer feedback, with numerical ratings alongside comments. Separating these data types allows for targeted analysis of ratings and sentiment in comments.
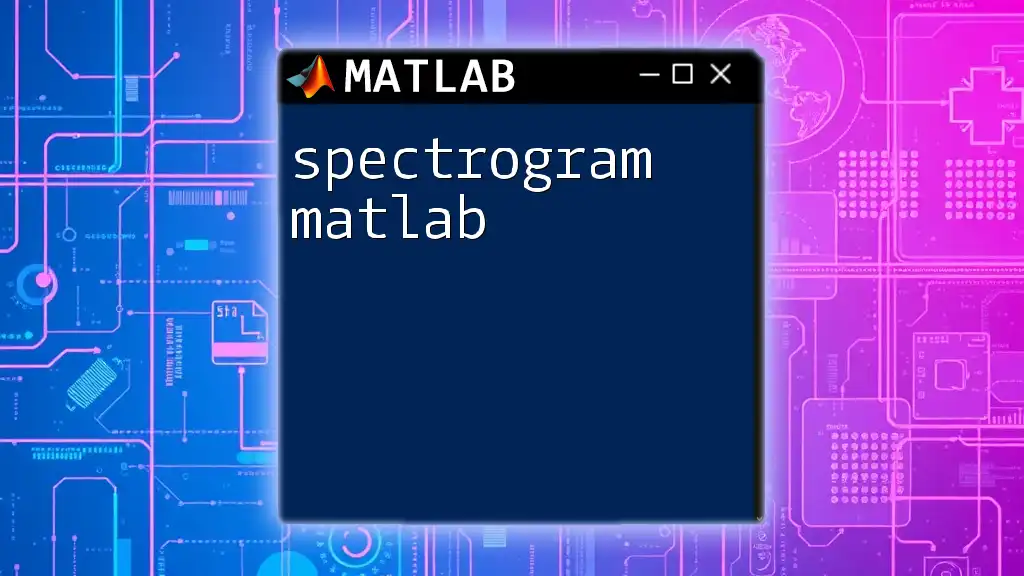
Basic Techniques for Separating Strings and Numbers
Using Regular Expressions
Introduction to Regular Expressions
Regular Expressions (regex) are a powerful tool in MATLAB for pattern matching and manipulation of string data. They enable users to search for patterns in strings, making it easy to separate strings and numbers.
Code Snippet: Basic Regex Example
Here’s how to use regex to extract numbers and strings from a mixed input:
data = 'abc123def456';
numbers = regexp(data, '\d+', 'match'); % Extracts numbers
strings = regexp(data, '[a-zA-Z]+', 'match'); % Extracts strings
In this example, `\d+` matches sequences of digits while `[a-zA-Z]+` matches sequences of letters. The output will be:
- `numbers` → `{'123', '456'}`
- `strings` → `{'abc', 'def'}`
Using `isstrprop` Function
Understanding `isstrprop`
MATLAB offers the `isstrprop()` function, which checks the properties of characters in strings. This function is instrumental when you want to verify whether characters are digits, letters, etc.
Code Snippet: Extracting Numbers and Strings
Utilize the `isstrprop` function as follows:
data = 'abc123def456';
numbers = data(isstrprop(data, 'digit'));
strings = data(isstrprop(data, 'alpha'));
In this example, `isstrprop(data, 'digit')` retrieves all digits while `isstrprop(data, 'alpha')` retrieves alphabetic characters. Thus, you’ll end up with:
- `numbers` → `123456`
- `strings` → `abcdef`
Using Cell Arrays for Mixed Data Types
What are Cell Arrays?
Cell arrays are a special type of array in MATLAB that can hold data of varying types and sizes. They are particularly useful when dealing with mixed data types, such as numbers and strings.
Code Snippet: Separating Data into Cell Arrays
You can separate mixed data using cell arrays:
data = {'apple', '42', 'banana', '24.5'};
numericData = cellfun(@str2double, data, 'UniformOutput', false);
stringData = data(cellfun(@ischar, data));
In this example, `cellfun` applies a function to each cell. Here, `str2double` is used to convert numerics stored as strings, while `ischar` filters for string cells.
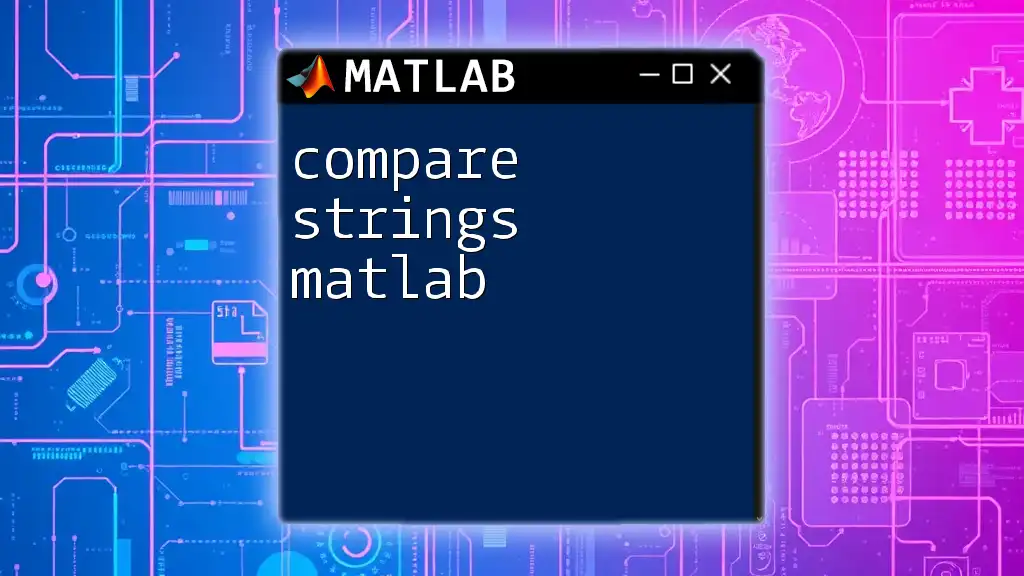
Advanced Techniques
Split and Convert Functions
Using `split` Function
MATLAB's `split` function is handy when you need to divide strings based on delimiters. It allows users to effectively parse complex string data.
Code Snippet: Example of Using `split`
Here is an example of splitting a string containing items with their prices:
data = 'item1:30,item2:50';
splitData = split(data, ',');
The output will separate the items into individual strings, allowing further processing to identify numeric values (like prices).
Custom Functions for Enhanced Flexibility
Writing a Custom Function
If you need to automate the separation process, writing a custom function could streamline your workflow. By encapsulating logic, you can't only promote code reusability but also enhance clarity.
Code Snippet: Example Custom Function
function [numArray, strArray] = separateData(inputArray)
numArray = inputArray(~cellfun('isempty', regexp(inputArray, '\d')));
strArray = inputArray(~cellfun('isempty', regexp(inputArray, '[a-zA-Z]')));
end
This function plays a vital role in identifying numeric and string data efficiently. Here’s how you can call the function:
inputData = {'apple', '42', 'banana', '24.5'};
[numArray, strArray] = separateData(inputData);
By executing this function, you will get:
- `numArray` → `{'42', '24.5'}`
- `strArray` → `{'apple', 'banana'}`
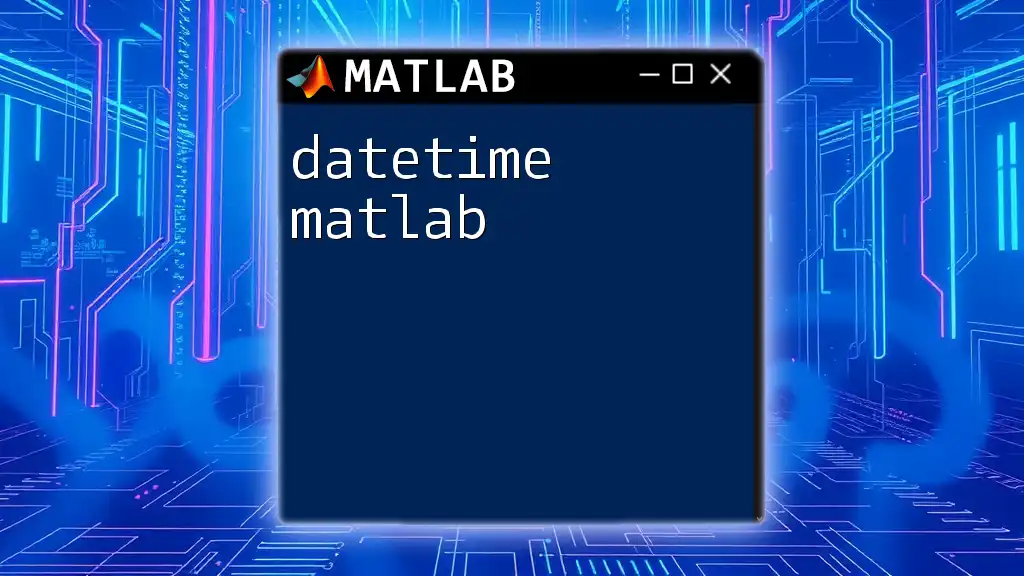
Practical Applications of Data Separation
Data Analysis
Data analysis often necessitates separating strings and numbers for effective processing. For instance, when trying to analyze customer ratings alongside text feedback, separating numbers from strings allows for precise statistical analysis of ratings and deeper exploration of sentiments expressed in comments.
Machine Learning
In machine learning applications, understanding how to separate features (input data) from labels (output data) is paramount. For example, if creating a model to predict housing prices based on descriptions and square footage, separating numerical values from textual descriptions is crucial for accurate model training.
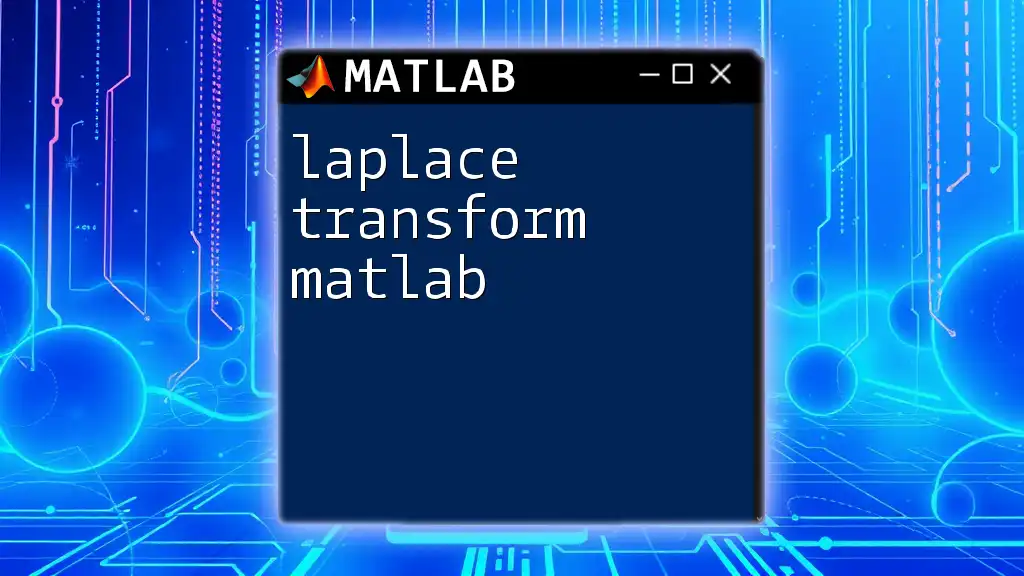
Common Pitfalls and Troubleshooting
Common Errors
Users may encounter several common errors when separating data in MATLAB, such as:
- Forgetting to specify the correct regex pattern.
- Misusing functions that return unexpected data types. To troubleshoot, ensure to review the documentation for each function and maintain clarity on data types.
Best Practices
- Always check and validate your output after executing code.
- Consider the data structure you are working with; clarity in code reduces errors.
- Document your code to ensure maintainability and comprehensibility.
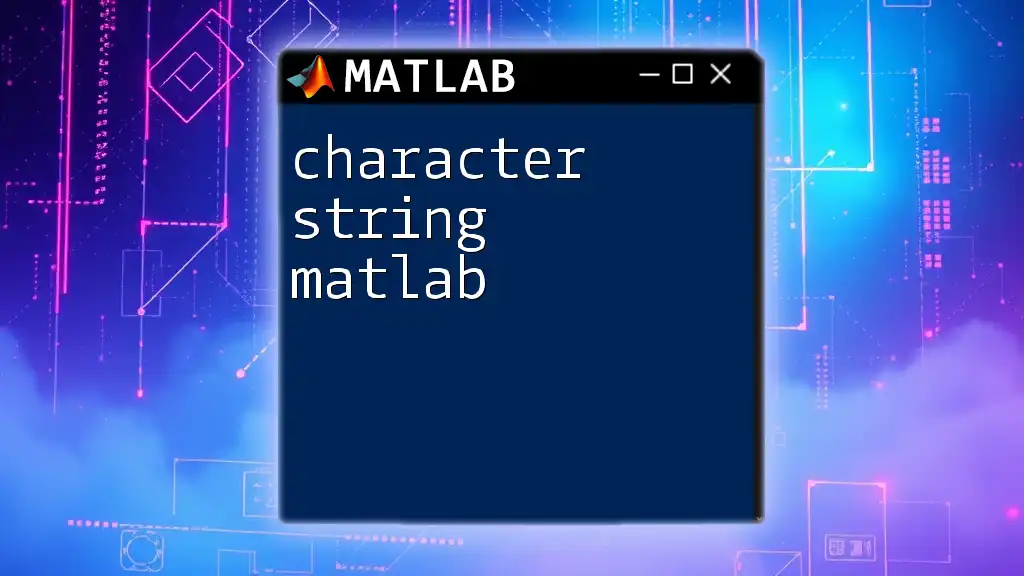
Conclusion
Separating strings and numbers in MATLAB is a foundational skill that enhances your ability to manipulate data effectively. By mastering various techniques, from regular expressions to custom functions, you not only bolster your coding toolkit but also unlock potential improvements in data analysis and machine learning endeavors. Practice these techniques, and you will find that your data processing capabilities expand significantly!
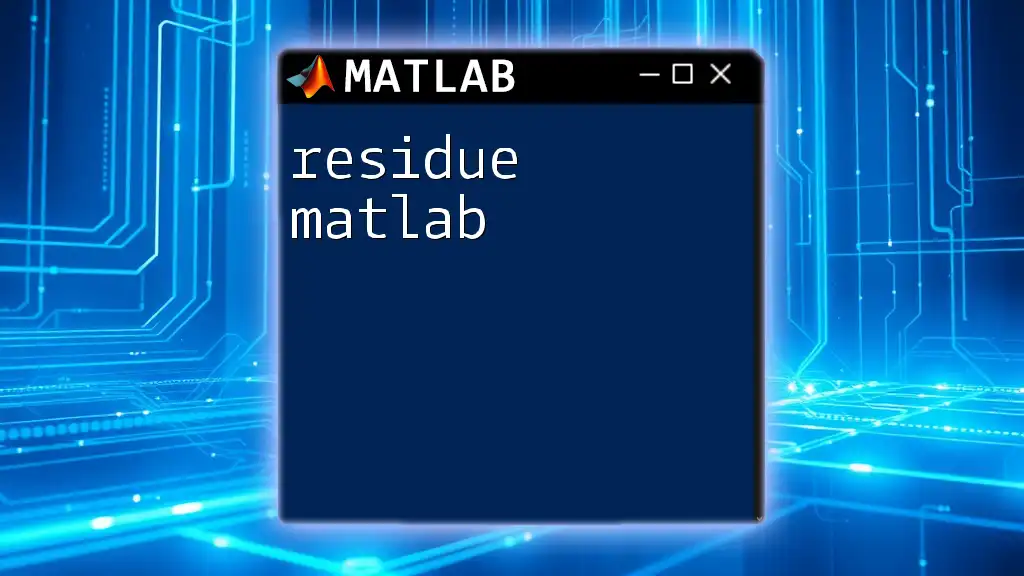
Additional Resources
For further reading, refer to the MATLAB documentation on string manipulation and regular expressions, along with recommended books and online courses that delve deeper into MATLAB programming.