A regression line in MATLAB can be easily computed using the `polyfit` function to fit a linear model to your data points and then `polyval` to evaluate the model.
% Sample data points
x = [1, 2, 3, 4, 5];
y = [2.2, 2.8, 3.6, 4.5, 5.1];
% Fit a linear regression line
p = polyfit(x, y, 1); % p(1) = slope, p(2) = intercept
% Evaluate the regression line
yfit = polyval(p, x);
% Plot the data points and regression line
scatter(x, y, 'filled'); % Plot original data
hold on;
plot(x, yfit, 'r-'); % Plot regression line
xlabel('X-axis');
ylabel('Y-axis');
title('Regression Line in MATLAB');
hold off;
What is a Regression Line?
Regression line is a fundamental concept in statistics and data analysis that represents the relationship between a dependent variable and one or more independent variables. It is essentially a statistical tool that helps predict the value of the dependent variable based on known values of independent variables.
Definition and Explanation
The regression line in its simplest form is achieved through a linear regression analysis, which assumes a linear relationship between the input variable (independent variable) and the output variable (dependent variable). The mathematical representation of a linear regression line is typically expressed as:
\[ y = mx + b \]
Where:
- \( y \) is the dependent variable,
- \( m \) represents the slope of the line,
- \( x \) is the independent variable, and
- \( b \) is the y-intercept.
Use Cases
Understanding and applying regression lines is crucial across various fields. For instance:
- Engineering: Used to predict outcomes based on design parameters.
- Finance: Helps forecast trends in stock prices based on historical data.
- Social Sciences: Aids in understanding relationships between socio-economic factors.
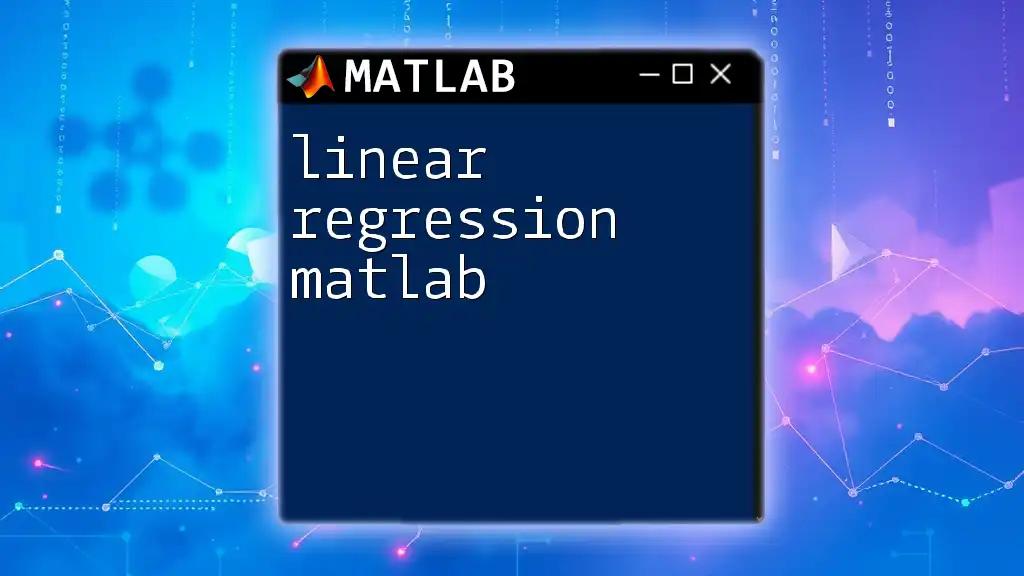
Understanding Linear Regression in MATLAB
Mathematical Foundation
The key aspect of linear regression is finding the best-fitting regression line, which minimizes the difference between the observed data points and the predicted values on the regression line. This is typically done using the least squares method.
Selection of Variables
In any regression analysis, clearly distinguishing between dependent and independent variables is essential. The dependent variable is the outcome you are trying to predict, while independent variables are the predictors that you think influence this outcome.
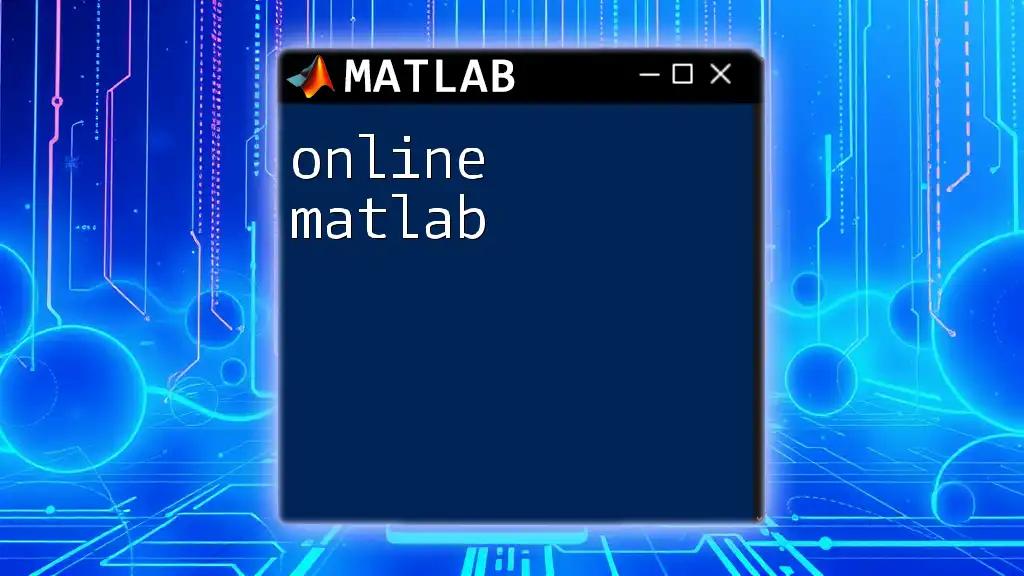
Getting Started with MATLAB
Setting Up MATLAB Environment
Before diving into regression line analysis in MATLAB, ensure that your environment is set up correctly. Download and install the latest version of MATLAB. Familiarize yourself with its interface, especially the Command Window, Workspace, and Editor.
Data Importing Techniques
You can import data into MATLAB using various functions:
- `readtable()`: Import data from text or spreadsheet files easily.
- `load()`: Load data from MAT-files into your workspace.
For example:
data = readtable('data_file.csv');
Preliminary Data Exploration
Before fitting a regression model, it's important to explore your data. Utilize functions like `mean()`, `median()`, and `std()` to grasp the data characteristics.
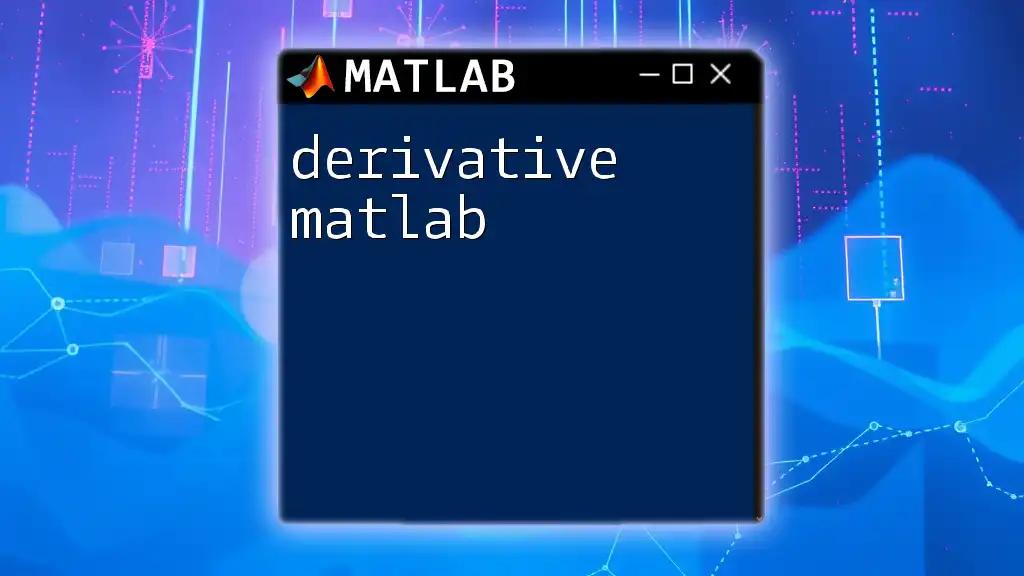
Creating a Simple Regression Line
Basic Syntax and Structure
To fit a simple regression line in MATLAB, the polyfit function is key. This function computes the coefficients of the polynomial that minimizes the error between the actual data points and the polynomial's values.
Here’s how to use it:
% Example data
x = [1, 2, 3, 4, 5]; % Independent variable
y = [2, 3, 5, 7, 11]; % Dependent variable
% Fitting a linear regression model
p = polyfit(x, y, 1); % 1 for linear regression
Visualizing the Regression Line
To visualize your regression line alongside your data points, you can use the plot function:
% Plot data
plot(x, y, 'o');
hold on;
% Generate x values for the regression line
x_fit = linspace(min(x), max(x), 100);
y_fit = polyval(p, x_fit);
% Plot regression line
plot(x_fit, y_fit, '-r');
hold off;
title('Regression Line');
xlabel('Independent Variable');
ylabel('Dependent Variable');
This code will create a scatter plot of your data points and overlay the regression line, allowing for a clear visual representation of the relationship.
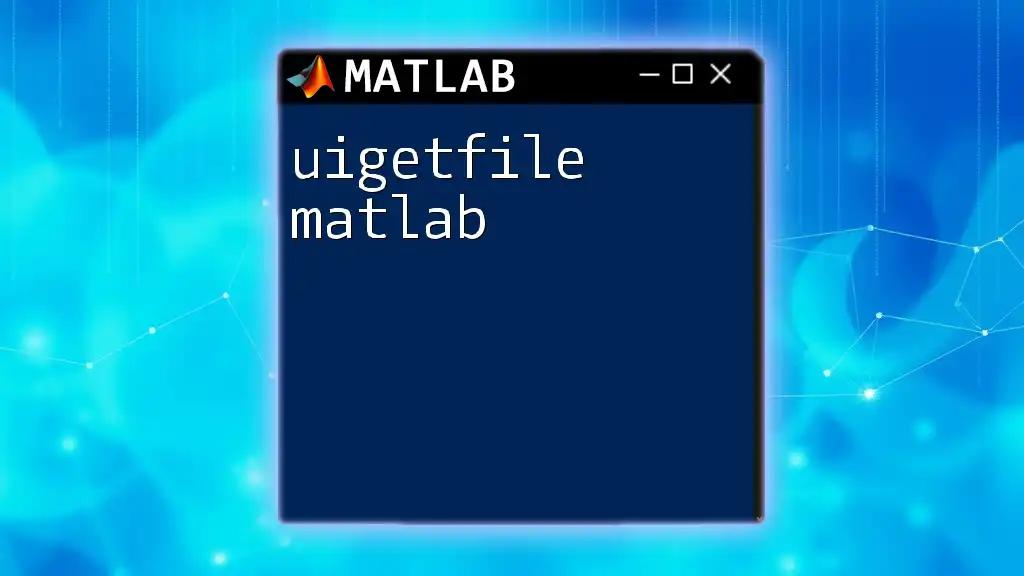
Evaluating the Regression Model
Goodness of Fit
A key metric in assessing how well your regression line fits the data is the R-squared statistic. This value ranges from 0 to 1, with higher values indicating a better fit.
To calculate R-squared in MATLAB, you can use the following approach:
y_hat = polyval(p, x);
SS_tot = sum((y - mean(y)).^2);
SS_res = sum((y - y_hat).^2);
R_squared = 1 - (SS_res / SS_tot);
Interpreting Results
The slope and intercept derived from the `polyfit` function provide insights into the relationship between the variables:
- The slope (m) tells you how much y changes with a unit change in x.
- The intercept (b) indicates the expected value of y when x is zero.
Additionally, analyzing the residuals—the differences between observed and predicted values—can provide further insights into the model's accuracy.
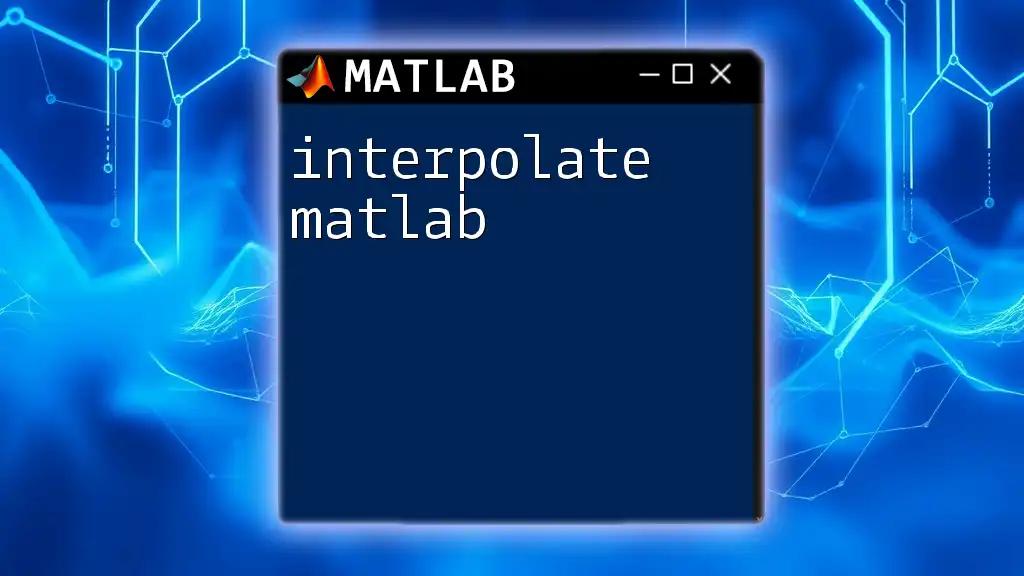
Advanced Techniques in Regression Analysis
Multiple Linear Regression
When working with more than one independent variable, you can extend your regression analysis to multiple linear regression. In MATLAB, the `regress` function allows for this expansion.
% Assuming X is the matrix of input variables (including a column of 1's for the intercept)
Y = [y']; % Reshaping to a column vector
b = regress(Y, [ones(length(x), 1), x']); % Adding intercept
Polynomial Regression
For cases where the relationship is not linear, polynomial regression can be employed. It allows the fitting of polynomial equations to the data rather than simply linear equations.
Here’s how to fit a quadratic polynomial:
p2 = polyfit(x, y, 2); % 2 for quadratic regression
Regularization Techniques
To prevent overfitting in your regression models, consider employing regularization techniques such as Ridge and Lasso regression. These methods include penalty terms that shrink coefficients to reduce model complexity, which is vital when dealing with high-dimensional data.
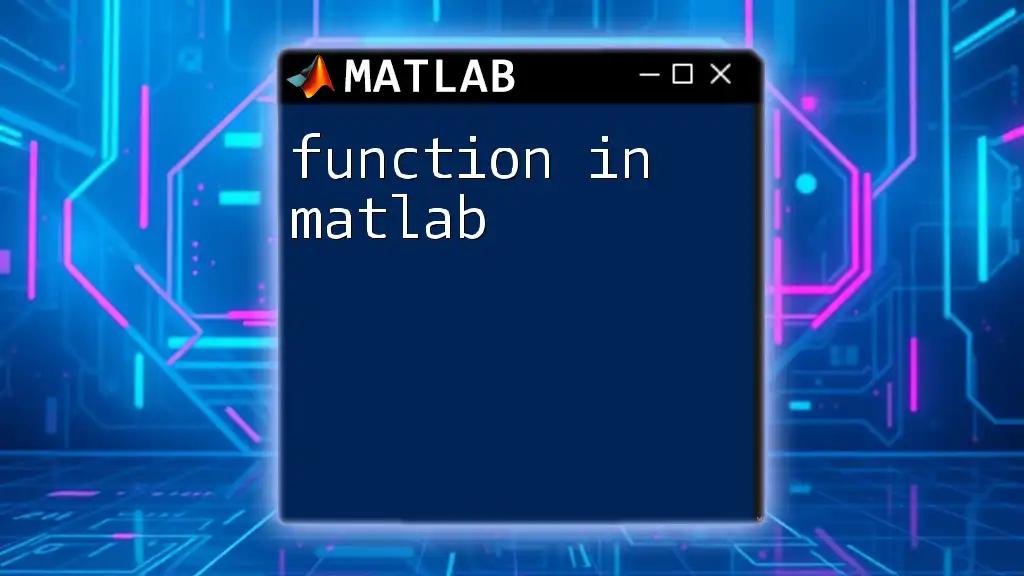
Practical Example: Regression in Real-world Data
Case Study: Predicting House Prices
Imagine you have a dataset on house prices with various features such as square footage, number of bedrooms, etc. Here’s a simplified workflow to perform regression analysis using `regression line MATLAB`:
- Import the dataset: Use `readtable()` to load your data.
- Explore the data: Use MATLAB functions to examine the structure and basic statistics.
- Fit the regression model: Select features and use `polyfit()` for the regression analysis.
- Visualize: Create plots to show the relationship between predicted and actual prices.
- Evaluate: Use R-squared and residual analysis to check the model's performance.
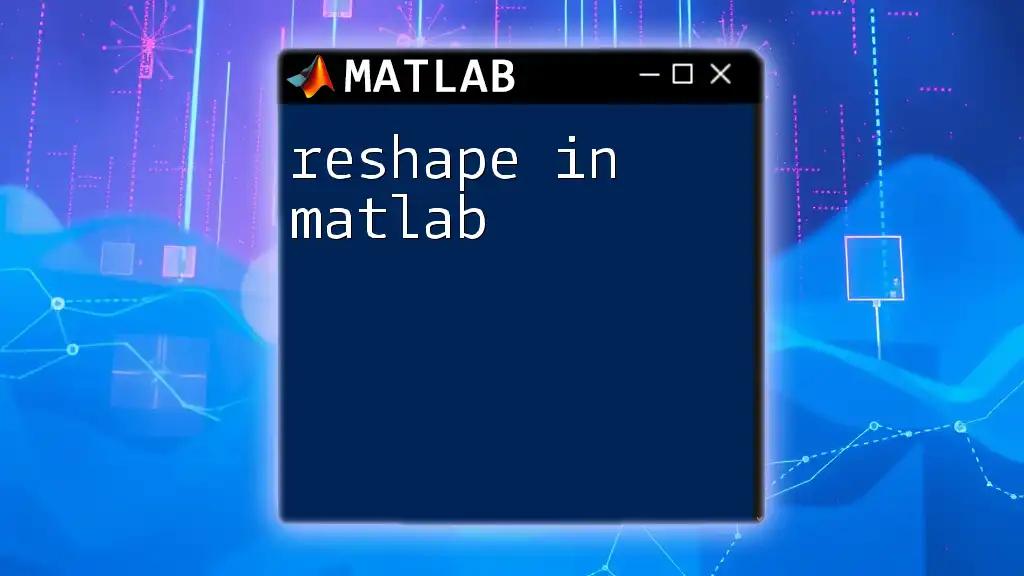
Best Practices for Regression Analysis in MATLAB
Data Cleaning and Preprocessing
Before any regression analysis, it's imperative to ensure that your data is clean. Check for missing values, outliers, and any inconsistencies. Techniques might include removing or imputing missing values and filtering out extreme values.
Choosing the Right Model
Be cautious about overfitting and underfitting:
- Overfitting occurs when the model is too complex, capturing noise rather than the underlying trend.
- Underfitting happens when the model is too simple to capture the underlying pattern in the data.
Documentation and Commenting Code
Remember to document your work effectively. Clear comments in your code can immensely help future users (including yourself) understand your logic and methodology.
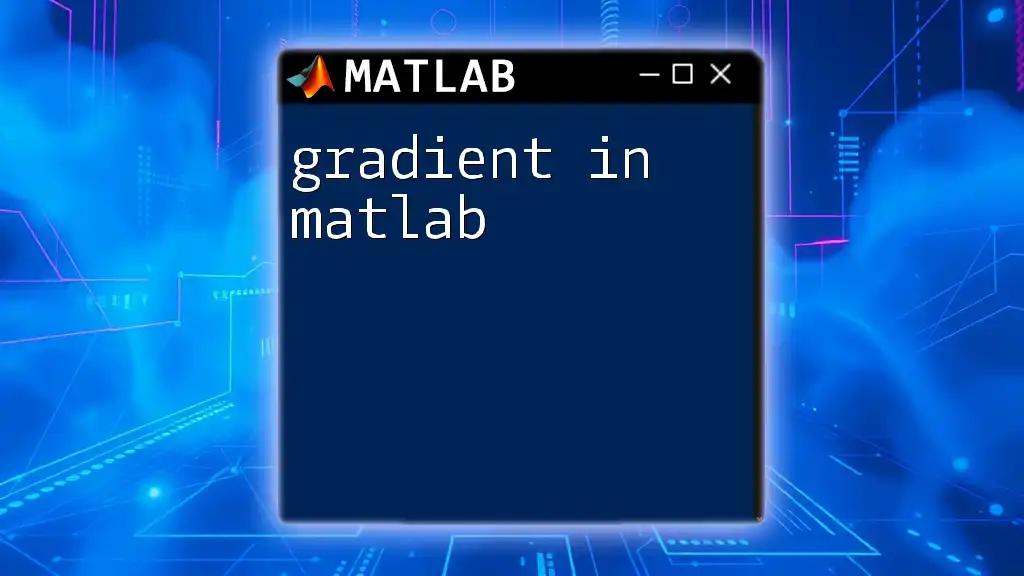
Conclusion
In this comprehensive examination of regression line MATLAB, we explored the definition and fundamentals of regression lines, practical steps to create and evaluate them in MATLAB, and advanced techniques to enhance your regression analysis. By practicing these techniques, experimenting with real datasets, and understanding best practices, you'll be well on your way to mastering regression analysis in MATLAB. Happy coding!
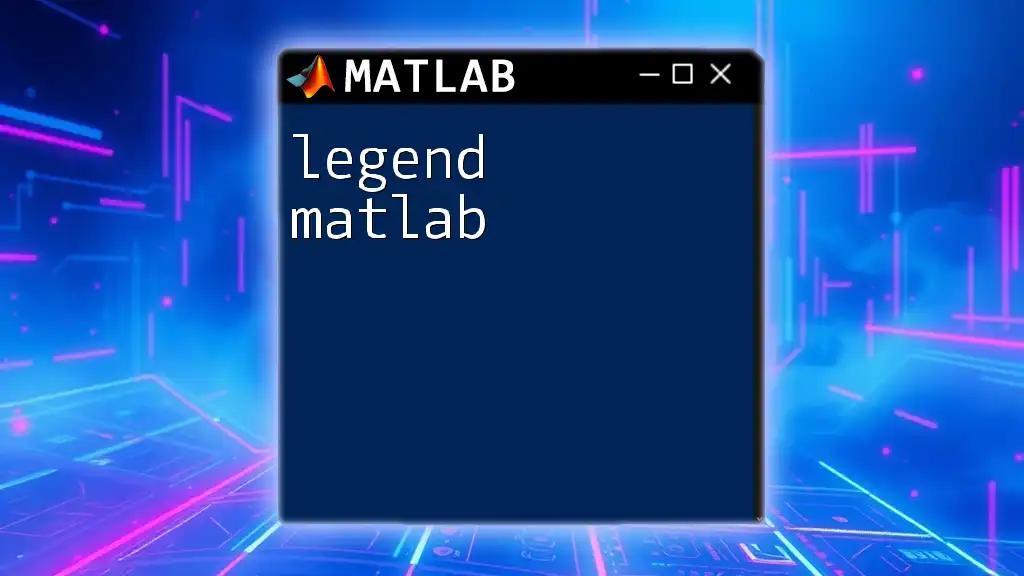
Additional Resources
To deepen your understanding, explore the official MATLAB documentation, consider recommended books on statistical modeling, and take advantage of online courses that can offer further insights into regression analysis and other advanced topics.