The `ss2tf` function in MATLAB converts state-space system representations into transfer function forms, allowing for easier analysis and design of control systems.
Here’s a simple example using `ss2tf`:
% Define state-space matrices
A = [0 1; -2 -3];
B = [0; 1];
C = [1 0];
D = 0;
% Convert state-space to transfer function
[num, den] = ss2tf(A, B, C, D);
Understanding State-Space Representation
What is State-Space Representation?
The state-space representation is a powerful mathematical framework used to model dynamic systems. In this format, a system is defined by a set of first-order differential equations. It encapsulates systems in the form of vector variables that represent the system's internal state. This method is widely used in control theory, signal processing, and various engineering fields due to its ability to handle multivariable systems and non-linearities effectively.
Importance of Conversion to Transfer Function
Converting state-space representations to transfer functions is essential for several reasons:
- Simpler Analysis: Transfer functions provide a more straightforward approach to analyze system behaviors such as stability and frequency response.
- Foundational Tool: Many classical control design techniques, such as root locus and frequency response methods, directly utilize transfer functions for successful implementation.
- Simulation Compatibility: Working with transfer functions often allows for quick simulations of system responses, which is significantly beneficial in system design and validation.
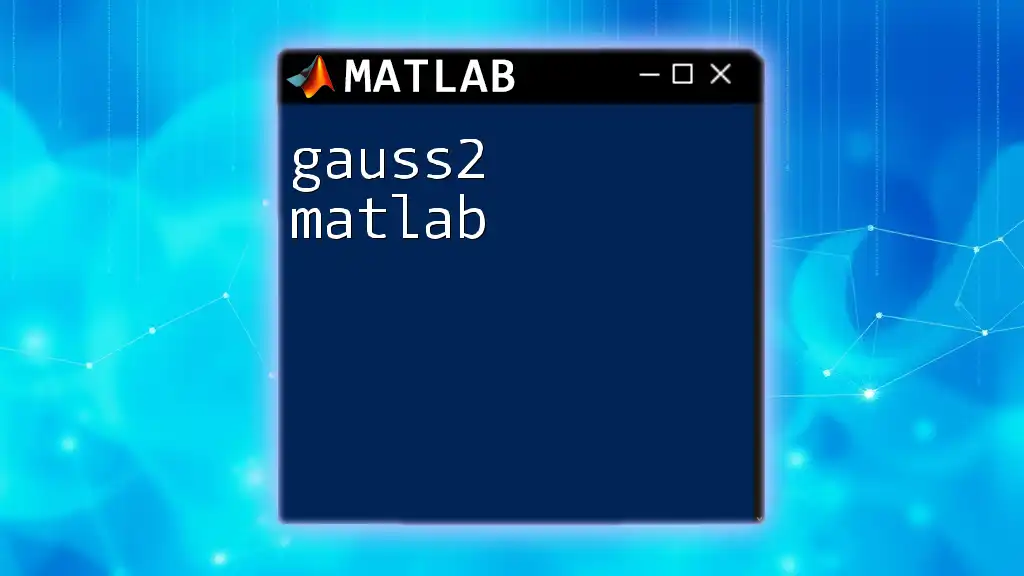
Understanding `ss2tf` Function in MATLAB
What is `ss2tf`?
The `ss2tf` function in MATLAB provides a way to convert state-space system representations directly into transfer function format. This MATLAB command streamlines the process of transforming the matrices that represent a system into the numerator and denominator polynomials of its transfer function. This command is pivotal for engineers and researchers working with dynamic systems.
Syntax of `ss2tf`
The general syntax for using `ss2tf` is as follows:
[num, den] = ss2tf(A, B, C, D, n)
Here, the parameters are defined as such:
- A: The state matrix that represents system dynamics.
- B: The input matrix linking inputs to states.
- C: The output matrix that connects states to outputs.
- D: The feedthrough matrix that connects inputs directly to outputs.
- n: This optional parameter specifies the output index when there are multiple outputs.
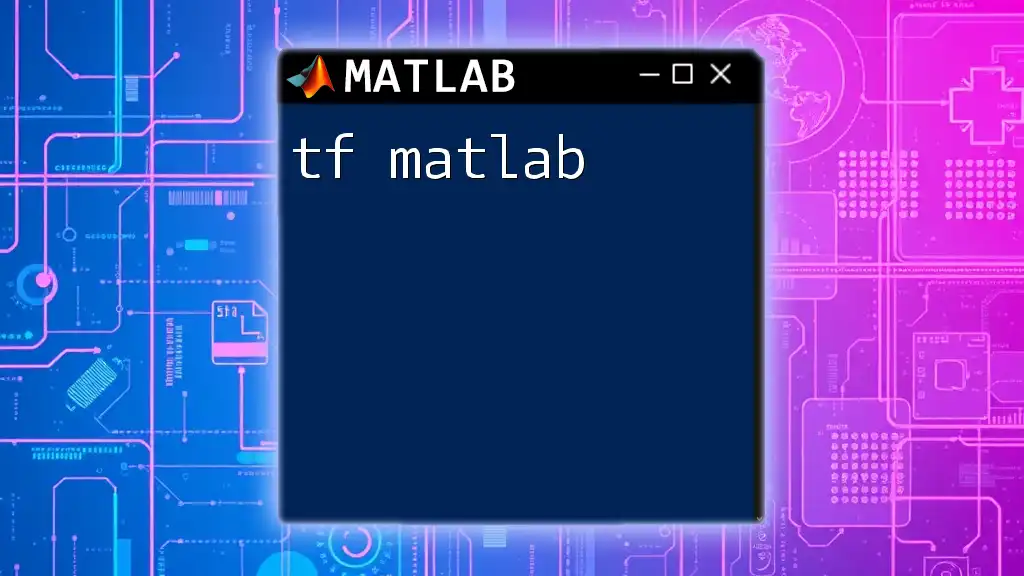
How to Use `ss2tf` in Practice
Setting Up Your State-Space Model
The first step in employing the `ss2tf` function is to create the matrices that represent your state-space model.
Creating Matrices
These matrices can typically be defined from system characteristics. For instance, consider a simple second-order system represented by the following matrices:
A = [0 1; -2 -3];
B = [0; 1];
C = [1 0];
D = 0;
In this example:
- The matrix A defines the dynamics of the system.
- The matrix B indicates how the input affects the state.
- The matrix C shows how the state is connected to the output.
- The matrix D represents any direct influence of the input on the output.
Applying `ss2tf` to Convert to Transfer Function
Once the matrices are established, the next step is to execute `ss2tf` for conversion.
Using the previously defined matrices, the conversion would look like this:
[num, den] = ss2tf(A, B, C, D);
disp('Numerator:');
disp(num);
disp('Denominator:');
disp(den);
Upon running this code, MATLAB will return the numerator and denominator, which together constitute the transfer function of the system.
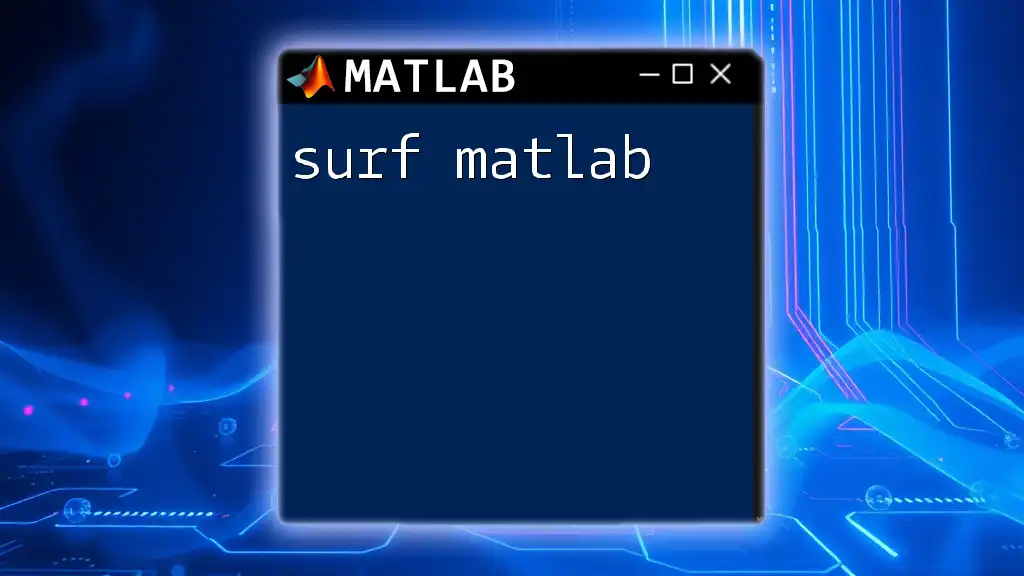
Detailed Example: Converting a State-Space System
Example State-Space System
For a more elaborate scenario, consider a mass-spring-damper system represented by the following state-space matrices:
Matrices for Example System
A = [0 1; -5 -6];
B = [0; 1];
C = [1 0];
D = 0;
Perform the Conversion
To convert the above matrices into transfer function format, simply run:
[num, den] = ss2tf(A, B, C, D);
Analyzing the Output
The output consists of two arrays: num (the numerator polynomial) and den (the denominator polynomial). The values in these arrays are essential for understanding the system's behavior; they indicate the relationship between the inputs and outputs of your system.
For example, if the output is:
- Numerator: `0 1`
- Denominator: `1 6 5`
The transfer function can be defined as:
\[ G(s) = \frac{0s^0 + 1}{1s^2 + 6s + 5} \]
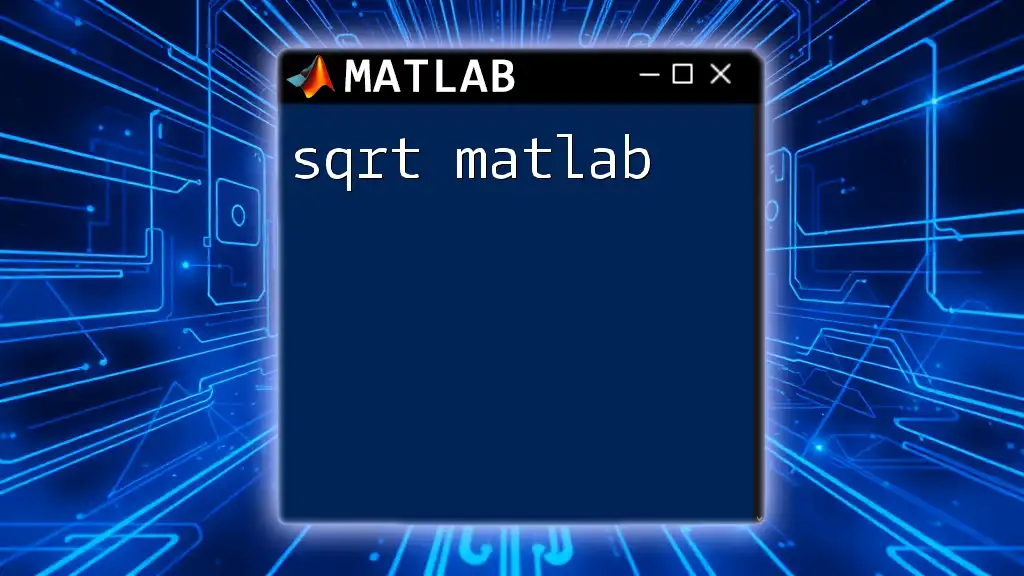
Practical Applications of `ss2tf`
Control System Design
The transformation from state-space to transfer function is crucial for control system designers. With the transfer function at hand, designers can employ techniques such as root locus, Bode plots, and Nyquist criteria to optimize system performance, ensuring stability and responsiveness in dynamic environments.
Simulation and System Response
Transfer functions are instrumental in simulating and analyzing system responses. For example, one can easily plot the step response of the system using the created transfer function:
sys = tf(num, den);
step(sys);
grid on;
title('Step Response of the System');
This script generates a visual representation of how the system reacts over time to a step input, providing insights into system dynamics and stability.
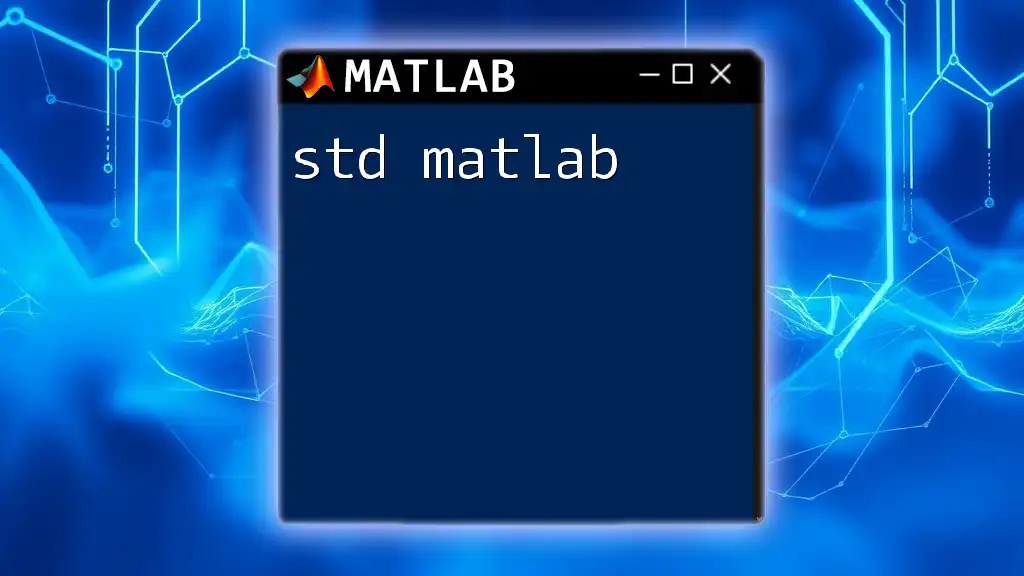
Common Issues and Troubleshooting
Common Errors with `ss2tf`
Users may encounter typical errors while using `ss2tf`, such as:
- Matrix Dimension Mismatch: Ensure that the matrices A, B, C, and D have compatible dimensions; otherwise, MATLAB will throw an error.
- Improper Matrix Definitions: Ensure that the matrices accurately reflect the physical system being modeled.
Tips for Effective Use
To optimize your use of `ss2tf`, consider the following best practices:
- Double-check matrix definitions and dimensions.
- Utilize MATLAB’s `size()` function to validate matrix formats before applying `ss2tf`.
- Validate and test the transformed transfer function by comparing outputs with known behaviors.
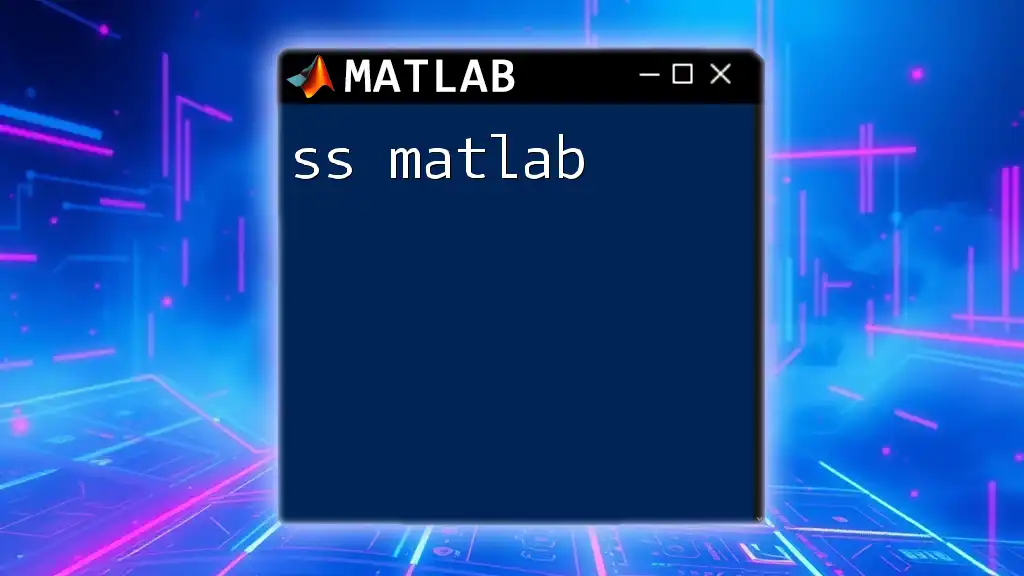
Conclusion
Recap of Key Points
In this guide, we explored the `ss2tf` function in MATLAB, which is instrumental in converting state-space representations into transfer functions. Understanding the syntax and application of `ss2tf` empowers users to perform essential analysis and design tasks in control systems.
Further Reading and Resources
To deepen your understanding, consider diving into MATLAB’s official documentation on system representation. Additionally, exploring tutorials on control systems and simulation will solidify your grasp on utilizing `ss2tf` effectively.
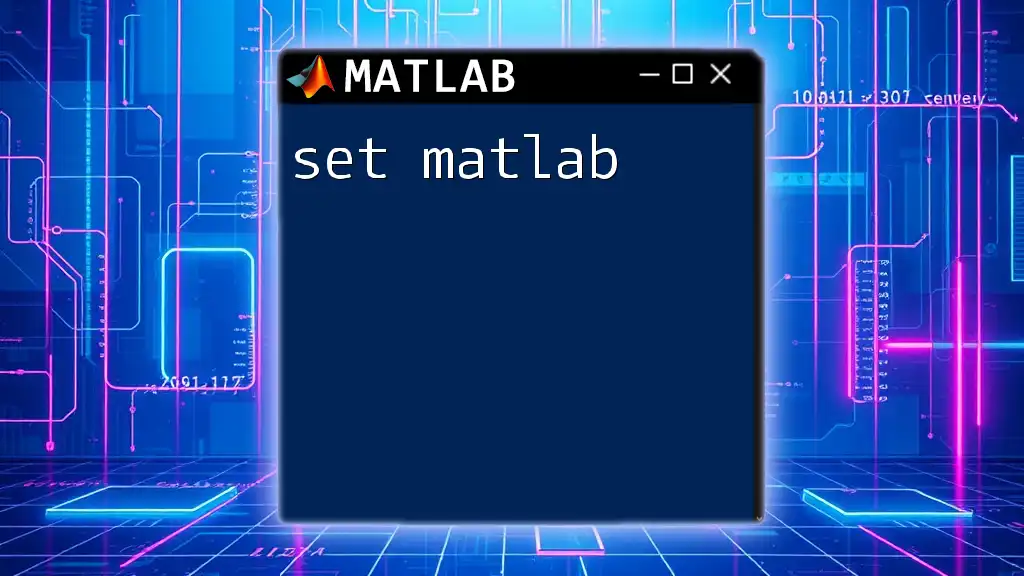
Call to Action
You are encouraged to practice using `ss2tf` with various examples and real-world systems. If you seek further assistance or structured training on MATLAB, consider seeking specialized courses tailored to enhance your skills and confidence in using MATLAB for dynamic systems.