In MATLAB, a table is a data type that organizes data in rows and columns, similar to a spreadsheet, allowing for easy access and manipulation of heterogeneous data.
Here's a simple example of creating a table in MATLAB:
% Create sample data
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 35];
Heights = [5.5; 6.0; 5.8];
% Create a table
T = table(Names, Ages, Heights)
% Display the table
disp(T);
What is a Table in MATLAB?
Tables in MATLAB are a type of data structure designed to store column-oriented or tabular data. They can hold variables of different types in each column, making them particularly useful for data analysis and manipulation. When you work with large datasets, tables organize the information in a way that is easy to read and understand.
Advantages of Using Tables
Using tables in MATLAB offers several advantages:
- Intuitive Organization: Tables display data in a clear and structured manner, with named columns for easy access.
- Versatile Data Types: Unlike traditional numerical arrays, tables can hold various data types, including strings, numbers, and categorical data.
- Built-In Functions: MATLAB provides powerful built-in functions specifically tailored for table operations, enhancing productivity and analysis efficiency.
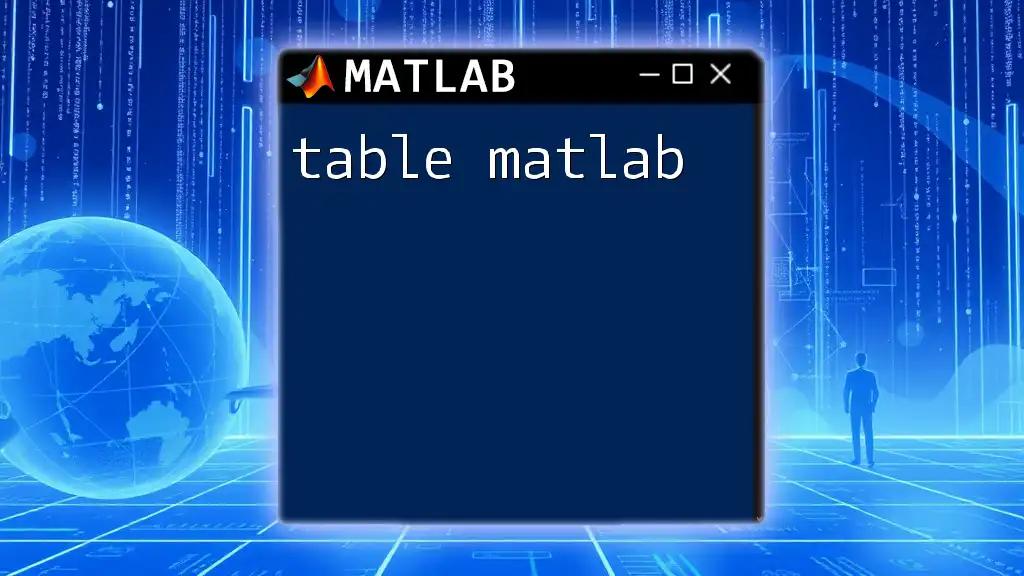
Creating Tables in MATLAB
Using Array Data to Create a Table
You can easily create a table from array data using the `table` function in MATLAB. For example:
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 35];
T = table(Names, Ages);
In this code snippet, we create a table `T` with two variables: `Names` and `Ages`. Each variable corresponds to a column in the table. The names of columns are derived directly from the variable names.
Importing Data to Create a Table
Another common way to create a table is by importing data from an existing file, such as a CSV. You can use `readtable` to import data easily:
T = readtable('data.csv');
When importing data, MATLAB automatically detects the data types and creates a table accordingly. Ensuring proper formatting during the import process is crucial for accurate analysis.
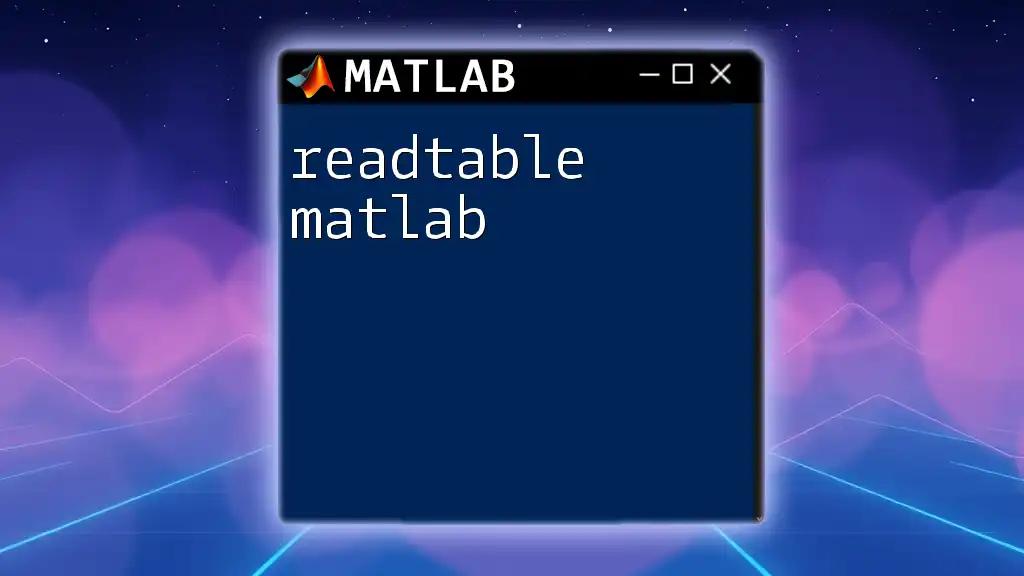
Accessing Data in Tables
Row and Column Access
Accessing data in tables is straightforward. You can use dot notation for columns and parentheses for rows. For example:
T.Ages % Accessing the column 'Ages'
T{1:2, :} % Accessing the first two rows
This manner of accessing data allows you to extract specific information quickly, tailoring your data analysis according to your needs.
Logical Indexing
Logical indexing is a powerful feature in MATLAB that allows you to filter data based on specific criteria. For example, if you want to find all individuals under the age of 30, you can write:
youngPeople = T(T.Ages < 30, :);
Logical indexing is beneficial in scenarios where you need to analyze subsets of data without manually sorting or filtering the original dataset.
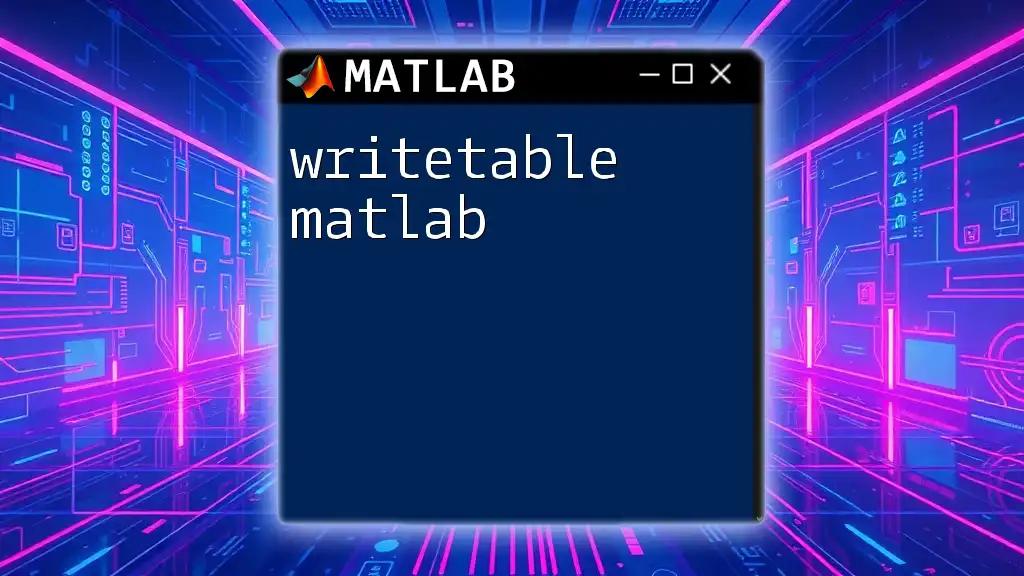
Modifying Tables
Adding New Variables (Columns)
You can expand the information in your table by adding new columns. For instance, if you want to include a column for `Height`, you can do the following:
T.Height = [5.5; 6.0; 5.8];
This modifies the original table `T`, adding a new variable `Height`, while maintaining the existing data.
Changing Existing Data
Changing values in specific rows and columns is also simple. For example, to update Alice's age, you could use:
T.Ages(1) = 26;
This code snippet directly modifies the existing age data, showcasing how easy it is to manage tabular data.
Removing Variables (Columns)
If you need to remove unnecessary data from your table, you can easily do so. To delete the `Ages` column, for example, use:
T.Ages = [];
This will remove the entire column from the table, allowing for cleaner data management.
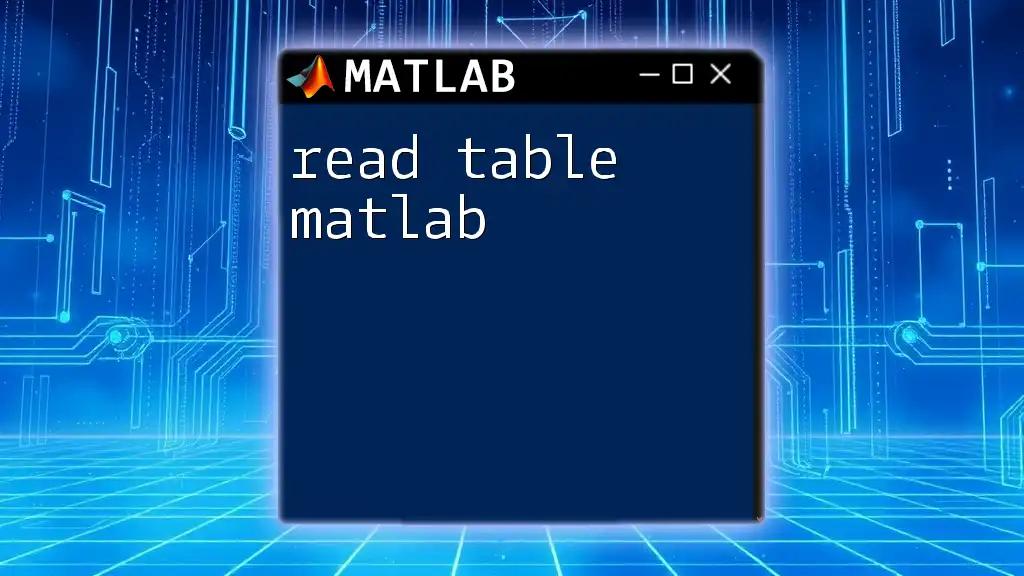
Sorting and Organizing Tables
Sorting Tables
Sorting your table data can help identify trends or organize information for analysis. You can sort tables based on one or more columns with the `sortrows` function:
sortedT = sortrows(T, 'Ages');
The new variable `sortedT` will contain the data sorted by age, streamlining your analysis process.
Grouping Data in Tables
Grouping data can provide insightful summaries rather than just raw data. You can achieve this using the `rowfun` function. For instance, to find the average age for each name:
meanAges = varfun(@mean, T, 'GroupingVariable', 'Names', 'InputVariable', 'Ages');
This operation is valuable for generating summaries that inform decision-making based on trends and averages.
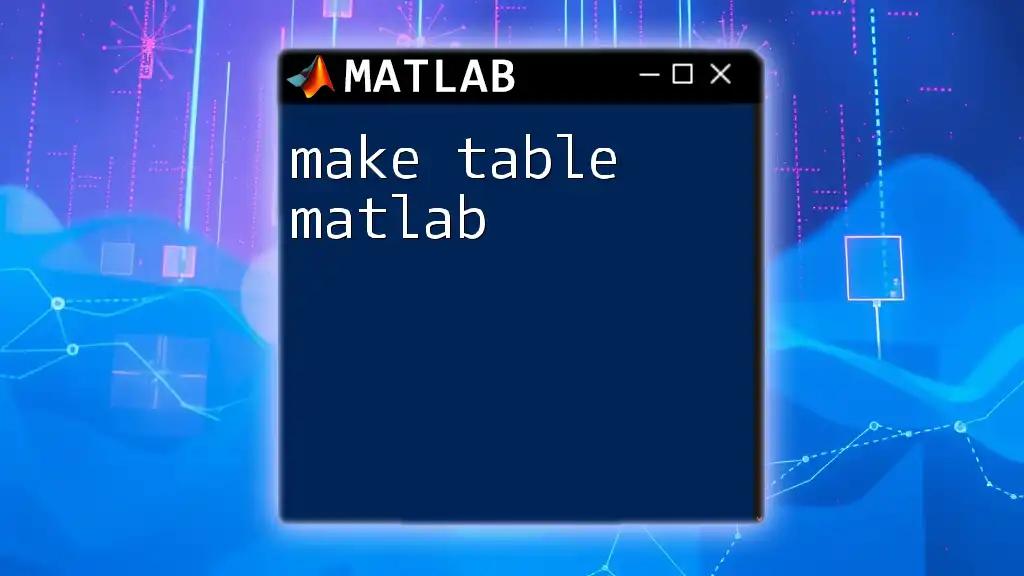
Advanced Table Operations
Merging Tables
Sometimes, you may need to combine data from multiple tables. You can use the `outerjoin` function to merge tables on a common variable:
T2 = table({'Dave'; 'Eva'}, [28; 22], 'VariableNames', {'Names', 'Ages'});
mergedT = outerjoin(T, T2, 'Keys', 'Names');
This will create a new table `mergedT` where all information relevant to the specified keys is consolidated.
Handling Missing Data
Handling missing data effectively is an essential skill in data analysis. You can use MATLAB functions to manage inconsistencies. For instance, to remove rows containing any missing values:
T = rmmissing(T);
This function ensures that subsequent analysis is not skewed by incomplete data.
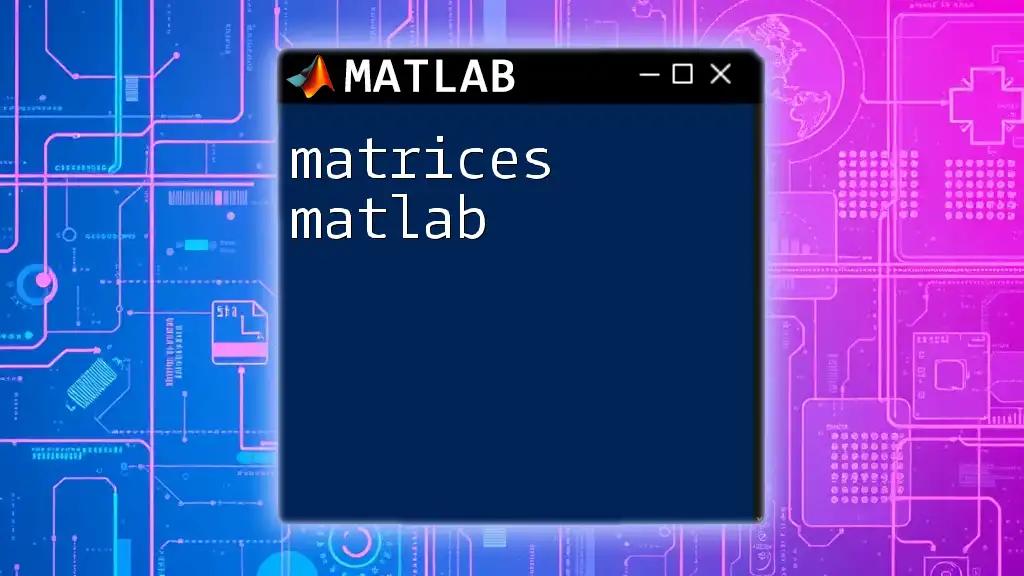
Best Practices for Using Tables in MATLAB
Naming Conventions
Using clear and concise naming conventions for your table variables enhances readability. This practice helps you and others understand the data without having to decipher ambiguous names.
Documentation and Comments
Adding comments to your code increases its maintainability. Clear documentation explaining your choice of methods and parameters can significantly help anyone reading the code, including your future self.
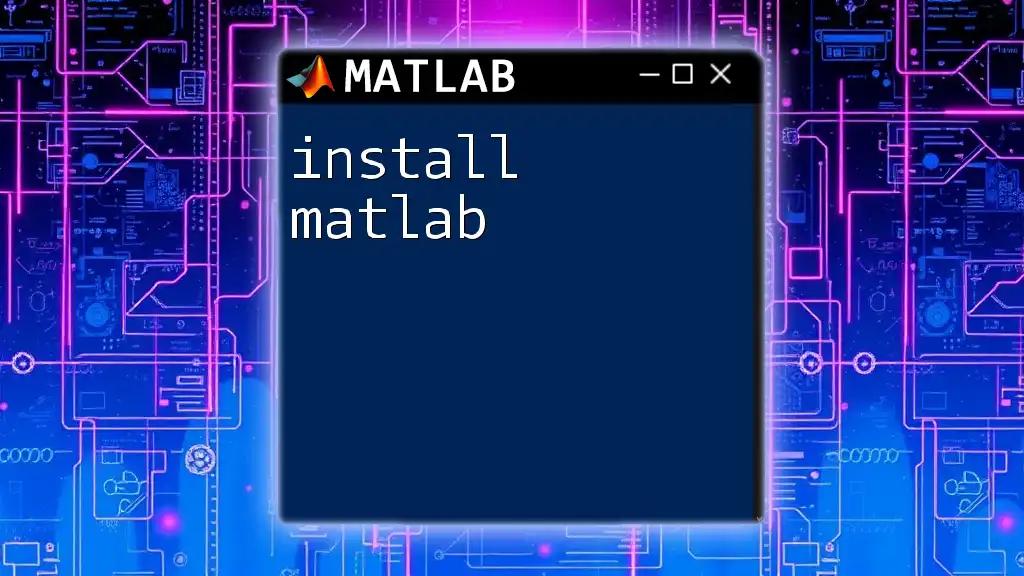
Conclusion
Tables in MATLAB are a robust option for organizing and analyzing data. With the ability to create, manipulate, and summarize data efficiently, they enhance productivity and clarity in data projects. Experimenting with the techniques outlined here will empower you to harness the full potential of tables in MATLAB, making your data analysis tasks more manageable and insightful.
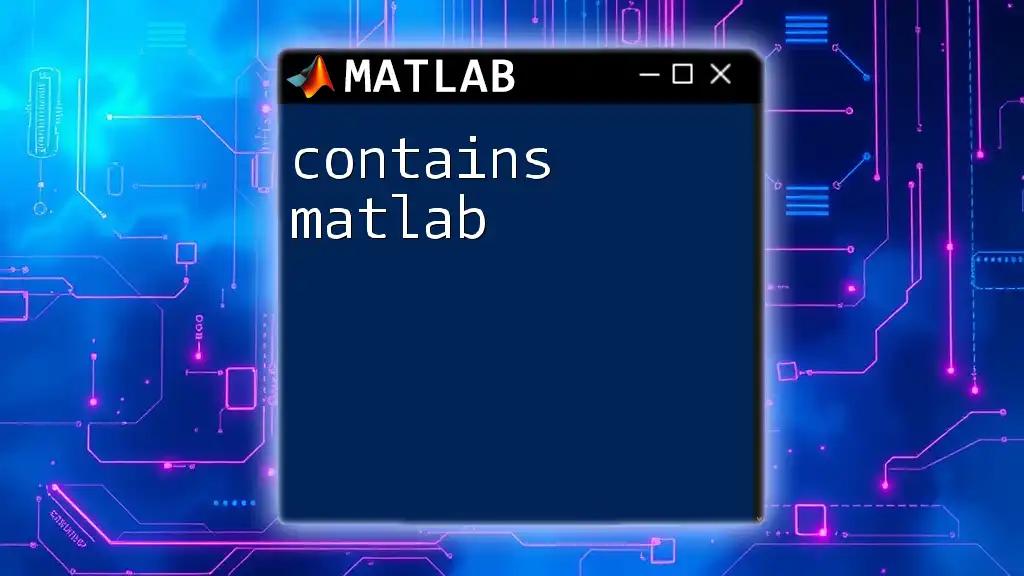
Additional Resources
For further reading, check out the official MATLAB documentation on tables. Participating in community forums and following tutorials will deepen your understanding and expand your skill set regarding tables in MATLAB.
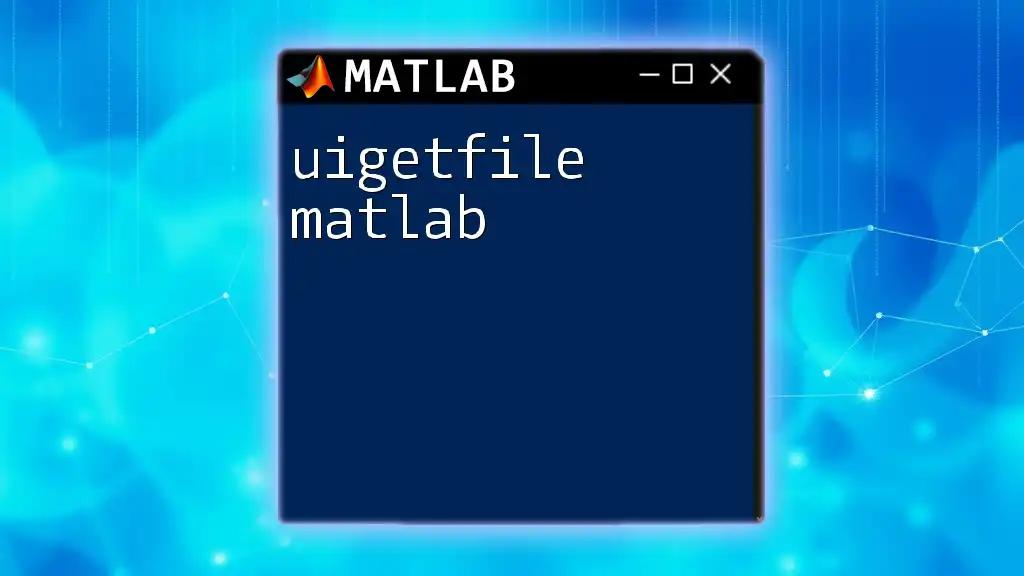
Call to Action
If you found this guide helpful, be sure to subscribe for more informative posts about MATLAB tips and tricks! We’d love to hear your experiences and any questions you might have about using tables in MATLAB in the comments section below.