`vpasolve` in MATLAB is a powerful function used to find numerical solutions to equations and systems of equations, allowing for higher precision results. Here’s a basic example of using `vpasolve` to solve the equation \( x^2 - 4 = 0 \):
syms x;
solution = vpasolve(x^2 - 4, x);
disp(solution);
Introduction to vpasolve
vpasolve is an essential function in MATLAB designed to solve equations numerically when traditional symbolic methods fall short. This makes it an invaluable tool in the arsenal of engineers, scientists, and researchers who often encounter complex equations that are difficult or impossible to solve analytically.
Importance of vpasolve in MATLAB
The vpasolve function is not just another tool; it fills a vital niche within MATLAB's broader technical computing environment. By providing approximate solutions to equations, it enables users to work with real-world problems efficiently. From optimization scenarios to engineering designs, vpasolve can help generate meaningful results where precise analytical solutions are elusive.
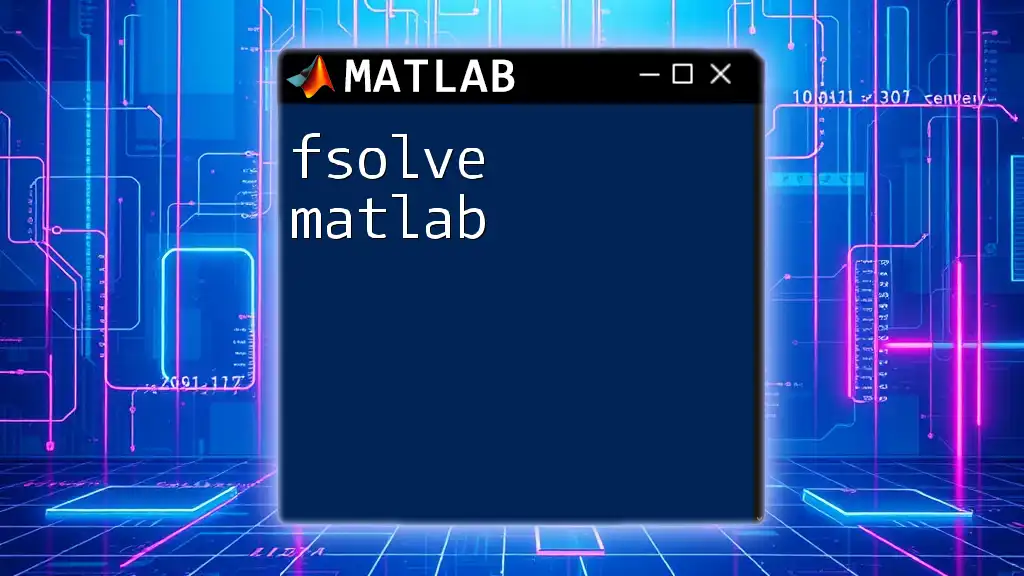
Understanding the Basics
What is Numerical Solving?
Numerical solving is a calculus-based approach that focuses on finding approximate solutions to mathematical problems that may not yield straightforward analytic answers. It contrasts with symbolic solving, where equations are manipulated algebraically to arrive at exact forms.
Key Concepts Behind vpasolve
The vpasolve function is instrumental in finding the roots of an equation by iteratively refining guess values until a sufficient degree of accuracy is achieved. It's essential to recognize that while solve provides exact solutions symbolically, vpasolve is tailored for approximations through numerical methods.
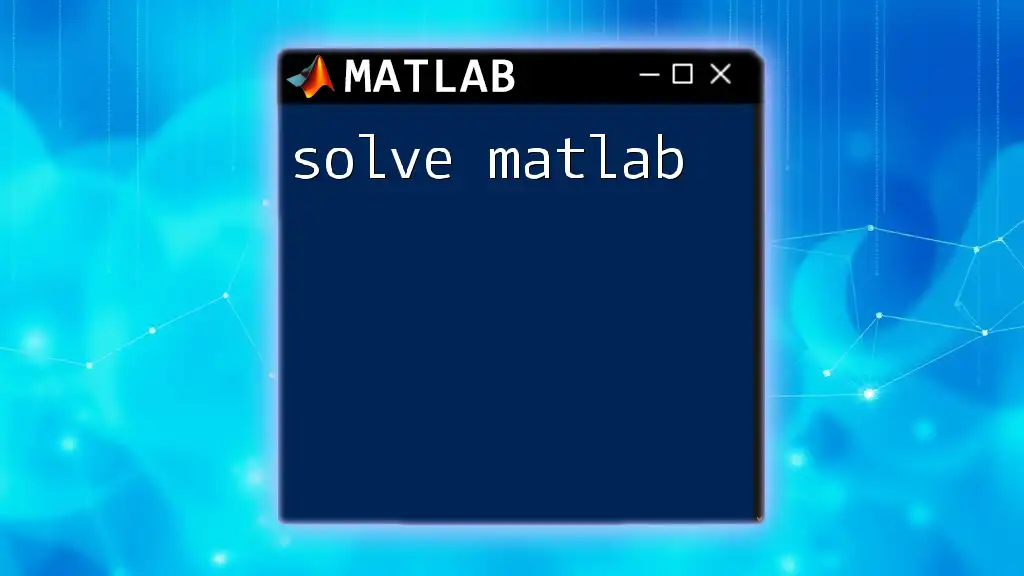
Syntax of vpasolve
Basic Syntax
To utilize the vpasolve function, users follow a simple syntax:
x = vpasolve(equation, variable)
This format highlights the essential elements required for solving problems with vpasolve.
Parameters of vpasolve
- Equation: Represents the mathematical expression you wish to solve.
- Variable: Denotes the specific variable you aim to solve for.
- Initial Guess: By offering an initial guess, you can significantly enhance the convergence speed towards the solution.
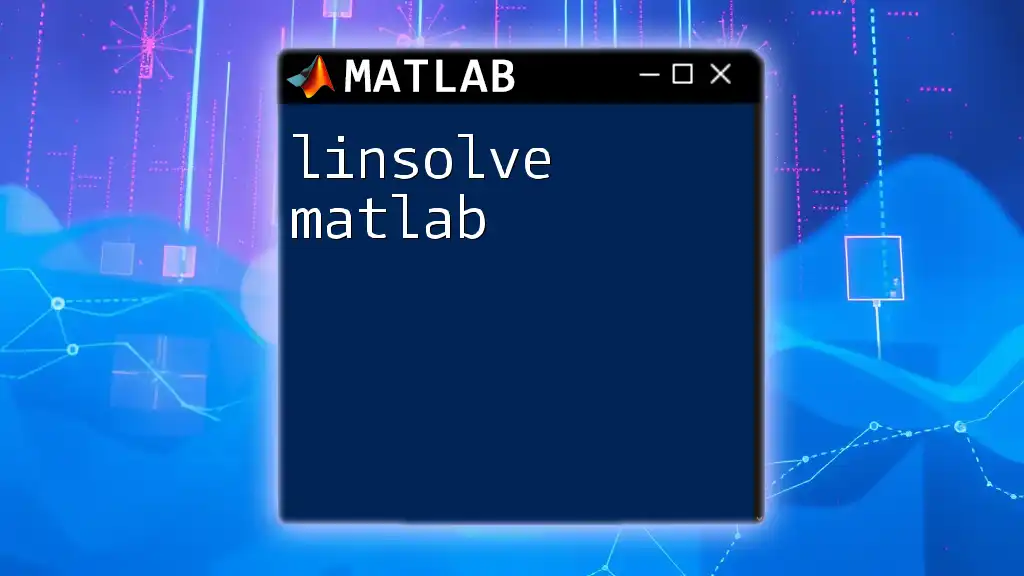
Examples of Using vpasolve
Example 1: Solving a Simple Equation
Problem Statement: Let’s solve the quadratic equation \( x^2 - 4 = 0 \).
MATLAB Code Example:
syms x;
eq = x^2 - 4 == 0;
solution = vpasolve(eq, x);
disp(solution);
Explanation of the Code: This code snippet begins by declaring a symbolic variable x. Next, we define a simple equation by equating \( x^2 - 4 \) to zero. Finally, we invoke vpasolve to find the numerical value of x satisfying the equation, then display the solution.
Example 2: Solving Trigonometric Equations
Problem Statement: Let’s tackle the equation involving trigonometric functions, \( \sin(x) - 0.5 = 0 \).
MATLAB Code Example:
syms x;
eq = sin(x) - 0.5 == 0;
solution = vpasolve(eq, x);
disp(solution);
Explanation of the Code: In this example, we once again define x symbolically. The equation is defined by subtracting 0.5 from \(\sin(x)\) and setting it to zero. vpasolve then helps find the values of x where the sine function equals 0.5.
Example 3: Multiple Solutions
Problem Statement: Let’s explore finding multiple roots for the cubic equation \( x^3 - 3*x + 2 = 0 \).
MATLAB Code Example:
syms x;
eq = x^3 - 3*x + 2 == 0;
solutions = vpasolve(eq, x);
disp(solutions);
Handling Multiple Results: vpasolve can return multiple solutions. Here, the code identifies the different values of x that satisfy the cubic equation, which is essential for a comprehensive understanding of polynomial roots.
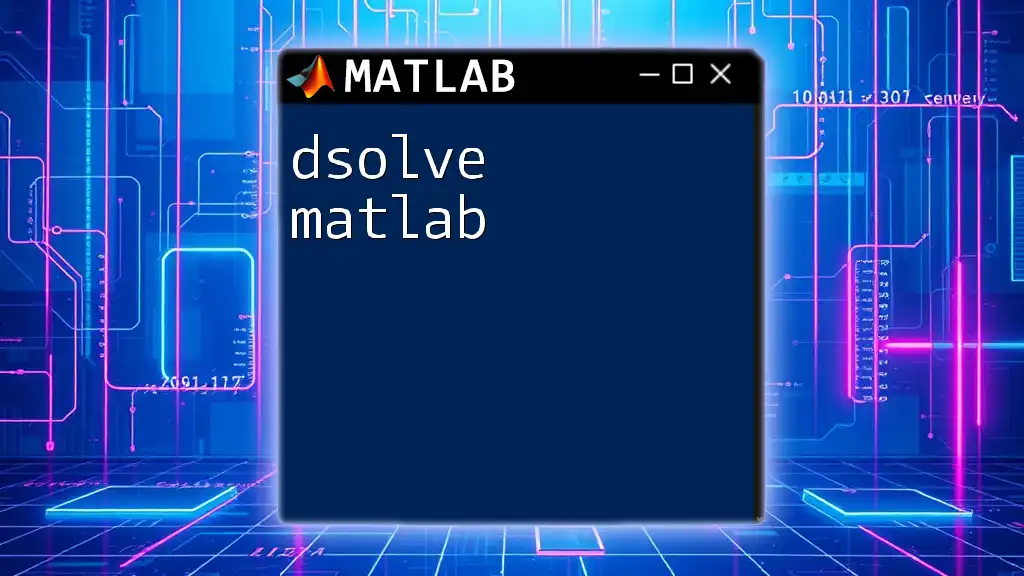
Advanced Usage of vpasolve
Providing Initial Guesses
Offering an initial guess can drastically improve the efficiency of vpasolve. This helps the algorithm to converge to the correct point more quickly.
solution = vpasolve(eq, x, 1); % Providing an initial guess of 1
Limiting Solutions
To restrict the solution search range, specify an interval:
solution = vpasolve(eq, x, [0, 2]); % Restricting search between 0 and 2
This is particularly useful when the potential solutions are known to lie within specific bounds.
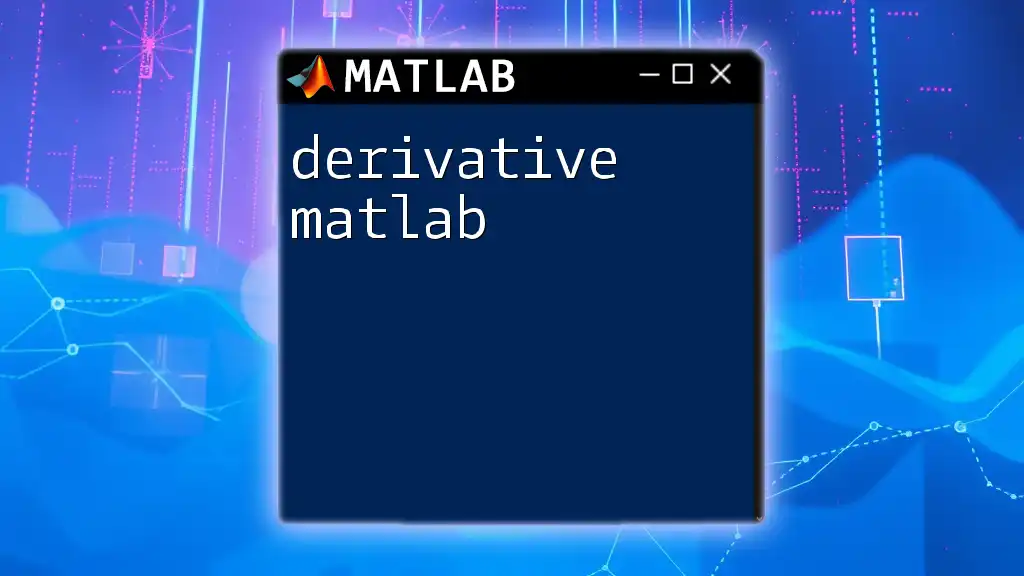
Common Issues and Troubleshooting
Non-Convergence Issues
One common frustration users may face with vpasolve is non-convergence. Such instances often arise from poorly chosen initial guesses or particularly complex equations. To mitigate this, it’s essential to experiment with different initial values and modify your approach to better guide the solver.
Precision Control
For applications needing higher accuracy, you can adjust the precision settings in vpasolve. For example:
vpasolve(eq, x, 'Precision', 50);
This command increases the precision of the solution, thereby making it more reliable for critical computations.
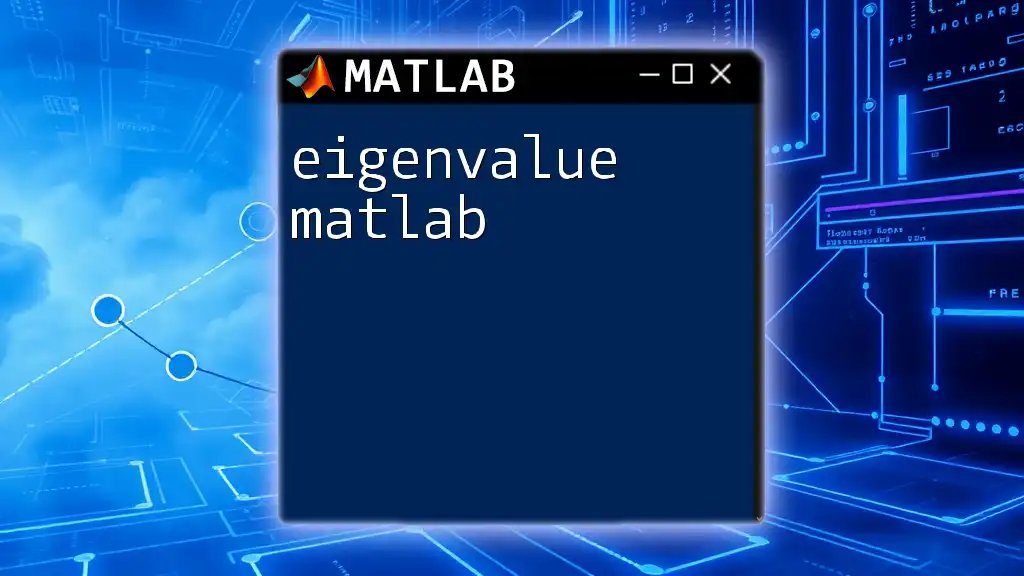
Conclusion
In summary, the vpasolve function in MATLAB stands out as a vital tool for tackling numerical equations across various domains. By mastering this function, users can access more efficient ways to solve complex equations, paving the way to deeper insights and practical solutions.
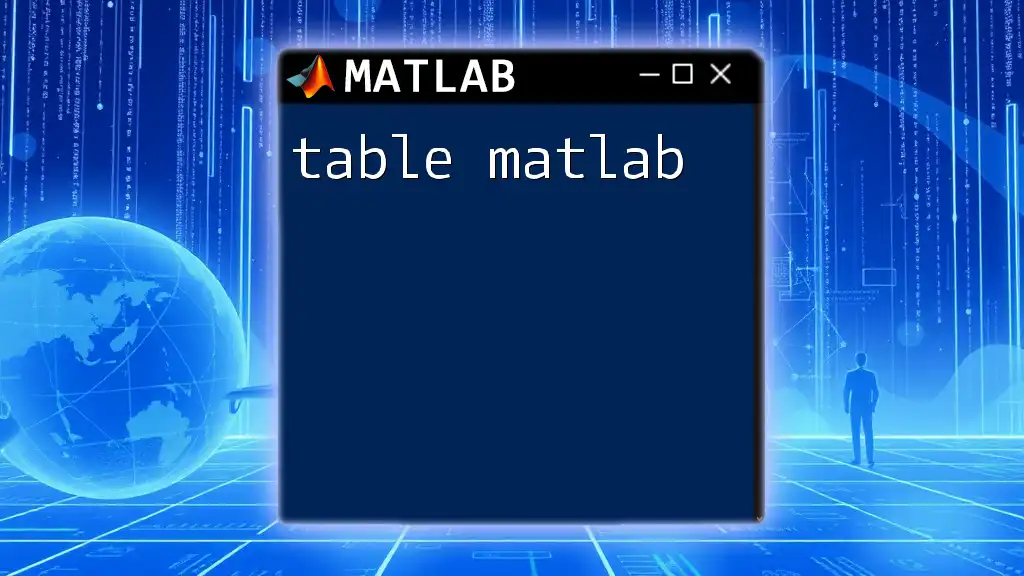
Further Resources
For readers keen on expanding their MATLAB skill set, consider exploring recommended books and online courses focused on MATLAB programming and numerical methods. Additionally, linking to the official MATLAB documentation for vpasolve and related functions can provide further guidance and thorough explanations.
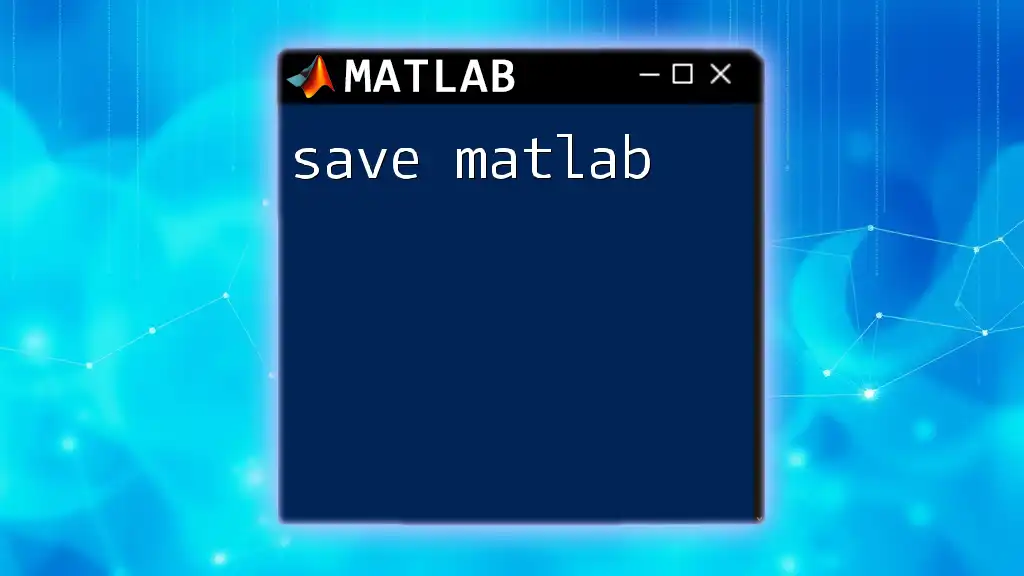
Call to Action
Join our classes to dive deeper into MATLAB commands and enhance your proficiency in solving equations efficiently with tools like vpasolve. Experience hands-on training that guides you through practical applications, ensuring you become adept at using MATLAB for your projects.