In MATLAB, a table is a convenient data type for storing and manipulating tabular data, where each column can contain different types of variables.
% Create a table with four variables: Name, Age, Height, and Weight
T = table({'John'; 'Jane'; 'Jim'}, [25; 30; 28], [5.6; 5.4; 5.9], [160; 150; 180], ...
'VariableNames', {'Name', 'Age', 'Height', 'Weight'});
What is a Table?
A table in MATLAB is a versatile data structure that allows for the storage of heterogeneous data types in a single entity. Similar to a spreadsheet, tables organize data into rows and columns, where each column can contain different types of data. This structure is particularly powerful for managing and analyzing tabular data, making it a favored choice for data scientists and engineers.
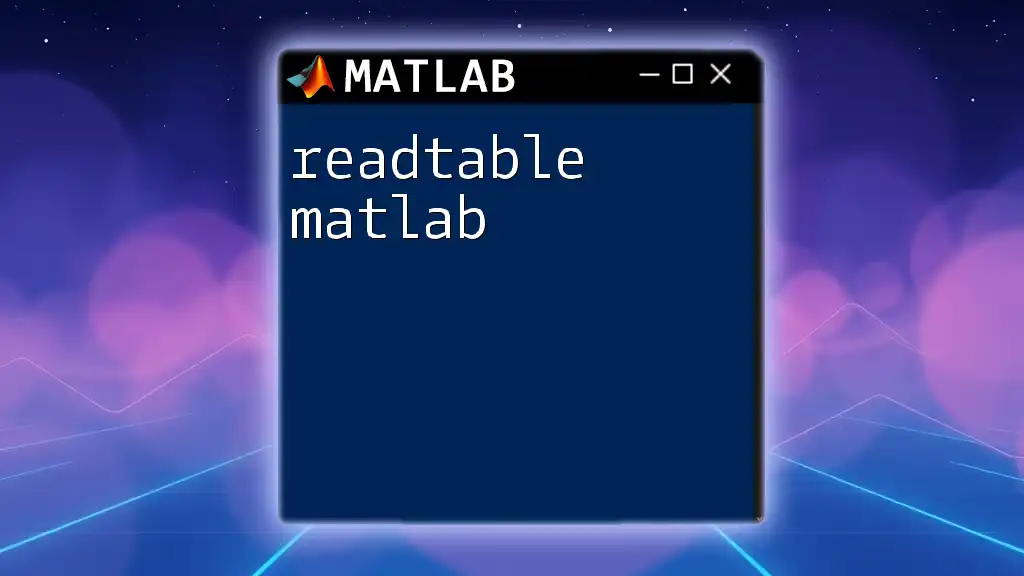
When to Use Tables?
Tables should be your go-to choice in several scenarios:
- When Handling Complex Data: If your dataset includes variables of different types (e.g., text, numbers, logical values), tables offer a more suitable organization than traditional matrices or arrays.
- For Enhanced Data Semantics: Tables come equipped with variable names, making it easier to refer to data without relying solely on numerical indices.
- For Streamlined Data Analysis: MATLAB provides a rich set of functions that leverage tables, facilitating easier analysis and manipulation of data.
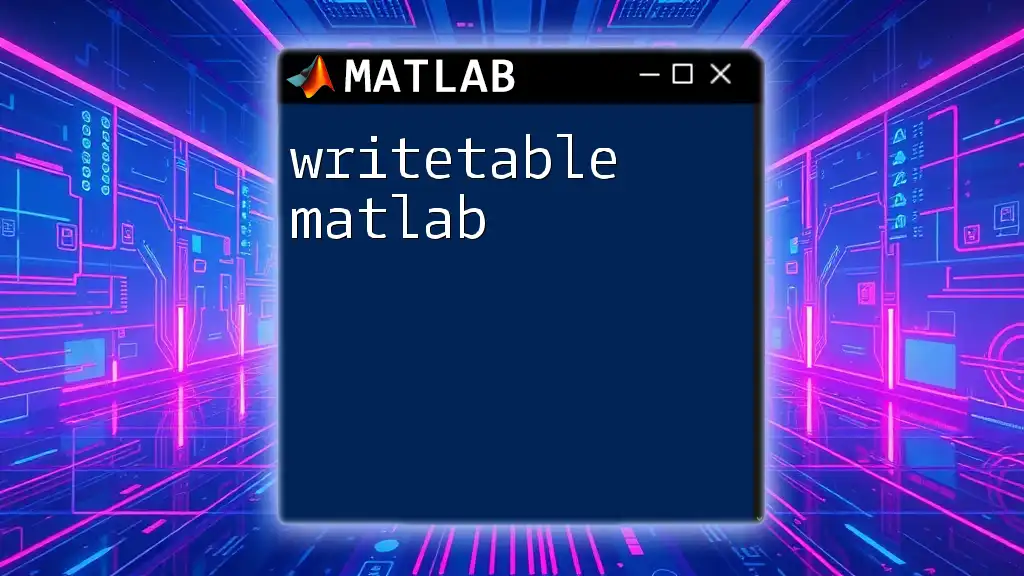
Creating Tables
How to Create a Table
Creating a table in MATLAB is straightforward using the `table` function. For instance:
% Creating a simple table
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 35];
T = table(Names, Ages);
disp(T);
The `table` function combines the given cell arrays and numeric arrays into a single table variable `T`.
Importing Data into a Table
You can also import external data directly into a table format using the `readtable` function. This method is ideal for loading data from files, such as CSV.
T = readtable('data.csv');
disp(T);
Creating Tables from Different Data Types
Tables can effectively store diverse data types. For example, you can create a table that includes strings, numeric values, and boolean flags:
Names = {'Alice'; 'Bob'};
Scores = [88; 92];
Attendance = [true; false];
T = table(Names, Scores, Attendance);
This flexible structure allows the seamless integration of various data types.
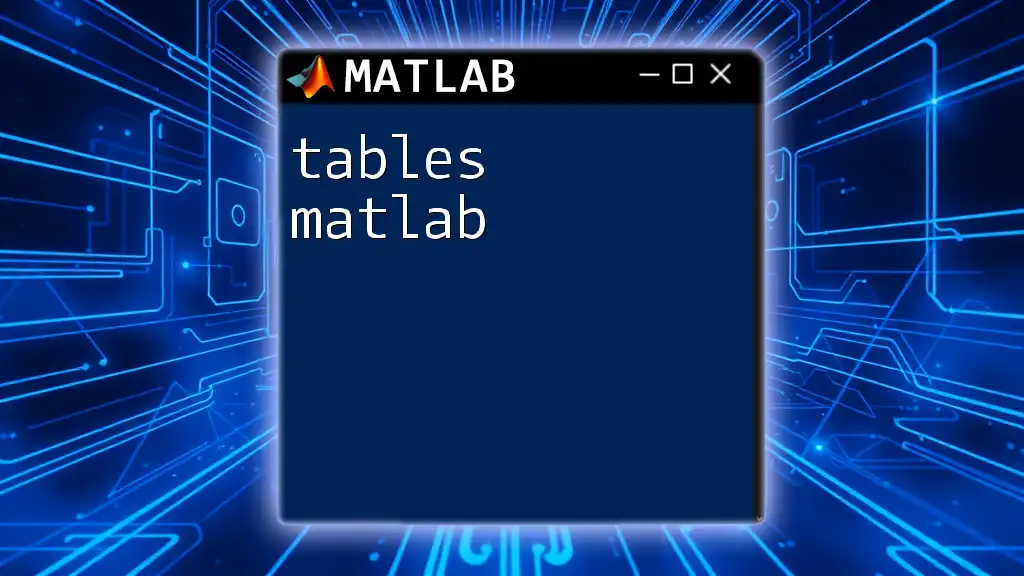
Manipulating Tables
Accessing Table Data
Accessing data within a table is a breeze. You can reference specific rows and columns easily, enhancing usability.
% Accessing the first row
firstRow = T(1, :);
This line retrieves the entire first row of the table `T`.
Adding and Removing Rows/Columns
Adding new rows or columns can be done dynamically. For instance, to add a new column called "Height":
T.Height = [1.65; 1.80];
To remove a column, you can simply set it to empty:
T.Height = [];
Renaming Table Variables
MATLAB makes it easy to rename your table variables for better clarity. Use the `Properties.VariableNames` property for renaming:
T.Properties.VariableNames{'Scores'} = 'TestScores';
This transforms the title of the "Scores" column into "TestScores," helping maintain clear semantics.
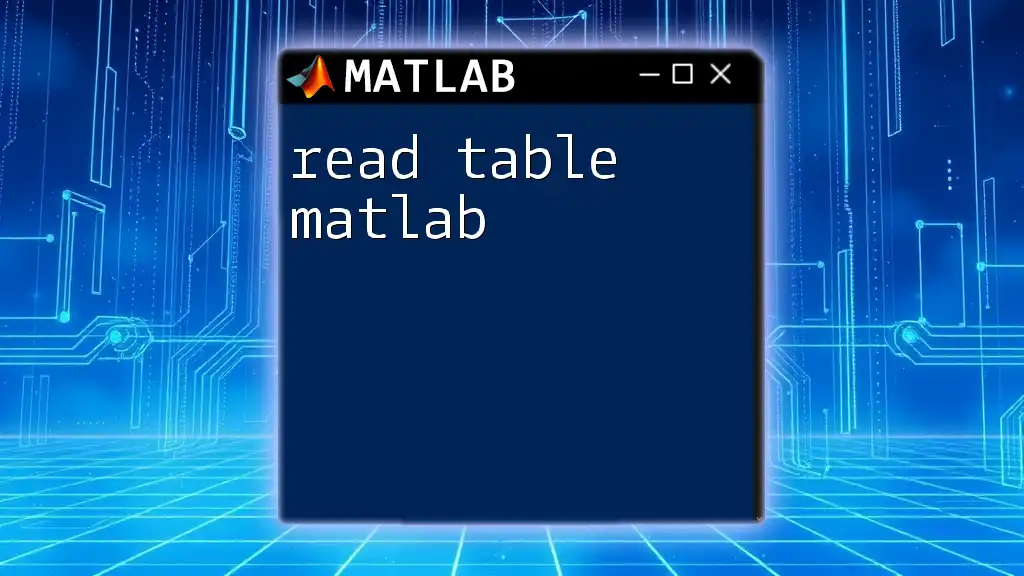
Analyzing and Summarizing Tables
Statistical Analysis with Tables
You can perform statistical calculations directly on table variables with built-in functions like `mean`, `std`, or `var`.
averageScore = mean(T.TestScores);
This code snippet computes the mean of the "TestScores" column, allowing for quick analysis.
Grouping Data
Grouping data and summarizing statistics is simple with functions like `groupsummary`. This tool helps you aggregate data based on specific criteria:
summaryT = groupsummary(T, 'Names', 'mean', 'TestScores');
This example computes the mean test score for each name in the table.
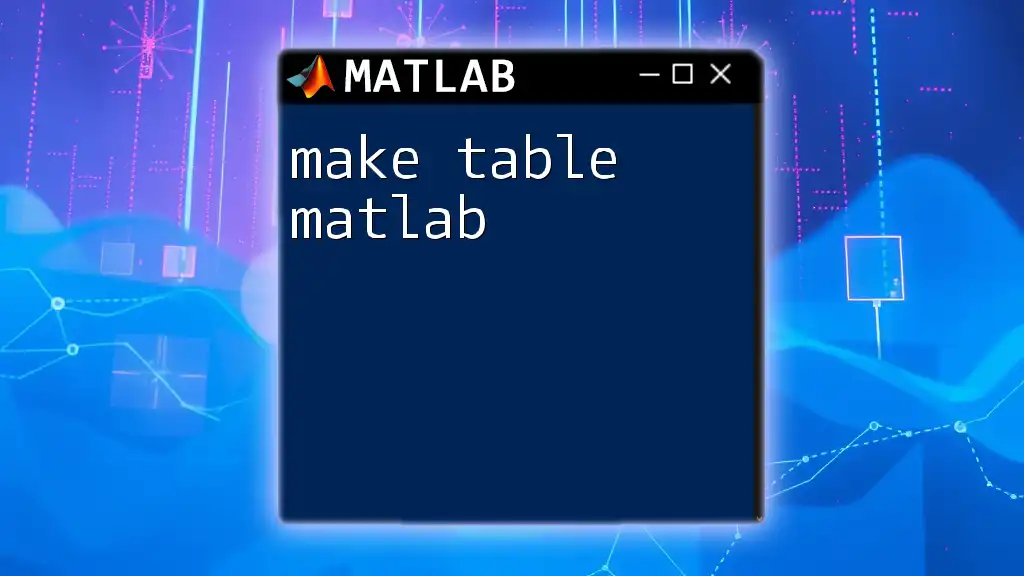
Advanced Table Manipulation
Joining Tables
Combining multiple tables is highly effective using the `innerjoin` or `outerjoin` functions. This allows you to create enriched datasets by merging based on shared columns.
T2 = table({'Alice'; 'Bob'}, [3; 4], 'VariableNames', {'Names', 'Courses'});
JoinedTable = innerjoin(T, T2, 'Keys', 'Names');
Here, the two tables are joined based on the "Names" column, yielding a single table with combined information.
Sorting Tables
Sorting table data enhances readability and analysis. You can easily sort rows based on one or more columns using `sortrows`.
SortedTable = sortrows(T, 'Ages', 'ascend');
This snippet sorts the rows of `T` in ascending order based on the "Ages" column.
Finding Data in Tables
To efficiently find and retrieve specific data, you can leverage logical indexing. For example, to locate rows where "Ages" is greater than 28:
foundRows = T(T.Ages > 28, :);
This command retrieves all rows corresponding to that condition, enabling powerful data retrieval.
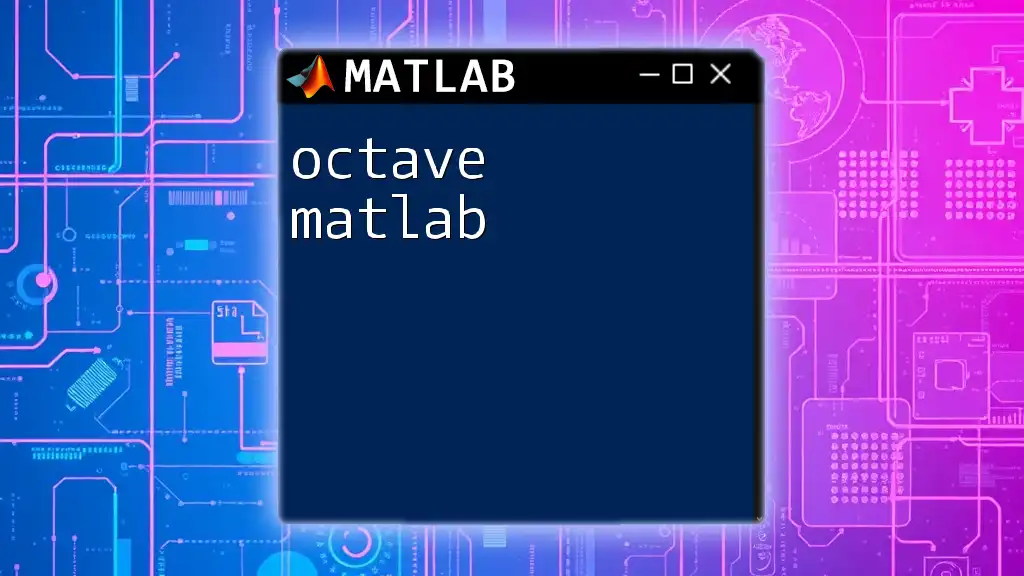
Visualizing Table Data
Tables integrate seamlessly with MATLAB’s plotting functions, allowing you to visualize your data effectively. Whether you're creating plots or bar graphs, you can easily use the table data:
bar(T.Names, T.TestScores);
This command generates a bar graph comparing test scores by name, offering a visual representation of your data.
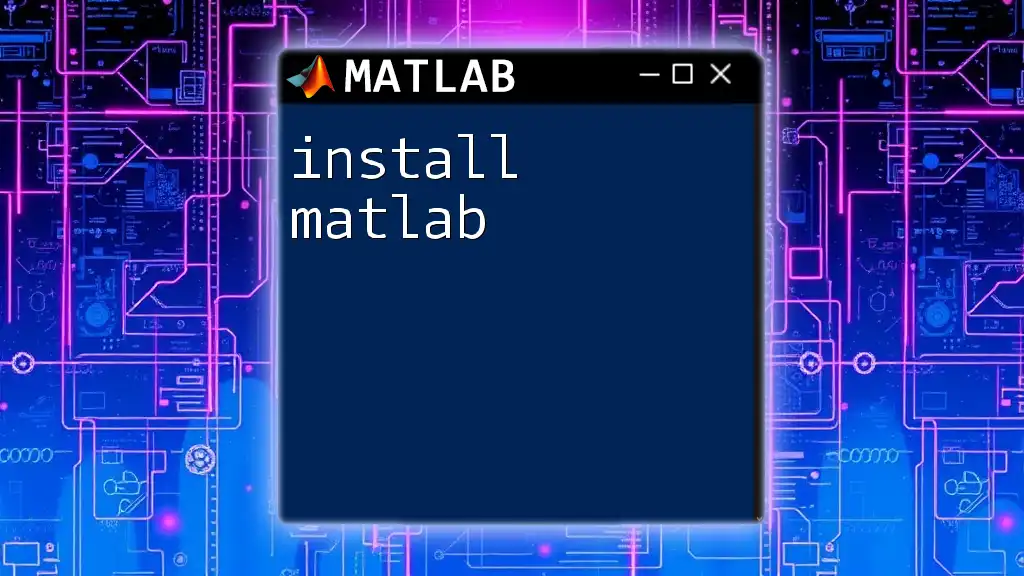
Exporting and Saving Tables
Once you’ve manipulated your data, you might want to export it. The `writetable` function allows you to save your table to various formats, such as CSV or Excel.
writetable(T, 'output.csv');
This line efficiently writes the table `T` to a file named "output.csv", maintaining all your data integrity.
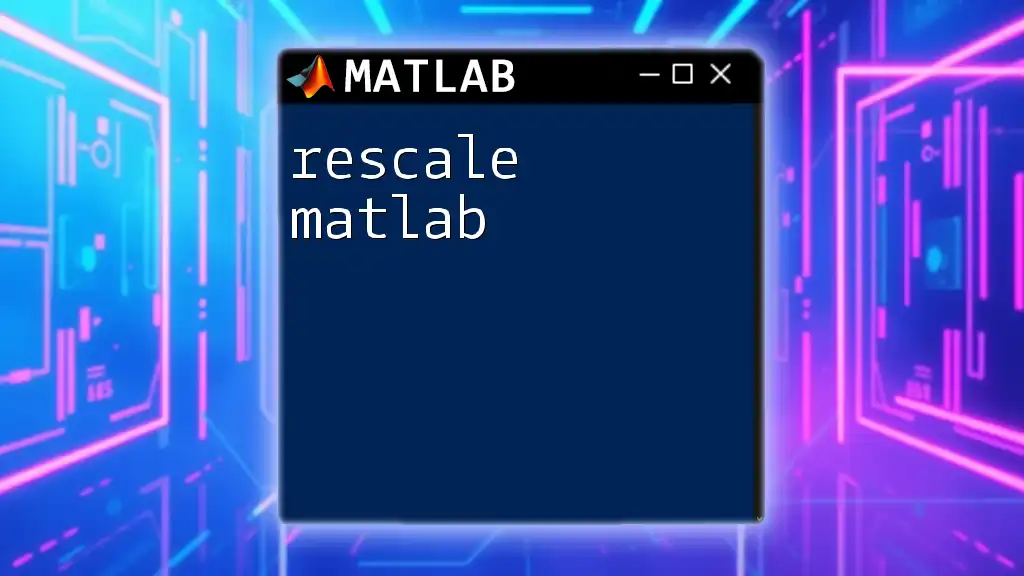
Conclusion
In summary, understanding how to use tables in MATLAB significantly enhances your ability to manage and analyze complex datasets. With tables, you can easily create, manipulate, and interpret data, providing a robust tool for data analysis and visualization.
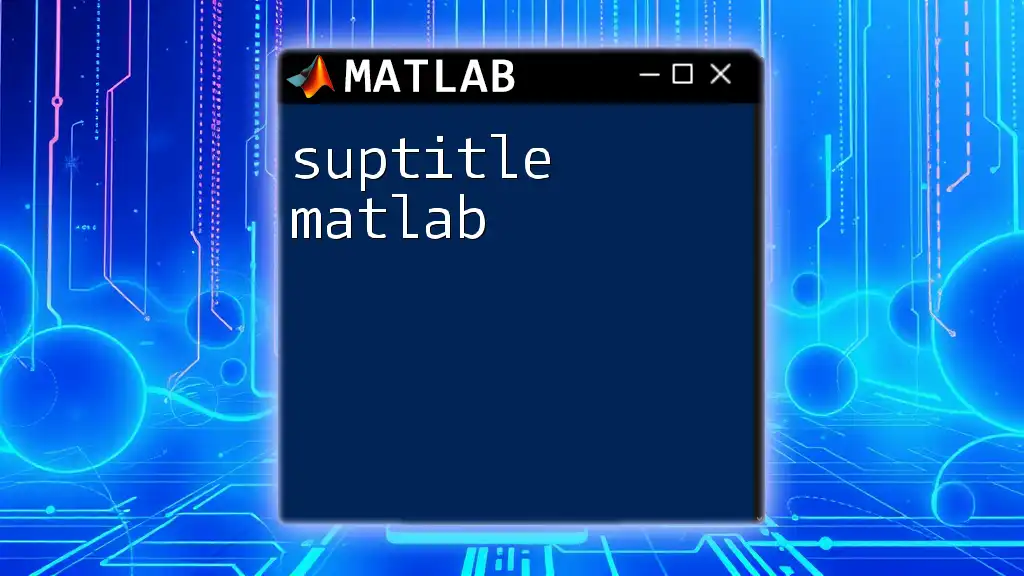
FAQs About Tables in MATLAB
What is the difference between a table and an array?
Tables can store heterogeneous data types and are easier to interpret because of their labeled columns. In contrast, arrays are strictly numerical and require careful indexing.
How to handle missing data in tables?
MATLAB provides various functions to identify and manage missing data, such as `rmmissing` (to remove rows with missing data) and logical operators for filtering.
Tips for Effective Table Management:
- Organize your data clearly with informative variable names.
- Use grouping and summarization techniques for efficient data analysis.
- Always verify your methods when merging or sorting data to ensure data integrity.