You can export a variety of algorithms from MATLAB, including neural networks, decision trees, and support vector machines, by utilizing the `fitctree` function for classification trees as an example.
Here's a quick code snippet demonstrating how to export a decision tree model:
% Sample data
X = [1 2; 2 3; 3 3; 5 4; 5 5]; % Features
Y = [0; 0; 1; 1; 1]; % Target class
% Train a decision tree
treeModel = fitctree(X, Y);
% Exporting the trained model
exportedModel = saveLearnerForCoder(treeModel, 'TreeModel');
Understanding Algorithm Exportation
What Does It Mean to Export an Algorithm?
Exporting an algorithm generally refers to the process of converting a developed algorithm into a format that can be used in different environments or applications. This can involve generating related code that can be run in various programming environments or creating stand-alone components ready for deployment. For many developers and researchers, knowing what algorithms can I export from MATLAB is critical, particularly when collaboration with software applications or platforms that use different programming languages is essential.
Why Use MATLAB for Algorithm Development?
MATLAB is a robust platform favored for algorithm development primarily due to its:
- Strong Mathematical Foundation: MATLAB is built for numerical computations, making it easy to implement complex algorithms.
- User-Friendly Interface: The visual environment of MATLAB allows for intuitive design and debugging of algorithms.
- Extensive Libraries: MATLAB provides various built-in functions and toolboxes tailored for specific applications such as machine learning, optimization, and signal processing.
Industries such as finance, academia, aerospace, and automotive frequently utilize MATLAB to develop and export algorithms due to its versatility and strength.
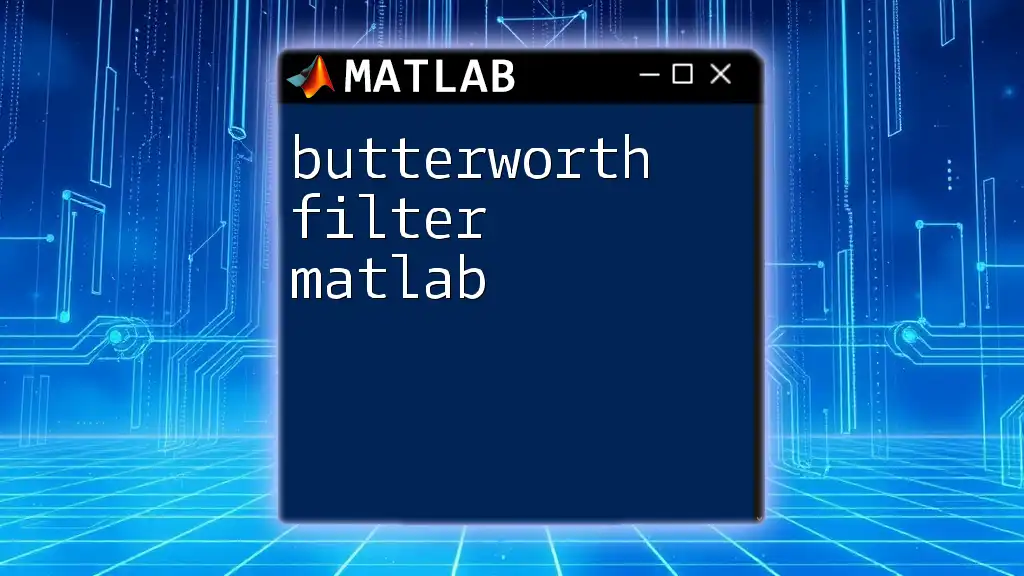
Common Algorithms That Can Be Exported from MATLAB
Machine Learning Algorithms
Overview of Machine Learning in MATLAB
Machine learning has become an integral part of many applications, and MATLAB provides an easy way to develop and export these algorithms. The platform includes multiple built-in functions and tools for various machine-learning tasks.
Examples of Exportable Machine Learning Algorithms
-
K-Nearest Neighbors: This is a simple, yet effective, classification algorithm.
- Explanation: The KNN algorithm classifies data points based on the distance to their nearest neighbors.
- Use Cases: Commonly used in pattern recognition and recommendations.
- Code Snippet:
% Load data load fisheriris % Prepare data for training trainingData = meas(1:100, :); trainingLabels = species(1:100); % Fit model mdl = fitcknn(trainingData, trainingLabels); % Export model to C code codegen -config:lib mdl
-
Support Vector Machines: SVM is a powerful classifier used widely for its effectiveness in high-dimensional spaces.
- Explanation: It works by finding the optimal hyperplane that separates classes in the feature space.
- Use Cases: Effective for email classification, image recognition, and more.
- Code Snippet:
% Load data load fisheriris % Prepare data for training trainingData = meas(1:100, :); trainingLabels = species(1:100); % Fit model mdl = fitcsvm(trainingData, trainingLabels); % Export model codegen -config:lib mdl
-
Decision Trees: These are used for classification and regression by forming a tree-like model.
- Explanation: Decision trees split the data into subsets based on feature values, leading to a decision outcome.
- Use Cases: Common in credit scoring and medical diagnosis.
- Code Snippet:
% Load data load fisheriris % Fit model mdl = fitctree(meas(:, 1:3), species); % Export model codegen -config:lib mdl
Optimization Algorithms
Overview of Optimization Techniques
Optimization algorithms are crucial for finding the best solution from a set of available alternatives under given constraints. MATLAB excels in providing tools to define and solve optimization problems efficiently.
Examples of Exportable Optimization Algorithms
-
Linear Programming: This optimization technique is used for maximizing or minimizing a linear objective function under linear constraints.
- Explanation: Typically involves functions that represent profits or costs subject to constraints defined by inequalities.
- Use Cases: Supply chain optimization and resource allocation problems.
- Code Snippet:
% Define the optimization problem f = [-5 -4]; % Coefficients for the objective function A = [1 2; 4 1]; % Coefficients for inequalities b = [8; 12]; % Right-hand side of inequalities % Solve using linprog x = linprog(f, A, b);
-
Genetic Algorithms: A heuristic optimization approach that mimics the process of natural selection.
- Explanation: Genetic algorithms evolve a population of candidate solutions over several generations.
- Use Cases: Suitable in complex optimization problems in engineering design and artificial intelligence.
- Code Snippet:
% Define the fitness function fitnessFcn = @(x) x(1)^2 + x(2)^2; % Minimization problem % Set options for the genetic algorithm options = optimoptions('ga', 'Display', 'iter'); % Solve using ga [x, fval] = ga(fitnessFcn, 2, [], [], [], [], [-10 -10], [10 10], [], options);
Signal Processing Algorithms
Overview of Signal Processing in MATLAB
Signal processing involves the analysis and manipulation of signals. MATLAB’s capabilities in this area are extensive, offering robust tools for a variety of processing tasks.
Examples of Exportable Signal Processing Algorithms
-
Fourier Transform: This algorithm transforms a signal from the time domain to the frequency domain.
- Explanation: It decomposes a function into its constituent frequencies, which is useful for filtering or signal analysis.
- Use Cases: Telecommunications, audio processing, and image analysis.
- Code Snippet:
% Define a signal t = 0:0.001:1; % 1 second duration x = sin(2 * pi * 50 * t) + randn(size(t)); % Signal with noise % Apply Fourier transform Y = fft(x); % Export results codegen -config:lib Y
-
Wavelet Transform: This algorithm allows time-frequency analysis of signals.
- Explanation: Wavelets are used to represent data or functions that have localized discontinuities.
- Use Cases: Image compression and feature extraction.
- Code Snippet:
% Load signal data load sampleSignal.mat; % Assuming sampleSignal is your signal % Perform wavelet transform [C, L] = wavedec(sampleSignal, 5, 'db1'); % 5 levels with Daubechies wavelets % Export the coefficients codegen -config:lib C
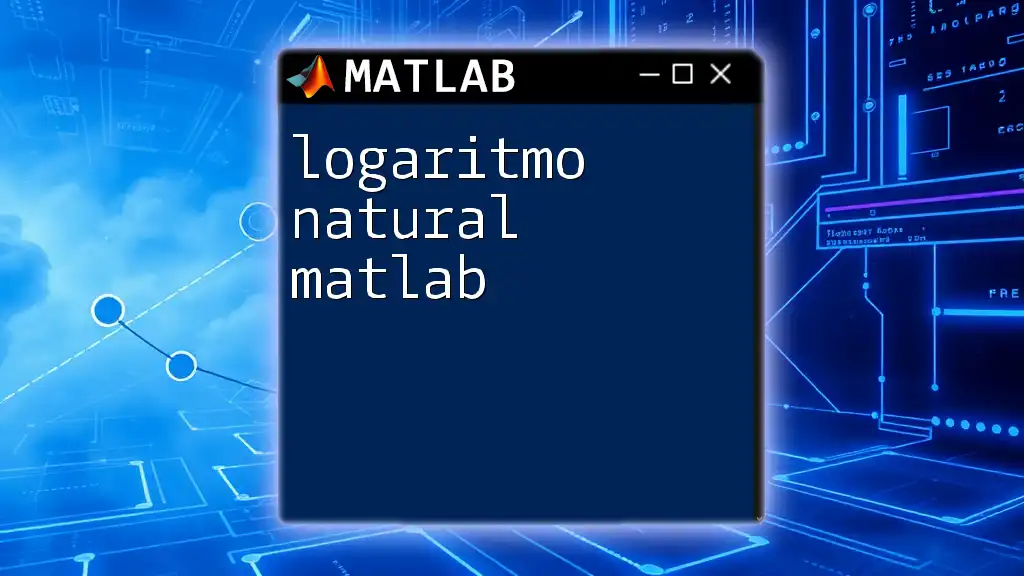
Exporting Algorithms: How to Get Started
Using MATLAB Coder
Introduction to MATLAB Coder
MATLAB Coder is a tool that allows you to automatically generate C or C++ code from MATLAB code. This can be particularly useful when you want to deploy your algorithms in embedded systems or need performance optimization.
Step-by-Step Guide on Exporting Code
- Write Your MATLAB Code: Ensure that your code is structured and adheres to best practices.
- Open MATLAB Coder: Navigate to the app from the MATLAB environment.
- Select a File or Function: Choose the function you want to export.
- Verify Code: Check for any compatibility issues or errors.
- Generate Code: Choose the output folder and generate code.
Example Export Process:
% Function to export
function y = myFunction(x)
y = x.^2;
end
% Run MATLAB Coder
codegen myFunction -o myFunctionExported
Using Simulink for Algorithm Exportation
What is Simulink?
Simulink is an extension of MATLAB designed for modeling, simulating, and analyzing dynamic systems. It provides rich capabilities to represent algorithms graphically, particularly useful in control systems and other engineering applications.
Guide to Exporting Simulink Models
- Develop your model: Use blocks to create the desired algorithm flow.
- Open the Code Generation Tool: This can be accessed from the Simulink environment.
- Configure Settings: Choose the settings based on the desired output (e.g., C code).
- Generate Code: Click ‘Build’ to create the exported code.
Example Export of a Simulink Model:
% This is a pseudocode representation to highlight the flow
% Assume you have a model named myModel
sim('myModel'); % Simulate your model
slbuild('myModel'); % Trigger code generation
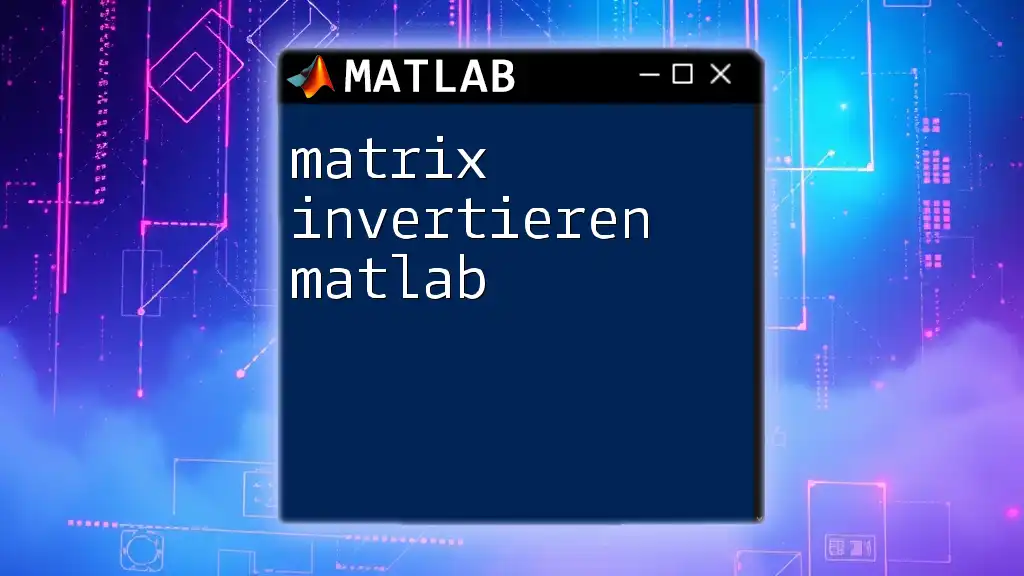
Best Practices for Exporting Algorithms
Optimize Code for Export
When exporting algorithms, efficiency and performance are paramount. During the coding phase, consider vectorization and preallocation where possible, as this enhances the speed of execution and subsequent exportation.
Testing and Validation
Prior to exporting, it’s imperative to test and validate your algorithms to ensure accuracy. Implement unit tests and perform regression testing to verify that the algorithm behaves as expected across different scenarios.
Documentation and Maintenance
Proper documentation is essential for future reference and maintenance. Include comments within your code and consider adding a README file that outlines the algorithm, usage instructions, and notes on potential limitations.
Example of a Simple Documentation Approach:
% myFunction - Squares the input
% Usage: y = myFunction(x)
% Input:
% x - Numeric value or array to be squared
% Output:
% y - Squared result
function y = myFunction(x)
y = x.^2; % Squares the input
end
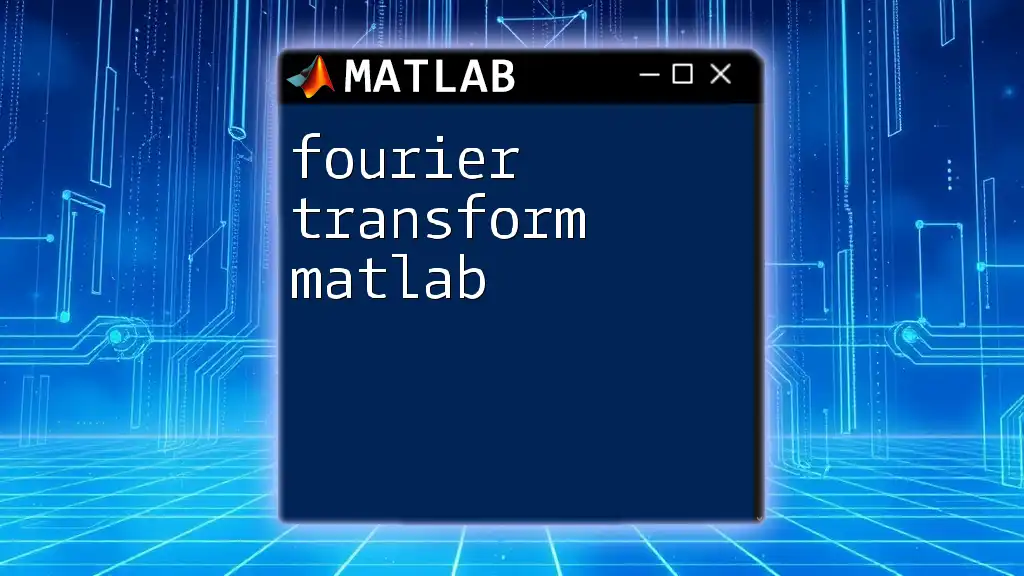
Use Cases for Exported Algorithms
Industry Applications
Exported algorithms have practical applications in many industries. For instance, in the healthcare sector, researchers export algorithms for predictive modeling in patient management systems, leading to improved patient outcomes.
Academic Research
Within academia, exported algorithms facilitate collaboration among researchers. By using standard programming languages, they can easily share and replicate results in studies involving complex mathematical models and simulations.
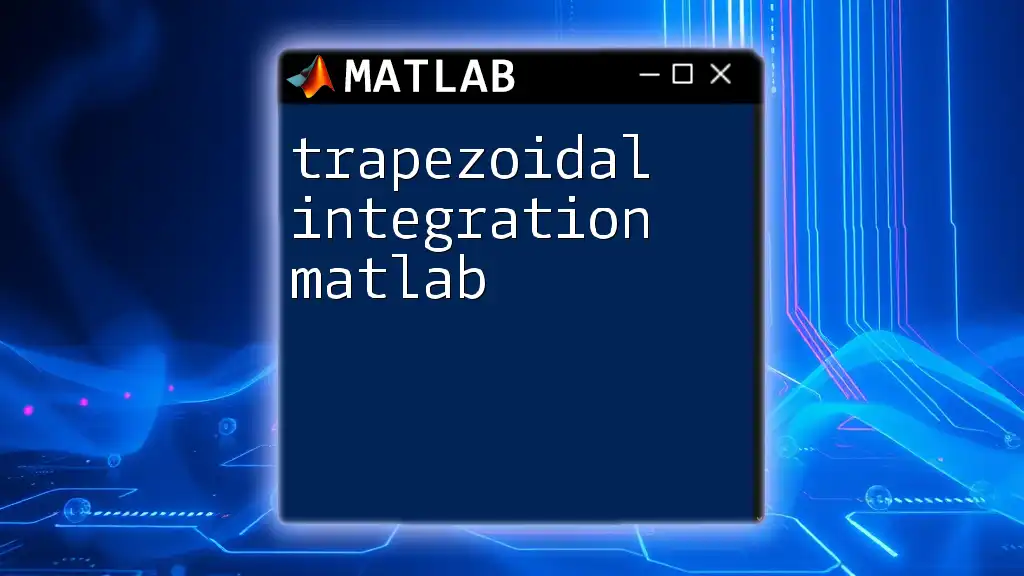
Conclusion
In summary, the question what algorithms can I export from MATLAB encompasses a diverse range of options across various domains including machine learning, optimization, and signal processing. Mastering the exporting process opens doors for collaboration and application in real-world scenarios, encouraging you to leverage MATLAB’s powerful features for efficient algorithm development.