A Butterworth filter in MATLAB is a type of signal processing filter characterized by a maximally flat frequency response in the passband, allowing for smooth filtering of signals.
% Design a Butterworth low-pass filter
fs = 1000; % Sampling frequency
fp = 100; % Passband frequency
fs2 = 150; % Stopband frequency
order = 4; % Order of the filter
% Calculate normalized frequencies
Wn = fp / (fs / 2);
% Design the Butterworth filter
[b, a] = butter(order, Wn, 'low');
% Apply the filter to a signal
filtered_signal = filter(b, a, input_signal);
Understanding Butterworth Filters
What is a Butterworth Filter?
A Butterworth filter is a type of signal processing filter that provides a frequency response with a smooth, maximally flat passband. Unlike other filters, the Butterworth filter is designed to have as flat a frequency response as possible within its passband. This characteristic makes it useful for applications where a smooth output is more desirable than a rapid roll-off.
Key characteristics of Butterworth filters include:
- Maximally flat frequency response in the passband.
- Gradual roll-off in the transition band, making it less aggressive than other filters.
- No ripples in the passband, creating a clean output signal.
Types of Butterworth Filters
Butterworth filters can be classified into the following types based on their frequency response:
- Low-pass Butterworth Filter: Allows signals with a frequency lower than a certain cut-off frequency to pass and attenuates frequencies higher than the cut-off.
- High-pass Butterworth Filter: Allows signals with frequencies higher than a specified cut-off frequency to pass, while attenuating frequencies below it.
- Band-pass Butterworth Filter: Permits frequencies within a certain range to pass while rejecting frequencies outside that range.
- Band-stop Butterworth Filter: Opposite of a band-pass filter; it eliminates frequencies within a certain range while allowing those outside that range to pass.
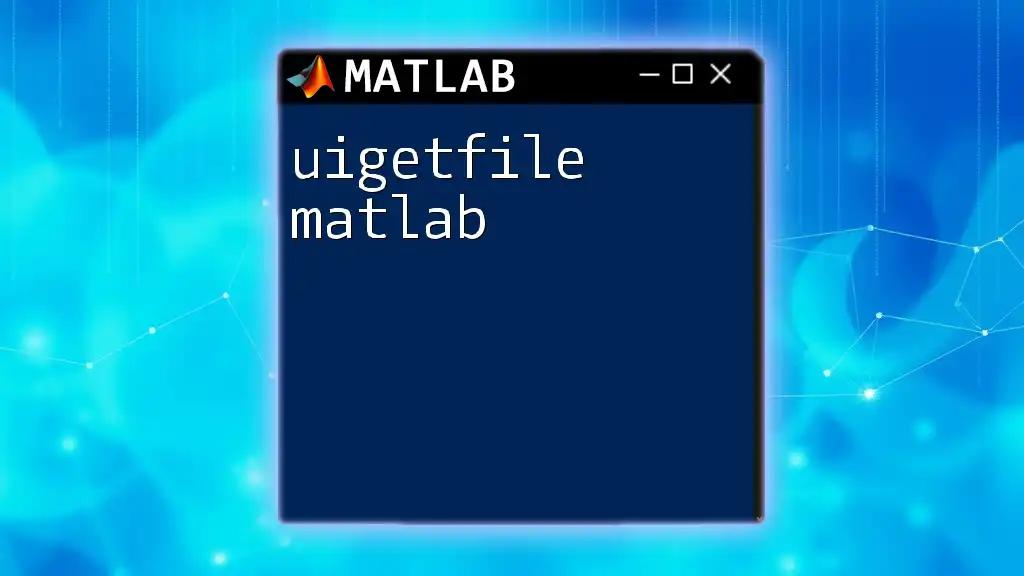
Theoretical Background
Mathematical Representation
Butterworth filters can be defined mathematically through their transfer function, which represents the relationship between the input and output of the filter in the frequency domain. The general transfer function for an n-th order Butterworth filter is given by:
\[ H(s) = \frac{1}{1 + \left(\frac{s}{\omega_c}\right)^{2n}} \]
where:
- \( s \) is the complex frequency variable.
- \( \omega_c \) is the cut-off frequency.
- \( n \) is the order of the filter.
Order of Butterworth Filters
The order of a Butterworth filter significantly influences its performance. The order determines the steepness of the filter's roll-off in the stopband:
- A lower order results in a gentler roll-off, which is suitable for applications where a smooth transition is essential.
- A higher order produces a steeper roll-off, providing better attenuation of unwanted frequencies.
To determine the required filter order for a specific application, you can analyze factors such as the desired cut-off frequency and the acceptable level of attenuation.
Comparison to Other Filter Types
Butterworth filters are often compared to other popular filter types, such as Chebyshev and Bessel filters. Here’s a brief comparison:
-
Butterworth vs. Chebyshev: Chebyshev filters can have a sharper roll-off compared to Butterworth filters, but they introduce ripples in the passband. In contrast, Butterworth filters maintain a smooth response but sacrifice sharpness.
-
Butterworth vs. Bessel: Bessel filters offer the best phase response, making them suitable for applications where preserving the waveform shape is critical. However, they generally have a slower roll-off than Butterworth filters.
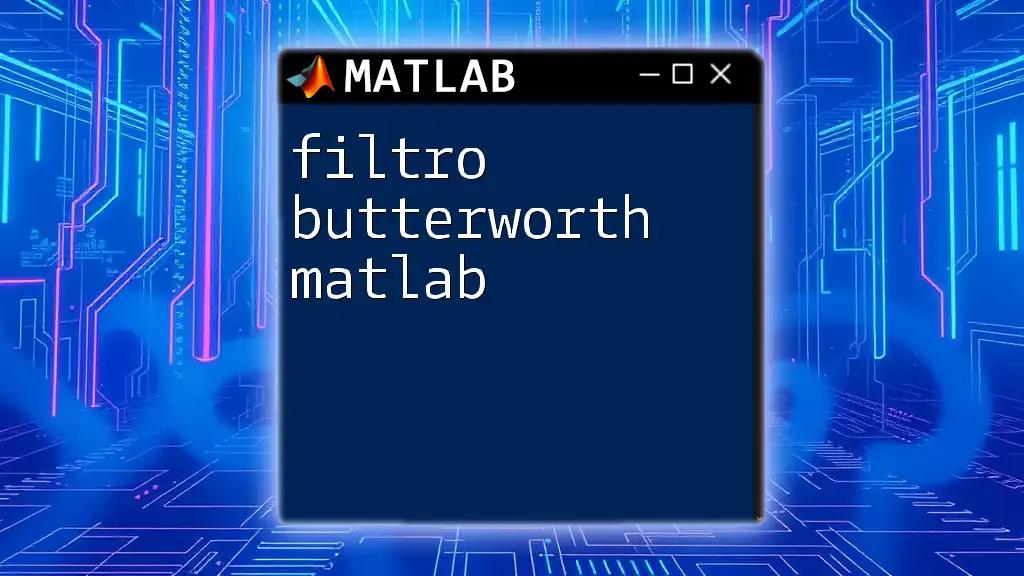
Implementing Butterworth Filters in MATLAB
Setting Up MATLAB for Filter Design
Before working with Butterworth filters in MATLAB, ensure you have the Signal Processing Toolbox installed. This toolbox contains essential functions required for filter design and analysis.
Low-Pass Butterworth Filter
Use-Cases: Low-pass filters are useful in applications like audio processing where you want to allow low-frequency signals (like bass) while attenuating higher frequencies (like treble).
MATLAB Code Example:
% Design parameters
fs = 1000; % Sampling frequency
fc = 100; % Cut-off frequency
order = 4; % Filter order
[b, a] = butter(order, fc/(fs/2), 'low'); % Create filter coefficients
High-Pass Butterworth Filter
Use-Cases: High-pass filters are ideal when you want to eliminate low-frequency noise or DC components from a signal, such as in audio or data signals.
MATLAB Code Example:
% Design parameters
fs = 1000; % Sampling frequency
fc = 200; % Cut-off frequency
order = 4; % Filter order
[b, a] = butter(order, fc/(fs/2), 'high'); % Create filter coefficients
Band-Pass and Band-Stop Butterworth Filters
Use-Cases: Band-pass filters are valuable in communications to allow specific ranges of frequencies (e.g., a radio station), while band-stop filters are used to eliminate unwanted frequencies (e.g., noise).
MATLAB Code Example for Band-Pass:
% Design parameters
fs = 1000; % Sampling frequency
fc = [100 200]; % Cut-off frequencies
order = 4; % Filter order
[b, a] = butter(order, fc/(fs/2), 'bandpass'); % Create filter coefficients
Visualizing the Filter Response
Visualizing the filter's frequency response is crucial for understanding its performance across different frequencies. The following MATLAB code snippet illustrates how to plot the frequency response:
[h, f] = freqz(b, a, 1024, fs);
plot(f, abs(h));
title('Frequency Response of the Butterworth Filter');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
grid on;
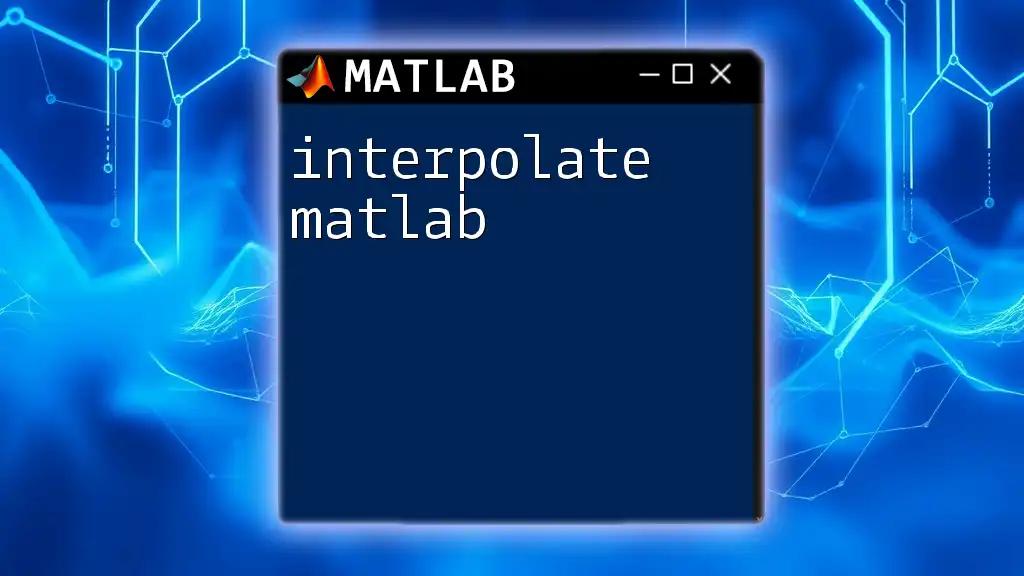
Practical Applications
Signal Processing
Butterworth filters are essential in various signal processing applications, from audio filtering to communications systems. They help in isolating frequency bands and eliminating unwanted noise.
Real-World Example
You can create a simple project to demonstrate the use of a Butterworth filter in audio signal processing. For instance, you might want to apply a low-pass filter to an audio signal to clean it up:
% Load an audio signal
[y, fs] = audioread('audio_signal.wav');
filtered_signal = filter(b, a, y); % Apply Butterworth filter
sound(filtered_signal, fs); % Play the filtered sound
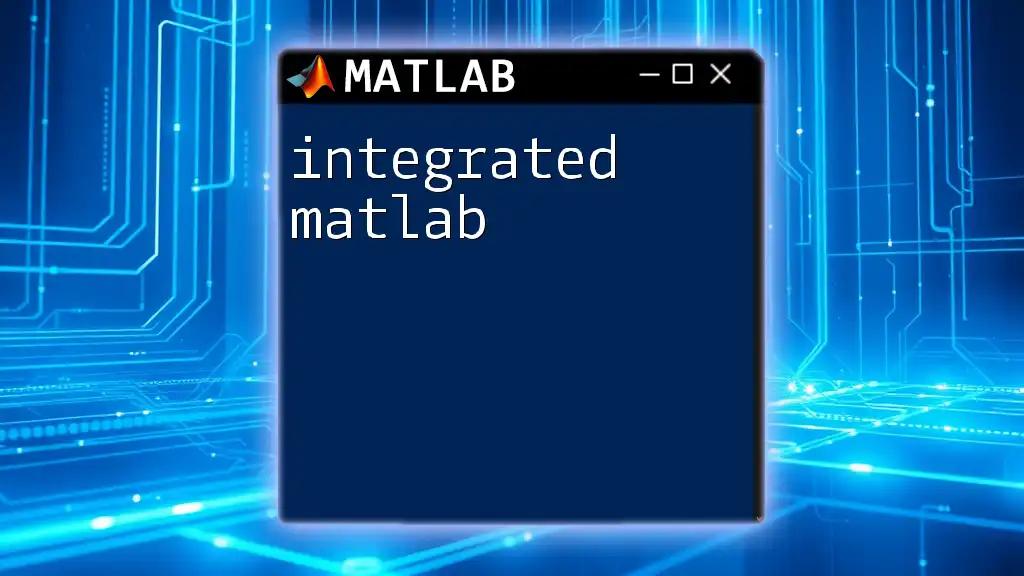
Tips for Effective Filter Design
Choosing the right parameters is crucial for achieving optimal performance with your Butterworth filter. Here are some tips:
- Cut-off Frequency: Always consider the cut-off frequency carefully. Choose a frequency that meets your application requirements without cutting off essential signals.
- Filter Order: Assess the order of the filter needed based on your desired roll-off characteristics. Higher orders provide better attenuation but may introduce unwanted phase shifts.
Avoiding Common Pitfalls
When designing your Butterworth filter, be cautious of sampling frequency issues:
- Aliasing: Ensure that your sampling frequency is at least twice the highest frequency you want to retain in the signal (Nyquist rate) to avoid aliasing.
- Implementation Errors: Always test your filter design with input signals to validate its performance in practical scenarios.
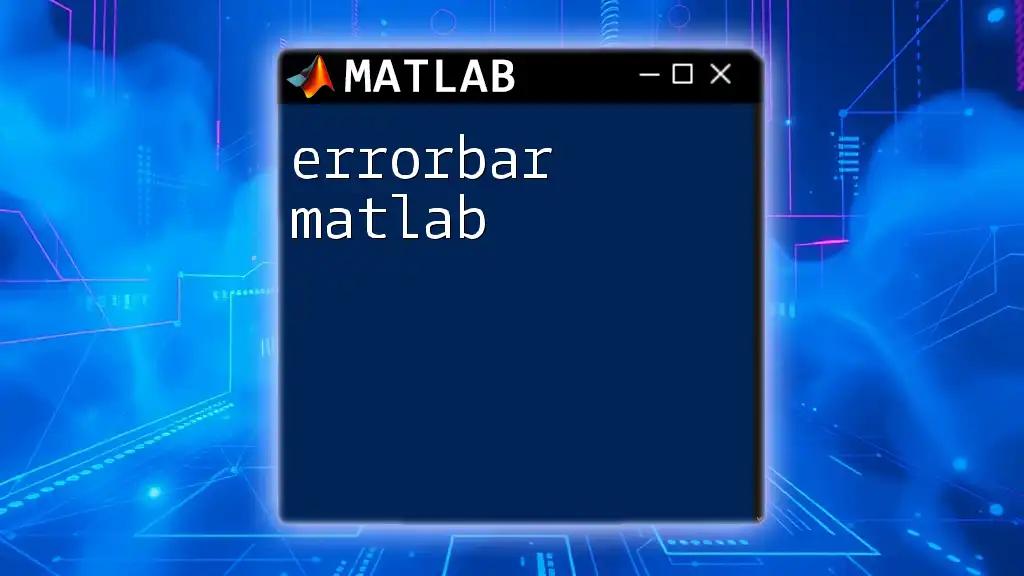
Conclusion
In this guide, we explored the intricacies of the butterworth filter in MATLAB, including its theoretical foundations, implementation strategies, and real-world applications. By following the rigorous design processes outlined and experimenting with MATLAB, you can effectively utilize Butterworth filters to enhance your signal processing projects. For further learning, consider reviewing MATLAB's official documentation and exploring filter design tutorials to expand your knowledge.
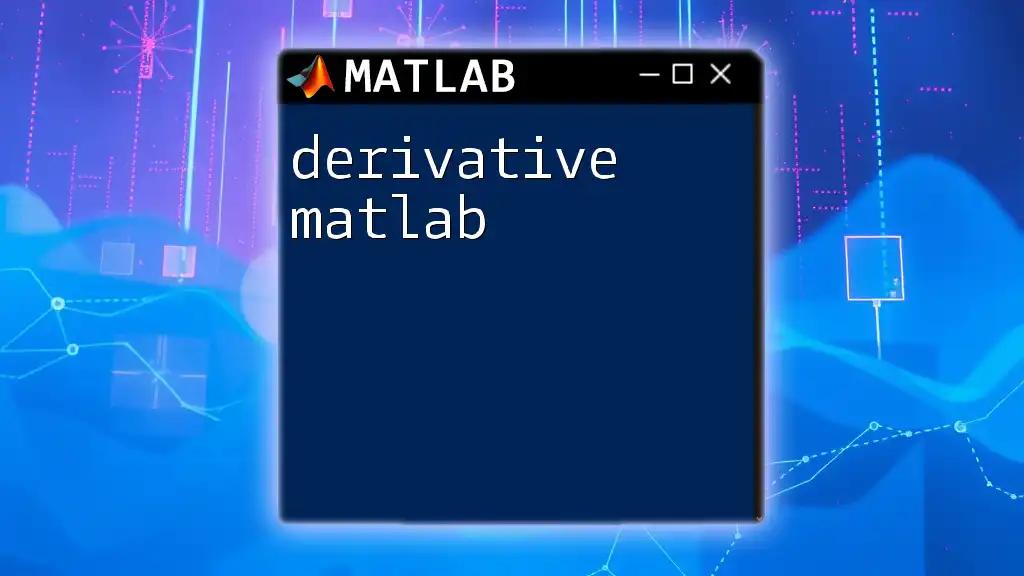
FAQs
What is the difference between Butterworth and Chebyshev filters?
Butterworth filters prioritize a smooth frequency response with no ripples, whereas Chebyshev filters allow for a sharper roll-off but introduce ripples in the passband.
How can I test the effectiveness of a Butterworth filter?
You can validate a Butterworth filter by analyzing its frequency response and applying it to test signals to observe its filtering capabilities.
What are some MATLAB functions for designing other types of filters?
In addition to `butter`, you can explore functions like `cheby1`, `cheby2`, and `bessel` for designing Chebyshev and Bessel filters, respectively.