A graphical interface in MATLAB allows users to create and interact with visual elements such as buttons, sliders, and plots, enhancing user engagement and simplifying complex programming tasks.
Here's a simple example to create a graphical user interface (GUI) with a push button that displays a message when clicked:
function simpleGUI
f = figure('Position', [100, 100, 300, 200]);
btn = uicontrol('Style', 'pushbutton', 'String', 'Click Me',...
'Position', [100, 80, 100, 40],...
'Callback', @buttonCallback);
function buttonCallback(~, ~)
msgbox('Hello, MATLAB!');
end
end
Understanding MATLAB’s GUI Components
What is a Graphical User Interface (GUI)?
A Graphical User Interface (GUI) is an interactive interface that allows users to interact with software applications through graphical elements like buttons, sliders, and text fields. In the context of graphical interface in MATLAB, this means providing users with a visual way to interact with their data and functions, making programming and data analysis more accessible to a wider audience.
Benefits of Using a GUI include:
- User-Friendliness: GUIs simplify complex tasks, allowing users with minimal programming knowledge to navigate and utilize MATLAB's power.
- Visual Feedback: Real-time graphical representations help users understand their data better.
Key GUI Components in MATLAB
Figure Windows
Figure windows serve as the primary containers for graphical outputs. Each figure can display plots, images, and other visual elements. Creating a basic figure in MATLAB is as simple as:
figure;
plot(1:10, rand(1,10));
title('Sample Plot');
xlabel('X-Axis');
ylabel('Y-Axis');
This code generates a new figure and plots a line graph of random data. You can easily customize these figures with titles, labels, and legends to provide context for your data visualizations.
Axes
Axes are pivotal for representing data. They allow you to define the data limits and labels, influencing how data is displayed. You can customize axes by adjusting their properties, such as tick marks, grid lines, and scaling. This customization enhances the clarity of plots, helping viewers better interpret the information being presented.
Uicontrols
User controls are interactive components within a MATLAB GUI. They include buttons, sliders, radio buttons, and edit boxes. By leveraging these controls, you can create intuitive interfaces.
Creating a Button
To create a simple push button within a figure:
% Create a figure window with a button
f = figure('Position', [100, 100, 400, 300]);
uicontrol('Style', 'pushbutton', 'String', 'Click Me', 'Position', [150, 130, 100, 30]);
This snippet generates a figure with a clickable button labeled "Click Me." This is your entry point for further interactivity within your GUI.
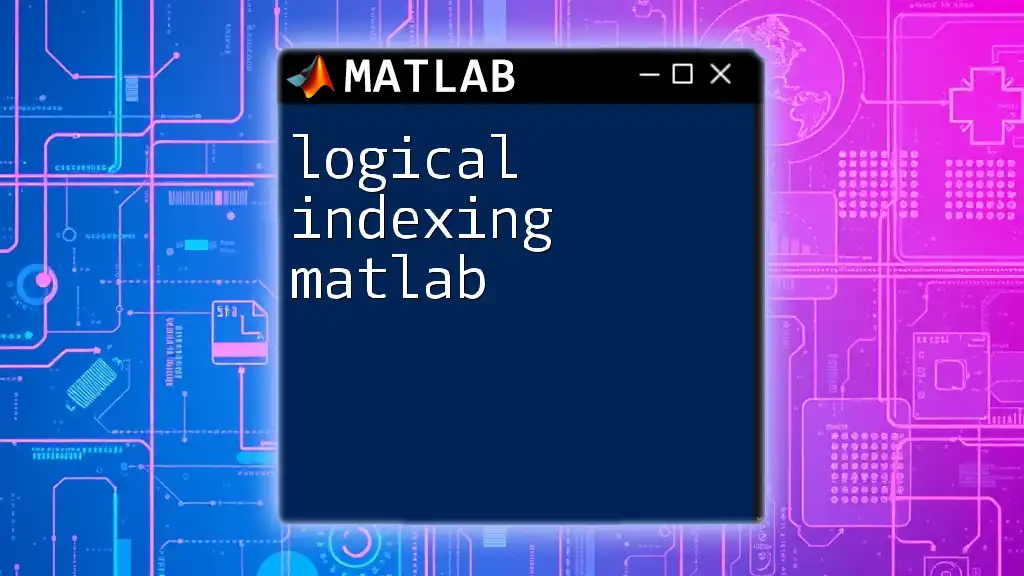
Designing a Simple GUI in MATLAB
Step-by-Step Guide to Create a Basic GUI
Step 1: Setting up the GUI Layout
Structuring your GUI effectively is crucial for user interaction. Consider the flow of activities and how users will navigate through the different components. Established best practices, such as grouping related controls and maintaining a consistent design, enhance usability.
Step 2: Adding UI Controls
You can mix various UI controls to create diverse functionalities. For example, sliders can help users set numerical parameters, while checkboxes can allow for multiple selections.
Step 3: Adding Functionality via Callbacks
Callbacks link your GUI controls to their actions. When a user interacts with a control—like clicking a button or moving a slider—the callback function is executed. This conversion of user actions into computational tasks makes your application dynamic and responsive.
Example: Creating a Simple Data Entry GUI
Here’s how you can create a simple data entry GUI to accept user input and print it to the command window:
function simpleDataEntryGUI()
f = figure('Position', [100, 100, 300, 200]);
uicontrol('Style', 'text', 'String', 'Enter your name:', 'Position', [20, 150, 100, 20]);
hEdit = uicontrol('Style', 'edit', 'Position', [150, 145, 100, 20]);
uicontrol('Style', 'pushbutton', 'String', 'Submit', ...
'Position', [100, 100, 100, 30], ...
'Callback', @(src, event) disp(get(hEdit, 'String')));
end
In this code, a text label prompts the user to enter their name in an edit box. Upon clicking the "Submit" button, the entered name is printed in the command window. This illustrates the interconnectedness of different GUI components and functions.
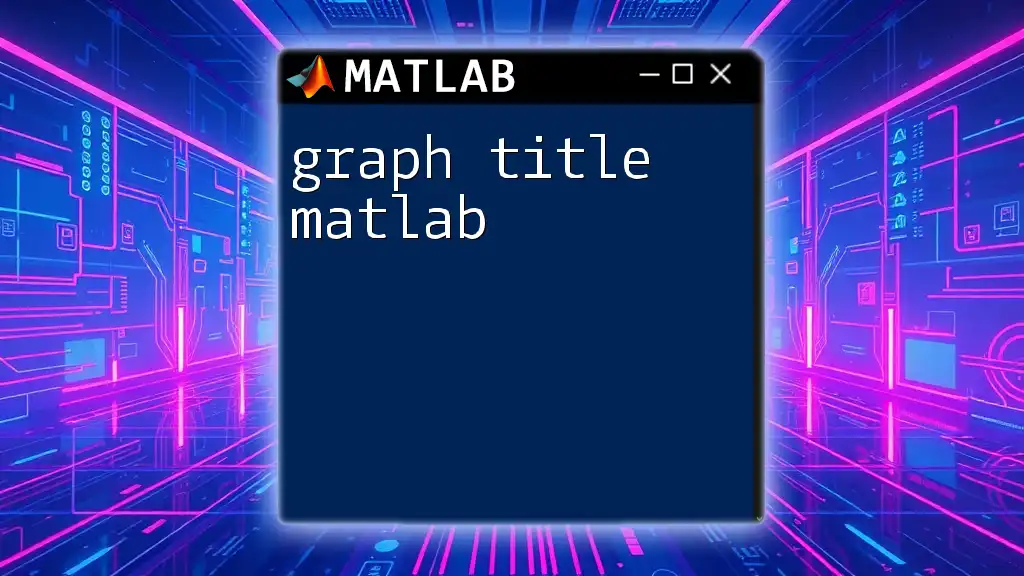
Advanced GUI Features and Customization
Enhancing User Experience with Custom Styles
Customizing the look and feel of your GUI enhances user satisfaction. You can change the colors of the UI components and adjust the fonts to align with branding or personal preferences.
Using App Designer for More Complex Applications
App Designer offers a more sophisticated environment for building GUIs in MATLAB. Unlike the traditional methods, it provides a drag-and-drop interface for designing apps. You can easily gain a preview of your GUI while developing.
Example of Creating a Basic App using App Designer
Creating a straightforward application is manageable with the App Designer's user-friendly interface. You can use its tools to add components, arrange them visually, and generate MATLAB code behind the scenes.
Deployment Options for MATLAB GUIs
Once your GUI is ready, you may want to share it with others. MATLAB provides deployment options that enable you to package your GUI as a standalone application. With the MATLAB Compiler, you can distribute your application, allowing users without MATLAB installed to run it smoothly.
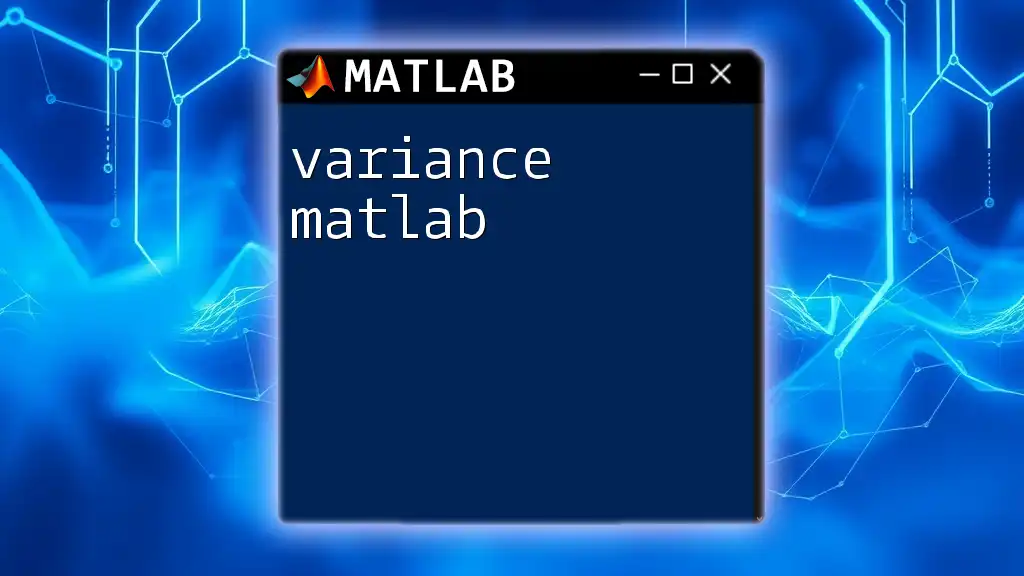
Common Problems and Troubleshooting Tips
Common GUI Issues and Fixes
Even the most well-designed GUIs can run into problems. Common issues may include unexpected behaviors from UI controls or performance hiccups during user interaction. Identifying the root cause is critical for effective troubleshooting.
Steps to Debugging GUI Applications
Debugging is essential to ensure a smooth user experience. Utilizing breakpoints in your code allows you to inspect the flow of execution at runtime. Additionally, examine callbacks closely, as many issues stem from problems in these function definitions.
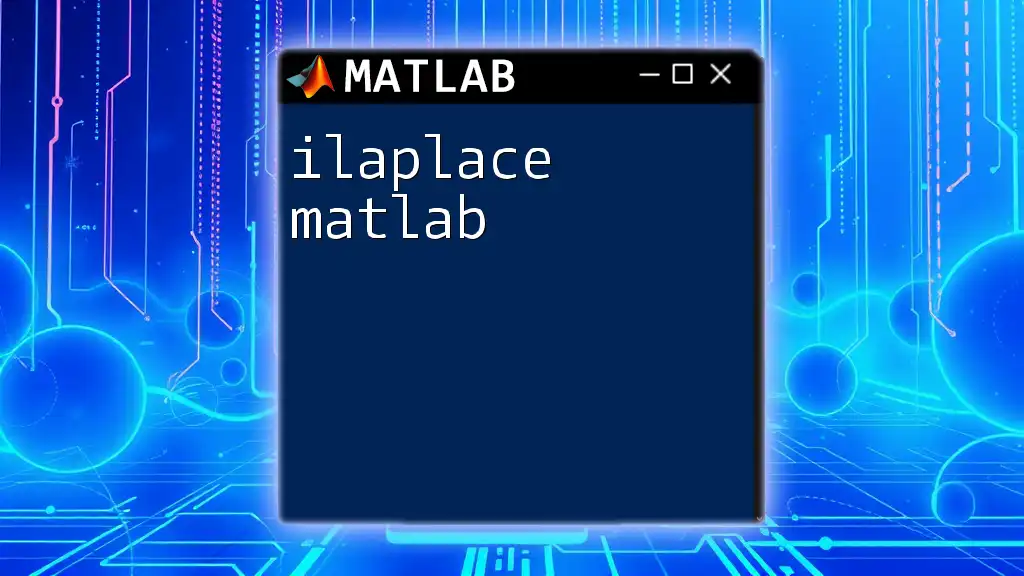
Conclusion
The versatility of a graphical interface in MATLAB empowers users to leverage MATLAB’s capabilities without extensive programming knowledge. By understanding the fundamentals, components, and advanced techniques, you create effective and user-friendly applications that can transform data analysis and visualization experiences.
Whether you are designing a simple entry form or a complex application, the ability to create intuitive GUIs in MATLAB opens the door to enhanced interactivity and engagement. So dive in, explore, and create your own graphical interfaces to make your MATLAB experience even more impactful!
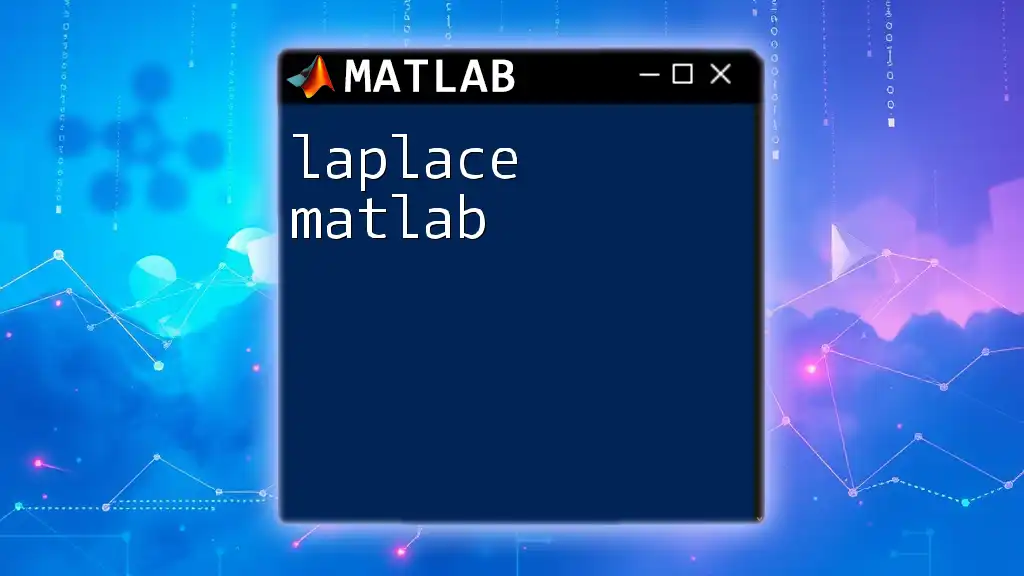
Additional Resources
For further understanding, consult the official MATLAB documentation and join online communities where experienced MATLAB users share their insights and solutions to common problems. This will enhance your learning and keep you updated with the latest features.
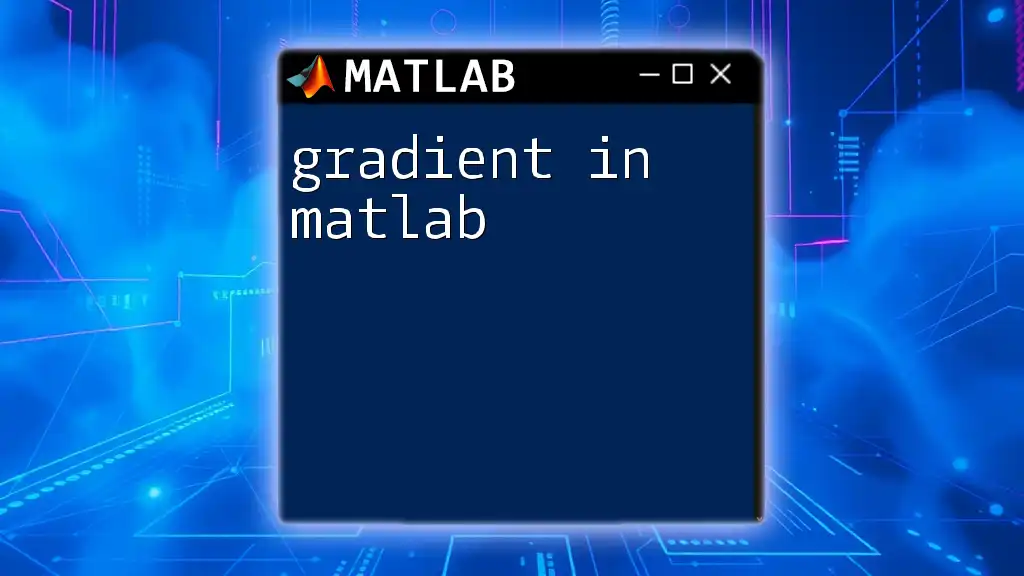
Call to Action
Start experimenting with your own GUI designs today! Share your creations and any questions you might have, and don’t hesitate to suggest topics for future exploration. Happy coding!