The Newton-Raphson method is an iterative numerical technique used to find roots of real-valued functions, which can be easily implemented in MATLAB for efficient convergence. Here's a simple MATLAB code snippet to illustrate its usage:
function root = newtonRaphson(func, dfunc, x0, tol, max_iter)
% func: the function for which we want to find the root
% dfunc: the derivative of the function
% x0: initial guess
% tol: tolerance for stopping criterion
% max_iter: maximum number of iterations
for i = 1:max_iter
x1 = x0 - func(x0)/dfunc(x0); % update rule
if abs(x1 - x0) < tol % check for convergence
root = x1;
return;
end
x0 = x1; % prepare for next iteration
end
error('Max iterations reached without convergence');
end
Understanding the Newton-Raphson Method
What is the Newton-Raphson Method?
The Newton-Raphson method is a powerful technique in numerical analysis used to find successively better approximations of the roots (or zeroes) of a real-valued function. This iterative method is particularly useful when dealing with functions that are difficult to analyze algebraically. Historically, it has found extensive applications in mathematics, physics, engineering, and computer science due to its speed and efficiency when compared to other numerical root-finding methods.
How the Newton-Raphson Method Works
The method relies on the idea of linear approximation. By utilizing the derivative of the function at a current guess, we can compute the next approximation. The core formula is:
\[ x_{n+1} = x_n - \frac{f(x_n)}{f'(x_n)} \]
Here, \(x_n\) is the current approximation, \(f(x_n)\) is the value of the function at this point, and \(f'(x_n)\) is the derivative. The process continues until the difference between successive approximations is less than a predefined tolerance level.
Choosing an appropriate initial guess is crucial because it can greatly affect the convergence of the method. If the guess is too far from the actual root, or if the function is not well-behaved near the guess, the method may fail to converge or may converge to the incorrect root.
Advantages and Limitations
The Newton-Raphson method boasts several advantages:
- Rapid convergence: When close to the root, it exhibits quadratic convergence, making it faster than methods like bisection or fixed-point iteration.
- Ease of use: Implementing the algorithm is straightforward, making it a popular choice for many numerical applications.
However, there are also notable drawbacks:
- Local behavior: If the initial guess is not close to the root, the method can diverge.
- Derivative requirement: It requires the computation of the derivative, which may not always be readily available or may be difficult to estimate for complex functions.

Implementing the Newton-Raphson Method in MATLAB
Setting Up Your MATLAB Environment
Before you start coding, ensure that you have a proper MATLAB environment set up. Familiarize yourself with the MATLAB interface, especially the Command Window and Editor. It’s also beneficial to understand how to access help documentation within MATLAB to resolve any issues you may encounter during development.
Basic Syntax for Implementing the Method
MATLAB syntax is intuitive and allows for a fluid implementation of the Newton-Raphson method. The main components involve function definitions, iterative loops, and conditional checks to determine convergence.
Example Problem: Finding Roots of a Function
Step 1: Define the Function
To find the root of a simple function, let’s define the function \(f(x) = x^2 - 2\) (which has a root at \(\sqrt{2}\)):
f = @(x) x^2 - 2; % Example function for finding the square root of 2
Step 2: Define the Derivative
Next, we need the derivative of this function, which is \(f'(x) = 2x\):
df = @(x) 2*x; % Derivative of the function
Step 3: Implement the Newton-Raphson Algorithm
With both the function and its derivative defined, you can now implement the Newton-Raphson algorithm:
x0 = 1; % Initial guess
tol = 1e-6; % Tolerance for convergence
max_iter = 100; % Set maximum iterations
for i = 1:max_iter
x1 = x0 - f(x0)/df(x0); % Update approximation
if abs(x1 - x0) < tol % Check for convergence
break; % Stop if within tolerance
end
x0 = x1; % Prepare for next iteration
end
fprintf('The root is: %f\n', x1); % Display the result
In this code snippet, the loop will continue iterating until either the maximum number of iterations is reached or the difference between two successive approximations is less than the defined tolerance.
Tips for Optimizing MATLAB Code
Avoiding Common Mistakes
Common pitfalls include using a bad initial guess or failing to check for division by zero in functions where the derivative may be zero. Always validate inputs and outputs to minimize errors.
Speeding Up Your Algorithm
For larger datasets or more complex functions, consider using vectorization instead of loops wherever possible. This can significantly enhance the performance of your code. Additionally, utilizing built-in MATLAB functions, like `fzero`, can provide quick solutions for root-finding problems without the need for manual implementations.
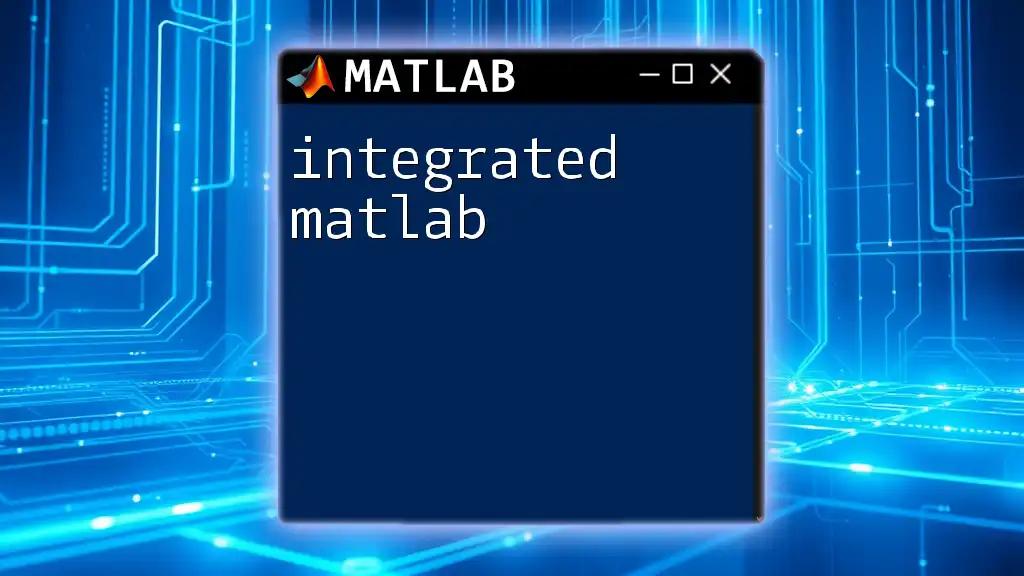
Visualizing Results in MATLAB
Creating Plots
Visualizing results can help significantly in understanding the effectiveness of the Newton-Raphson method. By plotting the function along with the found root, you can visually assess the convergence and validate results.
Here’s how you can create a plot of the function where the root is marked:
x = -2:0.1:2; % Define a range of x values
y = f(x); % Compute the function values
plot(x, y); % Plot the function
hold on; % Hold the plot for additional elements
plot(x1, f(x1), 'ro'); % Mark the found root with a red dot
xlabel('x'); % Label x-axis
ylabel('f(x)'); % Label y-axis
title('Newton-Raphson Method Example'); % Title of the plot
grid on; % Add a grid for easier readability
This snippet will generate a graph of the function \(f(x)\) along with a visual indication of where the root lies.
Interpreting the Plots
Interpreting plots is crucial for understanding the method's performance. Look for the behavior of the function around the root and assess if the iterations are concentrating towards the root as expected. A plot can often highlight issues such as non-convergence or unexpected behavior through visual cues.
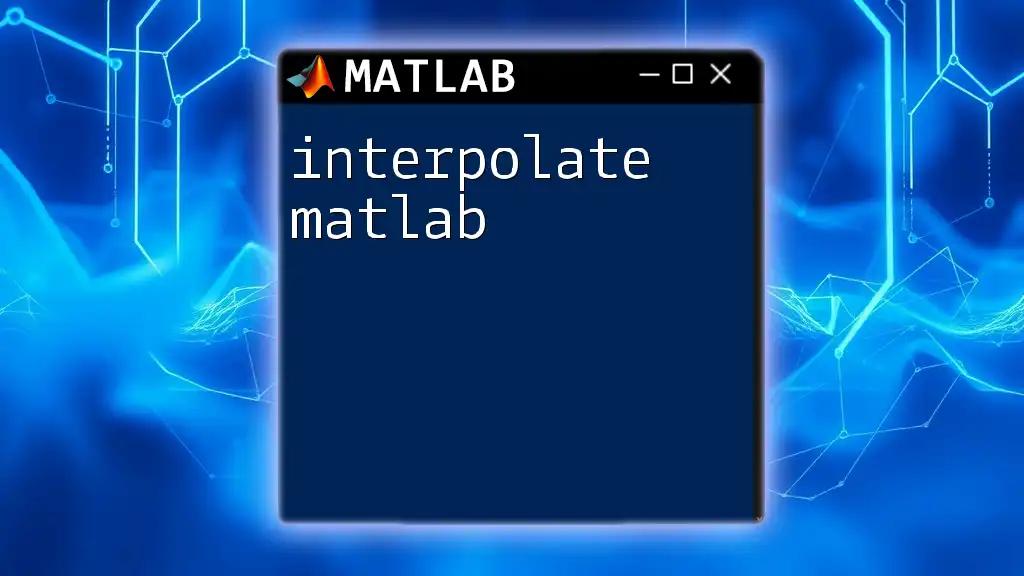
Case Studies and Practical Applications
Engineering Applications
The Newton-Raphson method is frequently used in engineering for solving nonlinear equations that arise in structural analysis, fluid dynamics, and other fields. For instance, it can assist in finding stresses and strains in materials subject to different loads, where the governing equations may be complex.
Scientific Research Applications
In scientific research, the method can be implemented in simulations involving systems of equations. For example, it is used in computational chemistry to determine equilibrium states of molecules based on potential energy surfaces, thereby predicting molecular structures.
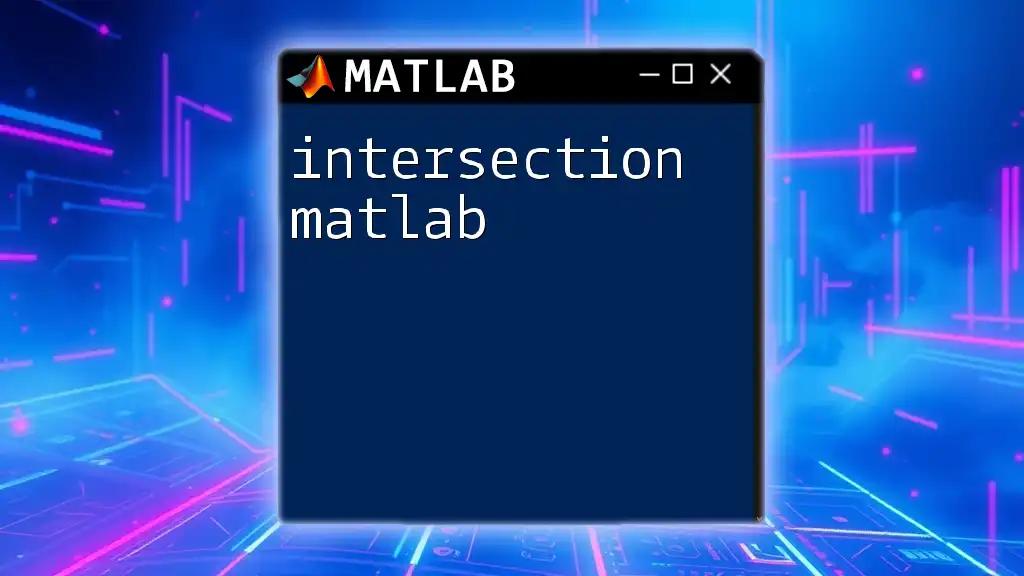
Conclusion
In summary, the Newton-Raphson method in MATLAB provides a robust framework for solving equations efficiently. Its iterative nature and reliance on derivatives lend it a speed that is invaluable in both academic and practical applications. By experimenting with different functions and initial guesses, practitioners can gain a deeper understanding of its capabilities. As you continue your journey into numerical methods, consider exploring MATLAB’s rich toolset to enhance your analytical skills further.

Additional Resources
To deepen your understanding of the Newton-Raphson method and its applications in MATLAB, refer to the official [MATLAB documentation](https://www.mathworks.com/help/matlab/). Further exploring numerical methods can also be beneficial; consider academic textbooks or online courses as a supplement to this information.

Frequently Asked Questions
What if the method does not converge?
Failure to converge can arise from several reasons, including poor initial guesses, flat or undefined derivatives, or functions without a real root. Review your function's behavior through plotting, and adjust your initial guess accordingly.
Can the Newton-Raphson method be used for multiple dimensions?
Yes, the Newton-Raphson method can be extended to multivariable functions. The technique generalizes to systems of equations where Jacobians replace simple derivatives, allowing for the simultaneous finding of multiple roots in higher dimensions. The implementation in MATLAB will involve defining a vector of functions and utilizing matrix operations.
Using this article as a guide, you can effectively apply the Newton-Raphson method within MATLAB, combining numerical techniques and programming practices for efficient problem-solving.