Double precision error in MATLAB refers to the potential inaccuracies that can occur in numerical computations due to the limitations of representing floating-point numbers, which can lead to unexpected results during calculations.
Here's a code snippet to illustrate how double precision can affect calculations:
% Example of double precision error in MATLAB
a = 0.1 + 0.2; % Expected result is 0.3
disp(a); % Displays 0.3000
% Check if a is exactly equal to 0.3
isEqual = (a == 0.3); % May return false due to precision error
disp(isEqual); % Displays 0
Understanding Double Precision in MATLAB
What is Double Precision?
Double precision is a numerical format that uses 64 bits to represent numbers in computing. In MATLAB, using double precision is significant because it allows for a more accurate representation of real numbers compared to single precision, which only utilizes 32 bits. This increased bit depth translates into a wider range of representable values and more decimal places, making it ideal for complex calculations.
In MATLAB, when you define a number without specifying its data type, it is automatically treated as a double. This default behavior is crucial for engineers and scientists relying on high precision for their mathematical computations.
How MATLAB Handles Double Precision
MATLAB supports several data types, but the most commonly used for floating-point arithmetic is the 'double' type. This data type adheres to the IEEE 754 standard for floating-point arithmetic, ensuring consistency across different computing platforms.
The efficient handling of double precision in MATLAB allows users to perform a variety of operations ranging from basic arithmetic to complex algorithms without manually adjusting precision settings. However, it's essential to understand that even double precision can be susceptible to errors due to the inherent limitations of binary representation.
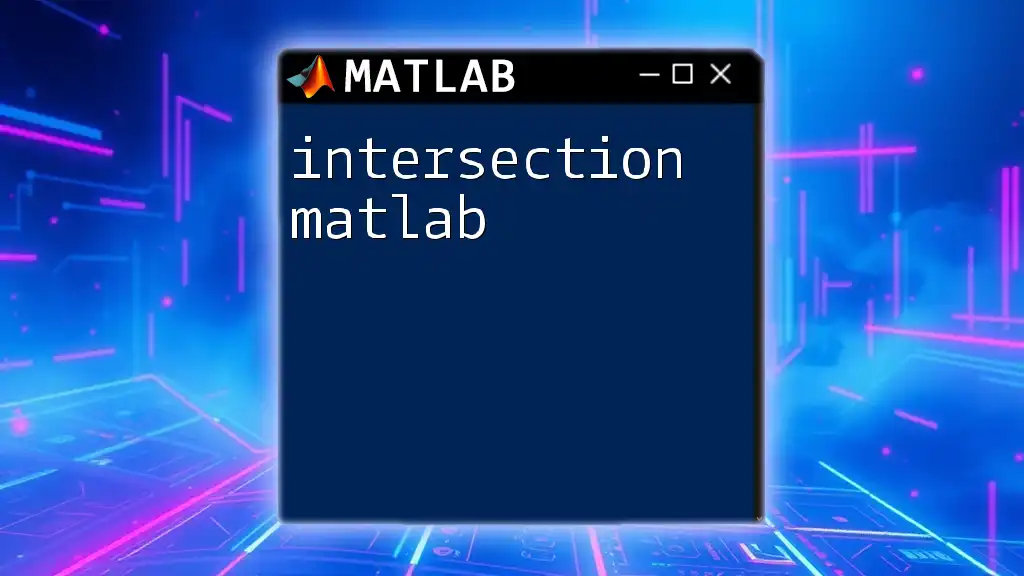
The Nature of Double Precision Error
Introduction to Floating-Point Arithmetic
At the core of double precision errors is the concept of floating-point arithmetic. Numbers are represented in binary, which can lead to inaccuracies when converting decimal numbers. For instance, many decimal fractions cannot be precisely represented in binary, leading to rounding errors.
Precision and rounding error arise when calculations result in numbers that exceed the representable range, prompting truncation or rounding. This is particularly critical in operations involving large scales or very small values.
Sources of Double Precision Error
Double precision errors can stem from a variety of operations. For example, subtracting two very similar numbers can amplify rounding errors, a phenomenon often termed catastrophic cancellation. Consider the following operation:
a = 1;
b = 1 + eps/2; % where eps is the floating-point relative accuracy
result = a - b;
disp(result); % result may not be what you expect
The precision loss can lead to unexpected outcomes, especially in iterative algorithms where each step might introduce additional errors.
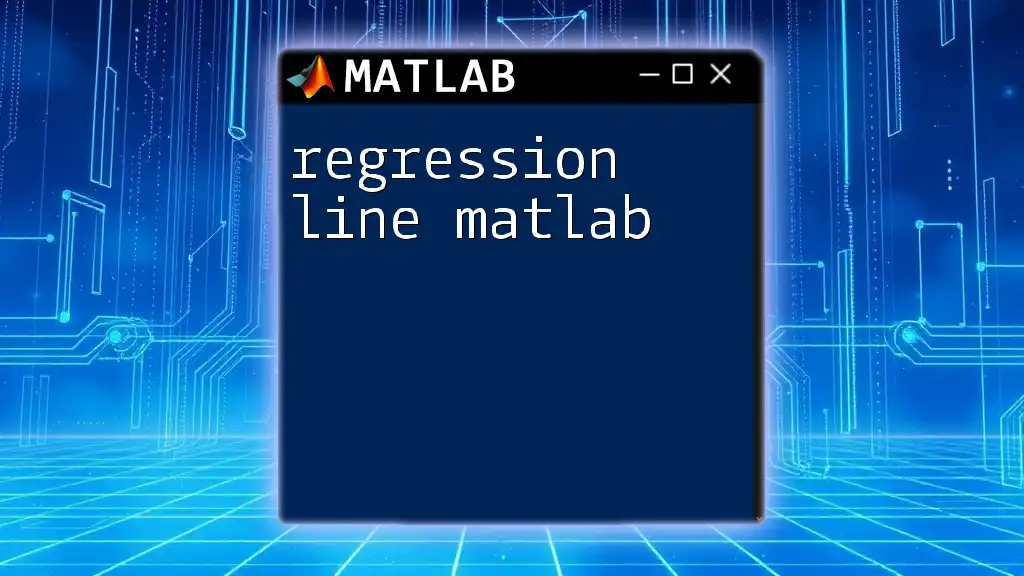
Recognizing Double Precision Errors in MATLAB
Symptoms of Double Precision Errors
Identifying double precision errors often comes from observing unexpected behavior in program output. Symptoms include:
- Discrepancies in calculation results that do not align with mathematical expectations.
- Problems in iterative calculations where results diverge from theoretical predictions.
These symptoms can often indicate hidden precision issues lurking in the code.
Tools for Diagnosing Precision Issues
MATLAB provides several built-in functions useful for diagnosing precision errors. The function `eps` returns the distance from 1.0 to the next larger double-precision number, serving as a benchmark for numerical stability.
You can use this function to assess precision like so:
a = 1e16;
b = a + 1;
precision_check = abs((b - a) - 1);
disp(precision_check); % Should ideally be zero but may not be.
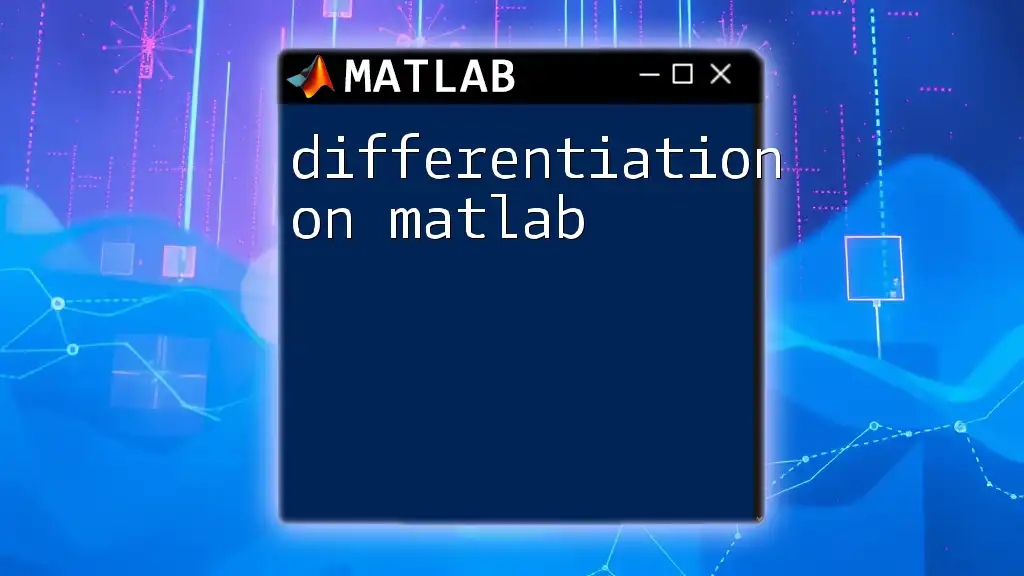
Mitigating Double Precision Errors
Techniques to Minimize Errors
To effectively mitigate double precision errors, here are some best practices:
- Use Variable Precision Arithmetic: MATLAB’s `vpa` function allows you to set arbitrary precision for calculations, useful for high-stakes computations.
Example using `vpa` can be illustrated as follows:
digits(50); % Setting precision to 50 decimal places
a = vpa('1.1');
b = vpa('1.2');
c = a + b;
disp(c);
This approach can significantly reduce the probability of encountering rounding errors.
Strategies for Robust Mathematical Modeling
Selecting numerical methods wisely plays a crucial role in minimizing double precision errors. Functions like `fminunc` and `ode45` often employ built-in techniques to handle precision issues effectively.
An algorithm designed with numerical stability in mind can help manage the pitfalls associated with floating-point arithmetic more effectively.
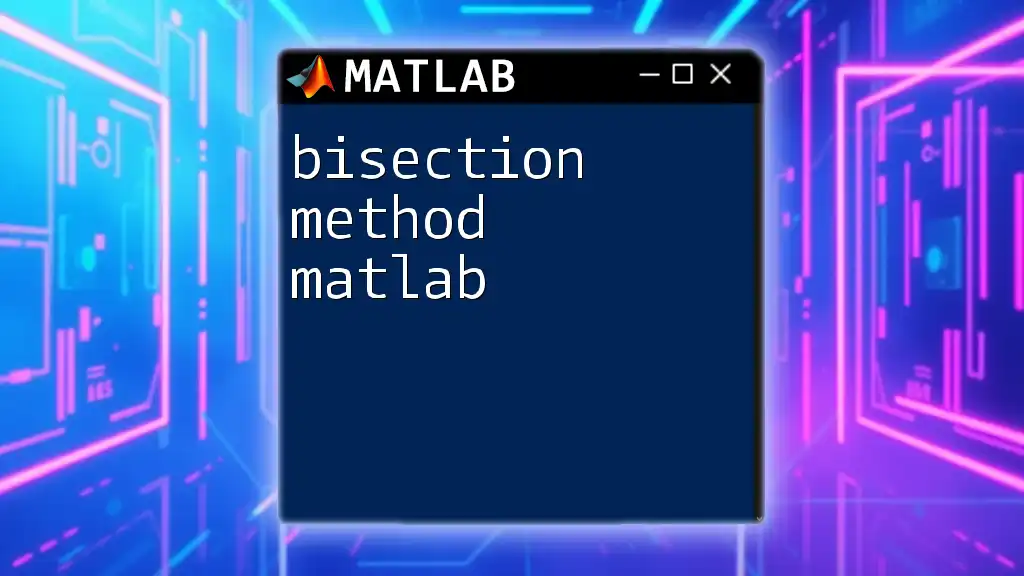
Practical Examples and Case Studies
Example 1: Simple Sum Inaccuracies
Consider the naive summation of a large number followed by a small increment:
a = 1e16;
b = a + 1;
disp(b - a); % Output might be 0 due to precision loss
In this example, the addition of 1 to `1e16` results in no change to the value, demonstrating the limits of double precision in capturing subtle distinctions when numeric differences fall within the threshold of rounding.
Example 2: Errors in Real-World Applications
In fields like engineering, double precision errors can lead to substantial real-world consequences. For instance, in structural load calculations, overlooking these errors could lead to designs that are unsafe or unfeasible. The accuracy of computed load conditions is paramount, and even minor errors can propagate through complex simulations and violate safety standards. By being able to recognize and mitigate these precision issues, practitioners can significantly improve reliability.
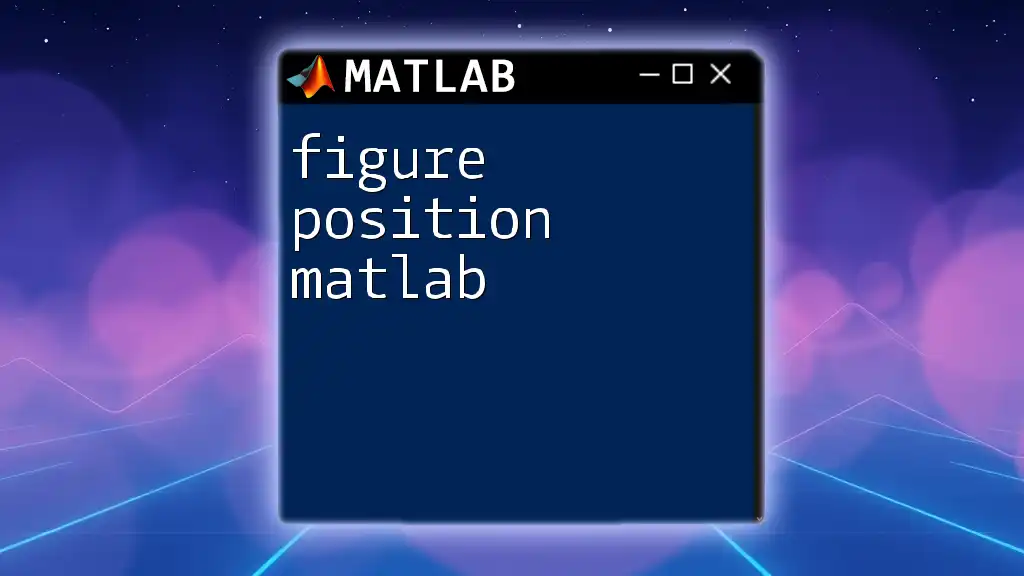
Conclusion
Recap of Key Points
Double precision errors in MATLAB can lead to unexpected results that affect the integrity of calculations. Understanding how MATLAB handles double precision, recognizing sources of error, and employing appropriate mitigation strategies are crucial for effective computational modeling.
Additional Resources
For further exploration into double precision and its implications, refer to MATLAB's official documentation on floating-point arithmetic and consider enrolling in online courses that specialize in robust numerical computing.
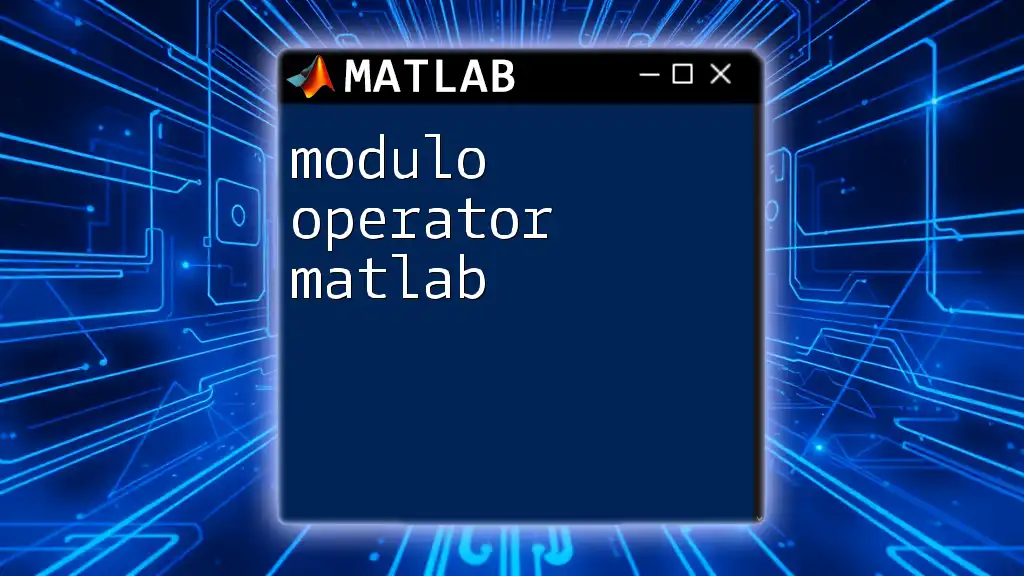
Call to Action
By practicing coding strategies that minimize double precision errors, users can enhance their MATLAB skills and build more reliable models. Join our MATLAB tutorials to delve deeper into these concepts and gain hands-on experience on how to navigate double precision errors effectively.