To export the GNU C Compiler (GCC) version of the phase transform (PHAT) algorithm from MATLAB to C, you can utilize MATLAB's code generation capabilities with the following command:
codegen -config:lib phatFunction -args {zeros(1024,1), zeros(1024,1)}
Make sure to replace `phatFunction` with the actual function name you want to generate C code for, and adjust the arguments as needed.
Understanding GCC PHAT
What is GCC PHAT?
GCC PHAT, or Generalized Cross Correlation with Phase Transform, is a powerful algorithm used in the field of signal processing for estimating the time delay between two signals. This technique excels in scenarios where noise and reverberation play significant roles, making it useful in applications such as audio processing, acoustic signal localization, and even in telecommunications. The essence of GCC PHAT lies in its ability to enhance the accuracy of time delay estimation, which is critical for applications like direction of arrival (DOA) estimation and source localization.
Applications of GCC PHAT
The versatility of GCC PHAT is reflected in its wide range of applications:
- Audio Processing: In applications such as music mixing or sound reinforcement, accurate time delay estimation between microphones can improve audio quality.
- Telecommunications: Enhancing voice signal clarity in mobile networks by accurately synchronizing signals from different antennas.
- Robotics: Determining the location of sound sources relative to a robot, enabling better interaction with environments.
The benefits of using GCC PHAT include greater speed and accuracy, leading to enhanced performance in critical systems.
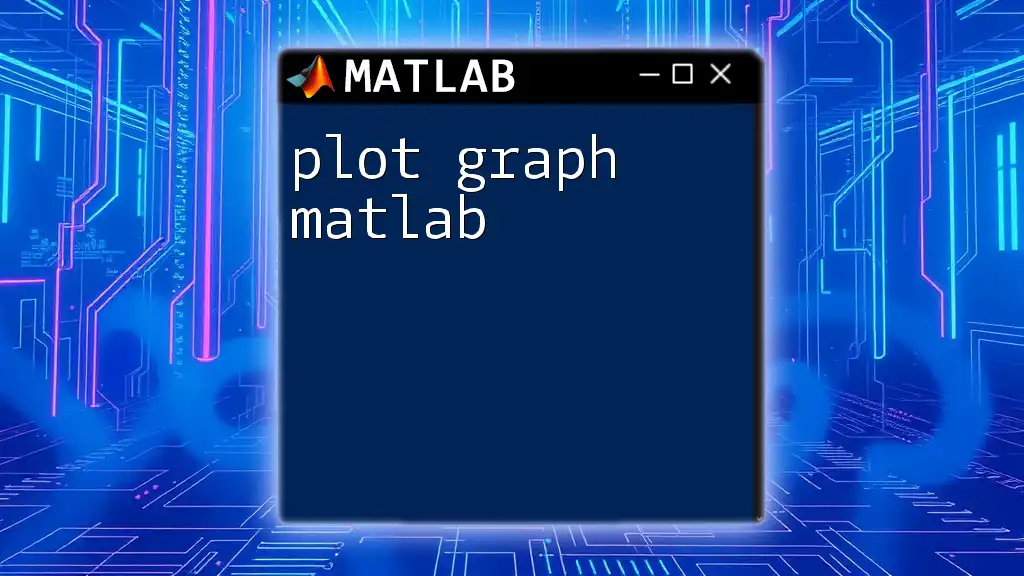
MATLAB's Implementation of GCC PHAT
Overview of MATLAB Functions for GCC PHAT
MATLAB provides a robust framework for implementing GCC PHAT through its Signal Processing Toolbox. Key functions often utilized in this context include:
- `xcorr`: Computes the cross-correlation of two signals.
- `gccphat`: Calculates the GCC PHAT estimate.
These functions leverage MATLAB's optimized libraries to provide efficient computations, facilitating the implementation of complex algorithms with minimal effort.
Example of GCC PHAT in MATLAB
Here’s a simple example demonstrating how to compute GCC PHAT in MATLAB:
% Sample code to compute GCC PHAT
[x, fs] = audioread('audiofile.wav'); % read audio file
[correlation, lags] = xcorr(x); % compute cross-correlation
gcc_phat = gcc_phat(correlation, fs); % compute GCC PHAT
plot(lags/fs, gcc_phat); % visualize the results
In this code:
- `audioread` reads the audio file and retrieves the sampling frequency.
- `xcorr` computes the cross-correlation of the signal `x`.
- The `gcc_phat` function is called to compute the GCC PHAT of the cross-correlated signal.
This example illustrates how straightforward it is to implement GCC PHAT using built-in MATLAB functions.
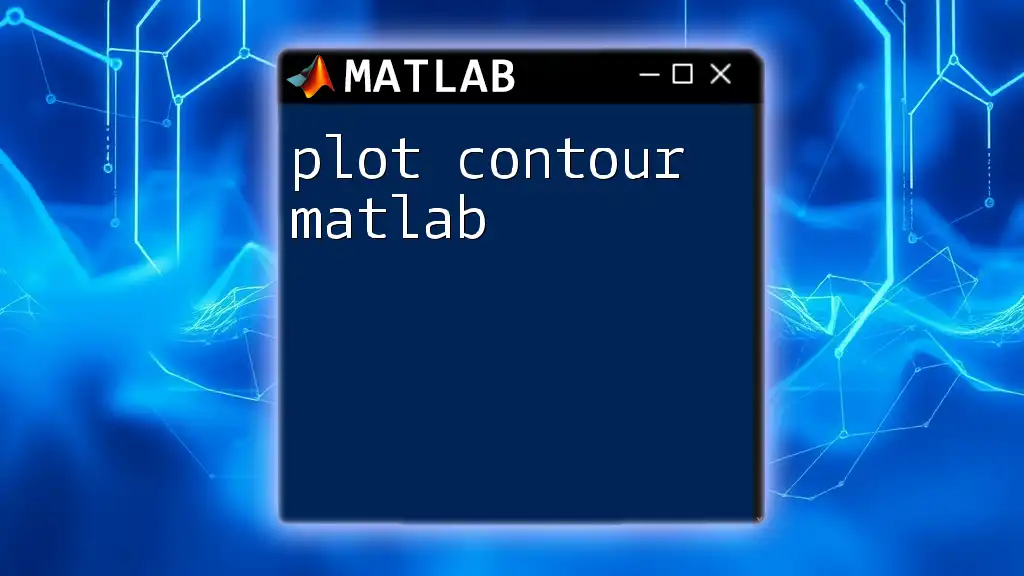
Preparing MATLAB Code for Export
Identifying Key Functions
When planning to export GCC PHAT from MATLAB to C, it’s essential to identify which functions will require conversion. Focus on core algorithm functions, especially those that handle computations relevant to GCC PHAT. Custom functions may need rewriting, while built-in functions can often be directly mapped.
Writing MATLAB Code for Compatibility
To ensure smooth export, adhere to these best practices:
- Utilize simple data types and structures (e.g., avoid complex MATLAB arrays).
- Write modular code by focusing on a single task per function to simplify the C conversion process.
Taking these steps can significantly reduce the complexity of transitioning your MATLAB code to C.
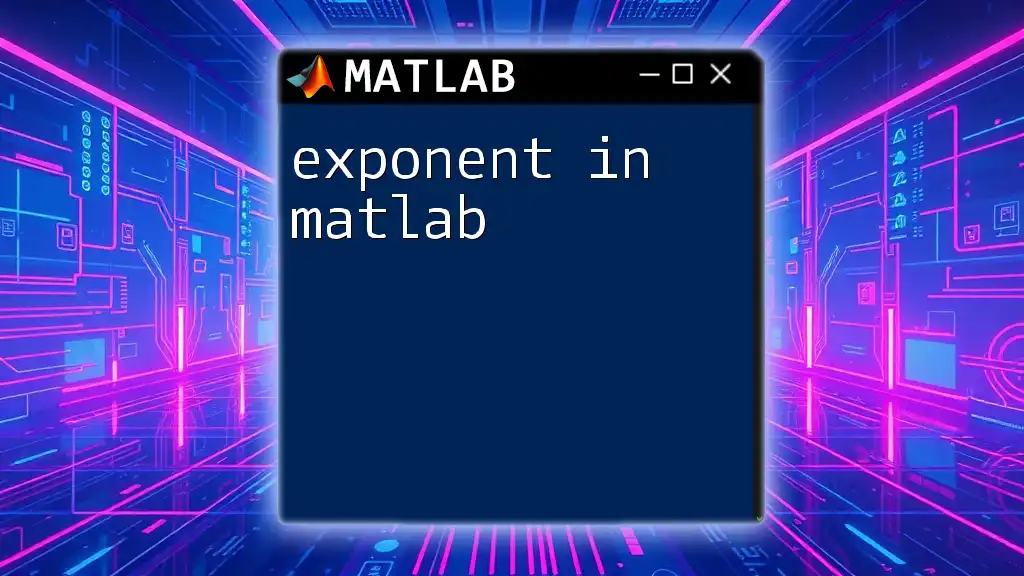
Exporting MATLAB Code to C
Using MATLAB Coder
MATLAB Coder is a tool that converts MATLAB code into C or C++ code, thus making it easier to integrate algorithms into applications that require high-performance execution. To set up MATLAB Coder, follow these steps:
- Open MATLAB Coder by navigating to the Apps tab.
- Select the program or function you intend to export.
- Choose the right settings based on your target hardware and desired output (e.g., static or dynamic memory allocation).
Exporting GCC PHAT Code
Once MATLAB Coder is set up, exporting GCC PHAT can be accomplished with the following command:
codegen myGccPhatFunction -args {input, fs} -o gcc_phat_c
This command generates C code from the specified function `myGccPhatFunction`, with `input` representing your data and `fs` your sampling frequency. Understanding the parameters in `codegen` is crucial for controlling the output type and format.
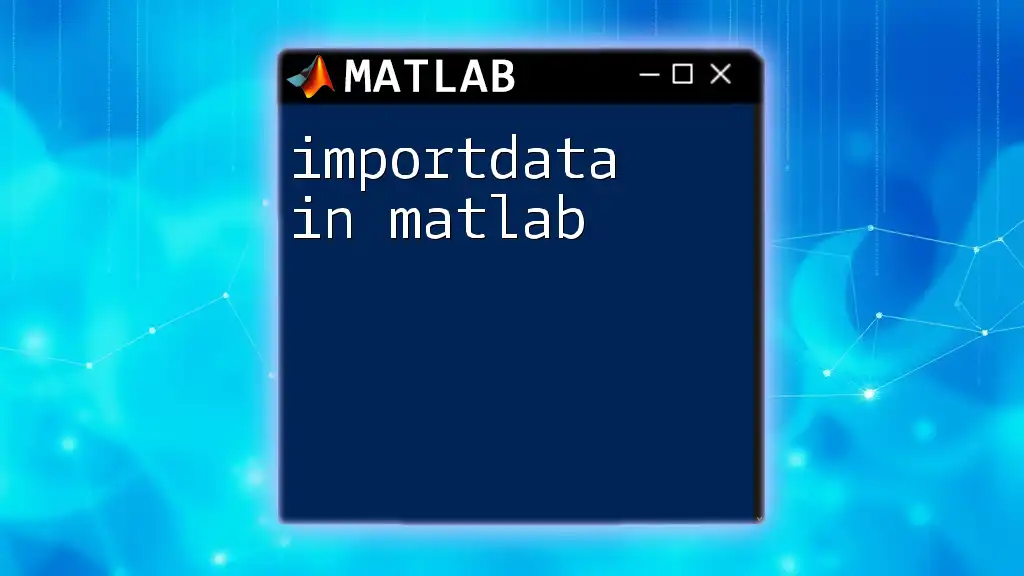
Compiling the C Code with GCC
Setting Up the GCC Environment
Before compiling, ensure your system is equipped with GCC (GNU Compiler Collection). Installation varies based on the operating system; for instance:
- Windows: Install MinGW or use WSL (Windows Subsystem for Linux).
- Linux: Check for GCC installation using `gcc --version`, and install it via package managers such as `apt` or `yum`.
- macOS: Use Homebrew with the command `brew install gcc`.
Compiling the Exported Code
Once your environment is ready, compile the C code using the terminal:
gcc gcc_phat_c.c -o gcc_phat_output -lm
In this command:
- `gcc_phat_c.c` is your generated C file.
- `-o gcc_phat_output` sets the name for the compiled output executable.
- `-lm` links the math library, necessary for using mathematical functions.
This step transforms your MATLAB algorithm into an executable C program.
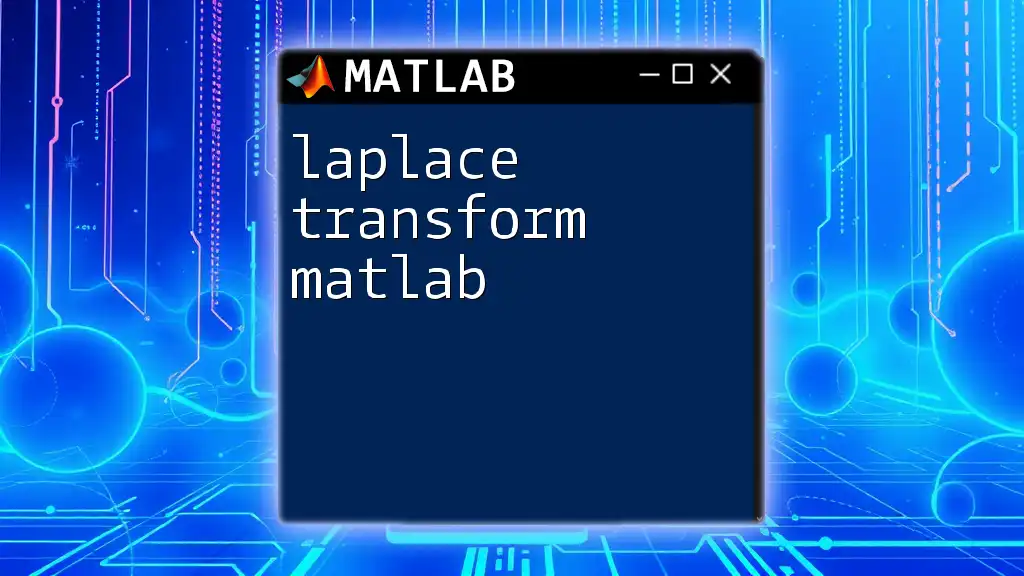
Testing the Exported C Code
Validating the Output
After compilation, validating the correctness of the C output is crucial. Run your executable using a test input file to ensure that the output aligns with the results obtained in MATLAB. Utilizing known inputs and expected outputs can streamline this process.
Performance Comparison
Once validated, assess the performance of your C implementation. Key metrics to evaluate include execution speed and memory usage. Optimize the C code if necessary, especially if performance falls short of expectations.
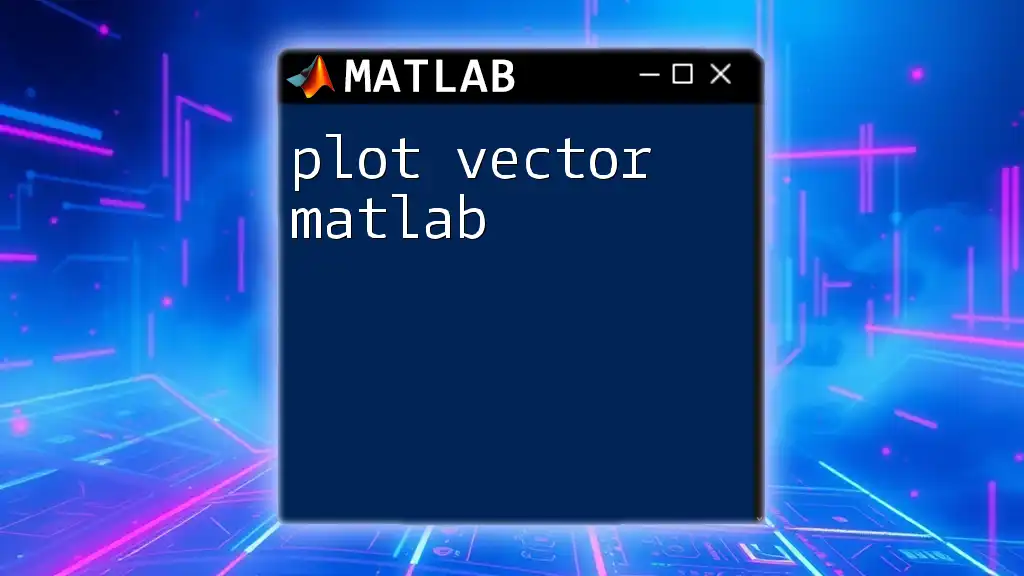
Troubleshooting Common Issues
Common Errors during Export
Exporting from MATLAB to C can lead to various issues such as unsupported MATLAB functions or data types. If you encounter error messages, consult MATLAB documentation or forums for insights.
Debugging the Compiled C Code
Should there be issues in your C output, debugging becomes critical. Utilize debugging tools like GDB (GNU Debugger) to step through your code and identify discrepancies. Print statements can also help trace the execution flow, allowing for better diagnosis of potential problems.
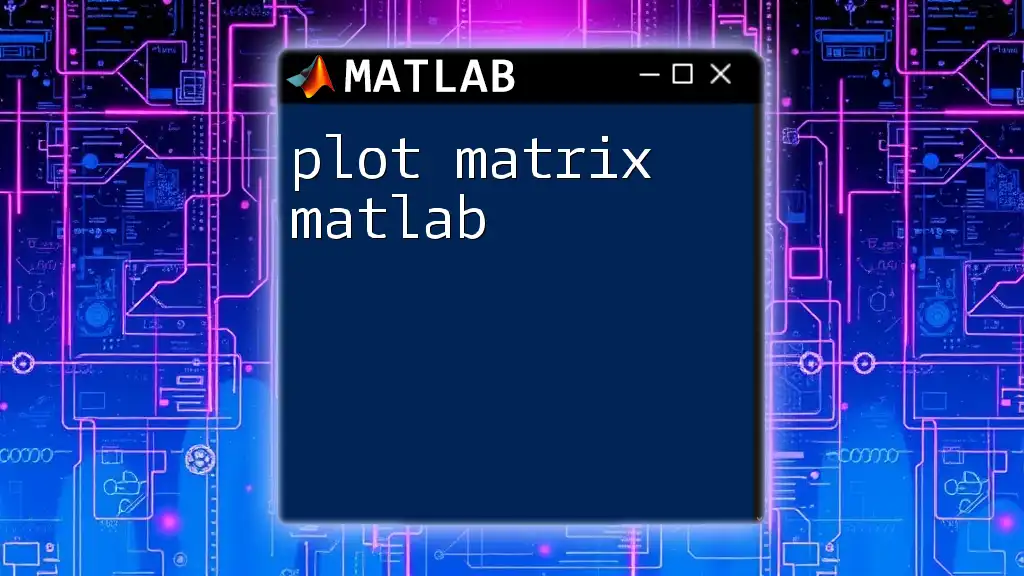
Conclusion
The journey to export gcc phat from matlab to c is not just a valuable exercise in optimizing your signal processing tasks, but also a way to leverage MATLAB's capabilities alongside high-performance C implementations. With the guidance provided in this article, you can confidently convert your MATLAB code, compile it, and validate its effectiveness in real-world applications.
In addition to the skills honed through this process, you are encouraged to dive deeper into MATLAB and C programming, exploring avenues such as online workshops and community forums to further enhance your coding skills.