Scientific notation in MATLAB is a way of displaying numbers that are either very large or very small by representing them in the form of a coefficient multiplied by a power of ten.
Here’s a code snippet that demonstrates how to use scientific notation in MATLAB:
% Define a number in scientific notation
number = 1.23e4; % This is equal to 1.23 x 10^4
disp(number);
What is Scientific Notation?
Scientific notation is a way of expressing very large or very small numbers in a concise format. It consists of two parts: a coefficient and an exponent. For instance, the number 5,000 can be represented as 5 x 10^3, while 0.00034 can be expressed as 3.4 x 10^-4. This method of notation allows for easier readability and arithmetic calculations, especially when dealing with numbers that can span several orders of magnitude.
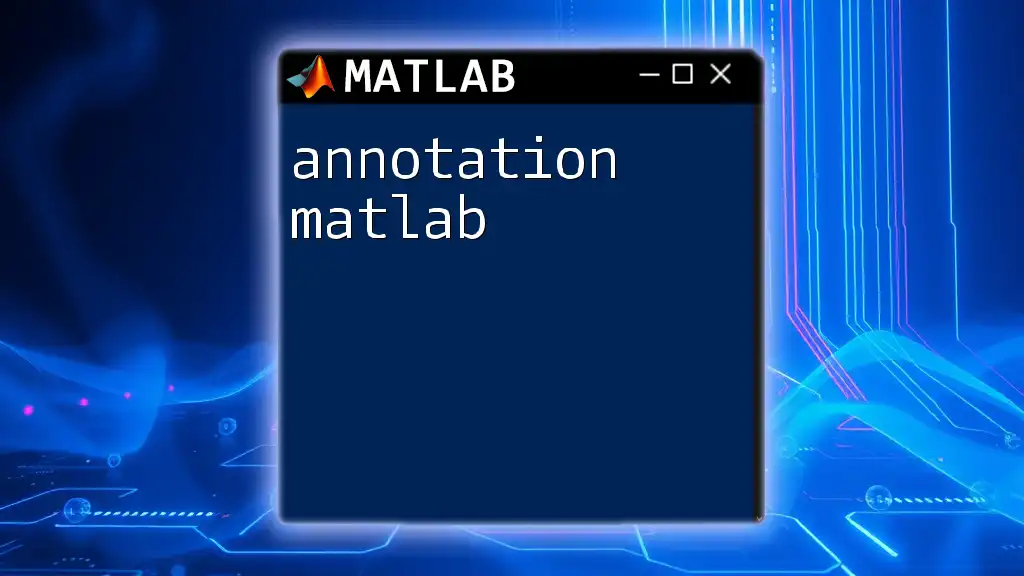
Importance of Scientific Notation in MATLAB
In MATLAB, scientific notation plays a crucial role in enhancing the visibility of numerical data. When working with large datasets or complex calculations, scientific notation allows for straightforward comprehension and manipulation of values. For example, instead of writing out several zeros or decimal places, you can simplify your code and results by using scientific notation.
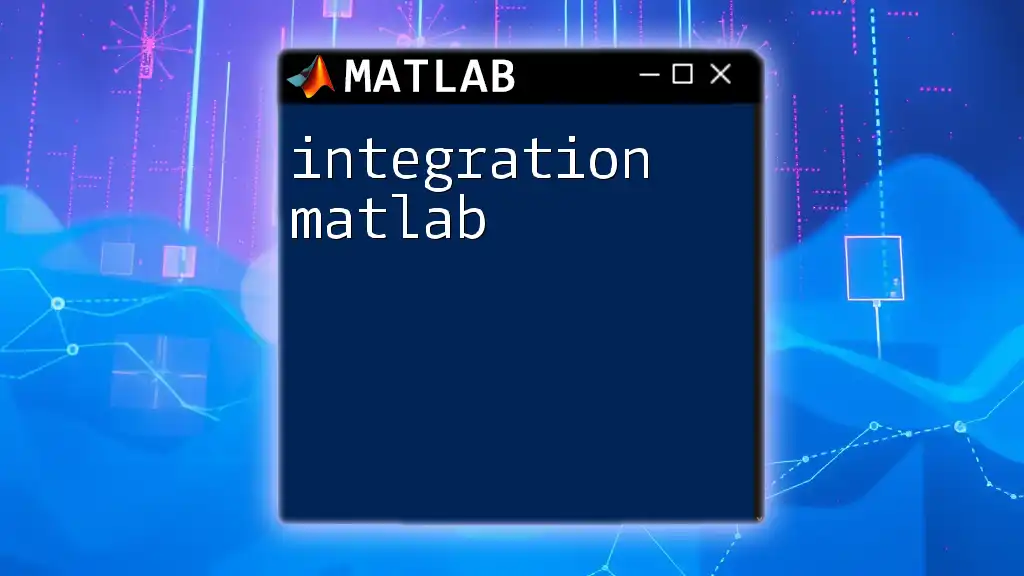
Understanding Scientific Notation
Base and Exponent
Scientific notation typically uses a base of 10. The exponent indicates how many times the base must be multiplied by itself. For instance, in the case of 6.02 x 10^23 (Avogadro's number), the base is 10, and the exponent is 23, which means you multiply 10 by itself 23 times to derive the full number.
Simple Examples
Converting numbers into scientific notation can clarify their size and give context for comparison. For instance:
- 5000 can be converted to 5 x 10^3
- 0.00034 can be expressed as 3.4 x 10^-4
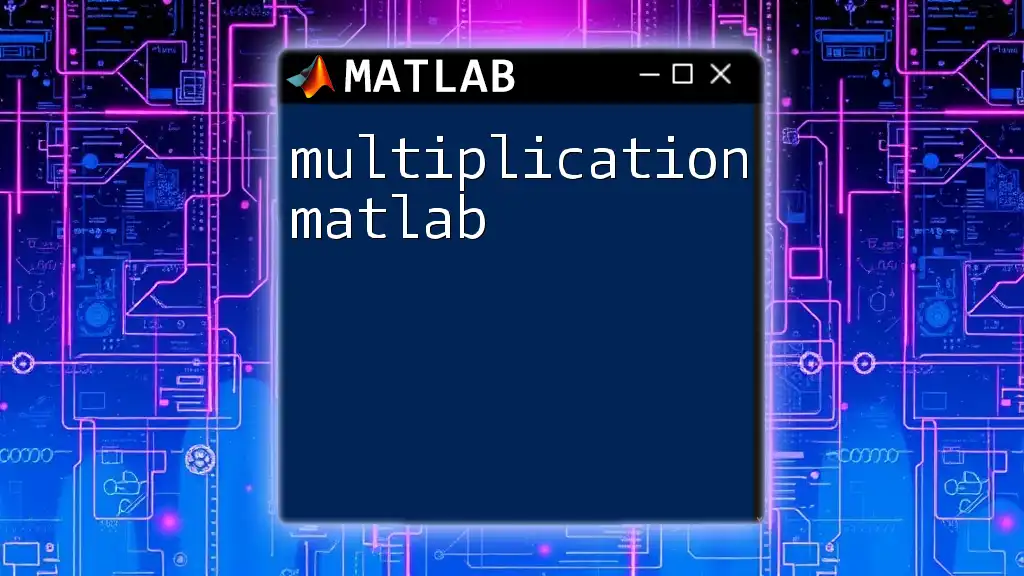
Working with Scientific Notation in MATLAB
Displaying Numbers in Scientific Notation
In MATLAB, displaying numbers in scientific notation is straightforward with the `format` command. This command changes how numbers are displayed without affecting their actual value.
You can use the following syntax:
format short e
disp(0.00012)
This will display 1.20e-04, representing 0.00012 in scientific notation. The `short` option provides a compact view, while `long` offers more significant digits.
Converting Numbers to Scientific Notation
For more controlled formatting, you can use MATLAB's `sprintf` function. This function allows for precision in how numbers are formatted as strings. The syntax is as follows:
formattedNum = sprintf('%.2e', number)
For example:
num = 1234.567;
formattedNum = sprintf('%.2e', num);
disp(formattedNum) % Output: 1.23e+03
This command converts 1234.567 into scientific notation as 1.23e+03.
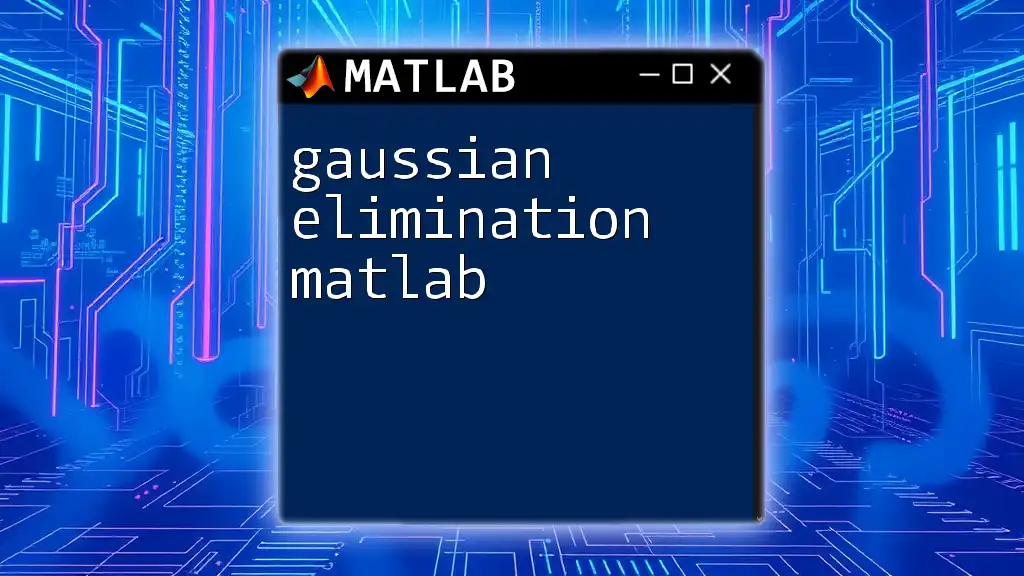
Setting Scientific Notation in MATLAB Figures
Controlling Axes in Plots
When plotting data in MATLAB, you may want to use scientific notation for your axes. This can be accomplished by setting a logarithmic scale using:
set(gca, 'XScale', 'log')
Here’s an example of how to use this in a plot:
x = logspace(0, 3, 100);
y = x.^2;
plot(x, y);
set(gca, 'XScale', 'log')
xlabel('X Axis (Log Scale)');
ylabel('Y Axis');
title('Plot with Logarithmic Scale');
In this case, the x-axis will be displayed in logarithmic scale, enhancing the readability of large datasets.
Automatic Scientific Notation for Axes
MATLAB automatically applies scientific notation for axis labels when the values exceed a certain range. This feature not only saves space but also helps maintain clarity when dealing with exponential data.
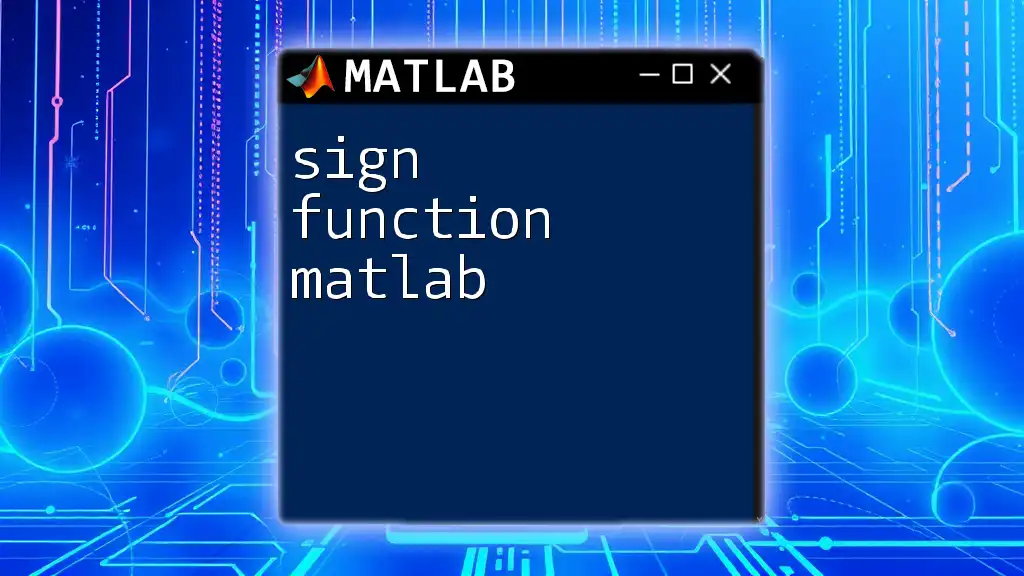
Performing Calculations with Scientific Notation
Using MATLAB’s Built-in Functions
MATLAB handles scientific notation seamlessly during arithmetic operations. For example, if you multiply numbers expressed in scientific notation, MATLAB automatically understands the context:
a = 3.2e5; % 320000
b = 2.1e3; % 2100
result = a * b; % Automatic handling of scientific notation
disp(result) % Output: 672000000
Here, MATLAB will compute the result without requiring any extra steps regarding the scientific notation.
Issues with Precision
It’s important to note that precision can vary depending on the data types you use within MATLAB. By default, MATLAB uses double-precision floating-point numbers, but you may encounter scenarios where single-precision is necessary. Always check your variables to ensure they handle values accurately.
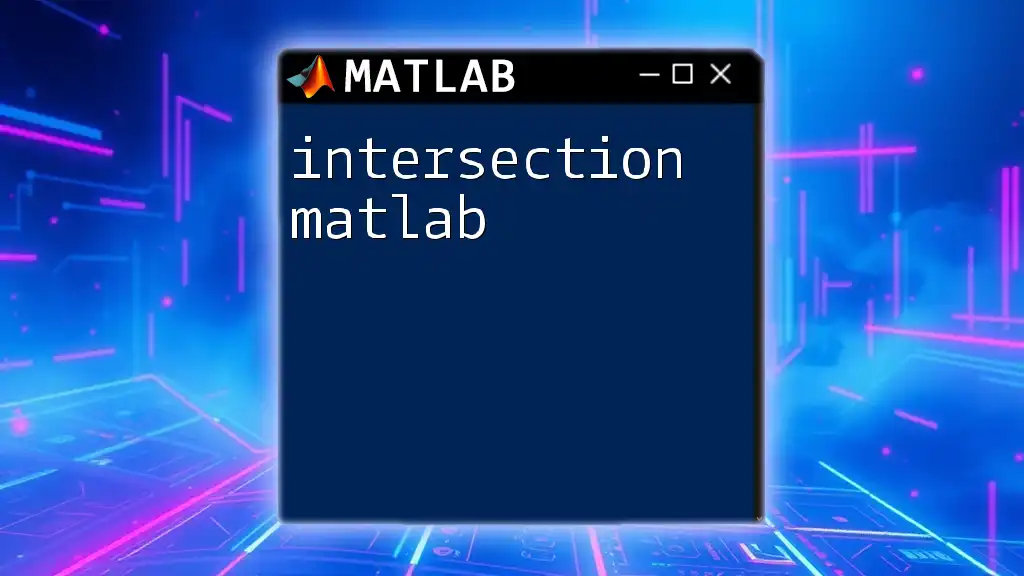
Advanced Topics in Scientific Notation
Custom Formatting Output
In some cases, you may want to specify a more customized output format. Using the `fprintf` function, you can achieve this level of detail. For example:
fprintf('%.3e\n', 56789.01);
This command formats the number to show three digits after the decimal point in scientific notation.
Creating a Custom Function for Input Handling
Creating a custom function can streamline the formatting process of your scientific notation. Here’s a simple example:
function formatted = formatScientific(num)
formatted = sprintf('%.2e', num);
end
You can call this function and pass any number to see it formatted in scientific notation:
result = formatScientific(123456);
disp(result) % Output: 1.23e+05
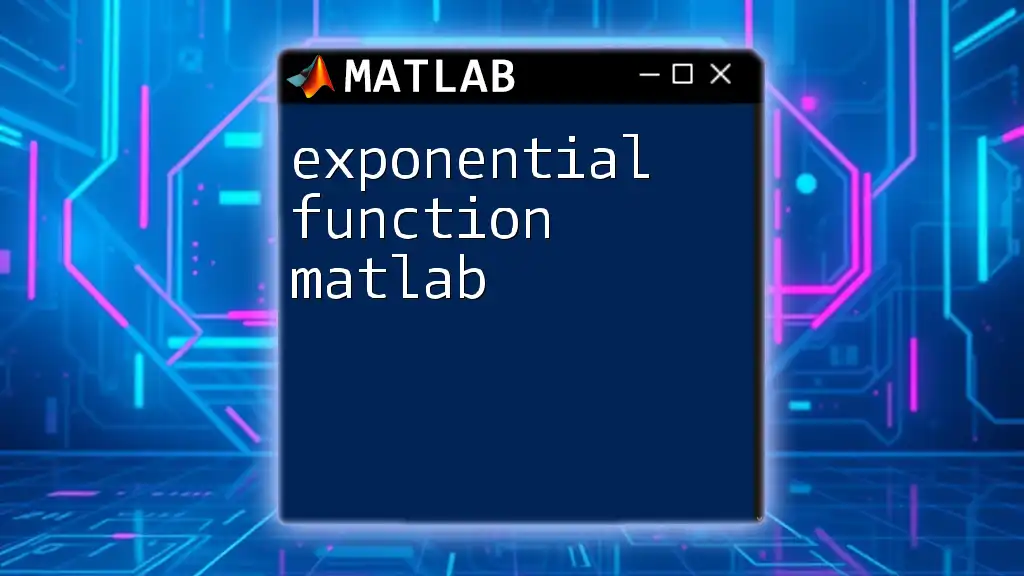
Conclusion
Scientific notation is a powerful tool in MATLAB that enhances both clarity and efficiency when dealing with numerical data. Understanding how to manipulate and display numbers in scientific notation allows for sophisticated data analysis and visualization. By practicing these techniques and utilizing MATLAB's functionalities, users can significantly improve their programming skills and data handling capabilities.
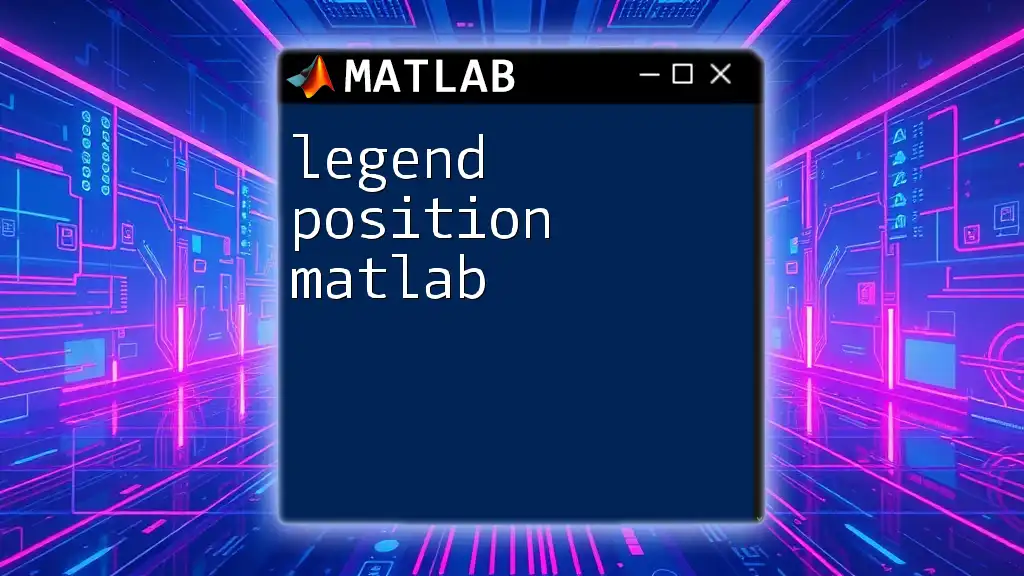
Additional Resources
For further reading, explore MATLAB's official documentation, which offers in-depth guidance on using scientific notation effectively. Additionally, consider looking into recommended books and tutorials that cover MATLAB programming in detail.
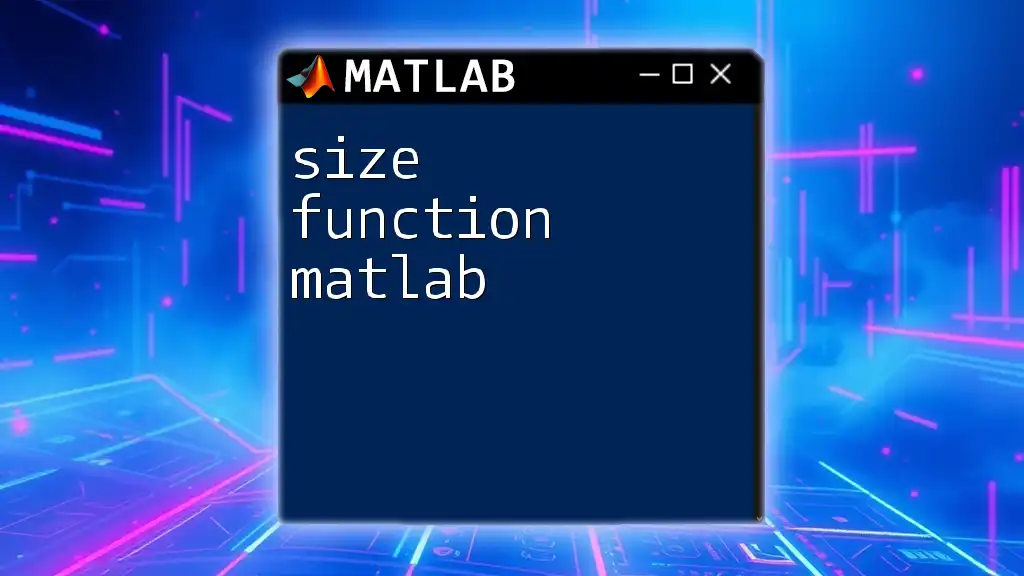
FAQs
Common Questions About Scientific Notation in MATLAB
-
What is the difference between `format short e` and `format long e`? `format short e` provides a shorter representation of numbers in scientific notation, while `format long e` displays more decimal points, giving a more precise representation.
-
How does MATLAB handle arithmetic with scientific notation? MATLAB automatically manages the conversion and output of scientific notation during calculations, simplifying operations with large and small numbers.
By equipping yourself with the information presented in this article, you will be well-prepared to navigate scientific notation effectively within MATLAB programming.