Steady state error in MATLAB refers to the difference between the desired final output and the actual output of a system as time approaches infinity, commonly analyzed in control systems.
Here's a simple code snippet to illustrate how to calculate steady state error using a unity feedback system in MATLAB:
% Define system parameters
K = 5; % Gain
num = K; % Numerator of the transfer function
den = [1 2 5]; % Denominator of the transfer function
% Create the transfer function
sys = tf(num, den);
% Define the reference input (step input)
t = 0:0.01:10; % Time vector
r = ones(size(t)); % Step input
% Get system response
[y, t] = step(sys, t);
% Calculate steady state error
steady_state_error = r(end) - y(end);
fprintf('Steady State Error: %f\n', steady_state_error);
What is Steady State Error?
Steady state error refers to the difference between the desired output (reference input) and the actual output of a control system as time approaches infinity. Essentially, it indicates how well a control system can reach and maintain the target value in response to various types of inputs, such as step, ramp, or even more complex signals. A system with zero steady state error is considered to perfectly track its reference input.
Types of Steady State Error
Understanding the different types of steady state error is crucial for designing effective control systems:
-
Position Error: This type of error occurs when a system is trying to reach a specific position. For example, a motor controlling the position of a robotic arm may have a position error if it doesn’t reach its intended location.
-
Velocity Error: This error type comes into play when a system’s output velocity does not match the desired velocity. For instance, a car's speed may not match the driver's command due to friction and inertia.
-
Acceleration Error: This error occurs during changes in speed; it indicates how well a system can adjust its acceleration to match the input signal, important in applications like drones or automated vehicles.
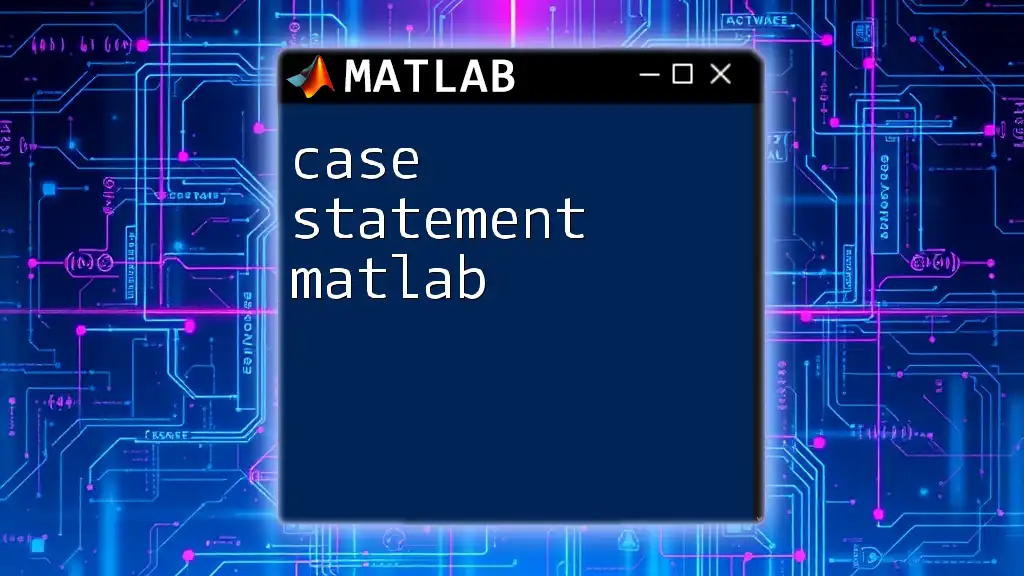
The Role of MATLAB in Analyzing Steady State Error
Benefits of Using MATLAB
MATLAB is a powerful tool for analyzing steady state error due to several reasons:
- Efficiency: MATLAB’s ability to perform complex calculations quickly saves time compared to manual calculations.
- Visual Representation: The software allows for easy visualization of responses through plots, making it easier to understand system behavior.
- Built-in Functions: MATLAB offers extensive functions and toolboxes that cater explicitly to control systems, simplifying the analysis process.
MATLAB Toolboxes for Control Systems
To analyze steady state error effectively, it’s essential to use the right MATLAB toolboxes:
- Control System Toolbox: This toolbox provides functions for the analysis and design of linear control systems, including functions for creating transfer functions and computing step responses.
- System Identification Toolbox: Useful for creating mathematical models of systems based on measured data, which can lead to more accurate descriptions of steady state behavior.
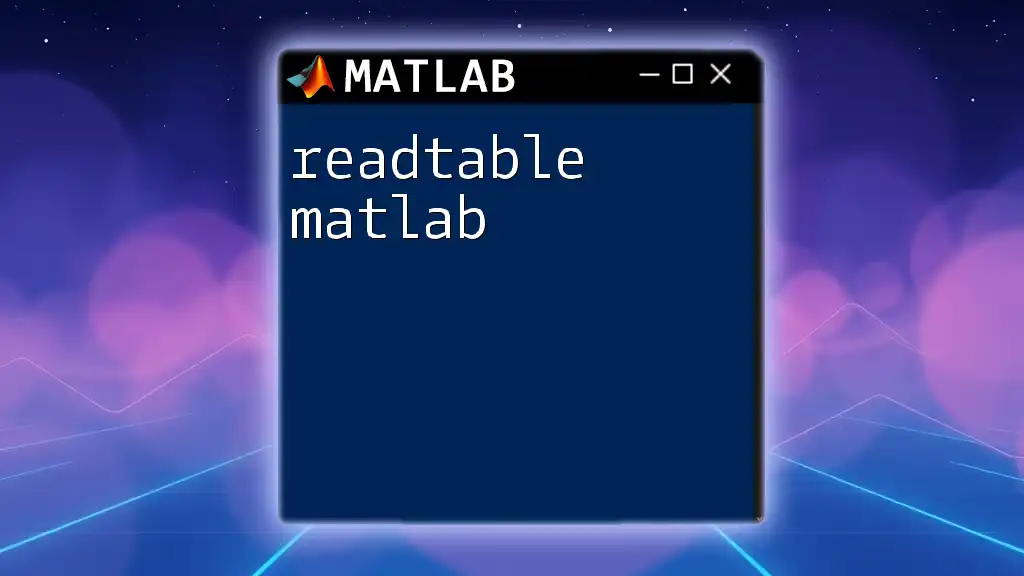
Understanding Control System Types
Open-Loop vs Closed-Loop Systems
Open-loop systems do not use feedback to determine if the desired output was achieved. As a result, they may exhibit significant steady state errors because they cannot self-correct based on output. For example, a simple water heater that runs for a fixed time without sensing the actual water temperature might produce inconsistent results.
In contrast, closed-loop systems use feedback to continuously adjust their output. This capability allows closed-loop control systems to minimize steady state errors effectively. An example of a closed-loop system is a thermostat-controlled heating system, which adjusts the heat output based on the temperature measured by a sensor.
Unity Feedback Systems
Unity feedback systems are a specific type of closed-loop system where the feedback loop is comprised of a direct connection from the output back to the input level without any gain. In these systems, the steady state error can often be determined simply and provides valuable insights into how well the system responds.
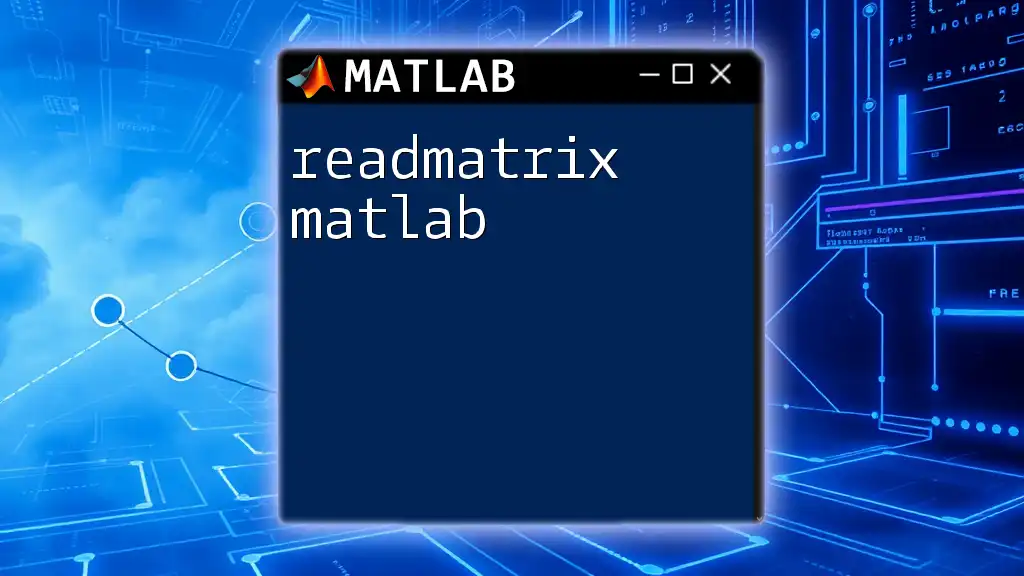
MATLAB Commands for Steady State Error
Basic MATLAB Commands
To analyze and compute steady state error in MATLAB, the following commands are essential:
- `step()`: Used for simulating the step response of a system.
- `lsim()`: Simulates the time response of a system to arbitrary inputs.
- `tf()`: Defines a system in terms of its transfer function.
Example Code Snippet: Step Response Analysis
To illustrate how to compute steady state error using MATLAB, consider the following example:
% Define system transfer function
num = [1]; % Numerator coefficients
den = [1, 3, 2]; % Denominator coefficients
system = tf(num, den);
% Step response
[y, t] = step(system);
% Calculate steady state error
steady_state_output = y(end);
reference_input = 1; % Assuming a step input of 1
steady_state_error = reference_input - steady_state_output;
fprintf('Steady State Error: %.4f\n', steady_state_error);
Explanation of the Code
- Define the Transfer Function: The transfer function is specified using numerator and denominator coefficients, which describe the system’s dynamics.
- Simulate Step Response: The `step()` function generates the system's response over time, providing output data that reveals how the system behaves in response to a step input.
- Calculate Steady State Error: The last value of the output array (`y(end)`) is compared to the desired reference input (in this case, 1) to compute the error.
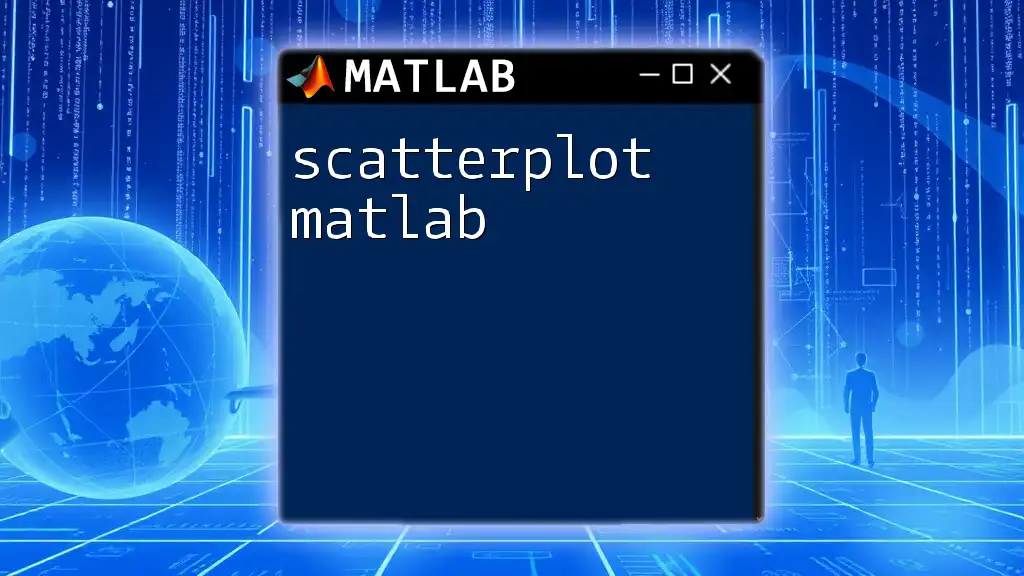
Calculating Steady State Error Using MATLAB
Steady State Error Formula
The formula for calculating steady state error can differ based on the type of input signal and system characteristics. Generally, the steady state error (Ess) for a system can be expressed as:
\[ E_{ss} = R - Y_{ss} \]
Where:
- \( R \) is the reference input.
- \( Y_{ss} \) is the steady state output.
Example: Steady State Error Calculation
Here’s how to perform the steady state error calculation using a simple MATLAB function:
% Define a second-order system
num = [1];
den = [1, 2, 1];
system = tf(num, den);
% Compute steady state error for a step input
t = 0:0.1:10;
[y, t] = step(system, t);
error = 1 - y(end); % Assuming step input is 1
disp('Steady State Error for Step Input:');
disp(error);
Visualizing the Response
Plotting system response is critical to interpreting results. Here’s an example of how you can visualize the step response:
plot(t, y);
grid on;
xlabel('Time (s)');
ylabel('System Output');
title('System Step Response');
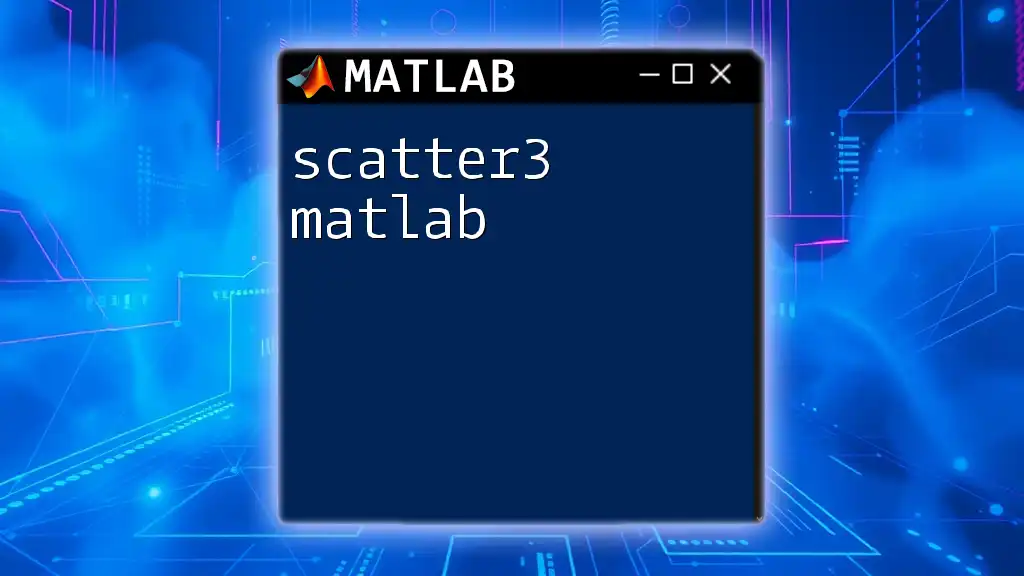
Common Scenarios and Troubleshooting
Typical Steady State Errors
Several factors can contribute to steady state error:
- System Gain: Insufficient gain can lead to significant steady state errors, particularly in feedback systems.
- Time Constants: Large time constants can slow the system response, affecting the error value.
Debugging in MATLAB
When working with MATLAB, common pitfalls to avoid include:
- Incorrectly defining the transfer function: Ensure the numerator and denominator coefficients accurately represent your system.
- Misinterpreting output: Always double-check calculations of the error and ensure you're referencing the correct steady state conditions.
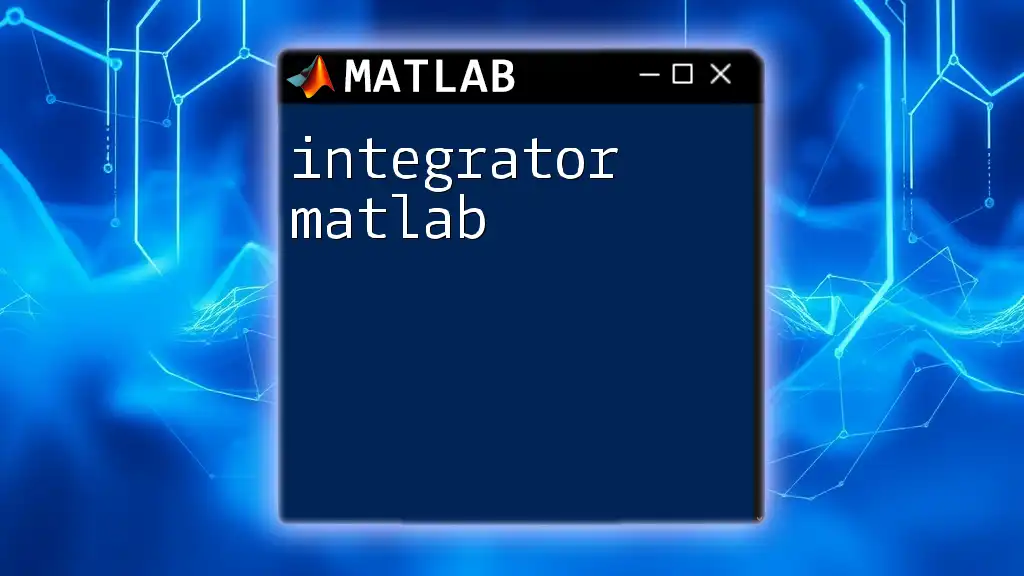
Conclusion
In conclusion, understanding and calculating steady state error in MATLAB is vital for designing effective control systems. By using MATLAB's powerful tools and commands, engineers can analyze how systems behave in steady state conditions and optimize their designs accordingly. Practicing these concepts will not only enhance your MATLAB skills but also improve your overall comprehension of control systems. Embrace the opportunity to experiment with the provided MATLAB commands, and you'll be well on your way to mastering steady state error analysis.
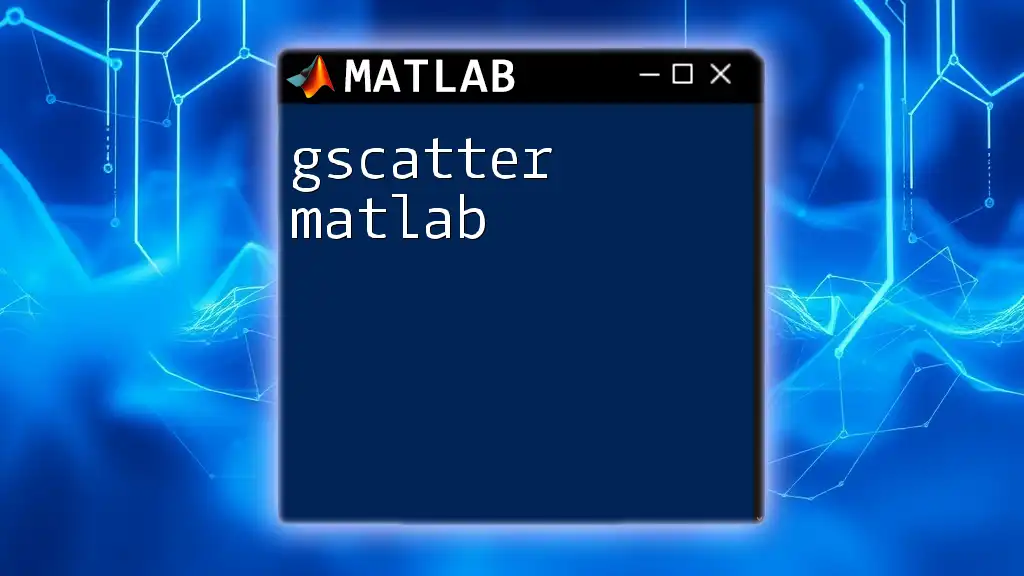
Additional Resources
For further insights into MATLAB and control systems, consider exploring:
- The official MATLAB documentation to deepen your understanding of command functionalities.
- Recommended books on control systems for theoretical knowledge and practical application.
- Online forums and communities where MATLAB enthusiasts share their knowledge and experiences.
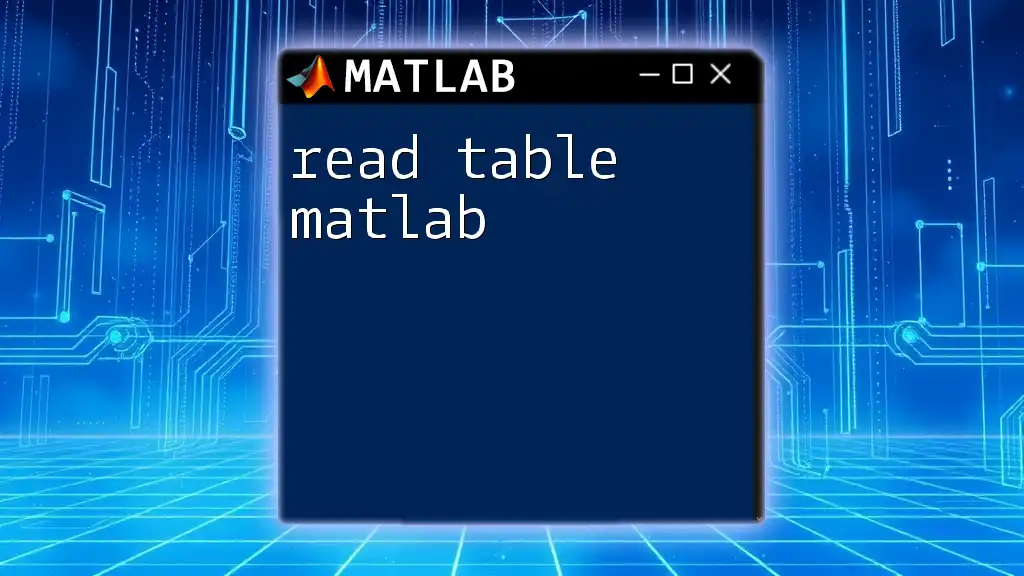
Call to Action
Now that you’re equipped with the knowledge to tackle steady state error calculations in MATLAB, it’s time to put this into practice! Try running the examples provided, calculate the steady state errors for different systems, and share your findings or inquiries in the comments below. Happy coding!