The `kron` function in MATLAB computes the Kronecker tensor product of two matrices, which results in a block matrix that combines all combinations of the elements from the input matrices.
% Example of using kron to compute the Kronecker product of two matrices
A = [1, 2; 3, 4];
B = [0, 5; 6, 7];
C = kron(A, B);
What is `kron`?
The `kron` function in MATLAB computes the Kronecker product of two matrices. This operation combines them in a way that is both mathematically interesting and useful for various applications. The Kronecker product merges two matrices \( A \) and \( B \) to produce a larger matrix \( C \), defined mathematically as follows:
If \( A \) is of size \( m \times n \) and \( B \) is of size \( p \times q \), then the resulting matrix \( C \) will be of size \( (m \times p) \times (n \times q) \).
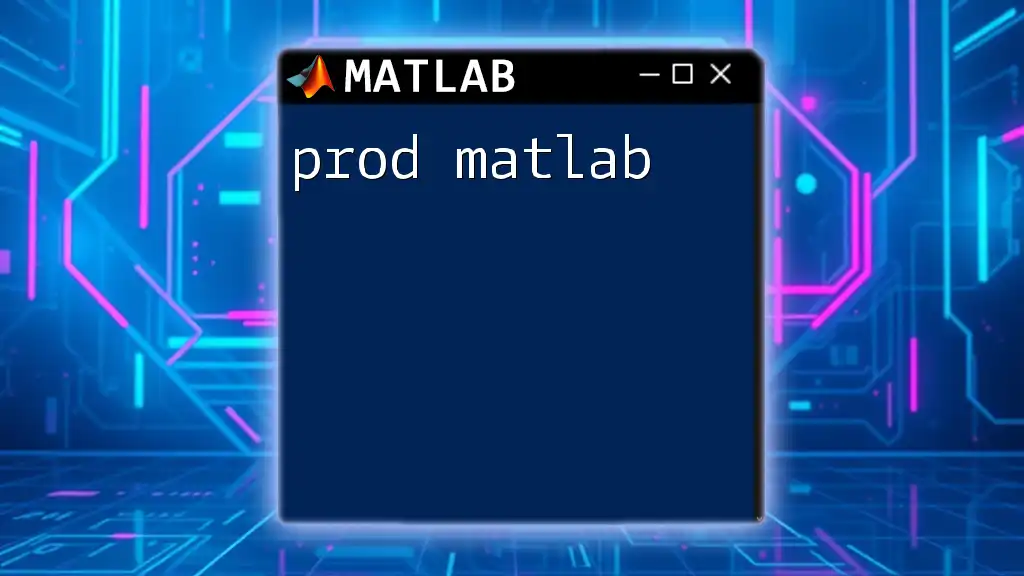
Why Use `kron`?
The Kronecker product has several applications across different fields, including signal processing, machine learning, and image processing. Understanding how to use `kron` enables you to perform complex transformations and calculations with ease.
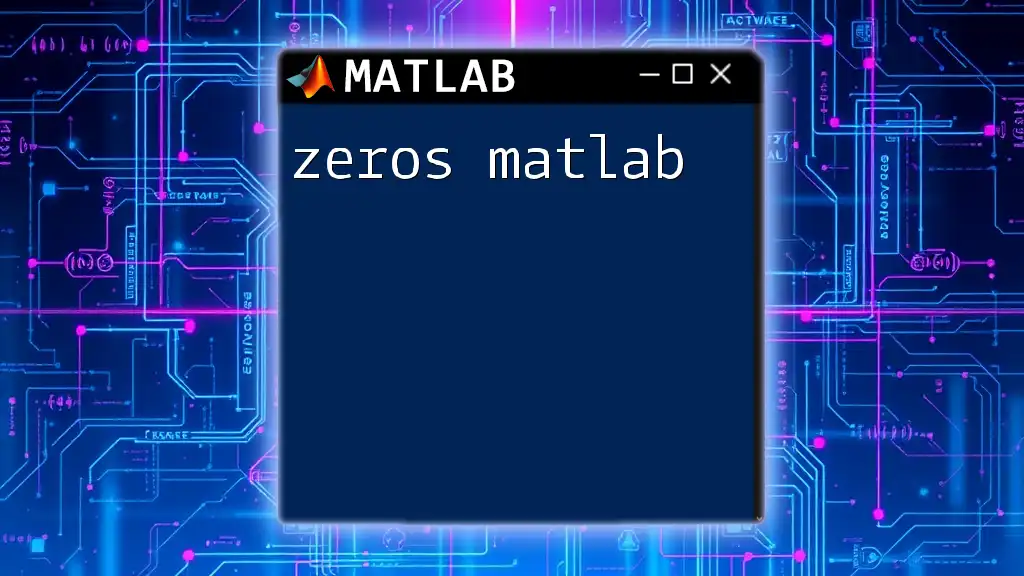
Definition of Kronecker Product
In mathematical terms, the Kronecker product \( C = A \otimes B \) is formed by multiplying each element of \( A \) by the entire matrix \( B \). For example, if
\[ A = \begin{bmatrix} a_{11} & a_{12} \\ a_{21} & a_{22} \end{bmatrix} \]
and
\[ B = \begin{bmatrix} b_{11} & b_{12} \\ b_{21} & b_{22} \end{bmatrix} \]
then
\[ C = \begin{bmatrix} a_{11}B & a_{12}B \\ a_{21}B & a_{22}B \end{bmatrix} \]
This creates a large matrix where each matrix \( B \) is scaled by each element of \( A \).
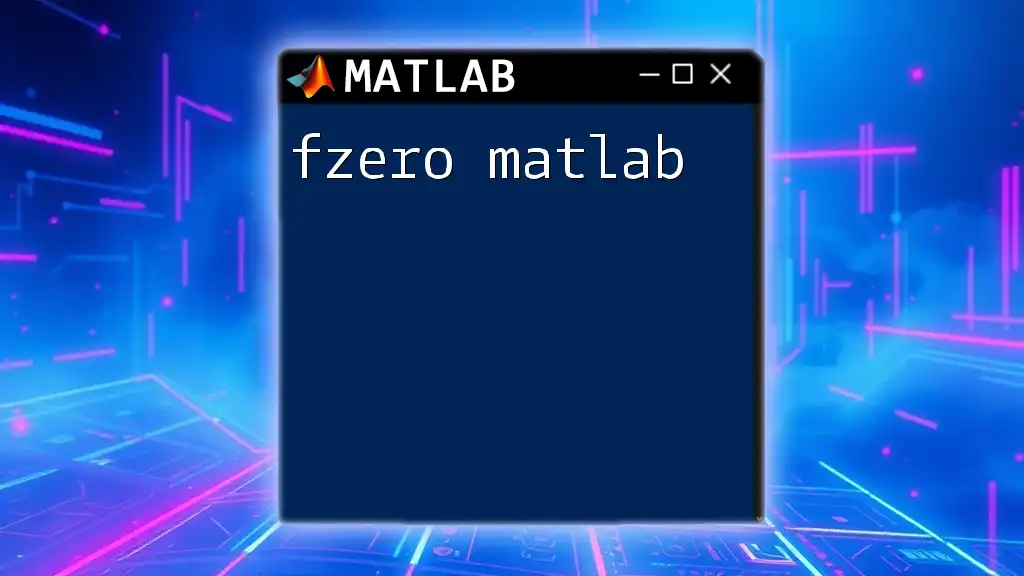
Properties of the Kronecker Product
Understanding the properties of the Kronecker product can provide insights into its behavior and facilitate its application:
-
Associativity:
\[ A \otimes (B \otimes C) = (A \otimes B) \otimes C \] -
Distributive Property:
\[ A \otimes (B + C) = A \otimes B + A \otimes C \] -
Mixed Product Property:
\[ (A \otimes B)(C \otimes D) = (AC) \otimes (BD) \]
These properties can greatly simplify computations in larger applications.
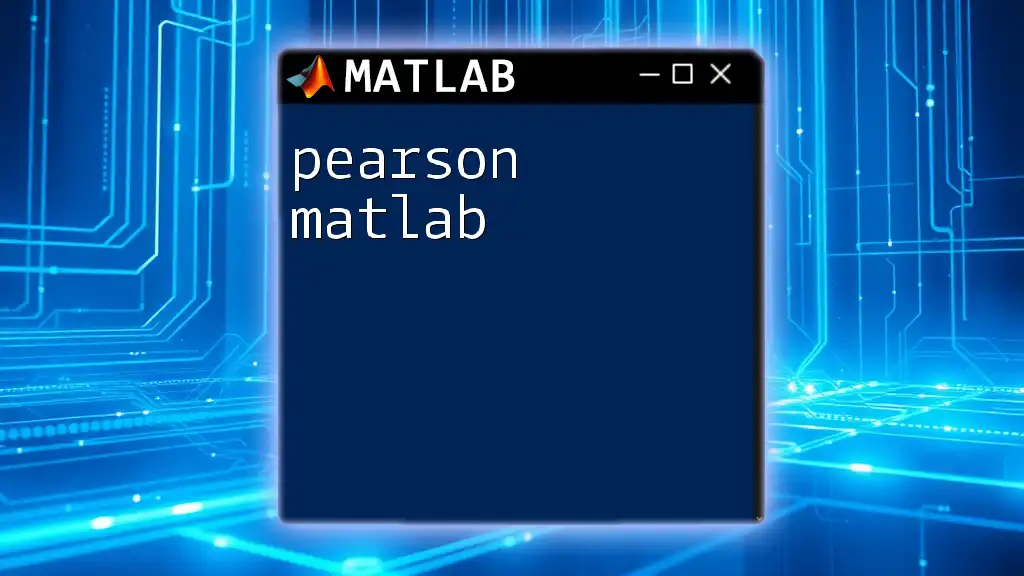
Basic Syntax
The syntax for using the `kron` function in MATLAB is straightforward:
C = kron(A, B)
In this command:
- `A` and `B` are the input matrices.
- `C` is the resulting Kronecker product of `A` and `B`.
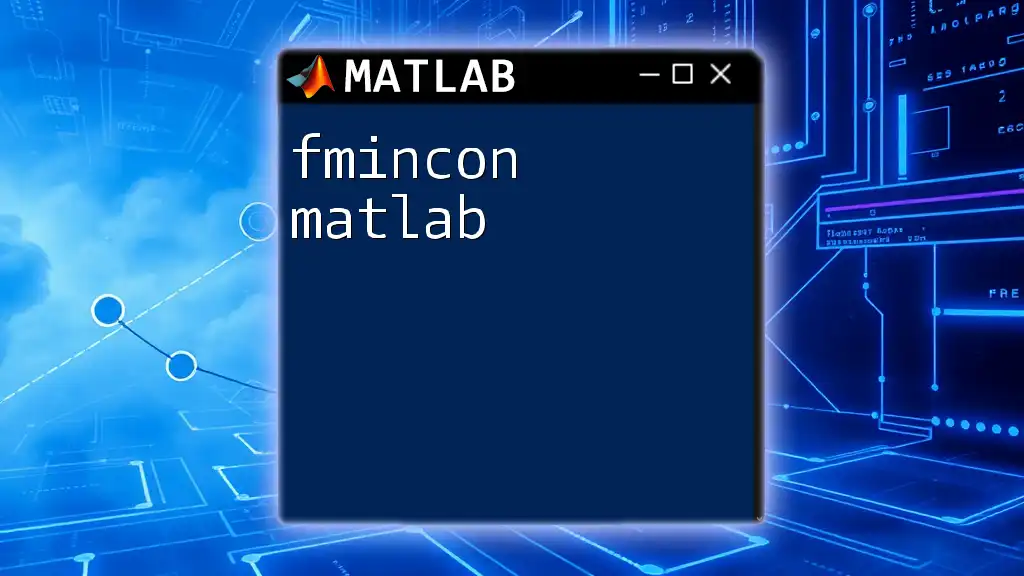
Common Use Cases
The `kron` function is particularly useful for tasks such as:
- Mathematical modeling: Generating higher-dimensional spaces from lower-dimensional representations.
- Tensor computations: Useful in machine learning and applied mathematics.
- Signal processing: Creating filters and manipulating signals.
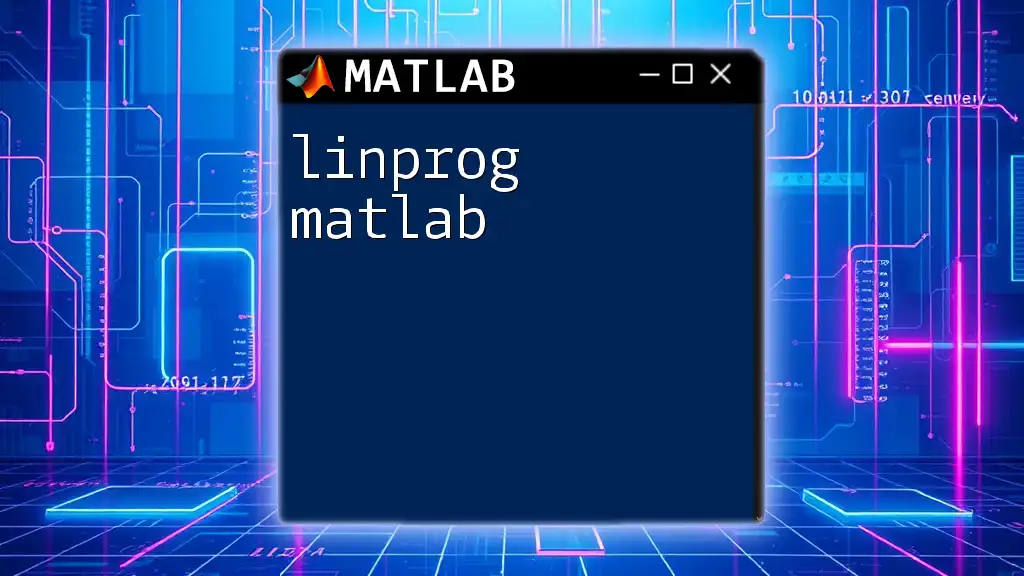
Examples of `kron` in Action
Example 1: Simple 2D Matrices
Consider two simple 2D matrices:
A = [1, 2; 3, 4];
B = [0, 5; 6, 7];
result = kron(A, B);
disp(result);
The output of this command will yield a \( 4 \times 4 \) matrix, where each element of \( A \) multiplies matrix \( B \).
Example 2: Higher-Dimensional Cases
The `kron` function can also handle matrices of different dimensions:
A = [1, 2; 3, 4];
B = [5; 6];
result = kron(A, B);
disp(result);
In this case, the resulting matrix will exhibit columns that are scaled versions of matrix \( B \) influenced by the corresponding element in \( A \).
Example 3: Using `kron` with Identity Matrices
Identity matrices can help maintain specific structural properties in matrix calculations:
A = eye(3);
B = [1, 0; 0, 1];
result = kron(A, B);
disp(result);
Here, the identity matrix expands the dimensions of matrix \( B \) without modifying its values, making it especially useful when working with complex systems.
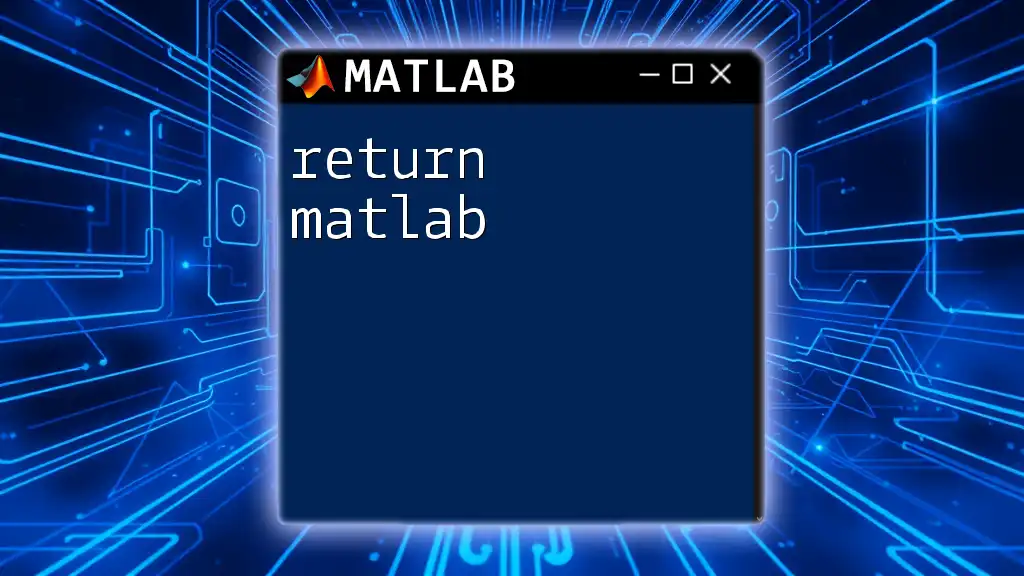
Performance Considerations
When utilizing the `kron` function, it’s essential to consider computational efficiency, especially for large matrices. The size of the output matrix can grow rapidly, leading to possible memory issues.
The Kronecker product involves a time complexity of approximately \( O(mnp) \), where \( m \) and \( n \) are the dimensions of matrix \( A \), and \( p \) and \( q \) are the dimensions of matrix \( B \). Thus, handling large matrices can be computationally intensive and may require performance optimization techniques.
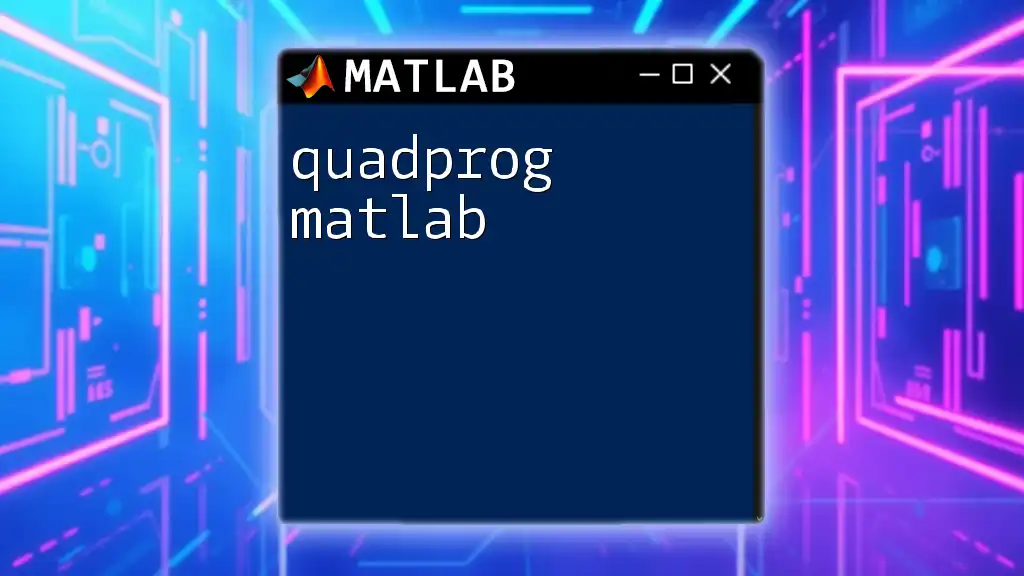
Applications of `kron`
Image Processing
In image processing, `kron` can be used to manipulate pixel grids, often employed in scenarios like resizing or filtering images, where interactions between different parts of the image are essential.
Signal Processing
In the realm of signal processing, the Kronecker product facilitates the transformation of signals, allowing for efficient implementation of certain filtering and processing techniques.
Machine Learning
`kron` is commonly applied in tensor computations, such as for creating complex neural network architectures that require multidimensional representations of data.
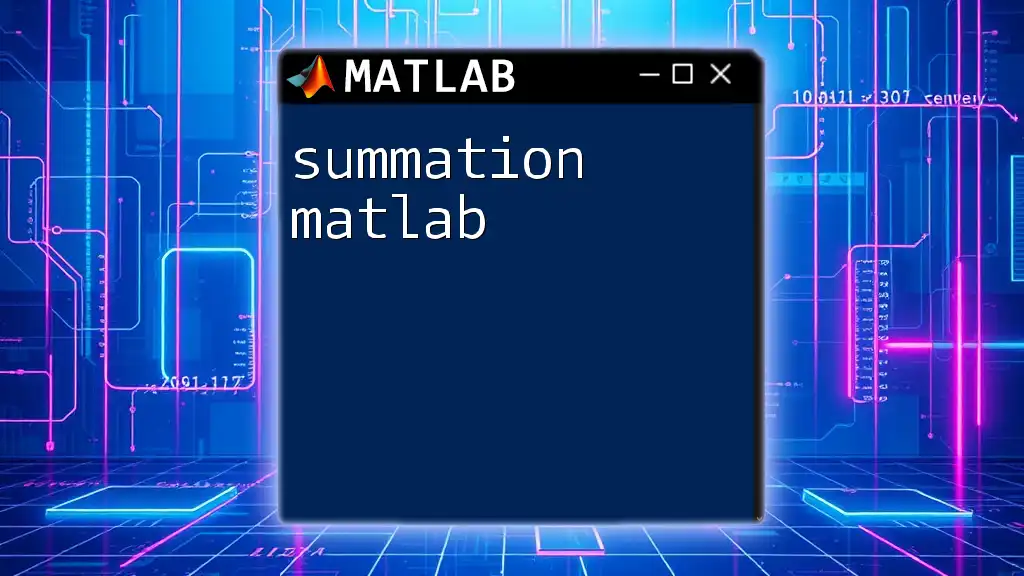
Troubleshooting Common Issues with `kron`
Mismatched Dimensions
One common problem when applying `kron` is mismatched dimensions between input matrices. Ensuring that matrices \( A \) and \( B \) are compatible for the desired outcome is crucial for obtaining correct results.
Data Type Compatibility
The types of the matrices should also be compatible. For example, mixing integers and floats can lead to unexpected results. Being mindful of data types ensures that you achieve accurate computations.
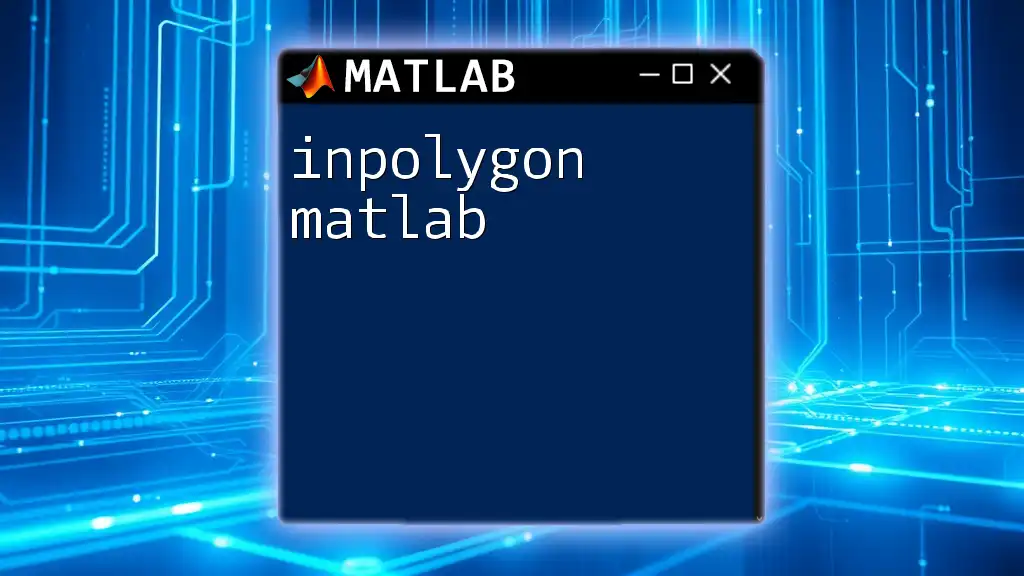
Advanced Uses of `kron`
Working with Sparse Matrices
Sparse matrices can be employed to enhance the efficiency of the `kron` function, especially when dealing with large datasets. Consider the following code snippet:
A = sparse([1, 0; 0, 1]);
B = [2, 3; 4, 5];
result = kron(A, B);
disp(result);
Here, the sparse nature of matrix \( A \) ensures that computational resources are used efficiently during the Kronecker product operation.
Kron Product of Higher-Dimensional Arrays
The `kron` function can also be applied to more than two dimensions, enabling complex maneuvers in higher-dimensional matrices and tensors. This flexibility is essential for various advanced applications in data science and engineering.
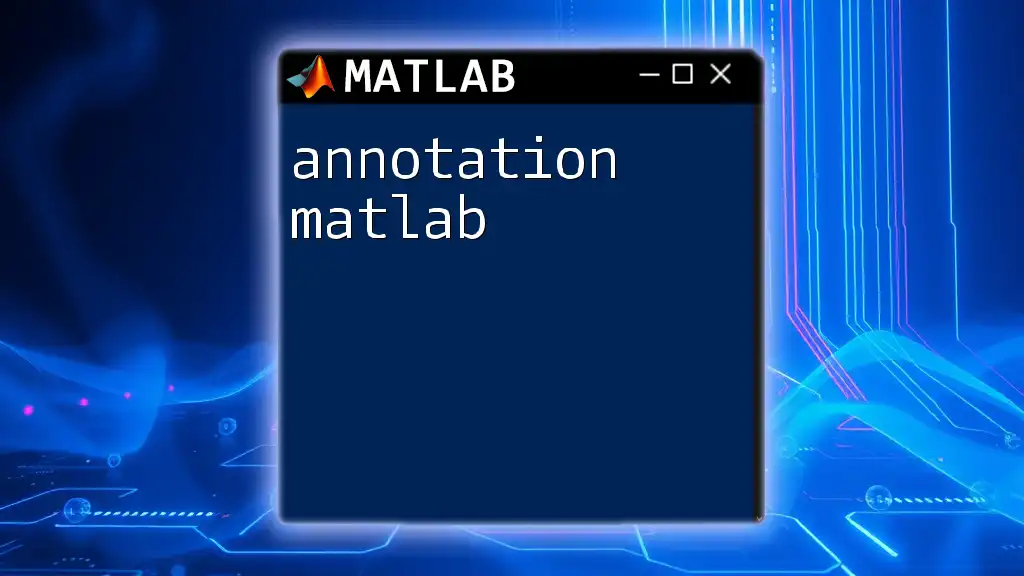
Recap of `kron` Importance
In summary, a thorough understanding of the `kron` function in MATLAB allows users to perform intricate mathematical operations efficiently and effectively. By mastering the Kronecker product, you can tackle advanced computations, optimize performance, and apply innovative solutions in your field.
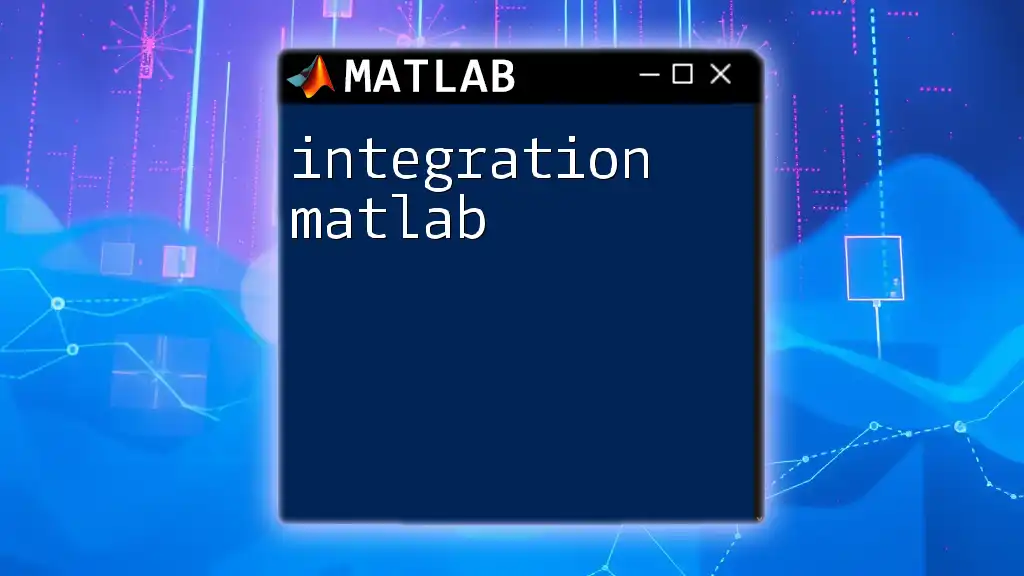
References and Further Reading
For more detailed information on `kron`, you can refer to the official MATLAB documentation and additional resources on matrix theory and its applications in MATLAB. Exploring these materials will deepen your understanding and broaden your skills in applying `kron` effectively in various scenarios.