In MATLAB, variance measures the spread of a set of data points around their mean, providing insights into data variability.
Here is a simple code snippet to calculate the variance of a dataset:
data = [10, 12, 23, 23, 16, 23, 21, 16]; % Sample data
variance = var(data); % Calculate variance
disp(variance); % Display the variance
What is Variance?
Variance is a statistical measurement that describes how far a set of data points are spread out from their average value. In essence, it quantifies the degree of dispersion or variability within a dataset. Understanding variance is critical for any data analysis, as it provides insight into trends and patterns, particularly when assessing risk and uncertainty in fields like finance, engineering, and scientific research.
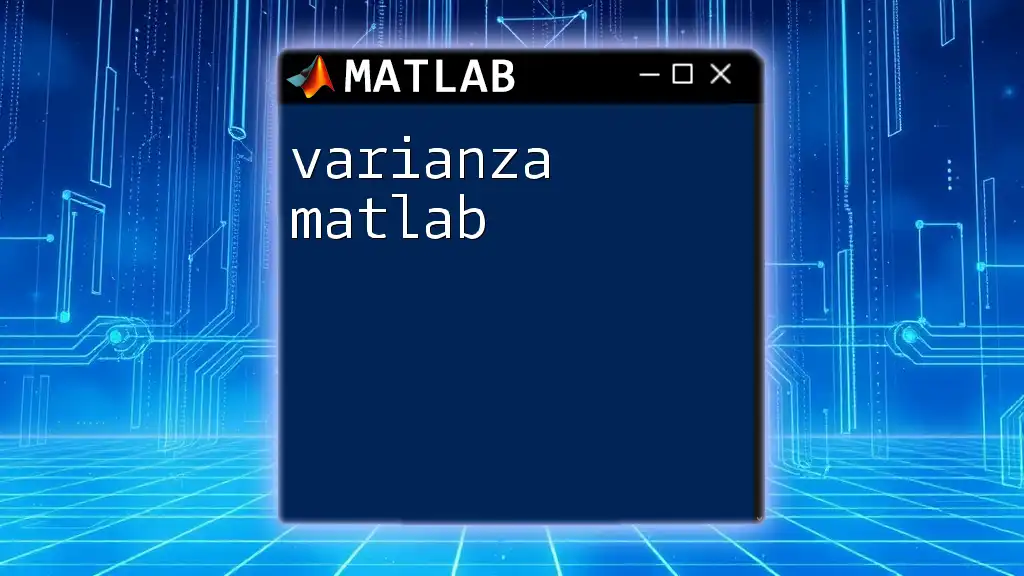
Applications of Variance
Variance plays an essential role in numerous disciplines. In finance, for example, it helps investors assess the risk associated with security prices. In engineering, it is vital for understanding tolerances in manufacturing processes. In research, variance aids in interpreting experimental results and determining the reliability of conclusions drawn from data.
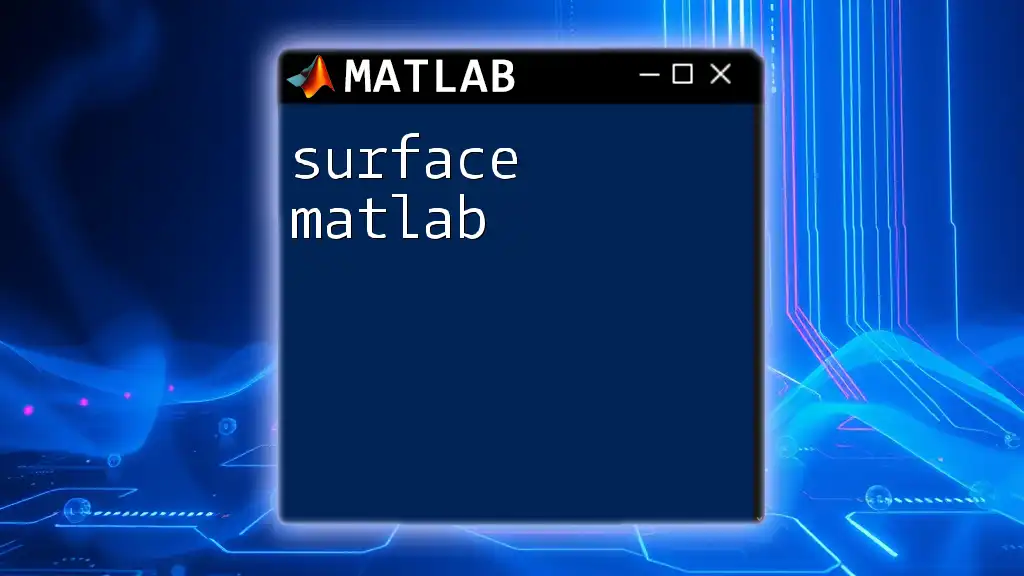
Mathematical Formula for Variance
The formula for calculating variance differs slightly depending on whether you are assessing a sample or a population:
-
Population Variance (σ²):
\[ \sigma^2 = \frac{\sum (x_i - \mu)^2}{N} \]
where \(x_i\) is each individual data point, \(\mu\) is the mean of the dataset, and \(N\) is the total number of data points. -
Sample Variance (s²):
\[ s^2 = \frac{\sum (x_i - \bar{x})^2}{n-1} \]
where \(\bar{x}\) is the sample mean, \(n\) is the number of observations, and the division by \(n-1\) instead of \(N\) corrects for bias in the estimation of the population variance.
Conceptual Understanding
Variance is a measure of how much the values in a dataset deviate from the mean. A low variance indicates that the data points tend to be close to the mean, while a high variance indicates that the data points are spread out over a larger range. This concept is closely related to standard deviation, which is simply the square root of the variance, and provides a more intuitive measure of spread since it is in the same units as the data points.
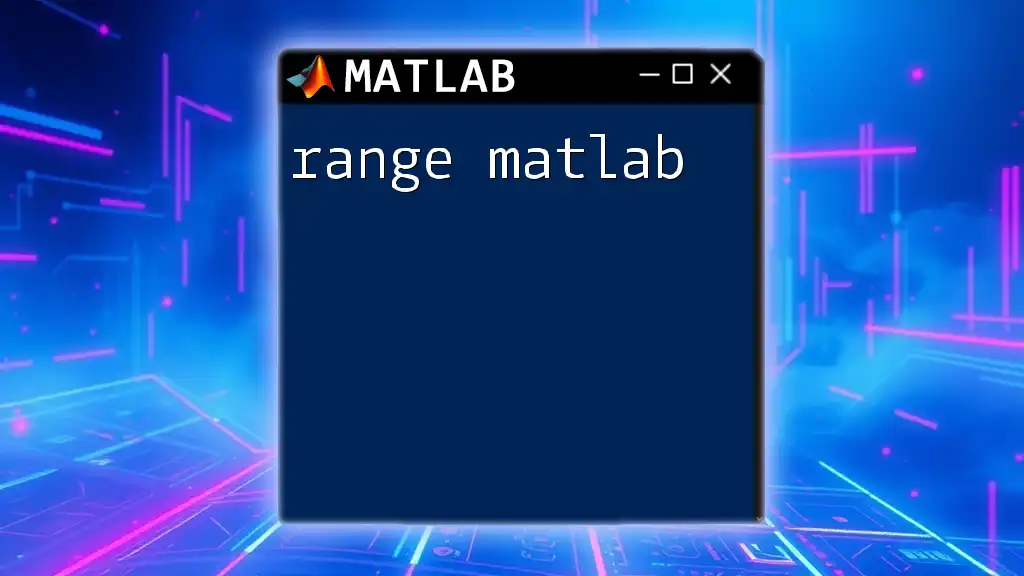
MATLAB's Built-in Functions for Variance
MATLAB provides powerful built-in functions to compute variance easily. The primary function used for calculating variance is `var()`, which can be utilized in various contexts to analyze different data structures.
Overview of Common Functions
The `var()` function can be employed on vectors and matrices in MATLAB:
- `var(x)`: Computes the variance of the elements of vector `x`.
- `var(x, flag)`: When used with the second argument, the `flag`, you can specify whether to calculate the population variance or the sample variance. By default, it calculates the sample variance.
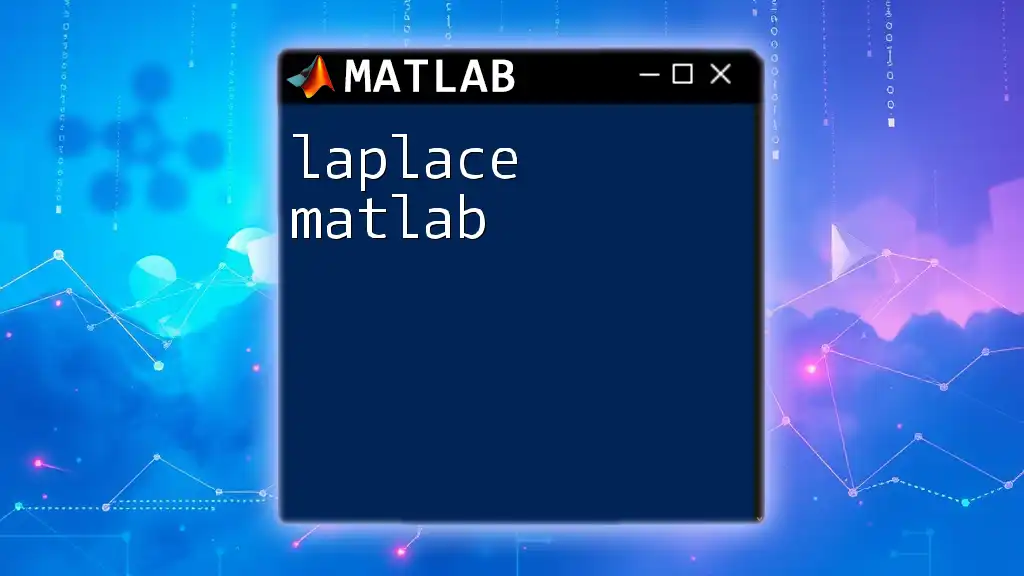
Calculating Variance in MATLAB
Basic Calculation of Variance
Performing variance calculation in MATLAB is straightforward. Here's a basic example of calculating the variance of a simple dataset:
data = [10, 12, 23, 23, 16, 23, 21, 16];
variance = var(data);
In the above example, `var(data)` computes the sample variance, resulting in a numeric output. This output can be interpreted as an indicator of how spread out the values in `data` are relative to their mean.
Calculating Variance for Different Data Types
MATLAB allows you to calculate variance for more complex data structures, such as matrices. When the input is a multidimensional array, `var()` operates along the specified dimension.
For instance, if you have a matrix and want to compute the variance across rows:
dataMatrix = [10, 12; 23, 23; 16, 21; 16, 23];
varianceMatrix = var(dataMatrix);
This command computes the variance for each column in the `dataMatrix`, providing an array of variance values for each variable represented in the columns.
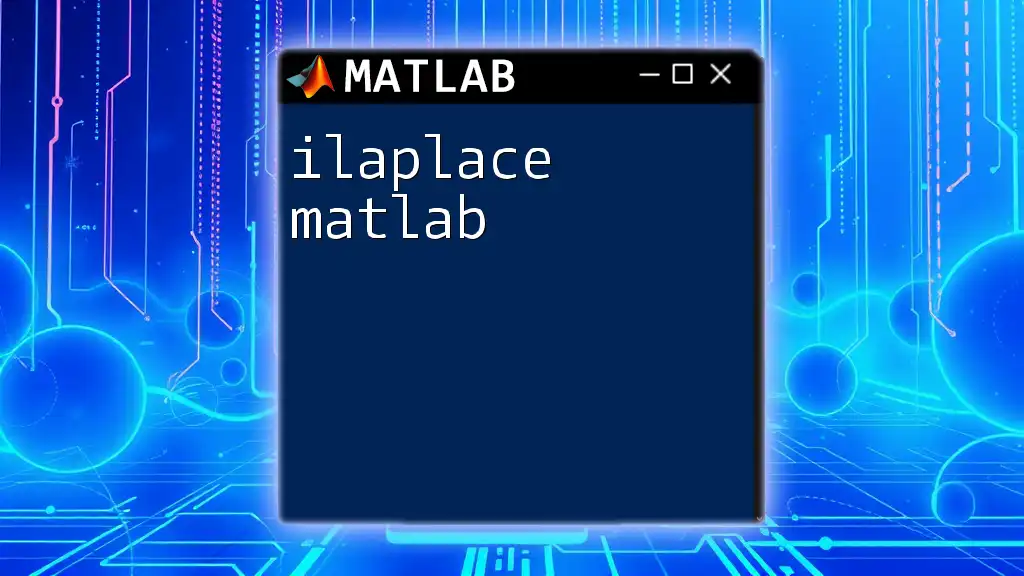
Advanced Variance Calculation Techniques
Weighted Variance
In some cases, individual data points may not contribute equally to the overall variance. This is where weighted variance comes into play. Weighted variance accounts for varying importance of each data point through specified weights.
Here's an example that illustrates calculating weighted variance:
data = [1, 2, 3, 4, 5];
weights = [0.1, 0.2, 0.3, 0.2, 0.2];
weightedVariance = sum(weights .* (data - mean(data)).^2) / sum(weights);
In this case, the `weights` array allows for each data point to be given a unique contribution to the variance calculation based on its importance.
Variance in Statistical Analysis
Variance is crucial in statistical methods such as hypothesis testing and regression analysis. For instance, when comparing two datasets, the difference in variance can inform decisions about data homogeneity or heterogeneity, which are pivotal in conducting significant tests.
Here’s a brief example where variance is employed in ANOVA (Analysis of Variance):
data1 = [1, 2, 3, 4];
data2 = [2, 3, 4, 5];
data3 = [3, 4, 5, 6];
% Combining data and calculating ANOVA
data = {data1, data2, data3};
p = anova1(cell2mat(data));
In this script, the `anova1()` function tests whether the means of the datasets differ significantly, with variance as a key component of the analysis.
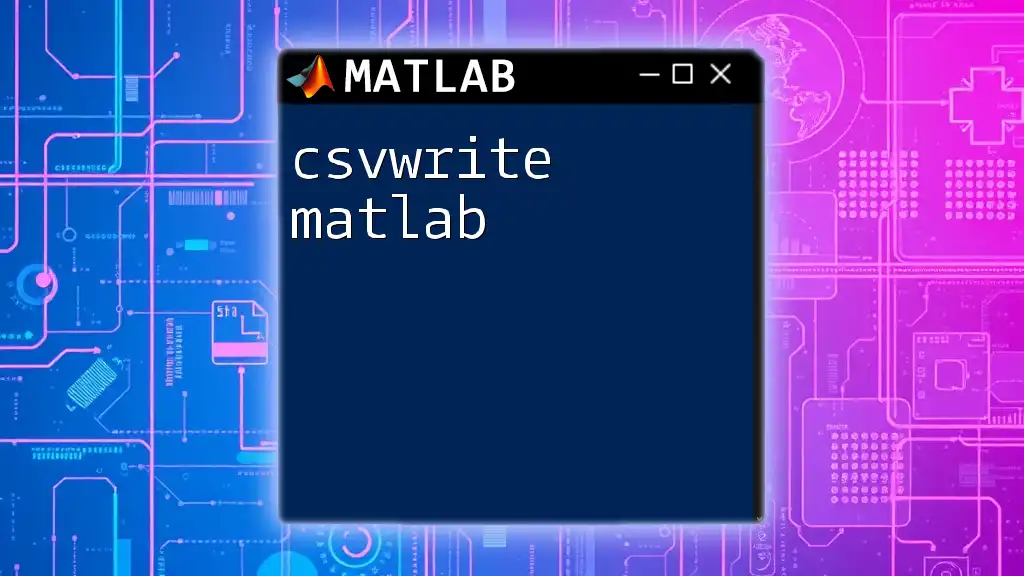
Visualization of Variance
Plotting Data with Error Bars
Visualizing variance can sometimes convey a more intuitive understanding of data spread. Error bars on plots can be utilized to illustrate the mean and variance effectively:
mu = mean(data);
sigma = std(data);
x = 1:length(data);
errorbar(x, mu, sigma);
title('Data with Variance');
The above code plots the mean values and adds error bars that represent the standard deviation, offering visual insight into the variability of the data.
Using Boxplots to Show Variance
Boxplots are valuable for visualizing the range and distribution of datasets. They can effectively showcase variance through the quartiles:
boxplot(dataMatrix);
title('Boxplot to Illustrate Variance');
Boxplots enable easy interpretation of the median, quartiles, and potential outliers, giving a comprehensive view of the data's dispersion and skewness.
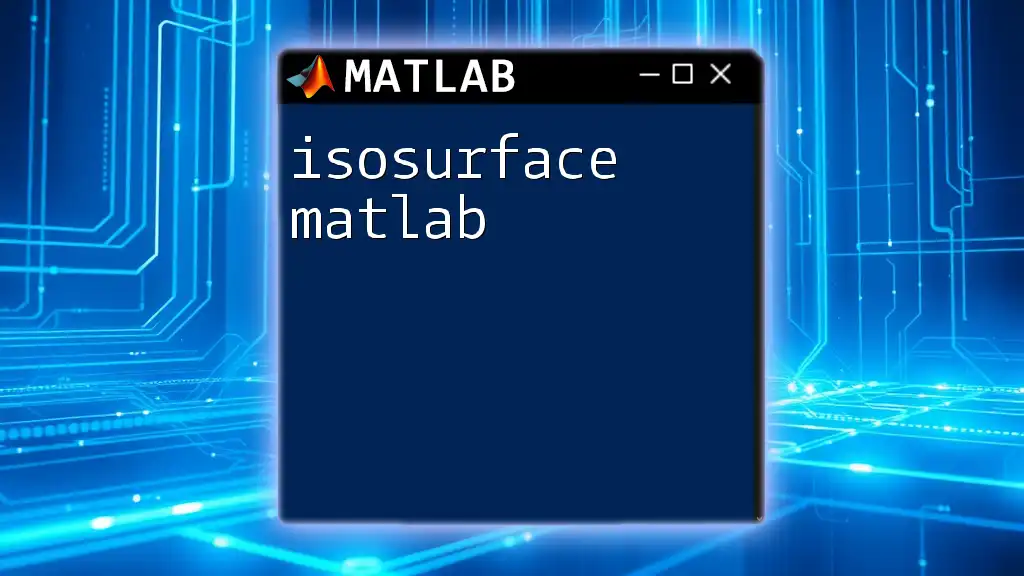
Troubleshooting Common Issues
When calculating variance in MATLAB, you may encounter common issues, especially concerning data types and dimensionality.
Common Errors in Variance Calculation
Understanding how to handle different data structures is vital. Be aware that:
- Input Vectorization: Ensure your data is in the correct format; for instance, a row vector versus a column vector can yield different variance outputs.
- NaN Values: Variance calculations can be affected by `NaN` values (Not a Number). Use `nanvar()` to ignore any NaN entries in your data when computing variance.
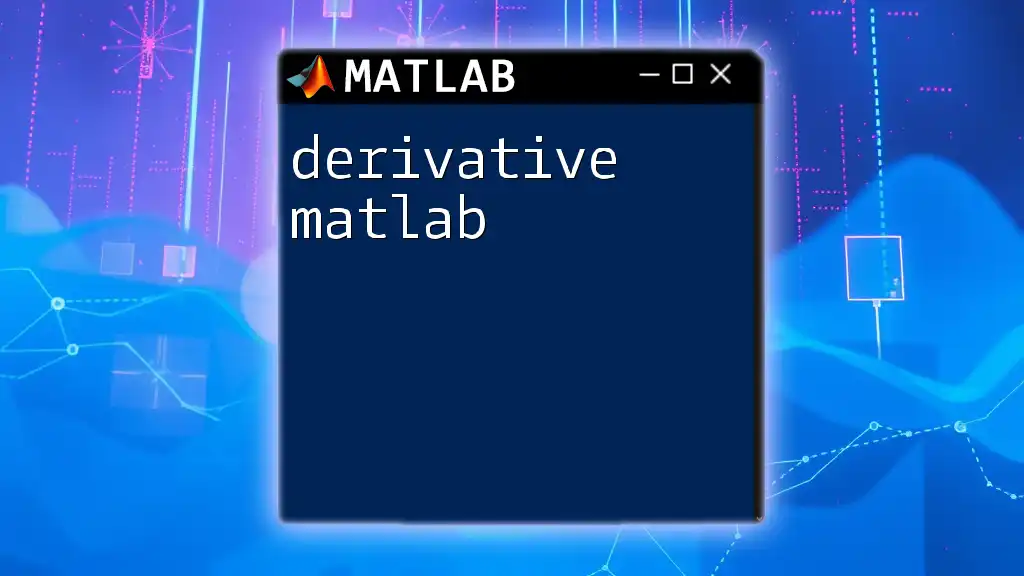
Best Practices for Using Variance in MATLAB
Choosing the Right Variance Function
When calculating variance, it is essential to choose between sample and population variance based on your data context. Familiarize yourself with the assumptions behind each to ensure accurate representation.
Ensuring Data Quality
Before performing any variance calculations, ensure that your data is clean and well-prepared. Removing outliers and addressing missing values can significantly enhance the utility of your variance analysis.
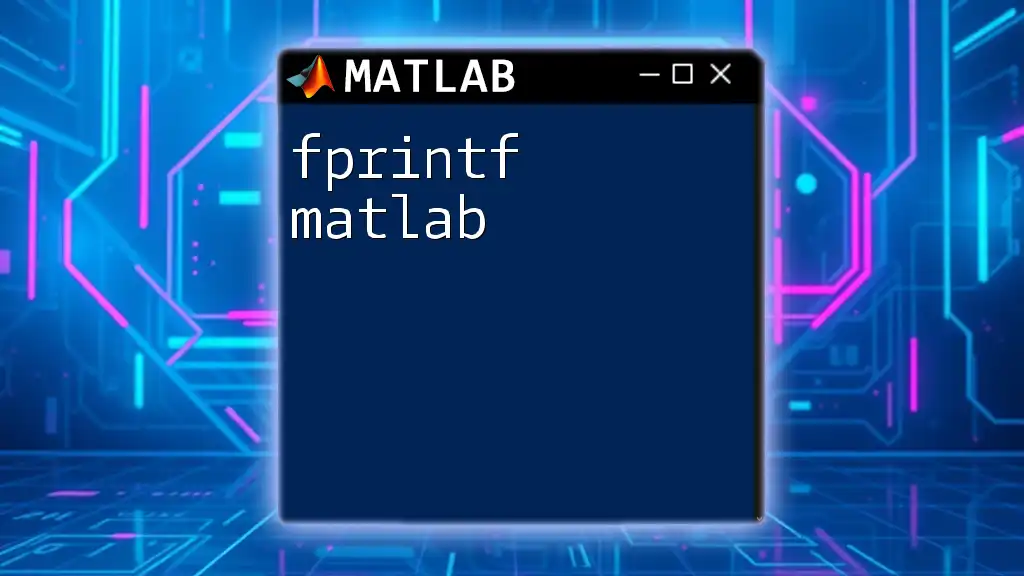
Conclusion
In summary, understanding variance in MATLAB is foundational for anyone looking to analyze data effectively. With built-in functions like `var()`, the process becomes simple and efficient, enabling deeper insights into the variability and distribution of data. As you explore variance further, remember to consider the context of your analysis, ensuring that you apply the appropriate techniques for optimal data interpretation and decision-making.
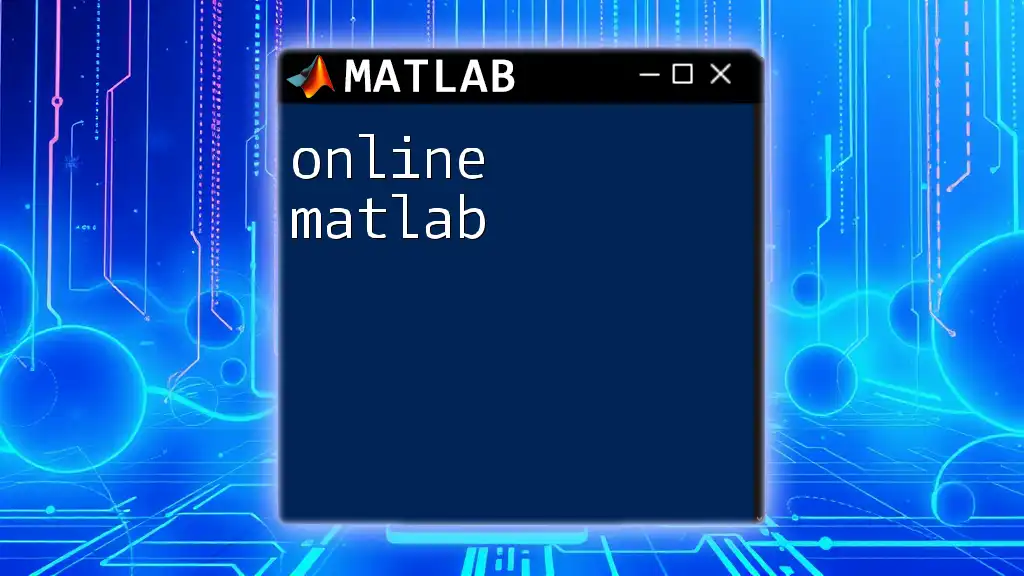
Additional Resources
For those interested in diving deeper into variance and its applications in MATLAB, numerous books and online courses can enhance your knowledge. Additionally, direct links to MATLAB’s official documentation on variance functions offer valuable insights and technical details to refine your understanding.