Applied numerical methods with MATLAB for engineers and scientists involves utilizing MATLAB's powerful computational tools to efficiently solve complex mathematical problems relevant to engineering and scientific applications.
Here's a simple implementation of the Newton-Raphson method to find roots of a function:
% Newton-Raphson method to find roots
f = @(x) x^2 - 2; % Function definition
df = @(x) 2*x; % Derivative of the function
x0 = 1; % Initial guess
tol = 1e-6; % Tolerance for convergence
maxIter = 100; % Maximum iterations
for i = 1:maxIter
x1 = x0 - f(x0)/df(x0); % Update rule
if abs(x1 - x0) < tol % Check for convergence
break;
end
x0 = x1; % Update guess
end
disp(['Root found at x = ', num2str(x1)]);
Understanding Numerical Methods
Numerical Methods are techniques used to find approximate solutions to mathematical problems that may not be solvable through analytical methods. They play a critical role in various engineering and scientific applications, facilitating problem-solving when closed-form solutions are impractical or impossible.
In the realm of engineering and scientific research, MATLAB serves as an exceptionally powerful tool for implementing these methods, thanks to its extensive built-in functions and ease of use.
Types of Numerical Methods
Numerical methods can be categorized into several types that address different mathematical problems:
Root Finding
Root finding methods determine where a function equals zero. This is essential in engineering scenarios like load analysis and electrical circuit design.
Optimization
Optimization techniques are used to find the best solution under given constraints. Applications range from minimizing manufacturing costs to maximizing system efficiency.
Integration and Differentiation
These methods enable analysis of dynamic systems by calculating areas under curves and rates of change, respectively. Applications include simulations in physics and engineering mechanics.
Solving Ordinary Differential Equations (ODEs)
ODEs model dynamical systems, making them essential in fields like control theory and fluid dynamics.
Linear Algebra Techniques
Linear algebra is fundamental in data science and engineering, providing solutions to systems of equations. Techniques involve matrix manipulation, eigenvalue problems, and others.
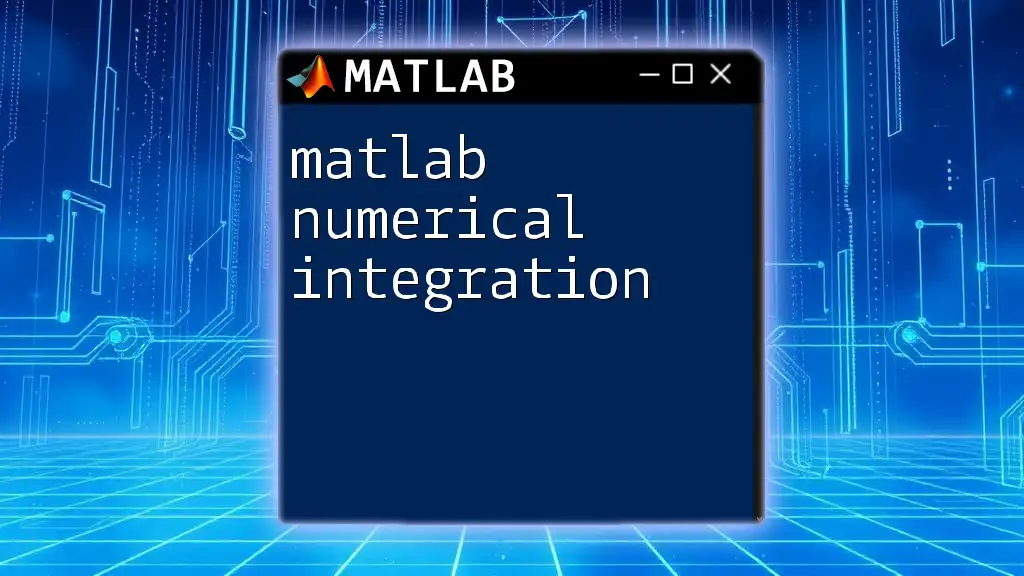
Getting Started with MATLAB
Introduction to MATLAB Environment
Before diving into numerical methods, familiarizing oneself with the MATLAB environment is crucial. The interface offers a straightforward layout with a command window for executing commands and a workspace for managing variables.
Basic Syntax and Commands
MATLAB features a unique syntax that focuses on matrix operations. Key concepts include variables, arrays, and matrix manipulation:
- Variables: Assigned using `=` (assignment operator).
- Arrays: Created using square brackets (e.g., `A = [1, 2, 3; 4, 5, 6]`).
- Matrices: Operations such as addition, subtraction, and multiplication follow specific rules essential in applied numerical methods.
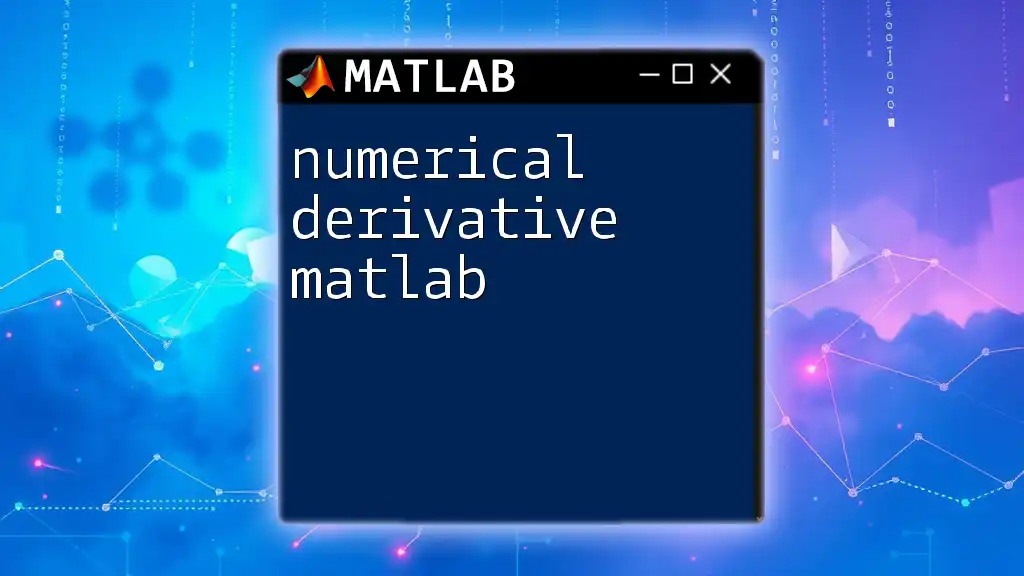
Root Finding Methods
Introduction to Root Finding
Root finding is crucial for solving equations in engineering problems. By identifying points where functions cross the x-axis, engineers can determine critical system parameters.
Bisection Method
Concept: The bisection method is a straightforward iterative technique that successively reduces the interval where a function changes sign.
MATLAB Implementation:
function root = bisectionMethod(f, a, b, tol)
if f(a) * f(b) > 0
error('f(a) and f(b) must have different signs.');
end
while (b - a) / 2 > tol
midpoint = (a + b) / 2;
if f(midpoint) == 0
return midpoint;
elseif f(a) * f(midpoint) < 0
b = midpoint;
else
a = midpoint;
end
end
root = (a + b) / 2;
end
Example Use: To find the intersection between two curves, one can define a function representing the difference and apply the bisection method, adjusting `a` and `b` accordingly.
Newton-Raphson Method
Concept: The Newton-Raphson method uses first derivatives to find roots, offering faster convergence than the bisection method.
MATLAB Implementation:
function root = newtonRaphson(f, df, x0, tol)
x = x0;
while abs(f(x)) > tol
x = x - f(x) / df(x);
end
root = x;
end
Example Use: Utilizing this method could effectively determine stress points in a mechanical component, ensuring product integrity.
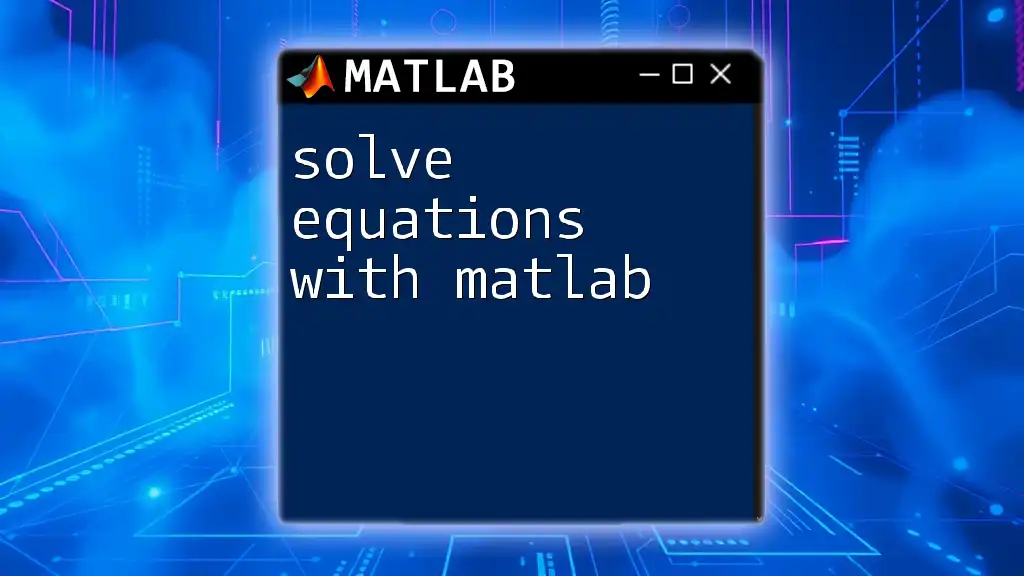
Optimization Techniques
Introduction to Optimization
Optimization techniques are vital in engineering design, enabling professionals to identify parameters that maximize performance or minimize costs.
Gradient Descent Algorithm
Concept: This iterative method adjusts parameters based on the gradient of a cost function, moving towards a local minimum.
MATLAB Implementation:
function [theta, J_history] = gradientDescent(X, y, theta, alpha, num_iters)
m = length(y); % number of training examples
J_history = zeros(num_iters, 1);
for iter = 1:num_iters
hypothesis = X * theta;
errors = hypothesis - y;
theta = theta - (alpha / m) * (X' * errors);
J_history(iter) = computeCost(X, y, theta);
end
end
Example Use: This method can optimize resource allocation in project management, ensuring that time and cost constraints are met efficiently.
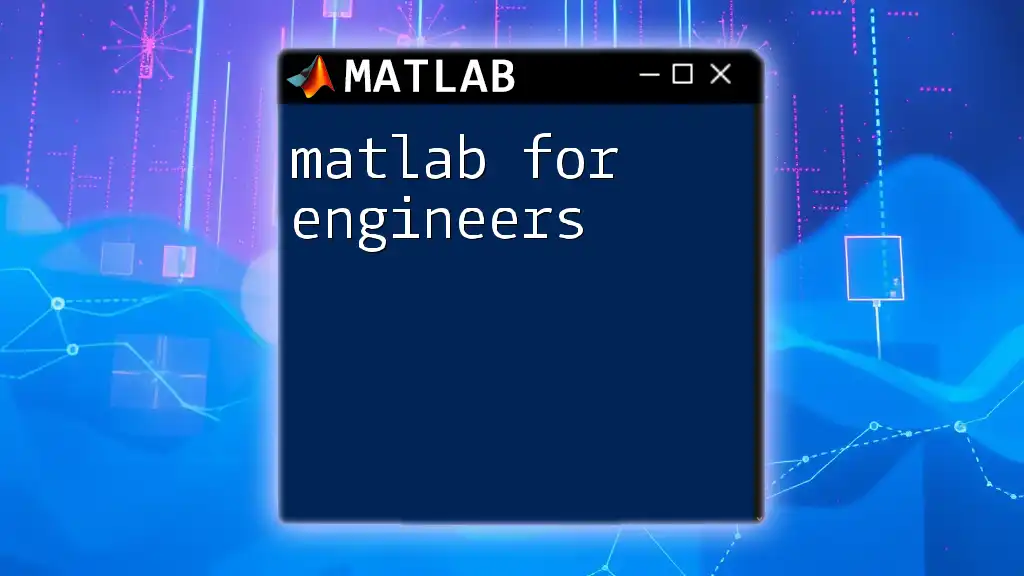
Integration and Differentiation
Numerical Integration
Trapezoidal Rule: A simple yet effective technique for estimating the definite integral of a function by dividing the area under the curve into trapezoidal sections.
MATLAB Implementation:
function integral = trapezoidalRule(f, a, b, n)
h = (b - a) / n;
x = a:h:b;
integral = (h/2) * (f(a) + f(b) + 2 * sum(f(x(2:end-1))));
end
Example Use: Engineers might use this rule when calculating the work done by varying forces over a given distance.
Numerical Differentiation
Forward Difference Method: This technique approximates derivatives by calculating the slope of secant lines.
MATLAB Implementation:
function derivative = forwardDifference(f, x, h)
derivative = (f(x + h) - f(x)) / h;
end
Example Use: This method can assist in determining velocity from displacement data in mechanical systems.
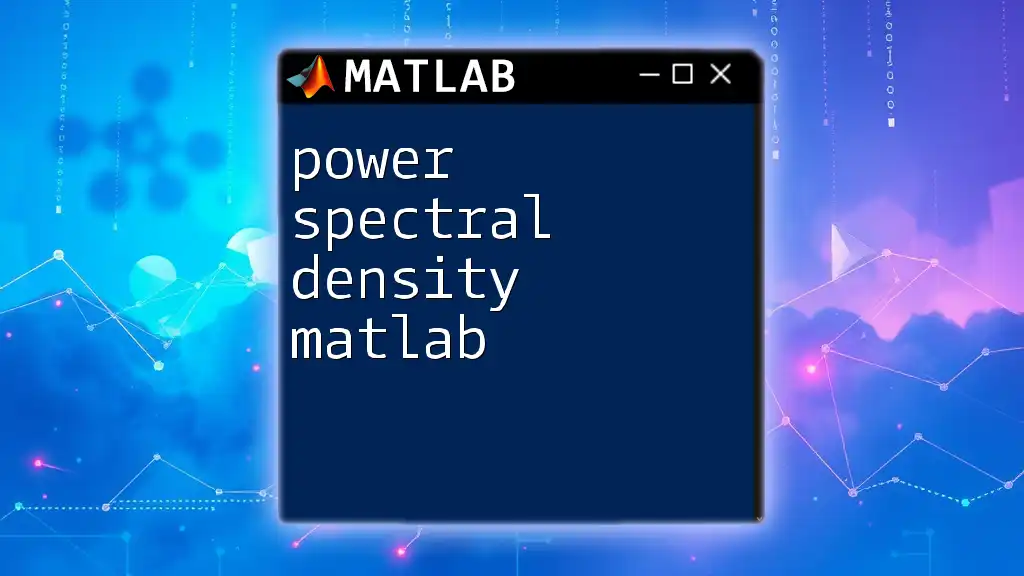
Solving Ordinary Differential Equations (ODEs)
Introduction to ODEs
Ordinary Differential Equations model the dynamics of systems, making them pivotal in fields such as control theory and fluid dynamics.
Euler’s Method
Concept: A basic numerical procedure for solving ODEs by using tangent line approximations.
MATLAB Implementation:
function [t, y] = eulerMethod(f, t0, y0, tEnd, dt)
t = t0:dt:tEnd;
y = zeros(size(t));
y(1) = y0;
for i = 2:length(t)
y(i) = y(i-1) + f(t(i-1), y(i-1)) * dt;
end
end
Example Use: Useful for simulating population growth over time, where the rate of change is influenced by current population density.
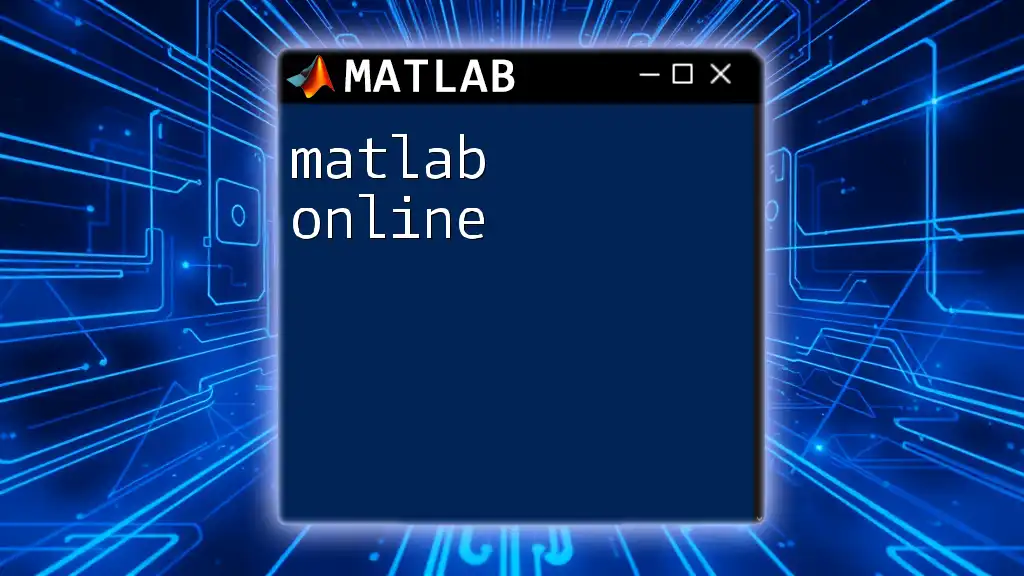
Linear Algebra Techniques
Matrix Operations and Applications
Linear algebra techniques are central to many applied numerical methods, facilitating the efficient manipulation of data. Understanding key operations such as matrix addition, multiplication, and inversion is indispensable.
Solving Systems of Linear Equations
Gaussian Elimination is a well-known method to solve systems of linear equations systematically by transforming the matrix into upper triangular form.
MATLAB Implementation:
function x = gaussianElimination(A, b)
Augmented = [A b]; % Form the augmented matrix
n = size(A, 1);
for i = 1:n
Augmented(i, :) = Augmented(i, :) / Augmented(i, i); % Pivot
for j = i+1:n
Augmented(j, :) = Augmented(j, :) - Augmented(j, i) * Augmented(i, :);
end
end
% Back substitution
x = zeros(n, 1);
for i = n:-1:1
x(i) = Augmented(i, end) - Augmented(i, 1:end-1) * x;
end
end
Example Use: This method can analyze electrical circuits where multiple voltage and current variables exist, thus ensuring compliance with Kirchhoff's laws.
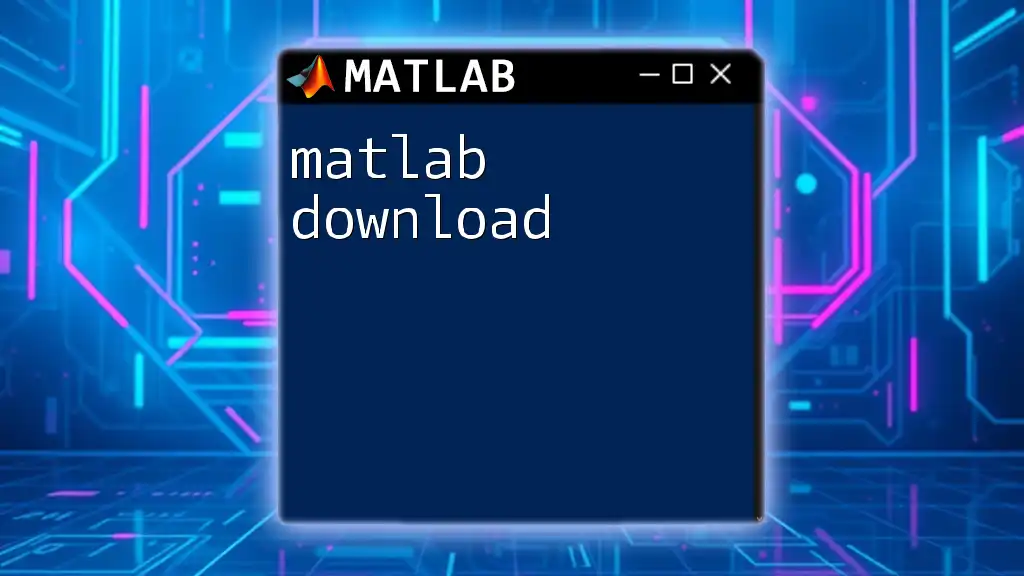
Conclusion
In conclusion, applied numerical methods with MATLAB for engineers and scientists form a cornerstone for tackling complex mathematical problems across various fields. By understanding and effectively implementing techniques such as root finding, optimization, integration, differentiation, ODE solving, and linear algebra operations, professionals can enhance their problem-solving capabilities.
The versatility of MATLAB adds immense value, allowing engineers and scientists to focus on modeling and analysis while streamlining computational tasks.
Continued exploration of MATLAB, along with reference to additional resources and practical applications, will further strengthen one's skillset and open doors to advanced computational analysis.