Learn how to solve equations in MATLAB using the `solve` function, which allows you to find the roots of algebraic equations efficiently.
syms x; % Declare the symbol
eq = x^2 - 4; % Define the equation
solution = solve(eq, x); % Solve the equation
disp(solution); % Display the solution
Understanding Equations
What are Equations?
Equations are mathematical statements that assert the equality of two expressions. They can take various forms, such as linear, quadratic, and polynomial equations, each possessing unique characteristics and solution methods. Equations are fundamental in fields such as engineering, finance, and the natural sciences, making the ability to solve them crucial for professionals in these areas.
Why Use MATLAB for Solving Equations?
MATLAB is a powerful computational tool designed for high-performance mathematical calculations and data visualization. It offers several advantages when you solve equations with MATLAB:
- Speed and Efficiency: MATLAB employs optimized algorithms that can solve large systems of equations faster than manual calculations or even some other software.
- Visualization: Providing graphical representations of equations can enhance understanding and confirm solutions.
- Robust Toolboxes: The availability of various toolboxes, like the Symbolic Math Toolbox, enables more advanced equation-solving capabilities.
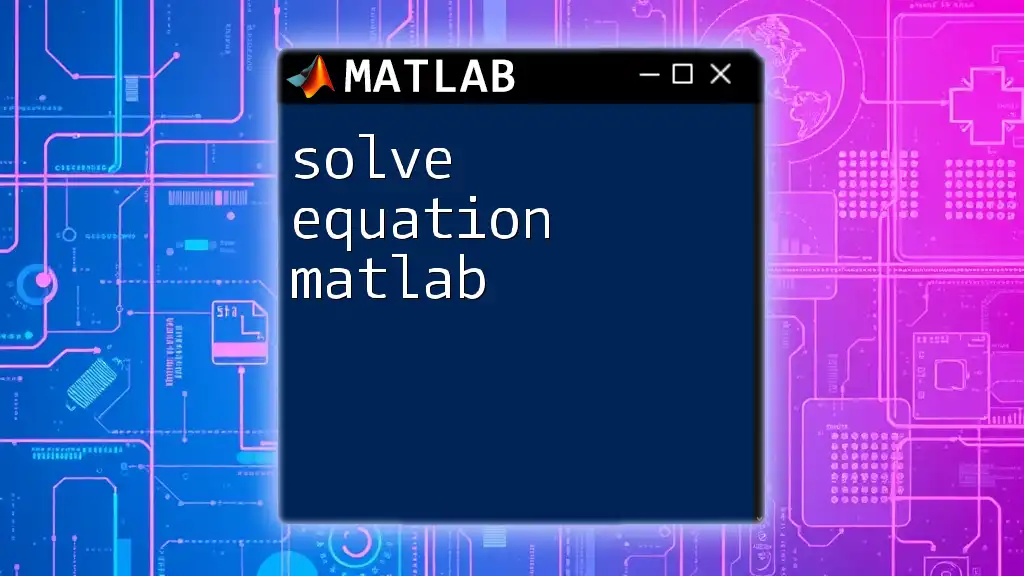
Getting Started with MATLAB
Setting Up Your MATLAB Environment
Before you can solve equations with MATLAB, you need to set up your working environment. First, install MATLAB, which you can download from the official MathWorks website. Familiarize yourself with the MATLAB interface, including the command window, workspace, and the editor. For equation-solving, make sure to explore relevant toolboxes, especially the Symbolic Math Toolbox and Optimization Toolbox.
Basic MATLAB Commands Overview
Understanding key commands will facilitate your work. The command window allows you to input commands directly, where you can quickly test scripts and functions. Below are some essential commands to know:
- `clc`: Clears the command window.
- `clear`: Removes all variables from the workspace.
- `close all`: Closes all figure windows.
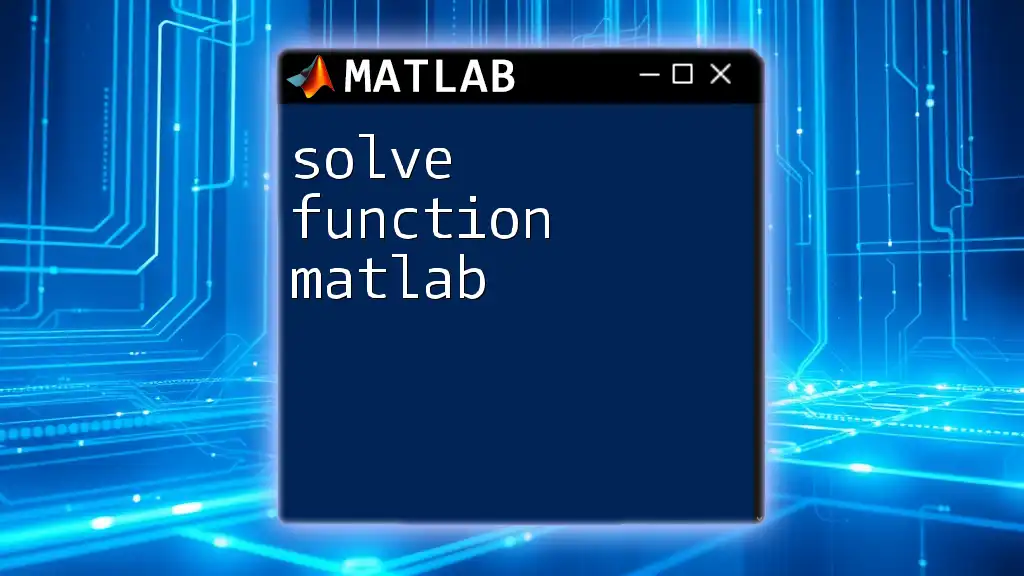
Types of Equations You Can Solve with MATLAB
Linear Equations
What are Linear Equations?
Linear equations represent relationships with no variables raised to an exponent greater than one. A common form is \(Ax = b\), where \(A\) is a matrix, \(x\) is the vector of variables, and \(b\) is a result vector.
Solving Linear Equations Using MATLAB
Using the `\` Operator
To solve the equation \(Ax = b\) in MATLAB, you can simply use the backslash operator (\). For example:
A = [3, 2; 1, 2];
b = [5; 3];
x = A \ b; % This solves Ax = b
This command computes the solution vector \(x\). The advantages of using the backslash operator include its direct approach and efficiency in handling various cases like square or over-determined systems.
Using the `linsolve` Function
Another robust method for solving linear equations is the `linsolve` function, which can provide greater detail about the solution process. The syntax is as follows:
x = linsolve(A, b);
This function is particularly useful for more complex systems and allows for additional options such as specifying properties of matrix \(A\) for optimizations.
Visualizing Linear Equations
To better understand linear equations and their intersections, consider visualizing them using the `plot` function. For instance:
x = -10:10;
y1 = (5 - 3*x)/2;
y2 = (3 - x)/2;
plot(x, y1, 'r', x, y2, 'b');
xlabel('x-axis');
ylabel('y-axis');
title('Visualization of Linear Equations');
legend('3x + 2y = 5', 'x + 2y = 3');
Non-linear Equations
What are Non-linear Equations?
Non-linear equations involve variables raised to powers greater than one or involve other non-linear functions (like sine, exponential, etc.). They often require more sophisticated methods to solve.
Steps to Solve Non-linear Equations with MATLAB
Using the `fzero` Function
MATLAB provides the `fzero` function for finding roots of non-linear equations. This function requires the definition of a function and an initial guess. Here’s a simple example:
fun = @(x) x^2 - 4; % Define the function
root = fzero(fun, 0); % Find root near initial guess 0
This code will return the root of the function, aiding in identifying the points where the function crosses the x-axis.
Using `fsolve` for Systems of Non-linear Equations
For systems of non-linear equations, `fsolve` is the go-to option. It requires the definition of a system of equations. An example code snippet would be:
fun = @(x) [x(1)^2 + x(2)^2 - 4; x(1) - x(2)];
x0 = [1; 1]; % Initial guess
solution = fsolve(fun, x0);
In this case, `fsolve` endeavors to find the values of \(x(1)\) and \(x(2)\) that satisfy both equations simultaneously.
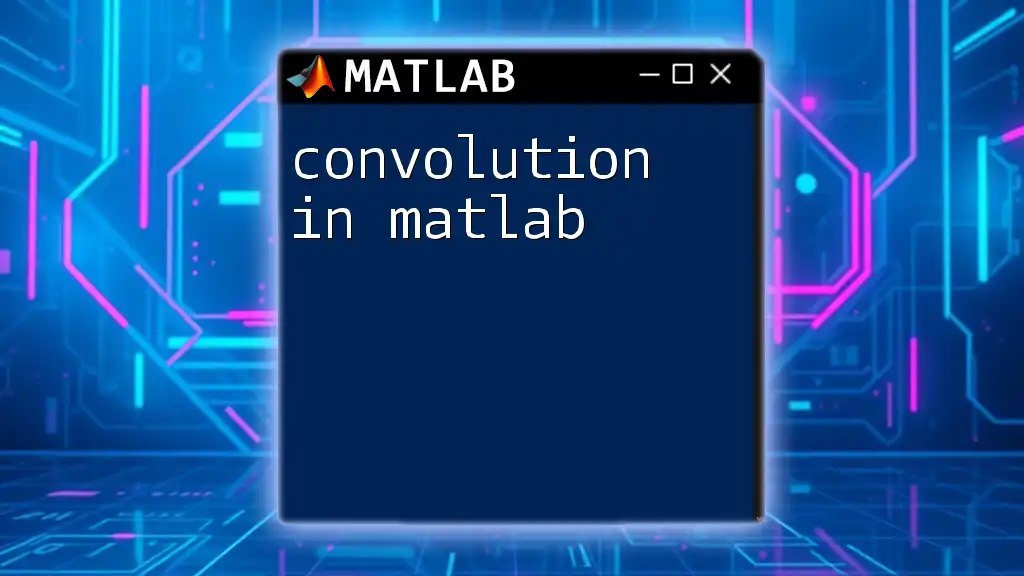
Advanced Topics in Solving Equations
Using Symbolic Math Toolbox
MATLAB's Symbolic Math Toolbox excels in offering analytical solutions rather than numerical approximations. This toolbox allows you to define symbolic variables and manipulate equations algebraically.
Defining Symbolic Variables and Equations
For example, if you want to solve the equation \(x^2 - 1 = 0\), you can do the following:
syms x
eqn = x^2 - 1 == 0;
sol = solve(eqn, x);
This code defines \(x\) symbolically and finds its roots, returning exact solutions instead of numeric approximations. The benefits of symbolic math include obtaining constant expressions, sums, or simplified rational functions that might aid theoretical understanding.
Numerical Approaches to Solve Equations
Introduction to Numerical Methods
When analytical solutions are difficult to derive, numerical methods—such as the Newton-Raphson method or bisection method—become necessary. These algorithms approximate solutions iteratively and are particularly effective for complex equations.
Implementing Numerical Methods in MATLAB
Example: Newton-Raphson Method
The Newton-Raphson method is an iterative procedure that starts with an initial guess and refines it to find roots. Below is a sample implementation in MATLAB:
f = @(x) x^2 - 3;
df = @(x) 2*x;
x0 = 1; % Initial guess
tolerance = 1e-6;
max_iterations = 100;
% Newton's iteration
x = x0;
for i = 1:max_iterations
x_new = x - f(x)/df(x);
if abs(x_new - x) < tolerance
break;
end
x = x_new;
end
The loop continues until the convergence condition is met, demonstrating the method's effectiveness in refining estimates.
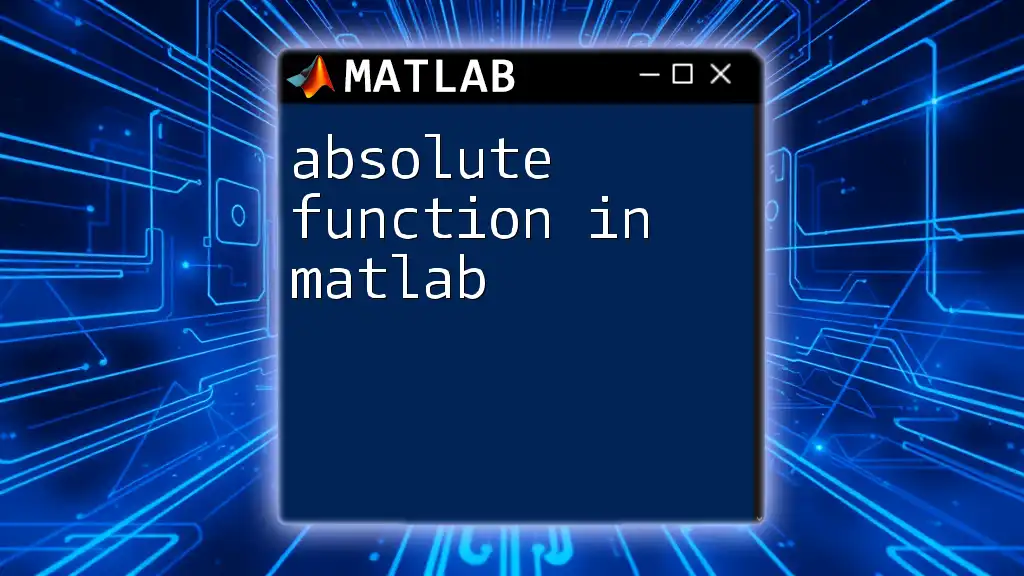
Practical Applications of Equation Solving in MATLAB
Case Studies
Numerous industries leverage MATLAB for solving equations in practical scenarios. For instance, engineers may use it to optimize designs, finance professionals might model investment returns, and scientists can simulate physical phenomena. Projects can range from simple equations to complex simulations like fluid dynamics.
Tips for Efficient Equation Solving
- Keep Code Clean: Maintain readability in your scripts; use comments liberally to document your processes.
- Understand Your Equations: Before diving into coding, comprehension of the mathematical background can significantly ease the solving process.
- Leverage Built-in Functions: MATLAB has extensive built-in functions tailored for many mathematical operations, so it’s often more efficient to use these rather than develop your solutions from scratch.
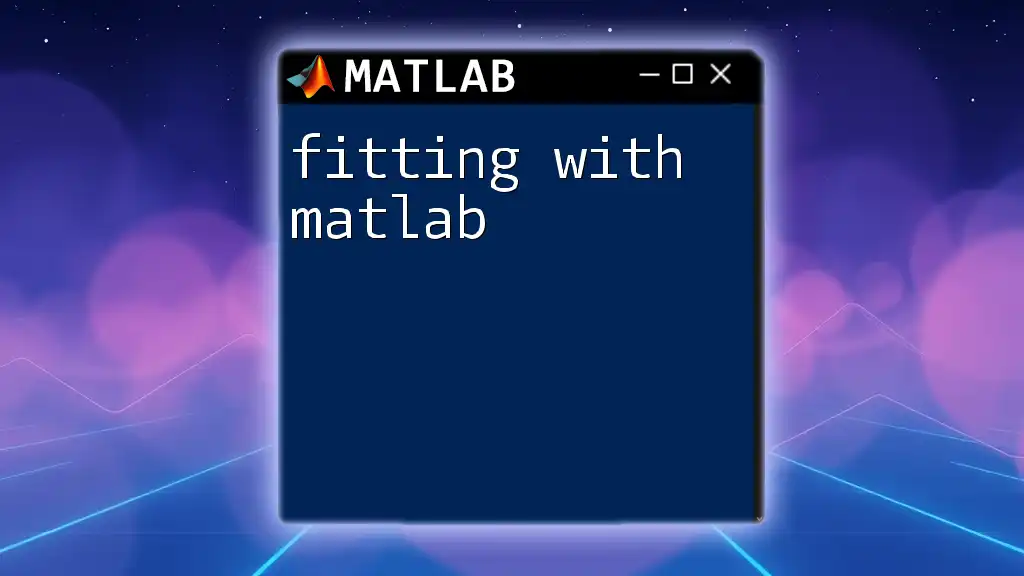
Conclusion
Whether you are dealing with simple linear equations or complex non-linear systems, knowing how to solve equations with MATLAB opens a wealth of possibilities in various fields. Mastering MATLAB’s capabilities will not only improve your mathematical efficiency but will also enrich your problem-solving skills.
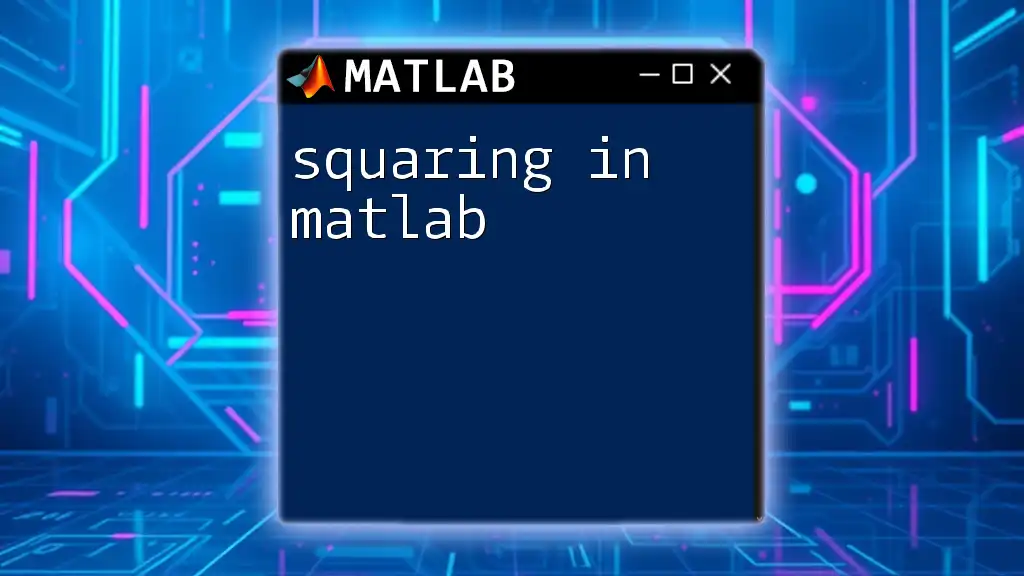
Additional Resources
For further exploration into equation-solving techniques in MATLAB, consider diving into textbooks on numerical methods, online MATLAB courses, and the extensive MATLAB documentation available through MathWorks. Engaging with forums can also enhance your understanding and help troubleshoot your specific problems.
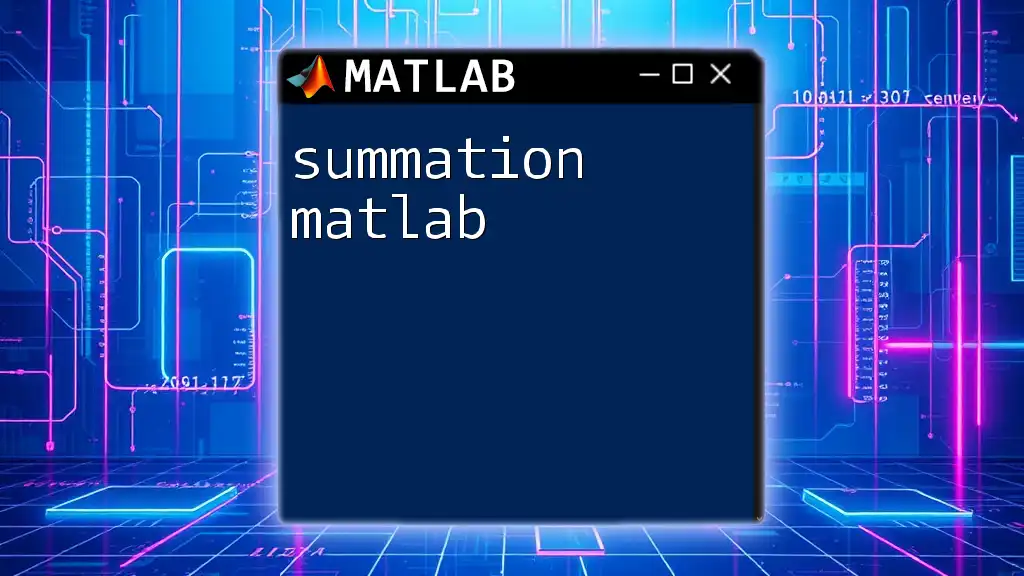
Call to Action
Join our MATLAB courses to deepen your understanding of these powerful tools and techniques. We welcome your questions and comments to foster a community of learning around MATLAB!