In MATLAB, a vector is a one-dimensional array that can hold a sequence of numbers and can be created using square brackets, with commas or spaces separating the elements.
% Creating a row vector
row_vector = [1, 2, 3, 4, 5];
% Creating a column vector
column_vector = [1; 2; 3; 4; 5];
What is a Vector?
A vector in mathematics is a quantity defined by both a magnitude and a direction. In MATLAB, a vector is a one-dimensional array that can contain numbers, and it is essential for various computations, especially in engineering, physics, and data analysis. Learning about vectors in MATLAB opens doors to understanding more complex data structures and calculations.
Why Learn About Vectors in MATLAB?
Vectors play a crucial role in scientific computing and data analysis, making it vital to become proficient with them in MATLAB. Their applications range from modeling physical phenomena to serving as data points in machine learning algorithms. Mastering vector operations helps you to work efficiently and effectively within MATLAB's environment.
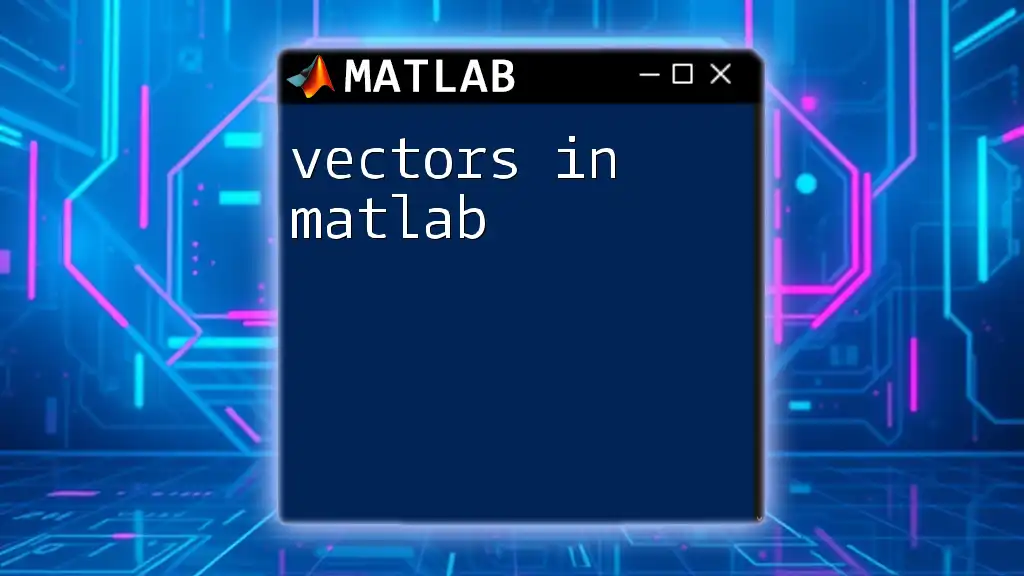
Creating Vectors in MATLAB
Different Methods to Create Vectors
Row Vectors: A row vector is a 1 × n matrix that can store elements in a single row. Creating a row vector is straightforward; you simply list the elements within square brackets, separated by commas.
rowVec = [1, 2, 3, 4, 5];
This defines a row vector containing the numbers 1 through 5.
Column Vectors: A column vector is an n × 1 matrix with its elements listed vertically. Unlike row vectors, you use semicolons to separate the elements.
colVec = [1; 2; 3; 4; 5];
In this case, the numbers are stored in a single column.
Using the `linspace` Function: The `linspace` function generates a vector with evenly spaced values between specified start and end points. This is particularly useful when you need a specified number of points in a given range.
linSpaceVec = linspace(1, 10, 5);
This code creates a vector containing 5 evenly spaced points between 1 and 10.
Using the Colon Operator: MATLAB provides a quick way to create vectors using the colon operator. This operator can define a sequence of numbers with a specified increment.
colonVec = 1:2:10;
In this example, the vector starts at 1 and increments by 2 until it reaches 10.
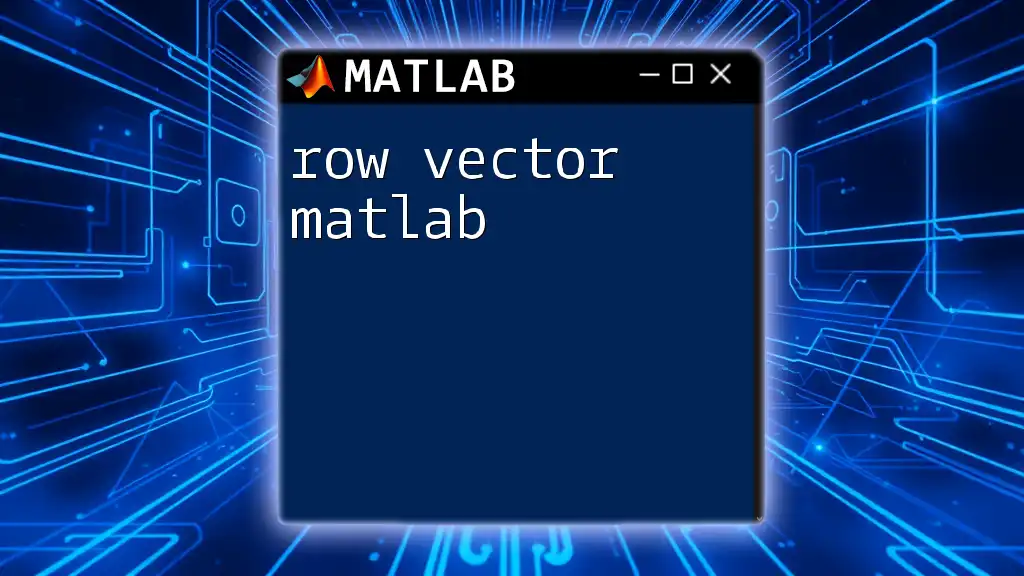
Accessing and Modifying Vectors
Indexing Vectors
Accessing Elements: MATLAB uses 1-based indexing, which means that the first element of a vector is accessed with the index 1.
thirdElement = rowVec(3);
In this case, `thirdElement` retrieves the third value from `rowVec`, which is 3.
Slicing Vectors: You can also extract a subarray, or slice, from a vector. This is accomplished by specifying a range of indices.
sliceVec = rowVec(2:4);
Here, `sliceVec` will contain elements from the second to the fourth position of `rowVec`, resulting in `[2, 3, 4]`.
Modifying Elements of a Vector
Changing Values: To update the value of a particular element, simply assign a new value to that index.
rowVec(2) = 10;
After running this code, the value at index 2 of `rowVec` will change from 2 to 10.
Appending and Concatenating Vectors: You can concatenate vectors to form a new vector. This is useful for expanding your data collection.
newVec = [rowVec, 6];
This code snippet will create `newVec`, which adds the value 6 to the end of `rowVec`.
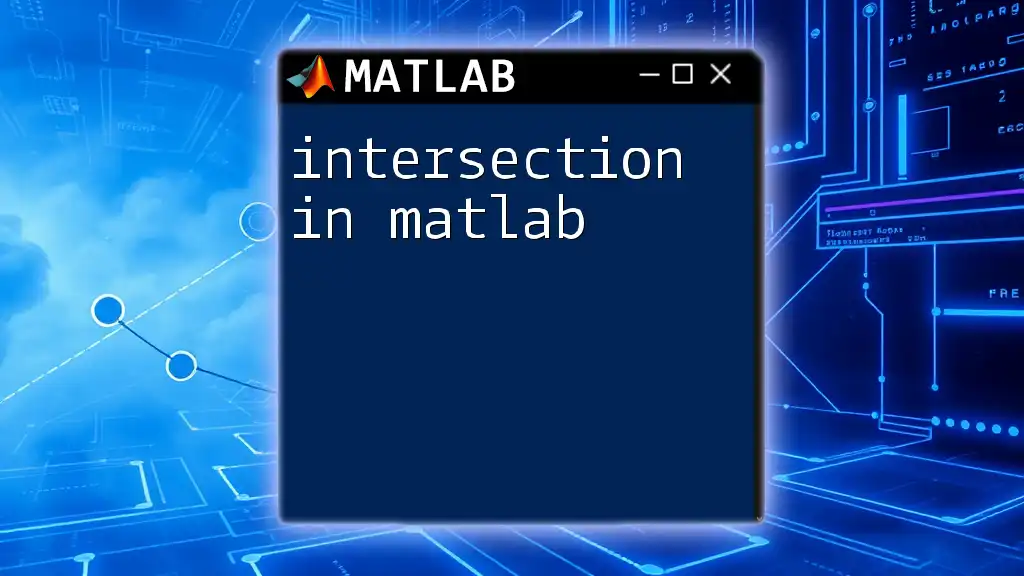
Performing Vector Operations
Basic Arithmetic Operations
Addition and Subtraction: In MATLAB, you can perform element-wise addition or subtraction straightforwardly, provided the vectors have the same orientation (row or column).
resultAdd = rowVec + [1, 1, 1, 1, 1];
This operation adds 1 to each element of `rowVec`, resulting in a new vector.
Scalar Multiplication: You can multiply every element in a vector by a scalar value, a common operation in many mathematical computations.
resultScale = 2 * rowVec;
In this example, each element of `rowVec` is multiplied by 2.
Dot and Cross Products
Dot Product: The dot product of two vectors is a key operation that combines two vectors to produce a scalar. This operation is only defined for vectors of the same size.
dotProd = dot(rowVec, [1, 2, 3, 4, 5]);
This computes the dot product of `rowVec` and the vector `[1, 2, 3, 4, 5]`.
Cross Product: The cross product is defined only for 3D vectors and produces a vector perpendicular to the plane formed by the two input vectors.
crossProd = cross([1, 0, 0], [0, 1, 0]);
This will yield `[0, 0, 1]`, a vector pointing in the z-direction.
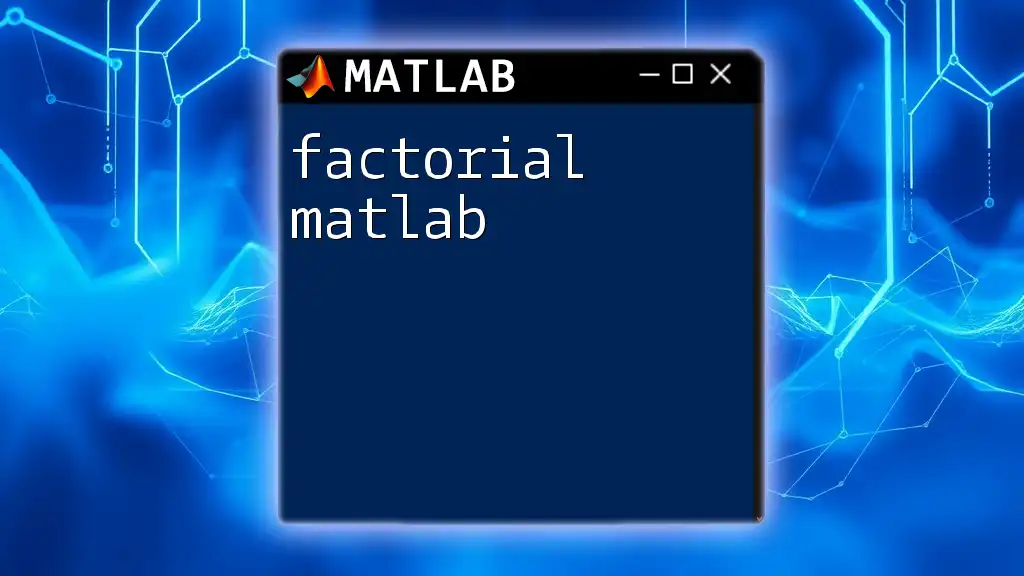
Visualizing Vectors in MATLAB
Plotting Vectors
2D Vectors: You can visualize 2D vectors using plotting functions. The `quiver` function is particularly useful for this purpose, as it plots arrows representing the vectors.
quiver(0,0,rowVec(1),rowVec(2),0);
xlim([-1, 6]);
ylim([-1, 6]);
grid on;
This example creates a 2D plot with an arrow starting from the origin pointing towards the coordinates specified by `rowVec`.
3D Vectors: For 3D visualization, the `quiver3` function helps you plot vectors in three-dimensional space.
quiver3(0, 0, 0, rowVec(1), rowVec(2), rowVec(3));
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
Here, a 3D arrow is drawn from the origin based on the elements of `rowVec`.
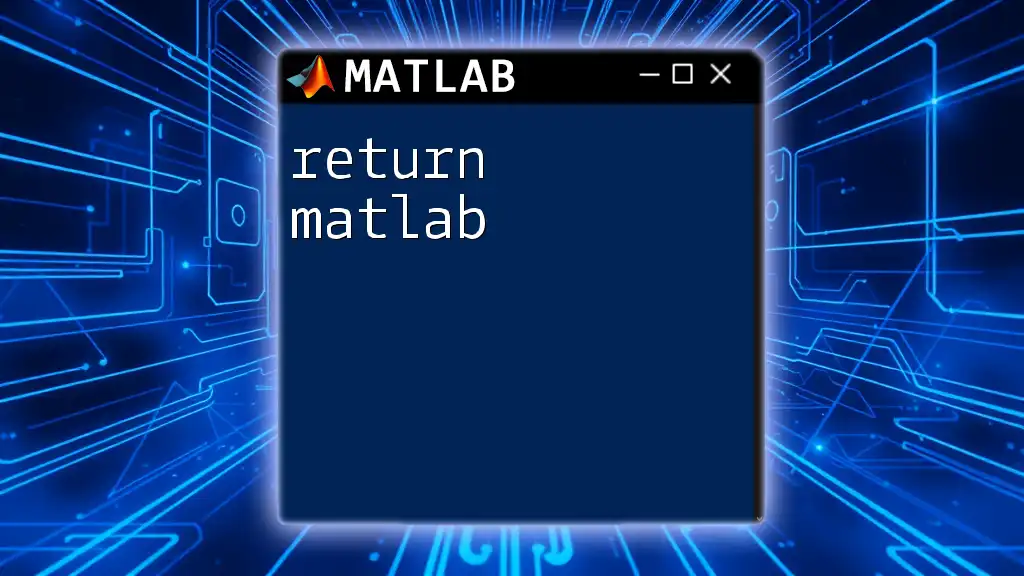
Practical Applications of Vectors
Vectors have numerous real-world applications. In physics, they are used to represent forces, velocities, and trajectories, providing insights into how objects move and interact in space.
In the realm of data analysis, vectors can represent datasets, making it easier to manipulate and visualize numerical information. In the context of machine learning, feature vectors capture the essential characteristics used by algorithms to make predictions and perform classifications.
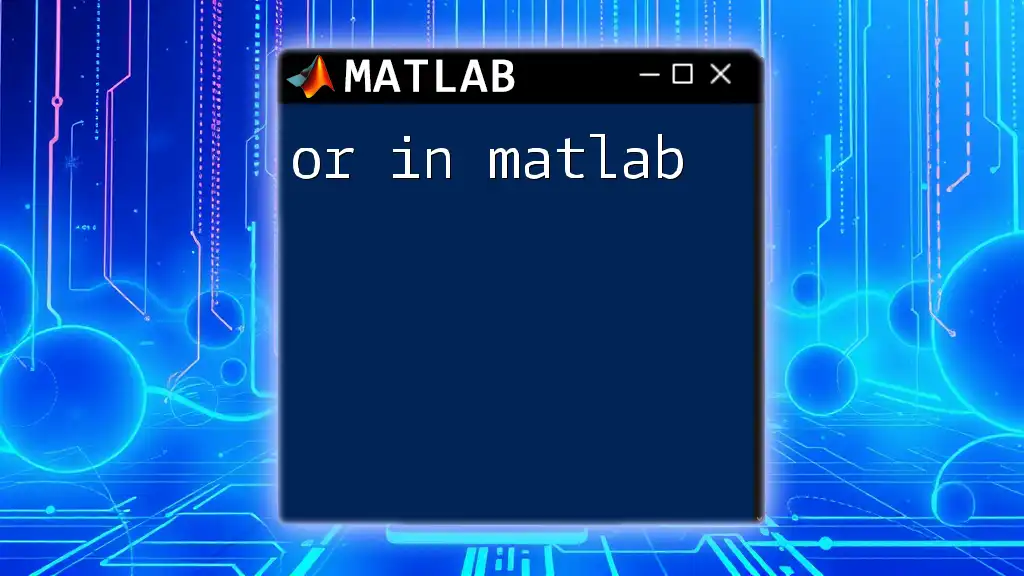
Conclusion
Vectors are foundational elements in MATLAB that enable you to perform a variety of operations efficiently and effectively. Understanding how to create, manipulate, and visualize vectors will significantly enhance your problem-solving capabilities within MATLAB. As you're mastering these operations, consider exploring more advanced topics and additional resources to deepen your knowledge and skill in using vectors in MATLAB.
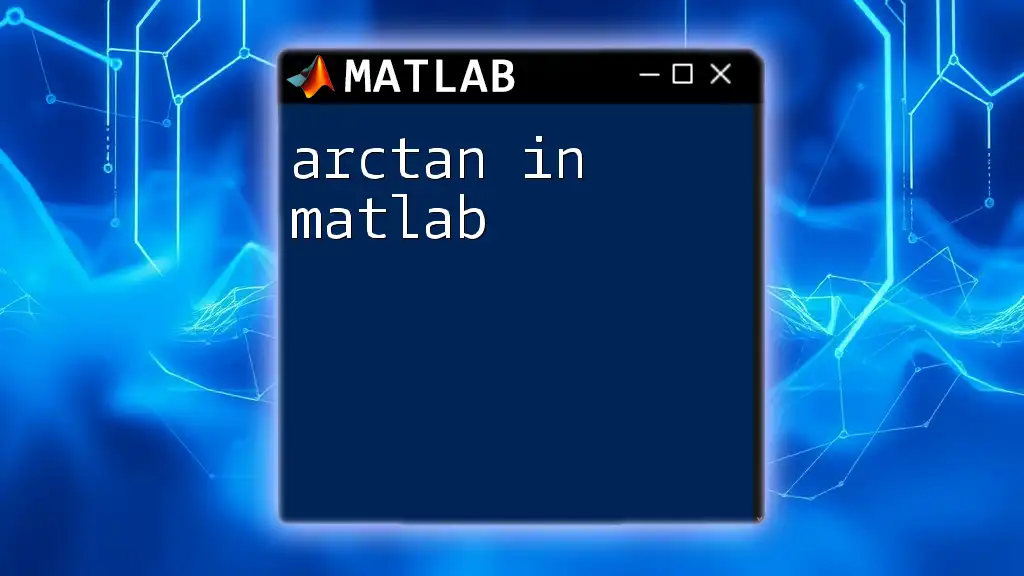
Further Learning Resources
For those eager to expand their understanding, MATLAB’s official documentation, online tutorials, and community forums are invaluable resources. By diving into these materials, you can continue to grow your knowledge and expertise in this powerful computing environment.
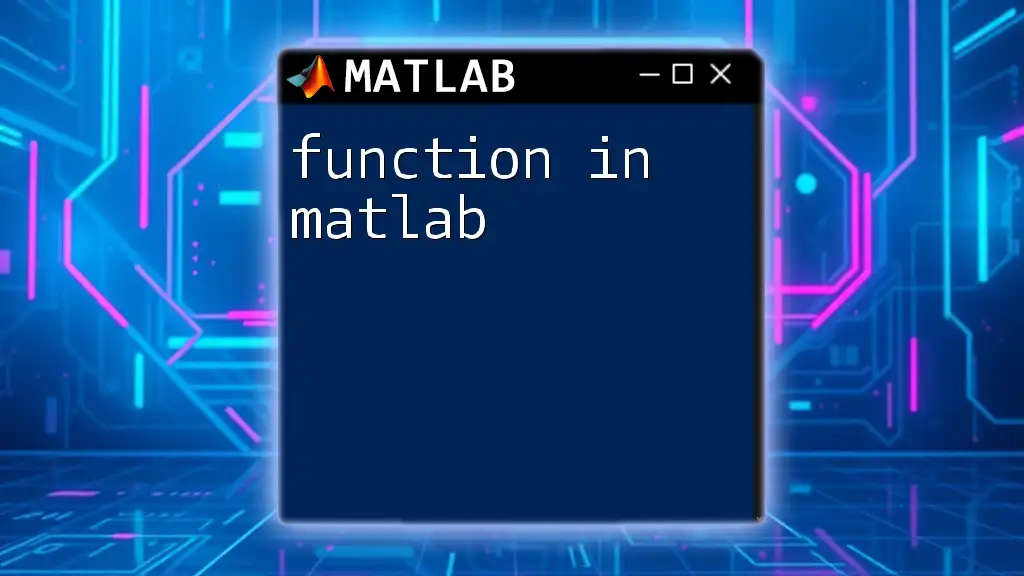
Call to Action
If you're looking to deepen your skills further, consider signing up for our MATLAB command tutorial service, where we provide concise and practical lessons. Also, feel free to share your experiences or ask questions in the comments section below!