The `audioread` function in MATLAB not only reads audio files but also allows you to determine the duration of the audio by computing the total number of samples and the sampling rate.
[y, fs] = audioread('audiofile.wav'); % Read audio file and sampling rate
duration = length(y) / fs; % Calculate duration in seconds
Understanding Audio Files
What is an Audio File?
An audio file is a digital representation of sound. It contains recorded sound waves, structured in a way that computers can read and play them. Common formats include WAV, MP3, and FLAC, each with its advantages regarding compression and quality. The structure of audio files usually consists of two primary components: the header, which contains metadata about the file (such as duration and sample rate), and the data, which contains the sound waveforms.
Why Audio Duration Matters
Understanding the duration of an audio file is crucial in various applications, especially in audio processing and analysis. Knowing the duration allows for better timing in editing, synchronization in multimedia projects, and thorough analysis in research contexts, such as music theory, speech recognition, and sound engineering.
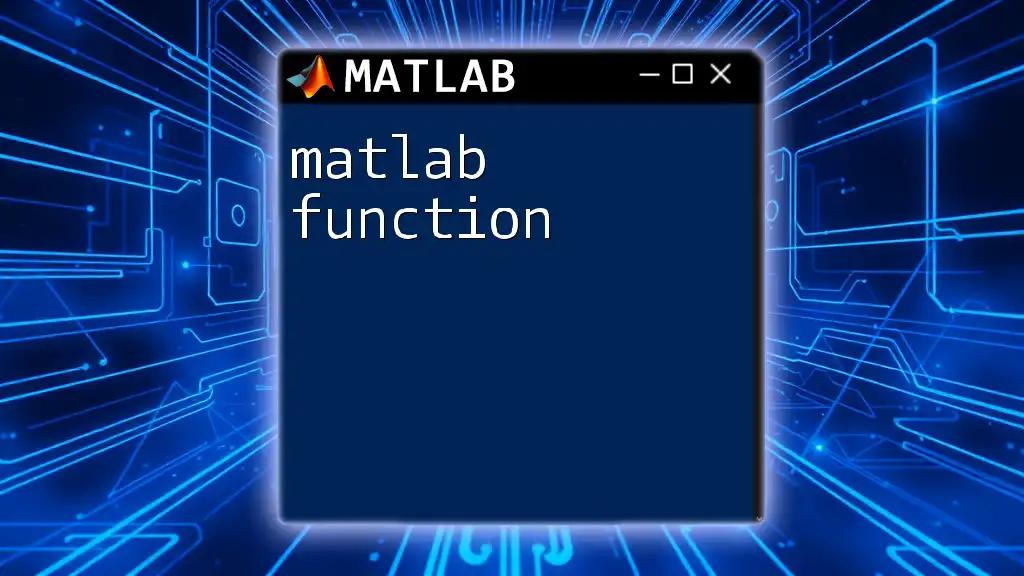
Getting Started with `audioread`
What is `audioread`?
The `audioread` function in MATLAB is a powerful tool for reading audio files. Its primary purpose is to extract audio data and metadata from an audio file, allowing users to manipulate and analyze sound within the MATLAB environment.
Basic Syntax of `audioread`
The general syntax for using `audioread` is:
[y, Fs] = audioread(filename)
Here, `y` represents the audio data, and `Fs` refers to the sampling rate (in Hertz) of the audio file. This function makes it easy to import audio for further processing or analysis, forming the foundation of audio-related tasks in MATLAB.
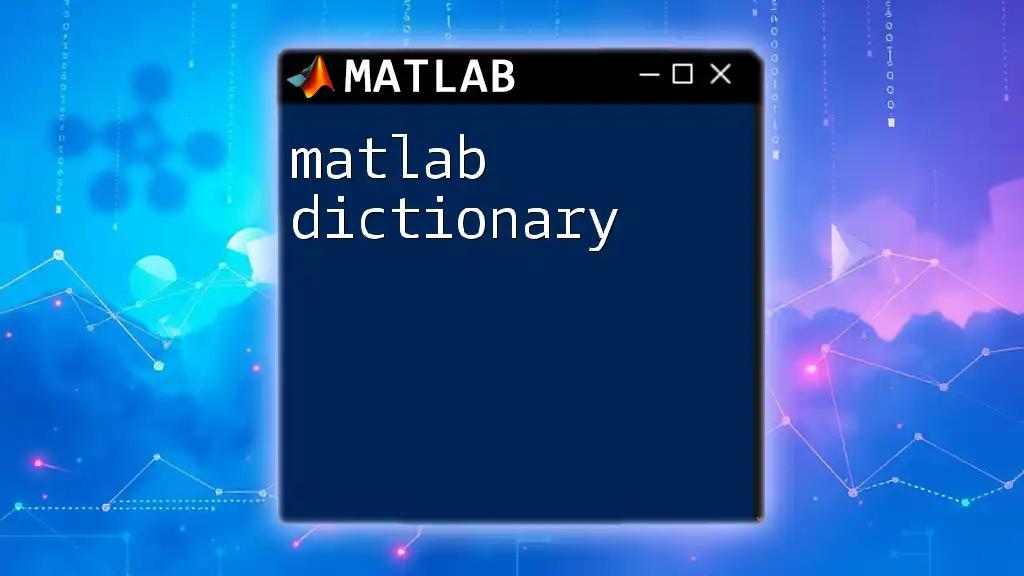
Calculating Audio Duration
What is Audio Duration?
Audio duration refers to the total time span of an audio file, measured in seconds. It is essential for determining how long a sound lasts and is calculated by relating the number of audio samples to the sampling rate.
How to Calculate Duration using `audioread`
To calculate the duration of an audio file read with `audioread`, you can use the following formula:
duration = length(y) / Fs
In this formula, `length(y)` provides the total number of samples in the audio data, while `Fs` is the sampling rate.
Example: Calculating Duration of an Audio File
To illustrate, consider the following code snippet that reads an audio file and calculates its duration:
% Read audio file
[y, Fs] = audioread('example.wav');
% Calculate duration
duration = length(y) / Fs;
% Display duration
fprintf('Duration of the audio file: %.2f seconds\n', duration);
In this example, the audio file `example.wav` is read into the variable `y`, and its sampling rate is stored in `Fs`. The duration is then calculated and printed to the console.
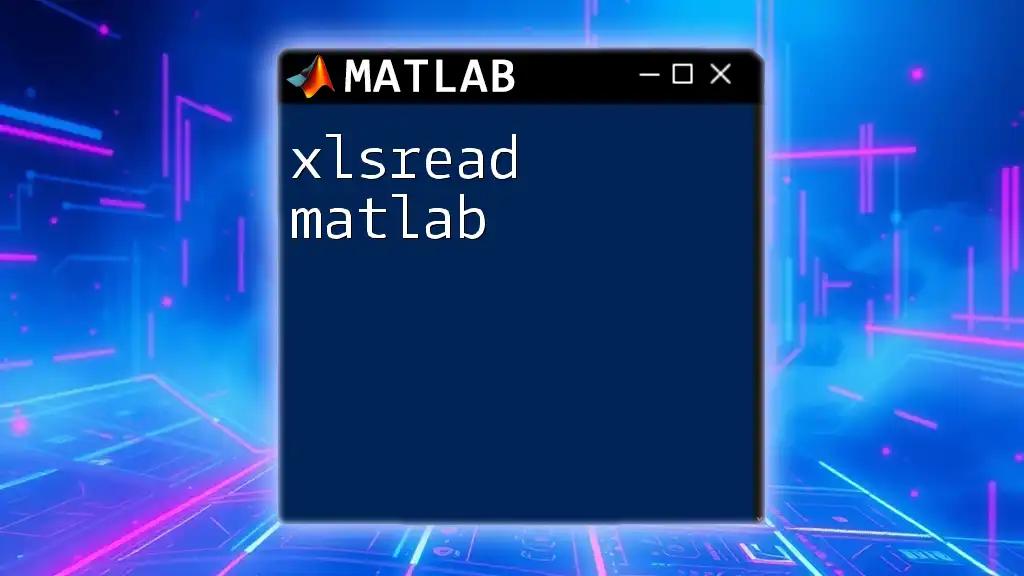
Additional Features of `audioread`
Handling Stereophonic and Monophonic Channels
Audio files can be mono or stereo. Mono audio is a single-channel signal, while stereo has two channels (left and right). When reading stereo audio using `audioread`, the data returned in `y` will be a two-column matrix, where each column represents one channel. It's important to note that the duration for both types remains the same, calculated as it is from the number of samples and sampling rate.
Reading Specific Portions of an Audio File
Sometimes, you may need to analyze only a portion of an audio file. This can be accomplished by specifying the range of samples you wish to read. Here’s a code snippet that reads the first 5 seconds of an audio file:
% Read only the first 5 seconds of audio
start_time = 0; % in seconds
end_time = 5; % in seconds
[y_partial, Fs_partial] = audioread('example.wav', [start_time*Fs end_time*Fs]);
duration_partial = length(y_partial) / Fs_partial;
fprintf('Duration of the partial audio: %.2f seconds\n', duration_partial);
In this example, `audioread` captures a specified segment of the audio, allowing for focused analysis or editing on just that part of the sound file.
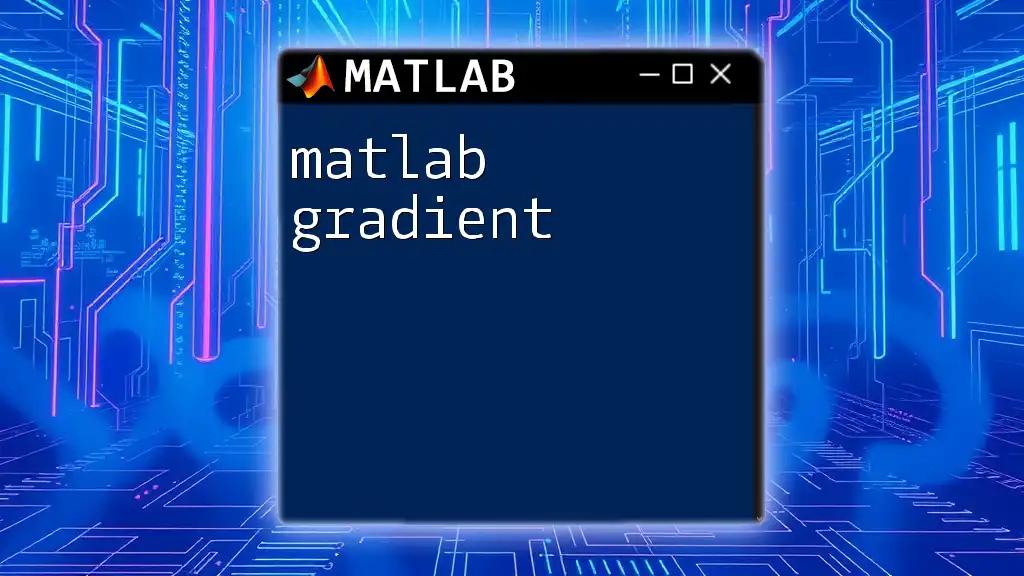
Common Errors and Troubleshooting
Common Errors When Using `audioread`
While working with `audioread`, users may encounter common errors:
- File Not Found: Ensure the filename and path are correct.
- Unsupported Format: Check if the audio file is in a format that `audioread` supports.
- Incompatible Sample Sizes: Make sure the sample sizes you specify align with the audio file's capabilities.
To avoid these issues, always verify file paths and formats before executing your code.
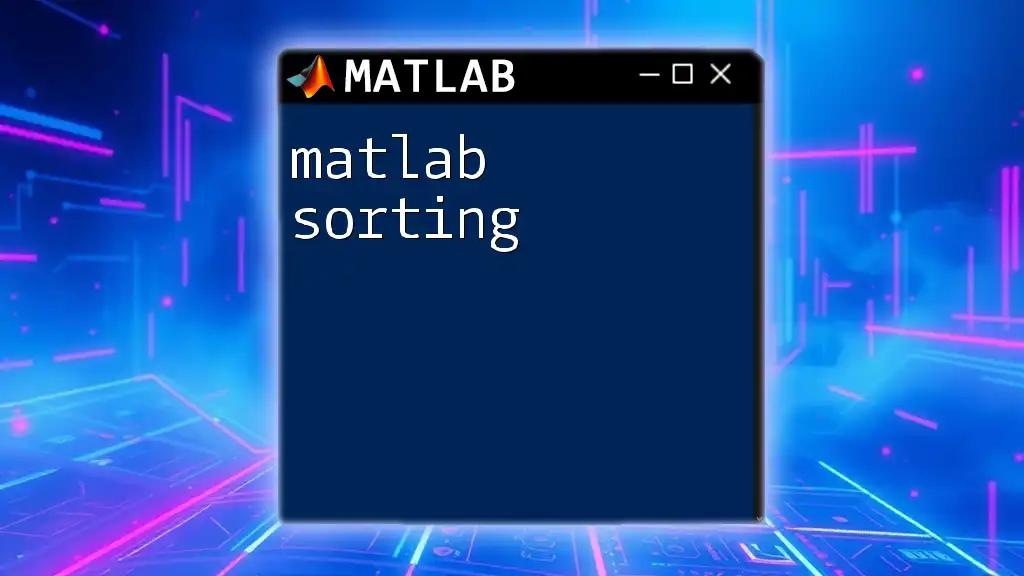
Best Practices
Ensuring Audio Quality
Using high-quality audio files ensures that your analysis yields accurate results. Pay attention to the sampling rates as well; commonly, 44.1 kHz is used for music, while 48 kHz is standard for video productions.
Optimizing Performance
For handling large audio files efficiently, consider loading only what you need. Instead of reading the entire file, use the range parameters in `audioread` to load segments as needed, which streamlines memory usage and processing time.
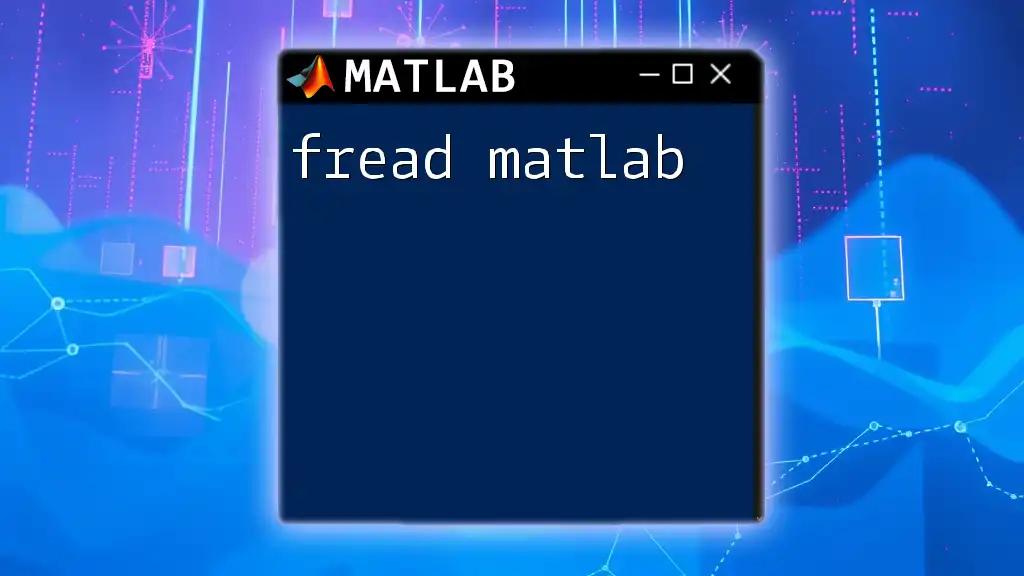
Conclusion
This guide has provided a comprehensive look into calculating audio duration using the `audioread` function in MATLAB. Understanding how to determine audio duration opens the door for more advanced audio analysis and processing techniques. Explore further to harness the full potential of MATLAB for your audio needs.
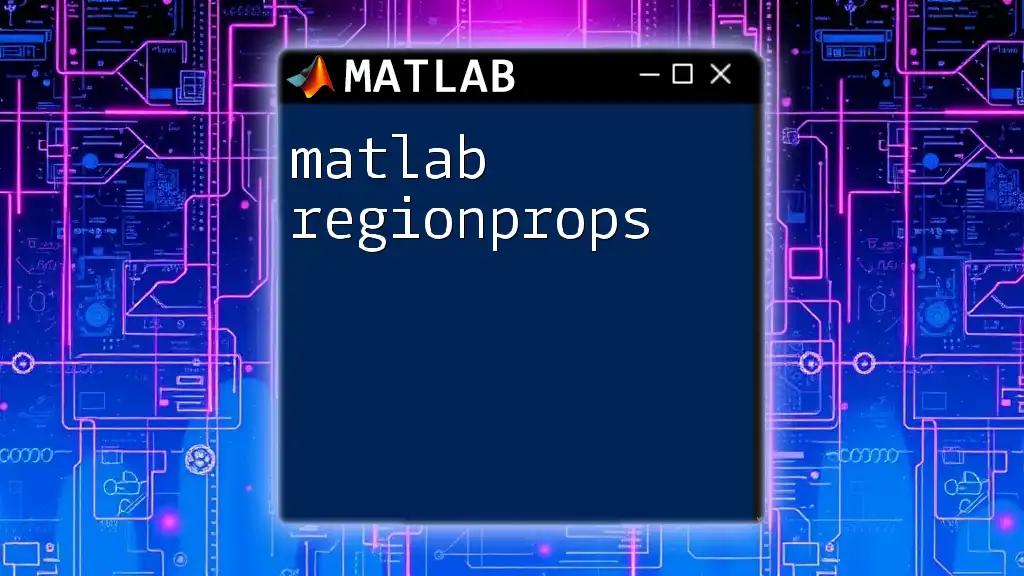
Additional Resources
MATLAB Documentation
For more detailed explanations and advanced functionalities, refer to the official MATLAB documentation on `audioread`.
Recommended Tutorials and Videos
Check out specific tutorials and videos that delve deeper into audio processing techniques in MATLAB.
Join the Community
Engage with forums and online communities focused on MATLAB and audio processing to enhance your learning and share insights with other enthusiasts.