To increase MATLAB precision, you can adjust numeric display format settings to provide more decimal places using the `format long` command.
format long
x = pi; % This will display pi with more precision
disp(x)
Understanding MATLAB Data Types
Key Data Types in MATLAB
To effectively increase MATLAB precision, it's crucial first to understand the different data types available in the environment. MATLAB predominantly uses two major floating-point types: `single` and `double`.
- `single`: This type uses 32 bits and can store approximately 7 decimal digits. It has less precision but is faster and consumes less memory.
- `double`: This is the default data type in MATLAB and employs 64 bits, capable of representing approximately 15 decimal digits. By utilizing `double`, calculations tend to be more accurate and reliable.
In addition to floating-point numbers, MATLAB supports various integer types such as `int8`, `int16`, `int32`, `int64`, and their unsigned versions. Using the right integer type can help with performance and storage efficiency in specific applications.
Choosing the Right Data Type
Choosing the appropriate data type is essential for balancing precision and performance. Here are some key considerations:
- For high precision requirements, prefer `double` over `single`.
- If memory usage is a concern, use integer types judiciously, keeping in mind the range you'll need.
- In operations that mix types (e.g., `double` with `int`), MATLAB often promotes the integer to `double`, which can introduce unexpected results if not anticipated.
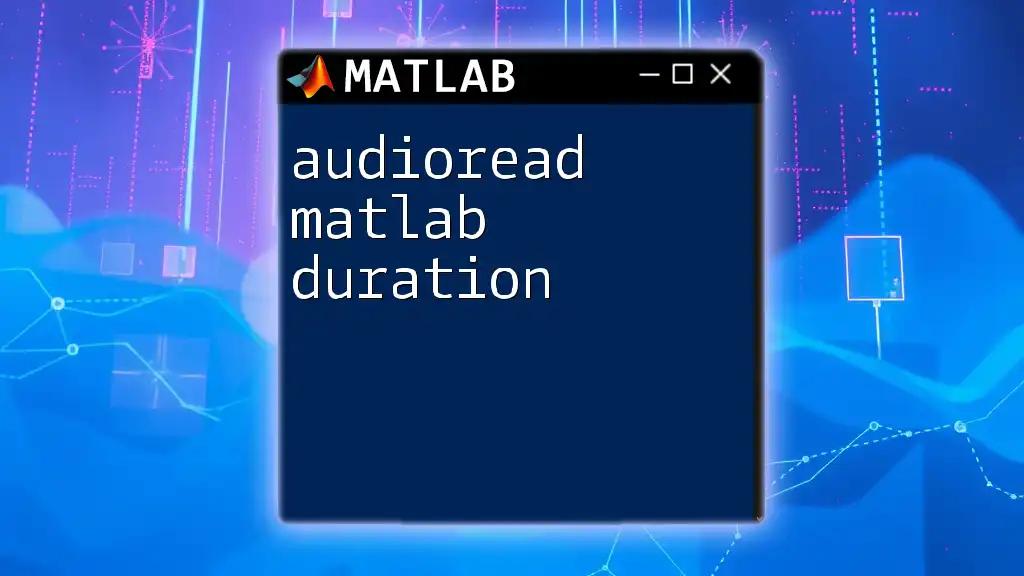
Precision in Floating-Point Arithmetic
IEEE 754 Standard
Most MATLAB floating-point arithmetic is governed by the IEEE 754 Standard, which defines how numbers are represented, stored, and manipulated. It has significant implications for numerical computations, particularly when dealing with precision and accuracy. Understanding this standard helps you grasp why certain operations may lead to unexpected results.
Code Example
Here is a simple example to demonstrate precision loss through basic operations:
a = 1e16;
b = a + 1;
% This will output 1e16 due to rounding errors in floating-point arithmetic
disp(b)
Relative and Absolute Error
It's essential to understand both absolute and relative error when working with precision in MATLAB. Absolute error is the difference between the exact value and the calculated value, while relative error is the absolute error divided by the exact value.
Code Snippet
To visualize errors, you can compute and display both types as follows:
exact_value = 0.1;
calculated_value = 0.1 + 1e-10;
absolute_error = abs(exact_value - calculated_value);
relative_error = absolute_error / abs(exact_value);
fprintf('Absolute Error: %.10f\n', absolute_error);
fprintf('Relative Error: %.10f\n', relative_error);
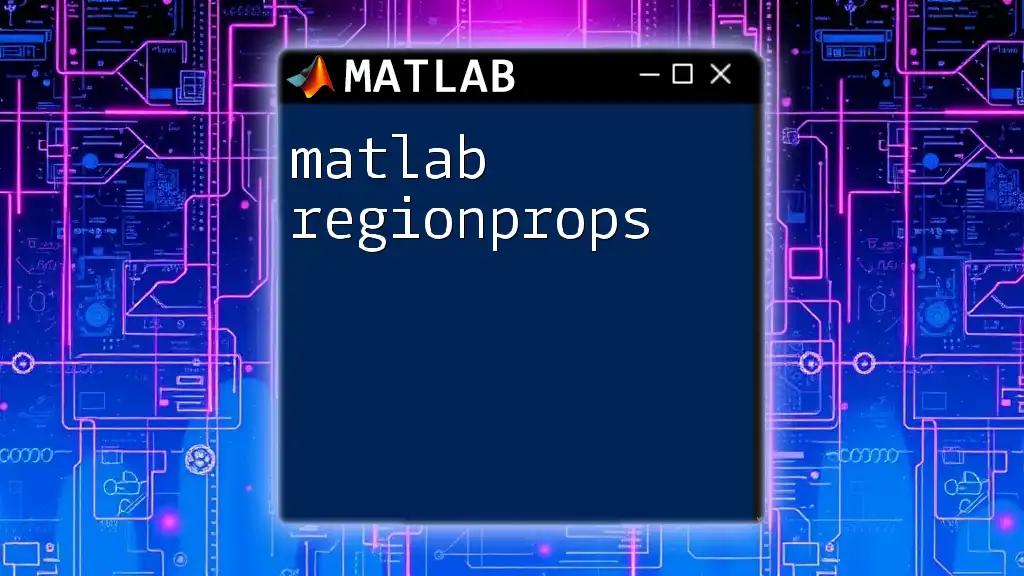
Using MATLAB Functions for High Precision
Built-in Functions for Increased Precision
MATLAB offers several built-in functions designed to aid precision-focused calculations.
`vpa()`
The Variable Precision Arithmetic function, `vpa()`, can handle numbers at arbitrary precision, which is especially beneficial in applications requiring great accuracy.
Here’s an example comparing `vpa()` to traditional floating-point calculations:
% Regular multiplication
a = 1.234567890123456789;
b = 1.000000000000000001;
result1 = a * b;
% Variable precision arithmetic
result2 = vpa(a) * vpa(b);
fprintf('Standard Result: %.15f\n', result1); % Less precise
fprintf('VPA Result: %s\n', char(result2)); % More precise
`sym()`
The Symbolic Math Toolbox (available via `sym()`) further enhances precision by allowing calculations in symbolic form, removing the issues tied to floating-point representation.
Example of using `sym()`:
syms x;
expr = (x^2 + 2*x + 1)/(x+1);
simplified_expr = simplify(expr); % Symbolically simplifying the expression
disp(simplified_expr)
Avoiding Common Pitfalls in Precision
Precision-related errors often stem from frequent pitfall practices, such as subtracting numbers that are very close in value. The loss of significance can drastically affect your results.
Control of Numerical Stability
Strategies to enhance numerical stability include:
- Revising algorithms: Adopt more stable algorithms, such as using `Cholesky` decomposition for solving linear systems instead of Gaussian elimination.
- Scaling and normalizing inputs: Adjust inputs to avoid operating on significantly large or small values.
Code Example
The following code illustrates the effect of cancelation:
x = 1e10;
result = (x - 1e10) + 1;
disp(result) % The result may not be as expected
By carefully crafting algorithms and operations, you can mitigate significant precision losses.
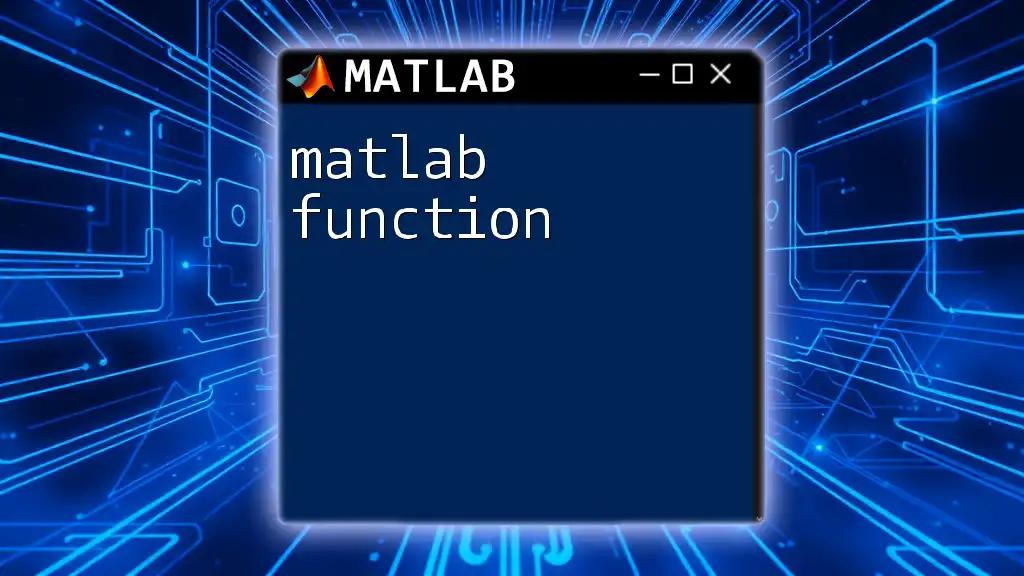
Increasing Display Precision
Setting Display Format
Changing the display format in MATLAB can enhance your ability to visualize precision. Common formats include `format short`, `format long`, and even custom formats.
Code Sample
For example, setting a long format allows you to see more digits in computations:
format long
pi_val = pi;
disp(pi_val)
Changing the display format doesn’t influence the underlying calculations but helps to verify what you're dealing with at a glance.
Utilizing `fprintf` for Enhanced Output
To achieve better control over how results appear in the output, use the `fprintf` function. This allows you to format numbers to the precision you need.
num = pi;
fprintf('The value of pi with high precision: %.15f\n', num);
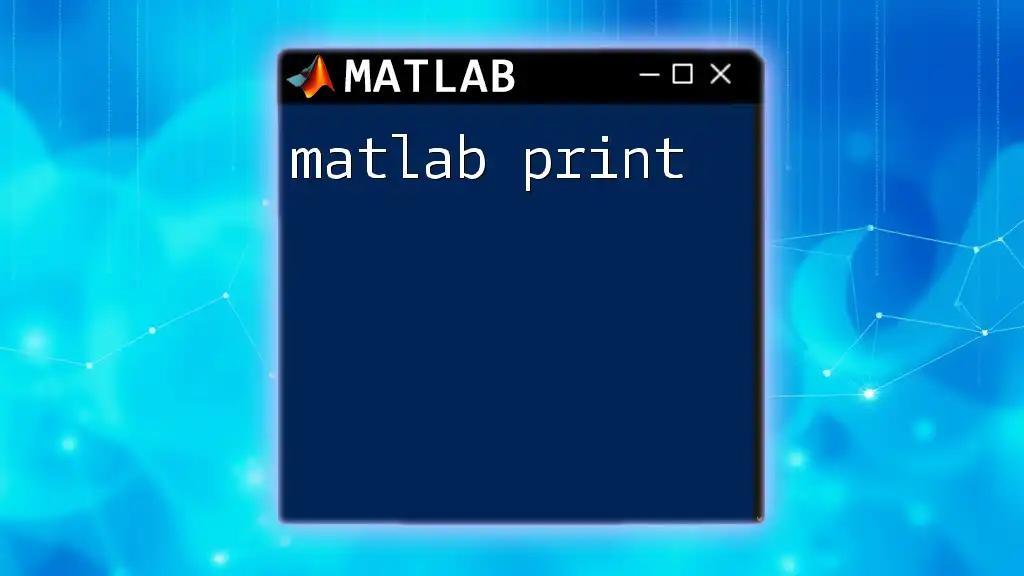
Best Practices for Code Writing in MATLAB
Writing Robust Numerical Code
Testing your code for precision-related issues is paramount. Implementing robust unit tests helps surface potential problems early in your development workflow.
Documentation and Commenting
Documenting your decisions around precision considerations in your code is also vital. Include comments that explain why certain data types or methods were employed, helping others (and your future self) understand your approach.
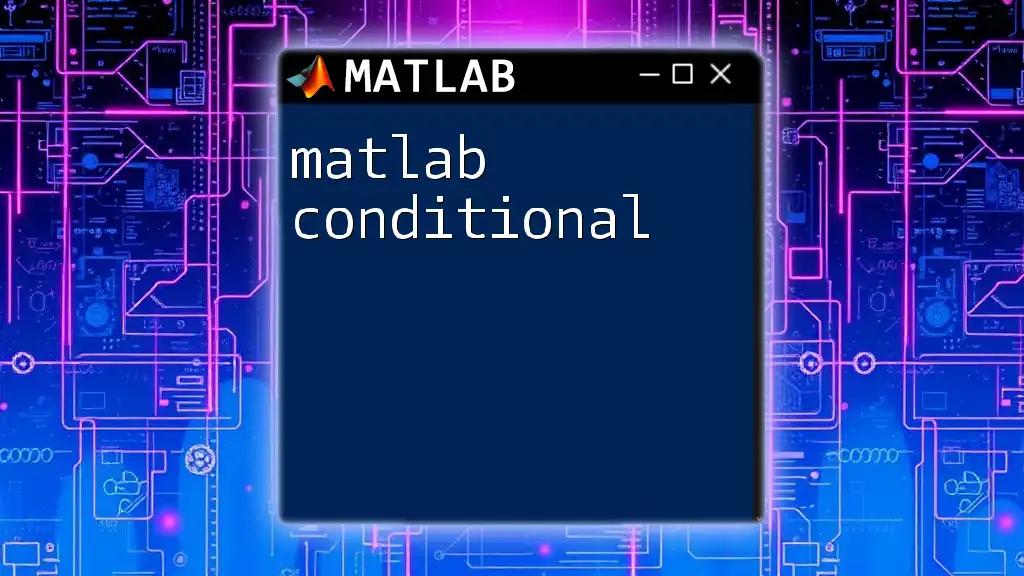
Real-World Applications of Increased Precision
Engineering and Scientific Applications
Certain fields like engineering, finance, and scientific research greatly benefit from enhanced precision. Examples include simulations involving small parameters or financial calculations requiring exact decimals.
A case study could highlight projects where precision is non-negotiable, showcasing the tangible benefits of increased precision techniques.
Benchmarks and Performance
Though increased precision can result in more accurate calculations, it might also impact performance. Evaluating this balance is essential.
Code Snippet
The following example compares the execution time of different precision levels:
tic
a = 1.234567890123456789;
b = 1.000000000000000001;
result1 = a * b; % Using default precision
toc
tic
result2 = vpa(a) * vpa(b); % Using variable precision
toc
By measuring execution times, you can make informed decisions about trade-offs regarding precision and performance.
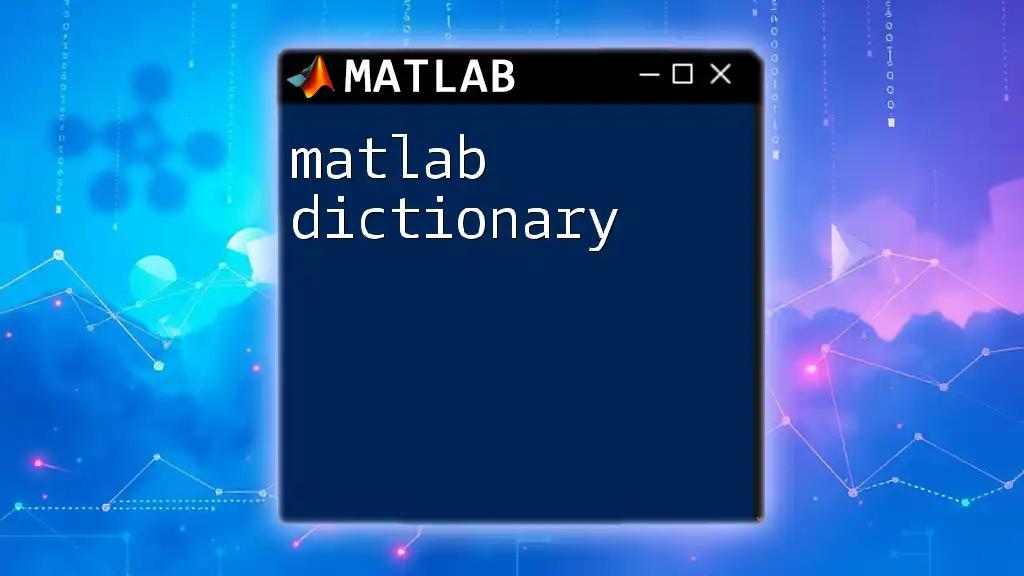
Conclusion
Increased MATLAB precision is indispensable for achieving accurate numerical results. By understanding different data types, leveraging built-in functions, and employing best practices, you can enhance your computational outcomes significantly. Implementing these strategies in your projects will not only improve accuracy but also increase reliability in your computations.
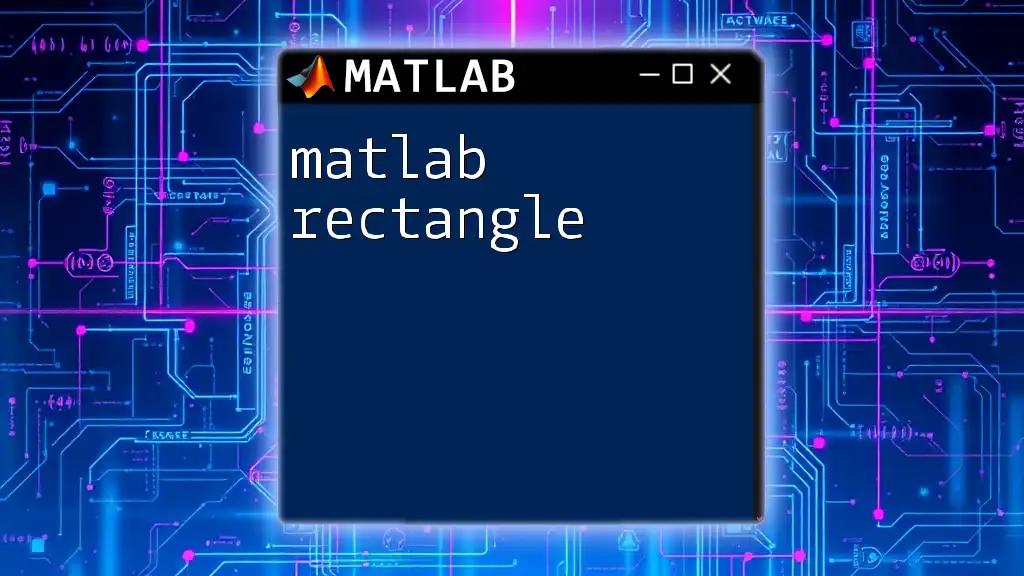
Additional Resources
Make use of official MATLAB documentation and join forums and communities that focus on MATLAB practices for ongoing discussions about precision challenges and solutions.
Final Thoughts
Consider sharing your experiences and approaches to increasing MATLAB precision with others in the community. Your insights could help others navigate similar challenges and enhance their MATLAB skills!