In MATLAB, you can customize tick marks on a duration axis by using the `xticks` and `xticklabels` functions to define specific tick positions and labels for better data visualization.
Here's a code snippet to illustrate this:
% Create sample duration data
durations = minutes(0:10:120);
values = rand(1, length(durations)); % Random values for demonstration
% Plot the data
plot(durations, values);
% Set custom tick marks and labels on the x-axis
xticks(minutes(0:30:120)); % Specify tick positions
xticklabels({'0 min', '30 min', '60 min', '90 min', '120 min'}); % Specify tick labels
xlabel('Duration'); % Label for the x-axis
ylabel('Values'); % Label for the y-axis
title('Plot with Customized Duration Axis Tick Marks'); % Title of the plot
grid on; % Add grid for better visibility
Understanding MATLAB Duration
What is MATLAB Duration?
In MATLAB, duration is a data type used to represent a length of time. It is particularly useful for handling time-related data, allowing for easy arithmetic operations, comparisons, and conversions. You can create duration objects with the `duration()` function, specifying hours, minutes, seconds, and even fractional components. Here’s a basic example:
t1 = duration(1, 30, 0); % 1 hour and 30 minutes
t2 = duration(0, 45, 0); % 45 minutes
With these objects, `t1` represents 1 hour and 30 minutes, while `t2` expresses 45 minutes.
Importance of Duration in Data Visualization
When visualizing time-series data, using duration data types enhances clarity and comprehension of the information being displayed. Duration axes allow the viewer to interpret changes over time effectively. For instance, if you plot durations related to traffic flow over a day, viewers will quickly see patterns like peak hours and slow periods. It’s a step towards better data storytelling.
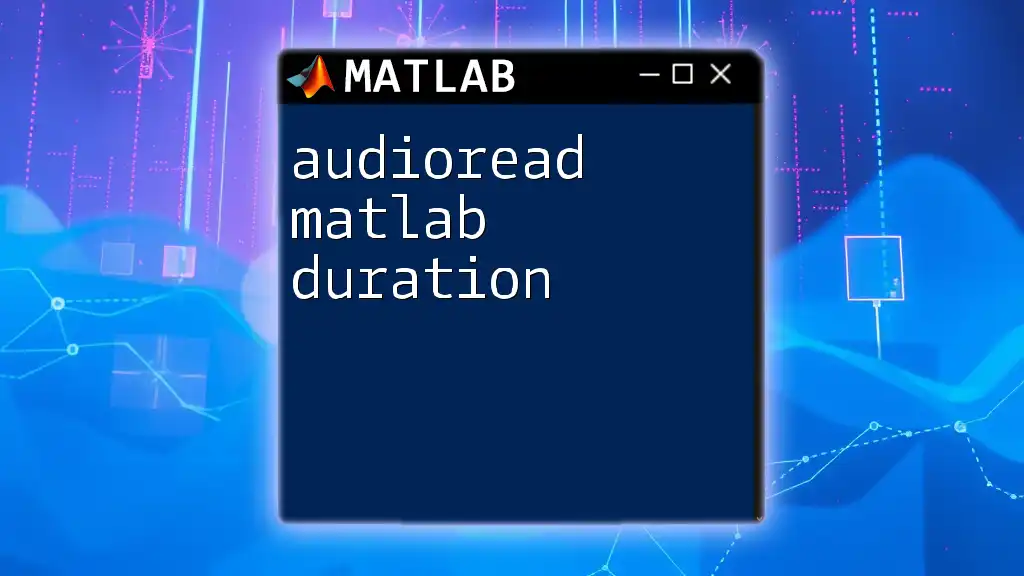
Basic Plotting with Duration
Creating a Simple Duration Plot
Plotting makes it easier to comprehend complex datasets. With the duration data type, you can create a simple plot that displays values over time. Here’s how you can do this:
timeData = duration(0, 0, 0, [0 1 2 3 4], 'Format', 'hh:mm:ss');
dataValues = rand(1, 5); % Random example data
figure;
plot(timeData, dataValues);
xlabel('Time');
ylabel('Random Values');
title('Simple Duration Plot');
This code generates a plot of random values against duration data, with x-axis labels formatted as hours, minutes, and seconds, allowing for an intuitive understanding of how the data changes over time.
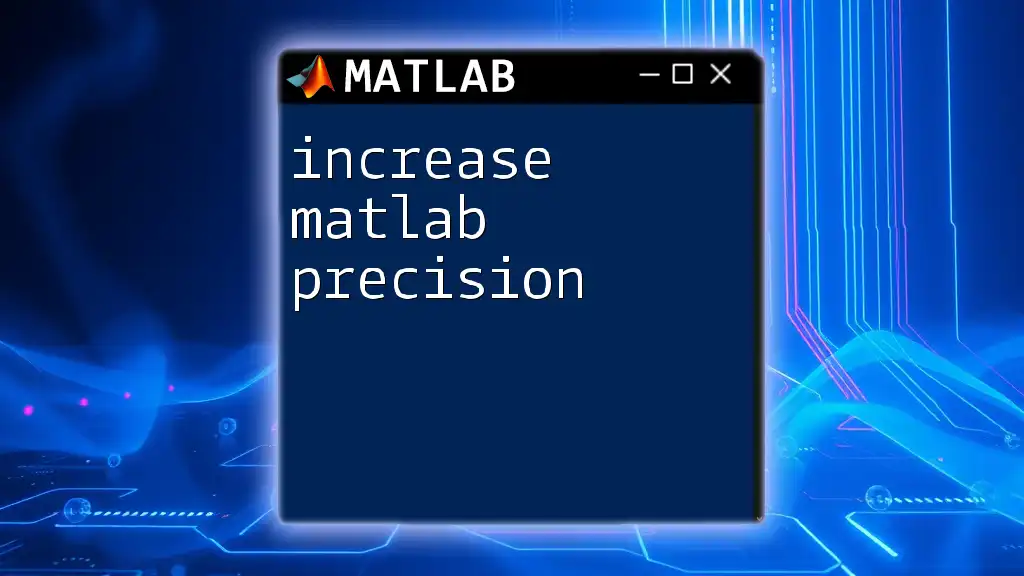
Customizing Tick Marks on Duration Axis
Default Tick Marks in MATLAB
By default, MATLAB generates tick marks automatically based on the data range. However, these automatic placements may not always meet your specific visualization needs, which is why custom tick marks can significantly enhance your plot.
Modifying Tick Marks
Set Specific Tick Marks
To have more control over your plot, you can manually set tick marks using the `xticks` function. This allows you to define meaningful intervals that accurately represent your data. For instance:
xticks([duration(0,0,0,0), duration(0,0,0,1), duration(0,0,0,2)]);
This command defines specific tick marks at 0, 1, and 2 minutes along the duration axis. This technique eliminates clutter and ensures that the viewer focuses on the most important points in your data.
Formatting Tick Labels
Once you’ve set your tick marks, you may want to customize their labels to reflect meaningful descriptions. This is done with the `xticklabels` function. Here’s an example of how to label these ticks:
xticklabels({'0m', '1m', '2m'}); % Label tick marks in minutes
Proper labeling helps guide the viewer’s comprehension of what each tick mark signifies, transforming vague marks into clear indicators of time intervals.
Adjusting Tick Mark Intervals
To ensure your tick marks are not overcrowded or too sparse, you can fine-tune the intervals using the `xtickformat` function. This function adjusts how the tick marks display their values more intuitively. Here’s how:
xtickformat('hh:mm:ss'); % Set the format for x-ticks
This command changes the format of the tick labels to hours, minutes, and seconds, maintaining precision while reducing viewer fatigue.
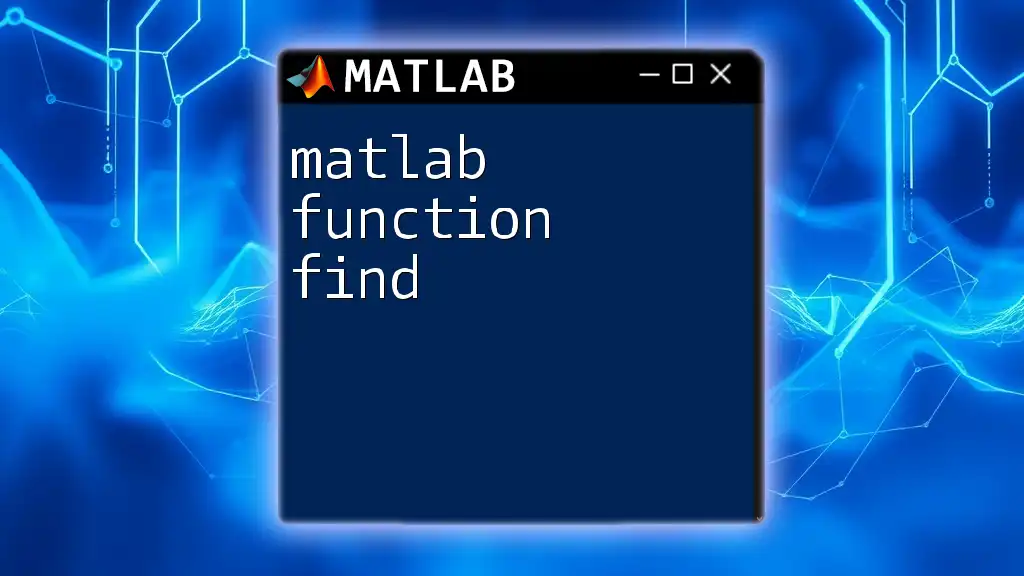
Advanced Customization Techniques
Using `Datetime` for Tick Marks
While duration is excellent for representing time lengths, using `datetime` can offer even more precision. When plotting events with specific timestamps, consider using `datetime` for your x-axis. For example:
datetimeData = datetime(2023, 10, 1, 0, 0, 0) + hours(0:4);
dataValues = rand(1, 5); % New dataset
figure;
plot(datetimeData, dataValues);
xlabel('Date and Time');
ylabel('Random Values');
title('Example with Datetime');
This approach supports time-based analysis at a granular level, particularly when you have data points tied to exact moments.
Combining Duration and Multiple Y-Axes
For more complex data representation, MATLAB lets you plot against a shared duration x-axis while utilizing multiple y-axes. This technique is beneficial when you want to demonstrate relationships between different datasets.
Example:
yyaxis left;
plot(timeData, dataValues); % Left y-axis for first dataset
yyaxis right;
plot(timeData, dataValues * 10); % Different dataset on right y-axis
xlabel('Time');
ylabel('Values');
title('Multi-Y Axis Example with Duration');
This code snippet highlights how to maintain clarity by visually distinguishing between two datasets while still presenting them on a unified temporal basis.
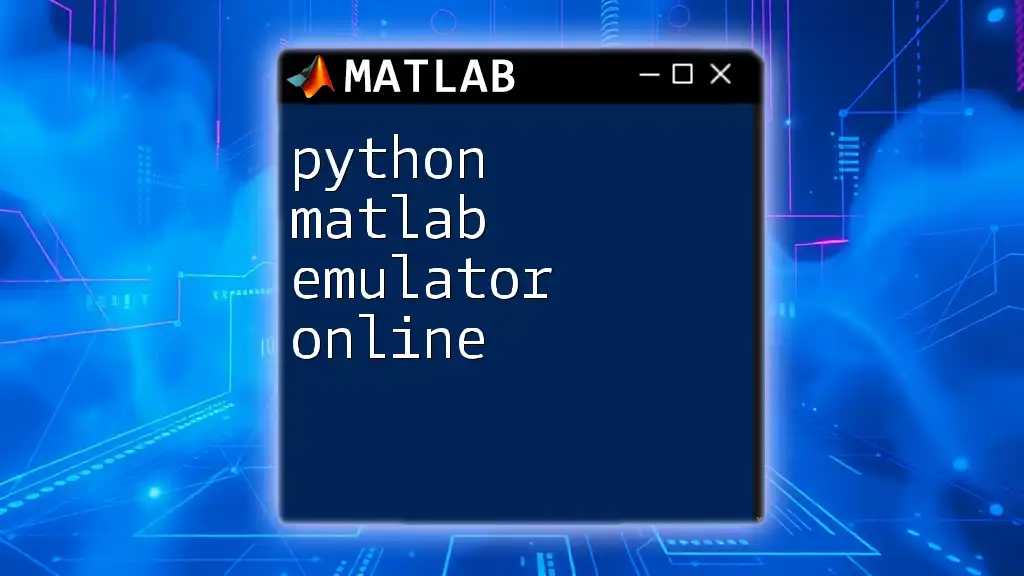
Troubleshooting Common Issues
Common Problems with Tick Marks on Duration Axis
When working with tick marks on the MATLAB duration axis, you might encounter several common issues:
- Overlapping tick labels can make a plot unreadable.
- Ticks not appearing properly if the duration range is not set correctly.
Each of these problems can disrupt the visual clarity of your data. Take careful note of your data ranges and ensure your ticks are appropriately defined for your specific dataset.
Accessing Properties of Axes
Understanding how to access and modify axis properties is key to troubleshooting issues. You can use the `gca` command to access the current axes object. This helps you debug your plot effectively.
ax = gca;
disp(ax.XTick); % Display current x-ticks
By examining the properties of your axes, you can get insights into what may be causing any tick-related issues and make informed adjustments.
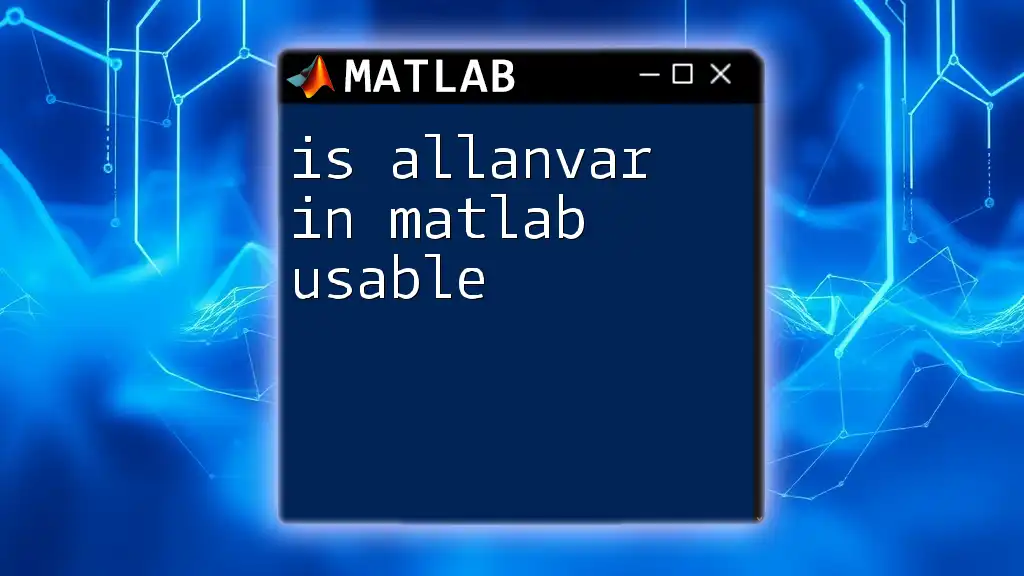
Conclusion
In conclusion, mastering tick marks on the MATLAB duration axis is a crucial skill for data visualization. By understanding the fundamentals of the duration data type and applying advanced customization techniques, you can create clear, informative visual representations of time-series data. This ability not only enhances your plots but also communicates your data story effectively to your audience. Don’t hesitate to experiment with varied functionalities and properties to enrich your MATLAB experience!
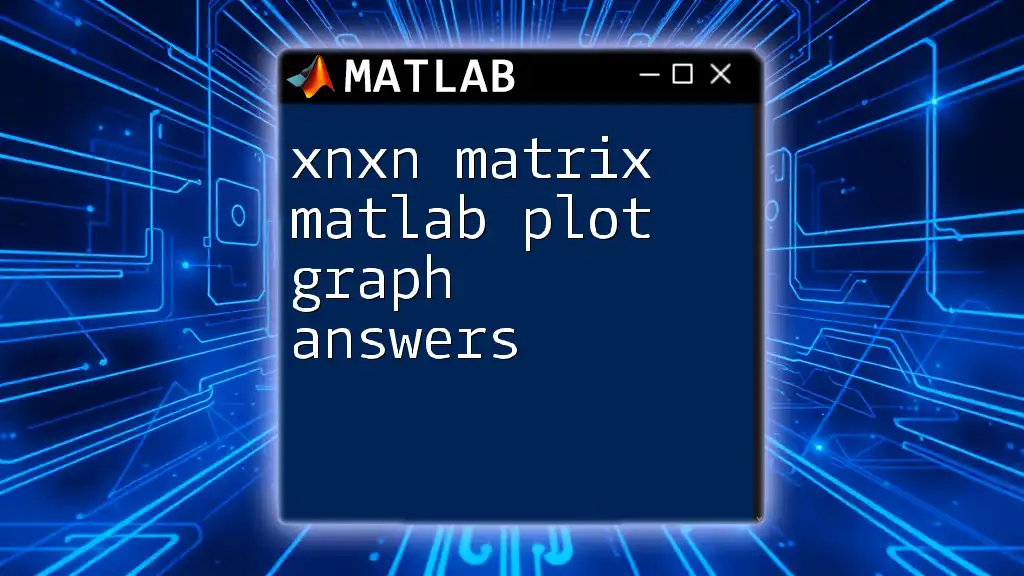
Additional Resources
For further exploration, refer to the official MATLAB documentation on `duration` and plotting functions. Practice enhancing your existing plots by applying the techniques covered in this guide.