The `datestr` function in MATLAB is used to convert serial date numbers or date strings into human-readable date formats.
% Example of using datestr to convert a serial date number to a formatted string
dateNum = now; % Current date and time as a serial date number
dateStr = datestr(dateNum, 'dd-mmm-yyyy HH:MM:SS'); % Convert to formatted string
disp(dateStr); % Display the formatted date and time
What is `datestr`?
The function `datestr` in MATLAB is used to convert date numbers, date vectors, or datetime objects into readable string representations. This functionality is crucial for effectively managing and presenting time-stamped data, which is especially important in data analysis, reporting, and visualization.
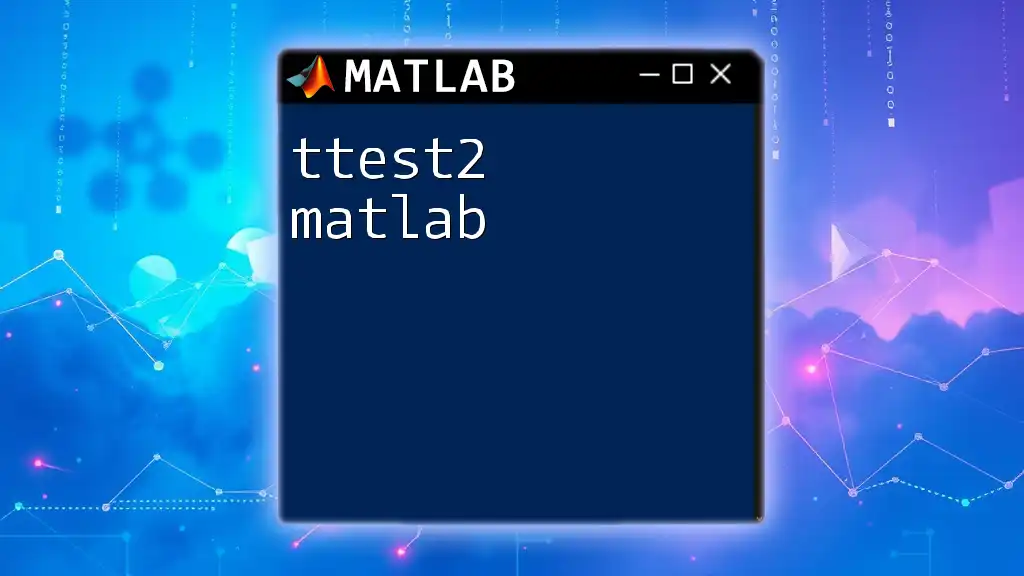
Use Cases for `datestr`
When working with large datasets or time-related data, consistent date formatting is essential. `datestr` is commonly used in scenarios such as:
- Formatting timestamps for reports or visualizations.
- Manipulating and displaying dates from datasets.
- Preparing data for time series analyses.
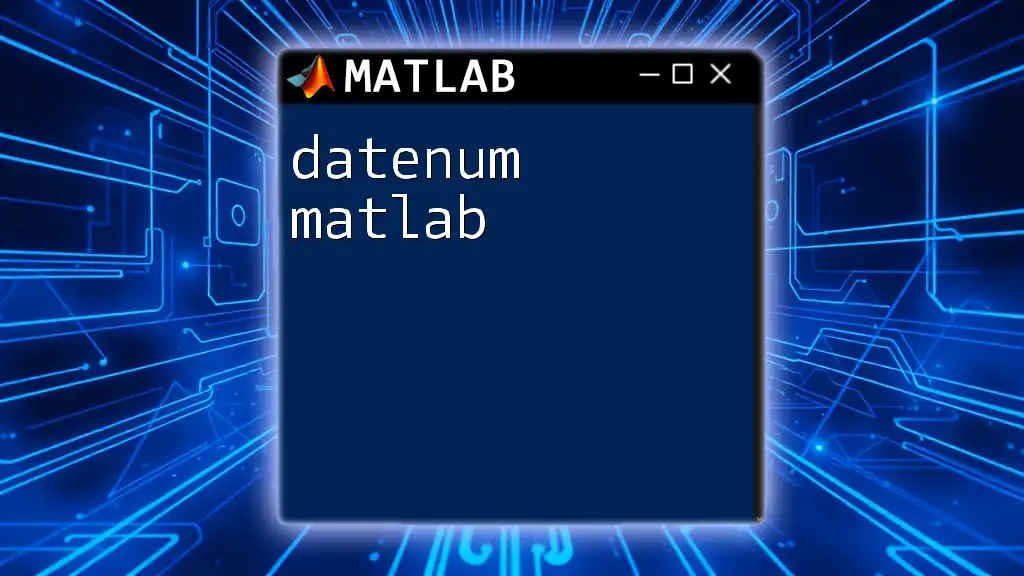
Understanding Date Formats in MATLAB
Overview of Date Representations
In MATLAB, dates are represented as serial date numbers. This means that a specific date is stored as a number, which counts the number of days from a fixed date (January 0, 0000). This numeric format allows for easy mathematical manipulation but is not always human-readable.
Common Date Formats
MATLAB supports a variety of date formats that can be specified in `datestr`. Some common formats include:
- `'dd-mmm-yyyy'`: Displays the date with a two-digit day, abbreviated month, and full year.
- `'mm/dd/yyyy'`: Uses slashes to separate the components of the date.
How MATLAB Stores Dates and Times
Understanding related functions is essential for mastering `datestr`. For instance:
- `datenum` converts datetime strings into serial date numbers.
- `datevec` translates date numbers into date vectors, which can then be manipulated for various applications.
- `datetime` is a newer object type in MATLAB designed to manage dates and times effectively, simplifying many date operations.
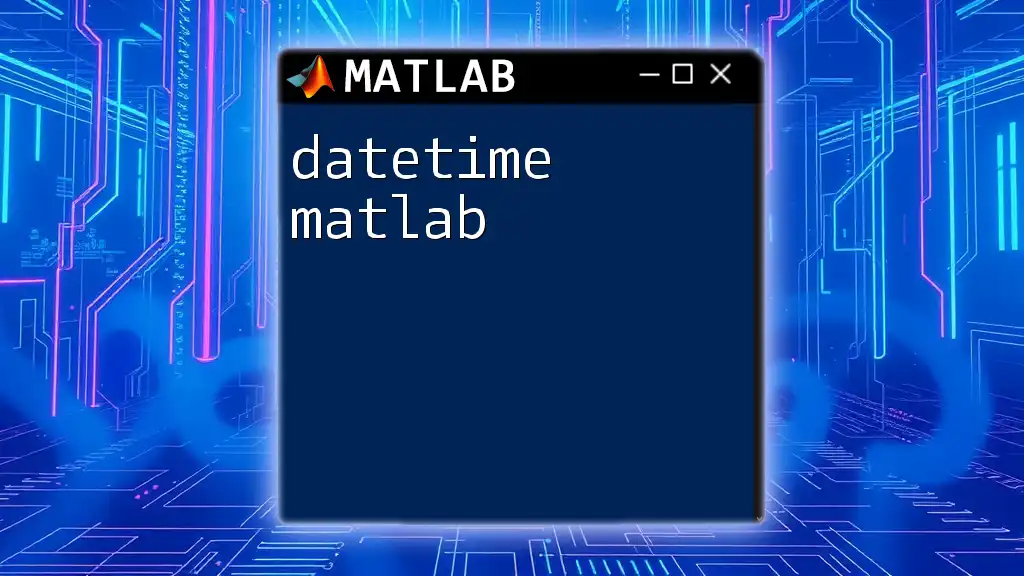
The Syntax of `datestr`
Basic Syntax
The basic syntax of `datestr` involves passing a date number (or datetime object) and a format string. The general form is as follows:
datestr(datenum, format)
Input Types for `datestr`
Date Serial Number
Using `datestr` with a date serial number is straightforward. For example, if you want to convert the date string '2023-10-01' into a more readable format, you can do the following:
dt = datenum('2023-10-01');
output = datestr(dt, 'dd-mmm-yyyy');
disp(output); % Output: '01-Oct-2023'
This example illustrates how you can easily convert a date into a format that is more understandable.
Datetime Objects
When using `datestr` with `datetime` objects, the function simplifies date management. For example, consider the following code:
dt = datetime('now');
output = datestr(dt);
disp(output); % Displays the current date and time in default format.
This representation is automatically formatted by `datestr`, showcasing the convenience of using `datetime` alongside this function.
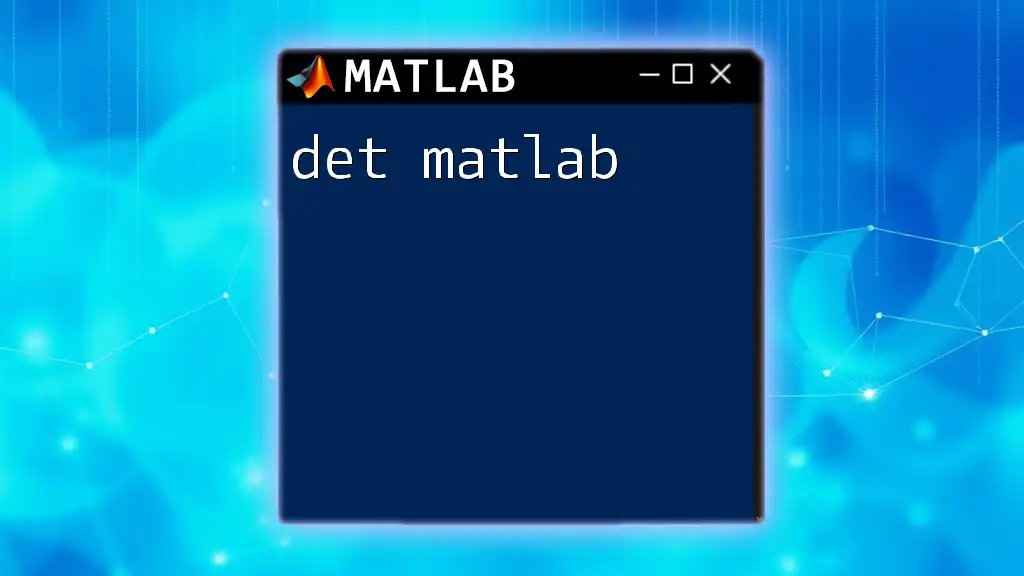
Customizing Date Formats
Built-in Format Options
MATLAB provides built-in formats for users’ convenience. Some common ones include:
- `'dd-mm-yyyy'`
- `'mmm dd, yyyy'`
You can leverage these built-in formats without additional effort. For example:
dt = now;
output1 = datestr(dt, 'dd-mm-yyyy');
output2 = datestr(dt, 'mmm dd, yyyy');
disp(output1); % Output: '01-10-2023'
disp(output2); % Output: 'Oct 01, 2023'
Creating Custom Formats
Creating custom formats gives you flexibility. You can specify your desired format by using symbols like:
- `yyyy` for the year.
- `MM` for the month.
- `dd` for the day. For example, you can write:
output = datestr(dt, 'yyyy/mm/dd HH:MM:SS');
disp(output); % Outputs as '2023/10/01 12:00:00' if executed at noon on that date.
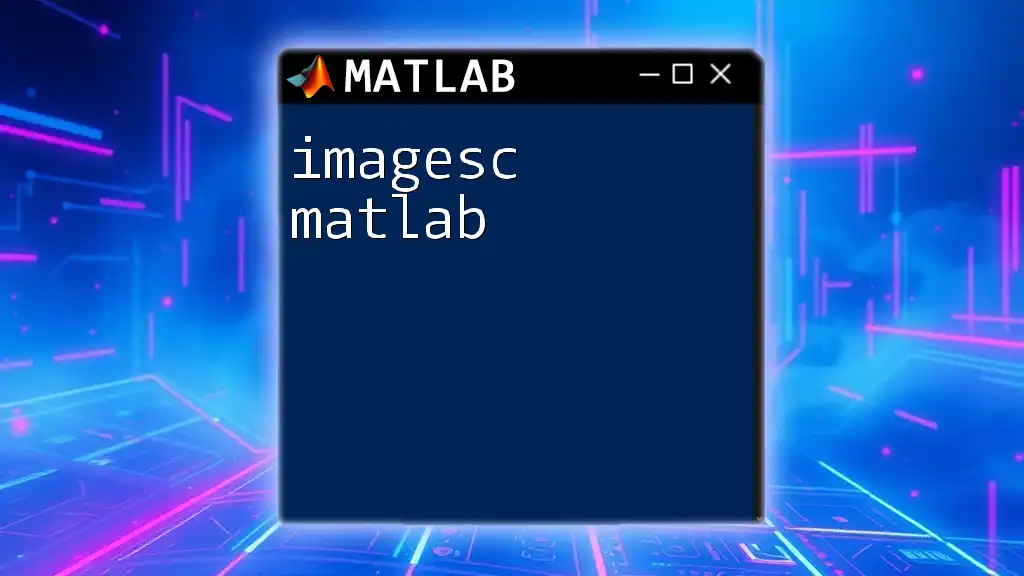
Practical Applications of `datestr`
Example 1: Formatting Timestamps for Reports
When generating reports, it's essential to display timestamps correctly. Consider a report where you want to format a timestamp for clarity:
reportDate = now; % Current date and time
formattedDate = datestr(reportDate, 'dddd, mmmm dd, yyyy HH:MM:SS');
disp(['Report generated on: ', formattedDate]); % E.g., 'Report generated on: Sunday, October 01, 2023 12:00:00'
This output enhances the presentation of critical information.
Example 2: Data Analysis with Date Formatting
Within data analysis, handling dates correctly is vital, especially in time series analysis. Here's how you might prepare dates for examination:
dates = {'2023-01-01', '2023-02-01', '2023-03-01'};
formatted_dates = cellfun(@(x) datestr(datenum(x)), dates, 'UniformOutput', false);
disp(formatted_dates); % Outputs cell array of formatted dates in default format.
This code shows how to work with a list of string dates and convert them collectively into a suitable format.
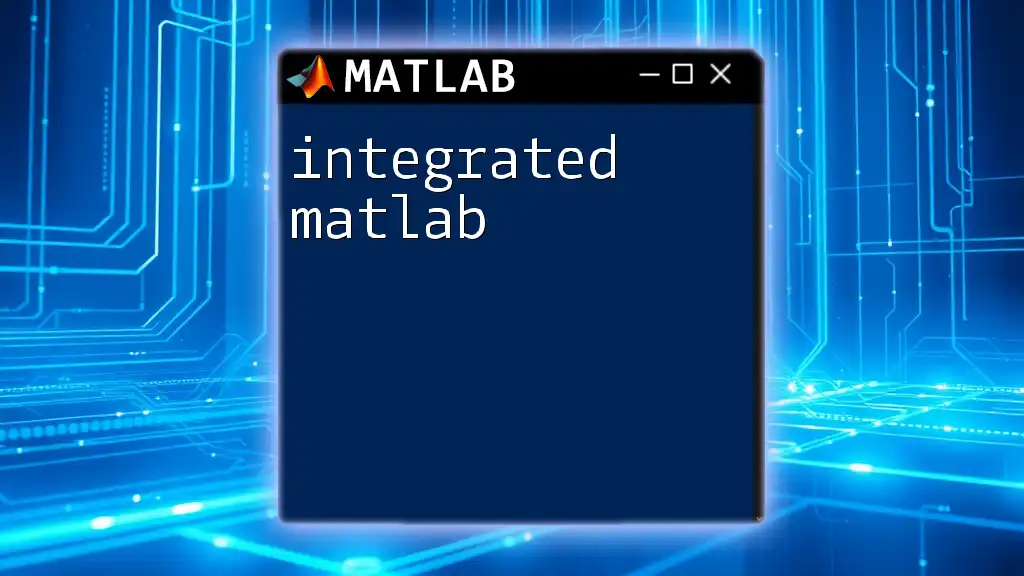
Common Errors and Troubleshooting
Common Issues with `datestr`
While using `datestr`, you might encounter some common pitfalls, such as:
- Invalid Date Formats: Make sure your format string uses the correct symbols. If a non-existent date format is applied, it may lead to errors or unexpected results.
Performance Considerations
When dealing with large datasets, performance becomes an issue. Excessive use of `datestr` in a loop may slow down your program. Consider vectorized operations or converting dates in batches to enhance performance.
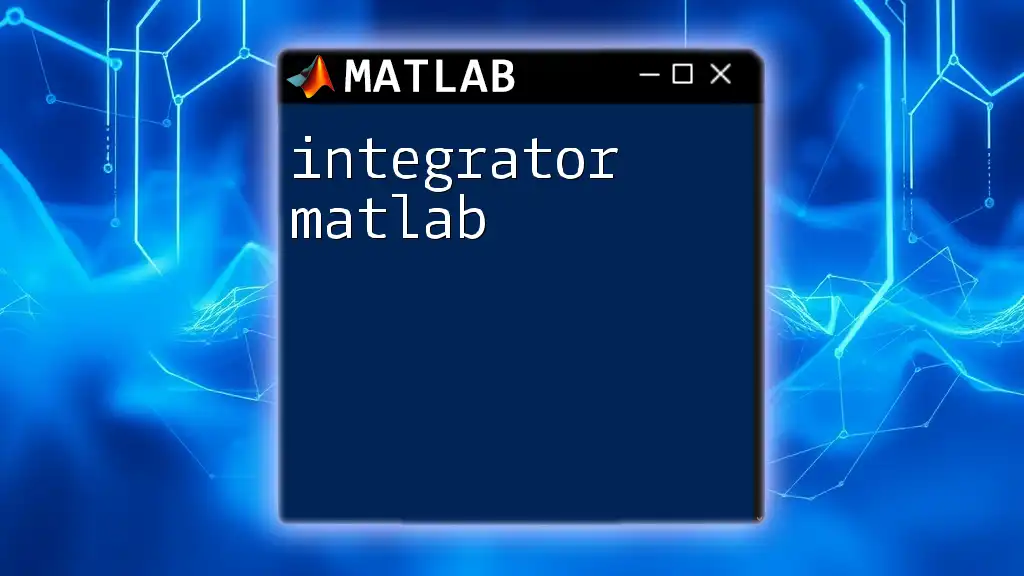
Conclusion
Mastering `datestr` significantly enhances your ability to manipulate and present dates in MATLAB. This function is invaluable for organizing and displaying your data effectively. By leveraging built-in and custom formats, you can adapt to various reporting needs and personal preferences.
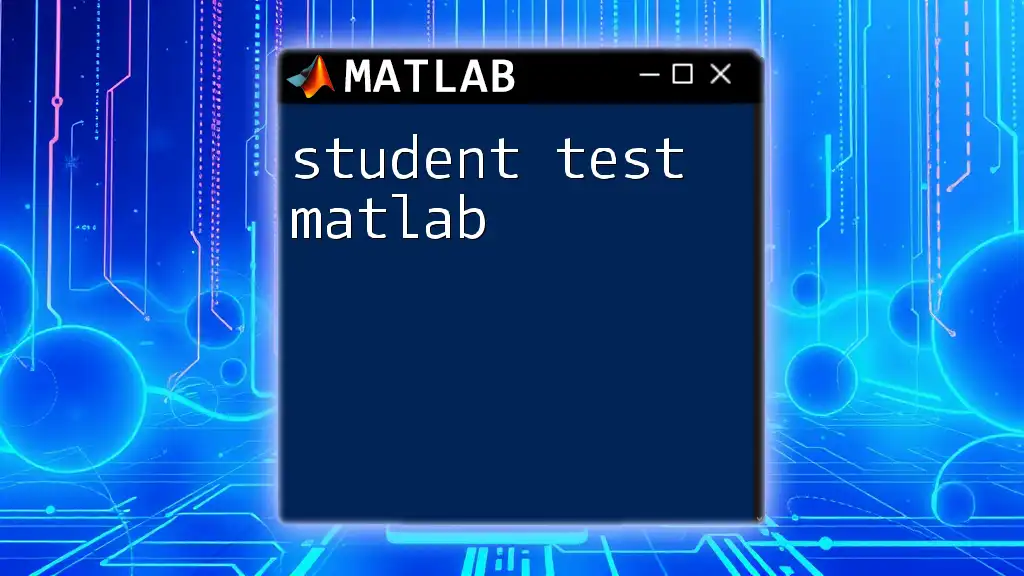
Call to Action
We encourage you to experiment with `datestr` in your MATLAB projects! Understanding how to effectively convert and format dates will simplify your programming tasks. If you're eager to enhance your MATLAB skills further or seek support, consider joining our community for more hands-on guidance and learning resources.