Indexing in MATLAB allows you to access and manipulate elements within arrays and matrices using their row and column indices.
Here’s a simple example of indexing a 2D matrix:
% Create a 3x3 matrix
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
% Access the element in the second row, third column
element = A(2, 3); % element = 6
What is Indexing?
Indexing in programming refers to the method of accessing and manipulating data stored in data structures like arrays and matrices. In the context of indexing MATLAB, it is crucial for efficiently managing and performing operations on large datasets due to MATLAB's matrix-based architecture. Mastery of indexing enables users to streamline tasks, optimize routines, and enhance data analysis capabilities.
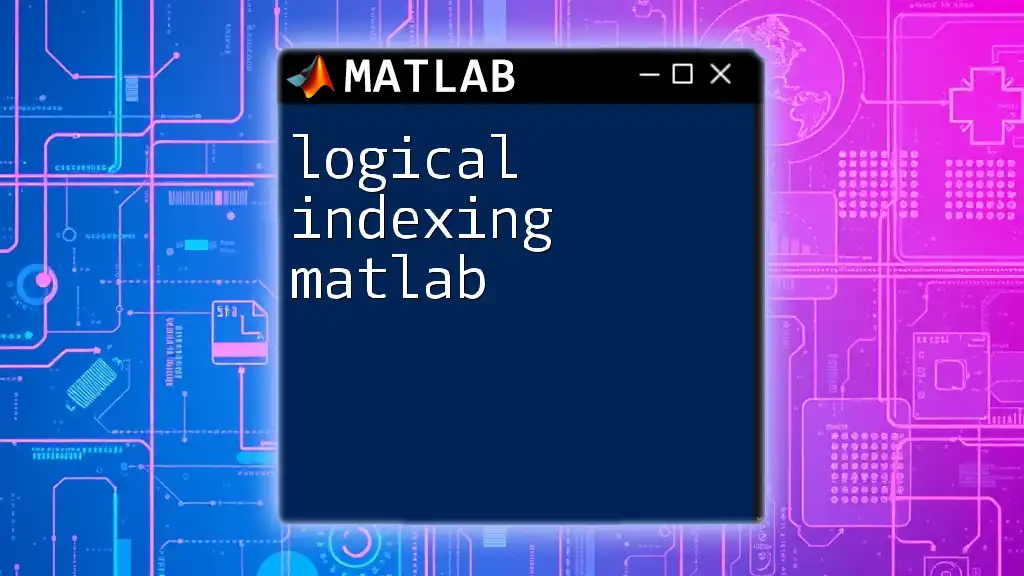
Why Indexing Matters in MATLAB
The significance of indexing in MATLAB cannot be overstated. MATLAB is inherently designed around matrices, and understanding indexing allows users to effectively retrieve and manipulate specific subsets of data. This proficiency is essential in various domains, including signal processing, machine learning, and engineering simulations. Furthermore, it simplifies complex operations, making code more readable and maintainable.
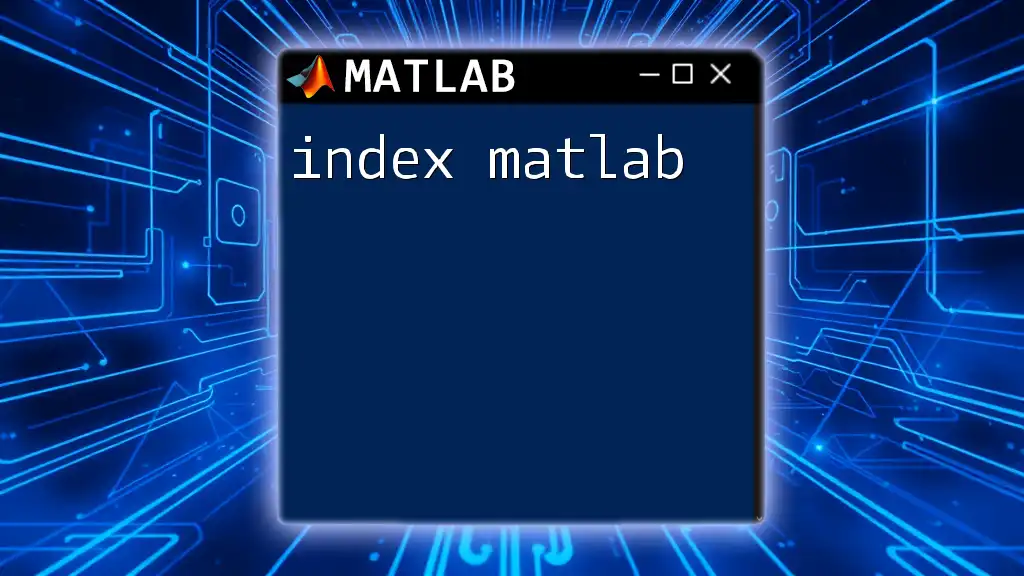
Types of Indexing in MATLAB
Linear Indexing
Linear indexing allows you to access elements of a matrix using a single index. MATLAB stores matrices in a column-major order, meaning elements are referenced linearly from top to bottom, and then left to right.
Using linear indexing provides a streamlined approach, especially when handling high-dimensional arrays.
Code Snippet Example
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
linear_index = A(5); % This retrieves the element '5' from the matrix.
In this example, `A(5)` accesses the fifth element in vectorized form, resulting in a clear and concise way to obtain elements.
Use Cases
Linear indexing can be particularly effective when performing operations on specific elements across different dimensions where traditional indexing may be cumbersome.
Row and Column Indexing
Row and column indexing enables targeting specific elements based on their row and column positions, ideal for focused manipulation of matrix data.
With this method, users can access entire rows or columns or even a subsection of the matrix.
Code Snippet Example
row_col_element = A(2, 3); % Accessing the element at the 2nd row and 3rd column
This line retrieves the element '6' from the matrix, highlighting how easily one can interact with specific data points.
Use Cases
This form of indexing is especially useful in scenarios where conditional operations need to be applied to entire rows or columns, such as normalization processes.
Logical Indexing
Logical indexing leverages logical arrays (arrays containing true/false values) to filter or manipulate data. This indexing method allows for powerful and expressive data operations.
Code Snippet Example
B = A > 5; % Creates a logical matrix indicating where elements of A are greater than 5
filtered_elements = A(B); % Retrieves the elements greater than 5
In the above example, the logical matrix `B` marks the positions where elements exceed 5, and `filtered_elements` isolates these values effectively.
Use Cases
Logical indexing shines in data analysis tasks where conditions must dictate data selection, such as filtering results or applying constraints.
End Indexing
End indexing utilizes the `end` keyword to refer to the last element of an array or matrix dimension. This promotes code simplicity and adaptability, particularly in dynamically sized datasets.
Code Snippet Example
last_element = A(end); % Accessing the last element of a linear array
last_row_elements = A(end, :); % Accessing all elements of the last row
Using `end` offers flexibility, allowing the code to adjust automatically to array size changes without manual updates.
Use Cases
This technique is beneficial in loops and iterative methods, especially when handling extensive arrays or datasets where dimensions may fluctuate.
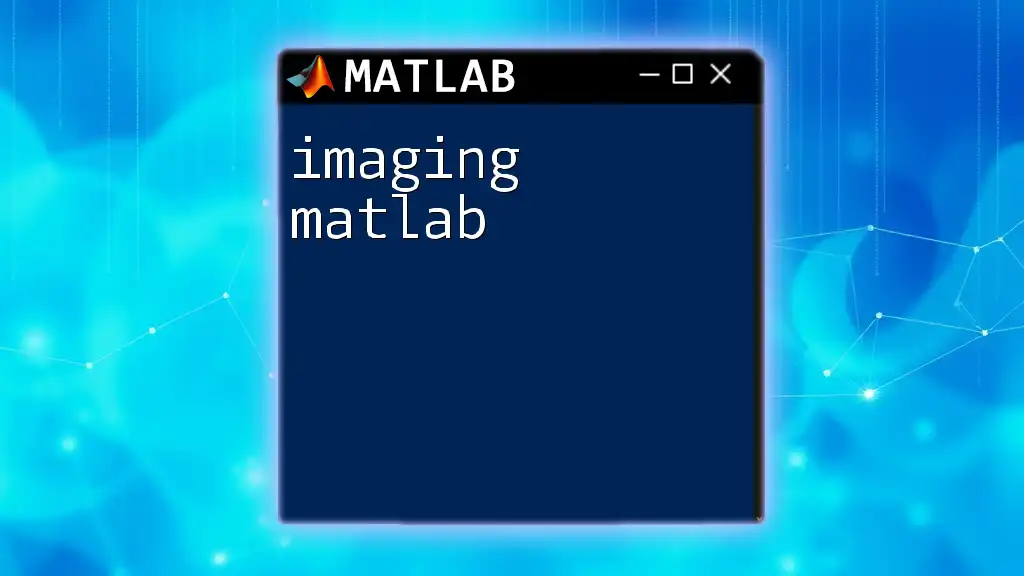
Advanced Indexing Techniques
Using the `find` Function
The `find` function is a powerful tool in indexing MATLAB. It returns the indices of non-zero or true elements in an array, making it ideal for conditional operations that require knowing where specific criteria are met.
Code Snippet Example
indices = find(A > 5); % Returns indices of elements greater than 5 in matrix A
This simple yet effective command provides a direct mapping of conditionally-selected elements, streamlining data retrieval processes.
Use Cases
The `find` function is invaluable in large datasets, allowing for efficient indexing where filtering and condition-based selection are necessary.
Multidimensional Indexing
With multidimensional indexing, MATLAB allows access to elements across arrays that extend beyond two dimensions, accommodating complex data structures. This capability is crucial in fields like 3D modeling or multi-frame data analysis.
Code Snippet Example
C = rand(2, 3, 4); % Creates a random 3D array with dimensions 2x3x4
element_3D = C(1, 2, 3); % Accessing a specific element in the 3rd dimension
This way of indexing empowers users to navigate and manipulate multidimensional datasets completely.
Use Cases
Applied in various contexts such as computer graphics, simulations, and tensor computations, multidimensional indexing is an essential aspect of advanced MATLAB functions.
Cell Array Indexing
Cell arrays allow for storage of mixed data types, and understanding how to properly index cell arrays is crucial for managing heterogeneous datasets.
Code Snippet Example
cellArray = {1, 'text', [1, 2, 3]};
firstElement = cellArray{1}; % Accessing the first cell, which is '1'
Cell array indexing differs from regular indexing in MATLAB, as it requires the use of curly braces for direct access.
Use Cases
This method serves in situations requiring the combination of different data types, such as algorithms that manage various data sources or integration tasks across datasets.
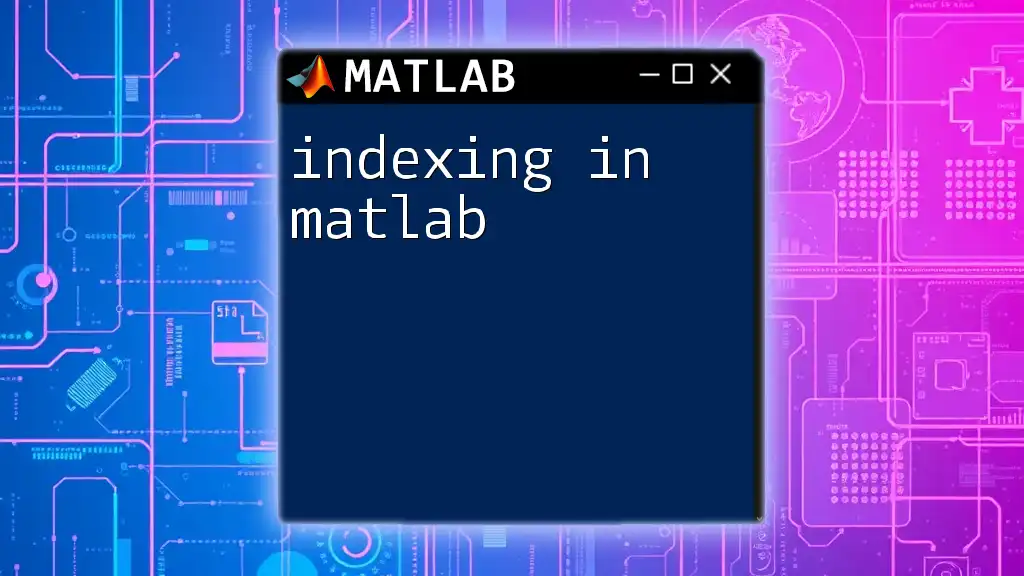
Common Indexing Pitfalls and Solutions
While indexing is a powerful tool, there are common pitfalls that users may encounter:
- Out-of-bounds errors: Attempting to access an index that does not exist in the array will throw an error.
- Confusion between linear and traditional indexing: Depending on the scenario, users may struggle with how matrices are structured and referenced.
To avoid indexing errors, always ensure the size and structure of your data match your indexing method. Debugging your code with smaller, simpler examples can help clarify any misunderstandings.
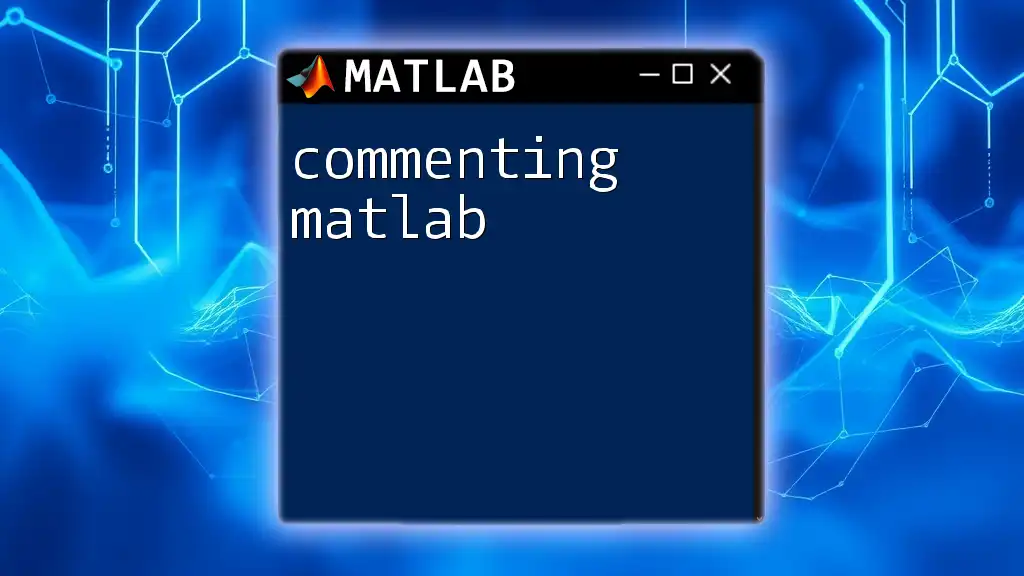
Conclusion
In summary, indexing MATLAB offers a robust toolkit for handling data within the MATLAB environment. From linear to logical indexing, understanding and applying these methods paves the way for better data management and manipulation. As you progress with MATLAB, practicing these indexing techniques will enhance your coding efficiency and effectiveness in various projects.
Additional Resources
For a deeper understanding, consider exploring the official MATLAB documentation, which is filled with examples and advanced techniques, or enrolling in specialized courses that delve into MATLAB programming.