To plot an \( n \times n \) matrix in MATLAB, you can use the `imagesc` function to visualize the data as a color-coded image.
% Example of plotting an nxn matrix
n = 10; % Size of the matrix
matrix = rand(n); % Create an nxn matrix with random values
imagesc(matrix); % Plot the matrix
colorbar; % Add a color scale
title('n x n Matrix Plot');
Understanding nxnxn Matrices
Definition of nxnxn Matrices
An nxnxn matrix is a three-dimensional array where each dimension has the same size, defined as n. Unlike two-dimensional matrices that contain rows and columns, nxnxn matrices are often visualized in a volumetric form, providing a rich representation of data in three-dimensional space. They are commonly used in various fields such as computer graphics, simulations, and scientific modeling.
For instance, in fluid dynamics, a 3D grid of points can represent velocity fields, while in medical imaging, volumetric data is often used to create 3D images of patients' internal structures.
Characteristics of nxnxn Matrices
A few important properties of nxnxn matrices include:
- They are usually symmetrical depending on the data being represented.
- The type of data that can be stored can vary; it can be integers, floating-point numbers, or complex data types. Understanding these properties is crucial as they affect how you manipulate and visualize your data in MATLAB.
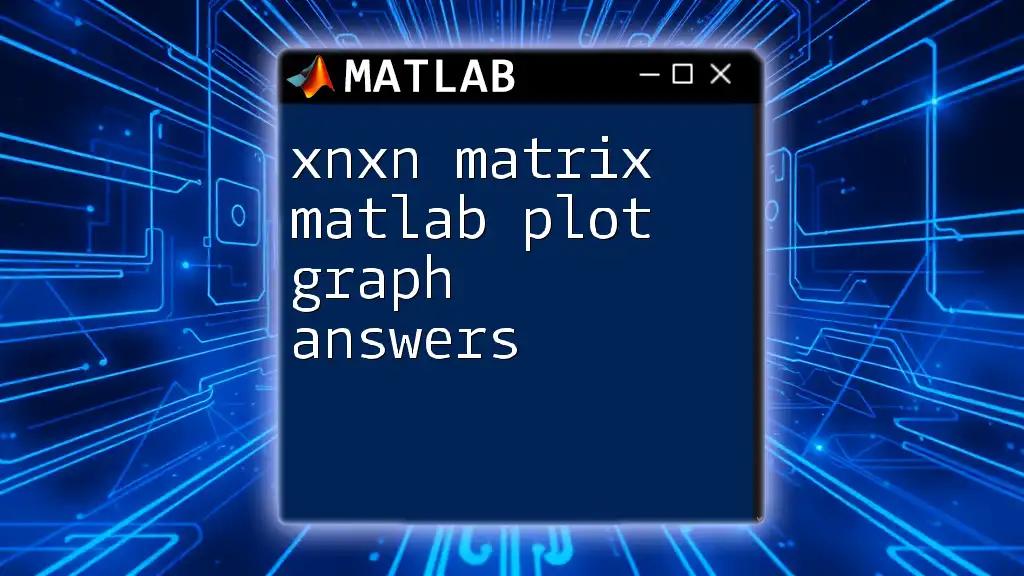
Setting Up Your MATLAB Environment
Installation and Configuration
To get started with MATLAB, ensure that you have the software installed on your system. You can find installation steps on the official MATLAB website.
Once installed, it’s essential to configure your workspace. Open MATLAB and familiarize yourself with the interface. This includes the Command Window, the Workspace, and the Editor, as each of these tools will be useful when executing commands related to your nxnxn matrix MATLAB plot.
Necessary Toolboxes
For 3D plotting, you may need specific toolboxes. The key toolbox for visualization in MATLAB is the MATLAB Graphics toolbox.
Make sure you have the following against your installation:
- Statistics and Machine Learning Toolbox: This toolbox is often useful for data analysis and manipulation.
- Image Processing Toolbox (if your work with nxnxn matrices involves image data).
To check if you have these toolboxes, use the `ver` command in the MATLAB Command Window. If they are missing, you can easily follow online guides to install them.
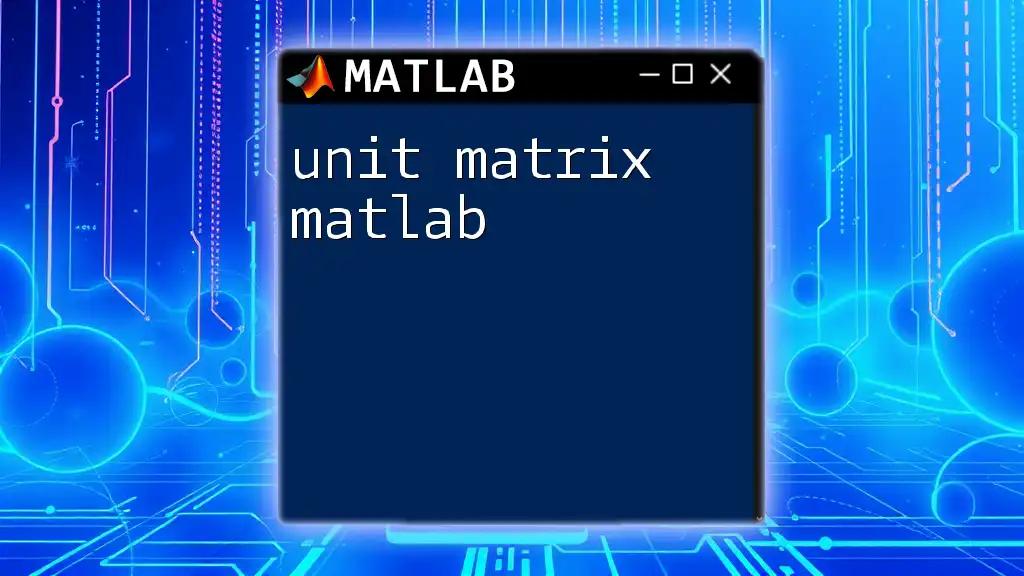
Basic Commands for Creating nxnxn Matrices
Generating Random Matrices
One of the easiest ways to get started with nxnxn matrices is to generate a random matrix using the `rand` function. Here’s how you can create an nxnxn matrix filled with random numbers between 0 and 1:
n = 3; % Size of matrix
A = rand(n, n, n);
This command initializes a 3x3x3 matrix `A` with random values. This is a common first step when experimenting with matrix visualization, as it allows you to generate varied datasets quickly.
Initializing Matrices Manually
You can also initialize a specific nxnxn matrix manually. For example, consider creating a 3D array of ones:
n = 3; % Size of matrix
A = ones(n, n, n);
This command constructs a matrix `A` where every element is 1. Such matrices can serve as base examples before you delve into more complex datasets.
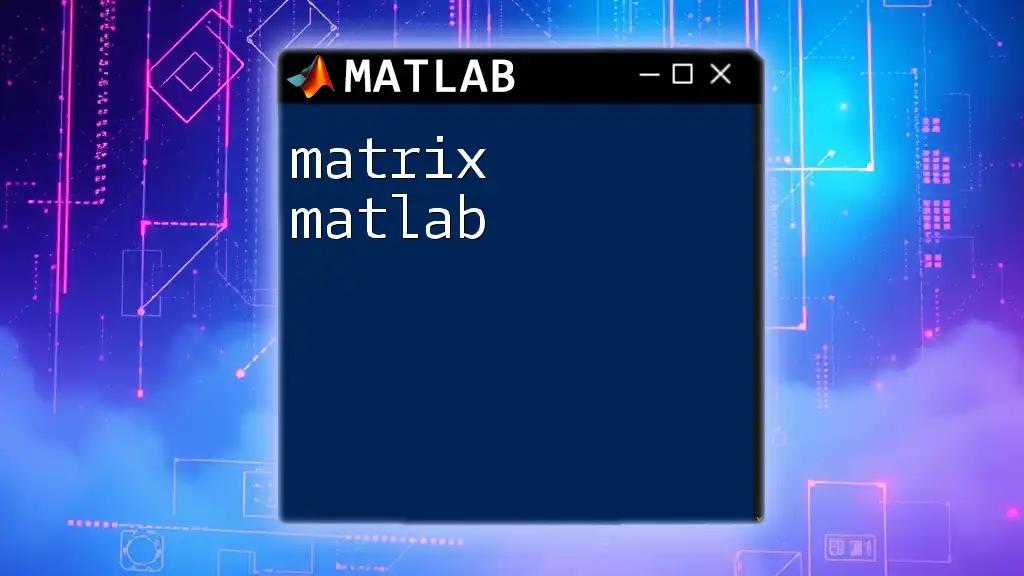
Plotting Techniques for nxnxn Matrices
3D Surface Plots
One of the most intuitive methods to visualize matrices in MATLAB is using surface plots. The `surf` function is designed for this purpose:
[X, Y, Z] = meshgrid(1:n, 1:n, 1:n); % Create a 3D grid of coordinates
surf(X(:, :, 1), Y(:, :, 1), A(:, :, 1)); % Plot the first slice
This code creates a surface plot for the first slice of your 3D matrix. Altering the third parameter in `A(:, :, 1)` changes which slice of the matrix you visualize, helping to better understand the changes across dimensions.
Slice Plots
Next, the slice function is particularly useful for visualizing multiple slices of the matrix at once:
slice(X, Y, Z, A, [], [], [1, 2, 3]); % Display slices at z = 1, 2, and 3
This command allows you to visualize cross-sections of the matrix along specified planes (in this case, `z = 1, 2, and 3`), providing clearer insight into the 3D data structure.
Volume Visualization
When dealing with volumetric data, using volume rendering tools can create a comprehensive visualization of your nxnxn matrix. The `vol3d` function is a useful command:
h = vol3d('cdata', A, 'xdata', X, 'ydata', Y, 'zdata', Z);
view(3); % Set view to 3D
This code will render an interactive volume plot, allowing you to inspect the data more thoroughly in a 3D context.
Heatmaps
Generating heatmaps can also provide valuable insights, especially when analyzing specific slices of your nxnxn matrix. Use the `imagesc` function, which is straightforward if you want to visualize a 2D slice:
imagesc(A(:, :, 1)); % Display the first slice as a heatmap
colorbar; % Add a color bar for reference
This generates a heatmap, where the colors represent different values in the slice of the matrix. Heatmaps are particularly useful for visual comparison among different data states.
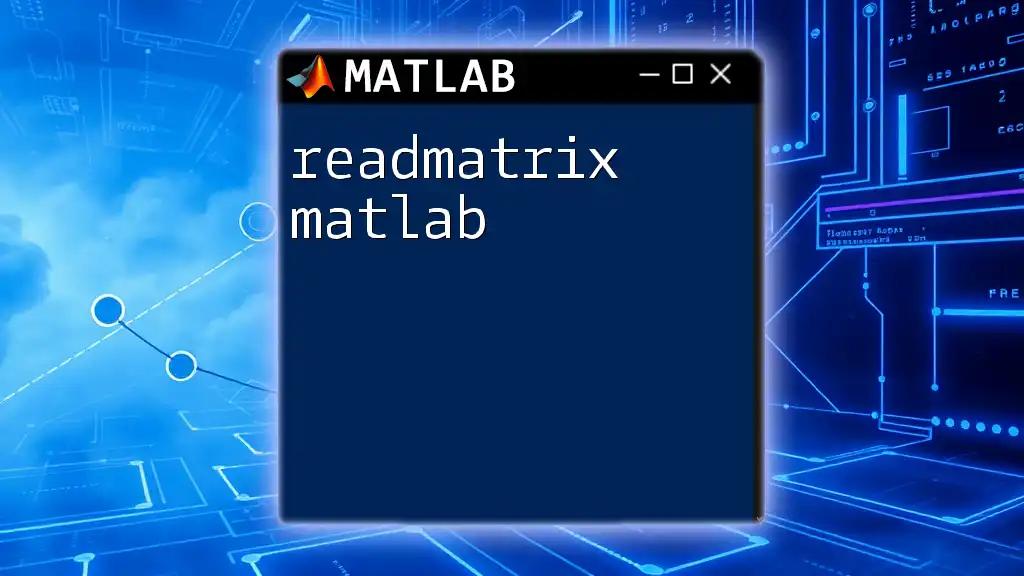
Advanced Visualization Techniques
Customizing Plots
Enhance your visual representations by customizing the appearance of your plots. You can change colors, labels, and titles using the following commands:
xlabel('X-axis label');
ylabel('Y-axis label');
zlabel('Z-axis label');
title('Custom 3D Plot');
Utilizing these commands can make your visualizations more informative and clearer to your audience, thereby enhancing data interpretation.
Animation of Matrices
Creating interactive and animated plots can be engaging and informative, especially when you need to represent changing data over time. Here’s a small example using `plot3` for animation:
for k = 1:n
plot3(X(:, :, k), Y(:, :, k), A(:, :, k), 'o');
pause(0.5); % Cool down period for viewing
end
This basic animation loops through the different slices of the matrix, plotting each in a 3D space. Animations can help visualize transformations or simulations effectively.
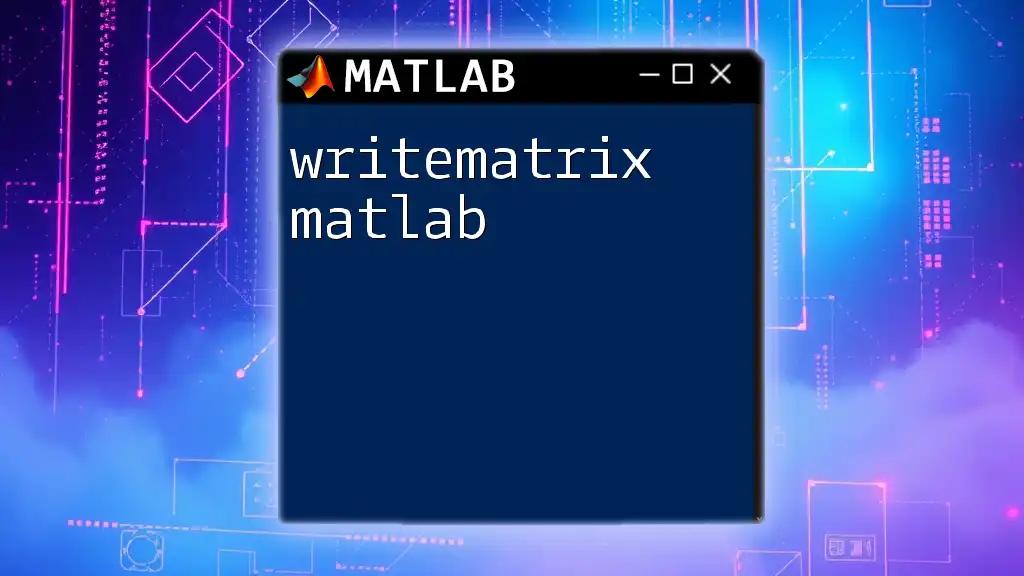
Troubleshooting Common Errors
Common Plotting Issues
While working with data visualization, you may run into errors, especially with 3D plotting. Common issues include mismatched dimensions or incorrect indexing. Here are a few troubleshooting tips:
- Check Dimensions: Use the `size` command to ensure your matrices align correctly.
- Debugging with Breakpoints: Insert breakpoints in your script to check variable states before plotting.
- Memory Management: For larger matrices, consider breaking them into smaller chunks to improve performance.
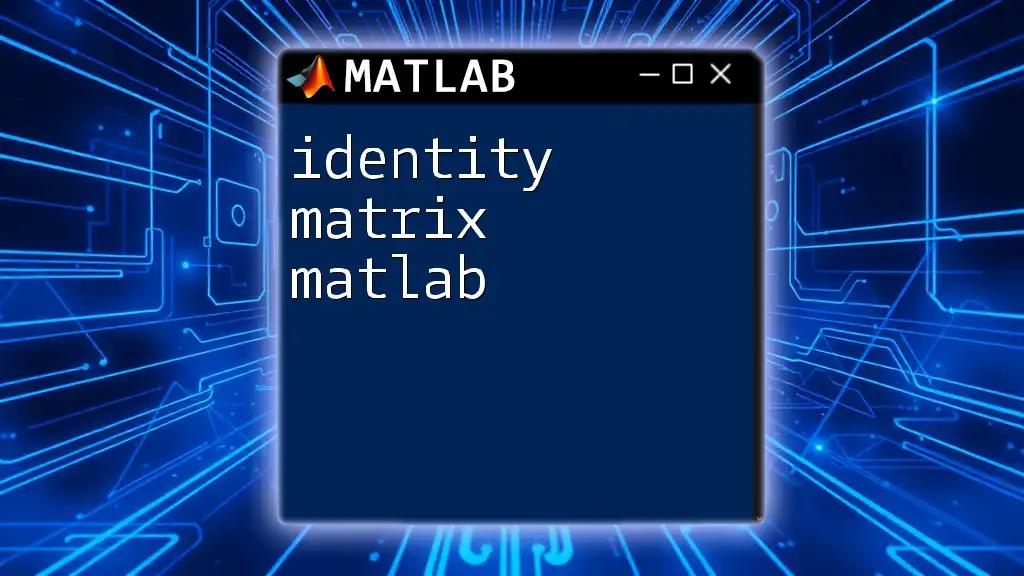
Conclusion
Visualizing nxnxn matrices in MATLAB through various plotting techniques is an invaluable skill for data analysis and presentation. Whether you start with random matrices or specific data, tools like `surf`, `slice`, and `vol3d` provide robust methods for graphical representation. By mastering these techniques and understanding how to manipulate your plots effectively, you will enhance your data storytelling capabilities.
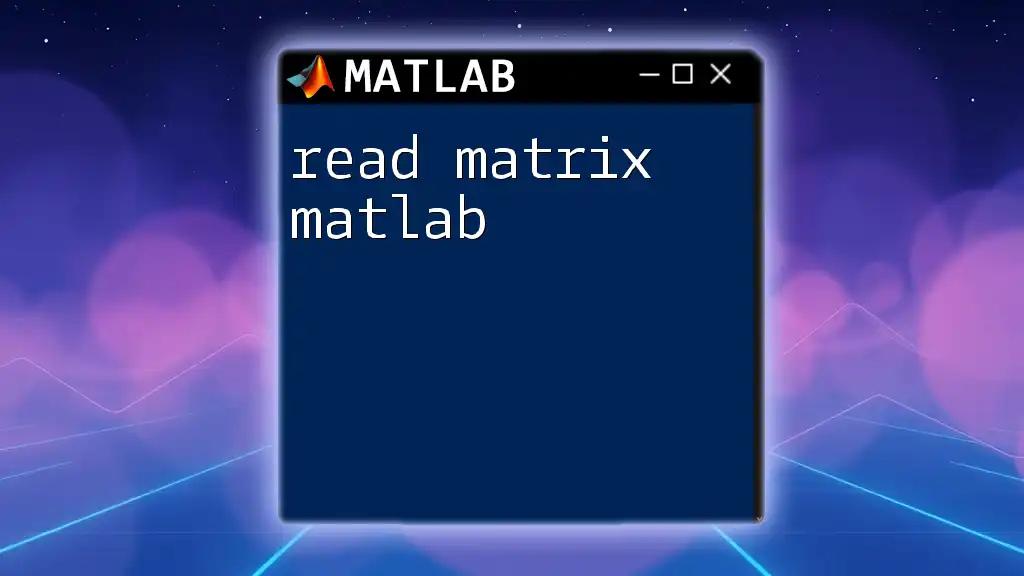
Additional Resources
Further Reading
Explore additional books, articles, or online forums dedicated to MATLAB and its extensive graphic capabilities. Engaging with the community helps deepen your understanding and uncovers more advanced strategies for data visualization.
MATLAB Documentation
Frequent reference to the official [MATLAB documentation](https://www.mathworks.com/help/matlab/) can provide further insights and examples. It’s highly recommended to keep it at hand as you navigate your data visualization journey.