To plot a matrix in MATLAB, you can use the `imagesc` function to visualize the data as a color-scaled image, where each element of the matrix is represented by a color.
matrix = rand(10); % Creates a 10x10 matrix of random values
imagesc(matrix); % Plots the matrix as a color-scaled image
colorbar; % Displays the color scale
Understanding Matrices in MATLAB
What is a Matrix?
A matrix is a two-dimensional array consisting of numbers arranged in rows and columns. In MATLAB, matrices play a crucial role in various computations, as they can represent data in a structured format. Each element in a matrix is identified by its position, defined by its row and column indices. Understanding how to manipulate and utilize matrices is fundamental to mastering MATLAB.
Creating Matrices in MATLAB
Creating matrices in MATLAB is straightforward and can be done in several ways:
-
Creating a Row and Column Matrix: To create a row matrix (also known as a vector), you can simply list the elements within square brackets:
row_matrix = [1, 2, 3, 4, 5]; % A 1x5 row matrix
For a column matrix, separate the elements with semicolons:
column_matrix = [1; 2; 3; 4; 5]; % A 5x1 column matrix
-
Creating a 2D Matrix: A 2D matrix can easily be formed using a combination of rows and columns:
matrix_2D = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % A 3x3 matrix
Exploring Matrix Properties
Understanding matrix properties is essential for effective data manipulation. You can use the following functions to explore matrix characteristics:
- `size()`: Returns the dimensions of the matrix.
- `length()`: Provides the length of the largest dimension.
- `class()`: Displays the data type of the matrix elements.
For instance:
dimensions = size(matrix_2D); % Get dimensions of matrix_2D
element_class = class(matrix_2D); % Get class type (e.g., double)
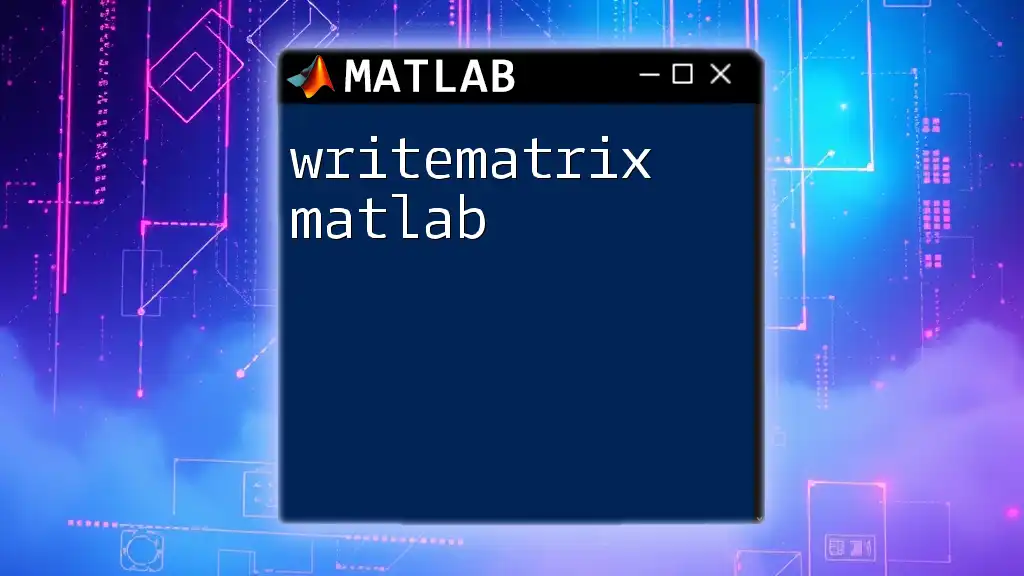
Plotting Basics in MATLAB
Introduction to MATLAB Plotting
MATLAB offers a robust framework for creating various types of plots that facilitate data visualization. Whether you are illustrating trends, comparing data sets, or highlighting discrepancies, plotting matrices is an invaluable tool in the analytical process.
Basic Plot Types
Several common plot types can be employed to visualize matrix data effectively:
- Line Plots: Ideal for showing trends over intervals, frequently used for time series data.
- Scatter Plots: Best for illustrating the relationship between two variables, particularly when data points are numerous.
- Bar Graphs: Useful for comparing discrete categories of data.
Each plot serves a unique purpose and can provide distinct insights based on the data being analyzed.
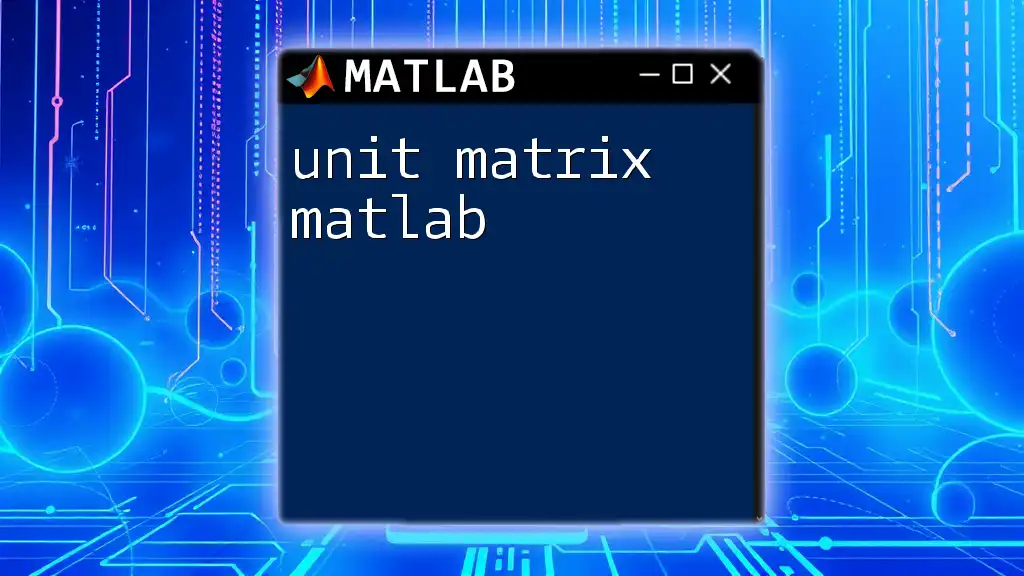
Plotting Matrices: Techniques and Steps
Visualizing Matrices with `imagesc()`
The `imagesc()` function is used to visualize a matrix as a colored image, where colors represent different matrix values.
- Purpose and Visual Representation: This method is incredibly effective for conveying variations in data, especially for larger matrices.
Here’s how it’s done:
M = rand(10); % Create a random 10x10 matrix
imagesc(M); % Plot the matrix using imagesc
colorbar; % Add a colorbar for reference
In this example, the `rand(10)` function generates a 10x10 matrix filled with random values between 0 and 1. The `colorbar` function adds a reference bar indicating the scale of the colors in the matrix.
Using `surf()` for 3D Representations
For a more dynamic representation, you can use `surf()` to create a three-dimensional surface plot. This technique adds depth to your analysis, especially suitable for functions of two variables.
- Example Code:
[X, Y] = meshgrid(-5:1:5, -5:1:5); % Generating a grid of points
Z = X.^2 + Y.^2; % Defining the Z matrix
surf(X, Y, Z); % Create a 3D surface plot
In this case, `meshgrid` creates a rectangular grid out of the X and Y input vectors, while `surf` renders the surface plot, allowing visual exploration of how Z varies with respect to X and Y.
Heatmaps and Contour Plots
Creating Heatmaps with `heatmap()`: Heatmaps are excellent for illustrating the intensity of data at different values.
- Example Code:
data = rand(10); % Generating random data
heatmap(data); % Creating heatmap
Heatmaps provide an immediate visual cue about data concentration, which can be helpful in identifying trends quickly.
Utilizing `contour()` for Contour Plots: Contour plots depict the contours of a matrix based on its values, allowing for an intuitive interpretation of 3D surfaces on a 2D plane.
- Example Code:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2)); % Sin function for Z values
contour(X, Y, Z); % Creating the contour plot
Here, the contour lines represent distinct levels of Z values, making it easier to identify areas of change and continuity.
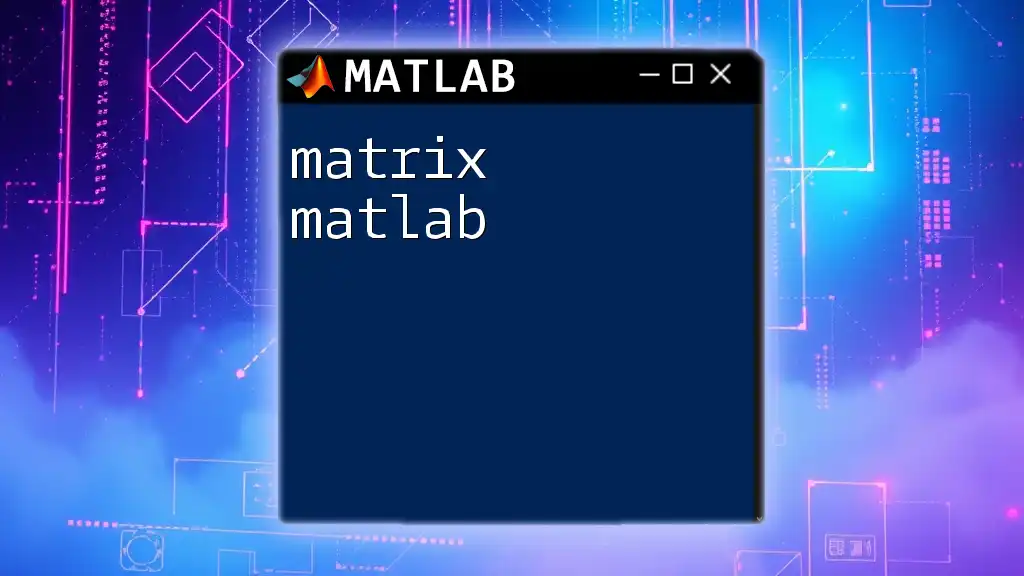
Customizing Your Plots
Enhancements to Visual Appeal
To make your plots more informative and visually appealing, consider adding:
- Titles: Clearly state what the plot represents.
- Labels: Provide concise information for each axis.
- Legends: Explain any color coding or symbols used within the plot.
Here’s how to enhance your plot:
title('3D Surface Plot Example');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
Adding Annotations and Customizing Axes
MATLAB provides options for adding annotations to your plots, which are instrumental for clarifying specific data points or trends.
Customize the tick marks using:
set(gca, 'XTick', -5:1:5); % Adjusting X-axis ticks
set(gca, 'YTick', -5:1:5); % Adjusting Y-axis ticks
grid on; % Enable grid for better readability
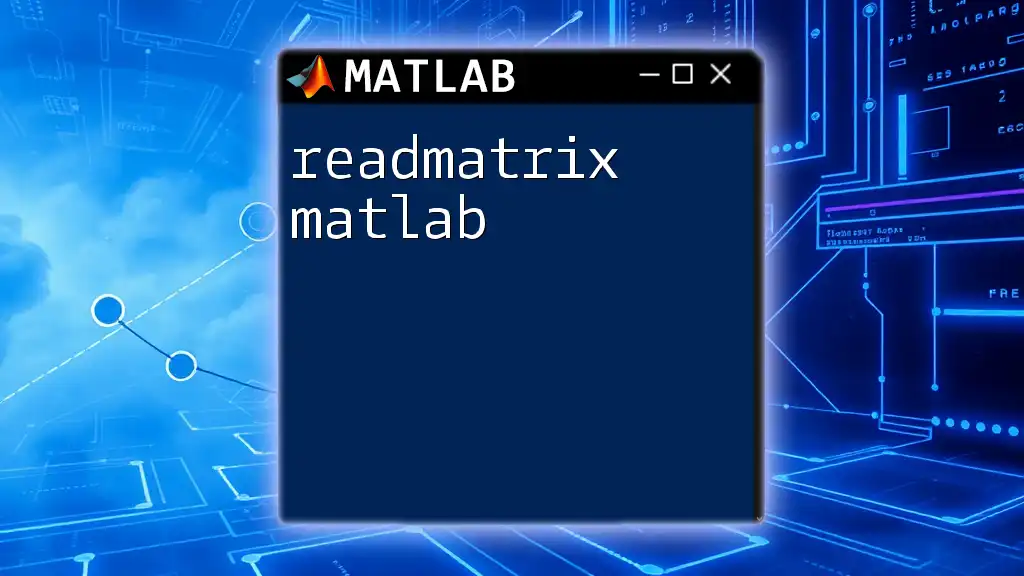
Advanced Techniques
Subplotting Matrices
Using subplots allows comparison between different matrices or different visualizations of the same matrix simultaneously. This is beneficial for cross-referencing or analyzing variations.
- Example Code:
subplot(1,2,1); % Divide the figure into 1 row and 2 columns
imagesc(matrix_2D); title('Matrix Visualization');
subplot(1,2,2);
imagesc(rand(10)); title('Random Matrix');
Here, the `subplot` function enables two plots to be displayed side by side for immediate comparison.
Using `plotmatrix()`
The `plotmatrix()` function creates a scatter plot matrix from the data, showing relationships between multiple variables effectively.
- Example Code:
data = rand(100, 3); % Generating random data for plotting
plotmatrix(data); % Generating a matrix plot
Through `plotmatrix`, you can observe the correlations among several dimensions simultaneously, providing a holistic view of your data.
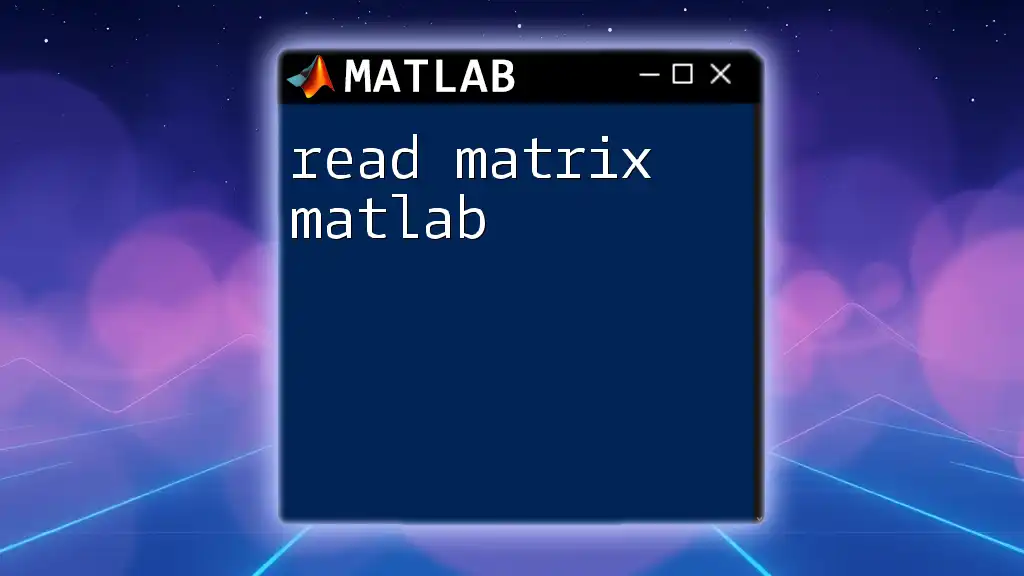
Conclusion
Mastering the techniques for plotting matrices in MATLAB is an essential skill that enhances data interpretation and presentation. From basic visualizations using `imagesc()` to advanced 3D plots with `surf()`, leveraging these tools ultimately aids in gaining deeper insights from your data. Engage with these examples and practice them in your MATLAB environment for a clearer understanding of how to effectively visualize matrices.
Feel free to explore additional resources, including MATLAB's official documentation and various online courses, to expand your knowledge further. Experimentation is key in this journey, and your skills will flourish through continuous practice and application.