An identity matrix in MATLAB is a square matrix with ones on the main diagonal and zeros elsewhere, which can be created using the `eye` function.
I = eye(3); % Creates a 3x3 identity matrix
What is an Identity Matrix?
Definition
An identity matrix is a fundamental concept in linear algebra, characterized as a square matrix in which all the elements of the principal diagonal are 1 and all other elements are 0. In simpler terms, it acts somewhat like the number 1 in regular multiplication: any matrix multiplied by an identity matrix remains unchanged.
Mathematical Representation
The identity matrix is typically denoted as \(I_n\), indicating a square matrix of size \(n \times n\). For example, a 2x2 identity matrix \(I_2\) appears as follows:
I_2 = [1 0;
0 1]
This matrix is crucial in various mathematical computations, especially in the context of matrix operations.
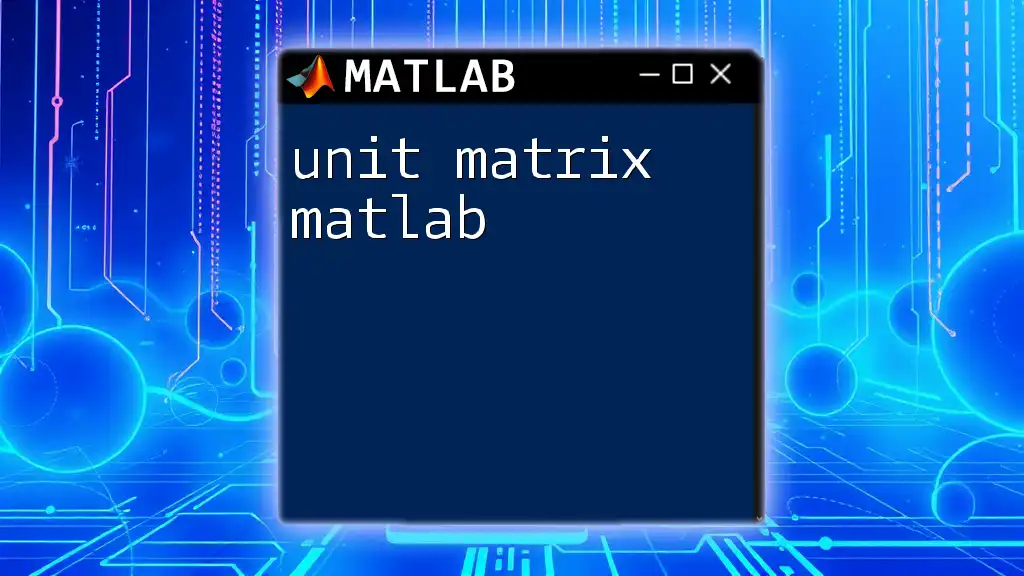
Creating an Identity Matrix in MATLAB
Using the `eye` Function
MATLAB provides a built-in function, `eye`, specifically designed to create identity matrices efficiently.
The syntax of the `eye` function is as follows:
I = eye(n) % creates an n x n identity matrix
Code Example
To create a 3x3 identity matrix in MATLAB, you can execute the following command:
I3 = eye(3)
When you run this code, MATLAB will output:
I3 =
1 0 0
0 1 0
0 0 1
This output confirms that I3 is indeed a 3x3 identity matrix, where all diagonal elements are 1 and all off-diagonal elements are 0.
Creating Non-Square Identity Matrices
Interestingly, MATLAB’s `eye` function is not limited to square matrices. You can create a non-square identity matrix by specifying both rows and columns.
For example, to create a 2x3 identity matrix, use the following code:
I2_3 = eye(2, 3)
This will generate:
I2_3 =
1 0 0
0 1 0
In this case, the resulting matrix has 2 rows and 3 columns, reflecting the specific dimensions you provided.
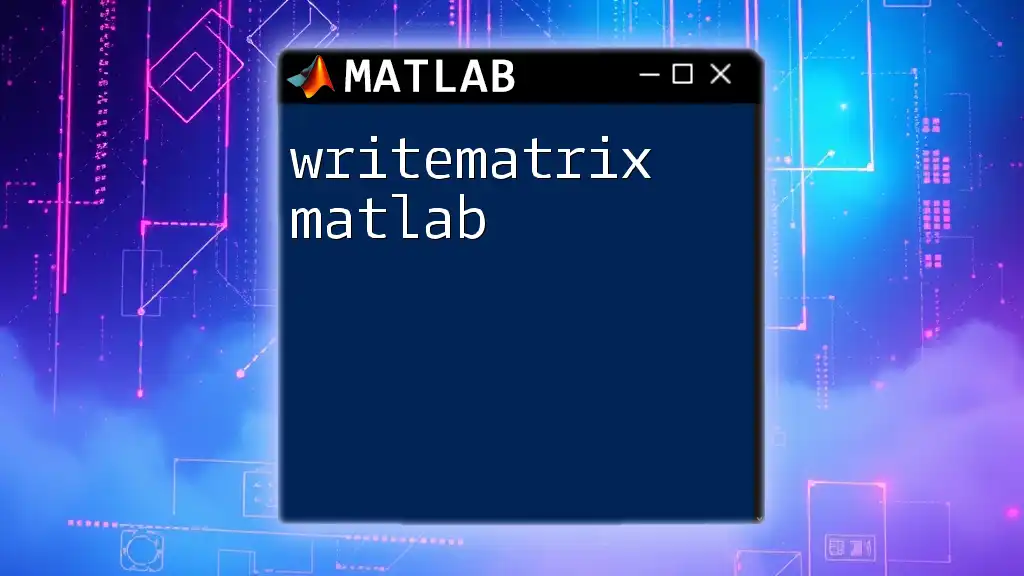
Applications of Identity Matrices in MATLAB
Algebraic Operations
Identity matrices are predominantly useful in matrix multiplication. When you multiply any matrix with an identity matrix of compatible dimensions, the original matrix remains unchanged. This property is foundational in a variety of mathematical operations.
Here’s a simple example using a 2x2 matrix:
A = [2 3; 4 5];
result = A * eye(2)
The output will be:
result =
2 3
4 5
As observed, the matrix A remains the same upon multiplication with the identity matrix.
Inverting Matrices
Another important application of identity matrices is in the context of matrix inversion. The product of a matrix and its inverse results in the identity matrix. This relationship is expressed mathematically as \(AA^{-1} = I\).
Here’s a demonstration of this concept:
A = [1 2; 3 4];
A_inv = inv(A);
check_identity = A * A_inv
The output will be:
check_identity =
1.0000 0
0 1.0000
The result indicates that the product of A and its inverse indeed yields the 2x2 identity matrix.
Solving Systems of Equations
Identity matrices also play a significant role in solving systems of linear equations. When applied in the context of an equation represented as \(Ax = b\), where \(A\) is a matrix and \(b\) is a vector, the identity matrix helps streamline the solution process.
Consider the following example where we solve the equation:
% Define a system of equations Ax = b
A = [2 1; 5 7];
b = [11; 43];
x = A \ b; % Using backslash operator to solve
In this snippet, the backslash operator efficiently handles the matrix inversion and multiplication under the hood. The result, vector x, will be the solution to the system of equations highlighted.
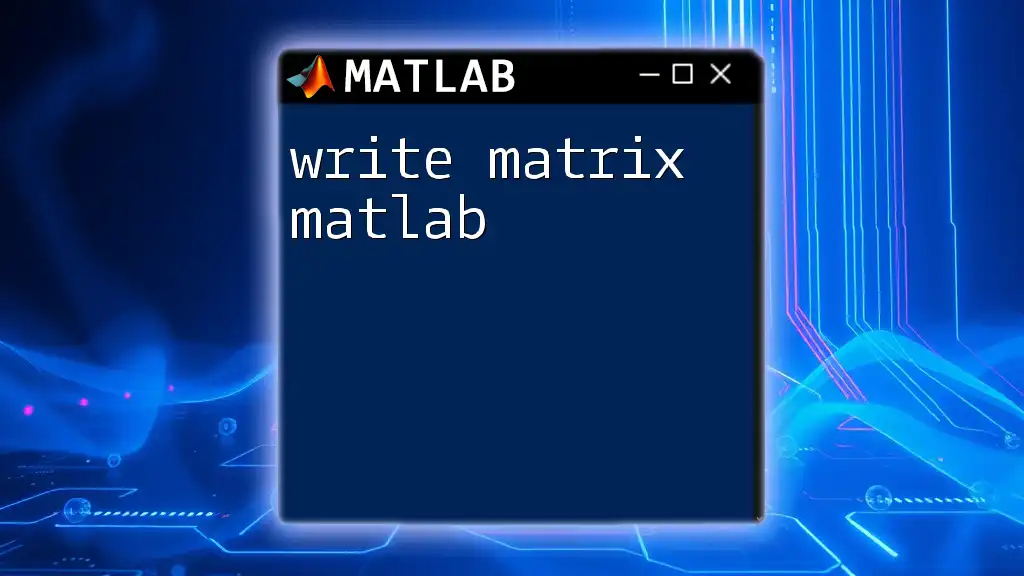
Common Mistakes and Troubleshooting
Misunderstandings about Identity Matrices
Many beginners confuse identity matrices with zero matrices. It's crucial to understand that an identity matrix contains 1s along the diagonal, while a zero matrix contains only 0s. Keeping a clear distinction between these two types of matrices is essential.
Debugging Tips in MATLAB
While using the `eye` function, beginners may encounter common errors related to matrix dimensions or incorrectly interpreting the outputs. Here are some strategies to avoid errors:
- Always check the dimensions of matrices involved in operations to ensure compatibility.
- Use `size(A)` to verify the dimensions when you are unsure.
- Familiarize yourself with MATLAB’s built-in functions documentation for deeper understanding.
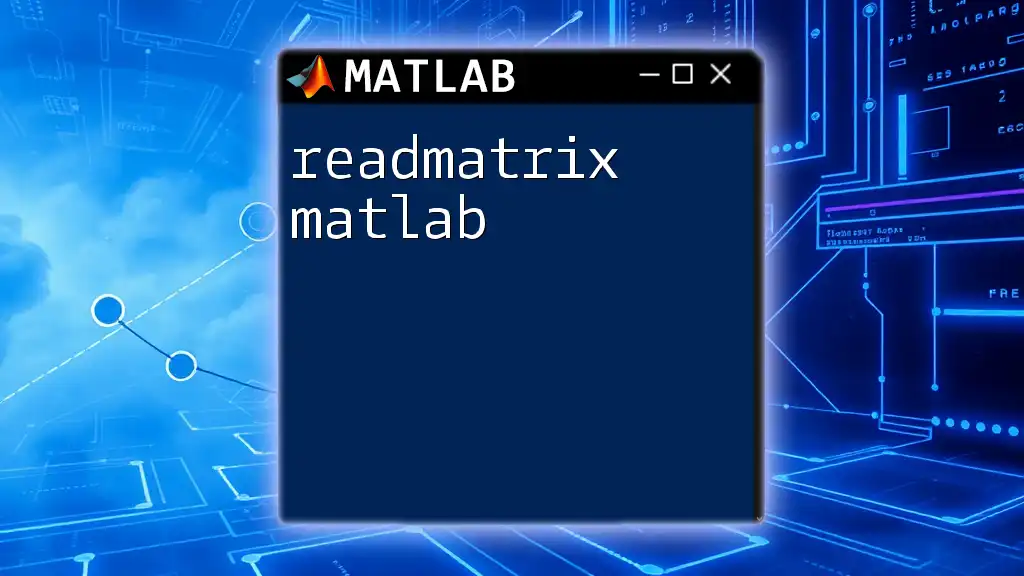
Conclusion
The identity matrix is a powerful tool in MATLAB that enables a multitude of mathematical operations. From creating and manipulating matrices to solving complex equations, understanding how to use the identity matrix will greatly enhance your MATLAB skills. Explore the `eye` function further in your projects, and leverage the unique properties of identity matrices to solve various mathematical problems.
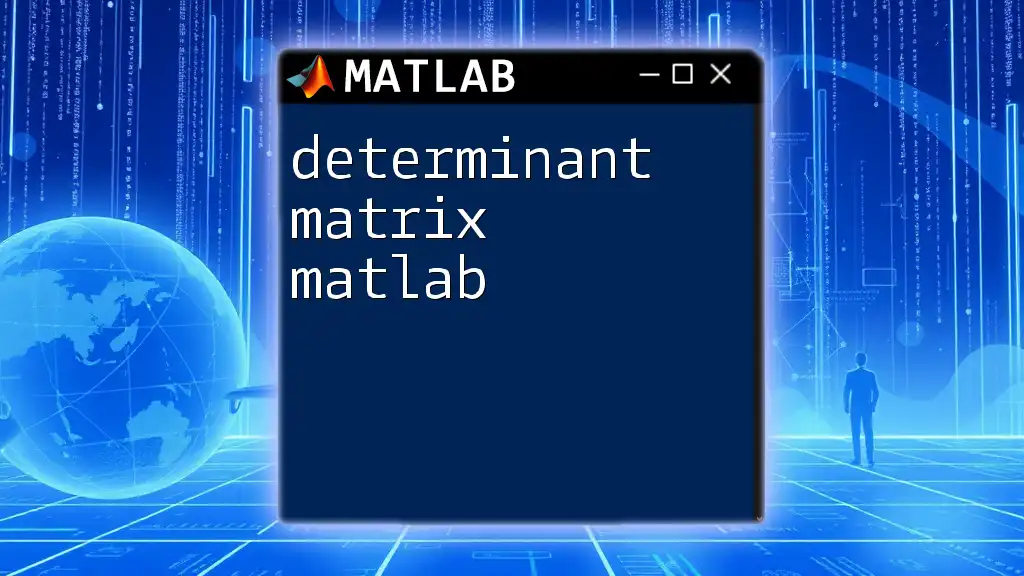
Additional Resources
For deeper learning, consider visiting the official MATLAB documentation on matrix operations. Engage in practical exercises using the identity matrix to reinforce your understanding and boost your confidence.
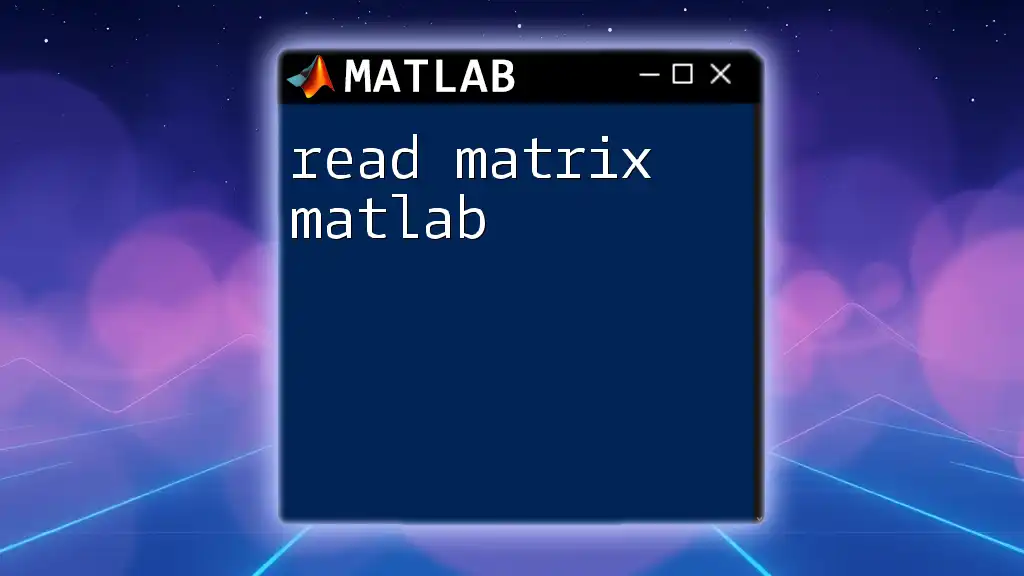
FAQs
What is the size of an identity matrix?
An identity matrix is characterized by its square shape but can vary in size, such as 1x1, 2x2, or n x n, depending on your needs.
Can identity matrices be used in machine learning?
Yes, identity matrices have applications in numerous algorithms and optimizations in the field of machine learning, especially when dealing with matrix computations and transformations.
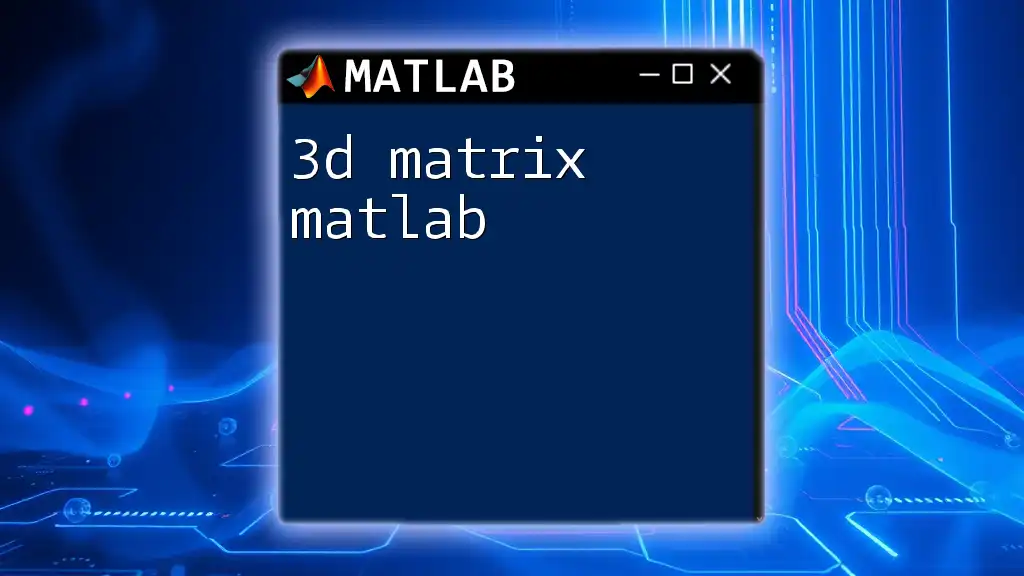
Call to Action
Now that you've explored the concept of the identity matrix in MATLAB, take the next step. Practice creating identity matrices and applying them in calculations to cement your understanding. Happy coding!