In MATLAB, the inverse of a matrix can be calculated using the `inv()` function, which takes a square matrix as input and returns its inverse if it exists.
A = [1, 2; 3, 4]; % Define a 2x2 matrix
A_inv = inv(A); % Calculate the inverse of matrix A
Understanding the Inverse of a Matrix
Definition of Matrix Inverse
The inverse of a matrix \(A\) is another matrix, denoted as \(A^{-1}\), which when multiplied by \(A\) yields the identity matrix \(I\): \[ A \cdot A^{-1} = I \]
For a matrix to be invertible, it must be square (having the same number of rows and columns) and its determinant must not be zero. This ensures that the matrix is non-singular, meaning that unique solutions exist for linear systems it represents.
Geometric Interpretation
In geometric terms, the inverse of a matrix can be seen as a transformation that reverses the effect of the original matrix. For instance, while one matrix might rotate and scale a vector, its inverse would undo these operations, returning the vector to its original orientation and magnitude.
Types of Matrices
Square Matrices vs. Non-square Matrices
Only square matrices can have an inverse. Non-square matrices lack the necessary properties to possess an inverse, as they do not maintain a consistent relationship between dimensions.
Singular vs. Non-singular Matrices
A singular matrix is one that does not have an inverse because its determinant is zero. Such matrices often represent linear transformations that collapse dimensions, leading to an infinite number of solutions or no solutions at all.
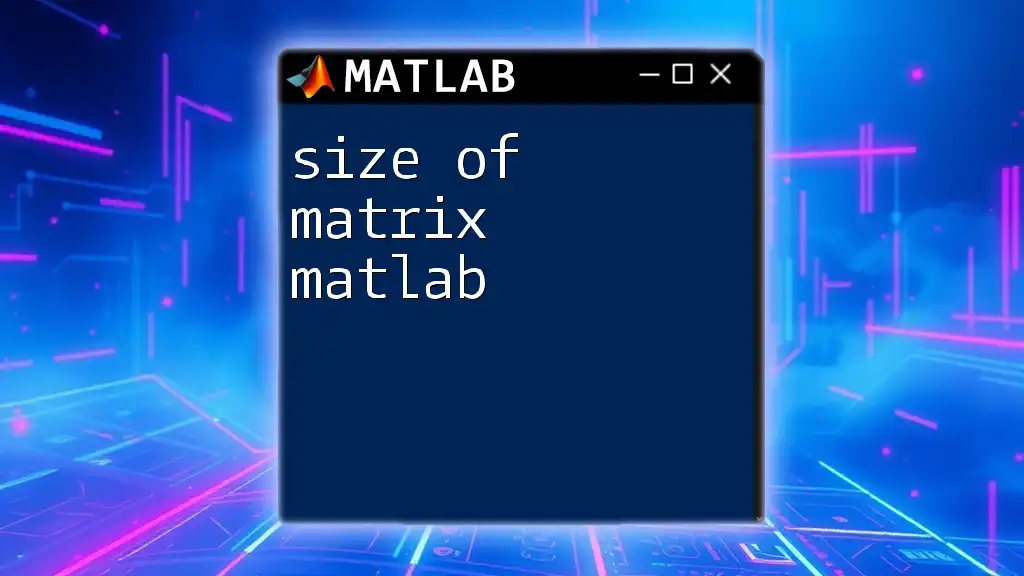
How to Calculate the Inverse of a Matrix in MATLAB
MATLAB Command for Inverse Calculation
In MATLAB, the most straightforward way to calculate the inverse of a matrix is by using the `inv` function. The basic syntax is as follows:
A = [1, 2; 3, 4]; % Example matrix
A_inv = inv(A); % Calculating the inverse
This command will return the inverse of matrix \(A\), provided that \(A\) is invertible.
Conditions for Inversion
Before attempting to find the inverse, it’s essential to check if the matrix is invertible. This is typically done using the `det` function, which calculates the determinant of the matrix. If the determinant is zero, the matrix is singular and cannot be inverted.
Here’s a code snippet demonstrating this validation:
if det(A) == 0
error('Matrix is singular and cannot be inverted');
end
This conditional check ensures that you avoid errors during the inversion process.
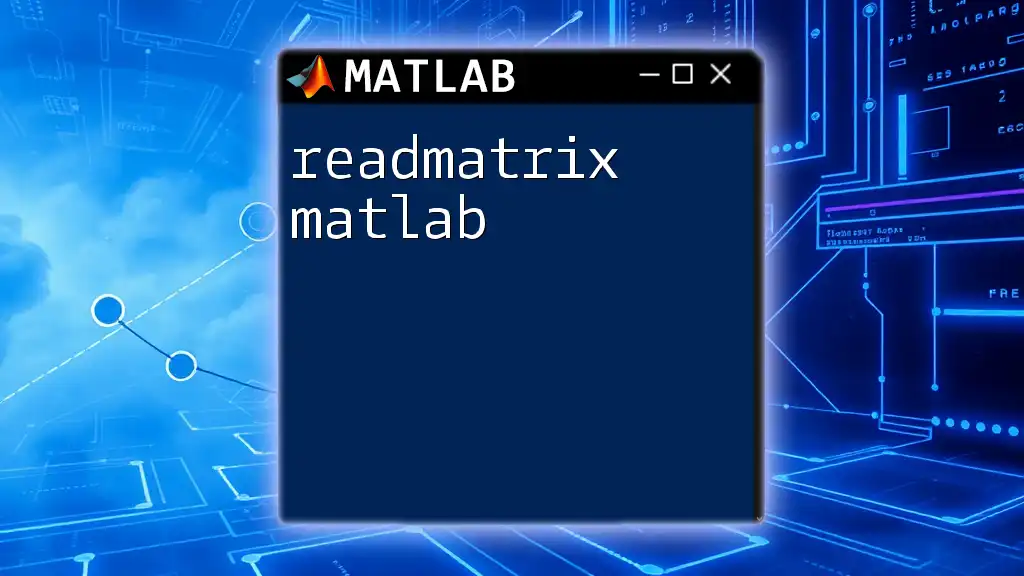
Advanced Techniques for Inverting Matrices
Alternative Methods to Calculate Inverses
Besides the `inv` function, MATLAB offers the backslash operator (`\`) as a highly efficient method for solving systems of equations. This method is preferred because it is numerically stable and computationally efficient. The syntax looks like this:
B = [5; 6]; % Example constant matrix
A_inv_2 = A \ B; % Solving Ax = B
In this context, using `A \ B` allows you to find \(x\) in the equation \(Ax = B\) without explicitly calculating \(A^{-1}\).
Symbolic Inversion with the Symbolic Toolbox
For matrices containing symbolic variables, the Symbolic Toolbox provides a way to compute their inverses symbolically. Here’s how you can use it with the `syms` function:
syms x y;
M = [x, y; y, x];
M_inv = inv(M);
This approach is particularly useful in theoretical applications where you need to analyze the properties of the inverse matrix rather than numerical results.
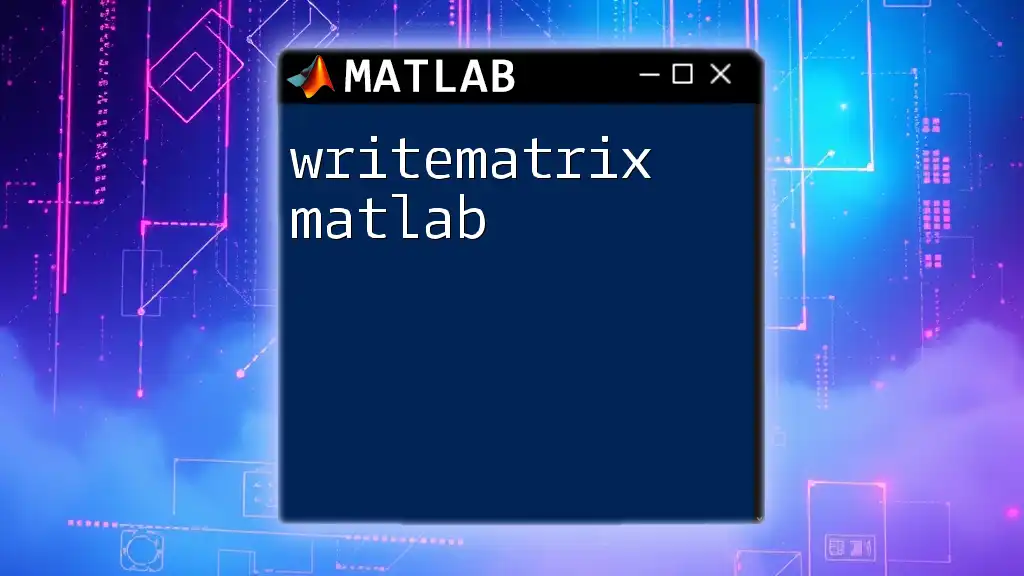
Common Pitfalls and Troubleshooting
Common Errors When Calculating Matrix Inverses
When working with matrix inverses, you may encounter common pitfalls:
-
Singular Matrix Error: Attempting to invert a singular matrix will lead to an error. Always perform a determinant check before inversion.
-
Dimension Mismatch: Ensure the matrix is square before trying to compute an inverse. Non-square matrices cannot be inverted.
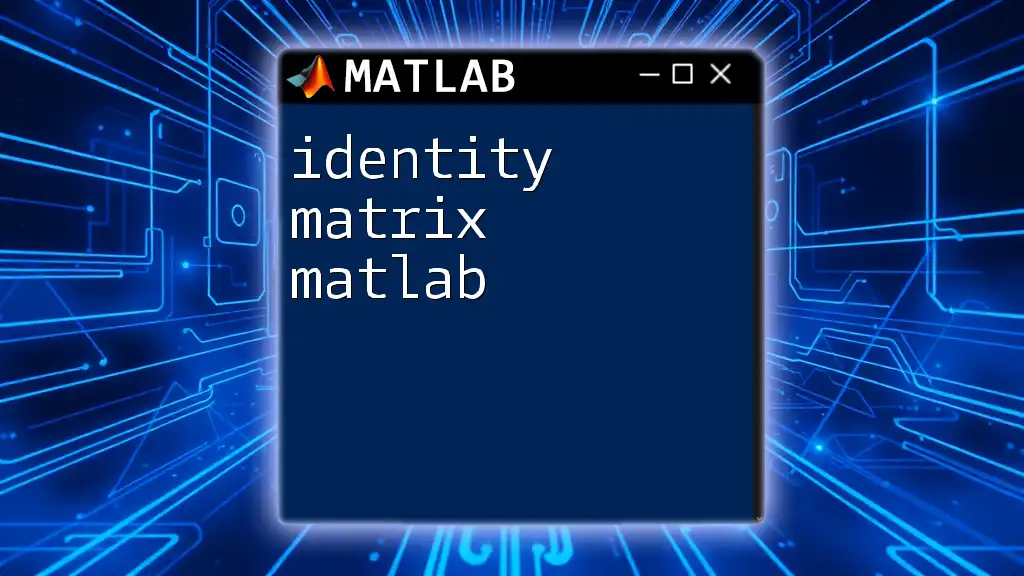
Practical Applications of Matrix Inversion
Systems of Linear Equations
One of the primary applications of the inverse of a matrix is solving systems of linear equations represented in the form \(Ax = b\). You can derive the solution by multiplying both sides of the equation by the inverse of \(A\): \[ x = A^{-1}b \]
In MATLAB, this is implemented very easily:
A = [2, 3; 5, 4];
b = [8; 16];
x = inv(A) * b; % Finding solutions
This results in the vector \(x\), which contains the solutions to the system.
Applications in Data Processing and Statistics
Matrix inversion is also crucial in statistics, particularly for calculating the covariance matrix of a dataset. The covariance matrix summarizes the pairwise covariances between features. In applications, you may need to invert this matrix to perform various analyses, such as multivariate regression.
To calculate the covariance matrix and its inverse in MATLAB, you can use:
data = rand(100, 2); % Example dataset
C = cov(data); % Covariance matrix
C_inv = inv(C); % Inverse of the covariance matrix
Understanding how to find the inverse of a matrix in MATLAB empowers you to tackle a variety of mathematical and engineering challenges effectively.
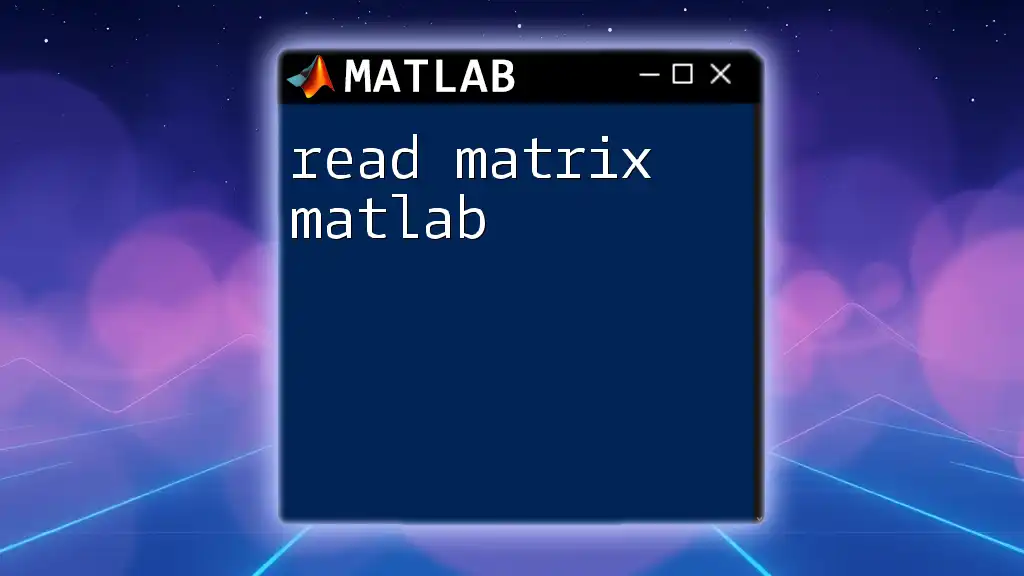
Conclusion
As we've explored, finding the inverse of a matrix in MATLAB is a fundamental operation that enables solutions to systems of equations and numerous applications in engineering and data analysis. By understanding different methods, as well as the significance of invertibility, you can leverage MATLAB’s capabilities to enhance your computational proficiency.
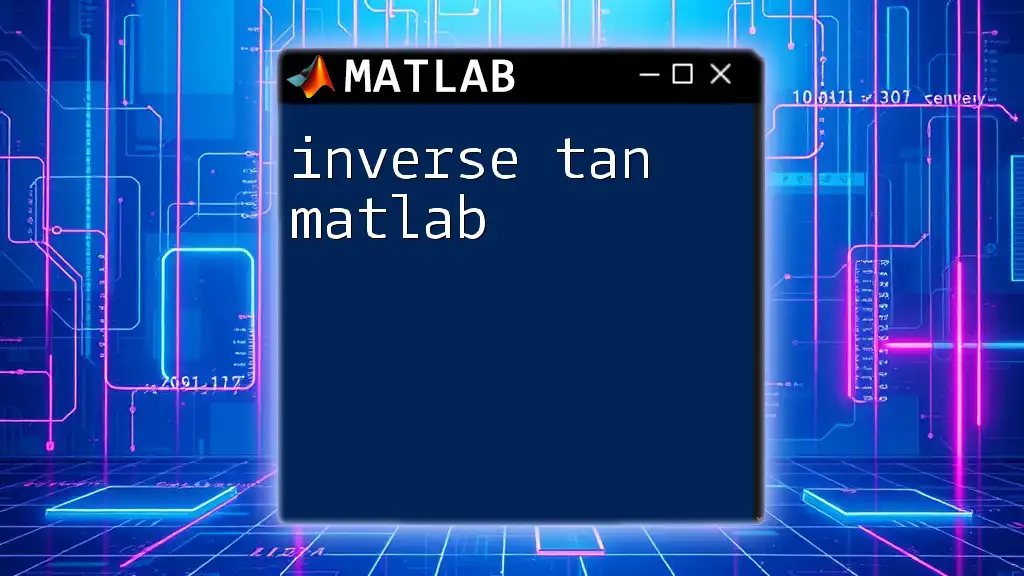
Further Reading and Resources
For a deeper understanding, consider exploring additional resources:
- Books and Tutorials: Look for texts covering linear algebra and MATLAB applications.
- MATLAB Documentation: Familiarize yourself with official documentation for advanced matrix functions and best practices.