A 3D matrix in MATLAB is a multidimensional array that allows you to store data in three dimensions, which can be manipulated efficiently using built-in functions.
% Create a 3D matrix of size 3x3x3 filled with random values
A = rand(3, 3, 3);
Understanding the Structure of 3D Matrices
In MATLAB, a 3D matrix can be thought of as an extension of a 2D matrix, where data is organized not only in rows and columns but also in layers. Each layer represents a "slice" of the matrix, much like how a stack of sheets can form a document.
When we talk about dimensions in MATLAB, it’s important to define:
- Scalar: A single number, represented as `A = 5`.
- Vector: An array of numbers, either a row or column, such as `B = [1, 2, 3]` or `C = [4; 5; 6]`.
- Matrix: A 2D array of numbers, for example, `D = [1, 2; 3, 4]`.
- 3D Matrix: A 3D array of numbers, which can be represented as `E = rand(4, 4, 3)`; this creates a random matrix with dimensions 4x4x3.
Understanding how these dimensions interact will aid you in manipulating and applying operations on 3D matrices.
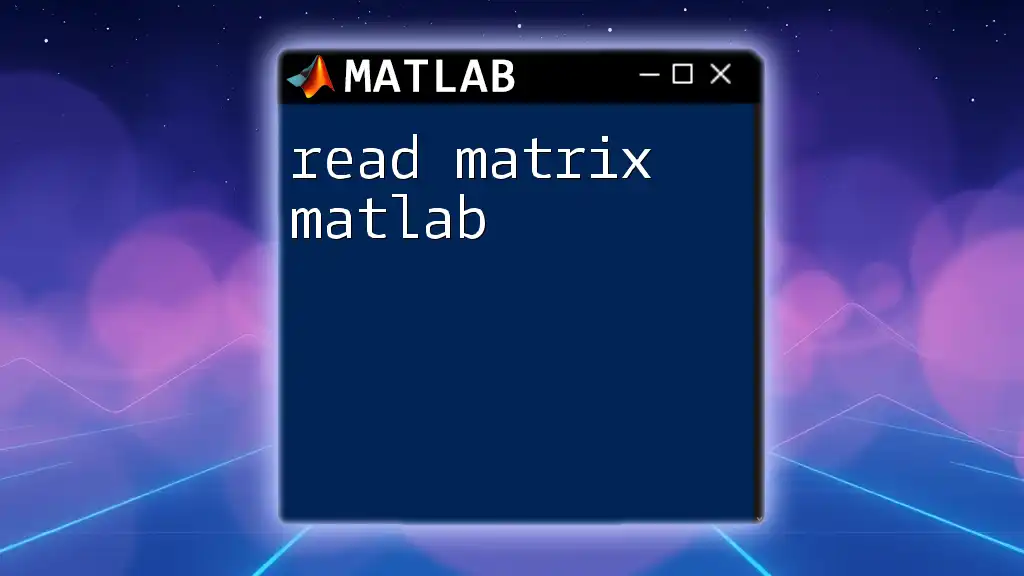
Creating 3D Matrices in MATLAB
Creating a 3D matrix in MATLAB can be achieved through several methods, including built-in functions, which provide a quick way to initialize matrices with desired properties.
Using Built-in Functions
MATLAB provides several built-in functions for creating 3D matrices:
- `rand`: Generates a matrix with random values.
- `zeros`: Creates a matrix filled with zeros.
- `ones`: Initializes a matrix filled with ones.
Here’s how you can create a few examples:
A = rand(4, 4, 3); % Creates a 4x4x3 matrix with random values
B = zeros(4, 4, 2); % Creates a 4x4x2 matrix filled with zeros
C = ones(5, 5, 1); % Creates a 5x5x1 matrix filled with ones
Manual Initialization
You can also manually define a 3D matrix in MATLAB. The `cat` function is very useful for concatenating matrices along a specified dimension. For example:
D = cat(3, [1, 2; 3, 4], [5, 6; 7, 8]); % Concatenating 2 matrices to form a 3D matrix
In this code, `D` becomes a 3D matrix made up of two layers, each containing a 2x2 matrix.
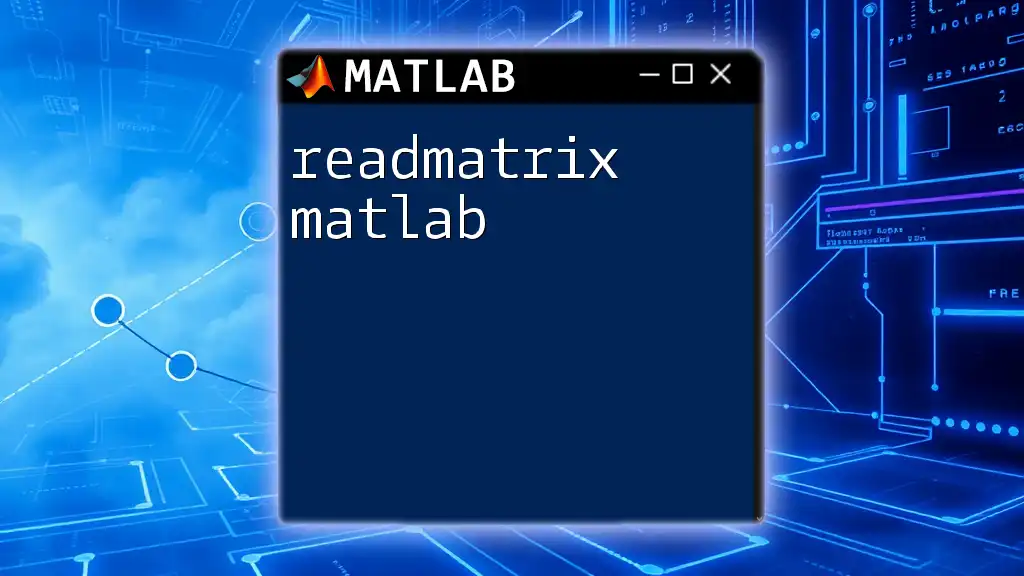
Accessing and Manipulating 3D Matrices
Indexing Elements
Accessing specific elements within a 3D matrix in MATLAB is straightforward. You can refer to an element by specifying its coordinates using the syntax `A(row, column, layer)`. For example, if you want to access a specific element:
value = A(2, 3, 1); % Accessing the element at (2,3,1)
You can also obtain an entire slice of the matrix. Slicing allows you to extract a layer of the matrix easily. For instance:
slice = A(:, :, 2); % Accessing the second layer of the 3D matrix
Modifying Elements
Once you have accessed the elements, you can modify them as well. This simple method allows you to update values directly:
A(1, 1, 1) = 10; % Changing the value of the first element to 10
Reshaping 3D Matrices
Reshaping provides flexibility in re-dimensioning your matrices. The `reshape` function allows you to adjust the size of a matrix without changing its data. Here is an example:
E = reshape(A, [2, 8]); % Reshaping the 3D matrix into a 2D matrix
This command alters the structure of matrix `A` into a 2D matrix with 2 rows and 8 columns.
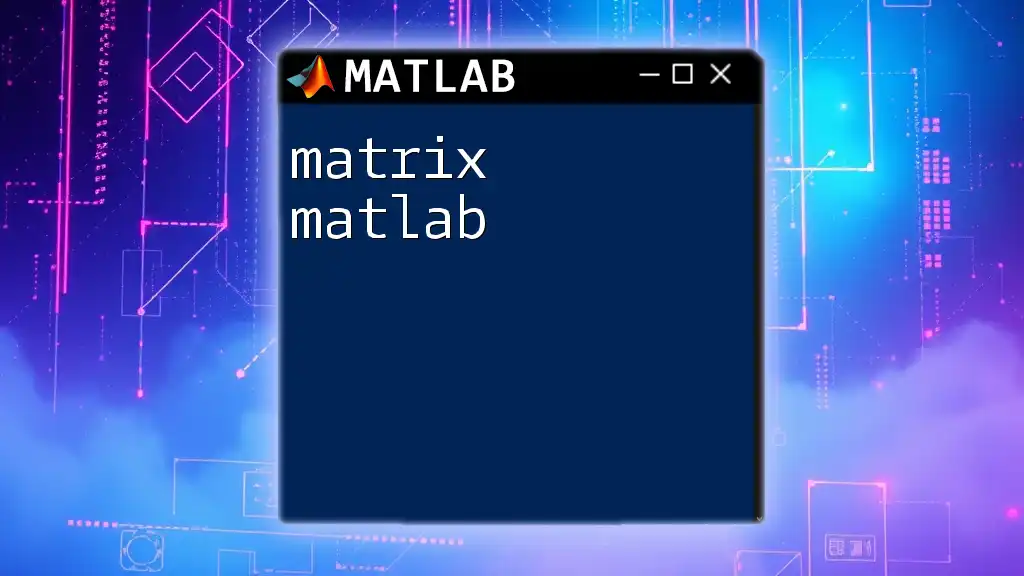
Performing Operations on 3D Matrices
Mathematical Operations
MATLAB allows you to perform a variety of mathematical operations on 3D matrices. Element-wise operations can be conducted using the usual arithmetic operators. Here’s how you can add two matrices:
F = A + B; % Element-wise addition of two 3D matrices
Similarly, for element-wise multiplication:
G = A .* B; % Element-wise multiplication of two 3D matrices
Statistical Functions
MATLAB provides built-in functions to analyze data effectively within 3D matrices. For instance, you can compute the mean and sum across a specified dimension:
meanVal = mean(A, 3); % Compute mean across the 3rd dimension
sumVal = sum(A, 3); % Compute sum across the 3rd dimension
These functions eliminate the need to manually loop through data, making your code cleaner and more efficient.
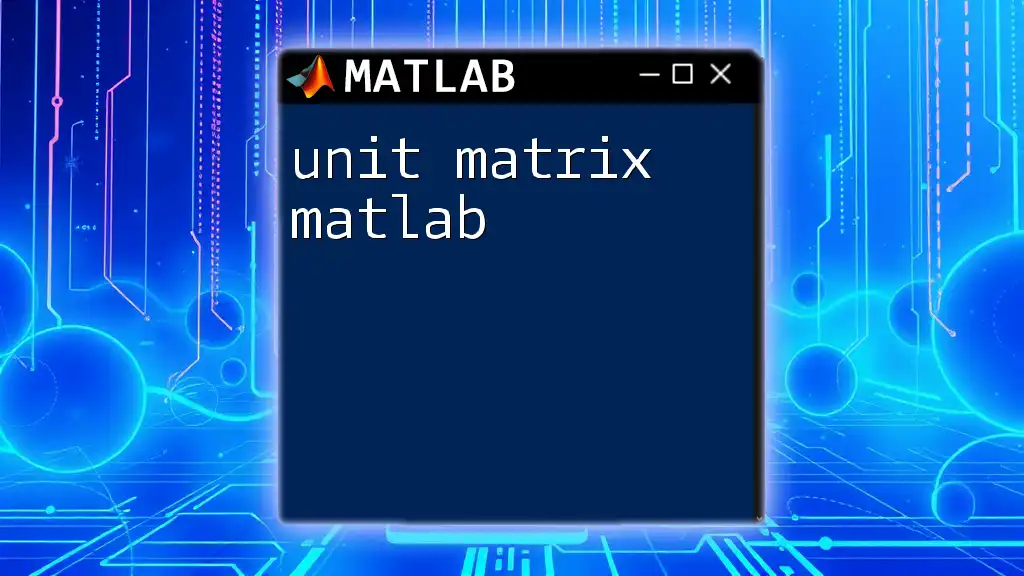
Visualizing 3D Matrices
Visualizing 3D matrices is crucial for better understanding your data. MATLAB offers multiple functions like `slice`, `surf`, and `mesh` to visualize 3D datasets effectively. Here’s a brief example of using the `slice` function:
x = 1:4; y = 1:4; z = 1:3;
[X, Y, Z] = meshgrid(x, y, z);
V = rand(4, 4, 3);
slice(X, Y, Z, V, [], [], 1:3);
In this example, `slice` allows you to visualize the layers in the 3D matrix `V`. Adjusting the ranges will help in focusing on specific slices of interest.
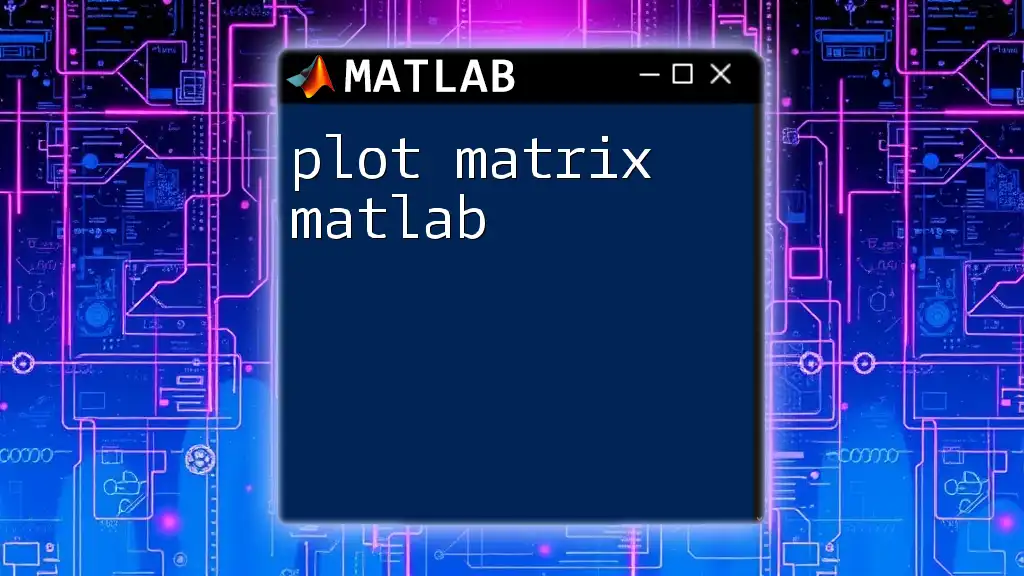
Examples and Use Cases
Practical Applications of 3D Matrices
3D matrices find extensive applications across various fields:
- Image Processing: Each layer of a 3D matrix can represent color channels in image data. For example, a colored image might have three layers corresponding to the RGB components.
- Scientific Computing: Simulations of physical systems like fluid dynamics or weather modeling often utilize 3D matrices to represent multidimensional datasets.
- Simulations: In engineering, simulations of mechanical structures and material properties can be modeled using 3D matrices.
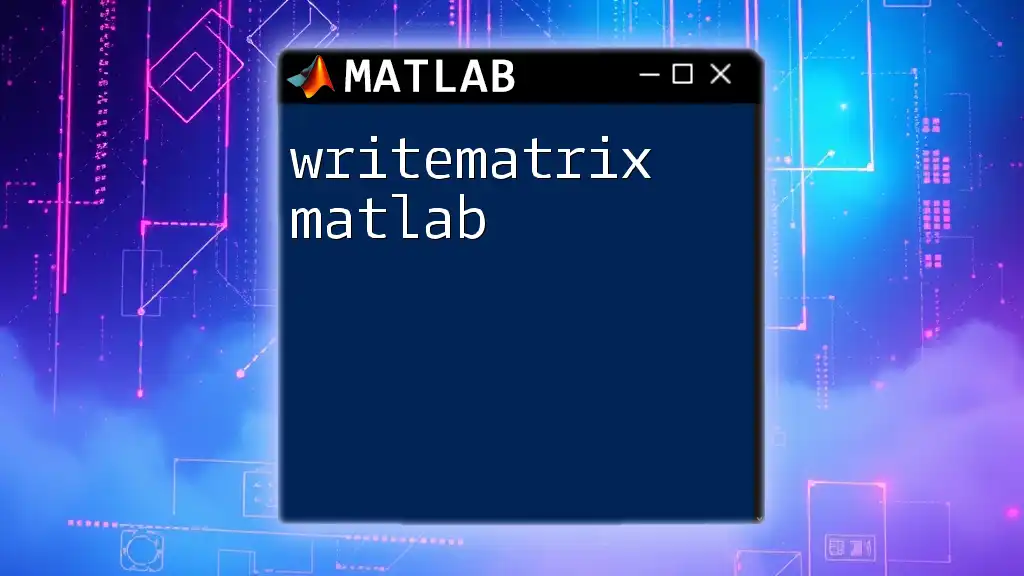
Tips and Best Practices
When working with 3D matrices in MATLAB, here are some tips to enhance the usability and performance:
- Aim to vectorize your code when possible, avoiding loops for better performance.
- Preallocate memory for large matrices to avoid dynamic resizing during execution.
- Be cautious with dimensions to avoid unintended errors when slicing or reshaping.
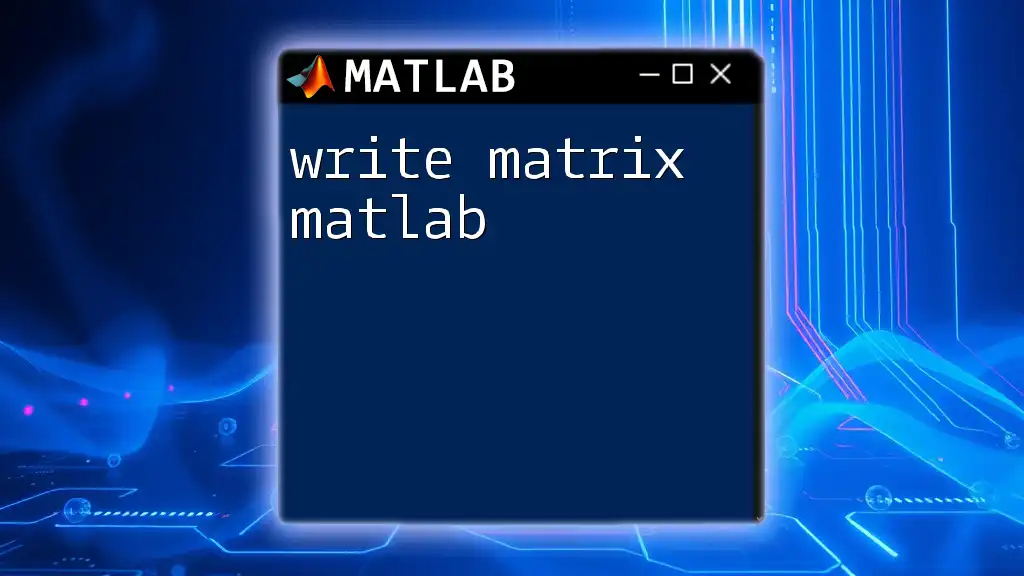
Conclusion
Understanding 3D matrices in MATLAB is essential for data manipulation and analysis. With proper knowledge and practical implementations of 3D matrices, you can unlock the potential of MATLAB for solving complex problems and visualizing datasets effectively. As you continue to explore MATLAB's capabilities, remember to experiment with various functions and techniques to enhance your skills. Embrace the journey and keep refining your MATLAB command knowledge for better outcomes.
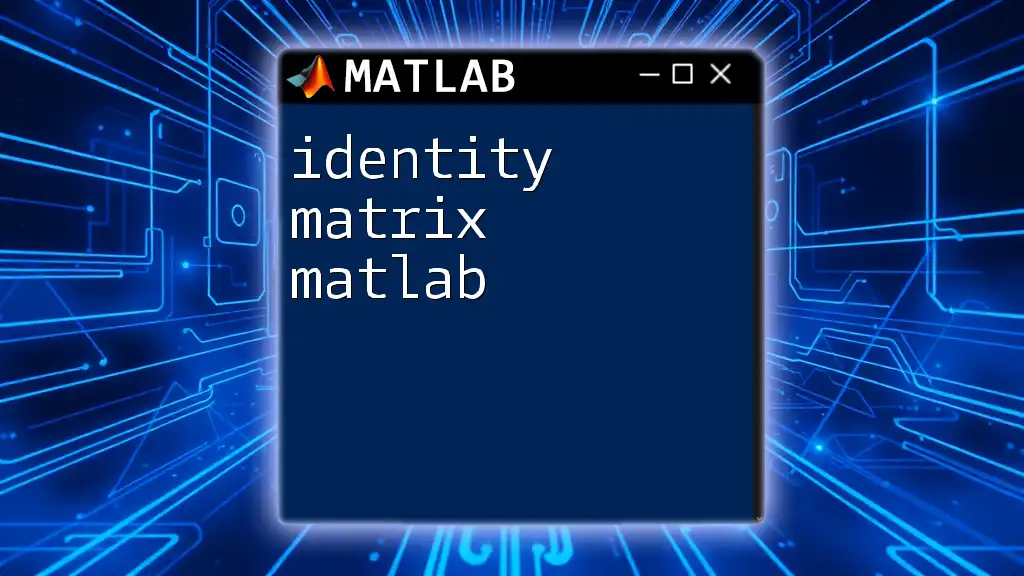
Additional Resources
For further exploration, consider referring to the MATLAB documentation on matrix operations, as it offers comprehensive details. You may also explore suggested readings or training courses to deepen your understanding of using 3D matrices effectively in various applications. If you're interested in personalized training, feel free to reach out!