A unit matrix, also known as an identity matrix, in MATLAB is created using the `eye` command, which generates a square matrix with ones on the main diagonal and zeros elsewhere.
A = eye(3); % Creates a 3x3 unit (identity) matrix
What is a Unit Matrix?
Definition
A unit matrix, also known as an identity matrix, is a fundamental type of square matrix. It is characterized by having 1s on the main diagonal (from the top left to the bottom right) and 0s elsewhere. For instance, a 2x2 unit matrix is represented as:
[ 1 0 ]
[ 0 1 ]
Mathematical Representation
In mathematical notation, a unit matrix of size \( n \) is denoted as \( I_n \). It is important to note that a unit matrix is always square, meaning it has the same number of rows and columns. Examples of small unit matrices include:
-
2x2:
I_2 = [ 1 0 ] [ 0 1 ]
-
3x3:
I_3 = [ 1 0 0 ] [ 0 1 0 ] [ 0 0 1 ]
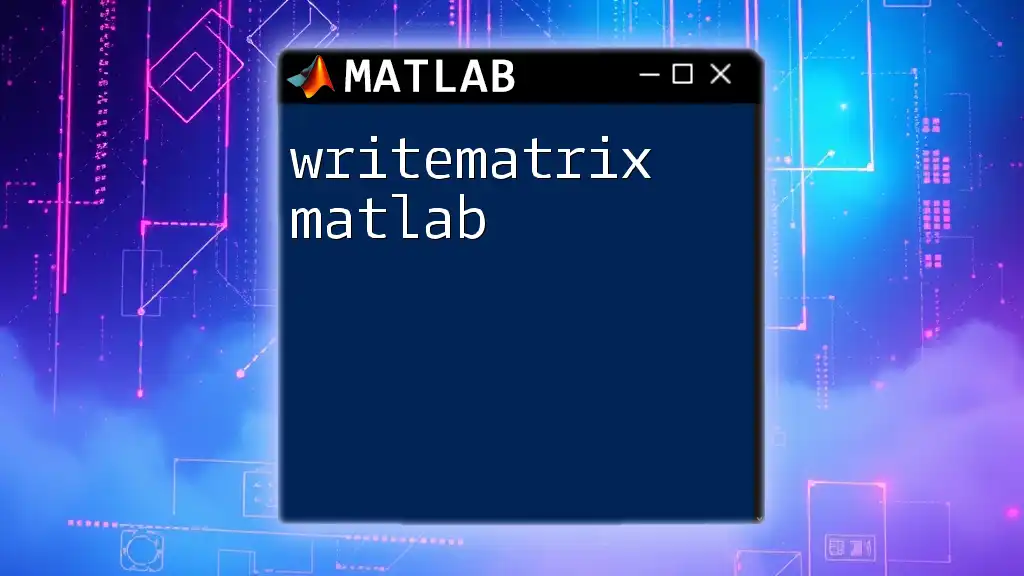
Creating a Unit Matrix in MATLAB
Using the `eye` Function
In MATLAB, the creation of a unit matrix is straightforward with the `eye` function. This function allows you to specify the size of the matrix easily. The syntax is:
I = eye(n)
Where \( n \) is the desired size of the unit matrix.
Example: To create a unit matrix of size 2x2:
I2 = eye(2); % creates a 2x2 unit matrix
For a 3x3 unit matrix:
I3 = eye(3); % creates a 3x3 unit matrix
Creating an Identity Matrix of Different Sizes
You can also create unit matrices of various dimensions. For instance, to create a 4x4 unit matrix, simply use:
I4 = eye(4);
If you want to create a rectangular unit matrix, such as 3x4, you can specify the number of rows and columns:
I_rect = eye(3, 4); % creates a 3x4 matrix with eye characteristics
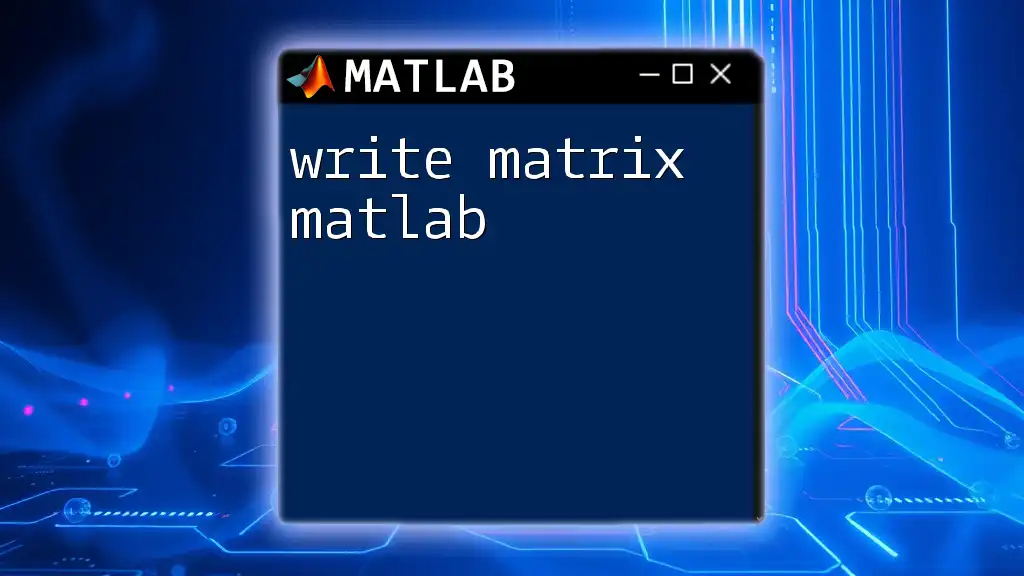
Properties of Unit Matrices
Multiplicative Identity
One of the primary features of a unit matrix is its function as the multiplicative identity in matrix multiplication. When any matrix \( A \) is multiplied by a unit matrix \( I \), the result is the original matrix \( A \).
Example:
A = [1 2; 3 4];
I = eye(2);
result = A * I; % result will be [1 2; 3 4]
Inverse
An interesting property of a unit matrix is that its inverse is the matrix itself. This means that if you take a unit matrix \( A \), and compute its inverse, you will get back \( A \).
Example:
A = eye(3);
inverse_A = inv(A); % returns A
Transpose
Another vital characteristic of a unit matrix is that it is symmetric. This means that the transpose of a unit matrix is equal to the matrix itself.
Example:
A = eye(3);
transpose_A = A'; % returns A
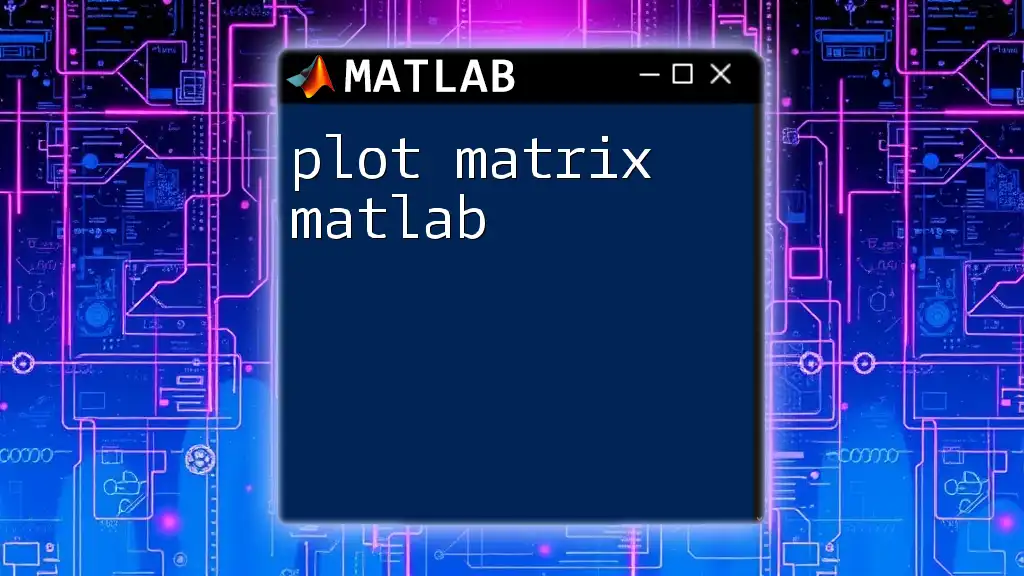
Applications of Unit Matrices in MATLAB
System of Equations
Unit matrices are heavily utilized in the solution of systems of linear equations. When solving a linear system represented as \( A \cdot x = b \), a unit matrix can be used in matrix operations to isolate variables and derive solutions.
Eigenvalue Problems
In linear algebra, unit matrices play an essential role in computing eigenvalues and eigenvectors. They help in transforming matrices into simpler forms to analyze linear transformations more straightforwardly.
Data Transformations
Unit matrices are also useful in data transformations, particularly in machine learning and statistical modeling. For example, in Principal Component Analysis (PCA), unit matrices are employed to maintain the relationships within datasets while reducing dimensions.
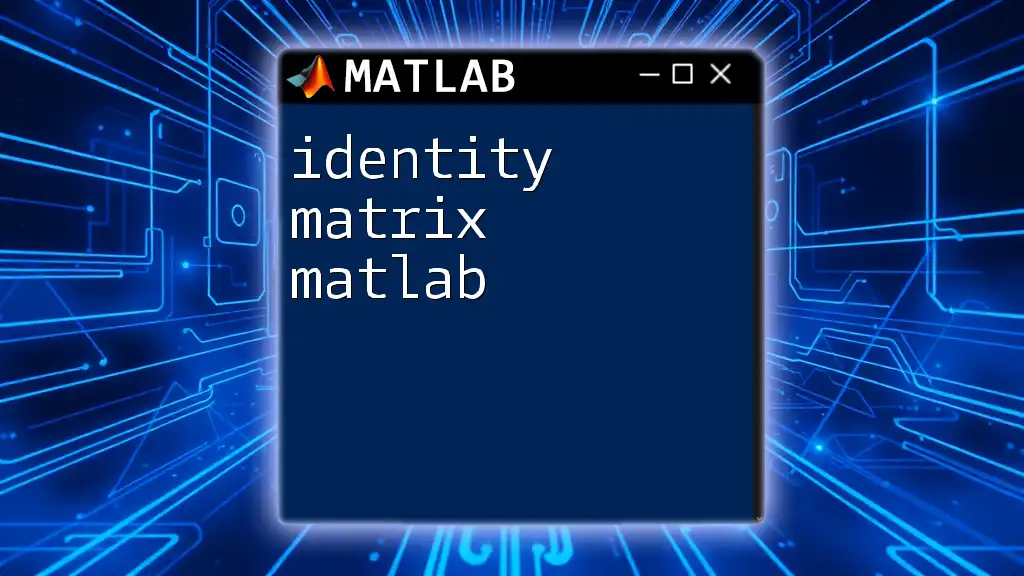
Advanced Usage of Unit Matrices
Custom Identity Matrices
You can create unit matrices with specific values other than 1 by scaling them. For example, you can multiply a unit matrix by a scalar to modify its entries.
Example:
I_custom = eye(3) * 5; % creates a unit matrix scaled by 5
Efficiency and Performance
Unit matrices provide computational efficiency in MATLAB, especially when used in algorithms involving matrix operations. They consume less memory and system resources compared to larger random matrices, leading to faster computation times.
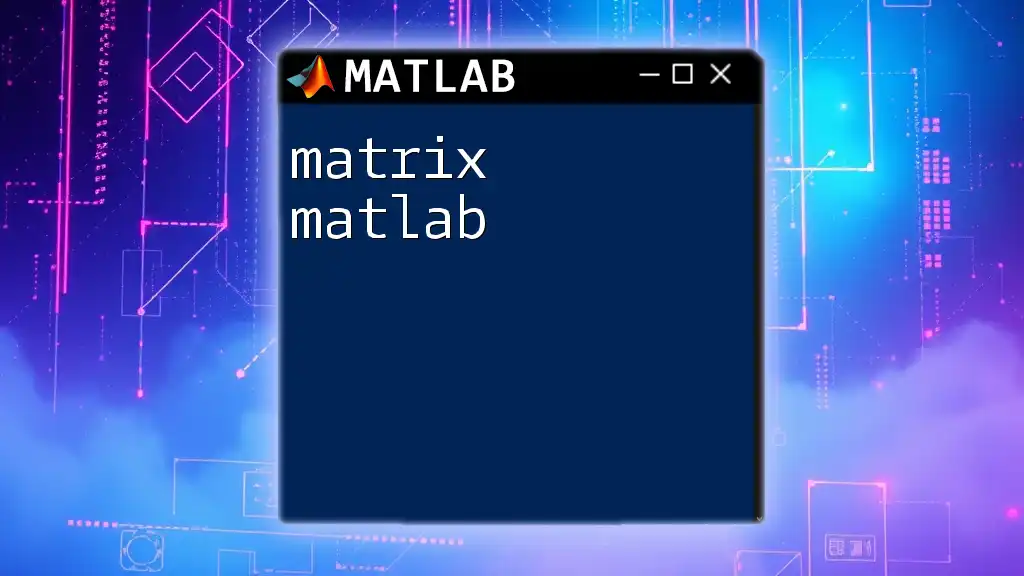
Common Mistakes and Troubleshooting
Misunderstanding Dimensions
One common mistake is misunderstanding matrix dimensions. When multiplying matrices, ensure that the number of columns in the first matrix matches the number of rows in the second. If you try to multiply compatible dimensions, MATLAB will return an error.
Notation Errors
Errors often occur in calling the `eye` function. A common mistake is to forget the parentheses or to misplace the size argument. Always double-check the syntax to avoid such pitfalls.
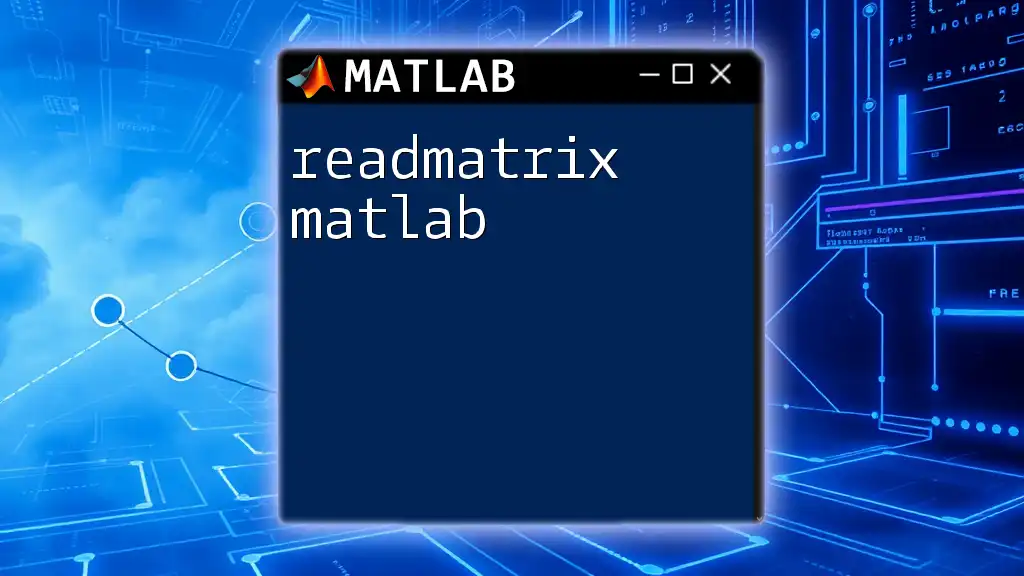
Conclusion
Understanding the concept of a unit matrix in MATLAB is essential for anyone looking to grasp linear algebra effectively. The `eye` function not only simplifies the creation of unit matrices but also supports a variety of operations. Mastering these commands will undoubtedly enhance your MATLAB skill set, making you more proficient in applying mathematical concepts in programming.