The dot product in MATLAB can be calculated using the `dot` function or the `*` operator for vector inputs, providing a concise way to measure the similarity between two vectors. Here's a simple code snippet demonstrating its usage:
% Define two vectors
A = [1, 2, 3];
B = [4, 5, 6];
% Calculate the dot product
result = dot(A, B); % Alternatively, you can use result = A * B';
disp(result);
What is a Dot Product?
The dot product is a fundamental operation between two vectors, resulting in a scalar value. It is calculated as a sum of the products of corresponding entries in the two sequences of numbers. This operation holds significant importance in various mathematical and scientific fields as it provides key insights into vector relationships.
Applications of Dot Product
The dot product is widely used in different domains such as:
- Physics: To determine work done, where work is defined as the dot product of force and displacement vectors.
- Computer Graphics: For lighting calculations and to determine angles between surfaces and light sources.
- Machine Learning: To assess similarity between feature vectors, which can impact decisions in classification tasks.
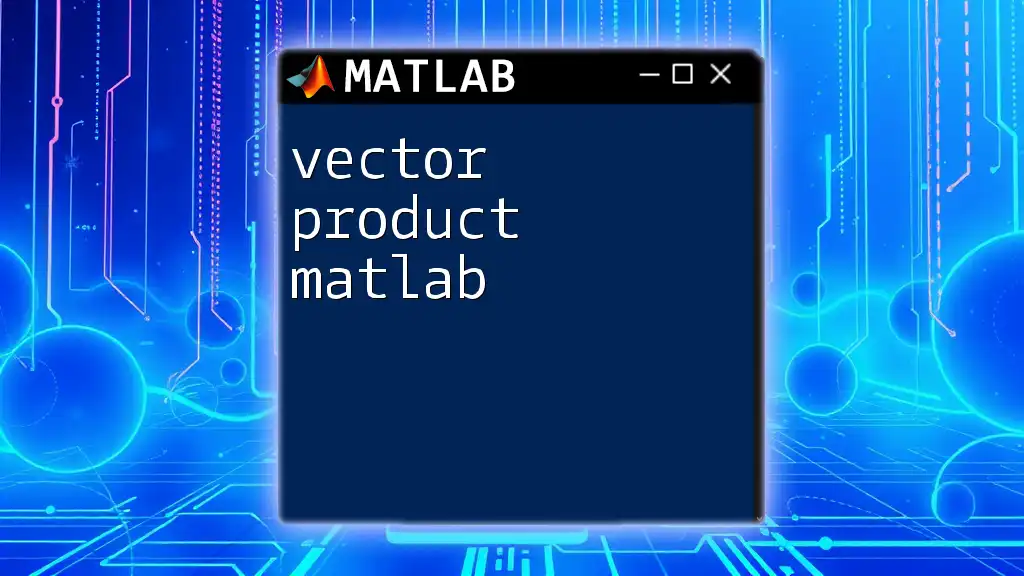
Understanding Vectors in MATLAB
Defining Vectors
In MATLAB, vectors can be easily created using square brackets. Vectors can either be row vectors or column vectors. For example, a row vector can be defined as:
A = [1, 2, 3]; % Row vector
Conversely, a column vector can be defined using a semicolon:
B = [1; 2; 3]; % Column vector
Vector Properties
Understanding vector properties is crucial when working with dot products. Important properties include:
- Length and Magnitude: The length of a vector can be calculated using the `norm` function.
- Direction and Normalization: A normalized vector has a length of 1 and provides a direction without magnitude.
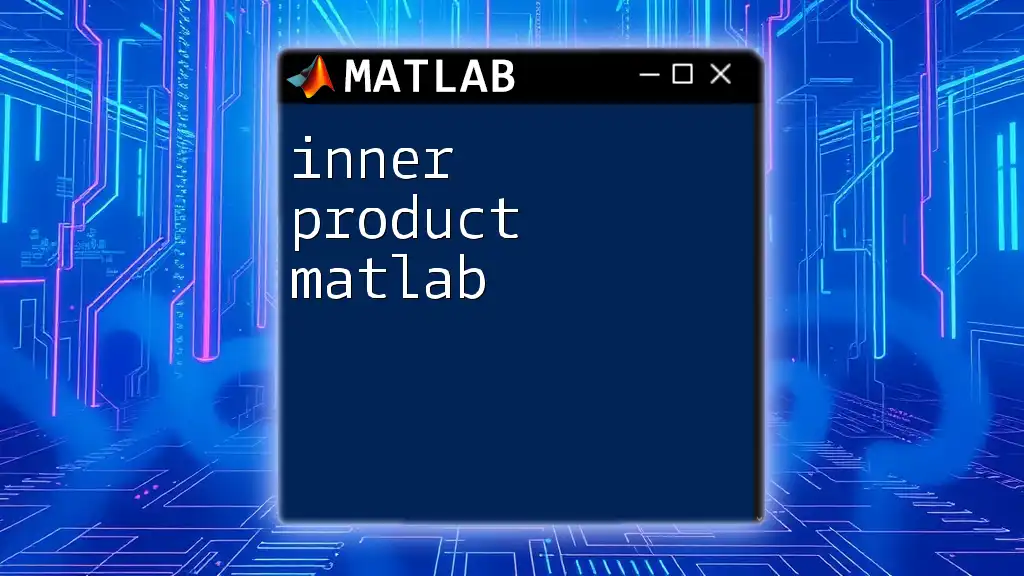
Calculating Dot Product in MATLAB
The `dot` Function
MATLAB provides a built-in function called `dot` to compute the dot product efficiently. The syntax follows the structure:
result = dot(A, B);
Where `A` and `B` are the input vectors. Here is a basic example of using the `dot` function:
A = [1, 2, 3];
B = [4, 5, 6];
result = dot(A, B);
disp(result); % Output: 32
This output is the scalar result of the dot product.
Manual Calculation of Dot Product
Alternatively, one can compute the dot product manually. The formula for the dot product between two vectors A and B is given as:
\[ \text{Dot Product} = A_1 \cdot B_1 + A_2 \cdot B_2 + A_3 \cdot B_3 + \ldots \]
Example: Manual Calculation
Let’s define vectors A and B and calculate the dot product manually:
A = [1, 2, 3];
B = [4, 5, 6];
manual_result = A(1)*B(1) + A(2)*B(2) + A(3)*B(3);
disp(manual_result); % Output should match the result from dot function
This exercise can help solidify the understanding of the dot product mechanics.
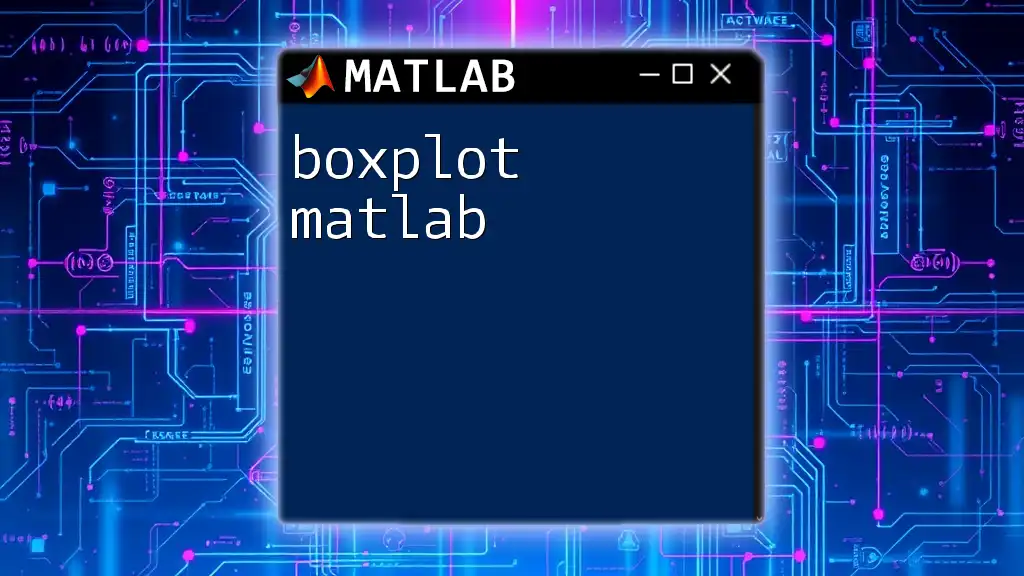
Exploring Properties of Dot Product
Commutative Property
The dot product exhibits the commutative property, meaning the order of operands does not affect the result:
\[ A \cdot B = B \cdot A \]
For instance, if we swap A and B in our earlier example, the result remains the same:
result_commutative = dot(B, A);
disp(result_commutative); % Output will still be 32
Distributive Property
The distributive property states that a dot product distributes over vector addition, which can be mathematically represented as:
\[ A \cdot (B + C) = A \cdot B + A \cdot C \]
This can be illustrated with an example where vectors C is also defined, and the resultant outputs can be confirmed through MATLAB calculations.
Dot Product and Angle Between Vectors
The dot product can be connected to the angle \( \theta \) between two vectors with the expression:
\[ A \cdot B = \|A\| \cdot \|B\| \cdot \cos(\theta) \]
This can be useful to find the angle between two vectors, which can be calculated using:
theta = acosd(dot(A, B) / (norm(A) * norm(B)));
disp(theta); % Degree output of the angle between vectors
This function computes \( \theta \) in degrees, providing a clear understanding of the geometric relationship between A and B.
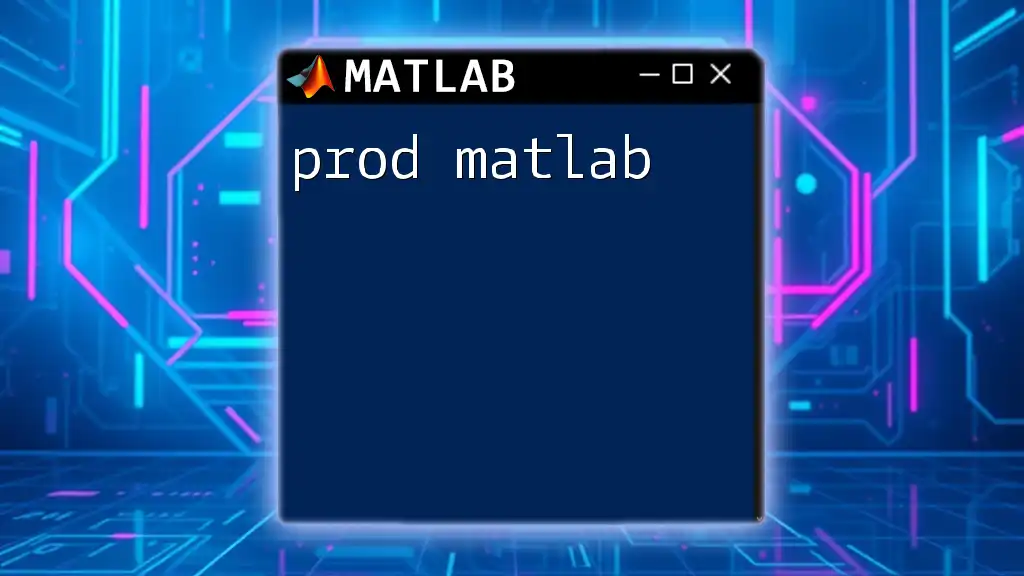
Visualizing Dot Product
2D Visualization
Visualizing vectors helps in understanding their properties and relationships. For 2D vectors, you can plot them using the `quiver` function.
figure;
quiver(0, 0, A(1), A(2), 'r');
hold on;
quiver(0, 0, B(1), B(2), 'b');
axis equal;
grid on;
xlim([-1 7]);
ylim([-1 7]);
title('Vectors A (Red) and B (Blue)');
3D Visualization
To visualize vectors in a 3D space, you can extend the `quiver3` function which allows plotting three-dimensional vectors:
figure;
quiver3(0, 0, 0, A(1), A(2), A(3), 'r');
hold on;
quiver3(0, 0, 0, B(1), B(2), B(3), 'b');
axis equal;
grid on;
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
title('3D Vectors A (Red) and B (Blue)');
This code will plot two vectors in a 3D space, facilitating a deeper understanding of their orientations.
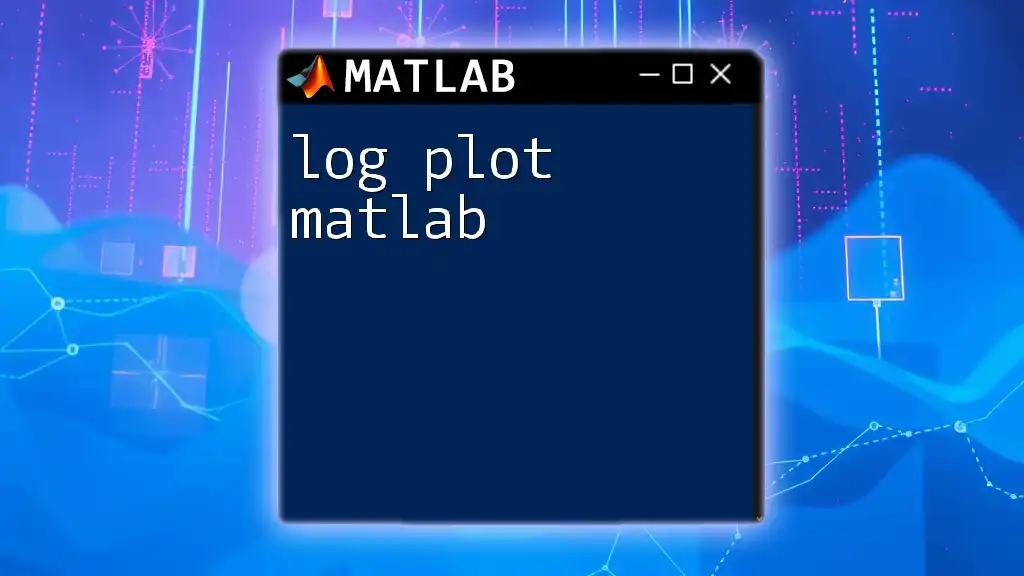
Common Mistakes and Troubleshooting
Dimension Mismatch Error
A common error encountered while calculating the dot product is dimension mismatch. This typically occurs when vectors of unequal lengths are input into the `dot` function:
C = [1, 2]; % Same dimension must match B
result_error = dot(A, C); % Will throw an error
Always ensure that the vectors being operated upon have the same number of elements.
Performance Issues with Large Vectors
When dealing with large vectors, performance can sometimes be an issue. It is recommended to utilize MATLAB’s optimized vectorized operations rather than looping constructs to improve performance.
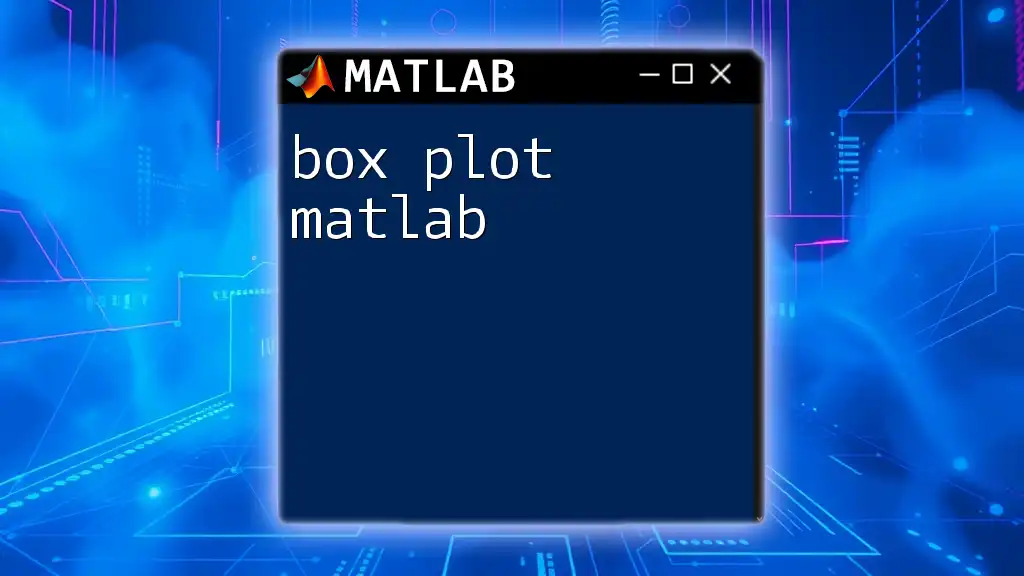
Conclusion
In summary, mastering the dot product in MATLAB is not only crucial for mathematical computations but also vital for tasks in various scientific domains. Understanding the properties, effective calculations, and visualizations of dot products will enhance your MATLAB programming efficiency.
On your journey to deepen your understanding of this crucial operation, remember that practice is key. Engage with exercises and challenge yourself to apply these concepts in various contexts.
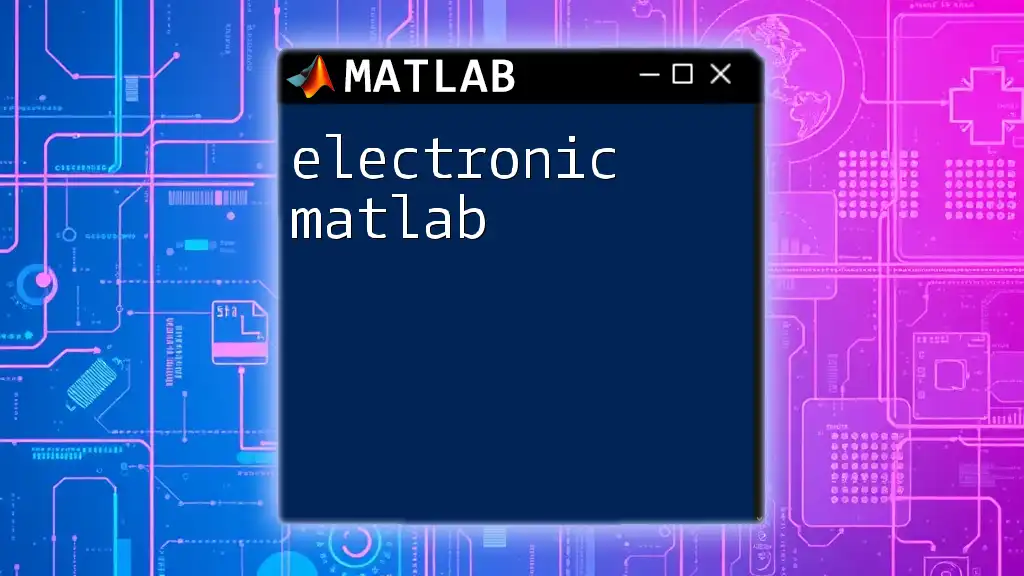
Additional Resources
Recommended Reading and Tutorials
For those interested in expanding their knowledge further, consider exploring recommended books and online courses that cover MATLAB programming comprehensively.
MATLAB Documentation Links
Direct links to MATLAB's official documentation provide additional insights and detailed explanations about the dot product function and related concepts.
Practice Exercises
Finally, seek out practice exercises that reinforce your understanding of the dot product. Applying these concepts in varying scenarios will help solidify your grasp on this fundamental mathematical operation.