An ODE (Ordinary Differential Equation) solver in MATLAB is a powerful tool that allows users to numerically solve differential equations using various built-in functions.
Here’s a simple example using the `ode45` solver in MATLAB:
% Define the ODE as a function
odefun = @(t, y) -2*y;
% Specify the time span and initial condition
tspan = [0 5];
y0 = 1;
% Use ode45 to solve the ODE
[t, y] = ode45(odefun, tspan, y0);
% Plot the results
plot(t, y);
xlabel('Time');
ylabel('y(t)');
title('Solution of ODE using ode45');
Understanding ODEs
What is an Ordinary Differential Equation?
An Ordinary Differential Equation (ODE) is a mathematical equation that relates a function of one independent variable to its derivatives. ODEs are fundamental in expressing various natural phenomena, from modeling motion in physics to predicting population growth in biology.
Types of ODEs include:
- First Order vs. Higher Order: First-order ODEs contain only the first derivative of the function, while higher-order ODEs involve second derivatives or higher.
- Linear vs. Non-linear: Linear ODEs can be expressed in a linear form, while non-linear ODEs include terms that are more complex, such as products of the solution function and its derivatives.
Key Terminology
To effectively work with ODEs, it's important to understand some key terms:
- Initial Value Problem (IVP): This is when the solution to an ODE is sought along with specified initial conditions.
- Boundary Value Problem (BVP): This involves solving ODEs under specific conditions at more than one value of the independent variable.
- Solution Space: The set of all possible solutions to a given differential equation.
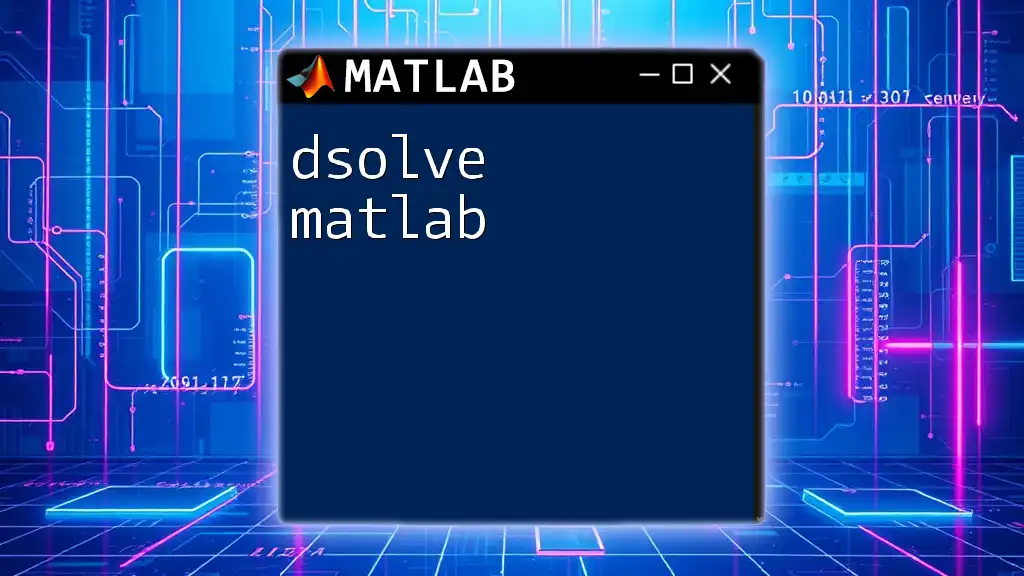
MATLAB ODE Solver Overview
Built-in ODE Solvers in MATLAB
MATLAB provides a variety of built-in ODE solvers that are particularly well-suited for different types of ODE problems. The most commonly used solvers include:
- `ode45`: A versatile solver for non-stiff problems based on the Dormand-Prince pair of formulas.
- `ode23`: Suitable for moderately stiff problems and especially effective when a simpler model suffices.
- `ode113`: Designed for higher accuracy in problems that require such precision.
- `ode15s`: Tailored for stiff ODEs, which are prevalent in chemical kinetics and other rapid phenomenon cases.
General Syntax for MATLAB ODE Solvers
The general syntax to use MATLAB ODE solvers typically follows this structure:
[t, y] = odeSolver(@(t, y) yourFunction(t, y), tspan, y0);
- `odeSolver` represents the solver you choose (like `ode45`).
- `@(t, y) yourFunction(t, y)` is an anonymous function that defines the ODE system.
- `tspan` is the interval over which you want to solve the ODE.
- `y0` is the initial condition for the solution.
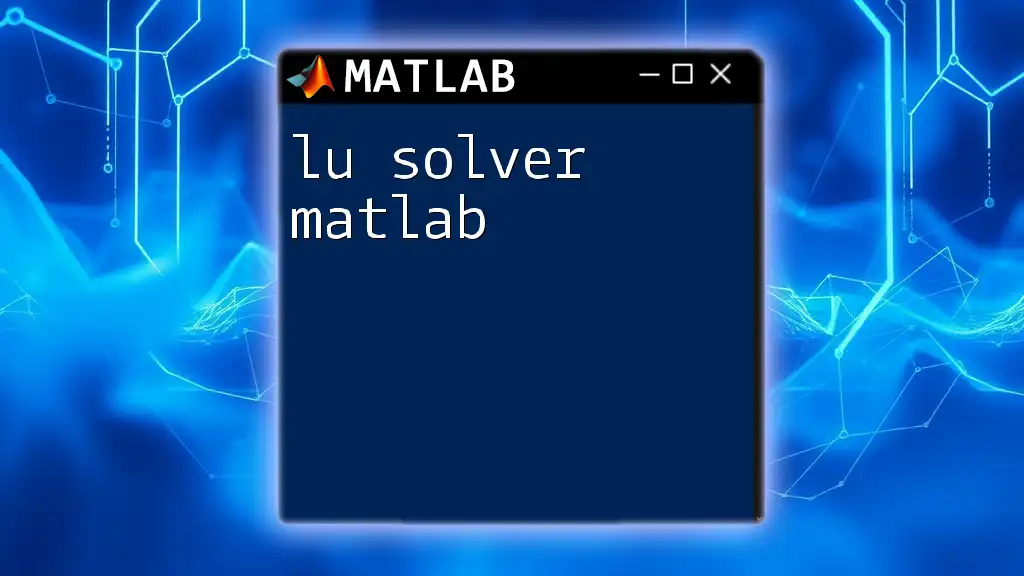
Setting Up an ODE Problem
Step-by-Step Guide to Defining ODEs
To solve an ODE using MATLAB, you must first define the equation you wish to solve. For instance, consider the first-order ODE:
\[ \frac{dy}{dt} = -2y + 1 \]
To solve this in MATLAB, follow these steps:
- Create a function file or an anonymous function. You can define this function as follows in MATLAB:
function dydt = myODE(t, y)
dydt = -2 * y + 1;
end
- Specify the initial conditions for the problem. In this case, if you want the initial value at \( t=0 \):
y0 = 0; % initial value at t=0
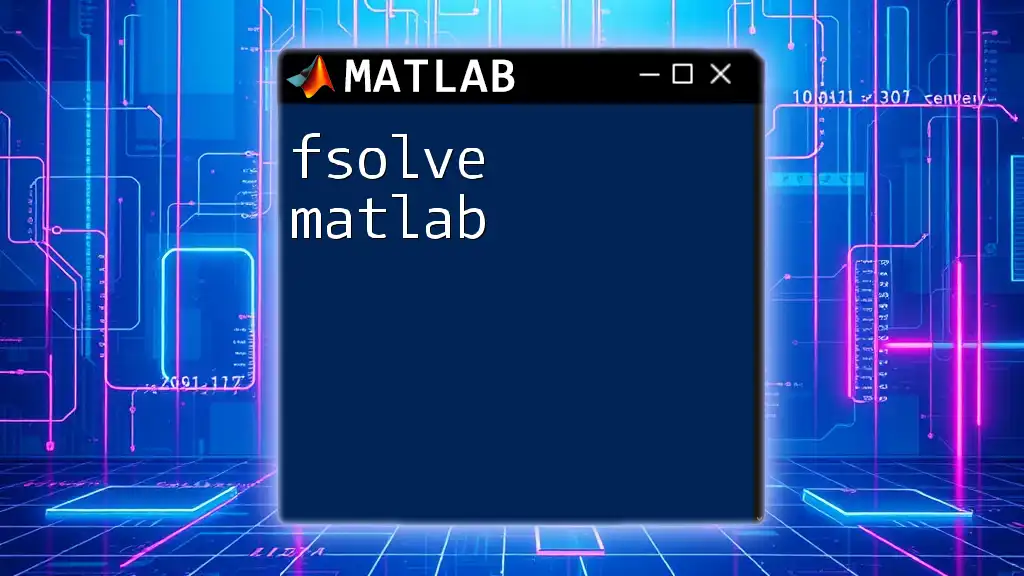
Solving ODEs Using MATLAB
Using `ode45` to Solve an ODE
`ode45` is the most commonly used solver, and it's suitable for a wide variety of problems. To apply it to our previous example, you can implement the following code:
tspan = [0 5]; % time interval
[t, y] = ode45(@myODE, tspan, y0);
plot(t, y);
title('Solution of ODE using ode45');
xlabel('Time t');
ylabel('Solution y');
In this code snippet:
- `tspan` sets the range of time over which we want to find the solution.
- `plot(t, y)` generates a graph of the solution over that range.
The resulting plot provides a visual insight into how the solution behaves over time, demonstrating the effect of the defined ODE.
Exploring Other Solvers
Using `ode23`
In scenarios where a slightly different method may be more efficient, `ode23` can be a good alternative. This solver is particularly effective for problems requiring moderate accuracy. An example might resemble:
[t, y] = ode23(@myODE, tspan, y0);
plot(t, y);
title('Solution of ODE using ode23');
xlabel('Time t');
ylabel('Solution y');
Using `ode15s`
If your ODE is stiff, it’s essential to switch to `ode15s`. This solver specifically handles stiff equations more effectively. Recognizing a stiff problem is crucial; you might gauge stiffness by observing whether solutions change rapidly relative to small changes in parameters.
Here's a potential application of the `ode15s` solver:
options = odeset('RelTol',1e-5,'AbsTol',1e-7);
[t, y] = ode15s(@myODE, tspan, y0, options);
plot(t, y);
title('Solution of Stiff ODE using ode15s');
xlabel('Time t');
ylabel('Solution y');
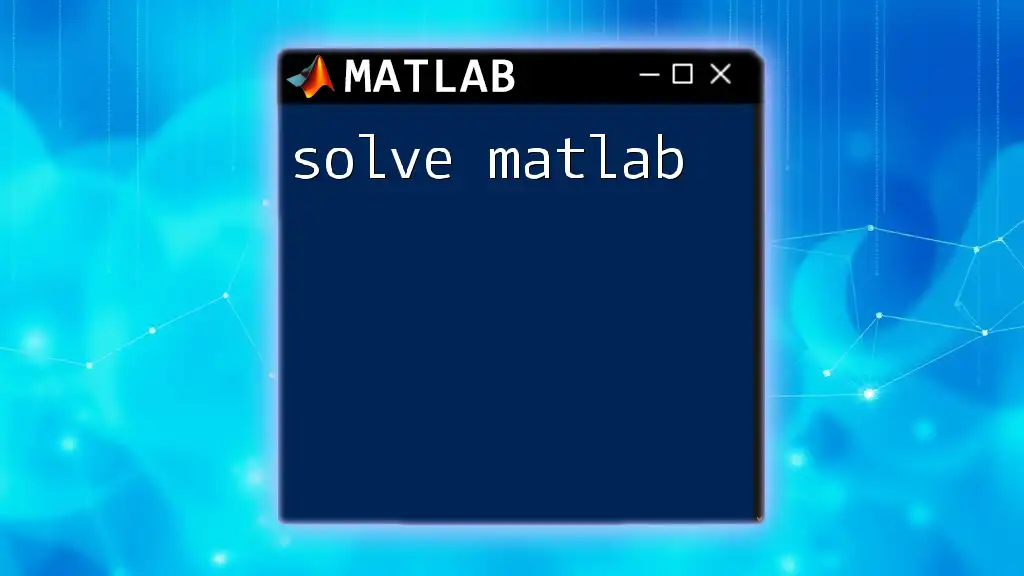
Advanced Topics in ODE Solving
Parameter Estimation in ODEs
MATLAB provides tools to fit ODE models to empirical data. This is critical in fields where parameters are not known a priori. Using functions like `lsqcurvefit`, you can optimize model parameters based on the available data. It allows for a comparison of modeled results against observed outcomes, improving accuracy.
Nonlinear ODEs with MATLAB
At times, you may encounter non-linear ODEs. To illustrate, consider a non-linear equation:
\[ \frac{dy}{dt} = y^2 - t \]
To solve this using MATLAB, define the function as follows:
function dydt = nonlinODE(t, y)
dydt = y^2 - t; % Non-linear term
end
You can then solve it in a similar way as before:
tspan = [0 5];
y0 = 0;
[t, y] = ode45(@nonlinODE, tspan, y0);
plot(t, y);
title('Solution of Non-linear ODE');
xlabel('Time t');
ylabel('Solution y');
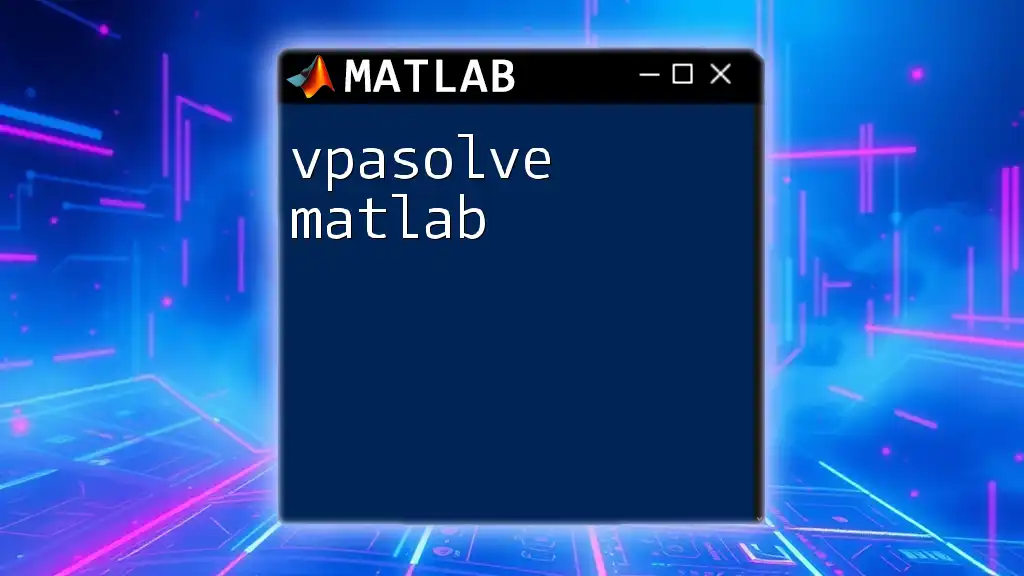
Visualization Techniques
Plotting Solutions
Visual representation of solutions is not just useful; it's indispensable. Plotting your results allows you to observe trends, stability, and fluctuations. You should implement customization options to enhance interpretability, such as adding legends or changing line colors.
For example:
plot(t, y, 'r-', 'LineWidth', 2);
hold on;
grid on;
legend('Solution');
xlabel('Time t');
ylabel('Solution y');
title('Modified Solution Plot for better visibility');
Analyzing Solutions
Beyond visualization, it’s crucial to analyze how solutions behave over time. Investigating aspects like stability and sensitivity to initial conditions can provide deeper insights. Consider varying parameters and noting changes in solutions, which aids in understanding system dynamics.
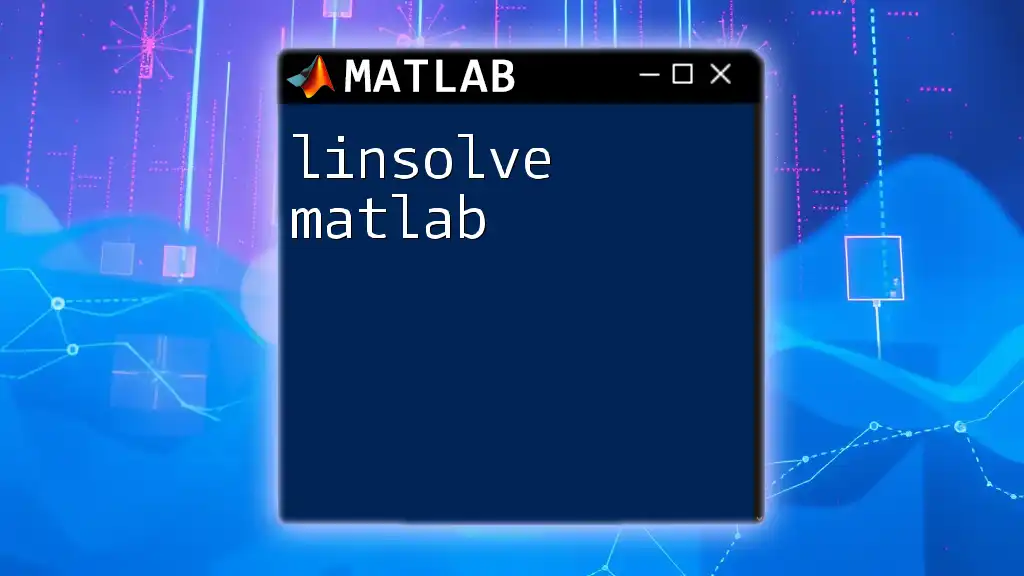
Conclusion
In this guide, we've explored the concept of ODEs and the efficient use of MATLAB's built-in solvers. From setting up simple first-order equations to tackling stiff and non-linear ODEs, we covered essential aspects to empower you to utilize the ode solver in MATLAB effectively.
Continuous practice with diverse scenarios will bolster your skills and confidence in solving ODEs. Embrace experimentation and don't hesitate to explore the vast capabilities MATLAB offers!
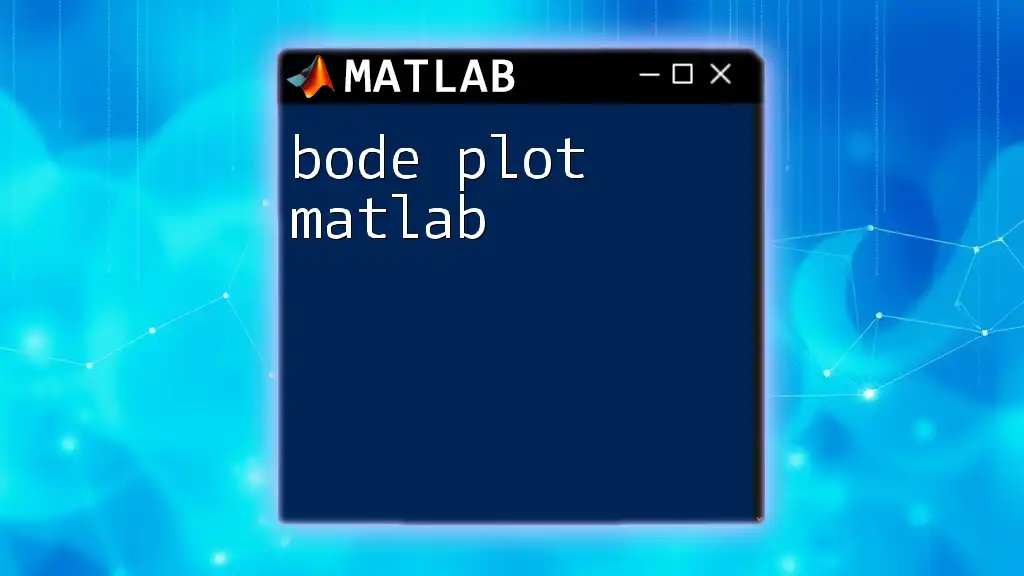
Additional Resources
For those looking to deepen their understanding of this topic:
- Consult the official MATLAB documentation available on the MathWorks website for detailed insights.
- Consider exploring specialized books focused on differential equations and MATLAB for more structured learning.
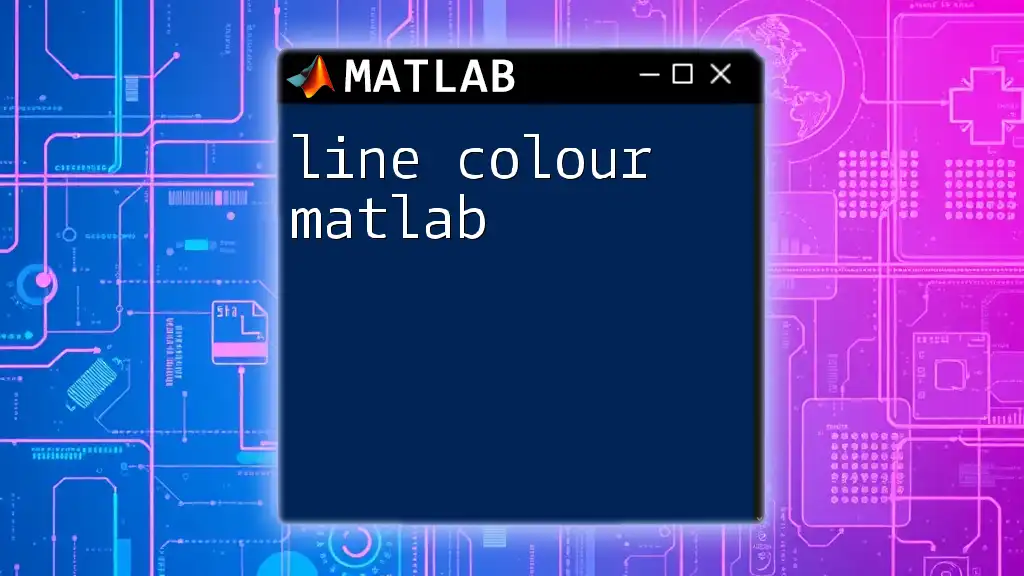
FAQs
Common Questions about ODE Solvers in MATLAB
-
What are the best practices for solving ODEs in MATLAB? Aim for clarity in your function definitions and utilize the appropriate solver for your problem type.
-
How do I choose the right ODE solver? Evaluate your equation's characteristics—stiffness, linearity, and required accuracy—to determine the most suitable solver.
-
Can I solve systems of ODEs with MATLAB? Absolutely! You can use similar patterns to define systems of ODEs by creating a vector function that returns derivatives for multiple dependent variables.
Call to Action
Now that you have a comprehensive guide on using the ODE solver in MATLAB, I encourage you to dive in and experiment with solving your own ODE problems. Challenge yourself with different scenarios, and feel free to reach out with questions or share your experiences. Happy coding!